UNIT 6
File Handling and Design Patterns
- Write in brief an introduction of File Handling.
Answer: In programming, we may require some specific input data to be generated several numbers of times. Sometimes, it is not enough to only display the data on the console. The data to be displayed may be very large, and only a limited amount of data can be displayed on the console, and since the memory is volatile, it is impossible to recover the programmatically generated data again and again. However, if we need to do so, we may store it onto the local file system which is volatile and can be accessed every time. Here, comes the need of file handling in C.
File handling in C enables us to create, update, read, and delete the files stored on the local file system through our C program. The following operations can be performed on a file.
- Creation of the new file
- Opening an existing file
- Reading from the file
- Writing to the file
- Deleting the file
Functions for file handling
There are many functions in the C library to open, read, write, search and close the file. A list of file functions are given below:
No. | Function | Description |
1 | Fopen() | Opens new or existing file |
2 | Fprintf() | Write data into the file |
3 | Fscanf() | Reads data from the file |
4 | Fputc() | Writes a character into the file |
5 | Fgetc() | Reads a character from file |
6 | Fclose() | Closes the file |
7 | Fseek() | Sets the file pointer to given position |
8 | Fputw() | Writes an integer to file |
9 | Fgetw() | Reads an integer from file |
10 | Ftell() | Returns current position |
11 | Rewind() | Sets the file pointer to the beginning of the file |
Opening File: fopen()
We must open a file before it can be read, write, or update. The fopen() function is used to open a file. The syntax of the fopen() is given below.
- FILE *fopen( const char * filename, const char * mode );
The fopen() function accepts two parameters:
- The file name (string). If the file is stored at some specific location, then we must mention the path at which the file is stored. For example, a file name can be like "c://some_folder/some_file.ext".
- The mode in which the file is to be opened. It is a string.
We can use one of the following modes in the fopen() function.
Mode | Description |
r | Opens a text file in read mode |
w | Opens a text file in write mode |
a | Opens a text file in append mode |
r+ | Opens a text file in read and write mode |
w+ | Opens a text file in read and write mode |
a+ | Opens a text file in read and write mode |
Rb | Opens a binary file in read mode |
Wb | Opens a binary file in write mode |
Ab | Opens a binary file in append mode |
Rb+ | Opens a binary file in read and write mode |
Wb+ | Opens a binary file in read and write mode |
Ab+ | Opens a binary file in read and write mode |
The fopen function works in the following way.
- Firstly, It searches the file to be opened.
- Then, it loads the file from the disk and place it into the buffer. The buffer is used to provide efficiency for the read operations.
- It sets up a character pointer which points to the first character of the file.
Consider the following example which opens a file in write mode.
- #include<stdio.h>
- Void main( )
- {
- FILE *fp ;
- Char ch ;
- Fp = fopen("file_handle.c","r") ;
- While ( 1 )
- {
- Ch = fgetc ( fp ) ;
- If ( ch == EOF )
- Break ;
- Printf("%c",ch) ;
- }
- Fclose (fp ) ;
- }
Output
The content of the file will be printed.
#include;
Void main( )
{
FILE *fp; // file pointer
Charch;
Fp = fopen("file_handle.c","r");
While ( 1 )
{
Ch = fgetc ( fp ); //Each character of the file is read and stored in the character file.
If ( ch == EOF )
Break;
Printf("%c",ch);
}
Fclose (fp );
}
Closing File: fclose()
The fclose() function is used to close a file. The file must be closed after performing all the operations on it. The syntax of fclose() function is given below:
- Int fclose( FILE *fp );
C fprintf() and fscanf()
C fputc() and fgetc()
C fputs() and fgets()
C fseek()
2. Explain Concepts of Stream
Answer: We are using the iostream standard library, it provides cin and cout methods for reading from input and writing to output respectively.
