UNIT 2
Control Structures, Arrays
- Explain arithmetic expression in detail with example
An arithmetic expression contains only arithmetic operators and operands. We know that the arithmetic operators in C language include unary operators (+ – ++ — ), multiplicative operators (* / %) and additive operators (+ – ). The arithmetic operands include integral operands (various int and char types) and floating-type operands (float, double and long double).
Example Simple arithmetic expressions
a) Several valid arithmetic expressions are given below. Assume that variables a and b are of type int.
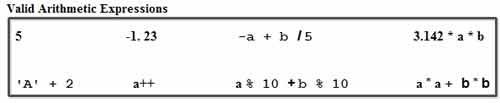
Observe that the operators are used correctly. The unary operators in expressions -1.23, a++ and -a + b /5 correctly operate on a single operand. The unary minus (-) is used as a prefix operator whereas the increment operator (++) is used in the postfix form. All other operators (multiplicative and additive) are used as infix operators and they operate on two operands. Also, the modulo-arithmetic(s) operator operates on integer operands as required. The expression ‘A’ + 2 is valid and its value is equal to 67, as the ASCII value of character ‘A’ is 65. Note that the operands and operators need not be separated by spaces. For example, the last two expressions in the first row can also be written as -a+b/5 and 3 .142*a*b. However, the spaces are often used to improve readability.
b) The expressions given below are invalid
The first two expressions are invalid because the operands a and b are not connected by any operator. This is true even for the third expression (a++ –b) as the++ and — operators operate on operands a and b, respectively, but there is no operator connecting these operands.
The last two expressions are invalid as they contain two binary operators in succession. These operators operate on a single operand instead of two. Note that the – operator in last expression cannot be interpreted as a unary operator as it does not precede an operand.
c) Can you tell which of the following expressions are valid?

Surprisingly, all are valid C expressions although they do not appear to be so. In expression +a +b, the first+ operator is a unary operator that operates on variable a, whereas the second+ is a binary operator that connects variables a and b.
Now observe the space between the two’-‘ symbols in the second expression. They cannot
Be interpreted as a decrement operator. As a result, the second – symbol is interpreted as a unary minus operator, whereas the first – is interpreted as a subtraction operator that operates on two operands a and -b. ·
The two consecutive operators in expression a % 10 I – b does not pose a problem as the –
Operator is interpreted as a unary operator and the I operator operates on two operands (a % 10 and -b). However, the space between – and b is misleading.
In the last expression, observe the spaces separating the middle + symbol from the increment operators that operate on variables a and b. The middle + is interpreted as a binary operator that operates on a and b.
d) Several simple mathematical equations are given below along with equivalent C expressions.
Assume that the symbolic constant PI has been defined as
#define PI 3.1415927
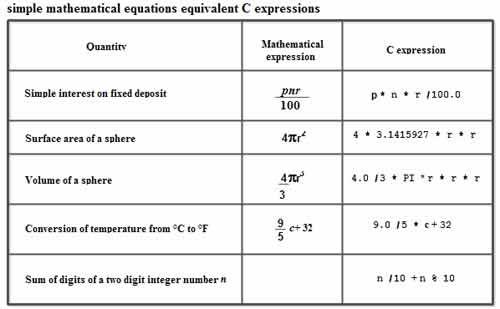
Observe that the literal 4 in expression 3 is written as floating constant 4.0 to avoid the integer division of 4 and 3. Similarly, constant 9 in expression 4 is also written as a floating constant. The sum of digits of a two-digit integer number is written as n I 10 + n % 10.Since n is assumed to be an integer variable, the expression n I 1o performs integer division and determines the digit at the 10’s place, whereas the expression n % 10 determines the digit at the unit’s place.
2. What is the Evaluation of Simple Arithmetic Expressions?
We use the operator precedence and associativity rules to determine the meaning and value of an expression in an unambiguous manner. Recall that the operators in an expression are bound to their operands in the order of their precedence. If the expression contains more than one operator at the same precedence level, they are associated with their operands using the associativity rules. Table summarizes these rules for arithmetic and assignment operators.
