Unit - 3
Interfaces and Packages
Q1) Introduce interface?
A1) In Java, an interface is a reference type. It's comparable to a class. It consists of a set of abstract methods. When a class implements an interface, it inherits the interface's abstract methods.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods. Method bodies are only available for default and static methods.
The process of creating an interface is identical to that of creating a class. A class, on the other hand, describes an object's features and behaviors. An interface, on the other hand, contains the behaviors that a class implements.
Unless the interface's implementation class is abstract, all of the interface's methods must be declared in the class.
In the following ways, an interface is analogous to a class:
● Any number of methods can be included in an interface.
● An interface is written in a file with the extension.java, with the interface's name matching the file's name.
● A.class file contains the byte code for an interface.
● Packages have interfaces, and the bytecode file for each must be in the same directory structure as the package name.
Why do we use interfaces?
There are three primary reasons to utilize an interface. Below is a list of them.
● It's a technique for achieving abstraction.
● We can offer various inheritance functionality via an interface.
● It's useful for achieving loose coupling.
Q2) How to declare an interface?
A2) The interface keyword is used to declare an interface. All methods in an interface are declared with an empty body, and all fields are public, static, and final by default. A class that implements an interface is required to implement all of the interface's functions.
Syntax
Interface <interface_name>{
// declare constant fields
// declare methods that abstract
// by default.
}
Q3) How to implement an interface?
A3) When a class implements an interface, it's like the class signing a contract, agreeing to perform the interface's defined actions. If a class doesn't perform all of the interface's actions, it must declare itself abstract.
To implement an interface, a class utilizes the implements keyword. Following the extends component of the declaration, the implements keyword appears in the class declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
Public void eat() {
System.out.println("Mammal eats");
}
Public void travel() {
System.out.println("Mammal travels");
}
Public int noOfLegs() {
Return 0;
}
Public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
Output
Mammal eats
Mammal travels
Q4) How to access implementations through interface references?
A4) Variables can be declared as object references that use an interface instead of a class.
Such a variable can refer to any instance that implements the stated interface.
When you use one of these references to invoke a method, the right version is used based on the actual instance of the interface being referred to.
This is one of the most important characteristics of interfaces. The method to be executed is dynamically looked up at runtime, allowing classes to be built after the code that calls methods on them.
This is analogous to accessing a subclass object using a superclass reference.
The callback() function is invoked in the following example using an interface reference variable:
Interface Callback {
Void callback(int param);
}
Class Client implements Callback {
// Implement Callback's interface
Public void callback(int p) {
System.out.println("callback called with " + p);
}
Void aa() {
System.out.println("hi.");
}
}
Public class Main{
Public static void main(String args[]) {
Callback c = new Client();
c.callback(42);
}
}
The following is the program's output:
Despite the fact that the variable c is of the interface type Callback, it has been assigned an instance of Client.
The callback() method can be used using c, but it cannot access any other members of the Client class.
Only the methods declared by the interface declaration are known to an interface reference variable.
As a result, because aa() is defined by Client but not Callback, c could not be used to access it.
Q5) How to extend the interface?
A5) In the same manner that a class can extend another class, an interface can extend another interface. When you use the extends keyword to extend an interface, the new interface inherits the parent interface's methods.
Hockey and Football interfaces are added to the Sports interface below.
Example
// Filename: Sports.java
Public interface Sports {
Public void setHomeTeam(String name);
Public void setVisitingTeam(String name);
}
// Filename: Football.java
Public interface Football extends Sports {
Public void homeTeamScored(int points);
Public void visitingTeamScored(int points);
Public void endOfQuarter(int quarter);
}
// Filename: Hockey.java
Public interface Hockey extends Sports {
Public void homeGoalScored();
Public void visitingGoalScored();
Public void endOfPeriod(int period);
Public void overtimePeriod(int ot);
}
Hockey has four methods, but it inherits two from Sports; as a result, a class that implements Hockey must implement all six methods. Similarly, a class that implements Football must specify the three Football methods as well as the two Sports methods.
Q6) What is the package?
A6) A java package is a group of similar types of classes, interfaces and sub-packages.
Package in java can be categorized in two form, built-in package and user-defined package.
