Unit - 1
Introduction to C++
Q1) Give a brief Introduction to procedural, modular, generic and object-oriented programming techniques
A1)
Procedure Oriented Programming
- Procedure Oriented Programming divides program into small procedures.
- A procedure is a set of instructions to perform specific independent task.
- A problem is viewed as a sequence of tasks and a separate procedure is written to perform that task.
- So the main emphasis is on procedure and data is given no/ little importance.
- Mostly data is global data which is shared by functions/procedures.
- It employs top down approach.
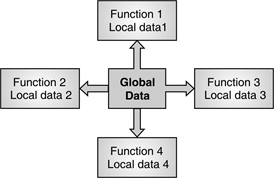
Fig.: Structure of procedure oriented programming
- Fig.shows how global data is accessed by all functions in a program while local data can be accessed by only that specific function.
Modular oriented programming
- Modular programming divides program into distinct independent units called as modules.
- Each module is a separate software component that is programmed and tested independently.
- Modular programming leads to effective development and maintenance of large programs.
Generic oriented programming
- Usually codes or programs are tied to specific types. If we are writing program for stack it is written for a specific data type like stack of integer values.
- If we want to create stack of characters, then we need to rewrite code taking ‘char’ as a data type.
- This rewriting same code for a specific data type can be avoided by generic programming technique.
- Generic programs create software components that can be used for any data type.
- Thus we will write generic program for stack which will work on any data type.
- In C++, class and templates are generic programming components.
Object Oriented Programming
- Program is divided into number of objects.
- Objects are considered as real life entities that contain data and functions.
- Data is given more importance than functions.
- Data is not accessible to functions of other classes and hence data security is ensured.
- It employs bottom up approach.
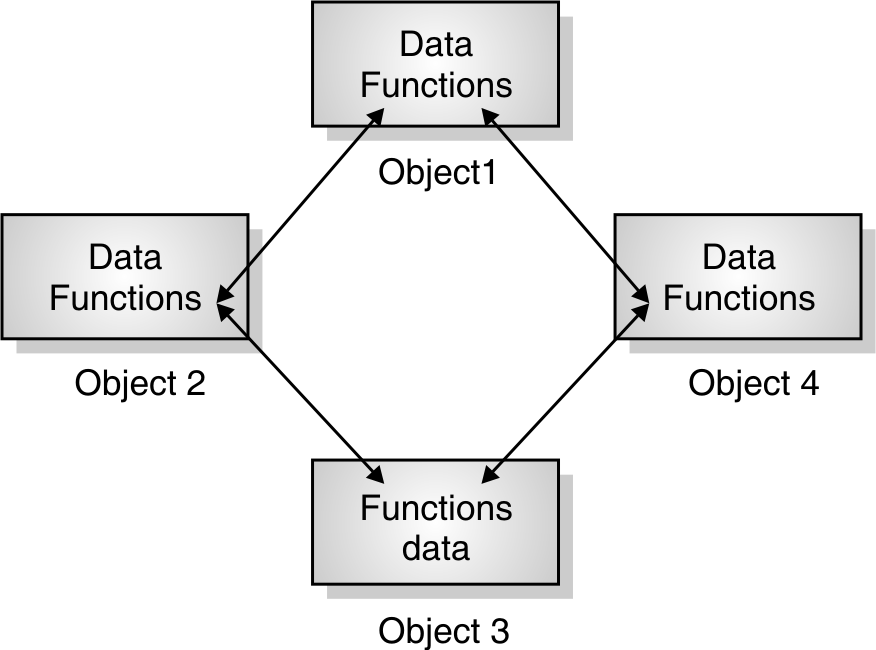
Fig.: Structure of Object Oriented Programming
- Fig. Shows how objects can communicate with other objects with the help of functions. Here data is not accessible to external functions which ensure data security.
Q2) What are the limitations of procedural programming
A2)
- Large procedural based programs may turn into spaghetti code.
- It does not model real world problems very well.
- Well, although procedural-oriented programs are extremely powerful, they do have some limitations.
- Perhaps the most serious limitation is the tendency for large procedural-based programs to turn into "spaghetti-code".
- Spaghetti code is code that has been modified so many times that the logical flow shown in the figures above becomes so convoluted that any new programmer coming onto the project needs a two month prep-course in order to even begin to understand the software innards.
- Why does this happen?
- Well, in reality, a programmer's job has just begun when she finishes writing version 1.0 of her software application. Before she knows it, she'll be bombarded with dozens of modification requests and bug reports as users actually get to batter and bruise her poor piece of code.
