Unit - 5
8085 Programming & Interrupts
Q1) Write a program to add two 8-bit numbers.
A1)
MEMORY ADDRESS | MNEMONICS | COMMENTS |
3000 | LDA 3050 |
|
3003 | MOV H, A | A -> H |
3004 | LDA 3051 | A<-[2051] |
3007 | ADD H | A + H -> A |
3006 | MOV L, A | A -> L |
3007 | MVI A 00 | 00 -> A |
3009 | ADC A | A+A+carry -> A |
300A | MOV H, A | A -> H |
300B | SHLD 4050 | H→4051, L→4050 |
300E | HLT |
|
Explanation:
- LDA 3050 moves the contents of 3050 memory location to the accumulator (A).
- MOV H, A copies contents of accumulator to register H .
- LDA 3051 moves the contents of 3051 memory location to the accumulator.
- ADD H adds contents of A and H. The result is then stored in A.
- MOV L, A copies contents of A to L.
- MVI A 00 moves immediate data (i.e., 00) to A.
- ADC A adds contents of A(00), contents of register specified (i.e A) and carry (1).
- MOV H, A copies contents of A (01) to H.
- SHLD 4050 moves the contents of L register in 4050 memory location and contents of H register in 4051 memory location.
- HLT stops program execution.
Q2) Write a program to subtract two 8-bit numbers
A2)
Mnemonics | Operand | Comments |
LXI H, | 3502 H | Get address of 2nd no. In H-L pair. |
MVI A, | 99 | Place 99 in accumulator. |
SUB | M | 9’s compliment of 2nd no. |
INR | A | 10’s compliment of 2nd no. |
DCX | H | Get address of 1st no. |
ADD | M | Add 1st no. & 10’s compliment 2nd no. |
DAA |
| Decimal Adjustment. |
STA | 3503 H | Store result in 3503 H. |
HLT
|
| Stop the program. |
DATA
3501- 96 H.
3502- 38 H.
The result is stored in memory location 3503 H.
RESULT
2503- 58 H.
Q3) Write a program to add two 16-bit numbers.
A3)
We are taking two numbers ABCD + FE2D = 1B9FA
INPUT:
…. | … |
5000H | CD |
5001H | AB |
5002H | 2D |
5003H | FE |
… | …. |
PROGRAM:
Labels | Mnemonics | Operand | Comments |
| LXI H, | 5000H | Point to LSB of first operand |
| LXI D, | 5002H | Point to LSB of second address |
| LDAX | D | Load Acc with content pointed by DE |
| ADD | M | Add memory element pointed by HL with Acc |
| MOV C, | A | Store LSB result at C |
| INX | H | Point to next byte of first operand |
| INX | D | Point to next byte of second operand |
| LDAX | D | Load Acc with content pointed by DE |
| ADC | M | Add memory element pointed by HL with Acc+ Carry |
| MOV B, | A | Store the MSB at B |
| MOV H, | B | Move B to H |
| MOV L, | C | Move C to L |
| SHLD | 5050H | Store the result at 5050H and 5051H |
| JNC | DONE | Skip to end |
| MVI A, | 01H | Load 1 to Acc |
| STA | 5052H | Store Acc content into 5052H |
DONE: | HLT |
| Stop the program. |
RESULT:
5052: FA
5053: B9
5054: 01
Q4 WAP for finding lowest no. In data array
A4)
Labels | Mnemonics | Operand | Comments |
| LXI H, | 5000H | Address for count in H-L pair |
| MOV C, | M | Count in C register |
| INX | H | Address for 1st no. |
| MOV A, | M | 1st no. Sent to the accumulator |
| DCR | C | Decrement count |
LOOP: | INX | H | Address of next no. |
| CMP | M | Compare next no. |
| JC | AHEAD | If no, get smaller no. In accumulator go to AHEAD |
| MOV A, | M | Get smaller no. In accumulator |
AHEAD: | DCR | C | Decrement count |
| JNZ | LOOP |
|
| STA | 4000H | Store in 4000H |
| HLT |
| Stop program |
DATA:
5000H : 03
5001H : 75
5002H : 98
5003H : 99
RESULT:
4000H : 03
Q4) WAP for finding highest no. In data array
A5)
Labels | Mnemonics | Operand | Comments |
| LXI H, | 5000H | Address for count in H-L pair |
| MOV C, | M | Count in C register |
| INX | H | Address for 1st no. |
| MOV A, | M | 1st no. Sent to the accumulator |
| DCR | C | Decrement count |
LOOP: | INX | H | Address of next no. |
| CMP | M | Compare next no. |
| JNC | AHEAD | If no, go to AHEAD |
| MOV A, | M | Or get larger no. In accumulator |
AHEAD: | DCR | C | Decrement count |
| JNC | LOOP |
|
| STA | 4000H | Store in 4000H |
| HLT |
| Stop program |
DATA:
5000H : 03
5001H : 75
5002H : 98
5003H : 99
RESULT:
4000H : 99
Q5) Write a program for delay subroutine using register
A6)
- The microprocessor does not execute other tasks, when the delay subroutine is executed.