If the given expression is simple, we can often directly convert it to its mathematical form and evaluate it. However, if the given expression is complex, i. e., it contains several operators at different precedence levels, we need a systematic approach to convert it to a mathematical equation and evaluate it. The steps to convert a given valid C expression to its mathematical form (if possible) and to evaluate it are as follows:
1. First determine the order in which the operators are bound to their operands by applying the precedence and associativity rules. Note that after an operator is bound to its operand(s), that sub-expression is considered as a single operand for the adjacent operators.
2. Obtain the equivalent mathematical equation for given C expression (if possible) by following the operator binding sequence (obtained in step 1).
3. Determine the value of the given expression by evaluating operators in the binding sequence.
The steps to determine operator binding in an arithmetic expression are explained below with the help of the expression -a+ b * c – d I e + f.
1. The unary operators (unary +, unary – , ++ and – -) have the highest precedence and right-to-left associativity. Thus, the given expression is first scanned from right to left and unary operators, if any, are bound to their operands. The order is indicated below the expression as follows:
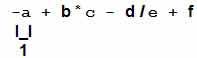
2. The multiplicative operators (*, I and %) have the next highest precedence and left to- right associativity. Thus, the expression is scanned from left-to-right and the multiplicative operators, if any, are bound to their operands as shown below. (Observe that after completion of the above step, sub-expressions -a, b * c and d I e will be operands for the remaining operator bindings.)
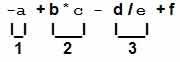
3. The additive operators (+ and -) have the next highest precedence and left-to-right associativity. Hence, the expression is scanned from left-to-tight and the additive operators, if any, are bound to their operands as shown below. Observe that the operands for the first + operator are the sub-expressions -a and b * c. Similarly, the operands for the – operator are -a+ b * c and d /e.
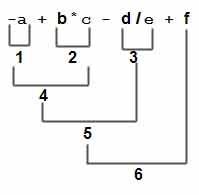
Now we can write the mathematical equation for the given C expression by following the operator binding sequence as shown below:
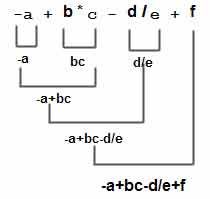
Now the given expression can be evaluated, again by following the operator binding sequence, as shown below. Assume that the variables a, b, c, ct, e and f are of type float and are initialized with values as a= 1. 5, b = 2. 0, c = 3. 5, ct= 5.0, e = 2. 5 and f = 1. 25.
Remember that except for some operators (& & || ? : and the comma operator), the C language does not specify the order of evaluation of sub-expressions. Thus, the sub expressions at the same level can be evaluated in any order. For example, the sub expressions -a, b * c and ct I e in the above expression can be evaluated in any order.
The procedure explained above can also be used to check the validity of an expression. The given expression is valid if we arrive at a single operand (or value) after all the operators in the given expression are considered. Consider a more complex arithmetic expression: -a–+ -b++ * –c. This expression appears to be invalid due to the excessive use of operators. It contains three operands a, b and c and seven operators, five of which are unary (-, ++ and –) and the other two are binary operators (+ and *). However, using the operator binding steps, we can easily verify that it is a valid expression:
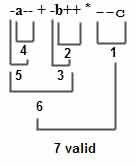
3. Explain operator precedence in detail with examples
Operator precedence determines which operator is performed first in an expression with more than one operators with different precedence.
For example: Solve
10 + 20 * 30
10 + 20 * 30 is calculated as 10 + (20 * 30)
And not as (10 + 20) * 30
Operators Associativity is used when two operators of same precedence appear in an expression. Associativity can be either Left to Right or Right to Left.
For example: ‘*’ and ‘/’ have same precedence and their associativity is Left to Right, so the expression “100 / 10 * 10” is treated as “(100 / 10) * 10”.
Operators Precedence and Associativity are two characteristics of operators that determine the evaluation order of sub-expressions in absence of brackets
For example: Solve
100 + 200 / 10 - 3 * 10
1) Associativity is only used when there are two or more operators of same precedence.
