UNIT 2
- What is data input and output?
When we say Input, it means to feed some data into a program. An input can be given in the form of a file or from the command line. C programming provides a set of built-in functions to read the given input and feed it to the program as per requirement.
When we say Output, it means to display some data on screen, printer, or in any file. C programming provides a set of built-in functions to output the data on the computer screen as well as to save it in text or binary files.
The Standard Files
C programming treats all the devices as files. So devices such as the display are addressed in the same way as files and the following three files are automatically opened when a program executes to provide access to the keyboard and screen.
Standard File | File Pointer | Device |
Standard input | Stdin | Keyboard |
Standard output | Stdout | Screen |
Standard error | Stderr | Your screen |
The file pointers are the means to access the file for reading and writing purpose. This section explains how to read values from the screen and how to print the result on the screen.
2. Explain getchar() and putchar() Functions and gets() and puts() Functions with example
The getchar() and putchar() Functions
The int getchar(void) function reads the next available character from the screen and returns it as an integer. This function reads only single character at a time. You can use this method in the loop in case you want to read more than one character from the screen.
The int putchar(int c) function puts the passed character on the screen and returns the same character. This function puts only single character at a time. You can use this method in the loop in case you want to display more than one character on the screen. Check the following example −
#include <stdio.h>
Int main( ) {
int c;
printf( "Enter a value :");
c = getchar( );
printf( "\nYou entered: ");
putchar( c );
return 0;
}
When the above code is compiled and executed, it waits for you to input some text. When you enter a text and press enter, then the program proceeds and reads only a single character and displays it as follows −
$./a.out
Enter a value : this is test
You entered: t
The gets() and puts() Functions
The char *gets(char *s) function reads a line from stdin into the buffer pointed to by s until either a terminating newline or EOF (End of File).
The int puts(const char *s) function writes the string 's' and 'a' trailing newline to stdout.
NOTE: Though it has been deprecated to use gets() function, Instead of using gets, you want to use fgets().
#include <stdio.h>
Int main( ) {
char str[100];
printf( "Enter a value :");
gets( str );
printf( "\nYou entered: ");
puts( str );
return 0;
}
When the above code is compiled and executed, it waits for you to input some text. When you enter a text and press enter, then the program proceeds and reads the complete line till end, and displays it as follows −
$./a.out
Enter a value : this is test
You entered: this is test
3. Explain scanf() and printf() Functions
The scanf() and printf() Functions
The int scanf(const char *format, ...) function reads the input from the standard input stream stdin and scans that input according to the format provided.
The int printf(const char *format, ...) function writes the output to the standard output stream stdout and produces the output according to the format provided.
The format can be a simple constant string, but you can specify %s, %d, %c, %f, etc., to print or read strings, integer, character or float respectively. There are many other formatting options available which can be used based on requirements. Let us now proceed with a simple example to understand the concepts better −
#include <stdio.h>
Int main( ) {
char str[100];
int i;
printf( "Enter a value :");
scanf("%s %d", str, &i);
printf( "\nYou entered: %s %d ", str, i);
return 0;
}
When the above code is compiled and executed, it waits for you to input some text. When you enter a text and press enter, then program proceeds and reads the input and displays it as follows −
$./a.out
Enter a value : seven 7
You entered: seven 7
Here, it should be noted that scanf() expects input in the same format as you provided %s and %d, which means you have to provide valid inputs like "string integer". If you provide "string string" or "integer integer", then it will be assumed as wrong input. Secondly, while reading a string, scanf() stops reading as soon as it encounters a space, so "this is test" are three strings for scanf().
4. What is basic structure of c program?
Hello World Example
A C program basically consists of the following parts −
- Pre-processor Commands
- Functions
- Variables
- Statements & Expressions
- Comments
Let us look at a simple code that would print the words "Hello World" −
#include <stdio.h>
Int main() {
/* my first program in C */
printf("Hello, World! \n");
return 0;
}
Let us take a look at the various parts of the above program −
- The first line of the program #include <stdio.h> is a preprocessor command, which tells a C compiler to include stdio.h file before going to actual compilation.
- The next line int main() is the main function where the program execution begins.
- The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. So such lines are called comments in the program.
- The next line printf(...) is another function available in C which causes the message "Hello, World!" to be displayed on the screen.
- The next line return 0; terminates the main() function and returns the value 0.
Compile and Execute C Program
Let us see how to save the source code in a file, and how to compile and run it. Following are the simple steps −
- Open a text editor and add the above-mentioned code.
- Save the file as hello.c
- Open a command prompt and go to the directory where you have saved the file.
- Type gcc hello.c and press enter to compile your code.
- If there are no errors in your code, the command prompt will take you to the next line and would generate a.out executable file.
