UNIT 3
Object & Classes
- Explain object and classes
Since C++ is an object-oriented language, program is designed using objects and classes in C++.
Object
In C++, Object is a real world entity, for example, chair, car, pen, mobile, laptop etc.
In other words, object is an entity that has state and behavior. Here, state means data and behavior means functionality.
Object is a runtime entity, it is created at runtime.
Object is an instance of a class. All the members of the class can be accessed through object.
Let's see an example to create object of student class using s1 as the reference variable.
- Student s1; //creating an object of Student
In this example, Student is the type and s1 is the reference variable that refers to the instance of Student class.
Class
In C++, object is a group of similar objects. It is a template from which objects are created. It can have fields, methods, constructors etc.
Let's see an example of C++ class that has three fields only.
- Class Student
- {
- Public:
- Int id; //field or data member
- Float salary; //field or data member
- String name;//field or data member
- }
Object and Class Example
Let's see an example of class that has two fields: id and name. It creates instance of the class, initializes the object and prints the object value.
- #include <iostream>
- Using namespace std;
- Class Student {
- Public:
- Int id;//data member (also instance variable)
- String name;//data member(also instance variable)
- };
- Int main() {
- Student s1; //creating an object of Student
- s1.id = 201;
- s1.name = "Sonoo Jaiswal";
- Cout<<s1.id<<endl;
- Cout<<s1.name<<endl;
- Return 0;
- }
Output:
201
Sonoo Jaiswal
2. Class Example: Initialize and Display data through method
Let's see another example of C++ class where we are initializing and displaying object through method.
- #include <iostream>
- Using namespace std;
- Class Student {
- Public:
- Int id;//data member (also instance variable)
- String name;//data member(also instance variable)
- Void insert(int i, string n)
- {
- Id = i;
- Name = n;
- }
- Void display()
- {
- Cout<<id<<" "<<name<<endl;
- }
- };
- Int main(void) {
- Student s1; //creating an object of Student
- Student s2; //creating an object of Student
- s1.insert(201, "Sonoo");
- s2.insert(202, "Nakul");
- s1.display();
- s2.display();
- Return 0;
- }
Output:
201 Sonoo
202 Nakul
3. Class Example: Store and Display Employee Information
Let's see another example of C++ class where we are storing and displaying employee information using method.
- #include <iostream>
- Using namespace std;
- Class Employee {
- Public:
- Int id;//data member (also instance variable)
- String name;//data member(also instance variable)
- Float salary;
- Void insert(int i, string n, float s)
- {
- Id = i;
- Name = n;
- Salary = s;
- }
- Void display()
- {
- Cout<<id<<" "<<name<<" "<<salary<<endl;
- }
- };
- Int main(void) {
- Employee e1; //creating an object of Employee
- Employee e2; //creating an object of Employee
- e1.insert(201, "Sonoo",990000);
- e2.insert(202, "Nakul", 29000);
- e1.display();
- e2.display();
- Return 0;
- }
Output:
201 Sonoo 990000
202 Nakul 29000
4. Explain Scope Resolution Operator
An operator is simply a symbol that is used to perform operations. There can be many types of operations like arithmetic, logical, bitwise etc.
There are following types of operators to perform different types of operations in C language.
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operator
- Unary operator
- Ternary or Conditional Operator
- Misc Operator
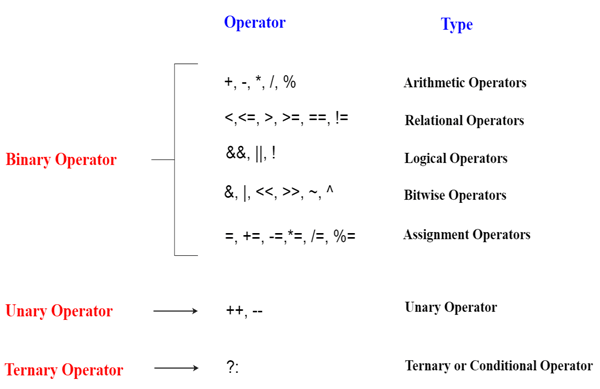
Precedence of Operators
The precedence of operator species that which operator will be evaluated first and next. The associativity specifies the operators direction to be evaluated, it may be left to right or right to left.
Let's understand the precedence by the example given below:
- Int data=5+10*10;
The "data" variable will contain 105 because * (multiplicative operator) is evaluated before + (additive operator).
The precedence and associativity of C++ operators is given below:
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* & sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Right to left |
Shift | << >> | Left to right |
Relational | < <= > >= | Left to right |
Equality | == !=/td> | Right to left |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Right to left |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
5. Explain constructor in detail
Constructors
- It is a member function of a class with same name as that of a class.