- In order to meet the demands of the evil user, the programmer is forced to modify the code. This can mean introducing new sub loops, new eddies of flow control and new methods, libraries and variables altogether.
- Unfortunately, there are no great tools for abstraction and modularization in procedural languages...thus, it is hard to add new functionality or change the work flow without going back and modifying all other parts of the program.
- Now, instead of redesigning the workflow and starting from scratch, most programmers, under intense time restrictions will introduce hacks to fix the code.
- This gets us to the second problem with procedural-based programming. Not only does procedural code have a tendency to be difficult to understand, as it evolves, it becomes even harder to understand, and thus, harder to modify.
- Since everything is tied to everything else, nothing is independent. If you change one bit of code in a procedural-based program, it is likely that you will break three other pieces in some other section that might be stored in some remote library file you'd forgotten all about.
- A final problem with spaghetification, is that the code you write today will not help you write the code you have to write tomorrow. Procedural-based code has a tenacious ability to resist being cut and pasted from one application to another. Thus, procedural programmers often find themselves reinventing the wheel on every new project.
Q3) What are the OOP Paradigms?
A3)
- The major motivating factor in the invention of object-oriented approch is to remove some of the flaws encountered in the procedural approch.
- OOP treats data as a critical element in the program development and does not allow it to flow freely around the systems.
- It ties data more closely to the functions that operate on it, and protects it from accidental modification from outside functions.
- OOP allows decomposition of a problem into a number of entities called objects and then builds data and functions around these objects.
- The data of an object can be accessed only by the function associated with that object.
- However, functions of one object can access the the functions of other objects.
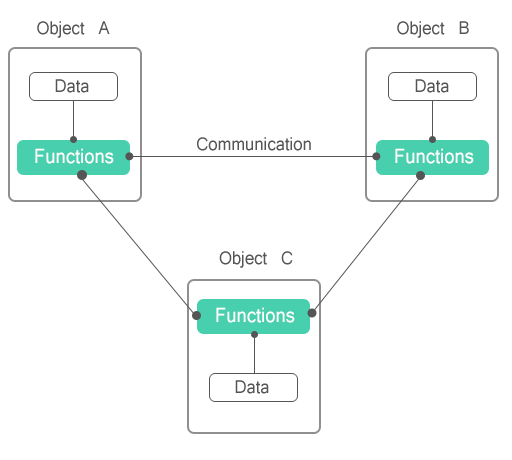
Some of the striking features of object-oriented programming are
- Emphasis is on data rather than procedure.
- Programs are divided into what are known as objects.
- Data structures are designed such that they characterize the objects.
- Data is hidden and cannot be accessed by external functions.
- Objects may communicate with each other through functions.
- New data and functions can be easily added whenever necessary.
- Follows bottom-up approach in program design.
Q4) Write a program to accept and display details of book.
A4)
#include<iostream>//Step 1 Header file
Using namespace std ;
Class Book //Step 2 Declares class Book
{
Char name[10];//Declares data members name, pages and price.
Int pages; These data members are private.
Float price;
Public://Starts public section of class book
Void accept()//Step 3 Defines function “accept”
{//Entering the details of book
Cout<<“Enter name of book”;
Cin>>name;
Cout<<“Enter pages of book”;
Cin>>pages;
Cout<<“Enter price of book”;
Cin>>price;
}//End of accept function definition
Void display()//Step 4 Defines function display
{// Displays all the details of book
Cout<<“Name of book is :”<<name;
Cout<<“No. Of Pages in book are :”<<pages;
Cout<<“Price of book is:”<<price;
} // End of display function
};//Step 5 End of class
Int main()//Step 6 Start of main() function
{
Book b1,b2; //Step 7 Creates objects b1,b2
b1.accept();//Step 8 Calls accept function for b1
b2.accept();// Calls accept function for b2
b1.display();//Step 9 Calls display function for b1
b2.display();//Calls display function for b2
Return 0;
}// End of main
Explanation
Step 1 :Every C++ program starts with header file “iostream.h” as it contains declarations of input and output streams in program.
#include<iostream>
Using namespace std ;
Step 2 :Class “Book” is declared and defined. It consists of data members name, pages and price. Access specifier is not mentioned for these members so they are considered as private.
Class Book //Declares class Book
{
Char name[10];//Declares data members name, pages and price. These int pages; data members are private.
Float price;
Step 3:Accept function accepts all the details of book. It shows new features cout, <<, cin, >>.
