UNIT- 4
An Overview of C
- What are Basic and Derived Data Types explain in brief Constants and Variables and Data types
Constant and Variables
Constant are same as variables but once initialized, cannot change by program.
Constants are fixed values and also called as literals.
Example –
- Integer constants – 2,7,0,454,1204.
- Real constants – 6.7, 4556.78,3432.988
- Character constants – ‘a’,’G’.
- String Constants – “Hello World”.
A variable is nothing but a name given to a storage area that our programs can manipulate. Each variable in C has a specific type, which determines the size and layout of the variable's memory; the range of values that can be stored within that memory; and the set of operations that can be applied to the variable.
Data Types
Variable are declared along with data types according to which data can be stored.
Compiler in C++ allocates memory to variable based on the data type is used to declare variable.
It consists of three types of data types – Primitive data type, Derived data types and user defined data types.
Primitive data type.
Integer
Int keyword is used for integer data types. Memory space required by integer is 4 bytes and range is from -2147483648 to 2147483647.
Character
Char keyword is used for character data types. It is used to store character data. . Memory space required by integer is 1 byte and range is from -128 to 127 or 0 to 255.
Boolean
Bool keyword is used for character data types. It is used to store Boolean values. It stores only true or false.
Floating point
Float keyword is used for character data types. It is used to store decimal values. Memory space required by integer is 4 byte.
Double Floating Point
Double keyword is used for character data types. It is used to store double precision decimal values. Memory space required by integer is 8 byte.
Derived Data types
Derived data types are the user defined data types , defined by the user.
It consists of struct, enumerations and classes.
Structure in which different data type variables can be defined.
Class is the basis of object oriented programming.
Enumeration is the set of integer constants that are assigned to variables.
Void Data type
Void data type is used for value les entity. It is used for function which do not return any value.
Void msg()
{
<< cout “Hello World”;
}
Int main()
{
Msg();
}
2. Difference between Structure and Union
Here is the important difference between structure and union:
Structure | Union |
You can use a struct keyword to define a structure. | You can use a union keyword to define a union. |
Every member within structure is assigned a unique memory location. | In union, a memory location is shared by all the data members. |
Changing the value of one data member will not affect other data members in structure. | Changing the value of one data member will change the value of other data members in union. |
It enables you to initialize several members at once. | It enables you to initialize only the first member of union. |
The total size of the structure is the sum of the size of every data member. | The total size of the union is the size of the largest data member. |
It is mainly used for storing various data types. | It is mainly used for storing one of the many data types that are available. |
It occupies space for each and every member written in inner parameters. | It occupies space for a member having the highest size written in inner parameters. |
You can retrieve any member at a time. | You can access one member at a time in the union. |
It supports flexible array. | It does not support a flexible array. |
3. Give example of Nested Structure
Let's see a simple example of the nested structure in C language.
- #include <stdio.h>
- #include <string.h>
- Struct Employee
- {
- Int id;
- Char name[20];
- Struct Date
- {
- Int dd;
- Int mm;
- Int yyyy;
- }doj;
- }e1;
- Int main( )
- {
- //storing employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- e1.doj.dd=10;
- e1.doj.mm=11;
- e1.doj.yyyy=2014;
- //printing first employee information
- Printf( "employee id : %d\n", e1.id);
- Printf( "employee name : %s\n", e1.name);
- Printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
- Return 0;
- }
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
4. Write an Example for array within structure
Struct Student
{
Int Roll;
Char Name[25];
Int Marks[3]; //Statement 1 : array of marks
Int Total;
Float Avg;
};
Void main()
{
Inti;
Struct Student S;
Printf("\n\nEnter Student Roll : ");
Scanf("%d",&S.Roll);
Printf("\n\nEnter Student Name : ");
Scanf("%s",&S.Name);
S.Total = 0;
For(i=0;i<3;i++)
{
Printf("\n\nEnter Marks %d : ",i+1);
Scanf("%d",&S.Marks[i]);
S.Total = S.Total + S.Marks[i];
}
S.Avg = S.Total / 3;
Printf("\nRoll : %d",S.Roll);
Printf("\nName : %s",S.Name);
Printf("\nTotal : %d",S.Total);
Printf("\nAverage : %f",S.Avg);
}
Output :
Enter Student Roll : 10
Enter Student Name : Kumar
Enter Marks 1 : 78
Enter Marks 2 : 89
Enter Marks 3 : 56
Roll : 10
Name : Kumar
Total : 223
Average : 74.00000
In the above example, we have created an array Marks[ ] inside structure representing
3 marks of a single student. Marks[ ] is now a member of structure student and to
Access Marks[ ] we have used dot operator(.) along with object S.
