Unit - 1
Introduction to Microcontrollers
Q1) Exchange the content of FFH and FF00H
A1)
MOV dptr, #0FF00H ; take the address in dptr
MOVX A, @dptr ; get the content of 0050H in a
MOV r0, 0FFH ; save the content of 50H in r0
MOV 0FFH, a ; move a to 50H
MOV A, r0 ; get content of 50H in a
MOVX @dptr, a ; move it to 0050H
Q2) Store the higher nibble of r7 in to both nibbles of r6
A2)
Firstly, we get the upper nibble of r7 in r6. Then we swap nibbles of r7 and make OR operation with r6 so the upper and lower nibbles are duplicated
MOV A, r7 ; get the content in acc
ANI A, #0F0h ; mask lower bit
MOV r6, A ; send it to r6
SWAP A ; xchange upper and lower nibbles of accumulator
ORL A, r6 ; OR operation
MOV r6, A ; finally load content in r6
Q3) Transfer the block of data from 20H to 30H to external location 1020H to 1030H.
A3)
MOV r7, #0AH ; initialize counter by 10d
MOV r0, #20H ; get initial source location
Mov dptr, #1020H ; get initial destination location
Nxt: MOV A, @r0 ; get first content in acc
MOVX @dptr, a ; move it to external location
INC r0 ; increment source location
INC dptr ; increase destination location
DJNZ r7, nxt ; decrease r7. If zero then over otherwise move next
Q4) Given block of 100h to 200h. Find out how many bytes from this block are greater then, the number in r2 and less then number in r3. Store the count in r4.
A4)
MOV dptr, #0100H ; get initial location
MOV r7, #0FFH ; counter
MOV r4, #00H ; number counter
MOV 20H, R2 ; get the upper and lower limits in
MOV 21H, R3 ; 20H and 21H
Nxt: MOVX A, @dptr ; get the content in acc
CJNE A, 21H, lower ; check the upper limit first
SJMP out ; if number is larger
Lower: JNC out ; jump out
CJNE A, 20H, limit ; check lower limit
SJMP out ; if number is lower
Limit: JC out ; jump out
INC r4 ; if number within limit increment count
Out: INC dptr ; get next location
DJNZ r7, nxt ; repeat until block completes
Q5) Find out how many equal bytes between two memory blocks 10H to 20H and 20H to 30H.
A5)
MOV r7, #0AH ; initialize counter by 10d
MOV r0, #10H ; get initial location of block1
MOV r1, #20H ; get initial location of block2
MOV r6, #00H ; equal byte counter. Starts from zero
Nxt: MOV A, @r0 ; get content of block 1 in acc
MOV b, a ; move it to B
MOV A, @r1 ; get content of block 2 in acc
CJNE A, B, nomatch ; compare both if equal
INC r6 ; increment the counter
Nomatch: INC r0 ; otherwise go for second number
INC r1
DJNZ r7, nxt ; decrease r7. If zero then over otherwise move next
Q6) Write a test program for the DS89C420/30 chip to toggle all the bits of PO, PI, and P2 every 1/4 of a second. Assume a crystal frequency of 11.0592 MHz.
A6)
ORG 0
BACK: MOV A,#55H
MOV P0, A
MOV P1, A
MOV P2, A
ACALL QSDELAY
SJUMP BACK
QSDELAY:
MOV R5, #11
H3: MOV R4, #248
H2: MOV R3, #255
H1: DJNZ R3, H1
DJNZ R4, H2,
DJUNZ R5, H3
Delay = 11 x 248 x 255 x 4MC x 90ns = 250,430s
Q7) Create a square wave of 50% duty cycle on bit 0 of port 1.
A7)
The 50% duty cycle means that the "on" and "off' states (or the high and low
Portions of the pulse) have the same length. Therefore, we toggle Pl.0 with a
Time delay in between each state.
