Unit - 7
Pointers and Arrays
Q1) Explain Pointer variable.
A1)
Pointers in C language is a variable that stores/points the address of another variable. A Pointer in C is used to allocate memory dynamically i.e. at run time. The pointer variable might be belonging to any of the data type such as int, float, char, double, short etc.
Pointer Syntax : data_type *var_name; Example : int *p; char *p;
Where, * is used to denote that “p” is pointer variable and not a normal variable.
Normal variable stores the value whereas pointer variable stores the address of the variable.
The content of the C pointer always be a whole number i.e. address.
Always C pointer is initialized to null, i.e. int *p = null.
The value of null pointer is 0.
& symbol is used to get the address of the variable.
* symbol is used to get the value of the variable that the pointer is pointing to.
If a pointer in C is assigned to NULL, it means it is pointing to nothing.
Two pointers can be subtracted to know how many elements are available between these two pointers.
But, Pointer addition, multiplication, division are not allowed.
The size of any pointer is 2 byte (for 16 bit compiler).
Example:
#include <stdio.h>
Int main()
{
int *ptr, q;
q = 50;
/* address of q is assigned to ptr */
ptr = &q;
/* display q's value using ptr variable */
printf("%d", *ptr);
return 0;
}
1
2
3
4
5
6
7
8
9
10
11
Q2) What is the different Pointer Arithmetic?
A2)
Pointer arithmetic is slightly different from arithmetic we normally use in our daily life. The only valid arithmetic operations applicable on pointers are:
- Addition of integer to a pointer
- Subtraction of integer to a pointer
- Subtracting two pointers of the same type
The pointer arithmetic is performed relative to the base type of the pointer. For example, if we have an integer pointer ip which contains address 1000, then on incrementing it by 1, we will get 1004 (i.e 1000 + 1 * 4) instead of 1001 because the
Size of the int data type is 4 bytes. If we had been using a system where the size of int is 2 bytes then we would get 1002 ( i.e 1000 + 1 * 2 ).
Similarly, on decrementing it we will get 996 (i.e 1000 - 1 * 4) instead of 999.
So, the expression ip + 4 will point to the address 1016 (i.e 1000 + 4 * 4 ).
Let's take some more examples.
1 2 3 | Int i = 12, *ip = &i; Double d = 2.3, *dp = &d; Char ch = 'a', *cp = &ch; |
Suppose the address of i, d and ch are 1000, 2000, 3000 respectively, therefore ip, dp and cp are at 1000, 2000, 3000 initially.
Q3) Explain different passing parameters.
A3)
A Parameter is the symbolic name for "data" that goes into a function. There are two ways to pass parameters in C: Pass by Value, Pass by Reference.
- Pass by Value
Pass by Value, means that a copy of the data is made and stored by way of the name of the parameter. Any changes to the parameter have NO affect on data in the calling function.
- Pass by Reference
A reference parameter "refers" to the original data in the calling function. Thus any changes made to the parameter are ALSO MADE TO THE ORIGINAL variable.
There are two ways to make a pass by reference parameter:
- ARRAYS
Arrays are always pass by reference in C. Any change made to the parameter containing the array will change the value of the original array.
2. The ampersand used in the function prototype.
Function ( & parameter_name )
To make a normal parameter into a pass by reference parameter, we use the "& param" notation. The ampersand (&) is the syntax to tell C that any changes made to the parameter also modify the original variable containing the data.
Q4) What is Declaration of structures?
A4)
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
- Why Use Structures
• Ordinary variables can hold one piece of information
• arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure
Called employee with three elements id, name and salary. The syntax of this structure is as
Follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Note:
Struct keyword is used to declare structure.
Members of structure are enclosed within opening and closing braces.
Usually structure type declaration appears at the top of the source code file, before any variables or functions are defined or maintained in separate header file.
Declaration of Structure reserves no space.
It is nothing but the “ Template / Map / Shape ” of the structure .
Memory is created , very first time when the variable is created / Instance is created.
- Structure variable declaration:
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1 ,e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
- Memory allocation for structure
Memory is allocated to the structure only when we create the variable of structure.