To read and write from a file we are using the standard C++ library called fstream. Let us see the data types define in fstream library is:
Data Type | Description |
Fstream | It is used to create files, write information to files, and read information from files. |
Ifstream | It is used to read information from files. |
Ofstream | It is used to create files and write information to the files. |
FileStream example: writing to a file
Let's see the simple example of writing to a text file testout.txt using C++ FileStream programming.
- #include <iostream>
- #include <fstream>
- Using namespace std;
- Int main () {
- Ofstream filestream("testout.txt");
- If (filestream.is_open())
- {
- Filestream << "Welcome to javaTpoint.\n";
- Filestream << "C++ Tutorial.\n";
- Filestream.close();
- }
- Else cout <<"File opening is fail.";
- Return 0;
- }
Output:
The content of a text file testout.txt is set with the data:
Welcome to javaTpoint.
C++ Tutorial.
FileStream example: reading from a file
Let's see the simple example of reading from a text file testout.txt using C++ FileStream programming.
- #include <iostream>
- #include <fstream>
- Using namespace std;
- Int main () {
- String srg;
- Ifstream filestream("testout.txt");
- If (filestream.is_open())
- {
- While ( getline (filestream,srg) )
- {
- Cout << srg <<endl;
- }
- Filestream.close();
- }
- Else {
- Cout << "File opening is fail."<<endl;
- }
- Return 0;
- }
Note: Before running the code a text file named as "testout.txt" is need to be created and the content of a text file is given below:
Welcome to javaTpoint.
C++ Tutorial.
Output:
Welcome to javaTpoint.
C++ Tutorial.
3. Read and Write Example
Answer: Let's see the simple example of writing the data to a text file testout.txt and then reading the data from the file using C++ FileStream programming.
- #include <fstream>
- #include <iostream>
- Using namespace std;
- Int main () {
- Char input[75];
- Ofstream os;
- Os.open("testout.txt");
- Cout <<"Writing to a text file:" << endl;
- Cout << "Please Enter your name: ";
- Cin.getline(input, 100);
- Os << input << endl;
- Cout << "Please Enter your age: ";
- Cin >> input;
- Cin.ignore();
- Os << input << endl;
- Os.close();
- Ifstream is;
- String line;
- Is.open("testout.txt");
- Cout << "Reading from a text file:" << endl;
- While (getline (is,line))
- {
- Cout << line << endl;
- }
- Is.close();
- Return 0;
- }
Output:
Writing to a text file:
Please Enter your name: Nakul Jain
Please Enter your age: 22
Reading from a text file: Nakul Jain
22
4. Explain Stream Classes
Answer: In C++ stream refers to the stream of characters that are transferred between the program thread and i/o.
Stream classes in C++ are used to input and output operations on files and io devices. These classes have specific features and to handle input and output of the program.
The iostream.h library holds all the stream classes in the C++ programming language.
Let's see the hierarchy and learn about them,
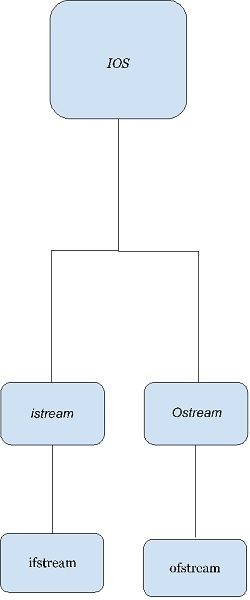
Now, let’s learn about the classes of the iostream library.
Ios class− This class is the base class for all stream classes. The streams can be input or output streams. This class defines members that are independent of how the templates of the class are defined.
Istream Class− The istream class handles the input stream in c++ programming language. These input stream objects are used to read and interpret the input as a sequence of characters. The cin handles the input.
Ostream class− The ostream class handles the output stream in c++ programming language. These output stream objects are used to write data as a sequence of characters on the screen. Cout and puts handle the out streams in c++ programming language.
