Unit - 5
Function and Pointers
Q1) Explain built in user define function
A1)
User defined functions are the functions defined by the programmer or user.
C++ provides the facility to user to define their own functions.
When the function gets call anywhere from program the function body gets execute.
Structure
#include<iostream>
Void function_name(){
}
Int main(){
Function_name();
}
Example
#include<iostream>
Using namespace std;
Int add(int, int);
Int main(){
int a, b, sum;
cout<<"Enters two numbers ";
cin >> a >> b;
sum = add(a, b);
cout << "Sum = " << sum;
return 0;
}
Int add(int a, int b)
{
int add;
add = a + b;
return add; }
}
Q2) What is function definition and declaration?
A2)
Function is declared globally to inform the compiler about function name, function parameters and return type.
Return_type function_name([arguments type)];
Example
Void create_function(){
}
Function call
Function call has parameter list with function name. Using function call function can be called anywhere in program.
Function_name([actual arguments]);
Example
Void createfunction() {
Cout << "Function created";
}
Int main() {
Createfunction();
Return 0;
}
Function definition.
Function definition consists of block of statements which contains actual execution code. When function gets called in program control comes to the function definition.
Return_type function_name([arguments])
{
Statements;
}
Example
Int main() {
createfunction();
return 0;
}
Void createfunction() {
cout <<”Functoin created.”;
}
Void mycreateunction();
Int main() {
createfunction();
return 0;
}
Void createfunction() {
cout << “Function created”;
}
Q3) What is pass by value and pass by reference in function explain with examples?
A3)
Function arguments in c programming
Basically, there are two types of arguments:
- Actual arguments
- Formal arguments
The variables declared in the function prototype or definition are known as Formal arguments and the values that are passed to the called function from the main function are known as Actual arguments.
The actual arguments and formal arguments must match in number, type, and order.
Following are the two ways to pass arguments to the function:
- Pass by value
- Pass by reference
Pass by Value
Pass by value is a method in which a copy of the value of the variables is passed to the function for the specific operation.
In this method, the arguments in the function call are not modified by the change in parameters of the called function. So the original variables remain unchanged.
Example of passing arguments by value to function in C
// arguments pass by value
# include <stdio.h>
Int add (int a, int b)
{
return( a + b );
}
Int main()
{
int x, y, z;
x = 5;
y = 5;
z = add(x,y); // call by value
Return 0;
}
//end of program
In this program, function add() is called by passing the arguments x and y.
The copy of the values of x and y are passed to a and b respectively and then are used in the function.
So by changing the values of a and b, there will be no change in the actual arguments x and y in the function call.
Pass by reference
Pass by reference is a method in which rather than passing direct value the address of the variable is passed as an argument to the called function.
When we pass arguments by reference, the formal arguments in the called function becomes the assumed name or aliases of the actual arguments in the calling function. So the function works on the actual data.
Example of passing arguments by reference to function in C
// arguments pass by reference
#include <stdio.h>
Void swap (int *a, int *b) // a and b are reference variables
{
int temp;
temp = *a;
*a = *b;
*b = temp;
}
Int main()
{
int x = 2, y = 4;
printf("before swapping x = %d and y = %d\n", x, y);
swap(&x, &y); // call by reference
return 0;
} //end of program
In the above program, the formal arguments a and b becomes the alias of actual arguments x and y when the function was called.
So when the variables a and b are interchanged x and y are also interchanged. So the output becomes like this.
Output
Before swapping x = 2 and y = 4
After swapping x = 4 and y = 2
Now, if the function was defined as:
Void swap(int a, int b)
{
int temp;
temp = a;
a = b;
b = temp;
}
This is the pass by value method so here even if the values are swapped in the function the actual value won’t interchange and output would become like this:
Before swapping x = 2 and y = 4
After swapping x = 4 and y = 2
Q4) What is calling by value explain with examples?
A4)
Call by value in C
- In call by value method, the value of the actual parameters is copied into the formal parameters. In other words, we can say that the value of the variable is used in the function call in the call by value method.
- In call by value method, we can not modify the value of the actual parameter by the formal parameter.
