Unit - 2
Control Structures and Functions
Q1) Explain C if else Statement with some examples
A1)
If-else Statement
The if-else statement is used to perform two operations for a single condition. The if-else statement is an extension to the if statement using which, we can perform two different operations, i.e., one is for the correctness of that condition, and the other is for the incorrectness of the condition. Here, we must notice that if and else block cannot be executed simiulteneously. Using if-else statement is always preferable since it always invokes an otherwise case with every if condition. The syntax of the if-else statement is given below.
- If(expression){
- //code to be executed if condition is true
- }else{
- //code to be executed if condition is false
- }
Flowchart of the if-else statement in C
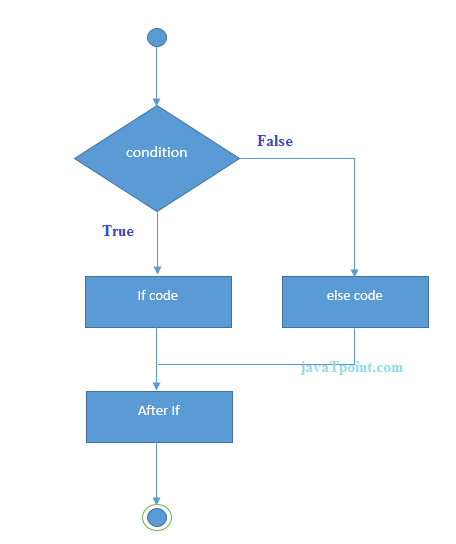
Let's see the simple example to check whether a number is even or odd using if-else statement in C language.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Else{
- Printf("%d is odd number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
5 is odd number
Program to check whether a person is eligible to vote or not.
- #include <stdio.h>
- Int main()
- {
- Int age;
- Printf("Enter your age?");
- Scanf("%d",&age);
- If(age>=18)
- {
- Printf("You are eligible to vote...");
- }
- Else
- {
- Printf("Sorry ... you can't vote");
- }
- }
Output
Enter your age?18
You are eligible to vote...
Enter your age?13
Sorry ... You can't vote
Q2) Explain If-Else-If ladder statement with some examples
A2)
The if-else-if ladder statement is an extension to the if-else statement. It is used in the scenario where there are multiple cases to be performed for different conditions. In if-else-if ladder statement, if a condition is true then the statements defined in the if block will be executed, otherwise if some other condition is true then the statements defined in the else-if block will be executed, at the last if none of the condition is true then the statements defined in the else block will be executed. There are multiple else-if blocks possible. It is similar to the switch case statement where the default is executed instead of else block if none of the cases is matched.
- If(condition1){
- //code to be executed if condition1 is true
- }else if(condition2){
- //code to be executed if condition2 is true
- }
- Else if(condition3){
- //code to be executed if condition3 is true
- }
- ...
- Else{
- //code to be executed if all the conditions are false
- }
Flowchart of else-if ladder statement in C
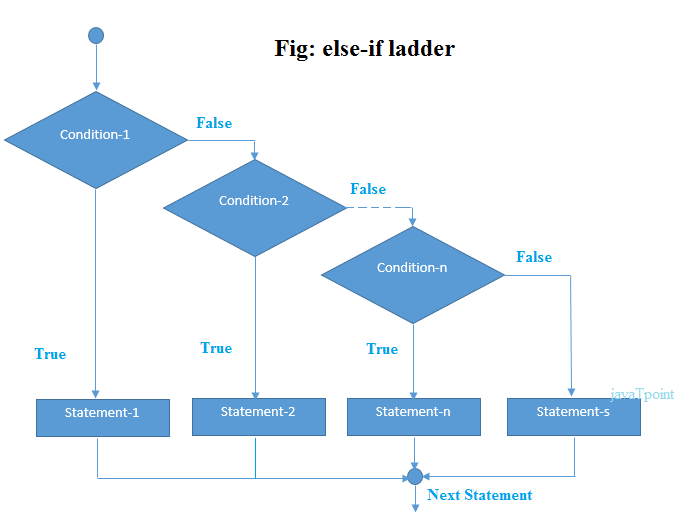
The example of an if-else-if statement in C language is given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number==10){
- Printf("number is equals to 10");
- }
- Else if(number==50){
- Printf("number is equal to 50");
- }
- Else if(number==100){
- Printf("number is equal to 100");
- }
- Else{
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Program to calculate the grade of the student according to the specified marks.
