Unit - 2
Client Side Technologies: JavaScript and DOM
Q1) Introduce javascripts?
A1) Javascripts
● JavaScript is a lightweight programming language (scripting language).
● JavaScript is used in millions of Web pages to improve the design, validate forms, create cookies, and much more.
● It is most commonly used as a part of web pages, whose implementations allow client-side scripts to interact with the user and make dynamic pages.
● JavaScript consists of lines of executable computer code.
● A JavaScript is usually embedded directly into HTML pages that means it is designed to add interactivity to HTML pages.
● JavaScript is an interpreted language that means that scripts execute without preliminary compilation.
● Everyone can use JavaScript without purchasing a license .
● JavaScript is Netscape’s cross-platform, object-based scripting language for client and server applications.
● JavaScript is the most popular scripting language on the internet, and works in all major browsers, such as Internet Explorer, Mozilla, Firefox, Netscape, Opera.
● JavaScript is not Java means not related to Java other than by name and some syntactic similarities. They are similar in some ways but fundamentally different in others.
● JavaScript enabled shopping carts, form validation, calculations, special graphic and text effects, image swapping, image mapping, clocks, and more.
● JavaScript gives HTML designers a programming tool. JavaScript can read and write HTML elements.
● JavaScript can react to events and can change HTML content, images, styles etc.
● JavaScript works together with HTML/CSS. HTML provides the contents , CSS specifies the presentation and JavaScript programs the behavior.
Are Java and JavaScript the Same?
● TheanswerisNO!
● Java and JavaScript are two completely different languages in both concept and design.
● Java (developed by Sun Microsystems) is a powerful and much more complex programming language - in the same category as C and C++.
● The JavaScript resembles Java but does not have Java’s static typing and strong type checking.
● JavaScript supports most Java expression syntax and basic control-flow constructs.
● JavaScript has a simple, instance-based object model that still provides significant capabilities.
Q2) Write the advantages and disadvantages?
A2) Advantages of JavaScript
Following are some merits of using JavaScript:
● Executed on the client side: For example, you can validate any user input before sending a request to the server. This makes less load on the server.
● No matter where you host JavaScript, Execute always on client environment to save a
● bandwidth and make execution process fast.
● Immediate feedback to the visitors: They don’t have to wait for a page reload to see if they have forgotten to enter something.
● Relatively an easy language: This is quite easy to leam and the syntax that is close to English.
● Fast to the end user: As the script is executed on the user’s computer, depending on task, the results are completed almost instantly.
● Rich interfaces: Drag and drop components or slider may give a rich interface to your site visitors.
● Interactivity increased: Creating interfaces that can react when the user hovers over them or activates them using the keyboard.
● Rapid Development: JavaScript syntax are easy and flexible for the developers. JavaScript small bit of code you can test easily on Console Panel At a time browser interpret return output result. In-short easy language to get picked up in development.
● Browser Compatible: The biggest advantages to a JavaScript having a ability to support all modern browser and produce the same result.
Disadvantages of JavaScript
Following are some demerits of using JavaScript:
● Client-side JavaScript does not allow the reading or writing of files. This has been kept for security reason that is any JavaScript snippets, while appended onto web pages on client side immediately can also be used for exploiting the user’s system.
● Doesn’t have any multiprocessor or multi-threading capabilities.
● As no supports are available, JavaScript cannot be used for any networking applications.
● JavaScript doesn’t have any multithreading or multiprocessor capabilities.
● JavaScript render varies: JavaScript may be rendered by different layout engines differently. As a result, this causes inconsistency in terms of interface and functionality.
● Once again, JavaScript is a lightweight, interpreted programming language that allows you to build interactivity into otherwise static HTML pages.
● Code Always Visible: The biggest disadvantages is code always visible to everyone anyone can view JavaScript code.
● Bit of Slow Execute: No matter how much fast JavaScript interpret, JavaScript DOM (Document Object Model) is slow and will be a never fast rendering with HTML.
Q3) What are the various and datatypes of javascripts?