- In call by value, different memory is allocated for actual and formal parameters since the value of the actual parameter is copied into the formal parameter.
- The actual parameter is the argument which is used in the function call whereas formal parameter is the argument which is used in the function definition.
Let's try to understand the concept of call by value in c language by the example given below:
- #include<stdio.h>
- Void change(int num) {
- Printf("Before adding value inside function num=%d \n",num);
- Num=num+100;
- Printf("After adding value inside function num=%d \n", num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(x);//passing value in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=100
Call by Value Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int , int); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(a,b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The value of actual parameters do not change by changing the formal parameters in call by value, a = 10, b = 20
- }
- Void swap (int a, int b)
- {
- Int temp;
- Temp = a;
- a=b;
- b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",a,b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
Q5) What is call by reference explain with examples?
A5)
Call by reference in C
- In call by reference, the address of the variable is passed into the function call as the actual parameter.
- The value of the actual parameters can be modified by changing the formal parameters since the address of the actual parameters is passed.
- In call by reference, the memory allocation is similar for both formal parameters and actual parameters. All the operations in the function are performed on the value stored at the address of the actual parameters, and the modified value gets stored at the same address.
Consider the following example for the call by reference.
- #include<stdio.h>
- Void change(int *num) {
- Printf("Before adding value inside function num=%d \n",*num);
- (*num) += 100;
- Printf("After adding value inside function num=%d \n", *num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(&x);//passing reference in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=200
Call by reference Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int *, int *); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(&a,&b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The values of actual parameters do change in call by reference, a = 10, b = 20
- }
- Void swap (int *a, int *b)
- {
- Int temp;
- Temp = *a;
- *a=*b;
- *b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",*a,*b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 20, b = 10
Q6) Explain pointer with example
A6)
The pointer in C language is a variable which stores the address of another variable. This variable can be of type int, char, array, function, or any other pointer. The size of the pointer depends on the architecture. However, in 32-bit architecture the size of a pointer is 2 byte.
Consider the following example to define a pointer which stores the address of an integer.
- Int n = 10;
- Int* p = &n; // Variable p of type pointer is pointing to the address of the variable n of type integer.
Declaring a pointer
The pointer in c language can be declared using * (asterisk symbol). It is also known as indirection pointer used to dereference a pointer.
- Int *a;//pointer to int
- Char *c;//pointer to char
Pointer Example
An example of using pointers to print the address and value is given below.
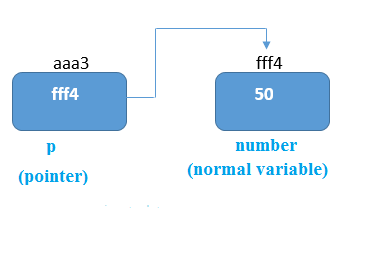
As you can see in the above figure, pointer variable stores the address of number variable, i.e., fff4. The value of number variable is 50. But the address of pointer variable p is aaa3.
By the help of * (indirection operator), we can print the value of pointer variable p.
Let's see the pointer example as explained for the above figure.
- #include<stdio.h>
- Int main(){
- Int number=50;
- Int *p;
- p=&number;//stores the address of number variable
- Printf("Address of p variable is %x \n",p); // p contains the address of the number therefore printing p gives the address of number.
- Printf("Value of p variable is %d \n",*p); // As we know that * is used to dereference a pointer therefore if we print *p, we will get the value stored at the address contained by p.
- Return 0;
- }
Output
Address of number variable is fff4
Address of p variable is fff4
Value of p variable is 50
Q7) Write Pointer Program to swap two numbers without using the 3rd variable
A7)
- #include<stdio.h>
- Int main(){
- Int a=10,b=20,*p1=&a,*p2=&b;
- Printf("Before swap: *p1=%d *p2=%d",*p1,*p2);
- *p1=*p1+*p2;
- *p2=*p1-*p2;
- *p1=*p1-*p2;
- Printf("\nAfter swap: *p1=%d *p2=%d",*p1,*p2);
- Return 0;
- }
Output
Before swap: *p1=10 *p2=20
After swap: *p1=20 *p2=10
Q8) How to read the pointer: int (*p)[10].