- Now, type a.out to execute your program.
- You will see the output "Hello World" printed on the screen.
$ gcc hello.c
$ ./a.out
Hello, World!
Make sure the gcc compiler is in your path and that you are running it in the directory containing the source file hello.c.
5. What is string input: Read a string explains with example?
String Input: Read a String
When writing interactive programs which ask the user for input, C provides the scanf(), gets(), and fgets() functions to find a line of text entered from the user.
When we use scanf() to read, we use the "%s" format specifier without using the "&" to access the variable address because an array name acts as a pointer. For example:
#include <stdio.h>
Int main() {
Char name[10];
Int age;
Printf("Enter your first name and age: \n");
Scanf("%s %d", name, &age);
Printf("You entered: %s %d",name,age);
}
Output:
Enter your first name and age:
John_Smith 48
The problem with the scanf function is that it never reads an entire string. It will halt the reading process as soon as whitespace, form feed, vertical tab, newline or a carriage return occurs. Suppose we give input as "Guru99 Tutorials" then the scanf function will never read an entire string as a whitespace character occurs between the two names. The scanf function will only read Guru99.
In order to read a string contains spaces, we use the gets() function. Gets ignores the whitespaces. It stops reading when a newline is reached (the Enter key is pressed).For example:
#include <stdio.h>
Int main() {
Char full_name[25];
Printf("Enter your full name: ");
Gets(full_name);
Printf("My full name is %s ",full_name);
Return 0;
}
Output:
Enter your full name: Dennis Ritchie
My full name is Dennis Ritchie
Another safer alternative to gets() is fgets() function which reads a specified number of characters. For example:
#include <stdio.h>
Int main() {
Char name[10];
Printf("Enter your name plz: ");
Fgets(name, 10, stdin);
Printf("My name is %s ",name);
Return 0;}
Output:
Enter your name plz: Carlos
My name is Carlos
The fgets() arguments are :
- The string name,
- The number of characters to read,
- Stdin means to read from the standard input which is the keyboard.
6. Explain String Output: Print/Display a String with example
String Output: Print/Display a String
The standard printf function is used for printing or displaying a string on an output device. The format specifier used is %s
Example,
Printf("%s", name);
String output is done with the fputs() and printf() functions.
Fputs() function
The fputs() needs the name of the string and a pointer to where you want to display the text. We use stdout which refers to the standard output in order to print to the screen.For example:
#include <stdio.h>
Int main()
{char town[40];
printf("Enter your town: ");
gets(town);
fputs(town, stdout);
return 0;}
Output:
Enter your town: New York
New York
Puts function
The puts function prints the string on an output device and moves the cursor back to the first position. A puts function can be used in the following way,
#include <stdio.h>
Int main() {
Char name[15];
Gets(name); //reads a string
Puts(name); //displays a string
Return 0;}
The syntax of this function is comparatively simple than other functions.
7. Explain formatted input and output in detail
C language provides us console input/output functions. As the name says, the console input/output functions allow us to -
- Read the input from the keyboard by the user accessing the console.
- Display the output to the user at the console.
Note : These input and output values could be of any primitive data type.
There are two kinds of console input/output functions -
- Formatted input/output functions.
- Unformatted input/output functions.
Formatted input/output functions
Formatted console input/output functions are used to take one or more inputs from the user at console and it also allows us to display one or multiple values in the output to the user at the console.
Some of the most important formatted console input/output functions are -
Functions | Description |
Scanf() |
|
Printf() |
|
Sscanf() |
|
Sprintf() |
|
Format specifiers in console formatted I/O functions
Some of the most commonly used format specifiers used in console formatted input/output functions are displayed in the table below -
Format Specifiers | Description |
%hi |
|
%hu |
|
%d |
|
%u |
|
%ld |
|
%lu |
|
%c |
|
%c |
|
%f |
|
%lf |
|
%Lf |
|
%s |
|
8. What is a control structure?
Control Structures are just a way to specify flow of control in programs. Any algorithm or program can be more clear and understood if they use self-contained modules called as logic or control structures. It basically analyzes and chooses in which direction a program flows based on certain parameters or conditions. There are three basic types of logic, or flow of control, known as:
- Sequence logic, or sequential flow
- Selection logic, or conditional flow
- Iteration logic, or repetitive flow
Let us see them in detail:
- Sequential Logic (Sequential Flow)
Sequential logic as the name suggests follows a serial or sequential flow in which the flow depends on the series of instructions given to the computer. Unless new instructions are given, the modules are executed in the obvious sequence. The sequences may be given, by means of numbered steps explicitly. Also, implicitly follows the order in which modules are written. Most of the processing, even some complex problems, will generally follow this elementary flow pattern.
