Unit - 3
Integrated Development Environment (IDE) for Microcontrollers
Q1) Write quotes to set PB2 and PB4 of PORTB to 1 and clear the other pins i) without the directive and b) using the directive
A1)
a) Without directives
LDI R20, 0X14
OUT PORTB, R20
LDI R20,0b00010100
OUT PORTB, R20
b) With directives
LDI R20, (1<<4) | (1<<2); R20 = (0b10000| 0b00100) =0b10100
OUT PORTB, R20; PORTB= R20
As we already know the names of the register beds are defined in the header files of each AVR microcontroller PB2 and PB4 are defined equal to 2 and 4 as well therefore we can write the code as shown below
LDI R20, (1<< PB4) | (1<< PB2); set the PB4 and PB2 bits
OUT PORTB, R20; PORTB = R20
Q2) What does the abr assembler do while assembling the following program .EQU C1 =2
.EQU C2 = 3
LDI R20, C1 | (1<<C2); R20 = 2| (1<<3) = 0b00000010 | 0b00001000 = 0b00001010
A2) .EQU is an assembler directive when assembling .EQU C1 =2 assembler assigns value to 2 to C1. Similarly while assembling the .EQU C2=3 instruction it assigns the value 3 to C2
When the assembler converts instruction LDI R20, C1 | (1<<C2) to machine language it knows the value of C1 and C2. Thus, it calculates the value of C1| (1<<C2) and then replaces the expression with its value. Therefore, LDI R20, C1 | (1<<C2) will be converted to LDI R20, 0b00001010. Then the assembler converts the instructions to machine language.
Q3) Write code to send $55 to PORTB. Include (a) the register name (b) the I/O address and (c) the data memory address?
A3)
a) LDI R20, 0XFF; R20 = 0XFF
OUT DDRB, R20; DDRB= R20 (Port B output)
LDI R20, 0X55; R20= $55
OUT PORTB, R20; Port B =0X55
b) LDI R20, 0XFF; R20 = 0XFF
OUT 0X17, R20; DDRB= R20 (Port B output)
LDI R20, 0X55; R20= $55
OUT 0X18, R20; Port B =0X55
c) LDI R20, 0XFF; R20 = 0XFF
STS 0X37, R20; DDRB= R20 (Port B output)
LDI R20, 0X55; R20= $55
OUT 0X38, R20; Port B =0X55
Q4) Write a program to copy the value $55 into memory locations $140 through $144 using (i) direct addressing mode (ii) register indirect addressing mode without loop and (iii) a loop
A4)
a) LDI R17,0X55; load R17 with value 0x55
STS 0X140, R17; copy R17 to memory location 0x140
STS 0X141, R17; copy R17 to memory location 0x141
STS 0X142, R17; copy R17 to memory location 0x142
STS 0X143, R17; copy R17 to memory location 0x143
STS 0X143, R17; copy R17 to memory location 0x144
b) LDI R16, 0X55; load R16 with value 0x55
LDI YL, 0X40; load R28 with value 0x40
LDI YH, 0X1; load R29 with value 0x1
ST Y, R16; copy R16 to memory location 0x140
INC YL; increment lower byte of Y
ST Y, R16; copy R16 to memory location 0x141
INC YL; increment the pointer
ST Y, R16; copy R16 to memory location 0x142
INC YL; increment the pointer
ST Y, R16; copy R16 to memory location 0x143
INC YL; increment the pointer
ST Y, R16; copy R16 to memory location 0x144
c) LDI R16, 0X5; R16=5
LDI R20, 0X55; load R20 with 0x55
LDI YL, 0X40; load YL with 0x 40
LDI YH,0X1; load YH with 0x1
L1:ST Y, R20; copy R20 to memory pointed to by Y
INC YL; increment the pointer
DEC R16; decrement the counter
BRNE L1; loop while counter is not 0
Q5) Assume that RAM locations $90-$94 have a string of ASCII data as shown below $90 =(‘H’), $91 =(‘E’), $92 =(‘L’), $94 =(‘O’),
WAP to get each character and send it to port B one byte at a time. Show that the program using (a) Direct addressing mode (b) Register indirect addressing mode.
A5)
a) Using direct addressing mode
LDI R20, 0XFF;
OUT DDRB, R20; make Port B an output
LDS R20, 0X90; R20=content of location 0x90
OUT PORTB, R20; PORTB =R20
LDS R20, 0X91; R20=content of location 0x91
OUT PORTB, R20; PORTB =R20
LDS R20, 0X92; R20=content of location 0x92
OUT PORTB, R20; PORTB =R20
LDS R20, 0X93; R20=content of location 0x93
OUT PORTB, R20; PORTB =R20
LDS R20, 0X94; R20=content of location 0x94
OUT PORTB, R20; PORTB =R20
b) Using register indirect addressing mode
LDI R16, 0X5; R10= 0X5
LDI R20, 0XFF
OUT DDRB, R20; make port B an output
LDI ZL, 0X90; the lower byte of address (ZL=0x90)
LDI ZH, 0X0; the higher byte of address (ZL=0x0)
L1: LD R20, Z; read from location pointed to by Z
INC ZL; increment pointer
OUT PORTB, R20; send to Port B the contents of R20
DEC R16; decrement counter
BRNE L1; if R16 is not zero go to L1
Q6) WAP to clear 16 memory locations starting at data memory address $60. Use the following (a) INC Rn (b) Auto-increment.