A8)
To read the pointer, we must see that () and [] have the equal precedence. Therefore, their associativity must be considered here. The associativity is left to right, so the priority goes to ().
Inside the bracket (), pointer operator * and pointer name (identifier) p have the same precedence. Therefore, their associativity must be considered here which is right to left, so the priority goes to p, and the second priority goes to *.
Assign the 3rd priority to [] since the data type has the last precedence. Therefore the pointer will look like following.
- Char -> 4
- * -> 2
- p -> 1
- [10] -> 3
The pointer will be read as p is a pointer to an array of integers of size 10.
Example
How to read the following pointer?
- Int (*p)(int (*)[2], int (*)void))
Explanation
This pointer will be read as p is a pointer to such function which accepts the first parameter as the pointer to a one-dimensional array of integers of size two and the second parameter as the pointer to a function which parameter is void and return type is the integer.
Q9) Explain declaration and initialization of pointer
A9)
Declaring a pointer
Like variables, pointers have to be declared before they can be used in your program. Pointers can be named anything you want as long as they obey C's naming rules. A pointer declaration has the following form.
Data_type * pointer_variable_name;
Here,
- Data_type is the pointer's base type of C's variable types and indicates the type of the variable that the pointer points to.
- The asterisk (*: the same asterisk used for multiplication) which is indirection operator, declares a pointer.
Let's see some valid pointer declarations
Int *ptr_thing; /* pointer to an integer */
Int *ptr1,thing;/* ptr1 is a pointer to type integer and thing is an integer variable */
Double *ptr2; /* pointer to a double */
Float *ptr3; /* pointer to a float */
Char *ch1 ; /* pointer to a character */
Float *ptr, variable;/*ptr is a pointer to type float and variable is an ordinary float variable */
Initialize a pointer
After declaring a pointer, we initialize it like standard variables with a variable address. If pointers are not uninitialized and used in the program, the results are unpredictable and potentially disastrous.
To get the address of a variable, we use the ampersand (&)operator, placed before the name of a variable whose address we need. Pointer initialization is done with the following syntax.
Pointer = &variable;
A simple program for pointer illustration is given below:
#include <stdio.h>
Int main()
{
int a=10; //variable declaration
int *p; //pointer variable declaration
p=&a; //store address of variable a in pointer p
printf("Address stored in a variable p is:%x\n",p); //accessing the address
printf("Value stored in a variable p is:%d\n",*p); //accessing the value
return 0;
}
Output:
Address stored in a variable p is:60ff08
Value stored in a variable p is:10
Q10) What are different types of a pointer?
A10)
Null pointer
We can create a null pointer by assigning null value during the pointer declaration. This method is useful when you do not have any address assigned to the pointer. A null pointer always contains value 0.
Following program illustrates the use of a null pointer:
#include <stdio.h>
Int main()
{
Int *p = NULL; //null pointer
Printf(“The value inside variable p is:\n%x”,p);
Return 0;
}
Output:
The value inside variable p is:
0
Void Pointer
In C programming, a void pointer is also called as a generic pointer. It does not have any standard data type. A void pointer is created by using the keyword void. It can be used to store an address of any variable.
Following program illustrates the use of a void pointer:
#include <stdio.h>
Int main()
{
Void *p = NULL; //void pointer
Printf("The size of pointer is:%d\n",sizeof(p));
Return 0;
}
Output:
The size of pointer is:4
Wild pointer
A pointer is said to be a wild pointer if it is not being initialized to anything. These types of pointers are not efficient because they may point to some unknown memory location which may cause problems in our program and it may lead to crashing of the program. One should always be careful while working with wild pointers.
Following program illustrates the use of wild pointer:
#include <stdio.h>
Int main()
{
Int *p; //wild pointer
Printf("\n%d",*p);
Return 0;
}
Output
Timeout: the monitored command dumped core
Sh: line 1: 95298 Segmentation fault timeout 10s main
Other types of pointers in 'c' are as follows:
- Dangling pointer
- Complex pointer
- Near pointer
- Far pointer
- Huge pointer
Unit - 5
Function and Pointers
Q1) Explain built in user define function
A1)
User defined functions are the functions defined by the programmer or user.