- For the delay, we use the instruction execution times.
- Execution of some instructions in a loop, the delay can be generated.
- Some methods for generating delays are as follows:
- Using NOP instructions
- Using 8-bit register as counter
- Using 16-bit register pair as counter.
Using NOP instructions
- It takes four clock pulses for fetching, decoding and executing.
- If the 8085 MPU is working on 4MHz clock frequency, then the internal clock frequency is 2MHz.
- Hence, can easily determine that each clock period is 1/2 of a microsecond.
- So the NOP will be executed in 1/2 * 4 = 2µs.
- If the entire memory uses NOP instruction, then 64K NOP instructions will be executed. Then the overall delay will be 216 * 2µs = 131072µs.
- Hence, it can be used to generate a short time delay of few milliseconds.
Using 8-bit register as counter
Labels | Mnemonics | Operand | Comments |
| MVI B, | FFH | 7 T states required |
LOOP: | DCR | B | 4 T states required and will be executed 255 (FF) times |
| JNZ | LOOP | 10 T states required when it jumps (It jumps 254 times), otherwise it take 7 T-States |
| RET |
| 10 T states required |
7 + ((4*255) + (10*254)) + 7 + 10 = 3584.
So the time delay will be 3584 * 1/3µs = 1194.66µs.
So when small delay is needed, then we can use this technique with some other values in the place of FF.
Using 16-bit register pair as counter
Labels | Mnemonics | Operand | Comments |
| LXI B, | FFFFH | 10 T states required |
LOOP: | DCX | B | 6 T states required |
| MOV A, | B | 4 T states required |
| ORA | C | 4 T states required |
| JNZ | LOOP | 10 T states required when it jumps, otherwise it take 7 T-States |
| RET |
| 10 T states required |
10 + (6 + 4 + 4 + 10) * 65535H – 3 + 10 = 17 + 24 * 65535H = 1572857.
Time delay = 1572857 * 1/3µs = 0.52428s.
Here we are getting nearly 0.5s delay.
Q6) Explain the use and how the memory locations of stack are affected?
A7) Stack is set of memory locations which are specified by the programmer in main program. These memory locations are used to store binary information temporarily during execution of program. The stack is shred by programmer and the microprocessor. There is a 16-bit memory address in stack pointer register (SP) of microprocessor. LXI SP, memory address instruction is used to load the SP with specific memory address.
The Stack is assigned the highest memory location so that the program is not affected. By using PUSH instruction, the data bytes in the register pair can be stored in the stack in reverse order. Using POP instruction, they can be again transferred to the respective registers.
The address in the SP register indicates the next two memory locations which are in descending order can be used for storage.