Class example
{
Int x,y;
Public:
Example() //constructor, same name as that of class
{
}
Void display()
{
:
}
};
- It is used to initialize data members of class.
Class example
{
Int x,y;
Public:
Example() //constructor initializing data members of class
{
x=10;
y=20;
}
Void display()
{
:
}
};
- It is always declared under public section.
Class example
{
Private:
Int x,y;
Example() // error, constructor declared under private section
{
x=10;
y=20;
}
:
:
};
- It does not have return type and hence cannot return any value
Class example
{
Int x, y;
Public:
Void example() // error, constructor does not have return type
{
x=10;
y=20;
}
:
:
};
- It is invoked automatically when object of class is created and initialize all data members of a class.
Class example
{
Int x, y;
Public:
Example() // constructor
{
x=10;
y=20;
}
Void display()
{
Cout<<x<<y;
}
};
Int main()
{
Example obj; //invokes constructor and initialize data
Member x and y to 10 and 20 respectively.
Obj.display();
Return 0 ;
}
- It cannot be declared as static, const and virtual.
- It can be defined inside a class or outside the class. While defining it outside the class scope resolution operator is used.
Class example
{
Int x, y;
Public:
Example(); // constructor declared
:
:
};
Example::example();// constructor defined outside class
{
x=10;
y=20;
}
6. What is the type of constructor?
Types of constructors with syntax
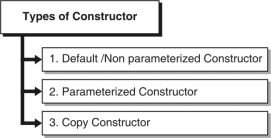
Fig: Types of Constructor
Default /Non parameterized Constructor
- A constructor with no parameter is default constructor.
- If constructor is not defined in program then compiler supplies default constructor.
Class Volume
{
Int x;
Public:
Volume () // default constructor
{
x=10;
}
Void display()
{
Cout<<x*x*x;
}
};
Void main()
{
Volume Obj; //invokes default constructor
Obj.display();
}
Parameterized Constructor
A constructor that receives parameters is parameterized constructor.
Class Volume {
Int x;
Public:
Volume ( int a) // parameterized constructor
{
x=a;
}
Void display()
{
Cout<<x*x*x;
}
};
Int main()
{
Volume obj(10); //invokes parameterized constructor
And pass argument 10 to it.
Obj.display();
}
Copy Constructor
Copy constructor initializes an object using values of another object passed to it as a parameter.
Class Volume
{
Int x;
Public:
Volume ( int & P) // copy constructor
{
x=P.x;//copies value
}
Void display()
{
Cout<<x*x*x;
}
};
Int main()
{
Volume obj1; //invokes default constructor
Volume obj2(obj1);//calls copy constructor
Obj2.display();
}
Constructor Overloading
Constructors can be overloaded just like member functions. Thus we can have multiple constructors in a class.
Write a C++ program to find volume of cube, cylinder.
Solution :
#include<iostream>
Using namespace std ;
Class Volume//Defines class Volume
{
Int x,y,z;
Public:
Volume ( int a) // Step 1Constructor with one argument
{
x=a;
}
Volume ( int a, int b) // Step 2 Constructor with two arguments
{
x=a;
y=b;
}
Void displaycube();
Void displaycylinder();
};
Void Volume::displaycube()
// Step 3 Function to calculate volume of cube
{
Cout<<x*x*x;
}
Void Volume::displaycylinder()
//Step 4 Function to calculate volume of cylinder
{
Cout<<3.14*x*x*y;
}
Int main()
{
Volume obj1(10);
// Step 5Calls constructor with one argument.
Volume obj2(10,5);
// Step 6 Calls constructor with two arguments.
Obj1. Displaycube ();//Step 7
Obj2. Displaycylinder ();
Return 0 ;
}
Explanation
This program calculates volume of cube and cylinder. For this we use two constructors in a class. One constructor is to initialize data member for cube and other to initialize data members for cylinder.
Step 1 :Defines constructor with one argument. Here x stands for side of a cube.
Volume ( int a) { x=a; } | //Constructor with one argument
|
Step 2 :Defines constructor with two arguments. Here x and y specifies radius and height.
Volume ( int a, int b) { x=a; y=b; } | //Constructor with two arguments
|
Step 3 :Defines Function to calculate volume of cube.
Void Volume::displaycube() { Cout<<x*x*x; } | //Function to calculate volume of cube
|
Step 4 :Defines Function to calculate volume of cylinder
Void Volume::displaycylinder() { Cout<<3.14*x*x*y; } | //Function to calculate volume of cylinder
|
Step 5 :Here we want to initialize side of cube so we pass only one argument to invoke constructor with one argument.