Example
OUT STREAM
COUT
#include<iostream>
Usingnamespacestd;
Int main(){
Cout<<"This output is printed on screen";
}
Output
This output is printed on screen
PUTS
#include<iostream>
Usingnamespacestd;
Int main(){
Puts("This output is printed using puts");
}
Output
This output is printed using puts
IN STREAM
CIN
#include<iostream>
Usingnamespacestd;
Int main(){
Intno;
Cout<<"Enter a number ";
Cin>>no;
Cout<<"Number entered using cin is "<
Output
Enter a number 3453
Number entered using cin is 3453
Gets
#include<iostream>
Usingnamespacestd;
Int main(){
Charch[10];
Puts("Enter a character array");
Gets(ch);
Puts("The character array entered using gets is : ");
Puts(ch);
}
Output
Enter a character array
Thdgf
The character array entered using gets is :
Thdgf
5. Explain Byte Stream Classes
Answer: These handle data in bytes (8 bits) i.e., the byte stream classes read/write data of 8 bits. Using these you can store characters, videos, audios, images etc.
The InputStream and OutputStream classes (abstract) are the super classes of all the input/output stream classes: classes that are used to read/write a stream of bytes. Following are the byte array stream classes provided by Java −
InputStream | OutputStream |
FIleInputStream | FileOutputStream |
ByteArrayInputStream | ByteArrayOutputStream |
ObjectInputStream | ObjectOutputStream |
PipedInputStream | PipedOutputStream |
FilteredInputStream | FilteredOutputStream |
BufferedInputStream | BufferedOutputStream |
DataInputStream | DataOutputStream |
Example
Following Java program reads data from a particular file using FileInputStream and writes it to another, using FileOutputStream.
Importjava.io.File;
Importjava.io.FileInputStream;
Importjava.io.FileOutputStream;
Importjava.io.IOException;
PublicclassIOStreamsExample{
Publicstaticvoid main(Stringargs[])throwsIOException{
//Creating FileInputStream object
Filefile=newFile("D:/myFile.txt");
FileInputStreamfis=newFileInputStream(file);
Byte bytes[]=newbyte[(int)file.length()];
//Reading data from the file
Fis.read(bytes);
//Writing data to another file
Fileout=newFile("D:/CopyOfmyFile.txt");
FileOutputStreamoutputStream=newFileOutputStream(out);
//Writing data to the file
OutputStream.write(bytes);
OutputStream.flush();
System.out.println("Data successfully written in the specified file");
}
}
Output
Data successfully written in the specified file
6. Explain Character Stream
Answer: Character Streams−These handle data in 16 bit Unicode. Using these you can read and write text data only.
The Reader and Writer classes (abstract) are the super classes of all the character stream classes: classes that are used to read/write character streams. Following are the character array stream classes provided by Java −
Reader | Writer |
BufferedReader | BufferedWriter |
CharacterArrayReader | CharacterArrayWriter |
StringReader | StringWriter |
FileReader | FileWriter |
InputStreamReader | InputStreamWriter |
FileReader | FileWriter |
Example
The following Java program reads data from a particular file using FileReader and writes it to another, using FileWriter.
Importjava.io.File;
Importjava.io.FileReader;
Importjava.io.FileWriter;
Importjava.io.IOException;
PublicclassIOStreamsExample{
Publicstaticvoid main(Stringargs[])throwsIOException{
//Creating FileReader object
Filefile=newFile("D:/myFile.txt");
FileReader reader =newFileReader(file);
Char chars[]=newchar[(int)file.length()];
//Reading data from the file
Reader.read(chars);
//Writing data to another file
Fileout=newFile("D:/CopyOfmyFile.txt");
FileWriter writer =newFileWriter(out);
//Writing data to the file
Writer.write(chars);
Writer.flush();
System.out.println("Data successfully written in the specified file");
}
}
Output
Data successfully written in the specified file
7. Explain in detail Other Useful I/O Classes with examples
Answer: The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Stream
A stream can be defined as a sequence of data. There are two kinds of Streams −
- InPutStream− The InputStream is used to read data from a source.
- OutPutStream− The OutputStream is used for writing data to a destination.