Example-1
LXI SP, xx99H | Load SP register with address xx99 |
PUSH H | It copies content of register pair (HL) on stack [H:xx98,L:xx97]. The SP register is decremented each time [SP = xx97] |
PUSH B | It copies content of register pair (BC) on stack [B:xx96, C:xx95] The SP register is decremented each time [SP = xx95] |
PUSH PSW | It is program status word which means content of A and F [A:xx94, F: xx93]. The SP register is decremented each time [SPxx93] |
POP H | It copies content of top 2 memory locations to HL. [H: xx94, L: xx93]. The SP is incremented each time [SP: xx95] |
POP B | It copies content of top 2 memory locations to HL. [H: xx95, L: xx96]. The SP is incremented each time [SP: xx97] |
POP PSW | It is program status word which means content of A and F [A: xx98, F: xx97]. The SP register is incremented each time [SP: xx99] |
Q7) How CALL and RETURN instructions are executed in subroutine?
A8) It is group of instructions written separately from the main program. We can use this sub program many times in main program by using CALL and RETURN instructions.
Example-1
Memory location | Instruction |
2000H | LX SP, 2300H |
2040H | CALL 2070H |
2043H | Next Instruction |
The CALL instruction requires 5 machine cycles and 18 T-states. The machine cycles are explained below.
M1 Opcode Fetch: The content of program counter 2040 are placed on address bus and the machine code is fetched using data bus. In the same time the program counter is incremented by one. So, now the new value in program counter is 2041H. After instruction is decoded and executed SP is decremented by 1. This machine cycle needs 6T-states.
M2-M3: These two are memory read cycles. The 16-bit address of CALL which is 2070H is fetched. The lower order 70H is fetched first and placed in internal register Z. Next the higher order address is fetched which is 20H and placed in register W. For machine cycle M3 the program counter is upgraded to location pointing next instruction. The both machine cycles require 3T states each.
M4-M5: It is storing of program counter. When M4 begins the content of SP register 23FFH is placed on address bus. The higher order is placed on the data bus 20H and stored in stack location 23FFH. Then simultaneously the SP is also decremented by 1(23FEH). In M5 the content of SP is placed on address bus and lower byte of program counter 43H is placed on data bus and stored in stack location 23FEH. The both machine cycles require 3T states each.
RET Execution
At the end of subroutine RET is executed. The program sequence is transferred to 2043H location. This was stored in top two stack locations 23FEH and 23FFH in CALL instruction. The RET requires 3 machine cycles. M1 is the opcade fetch. In M2 content of SP are placed on address bus. The data byte 43H from top of stack is fetched first and stored in Z and SP is incremented by 1. The next byte is copied in M3 i.e 20H and stored in W and SP is again incremented by 1(2400H).
Q8) What are conditional and continuous loop?
A9) The programming technique used to instruct the microprocessor to repeat task is called looping. The techniques such as counting and indexing are used to set up a loop. The loop can be of two types
Continuous Loop
The loop formed by using unconditional jump instruction. This loop will follow the instructions and repeat the same task until the system is reset.
Conditional Loop
The conditional jump instruction set up a conditional loop. These instructions check the flags such as zero, carry etc and then repeat the specified task if that condition is satisfied. These loops include counting and indexing.
Indexing means to refer objects with sequential numbers. The counting is set up by loading specific count value in the register. It can be incremental or decremental.
Q9) What are instructions and how many instructions are there for 8085?
A10) An instruction is a binary pattern designed inside a microprocessor to perform a specific function.
- The entire group of instructions that a microprocessor supports is called Instruction Set.
- 8085 has 246 instructions.
- Each instruction is represented by an 8-bit binary value.
- These 8-bits of binary value is called Op-Code or Instruction Byte.
Q10) Explain pin diagram of 8085 with pin functions?
A11)
- Address bus A15-A8.
- Data bus AD7-AD0.
- Three control signals are RD, WR & ALE.
- RD: It indicates that the selected IO or memory device can be read and is ready to accept data available on the data bus.
- WR: It indicates that the data on the data bus can be written into a selected memory or IO location.
- ALE: It is a positive going pulse that is generated when a new operation is started by the microprocessor. When high, it indicates address and when low, it indicates data.
- Three status signals are IO/M, S0 & S1.
IO/M: It is used for input/output or memory selection i.e when it is high it indicates IO operation and when it is low then it indicates memory operation.
