Unit - 4
Instruction Set and Programming
Q1) What is addressing mode?
A1)
The addressing mode is the method to specify the operand of an instruction. The operations require the following:
- The operator or opcode which determines what will be done.
- The operands which define data to be used in the operation.
Q2) Explain instruction syntax?
A2)
An 8051 Instruction consists of an Opcode followed by Operand(s) of size Zero Byte, One Byte or Two Bytes.
The Op-Code part contains the mnemonic, which specifies the type of operation to be performed. All Mnemonics or the Opcode part of the instruction are of One Byte size.
The Operand part of the instruction defines the data being processed by the instructions. There can multiple operands and the format of instruction is as follows:
Mnemonic Destination Operand, Source Operand
A simple instruction consists of just the opcode. Other instructions may include one or more operands. Instruction can be one-byte instruction, which contains only opcode, or two-byte instructions, where the second byte is the operand or three- byte instructions, where the operand makes up the second and third byte.
Q3) Explain the data types in 8051?
A3)
DB (define byte)
- It is used to define the 8-bit data.
- When DB is used to define data, the numbers can be in decimal, binary, hex, or ASCII formats.
- “B” (binary) and “H” (hexadecimal) is required.
- To indicate ASCII, simply place the characters in quotation marks
- The DB directive is the only directive that can be used to define ASCII strings larger than two characters; therefore, it should be used for all ASCII data definitions.
Following are some DB examples:
ORG 2000H
DATA1: DB 28 ;DECIMAL NUMBER 28
DATA2: DB 1234h ;HEXADECIMAL
DATA3: DB "ABCD" ;ASCII CHARACTER
Q4) What is a subroutine?
A4)
Subroutine is a group of instructions written separately from the main program to perform function that occurs repeatedly in the main program. When you call a subroutine implementation the current program is stopped and PC is loaded with the memory location of the subroutine running upto RET instruction which marks the end of the subroutine where we produce a return to the main program.
The functions of the subroutines are:
- Perform specific functions which are not operating on their own.
- Always linked to the major program or other subroutines.
- Can be called as many times as necessary to reduce the code of the program.
- Allow division of program blocks as per the function of the structure.
Q5) Explain Register and Direct Addressing mode?
A5)
Register Addressing
In this addressing mode the register will hold the data.
One of the eight general registers (R0 to R7) is used and specified as the operand.
Eg. MOV A,R0 ;
ADD A,R6
R0 – R7 will be selected from the current selection of register bank. The default register bank will be bank 0.
Direct addressing
There are two ways to access the internal memory that is by using direct address and indirect address.
In direct addressing mode we not only address the internal memory but SFRs also.
In direct addressing, an 8 bit internal data memory address is specified as part of the instruction and hence, it can specify the address only in the range of 00H to FFH.
In this addressing mode, data is obtained directly from the memory.
Eg. MOV A,60h
ADD A,30h
Q6) Explain Relative and Indexed addressing mode?
A6)
Relative addressing is used only with conditional jump instructions. The relative address, (offset), is an 8 bit signed number, which is automatically added to the PC to make the address of the next instruction.
The 8 bit signed offset value gives an address range of +127 to —128 locations.
The jump destination is usually specified using label and the assembler calculates the jump offset accordingly.
Eg. SJMP LOOP1 JC BACK
Indexed addressing
In indexed addressing, either the program counter (PC), or the data pointer (DTPR)—is used to hold the base address, and A is used to hold the offset address.
Adding the value of the base address to the value of the offset address forms the effective address.
Effective address = Base address + value of offset address.
Indexed addressing is used with JMP or MOVC instructions.
Look up tables are easily implemented with the help of index addressing.
Eg. MOVC A, @A+DPTR // copies the contents of memory location pointed by the sum of the accumulator A and the DPTR into accumulator A.
MOVC A, @A+PC // copies the contents of memory location pointed by the sum of the accumulator A and the program counter into accumulator A.
Q7) Explain Data Transfer Instructions?
A7)
In this group, the instructions perform data transfer operations of the following types.
a. Move the contents of a register Rn to A
- MOV A,R2
- MOV A,R7
b. Move the contents of a register A to Rn
i. MOV R4,A
Ii. MOV R1,A
c. Move an immediate 8 bit data to register A or to Rn or to a memory location(direct or indirect)
i. MOV A, #45H
Ii. MOV R6, #51H i
iii.MOV 30H, #44H
iv. MOV @R0, #0E8H
v. MOV DPTR, #0F5A2H
vi. MOV DPTR, #5467H
d. Move the contents of a memory location to A or A to a memory location using direct and indirect addressing
i. MOV A, 65H
Ii. MOV A, @R0
Iii.MOV 45H, A
Iii. MOV @R1, A
e. Move the contents of a memory location to Rn or Rn to a memory location using direct addressing
i. MOV R3, 65H
Ii. MOV 45H, R2
f. Move the contents of memory location to another memory location using direct and indirect addressing
i. MOV 47H, 65H
Ii. MOV 45H, @R0
g. Move the contents of an external memory to A or A to an external memory
i. MOVX A,@R1
Ii. MOVX @R0,A
Iv. MOVX A,@DPTR iv. MOVX@DPTR,A
h. Move the contents of program memory to A
i. MOVC A, @A+PC
Ii. MOVC A, @A+DPTR.
Q8) Explain Arithmetic Instructions?
A8)
The 8051 can perform addition, subtraction. Multiplication and division operations on 8- bit numbers.
Addition
In this group, we have instructions to
i. Add the contents of A with immediate data with or without carry.
- ADD A, #45H
- ADDC A, #OB4H
Ii. Add the contents of A with register Rn with or without carry.
- ADD A, R5
- ADDC A, R2
Iii. Add the contents of A with contents of memory with or without carry using direct and indirect addressing
- ADD A, 51H
- ADDC A, 75H
- ADD A, @R1
- ADDC A, @R0 CY AC and OV flags will be affected by this operation.
Subtraction
In this group, we have instructions to
i. Subtract the contents of A with immediate data with or without carry.
i. SUBB A, #45H
Ii. SUBB A, #OB4H
Ii. Subtract the contents of A with register Rn with or without carry.
- SUBB A, R5
- SUBB A, R2
- Subtract the contents of A with contents of memory with or without carry using direct and indirect addressing
- SUBB A, 51H
- SUBB A, 75H
- SUBB A, @R1
- SUBB A, @R0 CY AC and OV flags will be affected by this operation.
Multiplication
MUL AB.
This instruction multiplies two 8 bit unsigned numbers which are stored in A and B register. After multiplication the lower byte of the result will be stored in accumulator and higher byte of result will be stored in B register.
Eg. MOV A,#45H ;[A]=45H
MOV B,#0F5H ;[B]=F5H
MUL AB ;[A] x [B] = 45 x F5 = 4209 ;[A]=09H, [B]=42H
Division
DIV AB.
This instruction divides the 8 bit unsigned number which is stored in A by the 8 bit unsigned number which is stored in B register. After division the result will be stored in accumulator and remainder will be stored in B register.
Eg. MOV A,#45H ;[A]=0E8H
MOV B,#0F5H ;[B]=1BH
DIV AB ;[A] / [B] = E8 /1B = 08 H with remainder 10H ;[A] = 08H, [B]=10H
DA A (Decimal Adjust After Addition).
When two BCD numbers are added, the answer is a non-BCD number. To get the result in BCD, we use DA A instruction after the addition.
DA A works as follows.
If lower nibble is greater than 9 or auxiliary carry is 1, 6 is added to lower nibble.
If upper nibble is greater than 9 or carry is 1, 6 is added to upper nibble.
Eg 1: MOV A,#23H
MOV R1,#55H
ADD A,R1 // [A]=78
DA A // [A]=78 no changes in the accumulator after da a
Eg 2: MOV A,#53H
MOV R1,#58H
ADD A,R1 // [A]=ABh
DA A // [A]=11, C=1 .
ANSWER IS 111. Accumulator data is changed after DA A
Q9) Write a short note on Rotate instructions?
A9)
RR A
This instruction is rotate right the accumulator.
Its operation is illustrated below.
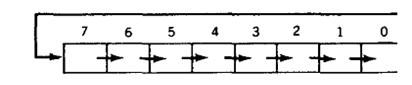
Each bit is shifted one location to the right, with bit 0 going to bit 7.
RL A Rotate left the accumulator.