Java provides strong but flexible support for I/O related to files and networks but this tutorial covers very basic functionality related to streams and I/O. We will see the most commonly used examples one by one −
Byte Streams
Java byte streams are used to perform input and output of 8-bit bytes. Though there are many classes related to byte streams but the most frequently used classes are, FileInputStream and FileOutputStream. Following is an example which makes use of these two classes to copy an input file into an output file −
Example
Import java.io.*;
PublicclassCopyFile{
Publicstaticvoid main(Stringargs[])throwsIOException{
FileInputStreamin=null;
FileOutputStreamout=null;
Try{
In=newFileInputStream("input.txt");
Out=newFileOutputStream("output.txt");
Int c;
While((c =in.read())!=-1){
Out.write(c);
}
}finally{
If(in!=null){
In.close();
}
If(out!=null){
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Character Streams
Java Byte streams are used to perform input and output of 8-bit bytes, whereas Java Character streams are used to perform input and output for 16-bit unicode. Though there are many classes related to character streams but the most frequently used classes are, FileReader and FileWriter. Though internally FileReader uses FileInputStream and FileWriter uses FileOutputStream but here the major difference is that FileReader reads two bytes at a time and FileWriter writes two bytes at a time.
We can re-write the above example, which makes the use of these two classes to copy an input file (having unicode characters) into an output file −
Example
Import java.io.*;
PublicclassCopyFile{
Publicstaticvoid main(Stringargs[])throwsIOException{
FileReaderin=null;
FileWriterout=null;
Try{
In=newFileReader("input.txt");
Out=newFileWriter("output.txt");
Int c;
While((c =in.read())!=-1){
Out.write(c);
}
}finally{
If(in!=null){
In.close();
}
If(out!=null){
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Standard Streams
All the programming languages provide support for standard I/O where the user's program can take input from a keyboard and then produce an output on the computer screen. If you are aware of C or C++ programming languages, then you must be aware of three standard devices STDIN, STDOUT and STDERR. Similarly, Java provides the following three standard streams −
- Standard Input−This is used to feed the data to user's program and usually a keyboard is used as standard input stream and represented as System.in.
- Standard Output−This is used to output the data produced by the user's program and usually a computer screen is used for standard output stream and represented as System.out.
- Standard Error− This is used to output the error data produced by the user's program and usually a computer screen is used for standard error stream and represented as System.err.
Following is a simple program, which creates InputStreamReader to read standard input stream until the user types a "q" −
Example
Import java.io.*;
PublicclassReadConsole{
Publicstaticvoid main(Stringargs[])throwsIOException{
InputStreamReadercin=null;
Try{
Cin=newInputStreamReader(System.in);
System.out.println("Enter characters, 'q' to quit.");
Char c;
Do{
c =(char)cin.read();
System.out.print(c);
}while(c !='q');
}finally{
If(cin!=null){
Cin.close();
}
}
}
}
Let's keep the above code in ReadConsole.java file and try to compile and execute it as shown in the following program. This program continues to read and output the same character until we press 'q' −
$javac ReadConsole.java
$java ReadConsole
Enter characters, 'q' to quit.
1
1
e
e
q
q
Reading and Writing Files
As described earlier, a stream can be defined as a sequence of data. The InputStream is used to read data from a source and the OutputStream is used for writing data to a destination.
Here is a hierarchy of classes to deal with Input and Output streams.
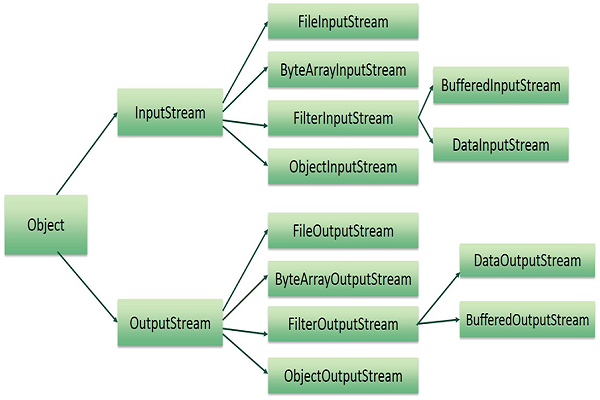
The two important streams are FileInputStream and FileOutputStream, which would be discussed in this tutorial.
