Unit 8
Character Arrays and Strings
- Explain character array with example
Strings are actually one-dimensional array of characters terminated by a null character '\0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word "Hello". To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word "Hello."
Char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
If you follow the rule of array initialization then you can write the above statement as follows −
Char greeting[] = "Hello";
Following is the memory presentation of the above defined string in C/C++ −
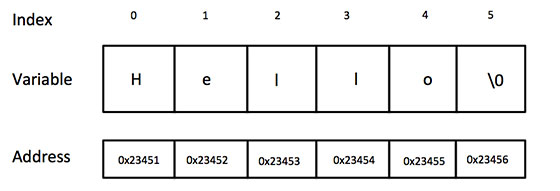
Actually, you do not place the null character at the end of a string constant. The C compiler automatically places the '\0' at the end of the string when it initializes the array. Let us try to print the above mentioned string −
#include <stdio.h>
Int main () {
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
printf("Greeting message: %s\n", greeting );
return 0;
}
When the above code is compiled and executed, it produces the following result −
Greeting message: Hello
2. Explain functions that manipulate null-terminated strings with examples
Sr.No. | Function & Purpose |
1 | Strcpy(s1, s2); Copies string s2 into string s1. |
2 | Strcat(s1, s2); Concatenates string s2 onto the end of string s1. |
3 | Strlen(s1); Returns the length of string s1. |
4 | Strcmp(s1, s2); Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | Strchr(s1, ch); Returns a pointer to the first occurrence of character ch in string s1. |
6 | Strstr(s1, s2); Returns a pointer to the first occurrence of string s2 in string s1. |
The following example uses some of the above-mentioned functions −
#include <stdio.h>
#include <string.h>
Int main () {
char str1[12] = "Hello";
char str2[12] = "World";
char str3[12];
int len ;
/* copy str1 into str3 */
strcpy(str3, str1);
printf("strcpy( str3, str1) : %s\n", str3 );
/* concatenates str1 and str2 */
strcat( str1, str2);
printf("strcat( str1, str2): %s\n", str1 );
/* total lenghth of str1 after concatenation */
len = strlen(str1);
printf("strlen(str1) : %d\n", len );
return 0;
}
When the above code is compiled and executed, it produces the following result −
Strcpy( str3, str1) : Hello
Strcat( str1, str2): HelloWorld
Strlen(str1) : 10
3. Explain Numeric Array in detail with example
Arrays a kind of data structure that can store a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Instead of declaring individual variables, such as number0, number1, ..., and number99, you declare one array variable such as numbers and use numbers[0], numbers[1], and ..., numbers[99] to represent individual variables. A specific element in an array is accessed by an index.
All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element.
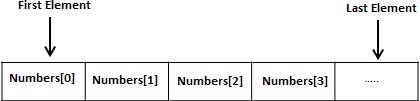
Declaring Arrays
To declare an array in C, a programmer specifies the type of the elements and the number of elements required by an array as follows −
Type arrayName [ arraySize ];
This is called a single-dimensional array. The arraySize must be an integer constant greater than zero and type can be any valid C data type. For example, to declare a 10-element array called balance of type double, use this statement −
Double balance[10];
Here balance is a variable array which is sufficient to hold up to 10 double numbers.
Initializing Arrays
You can initialize an array in C either one by one or using a single statement as follows −
Double balance[5] = {1000.0, 2.0, 3.4, 7.0, 50.0};
The number of values between braces { } cannot be larger than the number of elements that we declare for the array between square brackets [ ].
If you omit the size of the array, an array just big enough to hold the initialization is created. Therefore, if you write −
Double balance[] = {1000.0, 2.0, 3.4, 7.0, 50.0};
You will create exactly the same array as you did in the previous example. Following is an example to assign a single element of the array −
Balance[4] = 50.0;
The above statement assigns the 5th element in the array with a value of 50.0. All arrays have 0 as the index of their first element which is also called the base index and the last index of an array will be total size of the array minus 1. Shown below is the pictorial representation of the array we discussed above −

Accessing Array Elements
An element is accessed by indexing the array name. This is done by placing the index of the element within square brackets after the name of the array. For example −
Double salary = balance[9];
The above statement will take the 10th element from the array and assign the value to salary variable. The following example Shows how to use all the three above mentioned concepts viz. Declaration, assignment, and accessing arrays −
#include <stdio.h>
Int main () {
int n[ 10 ]; /* n is an array of 10 integers */
int i,j;
/* initialize elements of array n to 0 */
for ( i = 0; i < 10; i++ ) {
n[ i ] = i + 100; /* set element at location i to i + 100 */
}
/* output each array element's value */
for (j = 0; j < 10; j++ ) {
printf("Element[%d] = %d\n", j, n[j] );
}
return 0;
}
When the above code is compiled and executed, it produces the following result −
Element[0] = 100
Element[1] = 101
Element[2] = 102
Element[3] = 103
Element[4] = 104
Element[5] = 105
Element[6] = 106
Element[7] = 107
Element[8] = 108
Element[9] = 109
Arrays in Detail
Arrays are important to C and should need a lot more attention. The following important concepts related to array should be clear to a C programmer −
Sr.No. | Concept & Description |
1 | Multi-dimensional arrays C supports multidimensional arrays. The simplest form of the multidimensional array is the two-dimensional array. |
2 | Passing arrays to functions You can pass to the function a pointer to an array by specifying the array's name without an index. |
3 | Return array from a function C allows a function to return an array. |
4 | Pointer to an array You can generate a pointer to the first element of an array by simply specifying the array name, without any index. |