- S1 & S0: They are used to identify the type of current operation.
- Power supply: VCC & VSS.
- VCC: +5V and VSS : ground signal (0V).
- Clock signals: There are 3 types of clock signals, X1, X2 and CLK OUT.
- X1, X2: A crystal (RC, LC N/W) is connected at these two pins and is used to set frequency of the internal clock generator. This frequency is internally divided by 2.
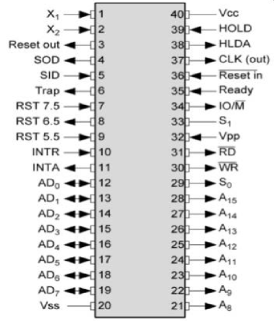
Fig: Pin diagram of 8085 (ref 1)
- CLK OUT: This signal is used as the system clock for devices connected with the microprocessor.
- Interrupts are the signals generated by external devices requesting the microprocessor to perform a certain task. There are 5 types of interrupt. They are TRAP, RST 7.5, RST 6.5, RST 5.5, and INTR.
- INTA: It is an interrupt acknowledge signal.
- RESET IN: It is used to reset the microprocessor by setting the program counter to zero.
- RESET OUT: It is used to reset all the devices connected to the microprocessor when microprocessor is reset.
- READY: It indicates that the device is ready to send or receive data. If it is low, then the CPU has to wait for it to go high.
- HOLD: It indicates that another master is requesting the use of the address and data buses.
- HLDA: It stands for HOLD Acknowledge. It indicates that the CPU has received the HOLD request and is ready to share the bus in the next clock cycle. After the HOLD signal is removed HLDA is set to low.
- SID and SOD signals are used for serial communication.
- SOD (Serial output data line): It is set/reset by the SIM instruction.
- SID (Serial input data line): when it is active, the data on this line is loaded into accumulator whenever a RIM instruction is executed.
Q11) Explain the architecture of 8085 microprocessor?
A12) It includes the timing & control unit, Arithmetic and logic unit, decoder, instruction register, interrupt control, a register array, serial input/output control and the central processing unit.
Operations of 8085 Microprocessor
It performs all arithmetical and logical operations like add, subtract, AND, OR etc. The temporary registers and accumulators hold the information throughout the operations and the result is stored in the accumulator. The different flags are arranged or rearranged on the basis of result.
Flag Registers
It is an 8 bit register. They are of five types namely sign, zero, auxiliary carry, parity and carry.
Control and Timing Unit
It coordinates with all the processes of the microprocessor by the clock and provides the control signals required for communication between the microprocessor and peripherals. It is used to control the internal as well as external circuits.
Decoder and Instruction Register
When an order is received from memory which is located in the instruction register, it is encoded & decoded into various device cycles.
Register Array
The general purpose registers are classified into several types such as B, C, D, E, H, & L. They are 8-bit registers. The register pairs are BC, DE & HL.
Special Purpose Registers
These registers are of four types namely program counter, stack pointer, increment or decrement register, address or data buffer.
Program Counter
It is a 16 bit register. It is used to store data, memory information etc. whenever memory is incremented, the PC then points to the next location.
Stack Pointer
It is a 16 bit register. It always points to stack which can be incremented or decremented by PUSH and POP instruction.
Increment or Decrement Register
It is an 8-bit register which can be increased or decreased by one. It is useful for incrementing or decrementing program counters as well as stack pointer register content with one. This operation can be done on any memory location or any kind of register.
Address Bus and Data Bus
Data bus carries the data required to be stored. It is bidirectional. Address bus carries the location to where the data should be stored and it is unidirectional. It is used to transfer the data & address.
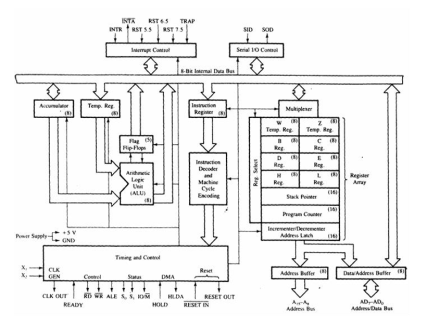
Fig: Architecture of 8085
Q12) Explain Register organisation of 8085?