Volume obj1(10); |
Step 6 :Here we want to initialize radius and height of cylinder so we pass two arguments to invoke constructor with two arguments.
Volume obj2(10,5); |
Step 7 :Calls functions to calculate volume of a cube and cylinder.
Obj1. Displaycube (); Obj2. Displaycylinder (); |
7. Explain destructor
- It is a special member function that is called when lifetime of an object ends.
- Destructor is used to free the resources occupied by an object.
- It is called automatically when the object is no longer needed.
- It has same name as that of class preceded by tilde(~).
Class example
{
Public:
~example();//destructor
};
- It is always declared or defined under public section.
- It neither returns any value nor takes any argument.
- It cannot be declared as static const and volatile.
8. What is friend function?
A friend function of a class is defined outside that class' scope but it has the right to access all private and protected members of the class. Even though the prototypes for friend functions appear in the class definition, friends are not member functions.
A friend can be a function, function template, or member function, or a class or class template, in which case the entire class and all of its members are friends.
To declare a function as a friend of a class, precede the function prototype in the class definition with keyword friend as follows −
Class Box {
double width;
public:
double length;
friend void printWidth( Box box );
void setWidth( double wid );
};
To declare all member functions of class ClassTwo as friends of class ClassOne, place a following declaration in the definition of class ClassOne −
Friend class ClassTwo;
Consider the following program –
#include <iostream>
Using namespace std;
Class Box {
double width;
public:
friend void printWidth( Box box );
void setWidth( double wid );
};
// Member function definition
Void Box::setWidth( double wid ) {
width = wid;
}
// Note: printWidth() is not a member function of any class.
Void printWidth( Box box ) {
/* Because printWidth() is a friend of Box, it can
directly access any member of this class */
cout << "Width of box : " << box.width <<endl;
}
// Main function for the program
Int main() {
Box box;
// set box width without member function
box.setWidth(10.0);
// Use friend function to print the wdith.
printWidth( box );
return 0;
}
When the above code is compiled and executed, it produces the following result −
Width of box : 10
9. What is inheritance?
In the above example we can say that “a Manager is-a- type of Employee”, “a Teamleader is-a- type of Employee” and “a Teammember is-a- type of Employee”, etc.
This shows that the manager ,Team Leader, Team member and Employee share some common attributes and behaviors.
The concept of inheritance have parent class which act as generic super class or an abstract class having the common attributes and methods inherited to child class or all sub classes.
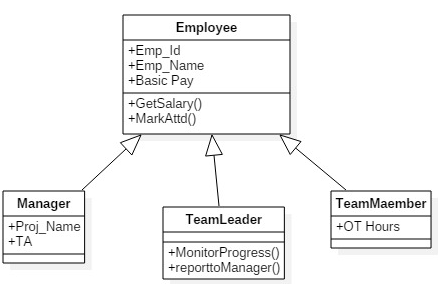
Class Manager, TeamLeader and class TeamMember inherits all common attributes and functions written in Employee class. The sub classes can have their own aatributes and function in addition to the super class attributes and functions.
10. What is polymorphism?
The word polymorphism means having many forms. Typically, polymorphism occurs when there is a hierarchy of classes and they are related by inheritance.
C++ polymorphism means that a call to a member function will cause a different function to be executed depending on the type of object that invokes the function.
Consider the following example where a base class has been derived by other two classes −
#include <iostream>
Using namespace std;
Class Shape {
protected:
int width, height;
public:
Shape( int a = 0, int b = 0){
width = a;
height = b;
}
int area() {
cout << "Parent class area :" <<endl;
return 0;
}
};
Class Rectangle: public Shape {
public:
Rectangle( int a = 0, int b = 0):Shape(a, b) { }
int area () {
cout << "Rectangle class area :" <<endl;
return (width * height);
}
};
Class Triangle: public Shape {
public:
Triangle( int a = 0, int b = 0):Shape(a, b) { }
int area () {
cout << "Triangle class area :" <<endl;
return (width * height / 2);
}
};
// Main function for the program
Int main() {
Shape *shape;
Rectangle rec(10,7);
Triangle tri(10,5);
// store the address of Rectangle
shape = &rec;
// call rectangle area.
shape->area();
// store the address of Triangle
shape = &tri;
// call triangle area.
shape->area();
return 0;
}
When the above code is compiled and executed, it produces the following result −
Parent class area :
Parent class area :