There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
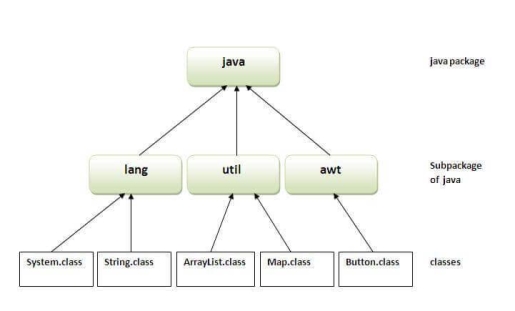
Fig - Package
Simple example of java package
The package keyword is used to create a package in java.
- //save as Simple.java
- Package mypack;
- Public class Simple{
- Public static void main(String args[]){
- System.out.println("Welcome to package");
- }
- }
How to compile java package
If you are not using any IDE, you need to follow the syntax given below:
- Javac -d directory javafilename
For example
- Javac -d . Simple.java
The -d switch specifies the destination where to put the generated class file. You can use any directory name like /home (in case of Linux), d:/abc (in case of windows) etc. If you want to keep the package within the same directory, you can use . (dot).
How to run java package program
You need to use fully qualified name e.g. Mypack.Simple etc to run the class.
To Compile: javac -d . Simple.java
To Run: java mypack.Simple
Output: Welcome to package
The -d is a switch that tells the compiler where to put the class file i.e. it represents destination. The . Represents the current folder.
Q7) Write the advantages of package?
A7) Advantage of Java Package
1) Java package is used to categorize the classes and interfaces so that they can be easily maintained.
2) Java package provides access protection.
3) Java package removes naming collision.
Q8) How to access a package from another package?
A8) There are three ways to access the package from outside the package.
- Import package.*;
- Import package.classname;
- Fully qualified name.
1) Using packagename.*
If you use package.* then all the classes and interfaces of this package will be accessible but not subpackages.
The import keyword is used to make the classes and interface of another package accessible to the current package.
Example of package that import the packagename.*
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Import pack.*;
- Class B{
- Public static void main(String args[]){
- A obj = new A();
- Obj.msg();
- }
- }
Output:
Hello
2) Using packagename.classname
If you import package.classname then only declared class of this package will be accessible.
Example of package by import package.classname
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Import pack.A;
- Class B{
- Public static void main(String args[]){
- A obj = new A();
- Obj.msg();
- }
- }
Output:
Hello
3) Using fully qualified name
If you use fully qualified name then only declared class of this package will be accessible. Now there is no need to import. But you need to use fully qualified name every time when you are accessing the class or interface.
It is generally used when two packages have same class name e.g. Java.util and java.sql packages contain Date class.
Example of package by import fully qualified name
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Class B{
- Public static void main(String args[]){
- Pack.A obj = new pack.A();//using fully qualified name
- Obj.msg();
- }
- }
Output:
Hello
Q9) Define subpackage with example?
A9) Subpackage in java
Package inside the package is called the subpackage. It should be created to categorize the package further.
Let's take an example, Sun Microsystem has defined a package named java that contains many classes like System, String, Reader, Writer, Socket etc. These classes represent a particular group e.g. Reader and Writer classes are for Input/Output operation, Socket and ServerSocket classes are for networking etc and so on. So, Sun has subcategorized the java package into subpackages such as lang, net, io etc. and put the Input/Output related classes in io package, Server and ServerSocket classes in net packages and so on.
The standard of defining a package is domain.company.package e.g. Com.javatpoint.bean or org.sssit.dao.
Example of Subpackage
- Package com.javatpoint.core;
- Class Simple{
- Public static void main(String args[]){
- System.out.println("Hello subpackage");
- }
- }
To Compile: javac -d . Simple.java
To Run: java com.javatpoint.core.Simple
Output: Hello subpackage
Q10) How to send the class file to another directory or drive?