A6)
a) LDI R16, 16; R16= 16 (counter value)
LDI XL, 0X60; XL = the low byte of address
LDI XH, 0X00; XH = the high byte of address
LDI R20, 0X0; R20=0
L1:ST X, R20; clear location X points to
INC XL; increment pointer
DEC R16; decrement counter
BRNE L1; loop until counter = 0
b) LDI R16, 16; R16= 16 (counter value)
LDI XL, 0X60; XL = the low byte of address
LDI XH, 0X00; XH = the high byte of address
LDI R20, 0X0; R20=0
L1:ST X+, R20; clear location X points to
DEC R16; decrement counter
BRNE L1; loop until counter = 0
Q7) Assume that data memory locations $240-$243 have following hex data. WAP to add them together and place the result in locations $220 and $221
$240 =(‘$7D’), $241 =(‘$EB’), $242 =(‘$C5’), $243=(‘$5B’)
A7)
.INCLUDE “M32DEF.INC”
.EQU L_BYTE = 0X220; RAM loc for L_Byte
.EQU H_ BYTE= 0X221; RAM loc for H_Byte
LDI R16,4
LDI R20,0
LDI R21,0
LDI XL,0X40; the low byte of x= 0x40
LDI XH, 0X20; the high byte of x= 02
L1: LD R22, X+; read content of location where X points to
ADD R20, R22
BRCC L2; branch if C=0
INC R21; increment R21
L2: DEC R16; decrement counter
BRNE L1; loop until counter is 0
ST L_BYTE, R20; store the low byte of the result in $220
ST H_BYTE, R21; store the high byte of the result in $221
Q8) WAP to copy a block of 5 bytes of data from data memory locations starting at $130 to RAM locations starting at $60.
A8)
LDI R16, 16; R16= 16 (counter value)
LDI XL, 0X30; the low byte of address
LDI XH, 0X01; the high byte of address
LDI YL, 0X60; the low byte of address
LDI YH, 0X00; the high byte of address
L1: LD R20, X+; read where X point to
ST Y+, R20; store R20 where Y points to
DEC R16; decrement counter
BRNE L1; loop until counter = zero
Q9) WAP to copy a block of 5bytes of data from data memory locations using $130 to RAM locations starting at $60.
A9)
LDI R16, 16; R16=16
LDI XL, 0X30; the low byte of address
LDI XH, 0X01; the high byte of address
LDI YL, 0X60; the low byte of address
LDI YH, 0X00; the high byte of address
L1: LD R20, X+; read where X points to
ST Y+, R20; store R20 where Y points to
DEC R16; decrement counter
BRNE L1; loop until counter =0
Q10) Two multibyte numbers are stored in locations $130-$133 and $150-$153. WAP to add thee multibyte numbers and save the result in address $160-$163.
$C7659812
+ $2978742A
A10)
.INCLUDE “M32DEF.INC”
LDI R16, 4; R16=4 counter value
LDI XL, 0X30
LDI XH, 0X1; load pointer Z= $130
LDI YL, 0X50
LDI YH, 0X1; load pointer Z= $150
LDI ZL, 0X60
LDI ZH, 0X1; load pointer Z= $160
CLC; clear carry
L1: LD R18, X+; copy memory to R18 and INC X
LD R19, Y+; copy memory to R19 and INC Y
ADC, R18, R19; R18= R18+R19+carry
ST Z+, R18; store R18 in memory and INC Z
DEC R16; decrement counter
BRNE L1; loop until counter =0
Q11) Write a function that adds the contents of three continuous locations of data space and stores the result in the first location. The Z register should point to the first location before the function is called?
A11)
.INCLUDE”M32DEF.INC”
LDI R16, HIGH
OUT SPH,R16
LDI R16, LOW
OUT SPL, R16
LDI ZL, 0X00
LDI ZH, 2
CALL ADD3LOC
HERE: JMP HERE
ADD3LOC:
LDI R21, 0
LD R20, Z
LDD R16, Z+!
ADD R20, R16
BRCC L1
INC R21
L1: LDD R16, Z+2
ADD R20, R16
BRCC L2
INC R21
L2: ST Z, R20
STD Z+1, R21
RET
Q12) Write a program to subtract 18H from 29 H and store the result in R21. Without using the SBI instruction and using the SUBI instruction.
A12)
a) Without SUBI instruction
LDI R21, 0X29
LDI R22, 0X18
SUB R21, R22
b) LDI R21, 0X29
SUBI R21, 0X18
Q13) Write a program to subtract two 16-bit numbers. 2762 H -1296 H assume R26 = 62 and R27 = 27. Place the difference in R26 and R 27 are 26. Should have the lower byte.