- #include <stdio.h>
- Int main()
- {
- Int marks;
- Printf("Enter your marks?");
- Scanf("%d",&marks);
- If(marks > 85 && marks <= 100)
- {
- Printf("Congrats ! you scored grade A ...");
- }
- Else if (marks > 60 && marks <= 85)
- {
- Printf("You scored grade B + ...");
- }
- Else if (marks > 40 && marks <= 60)
- {
- Printf("You scored grade B ...");
- }
- Else if (marks > 30 && marks <= 40)
- {
- Printf("You scored grade C ...");
- }
- Else
- {
- Printf("Sorry you are fail ...");
- }
- }
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats ! you scored grade A ...
Q3) Explain switch statement with some examples
A3)
The switch statement in C is an alternate to if-else-if ladder statement which allows us to execute multiple operations for the different possibles values of a single variable called switch variable. Here, We can define various statements in the multiple cases for the different values of a single variable.
The syntax of switch statement in c language is given below:
- Switch(expression){
- Case value1:
- //code to be executed;
- Break; //optional
- Case value2:
- //code to be executed;
- Break; //optional
- ......
- Default:
- Code to be executed if all cases are not matched;
- }
Rules for switch statement in C language
1) The switch expression must be of an integer or character type.
2) The case value must be an integer or character constant.
3) The case value can be used only inside the switch statement.
4) The break statement in switch case is not must. It is optional. If there is no break statement found in the case, all the cases will be executed present after the matched case. It is known as fall through the state of C switch statement.
Let's try to understand it by the examples. We are assuming that there are following variables.
- Int x,y,z;
- Char a,b;
- Float f;
Valid Switch | Invalid Switch | Valid Case | Invalid Case |
Switch(x) | Switch(f) | Case 3; | Case 2.5; |
Switch(x>y) | Switch(x+2.5) | Case 'a'; | Case x; |
Switch(a+b-2) |
| Case 1+2; | Case x+2; |
Switch(func(x,y)) |
| Case 'x'>'y'; | Case 1,2,3; |
Flowchart of switch statement in C
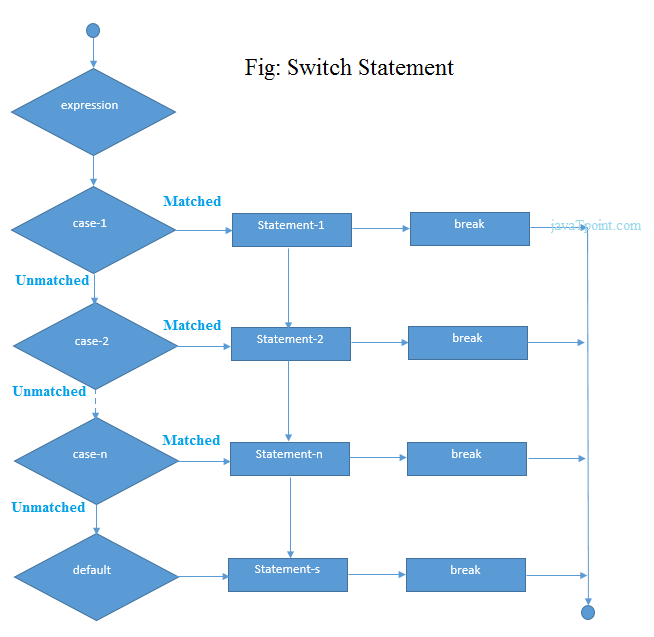
Functioning of switch case statement
First, the integer expression specified in the switch statement is evaluated. This value is then matched one by one with the constant values given in the different cases. If a match is found, then all the statements specified in that case are executed along with the all the cases present after that case including the default statement. No two cases can have similar values. If the matched case contains a break statement, then all the cases present after that will be skipped, and the control comes out of the switch. Otherwise, all the cases following the matched case will be executed.
