UNIT 6
QUESTIONS
Q1 Explain queue and its applications?
Queue
Basic features of Queue
Applications of Queue
Implementation of Queue arrangement
Q2 Explain queue operations?
Algorithms for basic primitive operations on queue
1. Insertion at rear
Function: QINSERT_REAR (Q, F, R, N,Y)
Given F and R tips that could the front and rear elements of a queue respectively. Queue Q consisting of N elements. This procedure inserts Y at rear end of Queue.
IF R >= N
2. Then write (‘OVERFLOW’)
Return
[Increment REAR pointer]
R ←R + 1
3. [Insert element ]
Q[R] ←Y
4. [Is front pointer properly set
IF F=0
Then F ←1
Return
2. Deletion from front
Function: QDELETE_FRONT (Q, F, R)
•Given F and R tips that could the front and rear elements of a queue respectively. Queue Q consisting of N elements. This function deleted and element from front of the Queue.
IF F= 0
Then write (‘UNDERFLOW’)
Return (0) (0 denotes an empty Queue)
2. [Decrement element]
Y ←Q[F]
3. [Queue empty?]
IF F=R
Then F←R←0
Else F←F+1 (increment front pointer)
4. [Return element]
Return (Y)
Q3 Explain dequeue and its operations?
Double Ended Queue
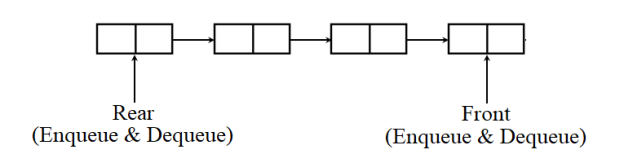
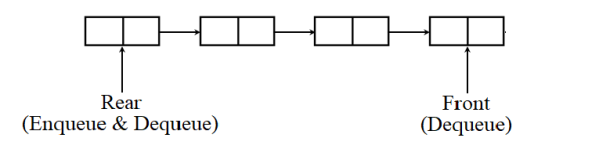
Implementation of Double ended Queue
Here we'll implement a double ended queue employing a circular array. It’ll have the subsequent methods:
1. Insert Elements at Front
When one element is added- 10
Now insert 12 at front
Now insert 14 at front
2. Insert Elements at back
Insert 21 at rear
3. Delete First Element
Delete from front
4. Delete Last Element
Q4 Explain operations in circular queue
Circular Queue
Algorithms for basic primitive operations in Circular Queue
1. Insertion in circular queue
Function: CQINSERT (F, R, Q, N, Y)
Given F and R tips that could the front and rear elements of a circular queue respectively. Circular queue Q consisting of N elements. This procedure inserts Y at buttocks of Circular queue.
If R = N
Then R← 1
Else R ← R + 1
2. [Overflow]
If F = R
Then Write (‘Overflow’)
Return
3. [Insert element]
Q[R] ← Y
4. [Is front pointer properly set?]
If F = 0
Then F ← 1
Return
2. Deletion in circular queue
Function CQDELETE (F, R, Q, N)
Given F and R tips that could the front and rear elements of a Circular queue respectively. Circular Queue Q consisting of N elements. This function deleted and element from front of the Circular Queue. Y is temporary pointer variable.
If F = 0
Then Write (‘UNDERFLOW’)
Return (0)
2. [Delete Element]
Y ←Q[F]
3. [Queue Empty?]
If F = R
Then F ← R ← 0
Return (Y)
4. [Increment front pointer]
If F = N
Then F ← 1
Else F ← F + 1
Return (Y)
Q5 Explain priority queue?
PriorityQueue
Figure:-Priority Queue viewed as a single queue with insertion allowed at any position
Figure: - Priority Queue viewed as a Viewed as a set of queue
Priority Queues
Operations
A max-priority queue also can provide these additional operations:
Implementations
1) Unordered array,a simple, yet inefficient implementation, as retrieving the max element would require searching the whole array.
2) Sorted array,this is not a really efficient implementation either. Inserting a replacement element requires linearly searching the array for the right position. Removing similarly requires a linear time: the remainder of the weather got to be adjusted (shifted) into correct positions.
3) Hash table,although inserting into a hash table takes constant time (given an honest hash function), finding the max element takes linear time. Therefore, this is able to be a poor choice for the underlying arrangement.
4) Heap, it seems that that a heap makes an efficient priority queue.
Q6 Explain circular queue and its advantages?
Circular Queue and its advantage
Figure: -Circular queue
Basic features of Circular Queue
1) In case of a circular queue, head pointer will always point to the front of the queue, and tail pointer will always point to the top of the queue.
2) Initially, the top and therefore the tail pointers are going to be pointing to an equivalent location, this is able to mean that the queue is empty.
3) New data is usually added to the situation pointed by the tail pointer, and once the info is added, tail pointer is incremented to point to subsequent available location.
4) In a circular queue, data isn't actually faraway from the queue. Only the top pointer is incremented by one position when dequeue is executed. Because the queue data is merely the info between head and tail, hence the info left outside isn't a neighborhood of the queue anymore, hence removed.
5) The head and therefore the tail pointer will get reinitialized to 0 whenever they reach the top of the queue.
6) Also, the top and therefore the tail pointers can cross one another. In other words, head pointers are often greater than the tail. This may happen once we dequeue the queue a few of times and therefore the tail pointer gets reinitialized upon reaching the top of the queue.
Q7 What is multiqueue and how it is implemented?
Multiple line queue
Implementation of k queues
Method 1 (Divide the array in slots of size n/k)
Method 2 (A space efficient implementation)
Q8 Write algorithm to insert and delete elements from dequeue?
Insert Elements at Front
When one element is added- 10
Now insert 12 at front
Now insert 14 at front
2. Insert Elements at back
Insert 21 at rear
3. Delete First Element
Delete from front
4. Delete Last Element
Q9 What are the types of queues?
Queues
Rear is that the end of queue at which new element is to be inserted
2. Circular Queue
3. Multi-queues
4. Double Ended Queue
Q10 How queue can be used as linked list?
Queue using link list
To implement queue using linked list,
1. To insert a new node into the queue...
enQueue(value) - Inserting an element into the Queue
2. To delete a node from the queue
deQueue() - Deleting an Element from Queue
3. To display the elements (nodes) of a queue...
display() - Displaying the elements of Queue