Cout
Standard predefined object that represents standard output stream in C++.
Q5) Give a brief Introduction to Class
A5)
- Class is used to create user defined data type.
- C++ provides various built in types like float, int and char.
- Similarly, if user wants to create his own data type then he can create it with the help of class.
Class Book
- Here user defined data type “Book” is created with the help of class keyword class.
- Class consists of data members (variables) and associated member functions.
Structure of a class
Class |
Data Members |
Member functions |
- Data members (variables) represent attributes and member functions represent behaviour of class.
Structure of a “Book” class
Book |
Data Members Name Pages Price |
Member functions Accept() Display() |
- Class only provides template for object and does not create any memory space for object. Hence class is logical abstract entity.
Structure of class declaration
Class class_name
{
Access specifier:
Data Members;
Access specifier:
Member Functions;
};
We will discuss new terms in above declaration.
Class
- Classes are created using keyword “class”.
- The body of class is enclosed within curly braces and terminated by semicolon.
- Class contains data members and Member functions. They are called as class members.
Access Specifier
It specifies if class members are accessible to external functions (functions of other classes).
C++ provides three access specifiers :
1.private
- Private members are accessible to functions of same class.
- They are not accessible to functions of other classes.
- By default members of class are private.
- Private members are specified by keyword “private”.
2.public
- Public members are accessible to functions of other classes.
- Public members are specified by keyword “public”.
3.protected
- It is used only when inheritance is involved.
- Protected members of super class are accessible to its immediate sub class.
- Protected members are specified by keyword “protected”.
Data members
- Data members are variables declared inside the class. They are declared as follows
Data _type Variable_name;
e.g. Int roll_no;
float price;
String name;
- They are declared under any of the access specifier viz. Private, public and protected.
Methods
Functions declared or defined within a class are known as member functions of that class. Member functions are also called as methods.
Table: Let us create a class Book
Class Definition | Explanation | |
Class Book
| Class “Book” is created | |
{ | Start of class definition | |
Char name[10]; | Declares data member “name” of the type char. | Here access specifier is not mentioned so all these data members are private as default access specifier is private. |
Int pages; | Declares data member “page” of the type int | |
Float price; | Declares data member “price” of the type float | |
Public: | Starts public section of a class | |
Void accept(); | Declares member function accept | |
Void display(); | Declares member function display | |
}; | End of class definition |
Q6) Explain messages in detail with example
A6)
Programming languages like Smalltalk and Objective-C are considered more flexible than C++ because they support dynamic message passing. C++ does not support dynamic message passing; it only supports static message passing: when a method of an object is invoked, the target object must have the invoked method; otherwise, the compiler outputs an error.
Although the way C++ does message passing is much faster than the way Smalltalk or Objective-C does it, sometimes the flexibility of Smalltalk or Objective-C is required. This little article shows how it is possible to achieve dynamic message passing in C++ with minimum code.
Example
The supplied header contains an implementation of dynamic message passing. In order to add dynamic message passing capabilities to an object, the following things must be done:
- Declare a global function which acts as a signature of the message.
- Inherit from dmp::object.
- In the class constructor, add one or more messages to the object using the method add_message.
- Send a message to an object using the function invoke(signature, ...parameters).
Here is an example:
Hide Copy Code
//the dynamic-message-passing header
#include "dmp.hpp"
//the message signature
Std::string my_message(int a, double b) { return ""; }
//a class that accepts messages dynamically
Class test : public dmp::object {
Public:
test() {
add_message(&::my_message, &test::my_message);
}
std::string my_message(int a, double b);
};
Int main() {
test t;
std::string s = t.invoke(&::my_message, 10, 3.14);
}
How it Works
Each object contains a shared ptr to a map. The map's key is the pointer to the signature of the message. The map's value is a pointer to an internal message structure which holds a pointer to the method to invoke.
When a method is invoked, the appropriate message structure is retrieved from the map, and the method of the object stored in the message structure is invoked, using this as the target object.
The map used internally is unordered, for efficiency reasons.
The message map of objects is shared between objects for efficiency reasons as well. When a message map is modified, it is duplicated if it is not unique, i.e., the copy-on-write pattern is applied.
The code is thread safe only when the message maps are not modified while used for look-up by different threads. If a message map is modified, then no thread must send messages to an object at the same time.
The code uses the boost library because of:
- Unordered maps; the class boost::unordered_map is used.
- The pre-processor; used for automatically constructing code for different number of parameters.
- Shared ptrs; used for internal memory management.