A3) JavaScript variables, like algebra variables, are used to store values or expressions. A variable can be given a simple name, such as x, or a longer name, such as length. A text value can also be stored in a JavaScript variable, as in carname="Volvo."
Rules for JavaScript variable names:
Case matters when it comes to variable names (y and Y are two different variables) A letter or the underscore character must be the first character in a variable name.
Example
The value of a variable can vary during the execution of a script. To show or update the value of a variable, you can refer to it by its name.
Declaring (Creating) JavaScript Variables
Declaring variables is the most common term for creating variables in JavaScript. The var statement can be used to declare JavaScript variables:
Var x;
Var carname;
The variables have no values after the declaration indicated above, but you can assign values to them while declaring them:
Var x=5;
Var carname="Volvo";
Assigning Values to JavaScript Variables
With JavaScript variables, you may assign values to them.
Assignment statements: x=5;
Carname="Volvo";
The name of the variable appears on the left side of the = sign, and the value you want to give to it appears on the right.
Following the following statements, the variable x will have the value 5, and the variable carname will have the value Volvo.
Assigning Values to Undeclared JavaScript Variables
If you assign values to variables that haven't been declared yet, the variables will be declared automatically. These are the statements:
x=5;
Carname="Volvo"; have the same effect as: var x=5;
Var carname="Volvo";
Redeclaring JavaScript Variables
A JavaScript variable will not lose its initial value if it is redeclared. Var x=5;
Var x;
The variable x will still have the value of 5 after the above statements have been executed. When you redeclare x, the value is not reset (or cleared).
Datatypes
The most fundamental characteristics of a programming language is the set of data types that it supports. These are the type of values that can be represented and manipulated in a programming language .JavaScript is object-oriented. But, It supports both primitive types and objects.
JavaScript allows you to work with three primitive data types -
1. Numbers: takes both integer (e.g., 5566) or floating-point (e.g., 3.14159265).
Examplovar width=20;
2. Strings: a sequence of characters. Strings literals are enclosed in a pair of single quotes or double quotes (e.g., ’’Hello”, ’Welcome’).
Examplovar name = ”Joy”;
3. Boolean: takes boolean literal of either true or false (in lowercase).
Example: 2.7:
Varx = 7;
Vary = 8;
Var z = 7;
(χ == y) ∕∕ Returns false(Boolean)
(χ == z) ∕∕ Returns true (Boolean)
JavaScript also defines two trivial data types, null and undefined, each of which defines only a single value. JavaScript also supports following object types:
1. Object: for general objects.
Example: var z = {name:”Joy”, surname:’’Smith};
2. Null: A special literal value for unallocated object. In JavaScript null means “nothing”. It is supposed to be something that doesn’t exist. You can empty an object by setting it to null.
Example:
Var customer = {name:”Joy”, sumame:”Smith,salary:5000};
Person = null; ∕∕ value is null, but type is still an object
3. Undefined: Is a special value assigned to an identifier after it has been declared but before a value has been assigned to it. In JavaScript, a variable without a value, has the value undefined.
Example:
Var salary; ∕∕ Value is undefined, type is undefined
Any variable can be emptied, by setting the value to undefined. The type will also be
Undefined.
Example
Salary = undefined; ∕∕ Value is undefined, type is undefined
4. The typeof operator: It is undefined. You can use the JavaScript type of operator to find the type of a JavaScript variable. Consider following examples where the typeof operator returns the type of a variable or an expression:
Example:
∕∕ Returns “string”
∕∕ Returns “string”
∕∕ Returns “string”
∕∕ Returns “number”
∕∕ Returns “number”
∕∕ Returns “number”
∕∕ Returns “number”
In JavaScript there is no distinction between integer values and floating-point
Values. All numbers in JavaScript are represented as floating-point values. Unlike most of the general programming languages such as Java/C/C++/C# which are strongly type, JavaScript is loosely type. You do not have to explicitly declare the type of a variable (such as int and float) during declaration. The type is decided when a value is assigned to that variable. If a number is assigned, the variable takes on the number type and can perform numeric operations such as addition and subtraction. If a string is assigned, the variable takes on the string type and can
Perform string operations such as string concatenation. It means JavaScript data types are dynamic in nature. Consider following Example, here the same variable can be used to hold different data types:
Example:
Varz; ∕∕ z is undefined
Var z = 7; /∕z is a Number
Var z = “Joy”// z is a String
Q4) Explain Operators and literals?