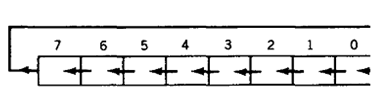
Each bit is shifted one location to the left, with bit 7 going to bit 0
RRC A Rotate right through the carry
Each bit is shifted one location to the right, with bit 0 going into the carry bit in the PSW, while the carry was at goes into bit 7
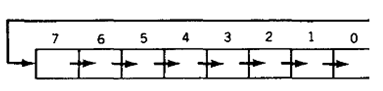
RLC A Rotate left through the carry.
Each bit is shifted one location to the left, with bit 7 going into the carry bit in the PSW, while the carry goes into bit 0.
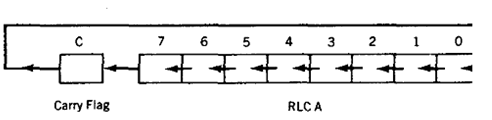
SWAP
The SWAP instruction can be thought of as a rotation of nibbles in the A register.
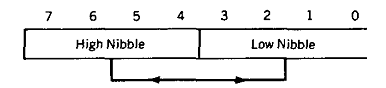
Example:
OY A,#OA5h | A = I0l00I0lb = A5h |
RR A | A = I I0I00I0b = D2h |
RR A | A = 0l I0l00lb = 69h |
RR A | A = I0I l0I00b = B4h |
RR A | A = 0I0l I0I0b = 5Ah |
SWAP A | A= I0I00I0lb = A5h |
CLR C | C = 0; A = I0I00I0lb = A5h |
RRC A | C = I; A= 0I0I00I0b = 52h |
RRC A | C = 0; A = l0I0J00lb = A9h |
RL A | A= 0I0I00llb = 53h |
RL A | A = I0I00l I0b = A6h |
SWAP A | C = 0; A = 0l 101010b = 6Ah |
RLC A | C = 0; A = 1 I0I0l00b = D4h |
RLC A | C = l; A= I0I0l000b = A8h |
SWAP A | C = l; A= l000I0I0b = 8Ah |
Q10) Write a program to add two 16 bit numbers stored at locations 51H-52H and 55H-56H and store the result in locations 40H, 41H and 42H. Assume that the least significant byte of data and the result is stored in low address and the most significant byte of data or the result is stored in high address.
A10)
ORG 0000H ; Set program counter 0000H
MOV A,51H ; Load the contents of memory location 51H into A
ADD A,55H ; Add the contents of 55H with contents of A
MOV 40H,A ; Save the LS byte of the result in location 40H
MOV A,52H ; Load the contents of 52H into A
ADDC A,56H ; Add the contents of 56H and CY flag with A
MOV 41H,A ; Save the second byte of the result in 41H
MOV A,#00 ; Load 00H into A
ADDC A,#00 ; Add the immediate data 00H and CY to A
MOV 42H,A ; Save the MS byte of the result in location 42H
END
Q11) Write a program to store data FFH into RAM memory locations 50H to 58H using direct addressing mode
A11)
ORG 0000H ; Set program counter 0000H
MOV A, #0FFH ; Load FFH into A
MOV 50H, A ; Store contents of A in location 50H
MOV 51H, A ; Store contents of A in location 5IH
MOV 52H, A ; Store contents of A in location 52H
MOV 53H, A ; Store contents of A in location 53H
MOV 54H, A ; Store contents of A in location 54H
MOV 55H, A ; Store contents of A in location 55H
MOV 56H, A ; Store contents of A in location 56H
MOV 57H, A ; Store contents of A in location 57H
MOV 58H, A ; Store contents of A in location 58H
END
Q12) Write a program to multiply two 8 bit numbers stored at locations 70H and 71H and store the result at memory locations 52H and 53H. Assume that the least significant byte of the result is stored in low address.
A12)
ORG 0000H ; Set program counter 00 OH
MOV A, 70H ; Load the contents of memory location 70h into A
MOV B, 71H ; Load the contents of memory location 71H into B
MUL AB ; Perform multiplication
MOV 52H,A ; Save the least significant byte of the result in location 52H
MOV 53H,B ;Save the most significant byte of the result in location 53H
END
Q13) Write a program to compute 1 + 2 + 3 + N (say N=15) and save the sum at70H
A13)
ORG 0000H ; Set program counter 0000H
N EQU 15
MOV R0,#00 ; Clear R0
CLR A ; Clear A
Again:INC R0 ; Increment R0
ADD A, R0 ; Add the contents of R0 with A
CJNE R0,#N,again ; Loop until counter, R0, N
MOV 70H,A ; Save the result in location 70H
END
Q14) Write a program to find the average of five 8 bit numbers. Store the result in 55H. (Assume that after adding five 8 bit numbers, the result is 8 bit only).
A14)
ORG 0000H
MOV 40H,#05H
MOV 41H,#55H
MOV 42H,#06H
MOV 43H,#1AH
MOV 44H,#09H
MOV R0,#40H
MOV R5,#05H
MOV B,R5
CLR A
Loop: ADD A,@RO
INC RO DJNZ R5,Loop
DIV AB
MOV 55H,A
END
Q15) Write a program to find the cube of an 8 bit number program is as follows
A15)
ORG 0000H
MOV R1,#N
MOV A,R1
MOV B,R1
MUL AB //SQUARE IS COMPUTED
MOV R2, B
MOV B, R1
MUL AB
MOV 50,A
MOV 51,B
MOV A,R2
MOV B, R1
MUL AB
ADD A, 51H
MOV 51H, A
MOV 52H, B
MOV A, # 00H
ADDC A, 52H
MOV 52H, A //CUBE IS STORED IN 52H,51H,50H
END
Q16) Write a program to count the number of and o's of 8 bit data stored in location 6000H.
A16)
ORG 00008 ; Set program counter 00008
MOV DPTR, #6000h ; Copy address 6000H to DPTR
MOVX A, @DPTR ; C o p y n u m b e r t o A
MOV R0,#08 ; C o py 0 8 i n R O
MOV R2,#00 ; C o p y 0 0 i n R 2
MOV R3,#00 ; C o p y 0 0 i n R 3
CLR C ; Clear carry flag
BACK: RLC A ; R o t a t e A t h r o u g h c a r r y f l a g
JC NEXT ; I f C F = 1 , b r a n c h t o n e x t
INC R2 ; I f C F = 0 , i n c r e m e n t R 2
AJMP NEXT2
NEXT: INC R3 ; I f C F = 1 , i n c r e m e n t R 3
NEXT2: DJNZ RO,BACK ; R e p e a t u n t i l R O i s z e r o
END
Q17) Explain Assemblers and Compilers.
A17)
Assembler
The assembly language is a low-level programming language which writes program code in terms of mnemonics. It can be used for direct hardware manipulations. It is used to write 8051 programming code efficiently with less number of clock cycles by consuming less memory compared to other high-level languages.
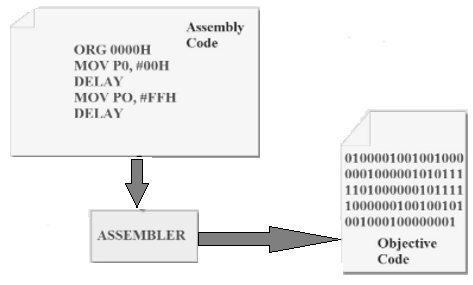
Fig. 8051 Programming
8051 Programming in Assembly Language
The assembly language is a completely hardware related programming language. It is essential that the embedded designers have sufficient knowledge on hardware of particular processor or controllers before writing the program. The assembly language is developed on mnemonics.
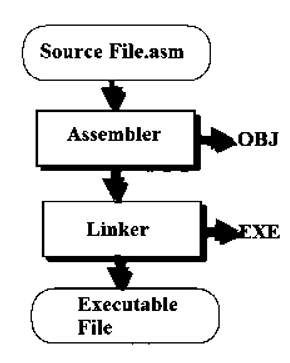
Fig. 8051 Programming in Assembly Language
- It is developed by various compilers and the “keiluvison” is best suitable for microcontroller programming development.
- Microcontrollers or processors can understand only binary language in the form of ‘0s or 1s’; An assembler converts the assembly language to binary language, and then stores in microcontroller memory to perform the specific task.
Compilers
- The name High-level language means the words and statements that are easily understood by humans.
- Few examples of High-level Languages are BASIC, C Pascal, C++ and Java. A program called Compiler will convert the Programs written in High-level languages to Machine Code.