Consider following example
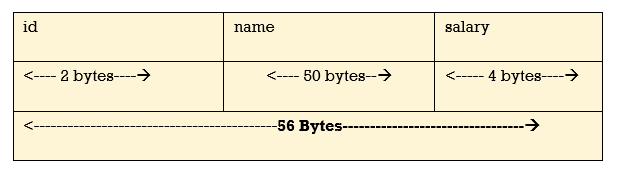
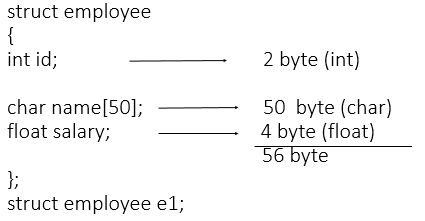
- Structure Initialization
1. When we declare a structure, memory is not allocated for un-initialized variable.
2. Let us discuss very familiar example of structure student , we can initialize structure variable
In different ways –
Way 1 : Declare and Initialize
Struct student
{
Char name[20];
Int roll;
Float marks;
}std1 = { "Poonam",89,78.3 };
In the above code snippet, we have seen that structure is declared and as soon as after declaration we
Have initialized the structure variable.
Std1 = { "Poonam",89,78.3 }
This is the code for initializing structure variable in C programming
Way 2 : Declaring and Initializing Multiple Variables
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Way 3 : Initializing Single member
Struct student
{
Int mark1;
Int mark2;
Int mark3;
} sub1={67};
Though there are three members of structure,only one is initialized , Then remaining two members
Are initialized with Zero. If there are variables of other data type then their initial values will be –
Data Type Default value if not initialized
Integer 0
Float 0.00
Char NULL
Way 4 : Initializing inside main
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
Void main()
{
Struct student s1 = {89,54,65};
- - - - --
- - - - --
- - - - --
};
When we declare a structure then memory won’t be allocated for the structure. i.e only writing below
Declaration statement will never allocate memory
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
We need to initialize structure variable to allocate some memory to the structure.
Struct student s1 = {89,54,65};
Q5) Explain Pointer to pointer.
A5)
Till this point we are clear about the pointer of different data types in this section we study the pointer of pointer which is also called as double pointer.
Pointer variable is responsible to hold the address of a variable but when user defines pointer to pointer variable then that variable is responsible to hold the address of pointer variable. Pointer to pointer variable is declare by putting extra asterisk sign before the pointer variable. Following is the declaration of pointer to pointer variable
Data-Type**variable-name
Data type is responsible to define type of value stored at the location of pointer variable.
Two asterisk signs indicated that it is pointer to pointer variable.
User can allocate any name to the pointer variable by following all the rules of allocating variable name.
Consider the following program
#include<stdio.h>
#include<conio.h>
Void main()
{
Int n=5;
Int *np,**npp;
Np=&n;
Npp=&np;
Printf("\n value of n is %d",n);
Printf("\n value of n is %d",*np);
Printf("\n value of n is %d",**npp);
Getch();
}
Above program is basic program for double pointer which contains three ‘printf’ statements. The explanation of these statements are as follows.
1.In the first ‘printf’ statement compiler will directly print the value of variable ‘n’ which is ‘5’.
2.In the second ‘printf’ statement compiler will print the value of pointer variable ‘np’. ‘np’ variable contains the address of variable ‘n’ but as we put the asterisk sign before it so it will print the value which is present at the address of variable ‘n’ which is ‘5’
3.In the third printf statement compiler will print the value of pointer to pointer variable ‘npp’. ‘npp’ variable contains the address of pointer variable ‘np’ and ‘np’ variable contains the address of variable n’ but as we put the two asterisk signs before it so it will print the value which is present at the address of variable ‘n’ which is ‘5’. If we put only one asterisk sign the it will print the address of variable ‘n’.
User can allocate any name to the pointer variable by following all the rules of allocating variable
Q6) Explain Pointer to structure.
A6)
A pointer to structures is where a pointer variable can point to the address of a structure variable. Here is how we can declare a pointer to a structure variable.
1 2 3 4 5 6 7 8 9 10 11 12 | Struct dog { Char name[10]; Char breed[10]; Int age; Char color[10]; };
Struct dog spike;
// declaring a pointer to a structure of type struct dog Struct dog *ptr_dog |
This declares a pointer ptr_dog that can store the address of the variable of type struct dog. We can now assign the address of variable spike to ptr_dog using & operator.
1 | Ptr_dog = &spike; |
Now ptr_dog points to the structure variable spike.
Accessing members using Pointer
There are two ways of accessing members of structure using pointer:
- Using indirection (*) operator and dot (.) operator.
- Using arrow (->) operator or membership operator.
Using indirection (*) operator and dot (.) operator
At this point ptr_dog points to the structure variable spike, so by dereferencing it we will get the contents of the spike. This means spike and *ptr_dog are functionally equivalent. To access a member of structure write *ptr_dog followed by a dot(.) operator, followed by the name of the member.