The point to note is associativity doesn’t define the order in which operands of a single operator are evaluated. For example, consider the following program, associativity of the + operator is left to right, but it doesn’t mean f1() is always called before f2(). The output of the following program is in-fact compiler dependent.
// Associativity is not used in the below program. // Output is compiler dependent.
#include <stdio.h>
Int x = 0; Int f1() { x = 5; Return x; } Int f2() { x = 10; Return x; } Int main() { Int p = f1() + f2(); Printf("%d ", x); Return 0; } |
2) All operators with the same precedence have same associativity
This is necessary, otherwise, there won’t be any way for the compiler to decide evaluation order of expressions which have two operators of same precedence and different associativity. For example + and – have the same associativity.
3) Precedence and associativity of postfix ++ and prefix ++ are different
Precedence of postfix ++ is more than prefix ++, their associativity is also different. Associativity of postfix ++ is left to right and associativity of prefix ++ is right to left.
4) Comma has the least precedence among all operators and should be used carefully For example consider the following program, the output is 1.
#include <stdio.h> Int main() { Int a; a = 1, 2, 3; // Evaluated as (a = 1), 2, 3 Printf("%d", a); Return 0; } |
5) There is no chaining of comparison operators in C
In Python, expression like “c > b > a” is treated as “c > b and b > a”, but this type of chaining doesn’t happen in C. For example consider the following program. The output of following program is “FALSE”.
#include <stdio.h> Int main() { Int a = 10, b = 20, c = 30;
// (c > b > a) is treated as ((c > b) > a), associativity of '>' // is left to right. Therefore the value becomes ((30 > 20) > 10) // which becomes (1 > 20) If (c > b > a) Printf("TRUE"); Else Printf("FALSE"); Return 0; } |
4. What is If else Statement explain with examples?
The if-else statement in C is used to perform the operations based on some specific condition. The operations specified in if block are executed if and only if the given condition is true.
There are the following variants of if statement in C language.
- If statement
- If-else statement
- If else-if ladder
- Nested if
If Statement
The if statement is used to check some given condition and perform some operations depending upon the correctness of that condition. It is mostly used in the scenario where we need to perform the different operations for the different conditions. The syntax of the if statement is given below.
- If(expression){
- //code to be executed
- }
Flowchart of if statement in C
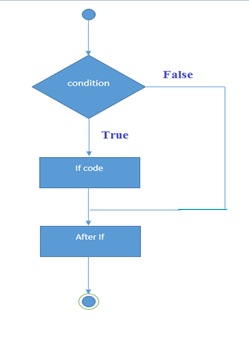
Let's see a simple example of C language if statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("Enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
Program to find the largest number of the three.
- #include <stdio.h>
- Int main()
- {
- Int a, b, c;
- Printf("Enter three numbers?");
- Scanf("%d %d %d",&a,&b,&c);
- If(a>b && a>c)
- {
- Printf("%d is largest",a);
- }
- If(b>a && b > c)
- {
- Printf("%d is largest",b);
- }
- If(c>a && c>b)
- {
- Printf("%d is largest",c);
- }
- If(a == b && a == c)
- {
- Printf("All are equal");
- }
- }
Output
Enter three numbers?
12 23 34
34 is largest
If-else Statement
The if-else statement is used to perform two operations for a single condition. The if-else statement is an extension to the if statement using which, we can perform two different operations, i.e., one is for the correctness of that condition, and the other is for the incorrectness of the condition. Here, we must notice that if and else block cannot be executed simiulteneously. Using if-else statement is always preferable since it always invokes an otherwise case with every if condition. The syntax of the if-else statement is given below.