C++ provides the facility to user to define their own functions.
When the function gets call anywhere from program the function body gets execute.
Structure
#include<iostream>
Void function_name(){
}
Int main(){
Function_name();
}
Example
#include<iostream>
Using namespace std;
Int add(int, int);
Int main(){
int a, b, sum;
cout<<"Enters two numbers ";
cin >> a >> b;
sum = add(a, b);
cout << "Sum = " << sum;
return 0;
}
Int add(int a, int b)
{
int add;
add = a + b;
return add; }
}
Q2) What is function definition and declaration?
A2)
Function is declared globally to inform the compiler about function name, function parameters and return type.
Return_type function_name([arguments type)];
Example
Void create_function(){
}
Function call
Function call has parameter list with function name. Using function call function can be called anywhere in program.
Function_name([actual arguments]);
Example
Void createfunction() {
Cout << "Function created";
}
Int main() {
Createfunction();
Return 0;
}
Function definition.
Function definition consists of block of statements which contains actual execution code. When function gets called in program control comes to the function definition.
Return_type function_name([arguments])
{
Statements;
}
Example
Int main() {
createfunction();
return 0;
}
Void createfunction() {
cout <<”Functoin created.”;
}
Void mycreateunction();
Int main() {
createfunction();
return 0;
}
Void createfunction() {
cout << “Function created”;
}
Q3) What is pass by value and pass by reference in function explain with examples?
A3)
Function arguments in c programming
Basically, there are two types of arguments:
- Actual arguments
- Formal arguments
The variables declared in the function prototype or definition are known as Formal arguments and the values that are passed to the called function from the main function are known as Actual arguments.
The actual arguments and formal arguments must match in number, type, and order.
Following are the two ways to pass arguments to the function:
- Pass by value
- Pass by reference
Pass by Value
Pass by value is a method in which a copy of the value of the variables is passed to the function for the specific operation.
In this method, the arguments in the function call are not modified by the change in parameters of the called function. So the original variables remain unchanged.
Example of passing arguments by value to function in C
// arguments pass by value
# include <stdio.h>
Int add (int a, int b)
{
return( a + b );
}
Int main()
{
int x, y, z;
x = 5;
y = 5;
z = add(x,y); // call by value
Return 0;
}
//end of program
In this program, function add() is called by passing the arguments x and y.
The copy of the values of x and y are passed to a and b respectively and then are used in the function.
So by changing the values of a and b, there will be no change in the actual arguments x and y in the function call.
Pass by reference
Pass by reference is a method in which rather than passing direct value the address of the variable is passed as an argument to the called function.
When we pass arguments by reference, the formal arguments in the called function becomes the assumed name or aliases of the actual arguments in the calling function. So the function works on the actual data.
Example of passing arguments by reference to function in C
// arguments pass by reference
#include <stdio.h>
Void swap (int *a, int *b) // a and b are reference variables
{
int temp;
temp = *a;
*a = *b;
*b = temp;
}
Int main()
{
int x = 2, y = 4;
printf("before swapping x = %d and y = %d\n", x, y);
swap(&x, &y); // call by reference
return 0;
} //end of program
In the above program, the formal arguments a and b becomes the alias of actual arguments x and y when the function was called.
So when the variables a and b are interchanged x and y are also interchanged. So the output becomes like this.
Output
Before swapping x = 2 and y = 4
After swapping x = 4 and y = 2
Now, if the function was defined as:
Void swap(int a, int b)
{
int temp;
temp = a;
a = b;
b = temp;
}
This is the pass by value method so here even if the values are swapped in the function the actual value won’t interchange and output would become like this:
Before swapping x = 2 and y = 4
After swapping x = 4 and y = 2
Q4) What is calling by value explain with examples?
A4)
Call by value in C
- In call by value method, the value of the actual parameters is copied into the formal parameters. In other words, we can say that the value of the variable is used in the function call in the call by value method.
- In call by value method, we can not modify the value of the actual parameter by the formal parameter.