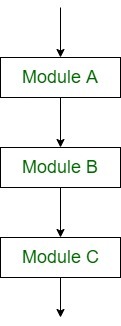
Sequential Control flow
2. Selection Logic (Conditional Flow)
Selection Logic simply involves a number of conditions or parameters which decides one out of several written modules. The structures which use these type of logic are known as Conditional Structures. These structures can be of three types:
- Single AlternativeThis structure has the form:
- If (condition) then:
- [Module A]
[End of If structure]
- Double AlternativeThis structure has the form:
- If (Condition), then:
- [Module A]
- Else:
- [Module B]
- [End if structure]
- Multiple AlternativesThis structure has the form:
- If (condition A), then:
- [Module A]
- Else if (condition B), then:
- [Module B]
- ..
- ..
- Else if (condition N), then:
- [Module N]
- [End If structure]
In this way, the flow of the program depends on the set of conditions that are written. This can be more understood by the following flow charts:
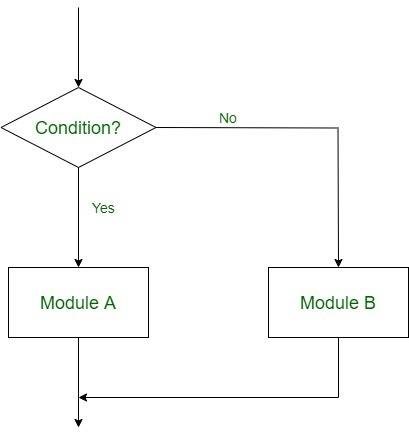
Double Alternative Control Flow
3. Iteration Logic (Repetitive Flow)
The Iteration logic employs a loop which involves a repeat statement followed by a module known as the body of a loop.
The two types of these structures are:
- Repeat-For Structure
This structure has the form: - Repeat for i = A to N by I:
- [Module]
- [End of loop]
Here, A is the initial value, N is the end value and I is the increment. The loop ends when A>B. K increases or decreases according to the positive and negative value of I respectively.
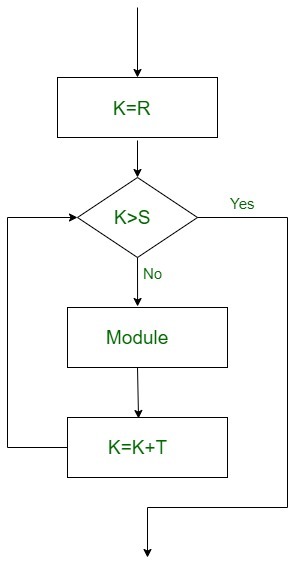
Repeat-For Flow
- Repeat-While Structure
It also uses a condition to control the loop. This structure has the form: - Repeat while condition:
- [Module]
- [End of Loop]
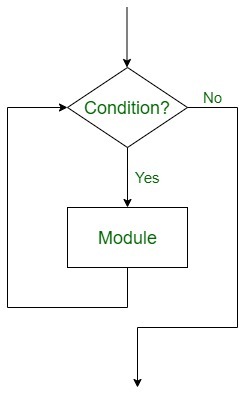
Repeat While Flow
Implementation:
In this, there requires a statement that initializes the condition controlling the loop, and there must also be a statement inside the module that will change this condition leading to the end of the loop.
9. Explain IF statement with example
C if else Statement
The if-else statement in C is used to perform the operations based on some specific condition. The operations specified in if block are executed if and only if the given condition is true.
There are the following variants of if statement in C language.
- If statement
- If-else statement
- If else-if ladder
- Nested if
If Statement
The if statement is used to check some given condition and perform some operations depending upon the correctness of that condition. It is mostly used in the scenario where we need to perform the different operations for the different conditions. The syntax of the if statement is given below.
- If(expression){
- //code to be executed
- }
Flowchart of if statement in C
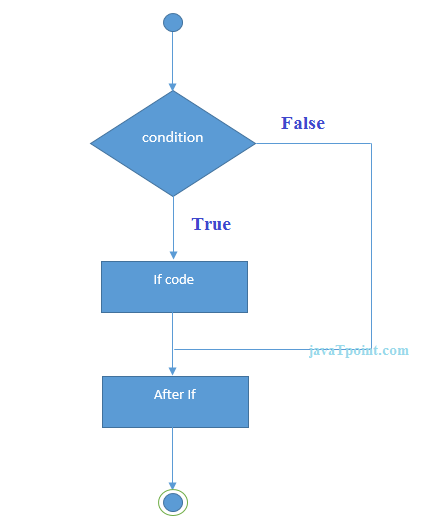
Let's see a simple example of C language if statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("Enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
Program to find the largest number of the three.