Let's see a simple example of c language switch statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equals to 10");
- Break;
- Case 50:
- Printf("number is equal to 50");
- Break;
- Case 100:
- Printf("number is equal to 100");
- Break;
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Switch case example 2
- #include <stdio.h>
- Int main()
- {
- Int x = 10, y = 5;
- Switch(x>y && x+y>0)
- {
- Case 1:
- Printf("hi");
- Break;
- Case 0:
- Printf("bye");
- Break;
- Default:
- Printf(" Hello bye ");
- }
- }
Output
Hi
C Switch statement is fall-through
In C language, the switch statement is fall through; it means if you don't use a break statement in the switch case, all the cases after the matching case will be executed.
Let's try to understand the fall through state of switch statement by the example given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equal to 10\n");
- Case 50:
- Printf("number is equal to 50\n");
- Case 100:
- Printf("number is equal to 100\n");
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:10
Number is equal to 10
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Output
Enter a number:50
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Q4) Explain nested switch case statement with examples
A4)
We can use as many switch statement as we want inside a switch statement. Such type of statements is called nested switch case statements. Consider the following example.
- #include <stdio.h>
- Int main () {
- Int i = 10;
- Int j = 20;
- Switch(i) {
- Case 10:
- Printf("the value of i evaluated in outer switch: %d\n",i);
- Case 20:
- Switch(j) {
- Case 20:
- Printf("The value of j evaluated in nested switch: %d\n",j);
- }
- }
- Printf("Exact value of i is : %d\n", i );
- Printf("Exact value of j is : %d\n", j );
- Return 0;
- }
Output
The value of i evaluated in outer switch: 10
The value of j evaluated in nested switch: 20
Exact value of i is : 10
Exact value of j is : 20
Q5) Explain break statement and break statement with nested loop with examples
A5)
The break is a keyword in C which is used to bring the program control out of the loop. The break statement is used inside loops or switch statement. The break statement breaks the loop one by one, i.e., in the case of nested loops, it breaks the inner loop first and then proceeds to outer loops. The break statement in C can be used in the following two scenarios:
- With switch case
- With loop
Syntax:
- //loop or switch case
- Break;
Flowchart of break in c
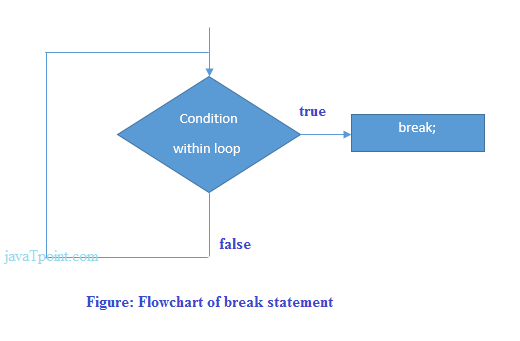
Example
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Int i;
- For(i = 0; i<10; i++)
- {
- Printf("%d ",i);
- If(i == 5)
- Break;
- }
- Printf("came outside of loop i = %d",i);
- }
Output
0 1 2 3 4 5 came outside of loop i = 5
Example of C breaks statement with switch case
C break statement with the nested loop
In such case, it breaks only the inner loop, but not outer loop.
- #include<stdio.h>
- Int main(){
- Int i=1,j=1;//initializing a local variable
- For(i=1;i<=3;i++){
- For(j=1;j<=3;j++){
- Printf("%d &d\n",i,j);
- If(i==2 && j==2){
- Break;//will break loop of j only
- }
- }//end of for loop
- Return 0;
- }
Output
1 1
1 2
1 3
2 1
2 2
3 1
3 2
3 3
As you can see the output on the console, 2 3 is not printed because there is a break statement after printing i==2 and j==2. But 3 1, 3 2 and 3 3 are printed because the break statement is used to break the inner loop only.
Break statement with while loop
Consider the following example to use break statement inside while loop.
- #include<stdio.h>
- Void main ()
- {
- Int i = 0;
- While(1)
- {
- Printf("%d ",i);
- i++;
- If(i == 10)
- Break;
- }
- Printf("came out of while loop");
- }
Output
0 1 2 3 4 5 6 7 8 9 came out of while loop
Break statement with do-while loop
Consider the following example to use the break statement with a do-while loop.