A4) Operators
Arithmetic operator
To conduct arithmetic between variables and/or values, arithmetic operators are employed. The table below describes the arithmetic operators given y=5.
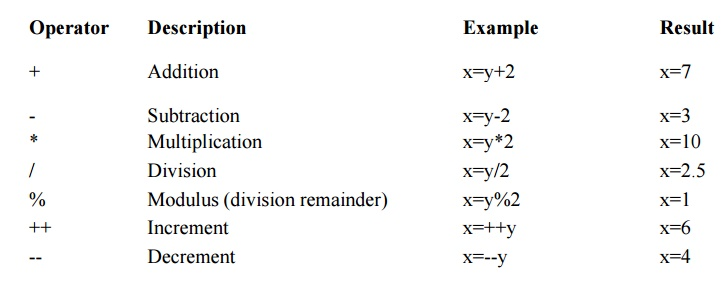
Assignment operators
JavaScript variables are assigned values using assignment operators. The assignment operators are explained in the table below, with x=10 and y=5.
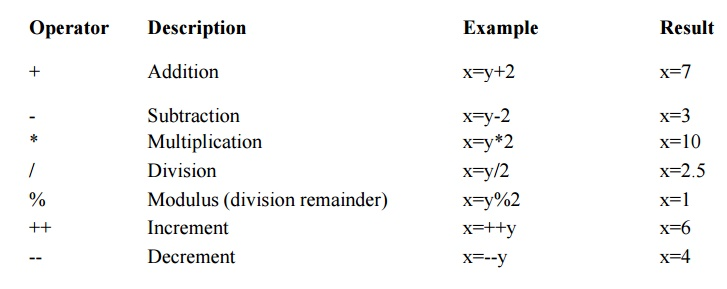
Comparison operators
In logical assertions, comparison operators are used to assess if variables or values are equal or different. The table below explains the comparison operators assuming x=5.
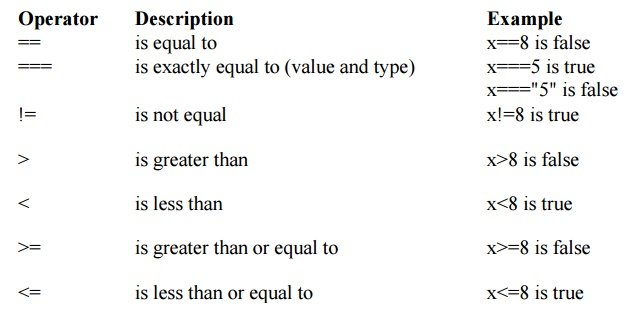
Conditional operator
A conditional operator in JavaScript assigns a value to a variable based on some condition.
Syntax
Variablename=(condition)?val ue1:value2 Example
Greeting=(visitor=="PRES")?"Dear President ":"Dear ";
If the variable visitor is set to "PRES," the variable greeting will be set to "Dear President," else it will be set to "Dear."
If the variable visitor is set to "PRES," the variable greeting will be set to "Dear President," else it will be set to "Dear."
In JavaScript, conditional statements are used to do different actions based on various criteria.
Literals
The literal values you type into mathematical or textual expressions are known as literal values. Consider the numbers 23 (integer), 12.32E23 (floating point), or 'flopsy the Hamster' (a string).
Single or double quotes can be used to enclose string literals. For
Example: 'literal string' "literal string"
'literal string with "double quotes" inside'
In literal strings, you can utilise five special characters. In the table below, you'll find a list of these.
Reference Meaning
\b Backspace
\f Form Feed
\n New Line
\r Carriage Return
\t Tab
Q5) What do you mean by functions?