5. Explain with example declaration of structure array
Structure is collection of different data type. An object of structure represents a singlerecord in memory, if we want more than one record of structure type, we have tocreate an array of structure or object. As we know, an array is a collection of similartype, therefore an array can be of structure type.
Syntax for declaring structure array
Struct struct-name
{
Datatype var1;
Datatype var2;
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj [ size ];
Example for declaring structure array
#include<stdio.h>
Struct Employee
{
Int Id;
Char Name[25];
Int Age;
Long Salary;
};
Void main()
{
Inti;
Struct Employee Emp[ 3 ]; //Statement 1
For(i=0;i<3;i++)
{
Printf("\nEnter details of %d Employee",i+1);
Printf("\n\tEnter Employee Id : ");
Scanf("%d",&Emp[i].Id);
Printf("\n\tEnter Employee Name : ");
Scanf("%s",&Emp[i].Name);
Printf("\n\tEnter Employee Age : ");
Scanf("%d",&Emp[i].Age);
Printf("\n\tEnter Employee Salary : ");
Scanf("%ld",&Emp[i].Salary);
}
Printf("\nDetails of Employees");
For(i=0;i<3;i++)
Printf("\n%d\t%s\t%d\t%ld",Emp[i].Id,Emp[i].Name,Emp[i].Age,Emp[i].Salary);
}
Output :
Enter details of 1 Employee
Enter Employee Id : 101
Enter Employee Name : Suresh
Enter Employee Age : 29
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name : Mukesh
Enter Employee Age : 31
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Ramesh
Enter Employee Age : 28
Enter Employee Salary : 47000
Details of Employees
101 Suresh 29 45000
102 Mukesh 31 51000
103 Ramesh 28 47000
In the above example, we are getting and displaying the data of 3 employee using
Array of object. Statement 1 is creating an array of Employee Emp to store the records
Of 3 employees.
6. What is Operations on individual members explain with examples?
Here we will see what type of operations can be performed on struct variables. Here basically one operation can be performed for struct. The operation is assignment operation. Some other operations like equality check or other are not available for stack.
Example
#include <stdio.h>
Typedef struct { //define a structure for complex objects
Int real, imag;
}complex;
Void displayComplex(complex c){
Printf("(%d + %di)\n", c.real, c.imag);
}
Main() {
Complex c1 = {5, 2};
Complex c2 = {8, 6};
Printf("Complex numbers are:\n");
DisplayComplex(c1);
DisplayComplex(c2);
}
Output
Complex numbers are:
(5 + 2i)
(8 + 6i)
This works fine as we have assigned some values into struct. Now if we want to compare two struct objects, let us see the difference.
Example
#include <stdio.h>
Typedef struct { //define a structure for complex objects
Int real, imag;
}complex;
Void displayComplex(complex c){
Printf("(%d + %di)\n", c.real, c.imag);
}
Main() {
Complex c1 = {5, 2};
Complex c2 = c1;
Printf("Complex numbers are:\n");
DisplayComplex(c1);
DisplayComplex(c2);
If(c1 == c2){
Printf("Complex numbers are same.");
} else {
Printf("Complex numbers are not same.");
}
}
Output
[Error] invalid operands to binary == (have 'complex' and 'complex')
7. Explain with examples Copying and comparing structures variables
Two variables of the same structure type can be copied the same way as ordinary variables. If persona1 and person2 belong to the same structure, then the following statements Are valid.
Person1 = person2;
Person2 = person1;
C does not permit any logical operators on structure variables In case, we need to compare them, we may do so by comparing members individually.
Person1 = = person2
Person1 ! = person2
Statements are not permitted.
Ex: Program for comparison of structure variables
Struct class
{
Int no;
Char name [20];
Float marks;
}
Main ( )
{
Int x;
Struct class stu1 = { 111, “Rao”, 72.50};
Struct class stu2 = {222, “Reddy”, 67.80};
Struct class stu3;
Stu3 = stu2;
x = ( ( stu3.no= = stu2.no) && ( stu3.marks = = stu2.marks))?1:0;
If ( x==1)
{
Printf (“ \n student 2 & student 3 are same \n”);
Printf (“%d\t%s\t%f “ stu3.no, stu3.name, stu3.marks);
}
Else
Printf ( “\n student 2 and student 3 are different “);
}
Out Put:
Student 2 and student 3 are same
222 reddy 67.0000
8. What is designated Initialization?
Designated Initialization allows structure members to be initialized in any order. This feature has been added in C99 standards.