A13)
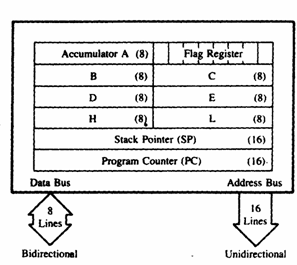
Fig: Register organisation of 8085
A=Accumulator
SP = Stack Pointer
PC =Program Counter
Flag Register


Register Pairs
There are three register pairs BC, DE and HL. Where HL is special register pair as it points current location of memory.
Stack Pointer (SP):
It holds address. It is important to initialise SP before using the stack. It is initialised by the highest memory location.
Program Counter (PC)
It will always hold the address. It sequences the execution of instructions. The microprocessor is a sequential logic device.
Q13) Explain the ALU unit of 8085?
A14) The word length of ALU depends upon of an internal data bus.
It is 8 bit and is always controlled by timing and control circuits.
It provides status or result of flag register.
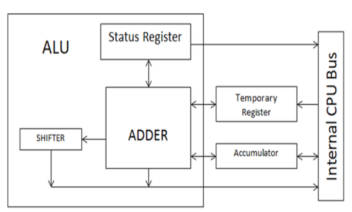
Fig: ALU of 8085
Adder: It performs arithmetic operations like addition, subtraction, increment, decrement, etc. The result of operation is stored into accumulator.
Shifter: It performs logical operations like rotate left, rotate right, etc. The result of operation is again stored into accumulator.
Status Register: Also known as flag register. It contains a no. Of flags either to indicate conditions arising after last ALU operation or to control certain operations.
Accumulator:
It is one of the general-purpose register of microprocessor also called as A register.
The accumulator is an 8-bit register that is a part of arithmetic/logic unit (ALU).
This register is used to store 8-bit data and to perform arithmetic and logical operations.
The result of an operation is stored in the accumulator.
The user can access this register by giving appropriate instructions (commands).
Temporary Register:
It is also called as operand register (8 bit).
It provides operands to ALU. ALU can store immediate result in temporary register.
It is not accessible by user.
Status or flag register:
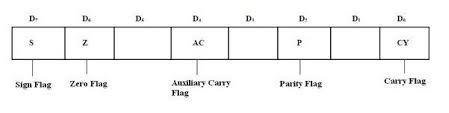
Flag register is a group of flip flops used to give status of different operations result.
The flag register is connected to ALU.
Once an operation is performed by ALU the result is transferred on internal data bus and status of result will be stored in flip flops.
They are called Zero (Z), Carry (CY), Sign (S), Parity (P), and Auxiliary Carry (AC) flags.
Q14) Explain control and status signal of 8085?
A15)
- ALE – It is an Address Latch Enable signal. It goes high during first T state of a machine cycle and enables the lower 8-bits of the address, if its value is 1 otherwise data bus is activated.
- IO/
– It is a status signal which determines whether the address is for input-output or memory. When it is high(1) the address on the address bus is for input-output devices. When it is low(0) the address on the address bus is for the memory.
- SO, S1 – These are status signals. They distinguish the various types of operations such as halt, reading, instruction fetching or writing.
– It is a signal to control READ operation. When it is low the selected memory or input-output device is read.
– It is a signal to control WRITE operation. When it goes low the data on the data bus is written into the selected memory or I/O location.
- READY – It senses whether a peripheral is ready to transfer data or not. If READY is high(1) the peripheral is ready. If it is low(0) the microprocessor waits till it goes high. It is useful for interfacing low speed devices.
Q15) What are interrupt signals explain the types of interrupts of 8085?
A16) The 8085 has five interrupt signals that can be used to interrupt a program execution.
INTR
RST 7.5
RST 6.5
RST 5.5
TRAP
The microprocessor acknowledges Interrupt Request by INTA’ signal. In addition to Interrupts, there are three externally initiated signals namely RESET, HOLD and READY. To respond to HOLD request, it has one signal called HLDA.