A5) Functions
● A JavaScript function is a block of code designed to perform a particular operation. A function is a group of reusable code which can be called anywhere in your program.
● It is executed when you call it. A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses ().
● Function names can contain letters, digits, underscores, and dollar signs.
● The parentheses may include parameter names separated by commas. The code that you want to execute by the function is kept inside curly brackets.
● The code inside the function will execute when it is invoked by something.
Syntax
Functionname(parameter_l,parameter_2.. .parametern)
{
Code to be executed
}
● A Function is similar to a Procedure or a Subroutine like other programming languages.
● Function parameters are the names listed in the function definition.
● Function parameters are the real values received by the function when it is invoked.
● Inside the function, the parameters behave as local variables. Consider following example:
Example
FunctiondemoFunction(xl, x2) {
Return xl + x2; H This function returns the addition of xl and x2
● The function will stop executing when JavaScript reaches a return statement. Functions often compute a return value. The return value is "returned” back to the ’’caller” program.
Example
Calculate the addition of two numbers, and return the result:
Varp = demoFunction(6,7); ∕∕ Function is called, return value will end up in p
FunctiondemoFunction(x, y)
{
Return x +y; ∕∕ returns the addition of x and y
}
Function Definition
Define a function before using it.In JavaScript function keyword is used to define a function followed by a unique function name, a list of parameters that might be empty, and a block of code to be executed enclosed in a set of curly braces.
Syntax
<script type- , text∕JavaScripf>
<!--
Functionfunction_name(parameter-list)
{
Code to be executed
}
//->
<∕script>
Example
Defines a function called display with no parameters:
<script type=”text/javascript”>
<!--
Functiondisplay()
{
Document.write(”Welcome”);
}
//->
<∕script>
Example
Try the following example. It defines a function that takes two parameters and concatenates them before returning the resultant in the calling program.
<html>
<head>
<script type=”text/javascript”>
Functionaddition(x,y)concatenate(fιrst, last) varz;
z=x+y;
Retumz;
Function Show()
{
Var result;
Result=addition(7,9);
Document.write (result);
}
<∕script>
<∕head>
<body>
<p>To execute program click the following button<∕p>
<form>
<input type-’button” onclick=”Show()” value=”Display Result’”
<∕form>
<∕body>
<∕html>
Output
To execute program click the following button
Display Result
After clicking on the above button, it will display the addition of two numbers.
Q6) Write about objects?
A6) Objects
● A number of predefined objects associated with the web browser and the HTML document loaded are present. JavaScript is an Object Oriented Programming (OOP) language. It is having basic features like:
○ Inheritance: The capability of a class to depend on another class or number of classes for some of its properties and methods.
○ Encapsulation: The capability to store related information, data or methods, together in an object.
○ Aggregation: The capability for storing one object inside another object.
○ Polymorphism: The capability to write one function which works in a different manner.
● Objects are composed of attributes. If an attribute contains a function, it is considered to be a method of the object, otherwise the attribute is considered as a property and each of these objects has certain properties associated with them.
● JavaScript objects belongs to following classes:
○ Navigator Objects that mostly correspond to HTML tags
○ Built-in Language Objects
Object Properties
The name: values pairs in JavaScript objects are called properties. Object properties can be primitive data types. Object properties are usually variables that are used internally in the object’s methods.
Syntax for adding a property to an object is -
ObjectName-ObjectProperty = propertyValue;
Example:
Var student = {RollnoT0, Name: “Amit”, age:20};
The above example, Rollno, name, age represents different properties and 10 ,“Amit” and 20 represents their values respectively.
Object Methods
Objects also can have methods which are distinguished from properties by the use of the open and close parentheses. Methods are stored in properties as function definition. Methods are actions that can be performed on objects. There is a small difference between a function and a method.
Function is a standalone unit of statements and a method is associated with an object and can be referenced by this keyword.
Example:
Document. Write( , “Welcome to JavaScript”);
In above example, write() method of document object is used to write the content.
User-Defined Objects
All user-defined objects and built-in objects are descendants of an object called Object.