- In call by value, different memory is allocated for actual and formal parameters since the value of the actual parameter is copied into the formal parameter.
- The actual parameter is the argument which is used in the function call whereas formal parameter is the argument which is used in the function definition.
Let's try to understand the concept of call by value in c language by the example given below:
- #include<stdio.h>
- Void change(int num) {
- Printf("Before adding value inside function num=%d \n",num);
- Num=num+100;
- Printf("After adding value inside function num=%d \n", num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(x);//passing value in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=100
Call by Value Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int , int); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(a,b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The value of actual parameters do not change by changing the formal parameters in call by value, a = 10, b = 20
- }
- Void swap (int a, int b)
- {
- Int temp;
- Temp = a;
- a=b;
- b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",a,b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
Q5) What is call by reference explain with examples?
A5)
Call by reference in C
- In call by reference, the address of the variable is passed into the function call as the actual parameter.
- The value of the actual parameters can be modified by changing the formal parameters since the address of the actual parameters is passed.
- In call by reference, the memory allocation is similar for both formal parameters and actual parameters. All the operations in the function are performed on the value stored at the address of the actual parameters, and the modified value gets stored at the same address.
Consider the following example for the call by reference.
- #include<stdio.h>
- Void change(int *num) {
- Printf("Before adding value inside function num=%d \n",*num);
- (*num) += 100;
- Printf("After adding value inside function num=%d \n", *num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(&x);//passing reference in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=200
Call by reference Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int *, int *); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(&a,&b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The values of actual parameters do change in call by reference, a = 10, b = 20
- }
- Void swap (int *a, int *b)
- {
- Int temp;
- Temp = *a;
- *a=*b;
- *b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",*a,*b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 20, b = 10
Q6) Explain pointer with example
A6)
The pointer in C language is a variable which stores the address of another variable. This variable can be of type int, char, array, function, or any other pointer. The size of the pointer depends on the architecture. However, in 32-bit architecture the size of a pointer is 2 byte.
Consider the following example to define a pointer which stores the address of an integer.
- Int n = 10;
- Int* p = &n; // Variable p of type pointer is pointing to the address of the variable n of type integer.
Declaring a pointer
The pointer in c language can be declared using * (asterisk symbol). It is also known as indirection pointer used to dereference a pointer.
- Int *a;//pointer to int
- Char *c;//pointer to char
Pointer Example
An example of using pointers to print the address and value is given below.
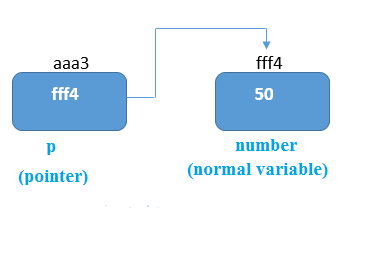
As you can see in the above figure, pointer variable stores the address of number variable, i.e., fff4. The value of number variable is 50. But the address of pointer variable p is aaa3.
By the help of * (indirection operator), we can print the value of pointer variable p.
Let's see the pointer example as explained for the above figure.
- #include<stdio.h>
- Int main(){
- Int number=50;
- Int *p;
- p=&number;//stores the address of number variable
- Printf("Address of p variable is %x \n",p); // p contains the address of the number therefore printing p gives the address of number.
- Printf("Value of p variable is %d \n",*p); // As we know that * is used to dereference a pointer therefore if we print *p, we will get the value stored at the address contained by p.
- Return 0;
- }
Output
Address of number variable is fff4
Address of p variable is fff4
Value of p variable is 50
Q7) Write Pointer Program to swap two numbers without using the 3rd variable
A7)
- #include<stdio.h>
- Int main(){
- Int a=10,b=20,*p1=&a,*p2=&b;
- Printf("Before swap: *p1=%d *p2=%d",*p1,*p2);
- *p1=*p1+*p2;
- *p2=*p1-*p2;
- *p1=*p1-*p2;
- Printf("\nAfter swap: *p1=%d *p2=%d",*p1,*p2);
- Return 0;
- }
Output
Before swap: *p1=10 *p2=20
After swap: *p1=20 *p2=10
Q8) How to read the pointer: int (*p)[10].