Unit - 4
Instruction Set and Programming
Q1) What is addressing mode?
A1)
The addressing mode is the method to specify the operand of an instruction. The operations require the following:
- The operator or opcode which determines what will be done.
- The operands which define data to be used in the operation.
Q2) Explain instruction syntax?
A2)
An 8051 Instruction consists of an Opcode followed by Operand(s) of size Zero Byte, One Byte or Two Bytes.
The Op-Code part contains the mnemonic, which specifies the type of operation to be performed. All Mnemonics or the Opcode part of the instruction are of One Byte size.
The Operand part of the instruction defines the data being processed by the instructions. There can multiple operands and the format of instruction is as follows:
Mnemonic Destination Operand, Source Operand
A simple instruction consists of just the opcode. Other instructions may include one or more operands. Instruction can be one-byte instruction, which contains only opcode, or two-byte instructions, where the second byte is the operand or three- byte instructions, where the operand makes up the second and third byte.
Q3) Explain the data types in 8051?
A3)
DB (define byte)
- It is used to define the 8-bit data.
- When DB is used to define data, the numbers can be in decimal, binary, hex, or ASCII formats.
- “B” (binary) and “H” (hexadecimal) is required.
- To indicate ASCII, simply place the characters in quotation marks
- The DB directive is the only directive that can be used to define ASCII strings larger than two characters; therefore, it should be used for all ASCII data definitions.
Following are some DB examples:
ORG 2000H
DATA1: DB 28 ;DECIMAL NUMBER 28
DATA2: DB 1234h ;HEXADECIMAL
DATA3: DB "ABCD" ;ASCII CHARACTER
Q4) What is a subroutine?
A4)
Subroutine is a group of instructions written separately from the main program to perform function that occurs repeatedly in the main program. When you call a subroutine implementation the current program is stopped and PC is loaded with the memory location of the subroutine running upto RET instruction which marks the end of the subroutine where we produce a return to the main program.
The functions of the subroutines are:
- Perform specific functions which are not operating on their own.
- Always linked to the major program or other subroutines.
- Can be called as many times as necessary to reduce the code of the program.
- Allow division of program blocks as per the function of the structure.
Q5) Explain Register and Direct Addressing mode?
A5)
Register Addressing
In this addressing mode the register will hold the data.
One of the eight general registers (R0 to R7) is used and specified as the operand.
Eg. MOV A,R0 ;
ADD A,R6
R0 – R7 will be selected from the current selection of register bank. The default register bank will be bank 0.
Direct addressing
There are two ways to access the internal memory that is by using direct address and indirect address.
In direct addressing mode we not only address the internal memory but SFRs also.
In direct addressing, an 8 bit internal data memory address is specified as part of the instruction and hence, it can specify the address only in the range of 00H to FFH.
In this addressing mode, data is obtained directly from the memory.
Eg. MOV A,60h
ADD A,30h
Q6) Explain Relative and Indexed addressing mode?
A6)
Relative addressing is used only with conditional jump instructions. The relative address, (offset), is an 8 bit signed number, which is automatically added to the PC to make the address of the next instruction.
The 8 bit signed offset value gives an address range of +127 to —128 locations.
The jump destination is usually specified using label and the assembler calculates the jump offset accordingly.
Eg. SJMP LOOP1 JC BACK
Indexed addressing
In indexed addressing, either the program counter (PC), or the data pointer (DTPR)—is used to hold the base address, and A is used to hold the offset address.
Adding the value of the base address to the value of the offset address forms the effective address.
Effective address = Base address + value of offset address.
Indexed addressing is used with JMP or MOVC instructions.
Look up tables are easily implemented with the help of index addressing.
Eg. MOVC A, @A+DPTR // copies the contents of memory location pointed by the sum of the accumulator A and the DPTR into accumulator A.
MOVC A, @A+PC // copies the contents of memory location pointed by the sum of the accumulator A and the program counter into accumulator A.
Q7) Explain Data Transfer Instructions?
A7)
In this group, the instructions perform data transfer operations of the following types.
a. Move the contents of a register Rn to A
- MOV A,R2
- MOV A,R7
b. Move the contents of a register A to Rn
i. MOV R4,A
Ii. MOV R1,A
c. Move an immediate 8 bit data to register A or to Rn or to a memory location(direct or indirect)
i. MOV A, #45H
Ii. MOV R6, #51H i
iii.MOV 30H, #44H
iv. MOV @R0, #0E8H
v. MOV DPTR, #0F5A2H
vi. MOV DPTR, #5467H
d. Move the contents of a memory location to A or A to a memory location using direct and indirect addressing
i. MOV A, 65H
Ii. MOV A, @R0
Iii.MOV 45H, A
Iii. MOV @R1, A
e. Move the contents of a memory location to Rn or Rn to a memory location using direct addressing
i. MOV R3, 65H
Ii. MOV 45H, R2
f. Move the contents of memory location to another memory location using direct and indirect addressing
i. MOV 47H, 65H
Ii. MOV 45H, @R0
g. Move the contents of an external memory to A or A to an external memory
i. MOVX A,@R1
Ii. MOVX @R0,A
Iv. MOVX A,@DPTR iv. MOVX@DPTR,A
h. Move the contents of program memory to A
i. MOVC A, @A+PC
Ii. MOVC A, @A+DPTR.
Q8) Explain Arithmetic Instructions?
A8)
The 8051 can perform addition, subtraction. Multiplication and division operations on 8- bit numbers.
Addition
In this group, we have instructions to
i. Add the contents of A with immediate data with or without carry.
- ADD A, #45H
- ADDC A, #OB4H
Ii. Add the contents of A with register Rn with or without carry.
- ADD A, R5
- ADDC A, R2
Iii. Add the contents of A with contents of memory with or without carry using direct and indirect addressing
- ADD A, 51H
- ADDC A, 75H
- ADD A, @R1
- ADDC A, @R0 CY AC and OV flags will be affected by this operation.
Subtraction
In this group, we have instructions to
i. Subtract the contents of A with immediate data with or without carry.
i. SUBB A, #45H
Ii. SUBB A, #OB4H
Ii. Subtract the contents of A with register Rn with or without carry.
- SUBB A, R5
- SUBB A, R2
- Subtract the contents of A with contents of memory with or without carry using direct and indirect addressing
- SUBB A, 51H
- SUBB A, 75H
- SUBB A, @R1
- SUBB A, @R0 CY AC and OV flags will be affected by this operation.
Multiplication
MUL AB.
This instruction multiplies two 8 bit unsigned numbers which are stored in A and B register. After multiplication the lower byte of the result will be stored in accumulator and higher byte of result will be stored in B register.
Eg. MOV A,#45H ;[A]=45H
MOV B,#0F5H ;[B]=F5H
MUL AB ;[A] x [B] = 45 x F5 = 4209 ;[A]=09H, [B]=42H
Division
DIV AB.
This instruction divides the 8 bit unsigned number which is stored in A by the 8 bit unsigned number which is stored in B register. After division the result will be stored in accumulator and remainder will be stored in B register.
Eg. MOV A,#45H ;[A]=0E8H
MOV B,#0F5H ;[B]=1BH
DIV AB ;[A] / [B] = E8 /1B = 08 H with remainder 10H ;[A] = 08H, [B]=10H
DA A (Decimal Adjust After Addition).
When two BCD numbers are added, the answer is a non-BCD number. To get the result in BCD, we use DA A instruction after the addition.
DA A works as follows.
If lower nibble is greater than 9 or auxiliary carry is 1, 6 is added to lower nibble.
If upper nibble is greater than 9 or carry is 1, 6 is added to upper nibble.
Eg 1: MOV A,#23H
MOV R1,#55H
ADD A,R1 // [A]=78
DA A // [A]=78 no changes in the accumulator after da a
Eg 2: MOV A,#53H
MOV R1,#58H
ADD A,R1 // [A]=ABh
DA A // [A]=11, C=1 .
ANSWER IS 111. Accumulator data is changed after DA A
Q9) Write a short note on Rotate instructions?
A9)
RR A
This instruction is rotate right the accumulator.
Its operation is illustrated below.
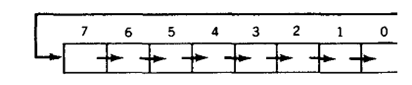
Each bit is shifted one location to the right, with bit 0 going to bit 7.
RL A Rotate left the accumulator.