HERE: SETB P1.0
LCALL
CLR PI. 0
LCALL DELAY
SJMP HERE
Another way to write the above program is:
HERE: CPL P1.0
LCALL DELAY
SJMP HERE
Q8) Create a square wave of 66% duty cycle on bit 3 of port 1
A8)
BACK: SETB Pl.3
LCALL DELAY
LCALL DELAY
CLR P1.3
LCALL DELAY
SJMP BACK
Q9) Write a program to perform the following:
(a) keep monitoring the Pl.2 bit until it becomes high
(b) when P 1.2 becomes high, write value 45H to port 0
(c) send a high-to-Iow (H-to-L) pulse to P2,3
A9)
SETB PI.2
MOV A,#45H
AGAIN JNB PI.2,AGAIN
MOV PO ,A
SETB P2.3
CLR P2.3
In this program, instruction "JNB PI 2 AGAING” (JNB means Jump if no bit) stays in the loop as long as P 1.2 is low. When P.12 becomes high. It gets out of the loop. Writes the value 45H to port 0 and creates an H-to-L pulse by the sequence of instruction SETB and CLR.
Q10) Assume that bit P2.3 is an input and represents the condition of an oven. If it goes high, it means that the oven is hot. Monitor the bit continuously. Whenever it goes high, send a high-to-low pulse to port P 1.5 to tum on a buzzer.
A10)
HERE: JNB P2.3, HERE
SETB Pl. 5
CLR Pl. 5
SJMP HERE
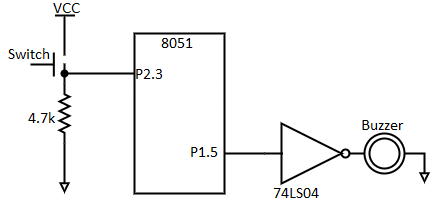
Q11) A switch is connected to pin P 1.7. Write a program to check the status of SW and perform
The following:
(a) If SW=O, send letter 'N' to P2.
(b) If SW=I, send letter 'Y'toP2.
A11)
SETB Pl.?
AGAIN JB Pl.2,OVER
MOV P2,#'N'
SJMP AGAIN
OVER MOV P2,#'Y'
SJMP AGAIN
Q12) A switch is connected to pin PI. 7. Write a program to check the status of the switch and
Perform the following:
(a) If switch = 0, send letter 'N' to P2.
(b) If switch = I, send letter 'Y'toP2.
A12)
SET P1.7
AGAIN: MOV C, P1.2
JC OVER
OVER: MOV P2, #’N’
SJMP AGAIN
Q13) A switch is connected to pin P1.0 and an LED to pin P2.7 status of the switch and send it to the LED
A13)
SET P1.7
AGAIN: MOV C, P1.0
MOV P2.7, C
SJMP AGAIN
Q14) Write 8051 C program to toggle all the bits of port P I continuously with some delay in between. Use Timer 0, 16-bit mode to generate the delay.
A14)
#include <reg5I.h>
Void TODelay(void);
Void main (void)
{
While(l) //repeat forever
{
Pl=Ox55; //toggle all bits of PI
T0Delay (); //delay size unknown
Pl=OxAA; //toggle all bits of PI
T0Delay ();
}
}
Void T0 Delay ()
{
TMOD=0x01; //Timer 0, Mode 1
TL0=0x00; //load TL0
TH0=0x35; //load TH0
TR0=l; //turn on T0
While (TF0==0); //wait for TF0 to rollover
TR0=0; //turn off T0
TF0=0; //clear TF0
}
FFFFH - 3500H = CAFFH = 51967 + 1 = 51968
51968 x 1.085 s = 56.384 ms is the approximate delay
Q15) Write an 8051 C program to toggle only bit P 1.5 continuously every 50 ms. Use Timer 0, mode I (l6-bit) to create the delay. Test the program (a) on the AT89C51 and (b) on the DS89C420.