A8)
To read the pointer, we must see that () and [] have the equal precedence. Therefore, their associativity must be considered here. The associativity is left to right, so the priority goes to ().
Inside the bracket (), pointer operator * and pointer name (identifier) p have the same precedence. Therefore, their associativity must be considered here which is right to left, so the priority goes to p, and the second priority goes to *.
Assign the 3rd priority to [] since the data type has the last precedence. Therefore the pointer will look like following.
- Char -> 4
- * -> 2
- p -> 1
- [10] -> 3
The pointer will be read as p is a pointer to an array of integers of size 10.
Example
How to read the following pointer?
- Int (*p)(int (*)[2], int (*)void))
Explanation
This pointer will be read as p is a pointer to such function which accepts the first parameter as the pointer to a one-dimensional array of integers of size two and the second parameter as the pointer to a function which parameter is void and return type is the integer.
Q9) Explain declaration and initialization of pointer
A9)
Declaring a pointer
Like variables, pointers have to be declared before they can be used in your program. Pointers can be named anything you want as long as they obey C's naming rules. A pointer declaration has the following form.
Data_type * pointer_variable_name;
Here,
- Data_type is the pointer's base type of C's variable types and indicates the type of the variable that the pointer points to.
- The asterisk (*: the same asterisk used for multiplication) which is indirection operator, declares a pointer.
Let's see some valid pointer declarations
Int *ptr_thing; /* pointer to an integer */
Int *ptr1,thing;/* ptr1 is a pointer to type integer and thing is an integer variable */
Double *ptr2; /* pointer to a double */
Float *ptr3; /* pointer to a float */
Char *ch1 ; /* pointer to a character */
Float *ptr, variable;/*ptr is a pointer to type float and variable is an ordinary float variable */
Initialize a pointer
After declaring a pointer, we initialize it like standard variables with a variable address. If pointers are not uninitialized and used in the program, the results are unpredictable and potentially disastrous.
To get the address of a variable, we use the ampersand (&)operator, placed before the name of a variable whose address we need. Pointer initialization is done with the following syntax.
Pointer = &variable;
A simple program for pointer illustration is given below:
#include <stdio.h>
Int main()
{
int a=10; //variable declaration
int *p; //pointer variable declaration
p=&a; //store address of variable a in pointer p
printf("Address stored in a variable p is:%x\n",p); //accessing the address
printf("Value stored in a variable p is:%d\n",*p); //accessing the value
return 0;
}
Output:
Address stored in a variable p is:60ff08
Value stored in a variable p is:10
Q10) What are different types of a pointer?
A10)
Null pointer
We can create a null pointer by assigning null value during the pointer declaration. This method is useful when you do not have any address assigned to the pointer. A null pointer always contains value 0.
Following program illustrates the use of a null pointer:
#include <stdio.h>
Int main()
{
Int *p = NULL; //null pointer
Printf(“The value inside variable p is:\n%x”,p);
Return 0;
}
Output:
The value inside variable p is:
0
Void Pointer
In C programming, a void pointer is also called as a generic pointer. It does not have any standard data type. A void pointer is created by using the keyword void. It can be used to store an address of any variable.
Following program illustrates the use of a void pointer:
#include <stdio.h>
Int main()
{
Void *p = NULL; //void pointer
Printf("The size of pointer is:%d\n",sizeof(p));
Return 0;
}
Output:
The size of pointer is:4
Wild pointer
A pointer is said to be a wild pointer if it is not being initialized to anything. These types of pointers are not efficient because they may point to some unknown memory location which may cause problems in our program and it may lead to crashing of the program. One should always be careful while working with wild pointers.
Following program illustrates the use of wild pointer:
#include <stdio.h>
Int main()
{
Int *p; //wild pointer
Printf("\n%d",*p);
Return 0;
}
Output
Timeout: the monitored command dumped core
Sh: line 1: 95298 Segmentation fault timeout 10s main
Other types of pointers in 'c' are as follows:
- Dangling pointer
- Complex pointer
- Near pointer
- Far pointer
- Huge pointer