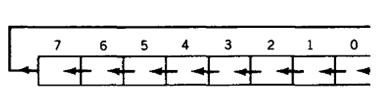
Each bit is shifted one location to the left, with bit 7 going to bit 0
RRC A Rotate right through the carry
Each bit is shifted one location to the right, with bit 0 going into the carry bit in the PSW, while the carry was at goes into bit 7
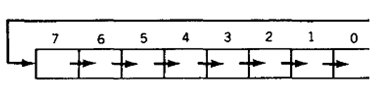
RLC A Rotate left through the carry.
Each bit is shifted one location to the left, with bit 7 going into the carry bit in the PSW, while the carry goes into bit 0.
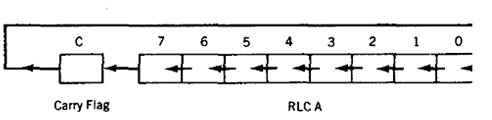
SWAP
The SWAP instruction can be thought of as a rotation of nibbles in the A register.
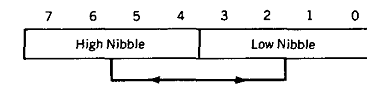
Example:
OY A,#OA5h | A = I0l00I0lb = A5h |
RR A | A = I I0I00I0b = D2h |
RR A | A = 0l I0l00lb = 69h |
RR A | A = I0I l0I00b = B4h |
RR A | A = 0I0l I0I0b = 5Ah |
SWAP A | A= I0I00I0lb = A5h |
CLR C | C = 0; A = I0I00I0lb = A5h |
RRC A | C = I; A= 0I0I00I0b = 52h |
RRC A | C = 0; A = l0I0J00lb = A9h |
RL A | A= 0I0I00llb = 53h |
RL A | A = I0I00l I0b = A6h |
SWAP A | C = 0; A = 0l 101010b = 6Ah |
RLC A | C = 0; A = 1 I0I0l00b = D4h |
RLC A | C = l; A= I0I0l000b = A8h |
SWAP A | C = l; A= l000I0I0b = 8Ah |
Q10) Write a program to add two 16 bit numbers stored at locations 51H-52H and 55H-56H and store the result in locations 40H, 41H and 42H. Assume that the least significant byte of data and the result is stored in low address and the most significant byte of data or the result is stored in high address.
A10)
ORG 0000H ; Set program counter 0000H
MOV A,51H ; Load the contents of memory location 51H into A
ADD A,55H ; Add the contents of 55H with contents of A
MOV 40H,A ; Save the LS byte of the result in location 40H
MOV A,52H ; Load the contents of 52H into A
ADDC A,56H ; Add the contents of 56H and CY flag with A
MOV 41H,A ; Save the second byte of the result in 41H
MOV A,#00 ; Load 00H into A
ADDC A,#00 ; Add the immediate data 00H and CY to A
MOV 42H,A ; Save the MS byte of the result in location 42H
END
Q11) Write a program to store data FFH into RAM memory locations 50H to 58H using direct addressing mode
A11)
ORG 0000H ; Set program counter 0000H
MOV A, #0FFH ; Load FFH into A
MOV 50H, A ; Store contents of A in location 50H
MOV 51H, A ; Store contents of A in location 5IH
MOV 52H, A ; Store contents of A in location 52H
MOV 53H, A ; Store contents of A in location 53H
MOV 54H, A ; Store contents of A in location 54H
MOV 55H, A ; Store contents of A in location 55H
MOV 56H, A ; Store contents of A in location 56H
MOV 57H, A ; Store contents of A in location 57H
MOV 58H, A ; Store contents of A in location 58H
END
Q12) Write a program to multiply two 8 bit numbers stored at locations 70H and 71H and store the result at memory locations 52H and 53H. Assume that the least significant byte of the result is stored in low address.
A12)
ORG 0000H ; Set program counter 00 OH
MOV A, 70H ; Load the contents of memory location 70h into A
MOV B, 71H ; Load the contents of memory location 71H into B
MUL AB ; Perform multiplication
MOV 52H,A ; Save the least significant byte of the result in location 52H
MOV 53H,B ;Save the most significant byte of the result in location 53H
END
Q13) Write a program to compute 1 + 2 + 3 + N (say N=15) and save the sum at70H
A13)
ORG 0000H ; Set program counter 0000H
N EQU 15
MOV R0,#00 ; Clear R0
CLR A ; Clear A
Again:INC R0 ; Increment R0
ADD A, R0 ; Add the contents of R0 with A
CJNE R0,#N,again ; Loop until counter, R0, N
MOV 70H,A ; Save the result in location 70H
END
Q14) Write a program to find the average of five 8 bit numbers. Store the result in 55H. (Assume that after adding five 8 bit numbers, the result is 8 bit only).
A14)
ORG 0000H
MOV 40H,#05H
MOV 41H,#55H
MOV 42H,#06H
MOV 43H,#1AH
MOV 44H,#09H
MOV R0,#40H
MOV R5,#05H
MOV B,R5
CLR A
Loop: ADD A,@RO
INC RO DJNZ R5,Loop
DIV AB
MOV 55H,A
END
Q15) Write a program to find the cube of an 8 bit number program is as follows
A15)
ORG 0000H
MOV R1,#N
MOV A,R1
MOV B,R1
MUL AB //SQUARE IS COMPUTED
MOV R2, B
MOV B, R1
MUL AB
MOV 50,A
MOV 51,B
MOV A,R2
MOV B, R1
MUL AB
ADD A, 51H
MOV 51H, A
MOV 52H, B
MOV A, # 00H
ADDC A, 52H
MOV 52H, A //CUBE IS STORED IN 52H,51H,50H
END
Q16) Write a program to count the number of and o's of 8 bit data stored in location 6000H.
A16)
ORG 00008 ; Set program counter 00008
MOV DPTR, #6000h ; Copy address 6000H to DPTR
MOVX A, @DPTR ; C o p y n u m b e r t o A
MOV R0,#08 ; C o py 0 8 i n R O
MOV R2,#00 ; C o p y 0 0 i n R 2
MOV R3,#00 ; C o p y 0 0 i n R 3
CLR C ; Clear carry flag
BACK: RLC A ; R o t a t e A t h r o u g h c a r r y f l a g
JC NEXT ; I f C F = 1 , b r a n c h t o n e x t
INC R2 ; I f C F = 0 , i n c r e m e n t R 2
AJMP NEXT2
NEXT: INC R3 ; I f C F = 1 , i n c r e m e n t R 3
NEXT2: DJNZ RO,BACK ; R e p e a t u n t i l R O i s z e r o
END
Q17) Explain Assemblers and Compilers.
A17)
Assembler
The assembly language is a low-level programming language which writes program code in terms of mnemonics. It can be used for direct hardware manipulations. It is used to write 8051 programming code efficiently with less number of clock cycles by consuming less memory compared to other high-level languages.
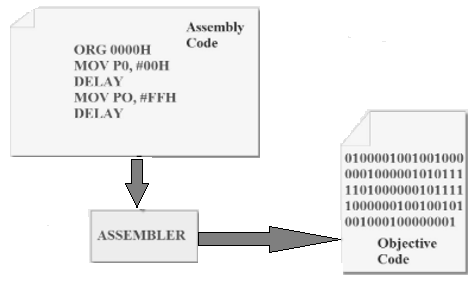
Fig. 8051 Programming
8051 Programming in Assembly Language
The assembly language is a completely hardware related programming language. It is essential that the embedded designers have sufficient knowledge on hardware of particular processor or controllers before writing the program. The assembly language is developed on mnemonics.
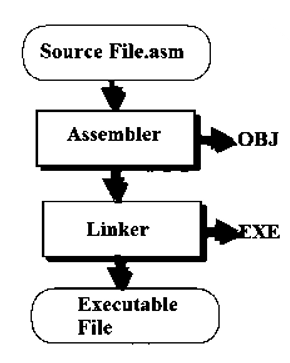
Fig. 8051 Programming in Assembly Language
- It is developed by various compilers and the “keiluvison” is best suitable for microcontroller programming development.
- Microcontrollers or processors can understand only binary language in the form of ‘0s or 1s’; An assembler converts the assembly language to binary language, and then stores in microcontroller memory to perform the specific task.
Compilers
- The name High-level language means the words and statements that are easily understood by humans.
- Few examples of High-level Languages are BASIC, C Pascal, C++ and Java. A program called Compiler will convert the Programs written in High-level languages to Machine Code.