- If(expression){
- //code to be executed if condition is true
- }else{
- //code to be executed if condition is false
- }
Flowchart of the if-else statement in C
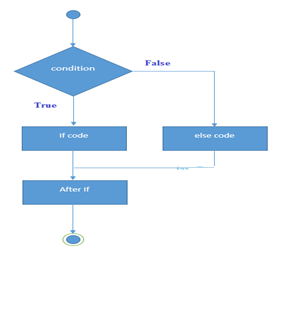
Let's see the simple example to check whether a number is even or odd using if-else statement in C language.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Else{
- Printf("%d is odd number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
5 is odd number
Program to check whether a person is eligible to vote or not.
- #include <stdio.h>
- Int main()
- {
- Int age;
- Printf("Enter your age?");
- Scanf("%d",&age);
- If(age>=18)
- {
- Printf("You are eligible to vote...");
- }
- Else
- {
- Printf("Sorry ... you can't vote");
- }
- }
Output
Enter your age?18
You are eligible to vote...
Enter your age?13
Sorry ... You can't vote
5. What is If else-if ladder Statement explain with examples?
The if-else-if ladder statement is an extension to the if-else statement. It is used in the scenario where there are multiple cases to be performed for different conditions. In if-else-if ladder statement, if a condition is true then the statements defined in the if block will be executed, otherwise if some other condition is true then the statements defined in the else-if block will be executed, at the last if none of the condition is true then the statements defined in the else block will be executed. There are multiple else-if blocks possible. It is similar to the switch case statement where the default is executed instead of else block if none of the cases is matched.
- If(condition1){
- //code to be executed if condition1 is true
- }else if(condition2){
- //code to be executed if condition2 is true
- }
- Else if(condition3){
- //code to be executed if condition3 is true
- }
- ...
- Else{
- //code to be executed if all the conditions are false
- }
Flowchart of else-if ladder statement in C
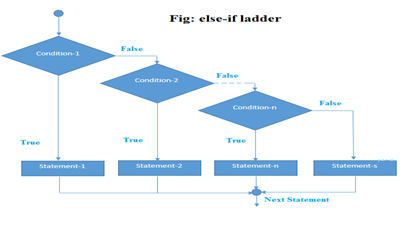
The example of an if-else-if statement in C language is given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number==10){
- Printf("number is equals to 10");
- }
- Else if(number==50){
- Printf("number is equal to 50");
- }
- Else if(number==100){
- Printf("number is equal to 100");
- }
- Else{
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Program to calculate the grade of the student according to the specified marks.
- #include <stdio.h>
- Int main()
- {
- Int marks;
- Printf("Enter your marks?");
- Scanf("%d",&marks);
- If(marks > 85 && marks <= 100)
- {
- Printf("Congrats ! you scored grade A ...");
- }
- Else if (marks > 60 && marks <= 85)
- {
- Printf("You scored grade B + ...");
- }
- Else if (marks > 40 && marks <= 60)
- {
- Printf("You scored grade B ...");
- }
- Else if (marks > 30 && marks <= 40)
- {
- Printf("You scored grade C ...");
- }
- Else
- {
- Printf("Sorry you are fail ...");
- }
- }
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats ! you scored grade A ...
6. What is Switch Statement explain?
The switch statement in C is an alternate to if-else-if ladder statement which allows us to execute multiple operations for the different possibles values of a single variable called switch variable. Here, We can define various statements in the multiple cases for the different values of a single variable.
The syntax of switch statement is given below:
- Switch(expression){
- Case value1:
- //code to be executed;
- Break; //optional
- Case value2:
- //code to be executed;
- Break; //optional
- ......
- Default:
- Code to be executed if all cases are not matched;
- }
Rules for switch statement in C language
1) The switch expression must be of an integer or character type.
2) The case value must be an integer or character constant.
3) The case value can be used only inside the switch statement.
4) The break statement in switch case is not must. It is optional. If there is no break statement found in the case, all the cases will be executed present after the matched case. It is known as fall through the state of C switch statement.
Let's try to understand it by the examples. We are assuming that there are following variables.