- #include<stdio.h>
- Void main ()
- {
- Int n=2,i,choice;
- Do
- {
- i=1;
- While(i<=10)
- {
- Printf("%d X %d = %d\n",n,i,n*i);
- i++;
- }
- Printf("do you want to continue with the table of %d , enter any non-zero value to continue.",n+1);
- Scanf("%d",&choice);
- If(choice == 0)
- {
- Break;
- }
- n++;
- }while(1);
- }
Output
2 X 1 = 2
2 X 2 = 4
2 X 3 = 6
2 X 4 = 8
2 X 5 = 10
2 X 6 = 12
2 X 7 = 14
2 X 8 = 16
2 X 9 = 18
2 X 10 = 20
Do you want to continue with the table of 3 , enter any non-zero value to continue.1
3 X 1 = 3
3 X 2 = 6
3 X 3 = 9
3 X 4 = 12
3 X 5 = 15
3 X 6 = 18
3 X 7 = 21
3 X 8 = 24
3 X 9 = 27
3 X 10 = 30
Do you want to continue with the table of 4 , enter any non-zero value to continue.0
Q6) Explain continue statement with examples
A6)
The continue statement in C language is used to bring the program control to the beginning of the loop. The continue statement skips some lines of code inside the loop and continues with the next iteration. It is mainly used for a condition so that we can skip some code for a particular condition.
Syntax:
- //loop statements
- Continue;
- //some lines of the code which is to be skipped
Continue statement example 1
- #include<stdio.h>
- Void main ()
- {
- Int i = 0;
- While(i!=10)
- {
- Printf("%d", i);
- Continue;
- i++;
- }
- }
Output
Infinite loop
Continue statement example 2
- #include<stdio.h>
- Int main(){
- Int i=1;//initializing a local variable
- //starting a loop from 1 to 10
- For(i=1;i<=10;i++){
- If(i==5){//if value of i is equal to 5, it will continue the loop
- Continue;
- }
- Printf("%d \n",i);
- }//end of for loop
- Return 0;
- }
Output
1
2
3
4
6
7
8
9
10
As you can see, 5 is not printed on the console because loop is continued at i==5.
C continue statement with inner loop
In such case, C continue statement continues only inner loop, but not outer loop.
- #include<stdio.h>
- Int main(){
- Int i=1,j=1;//initializing a local variable
- For(i=1;i<=3;i++){
- For(j=1;j<=3;j++){
- If(i==2 && j==2){
- Continue;//will continue loop of j only
- }
- Printf("%d %d\n",i,j);
- }
- }//end of for loop
- Return 0;
- }
Output
1 1
1 2
1 3
2 1
2 3
3 1
3 2
3 3
As you can see, 2 2 is not printed on the console because inner loop is continued at i==2 and j==2.
Q7) Explain goto statement with examples
A7)
The goto statement is known as jump statement in C. As the name suggests, goto is used to transfer the program control to a predefined label. The goto statment can be used to repeat some part of the code for a particular condition. It can also be used to break the multiple loops which can't be done by using a single break statement. However, using goto is avoided these days since it makes the program less readable and complecated.
Syntax:
- Label:
- //some part of the code;
- Goto label;
Goto example
Let's see a simple example to use goto statement in C language.
- #include <stdio.h>
- Int main()
- {
- Int num,i=1;
- Printf("Enter the number whose table you want to print?");
- Scanf("%d",&num);
- Table:
- Printf("%d x %d = %d\n",num,i,num*i);
- i++;
- If(i<=10)
- Goto table;
- }
Output:
Enter the number whose table you want to print?10
10 x 1 = 10
10 x 2 = 20
10 x 3 = 30
10 x 4 = 40
10 x 5 = 50
10 x 6 = 60
10 x 7 = 70
10 x 8 = 80
10 x 9 = 90
10 x 10 = 100
When should we use goto?
The only condition in which using goto is preferable is when we need to break the multiple loops using a single statement at the same time. Consider the following example.