Unit - 4
Instruction Set and Programming
Q1) What is addressing mode?
A1)
The addressing mode is the method to specify the operand of an instruction. The operations require the following:
- The operator or opcode which determines what will be done.
- The operands which define data to be used in the operation.
Q2) Explain instruction syntax?
A2)
An 8051 Instruction consists of an Opcode followed by Operand(s) of size Zero Byte, One Byte or Two Bytes.
The Op-Code part contains the mnemonic, which specifies the type of operation to be performed. All Mnemonics or the Opcode part of the instruction are of One Byte size.
The Operand part of the instruction defines the data being processed by the instructions. There can multiple operands and the format of instruction is as follows:
Mnemonic Destination Operand, Source Operand
A simple instruction consists of just the opcode. Other instructions may include one or more operands. Instruction can be one-byte instruction, which contains only opcode, or two-byte instructions, where the second byte is the operand or three- byte instructions, where the operand makes up the second and third byte.
Q3) Explain the data types in 8051?
A3)
DB (define byte)
- It is used to define the 8-bit data.
- When DB is used to define data, the numbers can be in decimal, binary, hex, or ASCII formats.
- “B” (binary) and “H” (hexadecimal) is required.
- To indicate ASCII, simply place the characters in quotation marks
- The DB directive is the only directive that can be used to define ASCII strings larger than two characters; therefore, it should be used for all ASCII data definitions.
Following are some DB examples:
ORG 2000H
DATA1: DB 28 ;DECIMAL NUMBER 28
DATA2: DB 1234h ;HEXADECIMAL
DATA3: DB "ABCD" ;ASCII CHARACTER
Q4) What is a subroutine?
A4)
Subroutine is a group of instructions written separately from the main program to perform function that occurs repeatedly in the main program. When you call a subroutine implementation the current program is stopped and PC is loaded with the memory location of the subroutine running upto RET instruction which marks the end of the subroutine where we produce a return to the main program.
The functions of the subroutines are:
- Perform specific functions which are not operating on their own.
- Always linked to the major program or other subroutines.
- Can be called as many times as necessary to reduce the code of the program.
- Allow division of program blocks as per the function of the structure.
Q5) Explain Register and Direct Addressing mode?
A5)
Register Addressing
In this addressing mode the register will hold the data.
One of the eight general registers (R0 to R7) is used and specified as the operand.
Eg. MOV A,R0 ;
ADD A,R6
R0 – R7 will be selected from the current selection of register bank. The default register bank will be bank 0.
Direct addressing
There are two ways to access the internal memory that is by using direct address and indirect address.
In direct addressing mode we not only address the internal memory but SFRs also.
In direct addressing, an 8 bit internal data memory address is specified as part of the instruction and hence, it can specify the address only in the range of 00H to FFH.
In this addressing mode, data is obtained directly from the memory.
Eg. MOV A,60h
ADD A,30h
Q6) Explain Relative and Indexed addressing mode?
A6)
Relative addressing is used only with conditional jump instructions. The relative address, (offset), is an 8 bit signed number, which is automatically added to the PC to make the address of the next instruction.
The 8 bit signed offset value gives an address range of +127 to —128 locations.
The jump destination is usually specified using label and the assembler calculates the jump offset accordingly.
Eg. SJMP LOOP1 JC BACK
Indexed addressing
In indexed addressing, either the program counter (PC), or the data pointer (DTPR)—is used to hold the base address, and A is used to hold the offset address.
Adding the value of the base address to the value of the offset address forms the effective address.
Effective address = Base address + value of offset address.
Indexed addressing is used with JMP or MOVC instructions.
Look up tables are easily implemented with the help of index addressing.
Eg. MOVC A, @A+DPTR // copies the contents of memory location pointed by the sum of the accumulator A and the DPTR into accumulator A.
MOVC A, @A+PC // copies the contents of memory location pointed by the sum of the accumulator A and the program counter into accumulator A.
Q7) Explain Data Transfer Instructions?
A7)
In this group, the instructions perform data transfer operations of the following types.
a. Move the contents of a register Rn to A
- MOV A,R2
- MOV A,R7
b. Move the contents of a register A to Rn
i. MOV R4,A
Ii. MOV R1,A
c. Move an immediate 8 bit data to register A or to Rn or to a memory location(direct or indirect)
i. MOV A, #45H
Ii. MOV R6, #51H i
iii.MOV 30H, #44H
iv. MOV @R0, #0E8H
v. MOV DPTR, #0F5A2H
vi. MOV DPTR, #5467H
d. Move the contents of a memory location to A or A to a memory location using direct and indirect addressing
i. MOV A, 65H
Ii. MOV A, @R0
Iii.MOV 45H, A
Iii. MOV @R1, A
e. Move the contents of a memory location to Rn or Rn to a memory location using direct addressing
i. MOV R3, 65H
Ii. MOV 45H, R2
f. Move the contents of memory location to another memory location using direct and indirect addressing
i. MOV 47H, 65H
Ii. MOV 45H, @R0
g. Move the contents of an external memory to A or A to an external memory
i. MOVX A,@R1
Ii. MOVX @R0,A
Iv. MOVX A,@DPTR iv. MOVX@DPTR,A
h. Move the contents of program memory to A
i. MOVC A, @A+PC
Ii. MOVC A, @A+DPTR.
Q8) Explain Arithmetic Instructions?
A8)
The 8051 can perform addition, subtraction. Multiplication and division operations on 8- bit numbers.
Addition
In this group, we have instructions to
i. Add the contents of A with immediate data with or without carry.
- ADD A, #45H
- ADDC A, #OB4H
Ii. Add the contents of A with register Rn with or without carry.
- ADD A, R5
- ADDC A, R2
Iii. Add the contents of A with contents of memory with or without carry using direct and indirect addressing
- ADD A, 51H
- ADDC A, 75H
- ADD A, @R1
- ADDC A, @R0 CY AC and OV flags will be affected by this operation.
Subtraction
In this group, we have instructions to
i. Subtract the contents of A with immediate data with or without carry.
i. SUBB A, #45H
Ii. SUBB A, #OB4H
Ii. Subtract the contents of A with register Rn with or without carry.
- SUBB A, R5
- SUBB A, R2
- Subtract the contents of A with contents of memory with or without carry using direct and indirect addressing
- SUBB A, 51H
- SUBB A, 75H
- SUBB A, @R1
- SUBB A, @R0 CY AC and OV flags will be affected by this operation.
Multiplication
MUL AB.
This instruction multiplies two 8 bit unsigned numbers which are stored in A and B register. After multiplication the lower byte of the result will be stored in accumulator and higher byte of result will be stored in B register.
Eg. MOV A,#45H ;[A]=45H
MOV B,#0F5H ;[B]=F5H
MUL AB ;[A] x [B] = 45 x F5 = 4209 ;[A]=09H, [B]=42H
Division
DIV AB.
This instruction divides the 8 bit unsigned number which is stored in A by the 8 bit unsigned number which is stored in B register. After division the result will be stored in accumulator and remainder will be stored in B register.
Eg. MOV A,#45H ;[A]=0E8H
MOV B,#0F5H ;[B]=1BH
DIV AB ;[A] / [B] = E8 /1B = 08 H with remainder 10H ;[A] = 08H, [B]=10H
DA A (Decimal Adjust After Addition).
When two BCD numbers are added, the answer is a non-BCD number. To get the result in BCD, we use DA A instruction after the addition.
DA A works as follows.
If lower nibble is greater than 9 or auxiliary carry is 1, 6 is added to lower nibble.
If upper nibble is greater than 9 or carry is 1, 6 is added to upper nibble.
Eg 1: MOV A,#23H
MOV R1,#55H
ADD A,R1 // [A]=78
DA A // [A]=78 no changes in the accumulator after da a
Eg 2: MOV A,#53H
MOV R1,#58H
ADD A,R1 // [A]=ABh
DA A // [A]=11, C=1 .
ANSWER IS 111. Accumulator data is changed after DA A
Q9) Write a short note on Rotate instructions?
A9)
RR A
This instruction is rotate right the accumulator.
Its operation is illustrated below.
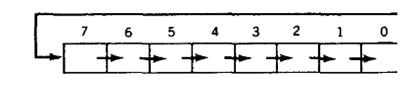
Each bit is shifted one location to the right, with bit 0 going to bit 7.
RL A Rotate left the accumulator.