- Int x,y,z;
- Char a,b;
- Float f;
Valid Switch | Invalid Switch | Valid Case | Invalid Case |
Switch(x) | Switch(f) | Case 3; | Case 2.5; |
Switch(x>y) | Switch(x+2.5) | Case 'a'; | Case x; |
Switch(a+b-2) |
| Case 1+2; | Case x+2; |
Switch(func(x,y)) |
| Case 'x'>'y'; | Case 1,2,3; |
Flowchart of switch statement in C
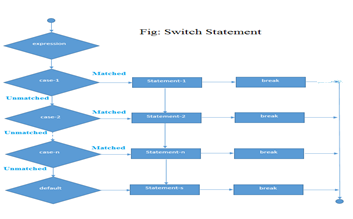
Functioning of switch case statement
First, the integer expression specified in the switch statement is evaluated. This value is then matched one by one with the constant values given in the different cases. If a match is found, then all the statements specified in that case are executed along with the all the cases present after that case including the default statement. No two cases can have similar values. If the matched case contains a break statement, then all the cases present after that will be skipped, and the control comes out of the switch. Otherwise, all the cases following the matched case will be executed.
Let's see a simple example of c language switch statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equals to 10");
- Break;
- Case 50:
- Printf("number is equal to 50");
- Break;
- Case 100:
- Printf("number is equal to 100");
- Break;
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Switch case example 2
- #include <stdio.h>
- Int main()
- {
- Int x = 10, y = 5;
- Switch(x>y && x+y>0)
- {
- Case 1:
- Printf("hi");
- Break;
- Case 0:
- Printf("bye");
- Break;
- Default:
- Printf(" Hello bye ");
- }
- }
Output
Hi
7. What is Switch statement is fall-through?
In C language, the switch statement is fall through; it means if you don't use a break statement in the switch case, all the cases after the matching case will be executed.
Let's try to understand the fall through state of switch statement by the example given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equal to 10\n");
- Case 50:
- Printf("number is equal to 50\n");
- Case 100:
- Printf("number is equal to 100\n");
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:10
Number is equal to 10
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Output
Enter a number:50
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Nested switch case statement
We can use as many switch statement as we want inside a switch statement. Such type of statements is called nested switch case statements. Consider the following example.
- #include <stdio.h>
- Int main () {
- Int i = 10;
- Int j = 20;
- Switch(i) {
- Case 10:
- Printf("the value of i evaluated in outer switch: %d\n",i);
- Case 20:
- Switch(j) {
- Case 20:
- Printf("The value of j evaluated in nested switch: %d\n",j);
- }
- }
- Printf("Exact value of i is : %d\n", i );
- Printf("Exact value of j is : %d\n", j );
- Return 0;
- }
Output
The value of i evaluated in outer switch: 10
The value of j evaluated in nested switch: 20
Exact value of i is : 10
Exact value of j is : 20
8. Explain If-else vs switch in detail
What is an if-else statement?
An if-else statement in C programming is a conditional statement that executes a different set of statements based on the condition that is true or false. The 'if' block will be executed only when the specified condition is true, and if the specified condition is false, then the else block will be executed.
Syntax of if-else statement is given below:
- If(expression)
- {
- // statements;
- }
- Else
- {
- // statements;
- }
What is a switch statement?
A switch statement is a conditional statement used in C programming to check the value of a variable and compare it with all the cases. If the value is matched with any case, then its corresponding statements will be executed. Each case has some name or number known as the identifier. The value entered by the user will be compared with all the cases until the case is found. If the value entered by the user is not matched with any case, then the default statement will be executed.
Syntax of the switch statement is given below:
- Switch(expression)
- {
- Case constant 1:
- // statements;
- Break;
- Case constant 2:
- // statements;
- Break;
- Case constant n:
- // statements;
- Break;
- Default:
- // statements;
- }
Similarity b/w if-else and switch
Both the if-else and switch are the decision-making statements. Here, decision-making statements mean that the output of the expression will decide which statements are to be executed.
Differences b/w if-else and switch statement
The following are the differences between if-else and switch statement are:
- Definition
If-else
Based on the result of the expression in the 'if-else' statement, the block of statements will be executed. If the condition is true, then the 'if' block will be executed otherwise 'else' block will execute.