For example:
(*ptr_dog).name – refers to the name of dog
(*ptr_dog).breed – refers to the breed of dog
Parentheses around *ptr_dog are necessary because the precedence of dot(.) operator is greater than that of indirection (*) operator.
Using arrow (->) operator or membership operator.
To access members using arrow (->) operator write pointer variable followed by -> operator, followed by name of the member.
Ptr_dog->name - refers to the name of dog
Ptr_dog->breed - refers to the breed of dog
We can also modify the value of members using pointer notation.
| Strcpy(ptr_dog->name, "new_name");
In the above expression precedence of arrow operator (->) is greater than that of prefix decrement operator (--), so first -> operator is applied in the expression then its value is decremented by 1. #include<stdio.h> Struct dog { char name[10]; char breed[10]; int age; char color[10]; };
Int main() { struct dog my_dog = {"tyke", "Bulldog", 5, "white"}; struct dog *ptr_dog; ptr_dog = &my_dog;
printf("Dog's name: %s\n", ptr_dog->name); printf("Dog's breed: %s\n", ptr_dog->breed); printf("Dog's age: %d\n", ptr_dog->age); printf("Dog's color: %s\n", ptr_dog->color);
// changing the name of dog from tyke to jack strcpy(ptr_dog->name, "jack");
// increasing age of dog by 1 year ptr_dog->age++;
printf("Dog's new name is: %s\n", ptr_dog->name); printf("Dog's age is: %d\n", ptr_dog->age);
// signal to operating system program ran fine return 0; } Expected Output:
1 2 3 4 5 6 7 8 9 Dog's name: tyke Dog's breed: Bulldog Dog's age: 5 Dog's color: white
After changes
Dog's new name is: jack Dog's age is: 6 |
Q7) Explain pointer to function.
A7)
Pointer to function is the very important concept in C language. With the help of pointer to function user can access the several different functions on the basis of input data. Function in C language is used to improve reusability of the code. With the help of function user can use the same sample of code for the multiple times. The process of debugging and error correction is also easier with the help of function. Functions divide the complete program into several different modules. Syntax for defining function is as follows
Syntax : Data_typeFunction_name();
Example: # int fun ( ) ;
In the above syntax ‘fun’ is the function name which is of type integer. The function name ‘fun’ is also work as pointer variable to function. But it is the constant pointer variable so it is illegal to assign value to ‘fun’. Following program illustrate the syntax and use of function
#include<stdio.h>
#include<conio.h>
Void sayhi()
{
Printf("hi everyone");
}
Void main()
{
Clrscr();
Sayhi();
Getch();
}
Output of above program is as follows
Hi everyone
In the above program we create function ‘sayhi’ and use it directly into the main function. Now we will see how to declare pointer to function. Pointer variable name should followed by asterisk sign. Syntax for declaring pointer to function is as follows.
Syntax :Data_type (*pointer_name)();
Example :int(*ptr)();
In the above example we declare pointer variable ‘ptr’ which is responsible to hold the pointer to a function and return an int. Pointer name should be enclosed with the parenthesis as lack of parenthesis pointer variable returns pointer to an int. Following program illustrate the syntax and use of pointer to function
#include<stdio.h>
#include<conio.h>
Void sayhi()
{
Printf("hi everyone");
}
Void main()
{
Void(*sayhiptr)()=sayhi;
Clrscr();
(*sayhiptr)();
Getch();
}
Output of above program is as follows
Hi everyone
In the above program we create function ‘sayhi’ and pointer to function ‘sayhiptr’. The function will not return anything so return type is void. Name of function works as a pointer to function and contains the address of function. So, we assign it to the pointer variable. At last line we call function with the help of pointer.
Function Pointer with Parameter:
Like routine function call, user is also allowed to send pameter with the function pointer.
Consider the following program of passing three integer type parameters to function with the help of function pointer.
#include<stdio.h>
#include<conio.h>
Void total(int m1,int m2, int m3)
{
Int result=m1+m2+m3;
Printf("\n Total marks is %d",result);
}
Void main()
{
Int m1,m2,m3;
Void (*totalptr)()=total;
Clrscr();
Printf("Enter the marks of three subjects");
Scanf("%d %d %d", &m1,&m2,&m3);
(*totalptr)(m1,m2,m3);
Getch();
}
Output of above program is as follows
Enter the marks of three subjects
25
35
30
Total marks is 90
In the above program we create function ‘total’ which accept three integer type values from the main function. Void (*totalptr)()=total; line is responsible to declare and define pointer to function variable. Whereas line(*totalptr)(m1,m2,m3); is used to call function with the help of pointer.