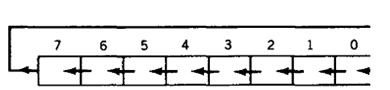
Each bit is shifted one location to the left, with bit 7 going to bit 0
RRC A Rotate right through the carry
Each bit is shifted one location to the right, with bit 0 going into the carry bit in the PSW, while the carry was at goes into bit 7
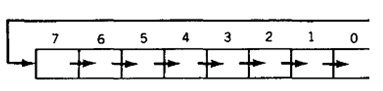
RLC A Rotate left through the carry.
Each bit is shifted one location to the left, with bit 7 going into the carry bit in the PSW, while the carry goes into bit 0.
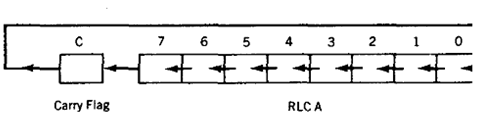
SWAP
The SWAP instruction can be thought of as a rotation of nibbles in the A register.
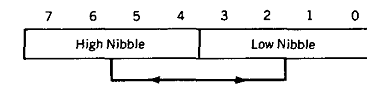
Example:
OY A,#OA5h | A = I0l00I0lb = A5h |
RR A | A = I I0I00I0b = D2h |
RR A | A = 0l I0l00lb = 69h |
RR A | A = I0I l0I00b = B4h |
RR A | A = 0I0l I0I0b = 5Ah |
SWAP A | A= I0I00I0lb = A5h |
CLR C | C = 0; A = I0I00I0lb = A5h |
RRC A | C = I; A= 0I0I00I0b = 52h |
RRC A | C = 0; A = l0I0J00lb = A9h |
RL A | A= 0I0I00llb = 53h |
RL A | A = I0I00l I0b = A6h |
SWAP A | C = 0; A = 0l 101010b = 6Ah |
RLC A | C = 0; A = 1 I0I0l00b = D4h |
RLC A | C = l; A= I0I0l000b = A8h |
SWAP A | C = l; A= l000I0I0b = 8Ah |
Q10) Write a program to add two 16 bit numbers stored at locations 51H-52H and 55H-56H and store the result in locations 40H, 41H and 42H. Assume that the least significant byte of data and the result is stored in low address and the most significant byte of data or the result is stored in high address.
A10)
ORG 0000H ; Set program counter 0000H
MOV A,51H ; Load the contents of memory location 51H into A
ADD A,55H ; Add the contents of 55H with contents of A
MOV 40H,A ; Save the LS byte of the result in location 40H
MOV A,52H ; Load the contents of 52H into A
ADDC A,56H ; Add the contents of 56H and CY flag with A
MOV 41H,A ; Save the second byte of the result in 41H
MOV A,#00 ; Load 00H into A
ADDC A,#00 ; Add the immediate data 00H and CY to A
MOV 42H,A ; Save the MS byte of the result in location 42H
END
Q11) Write a program to store data FFH into RAM memory locations 50H to 58H using direct addressing mode
A11)
ORG 0000H ; Set program counter 0000H
MOV A, #0FFH ; Load FFH into A
MOV 50H, A ; Store contents of A in location 50H
MOV 51H, A ; Store contents of A in location 5IH
MOV 52H, A ; Store contents of A in location 52H
MOV 53H, A ; Store contents of A in location 53H
MOV 54H, A ; Store contents of A in location 54H
MOV 55H, A ; Store contents of A in location 55H
MOV 56H, A ; Store contents of A in location 56H
MOV 57H, A ; Store contents of A in location 57H
MOV 58H, A ; Store contents of A in location 58H
END
Q12) Write a program to multiply two 8 bit numbers stored at locations 70H and 71H and store the result at memory locations 52H and 53H. Assume that the least significant byte of the result is stored in low address.
A12)
ORG 0000H ; Set program counter 00 OH
MOV A, 70H ; Load the contents of memory location 70h into A
MOV B, 71H ; Load the contents of memory location 71H into B
MUL AB ; Perform multiplication
MOV 52H,A ; Save the least significant byte of the result in location 52H
MOV 53H,B ;Save the most significant byte of the result in location 53H
END
Q13) Write a program to compute 1 + 2 + 3 + N (say N=15) and save the sum at70H
A13)
ORG 0000H ; Set program counter 0000H
N EQU 15
MOV R0,#00 ; Clear R0
CLR A ; Clear A
Again:INC R0 ; Increment R0
ADD A, R0 ; Add the contents of R0 with A
CJNE R0,#N,again ; Loop until counter, R0, N
MOV 70H,A ; Save the result in location 70H
END
Q14) Write a program to find the average of five 8 bit numbers. Store the result in 55H. (Assume that after adding five 8 bit numbers, the result is 8 bit only).
A14)
ORG 0000H
MOV 40H,#05H
MOV 41H,#55H
MOV 42H,#06H
MOV 43H,#1AH
MOV 44H,#09H
MOV R0,#40H
MOV R5,#05H
MOV B,R5
CLR A
Loop: ADD A,@RO
INC RO DJNZ R5,Loop
DIV AB
MOV 55H,A
END
Q15) Write a program to find the cube of an 8 bit number program is as follows
A15)
ORG 0000H
MOV R1,#N
MOV A,R1
MOV B,R1
MUL AB //SQUARE IS COMPUTED
MOV R2, B
MOV B, R1
MUL AB
MOV 50,A
MOV 51,B
MOV A,R2
MOV B, R1
MUL AB
ADD A, 51H
MOV 51H, A
MOV 52H, B
MOV A, # 00H
ADDC A, 52H
MOV 52H, A //CUBE IS STORED IN 52H,51H,50H
END
Q16) Write a program to count the number of and o's of 8 bit data stored in location 6000H.
A16)
ORG 00008 ; Set program counter 00008
MOV DPTR, #6000h ; Copy address 6000H to DPTR
MOVX A, @DPTR ; C o p y n u m b e r t o A
MOV R0,#08 ; C o py 0 8 i n R O
MOV R2,#00 ; C o p y 0 0 i n R 2
MOV R3,#00 ; C o p y 0 0 i n R 3
CLR C ; Clear carry flag
BACK: RLC A ; R o t a t e A t h r o u g h c a r r y f l a g
JC NEXT ; I f C F = 1 , b r a n c h t o n e x t
INC R2 ; I f C F = 0 , i n c r e m e n t R 2
AJMP NEXT2
NEXT: INC R3 ; I f C F = 1 , i n c r e m e n t R 3
NEXT2: DJNZ RO,BACK ; R e p e a t u n t i l R O i s z e r o
END
Q17) Explain Assemblers and Compilers.
A17)
Assembler
The assembly language is a low-level programming language which writes program code in terms of mnemonics. It can be used for direct hardware manipulations. It is used to write 8051 programming code efficiently with less number of clock cycles by consuming less memory compared to other high-level languages.
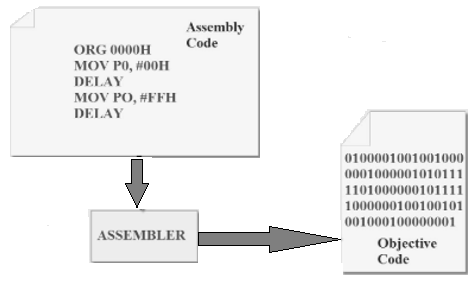
Fig. 8051 Programming
8051 Programming in Assembly Language
The assembly language is a completely hardware related programming language. It is essential that the embedded designers have sufficient knowledge on hardware of particular processor or controllers before writing the program. The assembly language is developed on mnemonics.
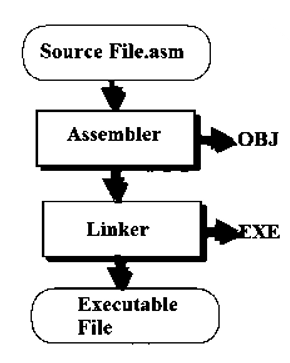
Fig. 8051 Programming in Assembly Language
- It is developed by various compilers and the “keiluvison” is best suitable for microcontroller programming development.
- Microcontrollers or processors can understand only binary language in the form of ‘0s or 1s’; An assembler converts the assembly language to binary language, and then stores in microcontroller memory to perform the specific task.
Compilers
- The name High-level language means the words and statements that are easily understood by humans.
- Few examples of High-level Languages are BASIC, C Pascal, C++ and Java. A program called Compiler will convert the Programs written in High-level languages to Machine Code.