Q7) Give a short description on data encapsulation , data abstraction and information hiding , inheritance and polymorphism
A7)
Data encapsulation
- It encapsulates data and methods into object.
- Data wrapped in an object is not accessible to functions outside the class.
- Functions of same class can access data and hence this feature ensures data security.
Data abstraction and information hiding
- It hides detailed implementation of an object from user.
- It completely ignores low level details of how things works internally and represents only essential feature to hide complexity from user.
- For example, we know how to withdraw money from ATM but are unaware of internal processing that happens in ATM during withdrawal. This internal processing is hidden to avoid complexity.
Inheritance
- It is a mechanism of extending functionality from one class to another.
- Class can reuse the code of another class if they are tied together by inheritance.
- The original class is called as base/super/parent class and inherited class is called derived/sub/child class.
- Sub class inherits some or all the features of base class.
- Sub class adds its own features to base class so that it has combined features of both the classes.
- In this way it supports the concept of reusability.
- It can very well model hierarchical classification as shown below.
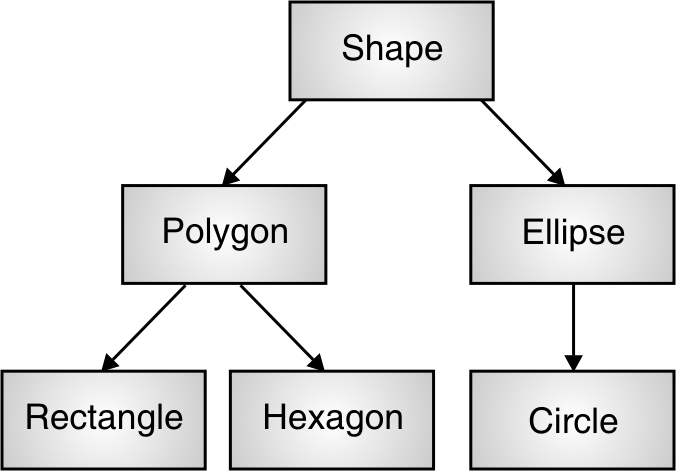
Fig. : Inheritance
- Here Shape is considered as base class.
- Polygon and Ellipse are subclasses of class Shape.
- Rectangle and Hexagon are subclasses of Polygon so they inherit features of Polygon class.
- Similarly, Circle inherits features of Ellipse class.
Polymorphism
- It is ability to take more than one form depending upon the data types used in program.
- Consider the operation of finding area of any geometrical figure shown in
Fig. 3.1.4. - The operation “Area” is same but will take different forms depending upon type and number of arguments passed to “Area”.
- “Area” of square calculates area of square whereas “Area” of rectangle calculates area of rectangle.
- Though operation “Area” is same but it takes different forms depending upon type of geometrical figure.
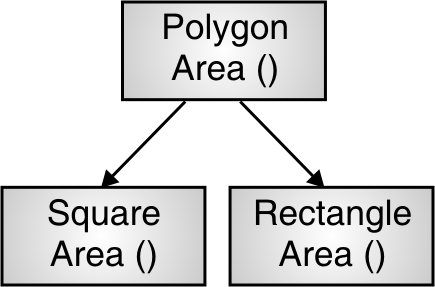
Fig. : Polymorphism
Q8) What are identifiers and keywords?
A8)
C++ Identifiers
A C++ identifier is a name used to identify a variable, function, class, module, or any other user-defined item. An identifier starts with a letter A to Z or a to z or an underscore (_) followed by zero or more letters, underscores, and digits (0 to 9).
C++ does not allow punctuation characters such as @, $, and % within identifiers. C++ is a case-sensitive programming language. Thus, Manpower and manpower are two different identifiers in C++.
Here are some examples of acceptable identifiers −
Mohd zara abc move_name a_123
Myname50 _temp j a23b9 retVal
C++ Keywords
The following list shows the reserved words in C++. These reserved words may not be used as constant or variable or any other identifier names.
Asm | Else | New | This |
Auto | Enum | Operator | Throw |
Bool | Explicit | Private | True |
Break | Export | Protected | Try |
Case | Extern | Public | Typedef |
Catch | False | Register | Typeid |
Char | Float | Reinterpret_cast | Typename |
Class | For | Return | Union |
Const | Friend | Short | Unsigned |
Const_cast | Goto | Signed | Using |
Continue | If | Sizeof | Virtual |
Default | Inline | Static | Void |
Delete | Int | Static_cast | Volatile |
Do | Long | Struct | Wchar_t |
Double | Mutable | Switch | While |
Dynamic_cast | Namespace | Template |
|
Q9) What is the fundamentals data type of C++?