- #include <stdio.h>
- Int main()
- {
- Int a, b, c;
- Printf("Enter three numbers?");
- Scanf("%d %d %d",&a,&b,&c);
- If(a>b && a>c)
- {
- Printf("%d is largest",a);
- }
- If(b>a && b > c)
- {
- Printf("%d is largest",b);
- }
- If(c>a && c>b)
- {
- Printf("%d is largest",c);
- }
- If(a == b && a == c)
- {
- Printf("All are equal");
- }
- }
Output
Enter three numbers?
12 23 34
34 s largest
10. Explain switch statement in detail with examples
C Switch Statement
The switch statement in C is an alternate to if-else-if ladder statement which allows us to execute multiple operations for the different possibles values of a single variable called switch variable. Here, We can define various statements in the multiple cases for the different values of a single variable.
The syntax of switch statement in c language is given below:
- Switch(expression){
- Case value1:
- //code to be executed;
- Break; //optional
- Case value2:
- //code to be executed;
- Break; //optional
- ......
- Default:
- Code to be executed if all cases are not matched;
- }
Rules for switch statement in C language
1) The switch expression must be of an integer or character type.
2) The case value must be an integer or character constant.
3) The case value can be used only inside the switch statement.
4) The break statement in switch case is not must. It is optional. If there is no break statement found in the case, all the cases will be executed present after the matched case. It is known as fall through the state of C switch statement.
Let's try to understand it by the examples. We are assuming that there are following variables.
- Int x,y,z;
- Char a,b;
- Float f;
Valid Switch | Invalid Switch | Valid Case | Invalid Case |
Switch(x) | Switch(f) | Case 3; | Case 2.5; |
Switch(x>y) | Switch(x+2.5) | Case 'a'; | Case x; |
Switch(a+b-2) |
| Case 1+2; | Case x+2; |
Switch(func(x,y)) |
| Case 'x'>'y'; | Case 1,2,3; |
Flowchart of switch statement in C
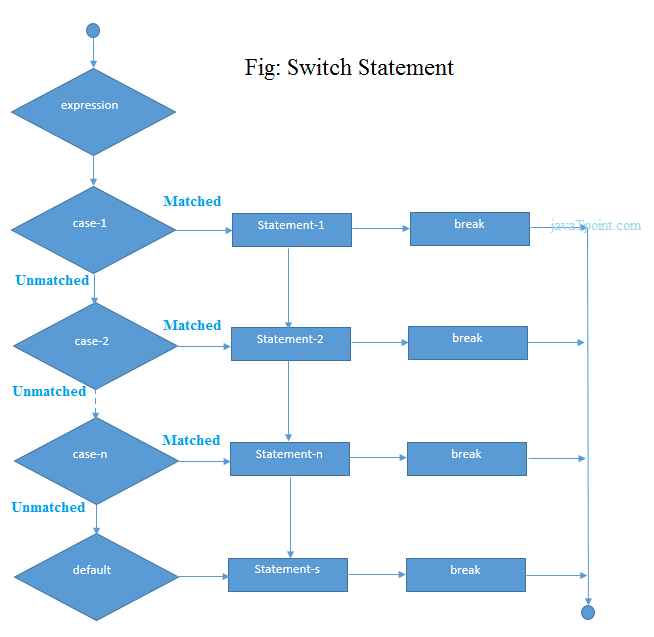
Functioning of switch case statement
First, the integer expression specified in the switch statement is evaluated. This value is then matched one by one with the constant values given in the different cases. If a match is found, then all the statements specified in that case are executed along with the all the cases present after that case including the default statement. No two cases can have similar values. If the matched case contains a break statement, then all the cases present after that will be skipped, and the control comes out of the switch. Otherwise, all the cases following the matched case will be executed.
Let's see a simple example of c language switch statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equals to 10");
- Break;
- Case 50:
- Printf("number is equal to 50");
- Break;
- Case 100:
- Printf("number is equal to 100");
- Break;
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Switch case example 2
- #include <stdio.h>
- Int main()
- {
- Int x = 10, y = 5;
- Switch(x>y && x+y>0)
- {
- Case 1:
- Printf("hi");
- Break;
- Case 0:
- Printf("bye");
- Break;
- Default:
- Printf(" Hello bye ");
- }
- }
Output
Hi
C Switch statement is fall-through
In C language, the switch statement is fall through; it means if you don't use a break statement in the switch case, all the cases after the matching case will be executed.
Let's try to understand the fall through state of switch statement by the example given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equal to 10\n");
- Case 50:
- Printf("number is equal to 50\n");
- Case 100:
- Printf("number is equal to 100\n");
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:10
Number is equal to 10
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Output
Enter a number:50
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100