4. What is Null Character?
A null character is a character with all its bits set to zero. Therefore, it has a numeric value of zero and can be used to represent the end of a string of characters, such as a word or phrase. This helps programmers determine the length of strings. In practical applications, such as database and spreadsheet programs, null characters are used as fillers for spaces.
5. Explain string with example
String is nothing but a collection of characters in a linear sequence. 'C' always treats a string a single data even though it contains whitespaces. A single character is defined using single quote representation. A string is represented using double quote marks.
Example, "Welcome to the world of programming!"
'C' provides standard library <string.h> that contains many functions which can be used to perform complicated string operations easily.
Declare and initialize a String
A string is a simple array with char as a data type. 'C' language does not directly support string as a data type. Hence, to display a string in 'C', you need to make use of a character array.
The general syntax for declaring a variable as a string is as follows,
Char string_variable_name [array_size];
The classic string declaration can be done as follow:
char string_name[string_length] = "string";
The size of an array must be defined while declaring a string variable because it used to calculate how many characters are going to be stored inside the string variable. Some valid examples of string declaration are as follows,
Char first_name[15]; //declaration of a string variable
Char last_name[15];
The above example represents string variables with an array size of 15. This means that the given character array is capable of holding 15 characters at most. The indexing of array begins from 0 hence it will store characters from a 0-14 position. The C compiler automatically adds a NULL character '\0' to the character array created.
6. Write program to read the 2D array of characters or array of String in C
#include<stdio.h>
Int main()
{
// declaring and initializing 2D String
char language[5][10] =
{"Java", "Python", "C++", "HTML", "SQL"};
// Dispaying strings
printf("Languages are:\n");
for(int i=0;i<5;i++)
puts(language[i]);
return 0;
}
Output:-
Languages are:
Java
Python
C++
HTML
SQL
Program:- Write a program to read and display a 2D array of strings in C language.
#include<stdio.h>
Int main()
{
char name[10][20];
int i,n;
printf("Enter the number of names (<10): ");
scanf("%d",&n);
// reading string from user
printf("Enter %d names:\n",n);
for(i=0; i<n; i++)
scanf("%s[^\n]",name[i]);
// Dispaying strings
printf("\nEntered names are:\n");
for(i=0;i<n;i++)
puts(name[i]);
return 0;
}
Output:-
Enter the number of names (<10): 5
Enter 5 names:
Emma
Olivia
Ava
Isabella
Sophia
Entered names are:
Emma
Olivia
Ava
Isabella
Sophia
7. Write a program for Reading and displaying 2d array of strings in C
The two-dimensional array of strings can be read by using loops. To read we can use scanf(), gets(), fgets() or any other methods to read the string.
// reading strings using for loop
For(i=0;i<n;i++)
{
scanf("%s[^\n]",name[i]);
}
Or,
// reading strings using while loop
Int i=0;
While(i<n)
{
scanf("%s[^\n]",name[i]);
i++;
}
The two-dimensional array of strings can be displayed by using loops. To display we can use printf(), puts(), fputs() or any other methods to display the string.
// displaying strings using for loop
For(i=0;i<n;i++)
{
puts(name[i]);
}
Or,
// displaying strings using while loop
Int i=0;
While (i<n)
{
puts(name[i]);
i++;
}
8. Explain Initialization of array of strings
Two dimensional (2D) strings in C language can be directly initialized as shown below,
Char language[5][10] = {"Java", "Python", "C++", "HTML", "SQL"};
Char largestcity[6][15] =
{"Tokyo", "Delhi", "Shanghai", "Mumbai", "Beijing", "Dhaka"};
The two dimensional (2D) array of Strings in C also can be initialized as,
Char language[5][10] ={
{'J','a','v','a','\0'},
{'P','y','t','h','o','n','\0'},
{'C','+','+','\0'},
{'H','T','M','L','\0'},
{'S','Q','L','\0'}
};
Since it is a two-dimension of characters, so each String (1-D array of characters) must end with null character i.e. ‘\0’
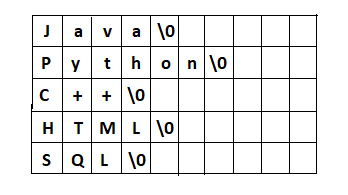
The second way of declaring the array of strings is a lengthy process, and other programmers can’t read them easily compared to the previous declaration, that why most of the time we prefer the first declaration.
The each String in this array can be accessed by using its index number. The index of array always starts with 0.