FileInputStream
This stream is used for reading data from the files. Objects can be created using the keyword new and there are several types of constructors available.
Following constructor takes a file name as a string to create an input stream object to read the file −
InputStream f = new FileInputStream("C:/java/hello");
Following constructor takes a file object to create an input stream object to read the file. First we create a file object using File() method as follows −
File f = new File("C:/java/hello");
InputStream f = new FileInputStream(f);
Once you have InputStream object in hand, then there is a list of helper methods which can be used to read to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public int read(int r)throws IOException{} This method reads the specified byte of data from the InputStream. Returns an int. Returns the next byte of data and -1 will be returned if it's the end of the file. |
4 | Public int read(byte[] r) throws IOException{} This method reads r.length bytes from the input stream into an array. Returns the total number of bytes read. If it is the end of the file, -1 will be returned. |
5 | Public int available() throws IOException{} Gives the number of bytes that can be read from this file input stream. Returns an int. |
There are other important input streams available, for more detail you can refer to the following links −
- ByteArrayInputStream
- DataInputStream
FileOutputStream
FileOutputStream is used to create a file and write data into it. The stream would create a file, if it doesn't already exist, before opening it for output.
Here are two constructors which can be used to create a FileOutputStream object.
Following constructor takes a file name as a string to create an input stream object to write the file −
OutputStream f = new FileOutputStream("C:/java/hello")
Following constructor takes a file object to create an output stream object to write the file. First, we create a file object using File() method as follows −
File f = new File("C:/java/hello");
OutputStream f = new FileOutputStream(f);
Once you have OutputStream object in hand, then there is a list of helper methods, which can be used to write to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public void write(int w)throws IOException{} This methods writes the specified byte to the output stream. |
4 | Public void write(byte[] w) Writes w.length bytes from the mentioned byte array to the OutputStream. |
There are other important output streams available, for more detail you can refer to the following links −
- ByteArrayOutputStream
- DataOutputStream
Example
Following is the example to demonstrate InputStream and OutputStream−
Import java.io.*;
PublicclassfileStreamTest{
Publicstaticvoid main(Stringargs[]){
Try{
BytebWrite[]={11,21,3,40,5};
OutputStreamos=newFileOutputStream("test.txt");
For(int x =0; x <bWrite.length; x++){
Os.write(bWrite[x]);// writes the bytes
}
Os.close();
InputStreamis=newFileInputStream("test.txt");
Int size =is.available();
For(inti=0;i< size;i++){
System.out.print((char)is.read()+" ");
}
Is.close();
}catch(IOException e){
System.out.print("Exception");
}
}
}
The above code would create file test.txt and would write given numbers in binary format. Same would be the output on the stdout screen.
File Navigation and I/O
There are several other classes that we would be going through to get to know the basics of File Navigation and I/O.
- File Class
- FileReader Class
- FileWriter Class
Directories in Java
A directory is a File which can contain a list of other files and directories. You use File object to create directories, to list down files available in a directory. For complete detail, check a list of all the methods which you can call on File object and what are related to directories.
Creating Directories
There are two useful File utility methods, which can be used to create directories −
- The mkdir( ) method creates a directory, returning true on success and false on failure. Failure indicates that the path specified in the File object already exists, or that the directory cannot be created because the entire path does not exist yet.
- The mkdirs() method creates both a directory and all the parents of the directory.
Following example creates "/tmp/user/java/bin" directory −
Example
Importjava.io.File;
PublicclassCreateDir{
Publicstaticvoid main(Stringargs[]){
Stringdirname="/tmp/user/java/bin";
File d =newFile(dirname);
// Create directory now.
d.mkdirs();
}
}
Compile and execute the above code to create "/tmp/user/java/bin".