Q8) Explain Unions dynamic memory allocations, unions
A8)
Unions are quite similar to the structures in C. Union is also a derived type as
Structure. Union can be defined in same manner as structures just the keyword
Used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union
Car is created.
- Memory allocation for union
Like structure memory is allocated to union only when we create the variable of it.
The memory is allocated to union according to the largest data members of the union.
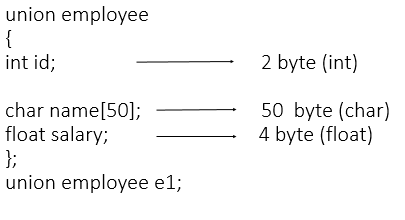
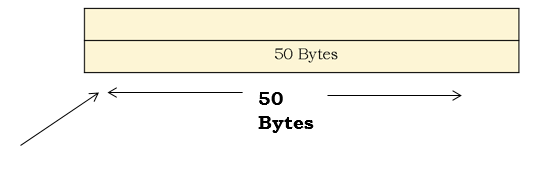
- Accessing members of an union
Array elements are accessed using the Subscript variable , Similarly Union members are accessed using dot [.] operator.
(.) is called as “union member Operator”.
Use this Operator in between “Union variable” & “member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
union employee e1= { 1, “ABC”, 50000 };
printf(“%d”, e. Id);
printf(“%s”, e. Name);
}
O/P- garbage value, ABC
Q9) Explain File management.
A9)
A File can be used to store a large volume of persistent data. Like many other languages 'C' provides following file management functions,
- Creation of a file
- Opening a file
- Reading a file
- Writing to a file
- Closing a file
Following are the most important file management functions available in 'C,'
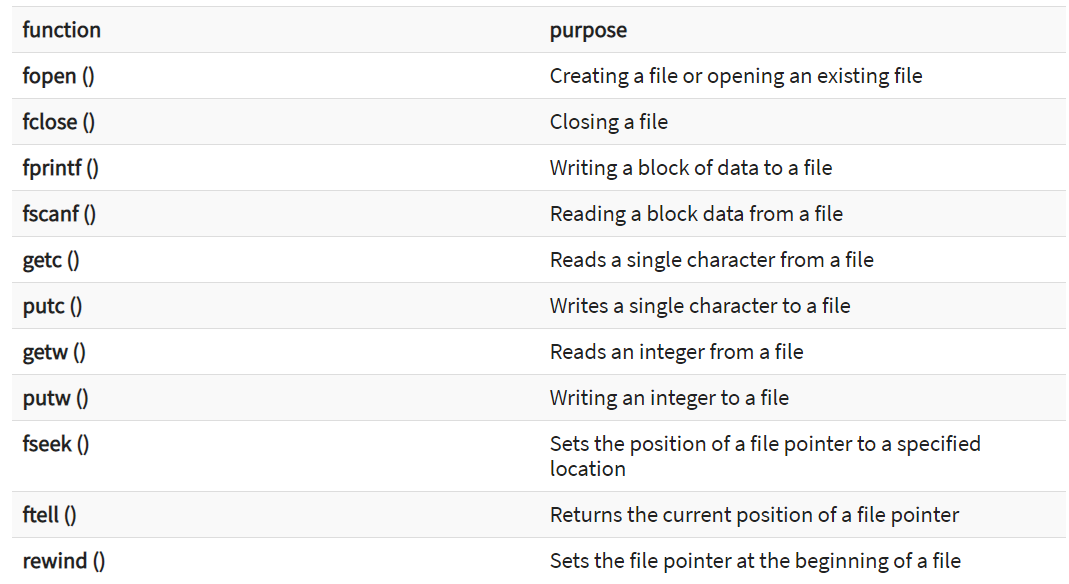
Q10) Explain Array of pointers using examples
A10)
Consider the following program:
#include<stdio.h> Int main() { Int arr[5] = { 1, 2, 3, 4, 5 }; Int *ptr = arr; Printf("%p\n", ptr); return 0; } |
In this program, we have a pointer ptr that points to the 0th element of the array. Similarly, we can also declare a pointer that can point to whole array instead of only one element of the array. This pointer is useful when talking about multidimensional arrays.
Syntax:
Data_type (*var_name)[size_of_array];
Example:
Int (*ptr)[10];
Here ptr is pointer that can point to an array of 10 integers. Since subscript have higher precedence than indirection, it is necessary to enclose the indirection operator and pointer name inside parentheses. Here the type of ptr is ‘pointer to an array of 10 integers’.