A15)
#include <regsl.h>
Void T0M1Delay(void);
Sbit mybit=PIAS;
Void main (void)
{
While(l)
{
Mybit=-mybit; //toggle Pl.5
T0MIDelay (); //Timer 0, mode 1(16-bit)
}
}
(a) Tested for AT89C51, XTAL=II.0592 MHz,
Void T0M1Delay(void)
{
TMOD=0x0l; //Timer 0, mode 1(16-bit)
TL0=0xFD; //load TL0
TH0=0x4B;//load TH0
TR0=l; //turn on T0
While (TF0==0); //wait for TF0 to rollover
TR0=0; //turn off T0
TF0=0; //clear TF0
}
(b) Tested for D589C420, XTAL=II.0592 MHz,
Void T0M1Delay(void)
{
TMOD=0x0l; //Timer 0, mode 1(16-bit)
TL0=0xFD; //load TL0
TH0=0x4B; //load TH0
TR0=l; //turn on T0
While (TF0==0); //wait for TFO to rollover
TR0=0; //turn off T0
TF0=0; //clear TF0
}
FFFFH - 4BFDH = B402H = 46082 + 1 =46083
Timer delay = 46083 x 1.085 s = 50 mS
Q16) Write an 8051 C program to toggle a11bits of P2 continuously every 500 ms.Timer 1, mode 1 to create the delay.
A16)
#include <regsl.h>
Void TIMIDelay(void);
Void main (void)
Unsigned
P2=OxSS;
While (1);
{
P2=-P2; //toggle all bits of P2
For(x=O;x<20;x++)
TIMlDelay();
}
}
Void TIMIDelay(void)
TMOD=0xl0; //Timer 1, mode 1(16-bit)
TLl=0xFE; //load TLI
THl=0xAS; //load THI
TRl=l; //turn on Tl
While (TFl==0); //wait for TFI to rollover
TRl=0; //turn off Tl
TFl=0; //clear TFI
}
A5FEH = 42494 in decimal
65536 - 42494 = 23042
23042 x 1.085 s = 25 ms and 20 x 25 ms = 500 ms
Q17) Write an 8051 C program to toggle only pin P1.5 continuously every 250 ms. Use Timer 0, mode 2 (8-bit auto-reload) to create the delay
A17)
#include <reg51.h>
Void T0M2Delay(void);
Sbit mybit=PIA5;
Void main (void)
{
Unsigned char x, y;
While (1)
{
Mybit=-mybit; //toggle Pl. 5
For(x=O,x<250;x++) //due to for loop overhead
For(y=O;y<36;y++) //we put 36 and not 40
T0M2Delay();
}
}
Void T0M2Delay(void)
{
TMOD=0x02; //Timer 0, mode 2(B-bit auto-reload)
TH0=-23; //load TH0 (auto-reload value)
TR0=I; //turn on T0
While (TF0==0); //wait for TFO to rollover
TR0=0; / / turn off. T0
TF0=0; //clear TF0
}
256 - 23 = 233
23 x 1.085 s = 25
s
25 s x 250 x 40 = 250 ms by calculation.
Q18) Write an 8051 C program to create a frequency of 2500 Hz on pin P2. 7. Mode 2 to create the delay.
A18)
#include <regS1.h>
Void T1M2Delay(void);
Sbit mybit=P2.7;
Void main (void)
(
Unsigned char X;
While (1)
(
Mybit=-mybit;
TlM2Delay ();
}
}
Void T1M2Delay(void)
TMOD=0x20; //Timer 1, mode 2(8-bit auto-reload)
TH1=-184; //load TH1(auto-reload value)
TR1=1;//turn on T1
While (TF1==0); //wait for TF1 to roll over
TR1=0; //turn off T1
TF1=0;//clear TF1
}
1 / 2500 Hz = 400 s
400 s / 2 = 200
s
200 s / 1.085
s =184
Q19) A switch is connected to pin P 1.2. Write an 8051 C program to monitor SW and create the following frequencies on pin PI.7:
SW=O: 500 Hz
SW=l: 750Hz
Use Timer 0, mode 1 for both of them.