Note− Java automatically takes care of path separators on UNIX and Windows as per conventions. If you use a forward slash (/) on a Windows version of Java, the path will still resolve correctly.
Listing Directories
You can use list( ) method provided by File object to list down all the files and directories available in a directory as follows −
Example
Importjava.io.File;
PublicclassReadDir{
Publicstaticvoid main(String[]args){
Filefile=null;
String[] paths;
Try{
// create new file object
File=newFile("/tmp");
// array of files and directory
Paths=file.list();
// for each name in the path array
For(Stringpath:paths){
// prints filename and directory name
System.out.println(path);
}
}catch(Exception e){
// if any error occurs
e.printStackTrace();
}
}
}
This will produce the following result based on the directories and files available in your /tmp directory −
Output
Test1.txt
Test2.txt
ReadDir.java
ReadDir.class
8. Explain Using the File Class
Answer: The File class is an abstract representation of file and directory pathname. A pathname can be either absolute or relative.
The File class have several methods for working with directories and files such as creating new directories or files, deleting and renaming directories or files, listing the contents of a directory etc.
Fields
Modifier | Type | Field | Description |
Static | String | PathSeparator | It is system-dependent path-separator character, represented as a string for convenience. |
Static | Char | PathSeparatorChar | It is system-dependent path-separator character. |
Static | String | Separator | It is system-dependent default name-separator character, represented as a string for convenience. |
Static | Char | SeparatorChar | It is system-dependent default name-separator character. |
Constructors
Constructor | Description |
File(File parent, String child) | It creates a new File instance from a parent abstract pathname and a child pathname string. |
File(String pathname) | It creates a new File instance by converting the given pathname string into an abstract pathname. |
File(String parent, String child) | It creates a new File instance from a parent pathname string and a child pathname string. |
File(URI uri) | It creates a new File instance by converting the given file: URI into an abstract pathname. |
Useful Methods
Modifier and Type | Method | Description |
Static File | CreateTempFile(String prefix, String suffix) | It creates an empty file in the default temporary-file directory, using the given prefix and suffix to generate its name. |
Boolean | CreateNewFile() | It atomically creates a new, empty file named by this abstract pathname if and only if a file with this name does not yet exist. |
Boolean | CanWrite() | It tests whether the application can modify the file denoted by this abstract pathname.String[] |
Boolean | CanExecute() | It tests whether the application can execute the file denoted by this abstract pathname. |
Boolean | CanRead() | It tests whether the application can read the file denoted by this abstract pathname. |
Boolean | IsAbsolute() | It tests whether this abstract pathname is absolute. |
Boolean | IsDirectory() | It tests whether the file denoted by this abstract pathname is a directory. |
Boolean | IsFile() | It tests whether the file denoted by this abstract pathname is a normal file. |
String | GetName() | It returns the name of the file or directory denoted by this abstract pathname. |
String | GetParent() | It returns the pathname string of this abstract pathname's parent, or null if this pathname does not name a parent directory. |
Path | ToPath() | It returns a java.nio.file.Path object constructed from the this abstract path. |
URI | ToURI() | It constructs a file: URI that represents this abstract pathname. |
File[] | ListFiles() | It returns an array of abstract pathnames denoting the files in the directory denoted by this abstract pathname |
Long | GetFreeSpace() | It returns the number of unallocated bytes in the partition named by this abstract path name. |
String[] | List(FilenameFilter filter) | It returns an array of strings naming the files and directories in the directory denoted by this abstract pathname that satisfy the specified filter. |
Boolean | Mkdir() | It creates the directory named by this abstract pathname. |
9. Explain Input/output Exceptions
Answer:
The java.io.Exceptions provides for system input and output through data streams, serialization and the file system.