A10) There is a scenario where I want to put the class file of A.java source file in classes folder of c: drive. For example:
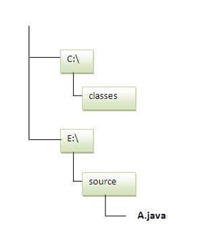
- //save as Simple.java
- Package mypack;
- Public class Simple{
- Public static void main(String args[]){
- System.out.println("Welcome to package");
- }
- }
To Compile:
e:\sources> javac -d c:\classes Simple.java
To Run:
To run this program from e:\source directory, you need to set classpath of the directory where the class file resides.
e:\sources> set classpath=c:\classes;.;
e:\sources> java mypack.Simple
Q11) What do you mean by access protection?
A11) Access protection
The scope of the class and its members is defined by access modifiers (data and methods). Private members, for example, can be accessed by other members of the same class (methods). Java has several degrees of security that allow members (variables and methods) within classes, subclasses, and packages to be visible.
Packages are used to encapsulate information and act as containers for classes and subpackages. Data and methods are contained in classes. When it comes to the visibility of class members between classes and packages, Java offers four options:
● Subclasses in the same package
● Non-subclasses in the same package
● Subclasses in different packages
● Classes that are neither in the same package nor subclasses
These categories require a variety of access methods, which are provided by the three main access modifiers private, public, and protected.
Simply remember that private can only be seen within its class, public can be seen from anyone, and protected can only be accessed in subclasses within the hierarchy.
There are just two access modifiers that a class can have: default and public. If a class has default access, it can only be accessed by other code inside the same package. However, if the class is public, it can be accessed from anywhere by any code.
Q12) What do you mean by classpath?
A12) CLASSPATH is an environment variable which is used by Application ClassLoader to locate and load the .class files. The CLASSPATH defines the path, to find third-party and user-defined classes that are not extensions or part of Java platform. Include all the directories which contain .class files and JAR files when setting the CLASSPATH.
You need to set the CLASSPATH if:
● You need to load a class that is not present in the current directory or any sub-directories.
● You need to load a class that is not in a location specified by the extensions mechanism.
The CLASSPATH depends on what you are setting the CLASSPATH. The CLASSPATH has a directory name or file name at the end. The following points describe what should be the end of the CLASSPATH.
● If a JAR or zip, the file contains class files, the CLASSPATH end with the name of the zip or JAR file.
● If class files placed in an unnamed package, the CLASSPATH ends with the directory that contains the class files.
● If class files placed in a named package, the CLASSPATH ends with the directory that contains the root package in the full package name, that is the first package in the full package name.
The default value of CLASSPATH is a dot (.). It means the only current directory searched. The default value of CLASSPATH overrides when you set the CLASSPATH variable or using the -classpath command (for short -cp). Put a dot (.) in the new setting if you want to include the current directory in the search path.
If CLASSPATH finds a class file which is present in the current directory, then it will load the class and use it, irrespective of the same name class presents in another directory which is also included in the CLASSPATH.
If you want to set multiple classpaths, then you need to separate each CLASSPATH by a semicolon (;).
The third-party applications (MySQL and Oracle) that use the JVM can modify the CLASSPATH environment variable to include the libraries they use. The classes can be stored in directories or archives files. The classes of the Java platform are stored in rt.jar.
There are two ways to ways to set CLASSPATH: through Command Prompt or by setting Environment Variable.
Q13) How to set the classpath of the mysql database?
A13) Let's see how to set CLASSPATH of MySQL database:
Step 1: Click on the Windows button and choose Control Panel. Select System.
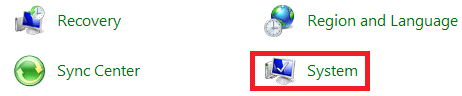
Step 2: Click on Advanced System Settings.
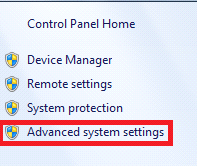
Step 3: A dialog box will open. Click on Environment Variables.
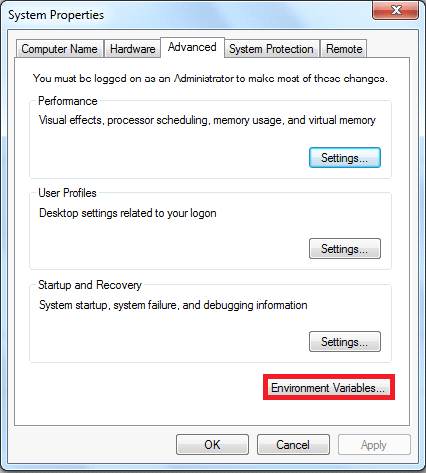
Step 4: If the CLASSPATH already exists in System Variables, click on the Edit button then put a semicolon (;) at the end. Paste the Path of MySQL-Connector Java.jar file.