A19)
#include <reg51.h>
Sbit mybit=P1^5;
Sbit SW=P1^7;
Void TOMIDelay(unsiged char);
Void main (void)
{
SW=l, //make PI.7 an input
While (1)
{
Mybit=-mybit;
If (SW==0)
TOMlDelay (0);
Else
T0MlDelay (1);
}
}
Void TOMIDelay(unsigned char c)
{
TMOD=0X01;
If (c==0)
{
TL0=0x67; //FC67
TH0=0xFC;
}
Else
{
TL0=0x9A; //FD9A
TH0=0xFD;
}
TRO=l;
While (TF0==0);
TR0=0;
TF0=0,
}
FC67H = 64615
65536 - 64615 = 921
921 X 1.085 s = 999.285
s
1 / (999.285 s X 2) = 500 Hz
Q20) Assume that a l-Hz external clock is being fed into pin T1 (P3.5). Write a C program for counter I in mode 2 (8-blt auto reload) to count up and display the state of the TLI count on P I. Start the count at 0H.
A20)
#include <regSI.h>
Sbit Tl = P3'S;
Void main (void)
{
T1=1; //make T1 an input
TMOD=0X60;
TH1=0; //set count to 0
While (1)
{
Do //repeat forever
{
TR1=1; //start timer
PhTL1; //place value on pins
}
While (TF1==O) .
TR1=0;
TFl=0;
}
}
Q21) Assume that a I-Hz external clock is being fed into pin TO (P3.4). Write a C program for counter 0 in mode 1 (I 6-bit) to count the pulses and display the TH0 and TL0 registers on P2 and PI, respectively.
A21)
#include <reg51.h>
Void main (void)
{
T0=l; //make TO an input
TMOD=0x05;
TL0=0; //set count to 0
TH0=0; //set count to 0
While (1) //repeat forever
{
Do
{
TR0=l; //start timer
Pl=TL0; //place value on pins
P2=TH0;
}
While (TF0==0); //wait here
TR0=0; //stop timer
TF0=0;
}
}
Q22) Assume that a 2-Hz external clock is being fed into pin TI (P3.5). Write a C program for counter 0 in mode 2 (8-bit auto reload) to display the count in ASCII. The 8-bit binary count must be converted to ASCII. Display the ASCII digits (in binary) on P0, PI, and P2 where P0 has the least significant digit. Set the initial value of TH0 to 0.
A22)
To display the TLI count we must convert 8-bit binary data to ASCII. The ASCII values will be shown in binary. For example, '9' will show as 00111001 on ports.
#include <reg51.h>
Void BinToASCII(unsigned char);
Void main ()
(
Unsigned char value;
T1=1;
TMOD=0X06;
TH0=0;
While(l)
(
Do
(
TR0=l;
Value=TL0;
BinTOASCII(value);
)
While (TF0==0);
TR0=0;
TF0=0;
}
}
Void BinToASCII(unsigned char value)
Unsigned char X,d1,d2 d3;
x = value/10;
Dl=value % 10;
d2= x % 10;
d3=x/10
P0=30|dl;
P1=30|d2;
P2=30Id3;
}
Q23) Assume that a 60-Hz external clock is being fed into pin T0 (P3.4). Write a C program for counter 0 in mode 2 (8-bit auto-reload) to display the seconds and minutes on P1 and P2, respectively.
A23)
#include <reg51.h>
Void ToTime(unsigned char);
Void main ()
{
Unsigned char val;
T0=1;
TMOD=0x06; //T0, mode 2, counter
TH0=-60; //sec = 60 pulses
While(1)
{
Do
{
TR0=1;
Sec=TL0;
ToTime (val);
}
}
While (TF0==0);
TR0=0;
TF0=0;
Void ToTime(unsigned char val)
{
Unsigned char
Min = valueI60;
Sec = value %60;
PI = sec;
P2 = min;
}