#include<stdio.h>
Struct Point { Int x, y, z; };
Int main() { // Examples of initialization using designated initialization Struct Point p1 = {.y = 0, .z = 1, .x = 2}; Struct Point p2 = {.x = 20};
Printf ("x = %d, y = %d, z = %d\n", p1.x, p1.y, p1.z); Printf ("x = %d", p2.x); Return 0; } |
Output:
x = 2, y = 0, z = 1
x = 20
This feature is not available in C++ and works only in C.
9. Give some examples of C Structure
Let's see a simple example of structure in C language.
- #include<stdio.h>
- #include <string.h>
- Struct employee
- { int id;
- Char name[50];
- }e1; //declaring e1 variable for structure
- Int main( )
- {
- //store first employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- //printing first employee information
- Printf( "employee 1 id : %d\n", e1.id);
- Printf( "employee 1 name : %s\n", e1.name);
- Return 0;
- }
Output:
Employee 1 id : 101
Employee 1 name : Sonoo Jaiswal
Let's see another example of the structure in C language to store many employees information.
- #include<stdio.h>
- #include <string.h>
- Struct employee
- { int id;
- Char name[50];
- Float salary;
- }e1,e2; //declaring e1 and e2 variables for structure
- Int main( )
- {
- //store first employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- e1.salary=56000;
- //store second employee information
- e2.id=102;
- Strcpy(e2.name, "James Bond");
- e2.salary=126000;
- //printing first employee information
- Printf( "employee 1 id : %d\n", e1.id);
- Printf( "employee 1 name : %s\n", e1.name);
- Printf( "employee 1 salary : %f\n", e1.salary);
- //printing second employee information
- Printf( "employee 2 id : %d\n", e2.id);
- Printf( "employee 2 name : %s\n", e2.name);
- Printf( "employee 2 salary : %f\n", e2.salary);
- Return 0;
- }
Output:
Employee 1 id : 101
Employee 1 name : Sonoo Jaiswal
Employee 1 salary : 56000.000000
Employee 2 id : 102
Employee 2 name : James Bond
Employee 2 salary : 126000.000000
10. Explain in detail Strings in C language with all the aspects and examples
The string can be defined as the one-dimensional array of characters terminated by a null ('\0'). The character array or the string is used to manipulate text such as word or sentences. Each character in the array occupies one byte of memory, and the last character must always be 0. The termination character ('\0') is important in a string since it is the only way to identify where the string ends. When we define a string as char s[10], the character s[10] is implicitly initialized with the null in the memory.
There are two ways to declare a string in c language.
- By char array
- By string literal
Let's see the example of declaring string by char array in C language.
- Char ch[10]={'j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't', '\0'};
As we know, array index starts from 0, so it will be represented as in the figure given below.
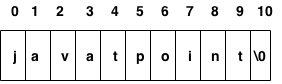
While declaring string, size is not mandatory. So we can write the above code as given below:
- Char ch[]={'j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't', '\0'};
We can also define the string by the string literal in C language. For example:
- Char ch[]="javatpoint";
In such case, '\0' will be appended at the end of the string by the compiler.
Difference between char array and string literal
There are two main differences between char array and literal.
- We need to add the null character '\0' at the end of the array by ourself whereas, it is appended internally by the compiler in the case of the character array.
- The string literal cannot be reassigned to another set of characters whereas, we can reassign the characters of the array.
String Example in C
Let's see a simple example where a string is declared and being printed. The '%s' is used as a format specifier for the string in c language.
- #include<stdio.h>
- #include <string.h>
- Int main(){
- Char ch[11]={'j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't', '\0'};
- Char ch2[11]="javatpoint";
- Printf("Char Array Value is: %s\n", ch);
- Printf("String Literal Value is: %s\n", ch2);
- Return 0;
- }
Output
Char Array Value is: javatpoint
String Literal Value is: javatpoint
Traversing String
Traversing the string is one of the most important aspects in any of the programming languages. We may need to manipulate a very large text which can be done by traversing the text. Traversing string is somewhat different from the traversing an integer array. We need to know the length of the array to traverse an integer array, whereas we may use the null character in the case of string to identify the end the string and terminate the loop.
Hence, there are two ways to traverse a string.
- By using the length of string
- By using the null character.
Let's discuss each one of them.
Using the length of string
Let's see an example of counting the number of vowels in a string.