If the CLASSPATH doesn't exist in System Variables, then click on the New button and type Variable name as CLASSPATH and Variable value as C:\Program Files\Java\jre1.8\MySQL-Connector Java.jar;.;
Remember: Put ;.; at the end of the CLASSPATH.
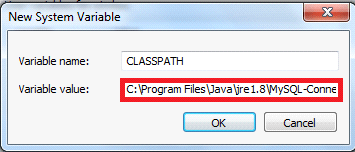
Q14) Write the difference between path and classpath?
A14) Difference between PATH and CLASSPATH
PATH | CLASSPATH |
PATH is an environment variable. | CLASSPATH is also an environment variable. |
It is used by the operating system to find the executable files (.exe). | It is used by Application ClassLoader to locate the .class file. |
You are required to include the directory which contains .exe files. | You are required to include all the directories which contain .class and JAR files. |
PATH environment variable once set, cannot be overridden. | The CLASSPATH environment variable can be overridden by using the command line option -cp or -CLASSPATH to both javac and java command. |
Q15) How to import a package?
A15) Import a Package
There are many packages to choose from. In the previous example, we used the Scanner class from the java.util package. This package also contains date and time facilities, random-number generator and other utility classes.
To import a whole package, end the sentence with an asterisk sign (*). The following example will import ALL the classes in the java.util package:
Example
Import java.util.*;
User-defined Packages
To create your own package, you need to understand that Java uses a file system directory to store them. Just like folders on your computer:
Example
└── root
└── mypack
└── MyPackageClass.java
To create a package, use the package keyword:
MyPackageClass.java
Package mypack;
Class MyPackageClass {
Public static void main(String[] args) {
System.out.println("This is my package!");
}
}
Save the file as MyPackageClass.java, and compile it:
C:\Users\Your Name>javac MyPackageClass.java
Then compile the package:
C:\Users\Your Name>javac -d . MyPackageClass.java
This forces the compiler to create the "mypack" package.
The -d keyword specifies the destination for where to save the class file. You can use any directory name, like c:/user (windows), or, if you want to keep the package within the same directory, you can use the dot sign ".", like in the example above.
Note: The package name should be written in lower case to avoid conflict with class names.
When we compiled the package in the example above, a new folder was created, called "mypack".
To run the MyPackageClass.java file, write the following:
C:\Users\Your Name>java mypack.MyPackageClass
The output will be:
This is my package!
Q16) Write a difference between package and interface?
A16) Key difference between Package and Interface
● An interface is a collection of abstract methods, whereas a package is a collection of classes and interfaces.
● A keyword package is used to construct a package, whereas a keyword interface is used to build an interface.
● If a package's class or interface is to be utilized, the package must be imported, and the interface must be implemented.
Q17) Write a sample program to implement an interface in Java?
A17) Program
Interface Results
{
Final static float pi = 3.14f;
Float areaOf(float l, float b);
}
Class Rectangle implements Results
{
Public float areaOf(float l, float b)
{
Return (l * b);
}
}
Class Square implements Results
{
Public float areaOf(float l, float b)
{
Return (l * l);
}
}
Class Circle implements Results
{
Public float areaOf(float r, float b)
{
Return (pi * r * r);
}
}
Public class InterfaceDemo
{
Public static void main(String args[])
{
Rectangle rect = new Rectangle();
Square square = new Square();
Circle circle = new Circle();
System.out.println("Area of Rectangle: "+rect.areaOf(20.3f, 28.7f));
System.out.println("Are of square: "+square.areaOf(10.0f, 10.0f));
System.out.println("Area of Circle: "+circle.areaOf(5.2f, 0));
}
}
Output:
Area of Rectangle: 582.61
Are of square: 100.0
Area of Circle: 84.905594
Unit - 3
Unit - 3
Interfaces and Packages
Q1) Introduce interface?