INTR – It is an interrupt request signal.
– It is an interrupt acknowledgment sent by the microprocessor after INTR is received.
Q16) Explain instruction format of microprocessor?
A17) According to the instruction word size, there are three types of instructions. They are:
- 1 byte instruction
- They include opcode and operand in the same byte.
- Operand are internal registers and coded into the instruction.
- Instruction requires one memory location to store one single byte of memory.
- For example:
MOV B,C
HLT
- 2 byte instruction
- Here 1st byte specifies opcode and the 2nd byte species operand.
- Instruction requires two memory location to store in memory.
- For example:
MOV B,20H
IN 25H
- 3 byte instruction
- Here 1st byte is opcode and the next two bytes species memory address.
- The 2nd byte holds the lower order address and the 3rd byte holds the higher order address.
- Instructions require three memory locations to store in memory.
- For example:
LDA 2000
JMP 5055H
Q17) Explain assembler directives?
A18)
They convert assembly language program to machine language. The basic aim of the assemble directive are to sow the beginning and end of program. They give storage location for data. They also define the start and end of different segments.
They are also called as pseudo-opcode. These instructions are neither translated into machine code nor assigned any memory location in the object file. Some of the important assembler directives for an assembler or a cross assembler are listed and described here.
Data -Bytes (Data Bytes): Assemble bytes of data are stored in successive memory location until all values are stored. This is a convenient way of writing a data string.
Exapmle-1
If we do not use
LDA, 4150H
MOV B,A
Or
MVI B, 25H
MOV A, B
We use DB, 25H, 29H
Data Word (DB)
It is used to just initialise an area of 2 bytes at a time. For example: DW 2050H
Data Quard Word (DQ)
It initialises 4 word or 8-bits in one instruction that means we take word which occupy a 8-bit number of decimal. For example: DQ 2050 95 76 30 75 95 86 H
Definite 10 Bit (DT)
It is similar to DQ but it occupies 2 bits extra. For example: DT 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 AB H.
Define Double Word (DD)
It is similar to DQ and DT. For example: DD 2050 60 75H
Definite Storage Location (DS)
Reserves a specified number of memory locations. In this example four memory locations are reserved for OUTBUF. For example: OUTBUF DS4
ORG
If we use ORG 4000H that means the next block of instructions should be stored in memory location starting 4000H.
EQU (equate)
Generally, we transfer any information in port address. Instead of
MVI A, 09H
OUT PA
PA EQU 40H
END
It is similar to HALT. But the HLT suggest the end of program but that does not necessarily mean it is the end of the assembly.
Q18) Write all the logical group of instructions?
A19) The logical operations are:
- AND
- OR
- XOR
- Rotate
- Compare
- Complement
OPCODE | OPERAND | DESCRIPTION |
CMP | R M | Compare the contents of register or memory with accumulator |
ANA | R M | Logical AND the contents of register with accumulator |
ANI | 8 bit data | Logical AND the data with accumulator |
XRA | R M | XOR the contents of register with accumulator |
ORA | R M | Logical OR the contents of register with accumulator |
RLC | - | Rotate accumulator left |
RRC | - | Rotate accumulator right |
CMA | - | Complement accumulator |
For example:
CMP C
ANA B
ANI 40H
XRA M
ORA C
RLC
Q19) Write the data transfer instruction for 8085?
A20) These instructions transfer data between registers or between memory and registers. These instructions are used to copy data from source to destination. While copying, the contents of source cannot be modified.
OPCODE | OPERAND | DESCRIPTION |
MOV | Rd, Rs Rd, M M, Rs | Transfer the contents of the source to the destination. |
MVI | Rd, data M, data | Move immediate data into the destination register |
LXI | Reg. Pair, data | Move 16 bit data into the register pair |
LDA | 16 bit address | Load accumulator with data present at the specified address |
XCHG | - | Exchange the contents of HL with DE |
For example:
MOV B, C
MVI B, 20H
LXI H, 4020H
LDA 6000H