Interface Summary
Sr.No. | Interface & Description |
1 | CharConversionException This is a base class for character conversion exceptions. |
2 | EOFException These are signals that an end of file or end of stream has been reached unexpectedly during input. |
3 | FileNotFoundException These are the signals that an attempt to open the file denoted by a specified pathname has failed. |
4 | InterruptedIOException This is signals that an I/O operation has been interrupted. |
5 | InvalidClassException This is thrown when the Serialization runtime detects one of the following problems with a Class. |
6 | InvalidObjectException This indicates that one or more deserialized objects failed validation tests. |
7 | IOException These are the signals that an I/O exception of some sort has occurred. |
8 | NotActiveException This is thrown when serialization or deserialization is not active. |
9 | NotSerializableException This is thrown when an instance is required to have a Serializable interface. |
10 | ObjectStreamException This is a superclass of all exceptions specific to Object Stream classes. |
11 | OptionalDataException This is an exception indicating the failure of an object read operation due to unread primitive data, or the end of data belonging to a serialized object in the stream. |
12 | StreamCorruptedException This is thrown when control information that was read from an object stream violates internal consistency checks. |
13 | SyncFailedException These are the signals that a sync operation has failed. |
14 | UnsupportedEncodingException This character encoding is not supported. |
15 | UTFDataFormatException This are signals that a malformed string in modified UTF-8 format has been read in a data input stream or by any class that implements the data input interface. |
16 | WriteAbortedException This are signals that one of the ObjectStreamExceptions was thrown during a write operation. |
10. Explain Handling Primitive Data Types
Answer: Not everything in Java is an object. There is a special group of data types (also known as primitive types) that will be used quite often in programming. For performance reasons, the designers of the Java language decided to include these primitive types. Java determines the size of each primitive type. These sizes do not change from one operating system to another. This is one of the key features of the language that makes Java so portable. Java defines eight primitive types of data: byte, short, int, long, char, float, double, and boolean. The primitive types are also commonly referred to as simple types which can be put in four groups
- Integers: This group includes byte, short, int, and long, which are for whole-valued signed numbers.
- Floating-point numbers: This group includes float and double, which represent numbers with fractional precision.
- Characters: This group includes char, which represents symbols in a character set, like letters and numbers.
- Boolean: This group includes boolean, which is a special type for representing true/false values.
Let’s discuss each in details:
Byte
The smallest integer type is byte. It has a minimum value of -128 and a maximum value of 127 (inclusive). The byte data type can be useful for saving memory in large arrays, where the memory savings actually matters. Byte variables are declared by use of the byte keyword. For example, the following declares and initialize byte variables called b:
Byte b =100;
Short:
The short data type is a 16-bit signed two's complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive). As with byte, the same guidelines apply: you can use a short to save memory in large arrays, in situations where the memory savings actually matters. Following example declares and initialize short variable called s:
Short s =123;
Int:
The most commonly used integer type is int. It is a signed 32-bit type that has a range from –2,147,483,648 to 2,147,483,647. In addition to other uses, variables of type int are commonly employed to control loops and to index arrays. This data type will most likely be large enough for the numbers your program will use, but if you need a wider range of values, use long instead.
Int v = 123543;
Intcalc = -9876345;
Long:
Long is a signed 64-bit type and is useful for those occasions where an int type is not large enough to hold the desired value. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Use of this data type might be in banking application when large amount is to be calculated and stored.
Long amountVal = 1234567891;
Float:
Floating-point numbers, also known as real numbers, are used when evaluating expressions that require fractional precision. For example interest rate calculation or calculating square root. The float data type is a single-precision 32-bit IEEE 754 floating point. As with the recommendations for byte and short, use a float (instead of double) if you need to save memory in large arrays of floating point numbers. The type float specifies a single-precision value that uses 32 bits of storage. Single precision is faster on some processors and takes half as much space as double precision. The declaration and initialization syntax for float variables given below, please note “f” after value initialization.
Float intrestRate = 12.25f;
Double:
Double precision, as denoted by the double keyword, uses 64 bits to store a value. Double precision is actually faster than single precision on some modern processors that have been optimized for high-speed mathematical calculations. All transcendental math functions, such as sin( ), cos( ), and sqrt( ), return double values. The declaration and initialization syntax for double variables given below, please note “d” after value initialization.