A1) In Java, an interface is a reference type. It's comparable to a class. It consists of a set of abstract methods. When a class implements an interface, it inherits the interface's abstract methods.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods. Method bodies are only available for default and static methods.
The process of creating an interface is identical to that of creating a class. A class, on the other hand, describes an object's features and behaviors. An interface, on the other hand, contains the behaviors that a class implements.
Unless the interface's implementation class is abstract, all of the interface's methods must be declared in the class.
In the following ways, an interface is analogous to a class:
● Any number of methods can be included in an interface.
● An interface is written in a file with the extension.java, with the interface's name matching the file's name.
● A.class file contains the byte code for an interface.
● Packages have interfaces, and the bytecode file for each must be in the same directory structure as the package name.
Why do we use interfaces?
There are three primary reasons to utilize an interface. Below is a list of them.
● It's a technique for achieving abstraction.
● We can offer various inheritance functionality via an interface.
● It's useful for achieving loose coupling.
Q2) How to declare an interface?
A2) The interface keyword is used to declare an interface. All methods in an interface are declared with an empty body, and all fields are public, static, and final by default. A class that implements an interface is required to implement all of the interface's functions.
Syntax
Interface <interface_name>{
// declare constant fields
// declare methods that abstract
// by default.
}
Q3) How to implement an interface?
A3) When a class implements an interface, it's like the class signing a contract, agreeing to perform the interface's defined actions. If a class doesn't perform all of the interface's actions, it must declare itself abstract.
To implement an interface, a class utilizes the implements keyword. Following the extends component of the declaration, the implements keyword appears in the class declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
Public void eat() {
System.out.println("Mammal eats");
}
Public void travel() {
System.out.println("Mammal travels");
}
Public int noOfLegs() {
Return 0;
}
Public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
Output
Mammal eats
Mammal travels
Q4) How to access implementations through interface references?
A4) Variables can be declared as object references that use an interface instead of a class.
Such a variable can refer to any instance that implements the stated interface.
When you use one of these references to invoke a method, the right version is used based on the actual instance of the interface being referred to.
This is one of the most important characteristics of interfaces. The method to be executed is dynamically looked up at runtime, allowing classes to be built after the code that calls methods on them.
This is analogous to accessing a subclass object using a superclass reference.
The callback() function is invoked in the following example using an interface reference variable:
Interface Callback {
Void callback(int param);
}
Class Client implements Callback {
// Implement Callback's interface
Public void callback(int p) {
System.out.println("callback called with " + p);
}
Void aa() {
System.out.println("hi.");
}
}
Public class Main{
Public static void main(String args[]) {
Callback c = new Client();
c.callback(42);
}
}
The following is the program's output:
Despite the fact that the variable c is of the interface type Callback, it has been assigned an instance of Client.
The callback() method can be used using c, but it cannot access any other members of the Client class.
Only the methods declared by the interface declaration are known to an interface reference variable.
As a result, because aa() is defined by Client but not Callback, c could not be used to access it.
Q5) How to extend the interface?
A5) In the same manner that a class can extend another class, an interface can extend another interface. When you use the extends keyword to extend an interface, the new interface inherits the parent interface's methods.
Hockey and Football interfaces are added to the Sports interface below.
Example
// Filename: Sports.java
Public interface Sports {
Public void setHomeTeam(String name);
Public void setVisitingTeam(String name);
}
// Filename: Football.java
Public interface Football extends Sports {
Public void homeTeamScored(int points);
Public void visitingTeamScored(int points);
Public void endOfQuarter(int quarter);
}
// Filename: Hockey.java
Public interface Hockey extends Sports {
Public void homeGoalScored();
Public void visitingGoalScored();
Public void endOfPeriod(int period);
Public void overtimePeriod(int ot);
}
Hockey has four methods, but it inherits two from Sports; as a result, a class that implements Hockey must implement all six methods. Similarly, a class that implements Football must specify the three Football methods as well as the two Sports methods.
Q6) What is the package?
A6) A java package is a group of similar types of classes, interfaces and sub-packages.