- #include <stdio.h>
- Int main()
- {
- Int i, j, k;
- For(i=0;i<10;i++)
- {
- For(j=0;j<5;j++)
- {
- For(k=0;k<3;k++)
- {
- Printf("%d %d %d\n",i,j,k);
- If(j == 3)
- {
- Goto out;
- }
- }
- }
- }
- Out:
- Printf("came out of the loop");
- }
0 0 0
0 0 1
0 0 2
0 1 0
0 1 1
0 1 2
0 2 0
0 2 1
0 2 2
0 3 0
Came out of the loop
Q8) Explain do-while loop with examples
A8)
The do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.
do while loop syntax
The syntax of the C language do-while loop is given below:
- Do{
- //code to be executed
- }while(condition);
Example 1
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Char c;
- Int choice,dummy;
- Do{
- Printf("\n1. Print Hello\n2. Print Javatpoint\n3. Exit\n");
- Scanf("%d",&choice);
- Switch(choice)
- {
- Case 1 :
- Printf("Hello");
- Break;
- Case 2:
- Printf("Javatpoint");
- Break;
- Case 3:
- Exit(0);
- Break;
- Default:
- Printf("please enter valid choice");
- }
- Printf("do you want to enter more?");
- Scanf("%d",&dummy);
- Scanf("%c",&c);
- }while(c=='y');
- }
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
Do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
Do you want to enter more?
n
Flowchart of do while loop
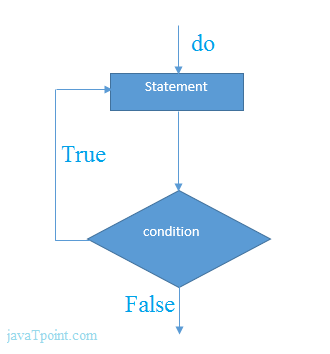
do while example
There is given the simple program of c language do while loop where we are printing the table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- Do{
- Printf("%d \n",i);
- i++;
- }while(i<=10);
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using do while loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- Do{
- Printf("%d \n",(number*i));
- i++;
- }while(i<=10);
- Return 0;
- }
Output
Enter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Infinitive do while loop
The do-while loop will run infinite times if we pass any non-zero value as the conditional expression.
- Do{
- //statement
- }while(1);
Q9) Explain while loop with examples
A9)
While loop is also known as a pre-tested loop. In general, a while loop allows a part of the code to be executed multiple times depending upon a given boolean condition. It can be viewed as a repeating if statement. The while loop is mostly used in the case where the number of iterations is not known in advance.
Syntax of while loop in C language
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
Flowchart of while loop in C
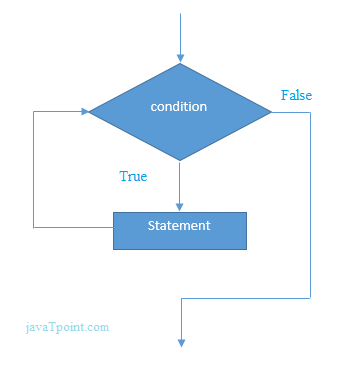
Example of the while loop in C language
Let's see the simple program of while loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- While(i<=10){
- Printf("%d \n",i);
- i++;
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using while loop in C
- #include<stdio.h>
- Int main(){
- Int i=1,number=0,b=9;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- While(i<=10){
- Printf("%d \n",(number*i));
- i++;
- }
- Return 0;
- }
Output
Enter a number: 50
50
100
150
200
250
300
350
400
450
500
Enter a number: 100
100
200
300
400
500
600
700
800
900
1000
Properties of while loop
- A conditional expression is used to check the condition. The statements defined inside the while loop will repeatedly execute until the given condition fails.
- The condition will be true if it returns 0. The condition will be false if it returns any non-zero number.
- In while loop, the condition expression is compulsory.
- Running a while loop without a body is possible.
- We can have more than one conditional expression in while loop.
- If the loop body contains only one statement, then the braces are optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int j = 1;
- While(j+=2,j<=10)
- {
- Printf("%d ",j);
- }
- Printf("%d",j);
- }
Output
3 5 7 9 11
Example 2
- #include<stdio.h>
- Void main ()
- {
- While()
- {
- Printf("hello Javatpoint");
- }
- }
Output
Compile time error: while loop can't be empty
Example 3
- #include<stdio.h>
- Void main ()
- {
- Int x = 10, y = 2;
- While(x+y-1)
- {
- Printf("%d %d",x--,y--);
- }
- }
Output
Infinite loop
Infinitive while loop in C
If the expression passed in while loop results in any non-zero value then the loop will run the infinite number of times.