Double sineVal = 12345.234d;
Boolean:
The boolean data type has only two possible values: true and false. Use this data type for simple flags that track true/false conditions. This is the type returned by all relational operators, as in the case of a < b. Boolean is also the type required by the conditional expressions that govern the control statements such as if or while.
Boolean flag = true;
Booleanval = false;
Char:
In Java, the data type used to store characters is char. The char data type is a single 16-bit Unicode character. It has a minimum value of '\u0000' (or 0) and a maximum value of '\uffff' (or 65,535 inclusive). There are no negative chars.
Char ch1 = 88; // code for X
Char ch2 = 'Y';
Primitive Variables can be of two types
(1) Class level (instance) variable:
It's not mandatory to initialize Class level (instance) variable. If we do not initialize instance variable compiler will assign default value to it. Generally speaking, this default will be zero or null, depending on the data type. Relying on such default values, however, is generally considered bad coding practice.The following chart summarizes the default values for the above data types.
Primitive Data Type | Default Value 3.6 |
Byte | 0 |
Short | 0 |
Int | 0 |
Long | 0L |
Float | 0.0f |
Double | 0.0d |
Char | '\u0000' |
Boolean | False |
(2) Method local variable:
Method local variables have to be initialized before using it. The compiler never assigns a default value to an un-initialized local variable. If you cannot initialize your local variable where it is declared, make sure to assign it a value before you attempt to use it. Accessing an un-initialized local variable will result in a compile-time error.
Let’s See simple java program which declares, initialize and print all of primitive types.
Java class PrimitiveDemo as below:
Package primitive;
Public class PrimitiveDemo {
Public static void main(String[] args) {
Byte b =100;
Short s =123;
Int v = 123543;
Intcalc = -9876345;
LongamountVal = 1234567891;
FloatintrestRate = 12.25f;
DoublesineVal = 12345.234d;
Boolean flag = true;
Booleanval = false;
Char ch1 = 88; // code for X
Char ch2 = 'Y';
System.out.println("byte Value = "+ b);
System.out.println("short Value = "+ s);
System.out.println("int Value = "+ v);
System.out.println("int second Value = "+ calc);
System.out.println("long Value = "+ amountVal);
System.out.println("float Value = "+ intrestRate);
System.out.println("double Value = "+ sineVal);
System.out.println("boolean Value = "+ flag);
System.out.println("boolean Value = "+ val);
System.out.println("char Value = "+ ch1);
System.out.println("char Value = "+ ch2);
}
}
Copy
Output of above PrimitiveDemo class as below:
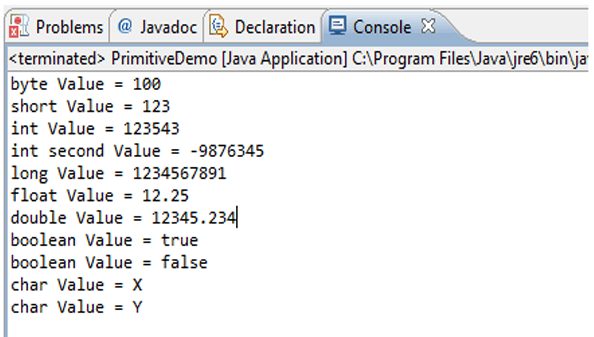
Summary of Data Types
Primitive Type | Size | Minimum Value | Maximum Value | Wrapper Type |
Char | 16-bit | Unicode 0 | Unicode 216-1 | Character |
Byte | 8-bit | -128 | +127 | Byte |
Short | 16-bit | -215 | +215-1 | Short |
Int | 32-bit | -231 | +231-1 | Integer |
Long | 64-bit | -263 | +263-1 | Long |
Float | 32-bit | Approx range 1.4e-045 to 3.4e+038 | Float | |
Double | 64-bit | Approx range 4.9e-324 to 1.8e+308 | Double | |
Boolean | 1-bit | True or false | Boolean |