Package in java can be categorized in two form, built-in package and user-defined package.
There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
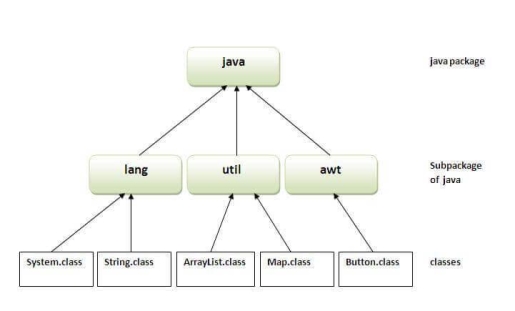
Fig - Package
Simple example of java package
The package keyword is used to create a package in java.
- //save as Simple.java
- Package mypack;
- Public class Simple{
- Public static void main(String args[]){
- System.out.println("Welcome to package");
- }
- }
How to compile java package
If you are not using any IDE, you need to follow the syntax given below:
- Javac -d directory javafilename
For example
- Javac -d . Simple.java
The -d switch specifies the destination where to put the generated class file. You can use any directory name like /home (in case of Linux), d:/abc (in case of windows) etc. If you want to keep the package within the same directory, you can use . (dot).
How to run java package program
You need to use fully qualified name e.g. Mypack.Simple etc to run the class.
To Compile: javac -d . Simple.java
To Run: java mypack.Simple
Output: Welcome to package
The -d is a switch that tells the compiler where to put the class file i.e. it represents destination. The . Represents the current folder.
Q7) Write the advantages of package?
A7) Advantage of Java Package
1) Java package is used to categorize the classes and interfaces so that they can be easily maintained.
2) Java package provides access protection.
3) Java package removes naming collision.
Q8) How to access a package from another package?
A8) There are three ways to access the package from outside the package.
- Import package.*;
- Import package.classname;
- Fully qualified name.
1) Using packagename.*
If you use package.* then all the classes and interfaces of this package will be accessible but not subpackages.
The import keyword is used to make the classes and interface of another package accessible to the current package.
Example of package that import the packagename.*
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Import pack.*;
- Class B{
- Public static void main(String args[]){
- A obj = new A();
- Obj.msg();
- }
- }
Output:
Hello
2) Using packagename.classname
If you import package.classname then only declared class of this package will be accessible.
Example of package by import package.classname
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Import pack.A;
- Class B{
- Public static void main(String args[]){
- A obj = new A();
- Obj.msg();
- }
- }
Output:
Hello
3) Using fully qualified name
If you use fully qualified name then only declared class of this package will be accessible. Now there is no need to import. But you need to use fully qualified name every time when you are accessing the class or interface.
It is generally used when two packages have same class name e.g. Java.util and java.sql packages contain Date class.
Example of package by import fully qualified name
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Class B{
- Public static void main(String args[]){
- Pack.A obj = new pack.A();//using fully qualified name
- Obj.msg();
- }
- }
Output:
Hello
Q9) Define subpackage with example?
A9) Subpackage in java
Package inside the package is called the subpackage. It should be created to categorize the package further.
Let's take an example, Sun Microsystem has defined a package named java that contains many classes like System, String, Reader, Writer, Socket etc. These classes represent a particular group e.g. Reader and Writer classes are for Input/Output operation, Socket and ServerSocket classes are for networking etc and so on. So, Sun has subcategorized the java package into subpackages such as lang, net, io etc. and put the Input/Output related classes in io package, Server and ServerSocket classes in net packages and so on.
The standard of defining a package is domain.company.package e.g. Com.javatpoint.bean or org.sssit.dao.
Example of Subpackage
- Package com.javatpoint.core;
- Class Simple{
- Public static void main(String args[]){
- System.out.println("Hello subpackage");
- }
- }
To Compile: javac -d . Simple.java
To Run: java com.javatpoint.core.Simple
Output: Hello subpackage
Q10) How to send the class file to another directory or drive?