Language[0] => "Java";
Language[1] => "Python";
Language[2] => "C++";
Language[3] => "HTML";
Language[4] => "SQL";
Note1:- the number of characters (column-size) must be declared at the time of the initialization of the two-dimensional array of strings.
// it is valid
Char language[ ][10] = {"Java", "Python", "C++", "HTML", "SQL"};
But that the following declarations are invalid.
// invalid
Char language[ ][ ] = {"Java", "Python", "C++", "HTML", "SQL"};
// invalid
Char language[5][ ] = {"Java", "Python", "C++", "HTML", "SQL"};
Note2:- Once we initialize the array of String then we can’t directly assign a new String.
Char language[5][10] = {"Java", "Python", "C++", "HTML", "SQL"};
// now, we can't directly assign a new String
Language[0] = "Kotlin"; // invalid
// we must copy the String
Strcpy(language[0], "Kotlin"); // valid
// Or,
Scanf(language[0], "Kotlin"); // valid
9. Explain 2 dimensional array in detail
Sometimes we often use lists of character strings, such as the list of names of students in a class, a list of names of employees in an organization, a list of places, etc. A list of names can be treated as a table of string. To store the entire list we use a 2d array of strings in C language.
The array of characters is called a string. “Hi”, “Hello”, and e.t.c are the examples of String. Similarly, the array of Strings is nothing but a two-dimensional (2D) array of characters. To declare an array of Strings in C, we must use the char data type.
Example of two dimensional characters or the array of Strings is,
Char language[5][10] = {"Java", "Python", "C++", "HTML", "SQL"};
Declaration of the array of strings
Syntax:-
Char string-array-name[row-size][column-size];
Here the first index (row-size) specifies the maximum number of strings in the array, and the second index (column-size) specifies the maximum length of every individual string.
For example, char language[5][10]; In the “language” array we can store a maximum of 5 Strings and each String can have a maximum of 10 characters.
In C language, each character take 1 byte of memory. For the “language” array it will allocate 50 bytes (1*5*10) of memory. Where each String will have 10 bytes (1*10) of memory space.
10. What are some of the Library Functions in header string?
Following are the functions defined in the header string.h −
Sr.No. | Function & Description |
1 | Void *memchr(const void *str, int c, size_t n) Searches for the first occurrence of the character c (an unsigned char) in the first n bytes of the string pointed to, by the argument str. |
2 | Int memcmp(const void *str1, const void *str2, size_t n) Compares the first n bytes of str1 and str2. |
3 | Void *memcpy(void *dest, const void *src, size_t n) Copies n characters from src to dest. |
4 | Void *memmove(void *dest, const void *src, size_t n) Another function to copy n characters from str2 to str1. |
5 | Void *memset(void *str, int c, size_t n) Copies the character c (an unsigned char) to the first n characters of the string pointed to, by the argument str. |
6 | Char *strcat(char *dest, const char *src) Appends the string pointed to, by src to the end of the string pointed to by dest. |
7 | Char *strncat(char *dest, const char *src, size_t n) Appends the string pointed to, by src to the end of the string pointed to, by dest up to n characters long. |
8 | Char *strchr(const char *str, int c) Searches for the first occurrence of the character c (an unsigned char) in the string pointed to, by the argument str. |
9 | Int strcmp(const char *str1, const char *str2) Compares the string pointed to, by str1 to the string pointed to by str2. |
10 | Int strncmp(const char *str1, const char *str2, size_t n) Compares at most the first n bytes of str1 and str2. |
11 | Int strcoll(const char *str1, const char *str2) Compares string str1 to str2. The result is dependent on the LC_COLLATE setting of the location. |
12 | Char *strcpy(char *dest, const char *src) Copies the string pointed to, by src to dest. |
13 | Char *strncpy(char *dest, const char *src, size_t n) Copies up to n characters from the string pointed to, by src to dest. |
14 | Size_t strcspn(const char *str1, const char *str2) Calculates the length of the initial segment of str1 which consists entirely of characters not in str2. |
15 | Char *strerror(int errnum) Searches an internal array for the error number errnum and returns a pointer to an error message string. |
16 | Size_t strlen(const char *str) Computes the length of the string str up to but not including the terminating null character. |
17 | Char *strpbrk(const char *str1, const char *str2) Finds the first character in the string str1 that matches any character specified in str2. |
18 | Char *strrchr(const char *str, int c) Searches for the last occurrence of the character c (an unsigned char) in the string pointed to by the argument str. |
19 | Size_t strspn(const char *str1, const char *str2) Calculates the length of the initial segment of str1 which consists entirely of characters in str2. |
20 | Char *strstr(const char *haystack, const char *needle) Finds the first occurrence of the entire string needle (not including the terminating null character) which appears in the string haystack. |
21 | Char *strtok(char *str, const char *delim) Breaks string str into a series of tokens separated by delim. |
22 | Size_t strxfrm(char *dest, const char *src, size_t n) Transforms the first n characters of the string src into current locale and places them in the string dest. |