Unit - 4
Instruction Set and Programming
Q1) What is addressing mode?
A1)
The addressing mode is the method to specify the operand of an instruction. The operations require the following:
- The operator or opcode which determines what will be done.
- The operands which define data to be used in the operation.
Q2) Explain instruction syntax?
A2)
An 8051 Instruction consists of an Opcode followed by Operand(s) of size Zero Byte, One Byte or Two Bytes.
The Op-Code part contains the mnemonic, which specifies the type of operation to be performed. All Mnemonics or the Opcode part of the instruction are of One Byte size.
The Operand part of the instruction defines the data being processed by the instructions. There can multiple operands and the format of instruction is as follows:
Mnemonic Destination Operand, Source Operand
A simple instruction consists of just the opcode. Other instructions may include one or more operands. Instruction can be one-byte instruction, which contains only opcode, or two-byte instructions, where the second byte is the operand or three- byte instructions, where the operand makes up the second and third byte.
Q3) Explain the data types in 8051?
A3)
DB (define byte)
- It is used to define the 8-bit data.
- When DB is used to define data, the numbers can be in decimal, binary, hex, or ASCII formats.
- “B” (binary) and “H” (hexadecimal) is required.
- To indicate ASCII, simply place the characters in quotation marks
- The DB directive is the only directive that can be used to define ASCII strings larger than two characters; therefore, it should be used for all ASCII data definitions.
Following are some DB examples:
ORG 2000H
DATA1: DB 28 ;DECIMAL NUMBER 28
DATA2: DB 1234h ;HEXADECIMAL
DATA3: DB "ABCD" ;ASCII CHARACTER
Q4) What is a subroutine?
A4)
Subroutine is a group of instructions written separately from the main program to perform function that occurs repeatedly in the main program. When you call a subroutine implementation the current program is stopped and PC is loaded with the memory location of the subroutine running upto RET instruction which marks the end of the subroutine where we produce a return to the main program.
The functions of the subroutines are:
- Perform specific functions which are not operating on their own.
- Always linked to the major program or other subroutines.
- Can be called as many times as necessary to reduce the code of the program.
- Allow division of program blocks as per the function of the structure.
Q5) Explain Register and Direct Addressing mode?
A5)
Register Addressing
In this addressing mode the register will hold the data.
One of the eight general registers (R0 to R7) is used and specified as the operand.
Eg. MOV A,R0 ;
ADD A,R6
R0 – R7 will be selected from the current selection of register bank. The default register bank will be bank 0.
Direct addressing
There are two ways to access the internal memory that is by using direct address and indirect address.
In direct addressing mode we not only address the internal memory but SFRs also.
In direct addressing, an 8 bit internal data memory address is specified as part of the instruction and hence, it can specify the address only in the range of 00H to FFH.
In this addressing mode, data is obtained directly from the memory.
Eg. MOV A,60h
ADD A,30h
Q6) Explain Relative and Indexed addressing mode?
A6)
Relative addressing is used only with conditional jump instructions. The relative address, (offset), is an 8 bit signed number, which is automatically added to the PC to make the address of the next instruction.
The 8 bit signed offset value gives an address range of +127 to —128 locations.
The jump destination is usually specified using label and the assembler calculates the jump offset accordingly.
Eg. SJMP LOOP1 JC BACK
Indexed addressing
In indexed addressing, either the program counter (PC), or the data pointer (DTPR)—is used to hold the base address, and A is used to hold the offset address.
Adding the value of the base address to the value of the offset address forms the effective address.
Effective address = Base address + value of offset address.
Indexed addressing is used with JMP or MOVC instructions.
Look up tables are easily implemented with the help of index addressing.
Eg. MOVC A, @A+DPTR // copies the contents of memory location pointed by the sum of the accumulator A and the DPTR into accumulator A.
MOVC A, @A+PC // copies the contents of memory location pointed by the sum of the accumulator A and the program counter into accumulator A.
Q7) Explain Data Transfer Instructions?
A7)
In this group, the instructions perform data transfer operations of the following types.
a. Move the contents of a register Rn to A
- MOV A,R2
- MOV A,R7
b. Move the contents of a register A to Rn
i. MOV R4,A
Ii. MOV R1,A
c. Move an immediate 8 bit data to register A or to Rn or to a memory location(direct or indirect)
i. MOV A, #45H
Ii. MOV R6, #51H i
iii.MOV 30H, #44H
iv. MOV @R0, #0E8H
v. MOV DPTR, #0F5A2H
vi. MOV DPTR, #5467H
d. Move the contents of a memory location to A or A to a memory location using direct and indirect addressing
i. MOV A, 65H
Ii. MOV A, @R0
Iii.MOV 45H, A
Iii. MOV @R1, A
e. Move the contents of a memory location to Rn or Rn to a memory location using direct addressing
i. MOV R3, 65H
Ii. MOV 45H, R2
f. Move the contents of memory location to another memory location using direct and indirect addressing
i. MOV 47H, 65H
Ii. MOV 45H, @R0
g. Move the contents of an external memory to A or A to an external memory
i. MOVX A,@R1
Ii. MOVX @R0,A
Iv. MOVX A,@DPTR iv. MOVX@DPTR,A
h. Move the contents of program memory to A
i. MOVC A, @A+PC
Ii. MOVC A, @A+DPTR.
Q8) Explain Arithmetic Instructions?
A8)
The 8051 can perform addition, subtraction. Multiplication and division operations on 8- bit numbers.
Addition
In this group, we have instructions to
i. Add the contents of A with immediate data with or without carry.
- ADD A, #45H
- ADDC A, #OB4H
Ii. Add the contents of A with register Rn with or without carry.
- ADD A, R5
- ADDC A, R2
Iii. Add the contents of A with contents of memory with or without carry using direct and indirect addressing
- ADD A, 51H
- ADDC A, 75H
- ADD A, @R1
- ADDC A, @R0 CY AC and OV flags will be affected by this operation.
Subtraction
In this group, we have instructions to
i. Subtract the contents of A with immediate data with or without carry.
i. SUBB A, #45H
Ii. SUBB A, #OB4H
Ii. Subtract the contents of A with register Rn with or without carry.
- SUBB A, R5
- SUBB A, R2
- Subtract the contents of A with contents of memory with or without carry using direct and indirect addressing
- SUBB A, 51H
- SUBB A, 75H
- SUBB A, @R1
- SUBB A, @R0 CY AC and OV flags will be affected by this operation.
Multiplication
MUL AB.
This instruction multiplies two 8 bit unsigned numbers which are stored in A and B register. After multiplication the lower byte of the result will be stored in accumulator and higher byte of result will be stored in B register.
Eg. MOV A,#45H ;[A]=45H
MOV B,#0F5H ;[B]=F5H
MUL AB ;[A] x [B] = 45 x F5 = 4209 ;[A]=09H, [B]=42H
Division
DIV AB.
This instruction divides the 8 bit unsigned number which is stored in A by the 8 bit unsigned number which is stored in B register. After division the result will be stored in accumulator and remainder will be stored in B register.
Eg. MOV A,#45H ;[A]=0E8H
MOV B,#0F5H ;[B]=1BH
DIV AB ;[A] / [B] = E8 /1B = 08 H with remainder 10H ;[A] = 08H, [B]=10H
DA A (Decimal Adjust After Addition).
When two BCD numbers are added, the answer is a non-BCD number. To get the result in BCD, we use DA A instruction after the addition.
DA A works as follows.
If lower nibble is greater than 9 or auxiliary carry is 1, 6 is added to lower nibble.
If upper nibble is greater than 9 or carry is 1, 6 is added to upper nibble.
Eg 1: MOV A,#23H
MOV R1,#55H
ADD A,R1 // [A]=78
DA A // [A]=78 no changes in the accumulator after da a
Eg 2: MOV A,#53H
MOV R1,#58H
ADD A,R1 // [A]=ABh
DA A // [A]=11, C=1 .
ANSWER IS 111. Accumulator data is changed after DA A
Q9) Write a short note on Rotate instructions?
A9)
RR A
This instruction is rotate right the accumulator.
Its operation is illustrated below.
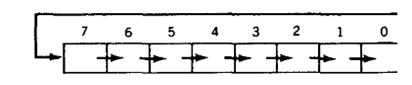
Each bit is shifted one location to the right, with bit 0 going to bit 7.
RL A Rotate left the accumulator.