A10) There is a scenario where I want to put the class file of A.java source file in classes folder of c: drive. For example:
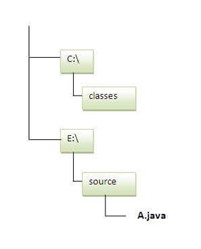
- //save as Simple.java
- Package mypack;
- Public class Simple{
- Public static void main(String args[]){
- System.out.println("Welcome to package");
- }
- }
To Compile:
e:\sources> javac -d c:\classes Simple.java
To Run:
To run this program from e:\source directory, you need to set classpath of the directory where the class file resides.
e:\sources> set classpath=c:\classes;.;
e:\sources> java mypack.Simple
Q11) What do you mean by access protection?
A11) Access protection
The scope of the class and its members is defined by access modifiers (data and methods). Private members, for example, can be accessed by other members of the same class (methods). Java has several degrees of security that allow members (variables and methods) within classes, subclasses, and packages to be visible.
Packages are used to encapsulate information and act as containers for classes and subpackages. Data and methods are contained in classes. When it comes to the visibility of class members between classes and packages, Java offers four options:
● Subclasses in the same package
● Non-subclasses in the same package
● Subclasses in different packages
● Classes that are neither in the same package nor subclasses
These categories require a variety of access methods, which are provided by the three main access modifiers private, public, and protected.
Simply remember that private can only be seen within its class, public can be seen from anyone, and protected can only be accessed in subclasses within the hierarchy.
There are just two access modifiers that a class can have: default and public. If a class has default access, it can only be accessed by other code inside the same package. However, if the class is public, it can be accessed from anywhere by any code.
Q12) What do you mean by classpath?
A12) CLASSPATH is an environment variable which is used by Application ClassLoader to locate and load the .class files. The CLASSPATH defines the path, to find third-party and user-defined classes that are not extensions or part of Java platform. Include all the directories which contain .class files and JAR files when setting the CLASSPATH.
You need to set the CLASSPATH if:
● You need to load a class that is not present in the current directory or any sub-directories.
● You need to load a class that is not in a location specified by the extensions mechanism.
The CLASSPATH depends on what you are setting the CLASSPATH. The CLASSPATH has a directory name or file name at the end. The following points describe what should be the end of the CLASSPATH.
● If a JAR or zip, the file contains class files, the CLASSPATH end with the name of the zip or JAR file.
● If class files placed in an unnamed package, the CLASSPATH ends with the directory that contains the class files.
● If class files placed in a named package, the CLASSPATH ends with the directory that contains the root package in the full package name, that is the first package in the full package name.
The default value of CLASSPATH is a dot (.). It means the only current directory searched. The default value of CLASSPATH overrides when you set the CLASSPATH variable or using the -classpath command (for short -cp). Put a dot (.) in the new setting if you want to include the current directory in the search path.
If CLASSPATH finds a class file which is present in the current directory, then it will load the class and use it, irrespective of the same name class presents in another directory which is also included in the CLASSPATH.
If you want to set multiple classpaths, then you need to separate each CLASSPATH by a semicolon (;).
The third-party applications (MySQL and Oracle) that use the JVM can modify the CLASSPATH environment variable to include the libraries they use. The classes can be stored in directories or archives files. The classes of the Java platform are stored in rt.jar.
There are two ways to ways to set CLASSPATH: through Command Prompt or by setting Environment Variable.
Q13) How to set the classpath of the mysql database?
A13) Let's see how to set CLASSPATH of MySQL database:
Step 1: Click on the Windows button and choose Control Panel. Select System.
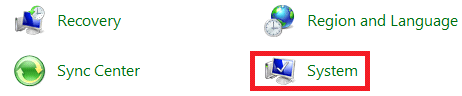
Step 2: Click on Advanced System Settings.
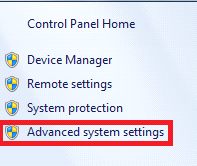
Step 3: A dialog box will open. Click on Environment Variables.
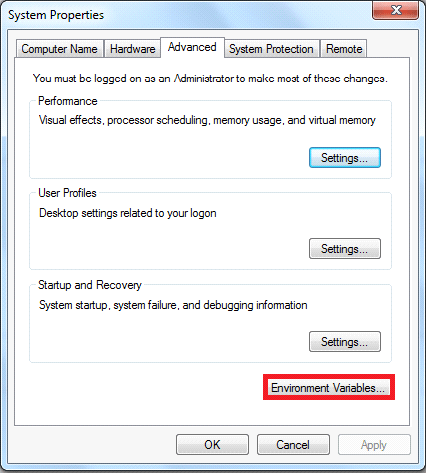
Step 4: If the CLASSPATH already exists in System Variables, click on the Edit button then put a semicolon (;) at the end. Paste the Path of MySQL-Connector Java.jar file.