A13)
; R26=62
; R27= 27
LDI R28, 0X96
LDI R29, 0X12
SUB R26, R28
SBC R27, R29
Q14) Assume that the data memory location 0x315 has well do FD H. Write a program to convert it to decimal. Save the digits in location 0X322, 0X 323, zero X3 to 4 and where the least significant digit is in location 0X322
A14)
.EQU HEX_NUM = 0X315
.EQU RMND_L= 0X322
.EQU RMND_M= 0X323
.EQU RMND_H= 0X324
.DEF NUM= R20
.DEF DENOMINATOR = R21
.DEF QUOTIENT = R22
LDI R16, 0XFD
STS HEX_NUM, R16
LDS NUM, HEX_NUM
LDI DENOMINATOR, 10
L1: INC QUOTIENT
SUB NUM, DENOMINATOR
BRCC L1
DEC QUOTIENT
ADD NUM, DENOMINATOR
STS RMND_L, NUM
MOV NUM, QUOTIENT
LDI QUOTIENT, 0
L2: INC QUOTIENT
SUB NUM, DENOMINATOR
BRCC L2
DEC QUOTIENT
ADD NUM, DENOMINATOR
STS RMND_M, NUM
STS RMND_H, QUOTIENT
HERE: JMP: HERE
Q15)
a) Show the results of the following
LDI R20, 0X04
ORI R20, 0X30
b) Assume that PB2 is used to control an outdoor light, and PB5 to control a light inside a building. Show how to turn “on” the outdoor light and turn “off” the inside one.
A15)
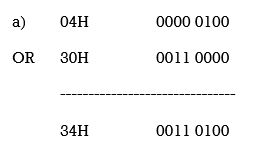
b) SBI DDRB,2; bit 2 of Port B is output
SBI DDRB, 5; bit 5 of Port B is output
IN R20, PORTB
ORI R20, 0b00000100
ANDI R20, 0b11011111
OUT PORTB, R20
HERE: JMP: HERE
Q16) Read and test port be to see whether it has the value 45H. If it does send 99 H to PORTC. Otherwise, it is clear.
A16)
LDI R20, 0XFF; R20 = 0xFF
OUT DDRC, R20; Port C is output
LDI R20, 0X00; R20= 0
OUT DDRB, R20; Port B is input
OUT PORTC, R20; PORTC =00
LDI R21, 0X45; R21= 45
HERE:
IN R20, PINB; get a byte
EOR R20, R21; Ex-or with 0x45
BRNE HERE
LDI R20, 0X99; R20 =0x99
OUT PORTC, R20; POTRC =99H
EXIT: JMP: EXIT; stop here
Q17) Write a program to monitor port. Be continuously for the value 63H. It should stop monitoring only if PORT B equals 63H
A17)
LDI R20, 0X00
OUT DDRB, R20
LDI R21, 0X63
AGAIN:
IN R20, PINB
CP R20, R21
BRNE AGAIN
Q18) Assume that won’t be is an input port connected to a temperature sensor. Write a program to read the temperature and test it for the value 75. According to the test results, place the temperature value into the registers indicated by the following.
If T=75 then R16=T ; R17=0; R18 =0
If T>75 then R16=0 ; R17=T; R18 =0
If T<75 then R16=0 ; R17=0; R18 =T
A18)
LDI R20, 0X00
OUT DDRB, R20
CLR R16
CLR R17
CLR R18
IN R20, PINB
CPI R20, 75
BRSH SAME_HI
MOV R18, R20
RJMP CNTNU
SAME_HI
BRNE HI
MOV R16, R20
RJMP CNTNU
HI:
MOV R17, R20
CNTNU: ………...
Q19) Write a program to add 2 signed numbers. The numbers are in R21 and R 22. The program should store the result in R 21. If the result is not correct, the program should put 0XAA on port. A and clear R21.
A19)
LDI R21, 0XFA
LDI R22, 0X05
LDI R23, 0XFF
OUT DDRA, R23
ADD R21, R22
BRVC NEXT
LDI R23, 0XAA
OUT PORTA, R23
LDI R21, 0X00
NEXT: ……...
Q20) Write a program to transfer the value 41 H serially. WAP in PB 1 put one high at the start and end of the data. Send the LSB first.
A20)
.INCLUDE”M32DEF.INC”
SBI DDRB, 1
LDI R20, 0X41
CLC
LDI R16, 8
SBI PORTB, 1
AGAIN:
ROR R20
BRCS ONE
CBI PORTB, 1
JMP NEXT
ONE: SBI PORTB, 1
NEXT:
DEC R16
BRNE AGAIN
SBI PORTB, 1
HERE: JMP HERE
Q21) WAP that find the number of 1s in a given byte
A21)
.INCLUDE “M32DEF.INC”
LDI R20, 0X97
LDI R30, 0
LDI R16, 8
AGAIN:
ROR R20
BRCC NEXT
INC R30
NEXT:
DEC R16
BRNE AGAIN
ROR R20
HERE: JMP HERE