(a) The new Operator
The new operator is used to create an instance of an object. The new operator is used to create an object.
Following are some constructors which are built-in JavaScript functions.
Var customer = new Object();
Var accessories = new Arrayf’Pendrive”, “Keyboard”, ’’Mouse”);
Var day = new Date(“December 20,2017”);
(b) The Object() Constructor
To build the object, JavaScript provides a special constructor function called Object() and the return value of the Object() constructor is assigned to a variable. The following example shows how to create an Object.
Example
<html>
<head>
<title>User-defmed objects<∕title>
<script type=”text/javascript”>
Var student = new Object(); ∕∕ Create the object
Studentname = ’’Amit”; ∕∕ Assign properties to the object student.course = ’’Computer”;
<∕script>
<∕head>
<body>
<script type=”text/javascript”>
Document.write( π Student Name is ” + Studentname + ”<br>”);
Document.write( π Student Course is” + Studentcourse + ”<br>”);
<∕script>
<∕body>
<∕html>
Output
Student Name is : Amit
Student Course is : Computer
The following example illustrates the use of user defined functions to create an object by using this keyword.
(c) Define Methods for an Object
The definition of an object by assigning methods to it is illustrated in following example:
Example:
<html>
<head>;
<title>Example of an User-defined objects<;∕title>
<script type=”text/javascript”>
∕∕ Define a function functionaddmarker(marks) { this.grade = marks;
}
Function student(name,course)
{ this.name = name;
This.course = course;
This.addmarks = addmarks;// Assign that method as property.
}
<∕script>
<∕head>
<body>
<script type=”text/javascript”>
Var studl = new student(“Amit”, ’’Computer”);
Stud 1. Addmarks( 9 5);
Document.write( π Student Name is ” + Studentmame + ”<br>”);
Document.write( π Student Course is” + student.course + ”<br>”);
Document.write( π Student Grade is : ” + Studentgrade + ”<br>”);
<∕script>
<∕body>
<∕html>
(d)Use of ‘with’ Keyword
The ‘with’ keyword is used for addressing an object’s properties or methods. The object specified as an argument to with becomes the default object for the duration of the block that follows. The syntax for with object is as follows -
Syntax
With (object) {properties used without the object name and dot
}
Example:
Try the following example.
<html>
<head>
<title>Example of an User-defined objects<∕title>
<script type=”text/javascript”>
∕∕ Define a function
Functionaddmarks(marks) {
With(this){
Grade = marks;
}
}
Function student(name,course)
{
This.name = name;
This.course = course;
This.addmarks = addmarks; ∕∕ Assign that method as property. This.grade=O;
}
<∕script>
<∕head>
<body>
<script type=”text/javascript”>
Var studl = new studentf’Amit”, ’’Computer”);
Stud 1. Addmarks( 9 5);
Document.write(“Student Name is ” + student.name + ”<br>”);
Document.write(“Student Course is” + student.course + ”<br>”);
Document.write(“Student Grade is ” + student.grade + ”<br>”);
<∕script>
<∕body>
<∕html>
Output
Student Name is :-Amit
Student Course is Computer
Student Grade is 95
Q7) Describe array?
A7) Arrays
An array is a special variable, which can hold more than one value at a time. If you have a list of fruit items, you can represent them in following manner:
Var frl = ’’Apple”;
Var fr2 = “Banana”;
Var fr3 = ’’Orange”;
By using an array, you can hold many values under a single name, and you can access the values by referring to an index number. The syntax for creating an array is shown below:
Syntax:
Vararray_name = [iteml, item2,...];
Example :
Var fruits = [’’Apple”, ’’Banana”, ’’Orange”];
Arrays in Javascript are also allocated using the new keyword.
Names = new Array(20);
Numbers = new Array(6);
Array indexes start at O and extend to the size of the array minus 1. To assign a value to an element of an array open and close brackets are used.