Switch statement
The switch statement contains multiple cases or choices. The user will decide the case, which is to execute.
- Expression
If-else
It can contain a single expression or multiple expressions for multiple choices. In this, an expression is evaluated based on the range of values or conditions. It checks both equality and logical expressions.
Switch
It contains only a single expression, and this expression is either a single integer object or a string object. It checks only equality expression.
- Evaluation
If-else
An if-else statement can evaluate almost all the types of data such as integer, floating-point, character, pointer, or Boolean.
Switch
A switch statement can evaluate either an integer or a character.
- Sequence of Execution
If-else
In the case of 'if-else' statement, either the 'if' block or the 'else' block will be executed based on the condition.
Switch
In the case of the 'switch' statement, one case after another will be executed until the break keyword is not found, or the default statement is executed.
- Default Execution
If-else
If the condition is not true within the 'if' statement, then by default, the else block statements will be executed.
Switch
If the expression specified within the switch statement is not matched with any of the cases, then the default statement, if defined, will be executed.
- Values
If-else
Values are based on the condition specified inside the 'if' statement. The value will decide either the 'if' or 'else' block is to be executed.
Switch
In this case, value is decided by the user. Based on the choice of the user, the case will be executed.
- Use
If-else
It evaluates a condition to be true or false.
Switch
A switch statement compares the value of the variable with multiple cases. If the value is matched with any of the cases, then the block of statements associated with this case will be executed.
- Editing
If-else
Editing in 'if-else' statement is not easy as if we remove the 'else' statement, then it will create the havoc.
Switch
Editing in switch statement is easier as compared to the 'if-else' statement. If we remove any of the cases from the switch, then it will not interrupt the execution of other cases. Therefore, we can say that the switch statement is easy to modify and maintain.
- Speed
If-else
If the choices are multiple, then the speed of the execution of 'if-else' statements is slow.
Switch
The case constants in the switch statement create a jump table at the compile time. This jump table chooses the path of the execution based on the value of the expression. If we have a multiple choice, then the execution of the switch statement will be much faster than the equivalent logic of 'if-else' statement.
Let's summarize the above differences in a tabular form.
| If-else | Switch |
Definition | Depending on the condition in the 'if' statement, 'if' and 'else' blocks are executed. | The user will decide which statement is to be executed. |
Expression | It contains either logical or equality expression. | It contains a single expression which can be either a character or integer variable. |
Evaluation | It evaluates all types of data, such as integer, floating-point, character or Boolean. | It evaluates either an integer, or character. |
Sequence of execution | First, the condition is checked. If the condition is true then 'if' block is executed otherwise 'else' block | It executes one case after another till the break keyword is not found, or the default statement is executed. |
Default execution | If the condition is not true, then by default, else block will be executed. | If the value does not match with any case, then by default, default statement is executed. |
Editing | Editing is not easy in the 'if-else' statement. | Cases in a switch statement are easy to maintain and modify. Therefore, we can say that the removal or editing of any case will not interrupt the execution of other cases. |
Speed | If there are multiple choices implemented through 'if-else', then the speed of the execution will be slow. | If we have multiple choices then the switch statement is the best option as the speed of the execution will be much higher than 'if-else'. |
9. Explain do while loop with examples
Do while loop
The do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.
Do while loop syntax
The syntax of the C language do-while loop is given below:
- Do{
- //code to be executed
- }while(condition);
Example 1
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Char c;
- Int choice,dummy;
- Do{
- Printf("\n1. Print Hello\n2. Print Javatpoint\n3. Exit\n");
- Scanf("%d",&choice);
- Switch(choice)
- {
- Case 1 :
- Printf("Hello");
- Break;
- Case 2:
- Printf("Javatpoint");
- Break;
- Case 3:
- Exit(0);
- Break;
- Default:
- Printf("please enter valid choice");
- }
- Printf("do you want to enter more?");
- Scanf("%d",&dummy);
- Scanf("%c",&c);
- }while(c=='y');
- }
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
Do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
Do you want to enter more?
n
Flowchart of do while loop
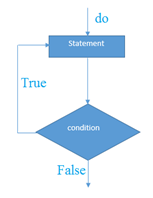
do while example
There is given the simple program of c language do while loop where we are printing the table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- Do{
- Printf("%d \n",i);
- i++;
- }while(i<=10);
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using do while loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- Do{
- Printf("%d \n",(number*i));
- i++;
- }while(i<=10);
- Return 0;
- }
Output
Enter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Infinitive do while loop
The do-while loop will run infinite times if we pass any non-zero value as the conditional expression.