- #include<stdio.h>
- Void main ()
- {
- Char s[11] = "javatpoint";
- Int i = 0;
- Int count = 0;
- While(i<11)
- {
- If(s[i]=='a' || s[i] == 'e' || s[i] == 'i' || s[i] == 'u' || s[i] == 'o')
- {
- Count ++;
- }
- i++;
- }
- Printf("The number of vowels %d",count);
- }
Output
The number of vowels 4
Using the null character
Let's see the same example of counting the number of vowels by using the null character.
- #include<stdio.h>
- Void main ()
- {
- Char s[11] = "javatpoint";
- Int i = 0;
- Int count = 0;
- While(s[i] != NULL)
- {
- If(s[i]=='a' || s[i] == 'e' || s[i] == 'i' || s[i] == 'u' || s[i] == 'o')
- {
- Count ++;
- }
- i++;
- }
- Printf("The number of vowels %d",count);
- }
Output
The number of vowels 4
Accepting string as the input
Till now, we have used scanf to accept the input from the user. However, it can also be used in the case of strings but with a different scenario. Consider the below code which stores the string while space is encountered.
- #include<stdio.h>
- Void main ()
- {
- Char s[20];
- Printf("Enter the string?");
- Scanf("%s",s);
- Printf("You entered %s",s);
- }
Output
Enter the string?javatpoint is the best
You entered javatpoint
It is clear from the output that, the above code will not work for space separated strings. To make this code working for the space separated strings, the minor changed required in the scanf function, i.e., instead of writing scanf("%s",s), we must write: scanf("%[^\n]s",s) which instructs the compiler to store the string s while the new line (\n) is encountered. Let's consider the following example to store the space-separated strings.
- #include<stdio.h>
- Void main ()
- {
- Char s[20];
- Printf("Enter the string?");
- Scanf("%[^\n]s",s);
- Printf("You entered %s",s);
- }
Output
Enter the string?javatpoint is the best
You entered javatpoint is the best
Here we must also notice that we do not need to use address of (&) operator in scanf to store a string since string s is an array of characters and the name of the array, i.e., s indicates the base address of the string (character array) therefore we need not use & with it.
Some important points
However, there are the following points which must be noticed while entering the strings by using scanf.
- The compiler doesn't perform bounds checking on the character array. Hence, there can be a case where the length of the string can exceed the dimension of the character array which may always overwrite some important data.
- Instead of using scanf, we may use gets() which is an inbuilt function defined in a header file string.h. The gets() is capable of receiving only one string at a time.
Pointers with strings
We have used pointers with the array, functions, and primitive data types so far. However, pointers can be used to point to the strings. There are various advantages of using pointers to point strings. Let us consider the following example to access the string via the pointer.
- #include<stdio.h>
- Void main ()
- {
- Char s[11] = "javatpoint";
- Char *p = s; // pointer p is pointing to string s.
- Printf("%s",p); // the string javatpoint is printed if we print p.
- }
Output
Javatpoint
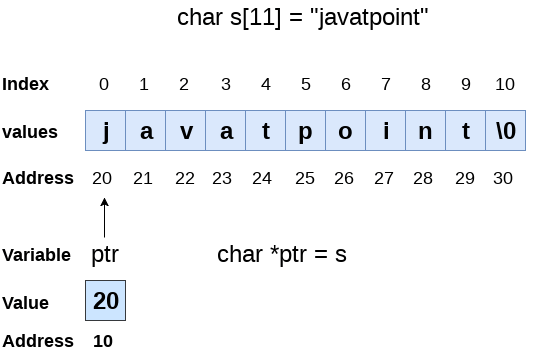
As we know that string is an array of characters, the pointers can be used in the same way they were used with arrays. In the above example, p is declared as a pointer to the array of characters s. P affects similar to s since s is the base address of the string and treated as a pointer internally. However, we can not change the content of s or copy the content of s into another string directly. For this purpose, we need to use the pointers to store the strings. In the following example, we have shown the use of pointers to copy the content of a string into another.
- #include<stdio.h>
- Void main ()
- {
- Char *p = "hello javatpoint";
- Printf("String p: %s\n",p);
- Char *q;
- Printf("copying the content of p into q...\n");
- q = p;
- Printf("String q: %s\n",q);
- }
Output
String p: hello javatpoint
Copying the content of p into q...
String q: hello javatpoint
Once a string is defined, it cannot be reassigned to another set of characters. However, using pointers, we can assign the set of characters to the string. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Char *p = "hello javatpoint";
- Printf("Before assigning: %s\n",p);
- p = "hello";
- Printf("After assigning: %s\n",p);
- }
Output
Before assigning: hello javatpoint
After assigning: hello