A9)
The table below shows the fundamental data types, their meaning, and their sizes (in bytes):
Data TypeMeaningSize (in Bytes)
IntInteger2 or 4
FloatFloating-point4
DoubleDouble Floating-point8
CharCharacter1
Wchar_tWide Character2
BoolBoolean1
VoidEmpty0
1. Int
The int keyword is used to indicate integers.
Its size is usually 4 bytes. Meaning, it can store values from -2147483648 to 214748647.
For example,
Int salary = 85000;
2. float and double
Float and double are used to store floating-point numbers (decimals and exponentials).
The size of float is 4 bytes and the size of double is 8 bytes. Hence, double has two times the precision of float. To learn more, visit C++ float and double.
For example,
Float area = 64.74;
Double volume = 134.64534;
As mentioned above, these two data types are also used for exponentials. For example,
Double distance = 45E12 // 45E12 is equal to 45*10^12
3. Char
Keyword char is used for characters.
Its size is 1 byte.
Characters in C++ are enclosed inside single quotes ' '.
For example,
Char test = 'h';
Note: In C++, an integer value is stored in a char variable rather than the character itself. To learn more, visit C++ characters.
4. wchar_t
Wide character wchar_t is similar to the char data type, except its size is 2 bytes instead of 1.
It is used to represent characters that require more memory to represent them than a single char.
For example,
Wchar_t test = L'ם' // storing Hebrew character;
Q10) Explain structure in detail with examples and difference of structure and class
A10)
C++ Structs
In C++, classes and structs are blueprints that are used to create the instance of a class. Structs are used for lightweight objects such as Rectangle, color, Point, etc.
Unlike class, structs in C++ are value type than reference type. It is useful if you have data that is not intended to be modified after creation of struct.
C++ Structure is a collection of different data types. It is similar to the class that holds different types of data.
The Syntax Of Structure
- Struct structure_name
- {
- // member declarations.
- }
In the above declaration, a structure is declared by preceding the struct keyword followed by the identifier(structure name). Inside the curly braces, we can declare the member variables of different types. Consider the following situation:
- Struct Student
- {
- Char name[20];
- Int id;
- Int age;
- }
In the above case, Student is a structure contains three variables name, id, and age. When the structure is declared, no memory is allocated. When the variable of a structure is created, then the memory is allocated. Let's understand this scenario.
How to create the instance of Structure?
Structure variable can be defined as:
Student s;
Here, s is a structure variable of type Student. When the structure variable is created, the memory will be allocated. Student structure contains one char variable and two integer variable. Therefore, the memory for one char variable is 1 byte and two ints will be 2*4 = 8. The total memory occupied by the s variable is 9 byte.
How to access the variable of Structure:
The variable of the structure can be accessed by simply using the instance of the structure followed by the dot (.) operator and then the field of the structure.
For example:
- s.id = 4;
In the above statement, we are accessing the id field of the structure Student by using the dot(.) operator and assigns the value 4 to the id field.
C++ Struct Example
Let's see a simple example of struct Rectangle which has two data members width and height.
- #include <iostream>
- Using namespace std;
- Struct Rectangle
- {
- Int width, height;
- };
- Int main(void) {
- Struct Rectangle rec;
- Rec.width=8;
- Rec.height=5;
- Cout<<"Area of Rectangle is: "<<(rec.width * rec.height)<<endl;
- Return 0;
- }
Output:
Area of Rectangle is: 40
C++ Struct Example: Using Constructor and Method
Let's see another example of struct where we are using the constructor to initialize data and method to calculate the area of rectangle.
- #include <iostream>
- Using namespace std;
- Struct Rectangle {
- Int width, height;
- Rectangle(int w, int h)
- {
- Width = w;
- Height = h;
- }
- Void areaOfRectangle() {
- Cout<<"Area of Rectangle is: "<<(width*height); }
- };
- Int main(void) {
- Struct Rectangle rec=Rectangle(4,6);
- Rec.areaOfRectangle();
- Return 0;
- }
Output:
Area of Rectangle is: 24
Structure v/s Class
Structure | Class |
If access specifier is not declared explicitly, then by default access specifier will be public. | If access specifier is not declared explicitly, then by default access specifier will be private. |
Syntax of Structure: | Syntax of Class: |
The instance of the structure is known as "Structure variable". | The instance of the class is known as "Object of the class". |