- Do{
- //statement
- }while(1);
10. Explain for loop in detail with examples
For loop
The for loop in C language is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like the array and linked list.
Syntax of for loop in C
The syntax of for loop in c language is given below:
- For(Expression 1; Expression 2; Expression 3){
- //code to be executed
- }
Flowchart of for loop in C
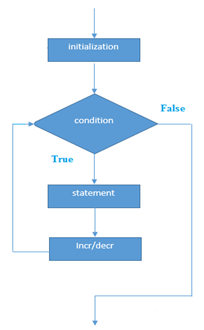
C for loop Examples
Let's see the simple program of for loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=0;
- For(i=1;i<=10;i++){
- Printf("%d \n",i);
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- For(i=1;i<=10;i++){
- Printf("%d \n",(number*i));
- }
- Return 0;
- }
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
Properties of Expression 1
- The expression represents the initialization of the loop variable.
- We can initialize more than one variable in Expression 1.
- Expression 1 is optional.
- In C, we can not declare the variables in Expression 1. However, It can be an exception in some compilers.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int a,b,c;
- For(a=0,b=12,c=23;a<2;a++)
- {
- Printf("%d ",a+b+c);
- }
- }
Output
35 36
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- For(;i<5;i++)
- {
- Printf("%d ",i);
- }
- }
Output
1 2 3 4
Properties of Expression 2
- Expression 2 is a conditional expression. It checks for a specific condition to be satisfied. If it is not, the loop is terminated.
- Expression 2 can have more than one condition. However, the loop will iterate until the last condition becomes false. Other conditions will be treated as statements.
- Expression 2 is optional.
- Expression 2 can perform the task of expression 1 and expression 3. That is, we can initialize the variable as well as update the loop variable in expression 2 itself.
- We can pass zero or non-zero value in expression 2. However, in C, any non-zero value is true, and zero is false by default.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;i<=4;i++)
- {
- Printf("%d ",i);
- }
- }
Output
0 1 2 3 4
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i,j,k;
- For(i=0,j=0,k=0;i<4,k<8,j<10;i++)
- {
- Printf("%d %d %d\n",i,j,k);
- j+=2;
- k+=3;
- }
- }
Output
0 0 0
1 2 3
2 4 6
3 6 9
4 8 12
Example 3
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;;i++)
- {
- Printf("%d",i);
- }
- }
Output
Infinite loop
Properties of Expression 3
- Expression 3 is used to update the loop variable.
- We can update more than one variable at the same time.
- Expression 3 is optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int i=0,j=2;
- For(i = 0;i<5;i++,j=j+2)
- {
- Printf("%d %d\n",i,j);
- }
- }
Output
0 2
1 4
2 6
3 8
4 10
Loop body
The braces {} are used to define the scope of the loop. However, if the loop contains only one statement, then we don't need to use braces. A loop without a body is possible. The braces work as a block separator, i.e., the value variable declared inside for loop is valid only for that block and not outside. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Int i;
- For(i=0;i<10;i++)
- {
- Int i = 20;
- Printf("%d ",i);
- }
- }
Output
20 20 20 20 20 20 20 20 20 20
Infinitive for loop in C
To make a for loop infinite, we need not give any expression in the syntax. Instead of that, we need to provide two semicolons to validate the syntax of the for loop. This will work as an infinite for loop.
- #include<stdio.h>
- Void main ()
- {
- For(;;)
- {
- Printf("welcome to javatpoint");
- }
- }
If you run this program, you will see above statement infinite times.