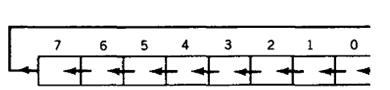
Each bit is shifted one location to the left, with bit 7 going to bit 0
RRC A Rotate right through the carry
Each bit is shifted one location to the right, with bit 0 going into the carry bit in the PSW, while the carry was at goes into bit 7
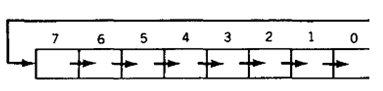
RLC A Rotate left through the carry.
Each bit is shifted one location to the left, with bit 7 going into the carry bit in the PSW, while the carry goes into bit 0.
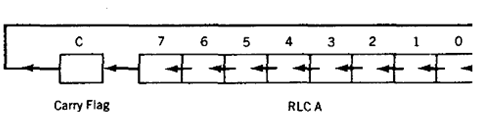
SWAP
The SWAP instruction can be thought of as a rotation of nibbles in the A register.
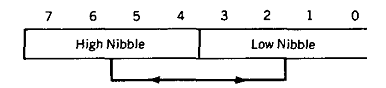
Example:
OY A,#OA5h | A = I0l00I0lb = A5h |
RR A | A = I I0I00I0b = D2h |
RR A | A = 0l I0l00lb = 69h |
RR A | A = I0I l0I00b = B4h |
RR A | A = 0I0l I0I0b = 5Ah |
SWAP A | A= I0I00I0lb = A5h |
CLR C | C = 0; A = I0I00I0lb = A5h |
RRC A | C = I; A= 0I0I00I0b = 52h |
RRC A | C = 0; A = l0I0J00lb = A9h |
RL A | A= 0I0I00llb = 53h |
RL A | A = I0I00l I0b = A6h |
SWAP A | C = 0; A = 0l 101010b = 6Ah |
RLC A | C = 0; A = 1 I0I0l00b = D4h |
RLC A | C = l; A= I0I0l000b = A8h |
SWAP A | C = l; A= l000I0I0b = 8Ah |
Q10) Write a program to add two 16 bit numbers stored at locations 51H-52H and 55H-56H and store the result in locations 40H, 41H and 42H. Assume that the least significant byte of data and the result is stored in low address and the most significant byte of data or the result is stored in high address.
A10)
ORG 0000H ; Set program counter 0000H
MOV A,51H ; Load the contents of memory location 51H into A
ADD A,55H ; Add the contents of 55H with contents of A
MOV 40H,A ; Save the LS byte of the result in location 40H
MOV A,52H ; Load the contents of 52H into A
ADDC A,56H ; Add the contents of 56H and CY flag with A
MOV 41H,A ; Save the second byte of the result in 41H
MOV A,#00 ; Load 00H into A
ADDC A,#00 ; Add the immediate data 00H and CY to A
MOV 42H,A ; Save the MS byte of the result in location 42H
END
Q11) Write a program to store data FFH into RAM memory locations 50H to 58H using direct addressing mode
A11)
ORG 0000H ; Set program counter 0000H
MOV A, #0FFH ; Load FFH into A
MOV 50H, A ; Store contents of A in location 50H
MOV 51H, A ; Store contents of A in location 5IH
MOV 52H, A ; Store contents of A in location 52H
MOV 53H, A ; Store contents of A in location 53H
MOV 54H, A ; Store contents of A in location 54H
MOV 55H, A ; Store contents of A in location 55H
MOV 56H, A ; Store contents of A in location 56H
MOV 57H, A ; Store contents of A in location 57H
MOV 58H, A ; Store contents of A in location 58H
END
Q12) Write a program to multiply two 8 bit numbers stored at locations 70H and 71H and store the result at memory locations 52H and 53H. Assume that the least significant byte of the result is stored in low address.
A12)
ORG 0000H ; Set program counter 00 OH
MOV A, 70H ; Load the contents of memory location 70h into A
MOV B, 71H ; Load the contents of memory location 71H into B
MUL AB ; Perform multiplication
MOV 52H,A ; Save the least significant byte of the result in location 52H
MOV 53H,B ;Save the most significant byte of the result in location 53H
END
Q13) Write a program to compute 1 + 2 + 3 + N (say N=15) and save the sum at70H
A13)
ORG 0000H ; Set program counter 0000H
N EQU 15
MOV R0,#00 ; Clear R0
CLR A ; Clear A
Again:INC R0 ; Increment R0
ADD A, R0 ; Add the contents of R0 with A
CJNE R0,#N,again ; Loop until counter, R0, N
MOV 70H,A ; Save the result in location 70H
END
Q14) Write a program to find the average of five 8 bit numbers. Store the result in 55H. (Assume that after adding five 8 bit numbers, the result is 8 bit only).
A14)
ORG 0000H
MOV 40H,#05H
MOV 41H,#55H
MOV 42H,#06H
MOV 43H,#1AH
MOV 44H,#09H
MOV R0,#40H
MOV R5,#05H
MOV B,R5
CLR A
Loop: ADD A,@RO
INC RO DJNZ R5,Loop
DIV AB
MOV 55H,A
END
Q15) Write a program to find the cube of an 8 bit number program is as follows
A15)
ORG 0000H
MOV R1,#N
MOV A,R1
MOV B,R1
MUL AB //SQUARE IS COMPUTED
MOV R2, B
MOV B, R1
MUL AB
MOV 50,A
MOV 51,B
MOV A,R2
MOV B, R1
MUL AB
ADD A, 51H
MOV 51H, A
MOV 52H, B
MOV A, # 00H
ADDC A, 52H
MOV 52H, A //CUBE IS STORED IN 52H,51H,50H
END
Q16) Write a program to count the number of and o's of 8 bit data stored in location 6000H.
A16)
ORG 00008 ; Set program counter 00008
MOV DPTR, #6000h ; Copy address 6000H to DPTR
MOVX A, @DPTR ; C o p y n u m b e r t o A
MOV R0,#08 ; C o py 0 8 i n R O
MOV R2,#00 ; C o p y 0 0 i n R 2
MOV R3,#00 ; C o p y 0 0 i n R 3
CLR C ; Clear carry flag
BACK: RLC A ; R o t a t e A t h r o u g h c a r r y f l a g
JC NEXT ; I f C F = 1 , b r a n c h t o n e x t
INC R2 ; I f C F = 0 , i n c r e m e n t R 2
AJMP NEXT2
NEXT: INC R3 ; I f C F = 1 , i n c r e m e n t R 3
NEXT2: DJNZ RO,BACK ; R e p e a t u n t i l R O i s z e r o
END
Q17) Explain Assemblers and Compilers.
A17)
Assembler
The assembly language is a low-level programming language which writes program code in terms of mnemonics. It can be used for direct hardware manipulations. It is used to write 8051 programming code efficiently with less number of clock cycles by consuming less memory compared to other high-level languages.
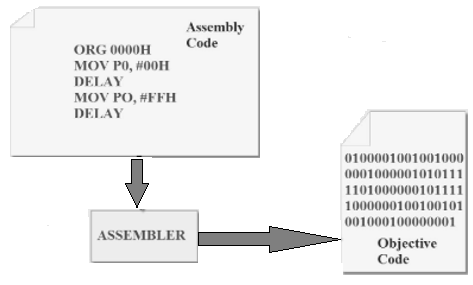
Fig. 8051 Programming
8051 Programming in Assembly Language
The assembly language is a completely hardware related programming language. It is essential that the embedded designers have sufficient knowledge on hardware of particular processor or controllers before writing the program. The assembly language is developed on mnemonics.
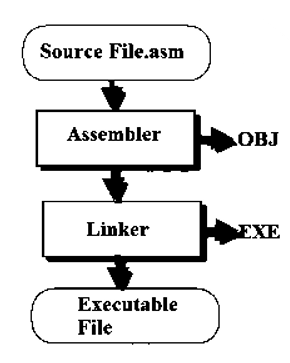
Fig. 8051 Programming in Assembly Language
- It is developed by various compilers and the “keiluvison” is best suitable for microcontroller programming development.
- Microcontrollers or processors can understand only binary language in the form of ‘0s or 1s’; An assembler converts the assembly language to binary language, and then stores in microcontroller memory to perform the specific task.
Compilers
- The name High-level language means the words and statements that are easily understood by humans.
- Few examples of High-level Languages are BASIC, C Pascal, C++ and Java. A program called Compiler will convert the Programs written in High-level languages to Machine Code.