- While(1){
- //statement
- }
Q10) Explain for loop with examples
A10)
The for loop in C language is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like the array and linked list.
Syntax of for loop in C
The syntax of for loop in c language is given below:
- For(Expression 1; Expression 2; Expression 3){
- //code to be executed
- }
Flowchart of for loop in C
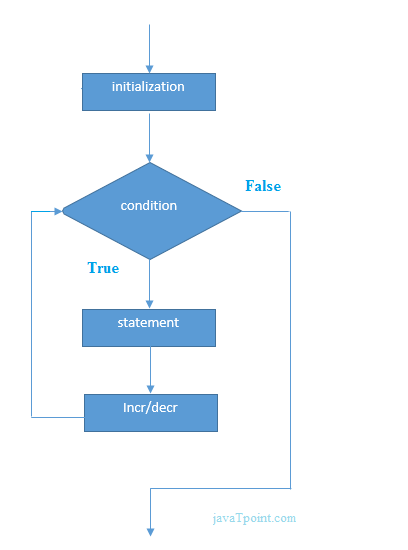
C for loop Examples
Let's see the simple program of for loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=0;
- For(i=1;i<=10;i++){
- Printf("%d \n",i);
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- For(i=1;i<=10;i++){
- Printf("%d \n",(number*i));
- }
- Return 0;
- }
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
Properties of Expression 1
- The expression represents the initialization of the loop variable.
- We can initialize more than one variable in Expression 1.
- Expression 1 is optional.
- In C, we can not declare the variables in Expression 1. However, It can be an exception in some compilers.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int a,b,c;
- For(a=0,b=12,c=23;a<2;a++)
- {
- Printf("%d ",a+b+c);
- }
- }
Output
35 36
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- For(;i<5;i++)
- {
- Printf("%d ",i);
- }
- }
Output
1 2 3 4
Properties of Expression 2
- Expression 2 is a conditional expression. It checks for a specific condition to be satisfied. If it is not, the loop is terminated.
- Expression 2 can have more than one condition. However, the loop will iterate until the last condition becomes false. Other conditions will be treated as statements.
- Expression 2 is optional.
- Expression 2 can perform the task of expression 1 and expression 3. That is, we can initialize the variable as well as update the loop variable in expression 2 itself.
- We can pass zero or non-zero value in expression 2. However, in C, any non-zero value is true, and zero is false by default.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;i<=4;i++)
- {
- Printf("%d ",i);
- }
- }
Output
0 1 2 3 4
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i,j,k;
- For(i=0,j=0,k=0;i<4,k<8,j<10;i++)
- {
- Printf("%d %d %d\n",i,j,k);
- j+=2;
- k+=3;
- }
- }
Output
0 0 0
1 2 3
2 4 6
3 6 9
4 8 12
Example 3
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;;i++)
- {
- Printf("%d",i);
- }
- }
Output
Infinite loop
Properties of Expression 3
- Expression 3 is used to update the loop variable.
- We can update more than one variable at the same time.
- Expression 3 is optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int i=0,j=2;
- For(i = 0;i<5;i++,j=j+2)
- {
- Printf("%d %d\n",i,j);
- }
- }
Output
0 2
1 4
2 6
3 8
4 10
Loop body
The braces {} are used to define the scope of the loop. However, if the loop contains only one statement, then we don't need to use braces. A loop without a body is possible. The braces work as a block separator, i.e., the value variable declared inside for loop is valid only for that block and not outside. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Int i;
- For(i=0;i<10;i++)
- {
- Int i = 20;
- Printf("%d ",i);
- }
- }
Output
20 20 20 20 20 20 20 20 20 20
Infinitive for loop in C
To make a for loop infinite, we need not give any expression in the syntax. Instead of that, we need to provide two semicolons to validate the syntax of the for loop. This will work as an infinite for loop.
- #include<stdio.h>
- Void main ()
- {
- For(;;)
- {
- Printf("welcome to javatpoint");
- }
- }
If you run this program, you will see above statement infinite times.