If the CLASSPATH doesn't exist in System Variables, then click on the New button and type Variable name as CLASSPATH and Variable value as C:\Program Files\Java\jre1.8\MySQL-Connector Java.jar;.;
Remember: Put ;.; at the end of the CLASSPATH.
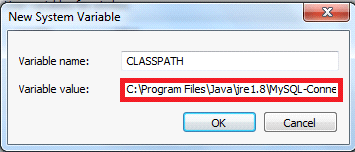
Q14) Write the difference between path and classpath?
A14) Difference between PATH and CLASSPATH
PATH | CLASSPATH |
PATH is an environment variable. | CLASSPATH is also an environment variable. |
It is used by the operating system to find the executable files (.exe). | It is used by Application ClassLoader to locate the .class file. |
You are required to include the directory which contains .exe files. | You are required to include all the directories which contain .class and JAR files. |
PATH environment variable once set, cannot be overridden. | The CLASSPATH environment variable can be overridden by using the command line option -cp or -CLASSPATH to both javac and java command. |
Q15) How to import a package?
A15) Import a Package
There are many packages to choose from. In the previous example, we used the Scanner class from the java.util package. This package also contains date and time facilities, random-number generator and other utility classes.
To import a whole package, end the sentence with an asterisk sign (*). The following example will import ALL the classes in the java.util package:
Example
Import java.util.*;
User-defined Packages
To create your own package, you need to understand that Java uses a file system directory to store them. Just like folders on your computer:
Example
└── root
└── mypack
└── MyPackageClass.java
To create a package, use the package keyword:
MyPackageClass.java
Package mypack;
Class MyPackageClass {
Public static void main(String[] args) {
System.out.println("This is my package!");
}
}
Save the file as MyPackageClass.java, and compile it:
C:\Users\Your Name>javac MyPackageClass.java
Then compile the package:
C:\Users\Your Name>javac -d . MyPackageClass.java
This forces the compiler to create the "mypack" package.
The -d keyword specifies the destination for where to save the class file. You can use any directory name, like c:/user (windows), or, if you want to keep the package within the same directory, you can use the dot sign ".", like in the example above.
Note: The package name should be written in lower case to avoid conflict with class names.
When we compiled the package in the example above, a new folder was created, called "mypack".
To run the MyPackageClass.java file, write the following:
C:\Users\Your Name>java mypack.MyPackageClass
The output will be:
This is my package!
Q16) Write a difference between package and interface?
A16) Key difference between Package and Interface
● An interface is a collection of abstract methods, whereas a package is a collection of classes and interfaces.
● A keyword package is used to construct a package, whereas a keyword interface is used to build an interface.
● If a package's class or interface is to be utilized, the package must be imported, and the interface must be implemented.
Q17) Write a sample program to implement an interface in Java?
A17) Program
Interface Results
{
Final static float pi = 3.14f;
Float areaOf(float l, float b);
}
Class Rectangle implements Results
{
Public float areaOf(float l, float b)
{
Return (l * b);
}
}
Class Square implements Results
{
Public float areaOf(float l, float b)
{
Return (l * l);
}
}
Class Circle implements Results
{
Public float areaOf(float r, float b)
{
Return (pi * r * r);
}
}
Public class InterfaceDemo
{
Public static void main(String args[])
{
Rectangle rect = new Rectangle();
Square square = new Square();
Circle circle = new Circle();
System.out.println("Area of Rectangle: "+rect.areaOf(20.3f, 28.7f));
System.out.println("Are of square: "+square.areaOf(10.0f, 10.0f));
System.out.println("Area of Circle: "+circle.areaOf(5.2f, 0));
}
}
Output:
Area of Rectangle: 582.61
Are of square: 100.0
Area of Circle: 84.905594