Names[O] = ’’Apple”;
Names[l] = “Banana”;
Names[9] = ’’Grapes”;
The size of an array can be increased dynamically by assigning a value to an element pass the end of the array. Arrays can be created that initially have no elements at all. In addition, they are not of a fixed size but can grow dynamically.
Consider the following array creation in which an array “Host” initially has no elements.
Host = new Array();
While in following example, the Host array is initialized with “Keyboard”
Host[20] = “Keyboard”;
After ’’Keyboard” has been assigned the array has 21 elements. The unassigned elements are set to Undefined. The length property of arrays returns the number of elements in the array.
Example
<html>
<head>
<title>JavaScript Example<;∕title>
<script Ianguage= n JavaScript 1.2”>
<!--
Names[O] = ’’Apple”;
Names[l] = ’’Banana”;
Names[9] = ’’Grapes”;
Document.write(“<br>names[0] - ” + names[0]);
Document.write(“<br>names[l] - ” + names[l]);
Document.write(“<br>names[2] - ” + names[2]);
Host = new Array();
Host[20] = ’’Keyboard”;
Document.write(“<br>Host[20] - ” + Host[20]);
Document.write(“<br>Host[9] - ” + Host[9]);
Document.write(“<br>Host.length - ” +HostJength);
//->
<∕script>
<∕head>
<body>
<∕body>
<∕html>
Output
Names[0] -Apple
Names[l] - Banana
Names[9] - undefined
Host[20]-Keyboard
Host[9]-undefιned
HostJength-21
You can access the full array using JavaScript in following manner:
Var fruits = [’’Apple”, “Banana”, ’’Orange”];
Document.getElementById(”sample”).innerHTML = fruits;
Arrays are Objects
The type of operator in JavaScript returns ’’object” for arrays. Arrays use numbers to access its ’’elements”. In following example which represents an array, fruits[O] returns Apple:
Var person = [’’Apple”, ’’Mango”,’’Kiwi”];
Objects use names to access its ’’members”. So in following example, fruits, first returns Apple:
Varperson= {first:’’Apple”, second:”Mango”,third:”Kiwi”};
Q8) What is DOM?
A8) DOM
● The Document Object Model (DOM) is a programming API for HTML and XML documents, it is a W3c standard. The Document Object Model can be used with any programming language.
● It defines how XML documents can be programmatically accessed and manipulated. It is a platform- and language-neutral interface.
● The Document Object Model (DOM) is a cross-platform and language independent APT that treats an HTML, XML document as a tree structure in which each node is an object representing a part of the document.
● Following fig. shows the structure of the DOM tree.
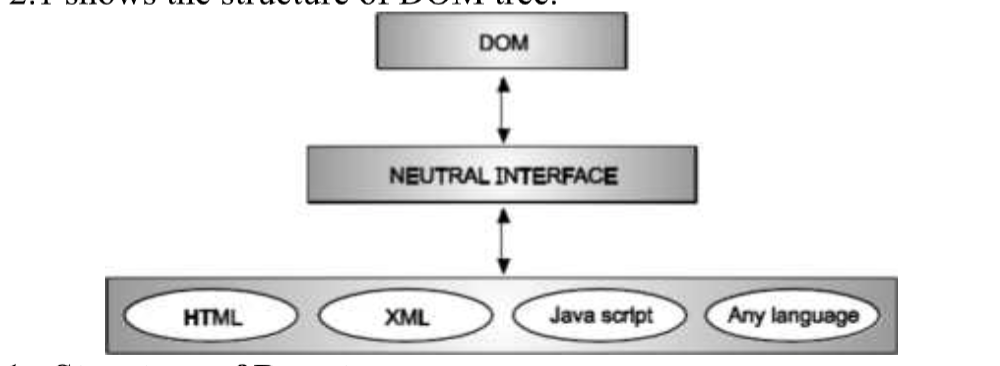
Fig 1: Structure of DOM tree
● It defines the logical structure of documents and the way a document is accessed and manipulated. With the Document Object Model, programmers can create and build documents, navigate their structure, and add, modify, or delete elements and content.
● Anything found in an HTML or XML document can be accessed, changed, deleted, or added using the Document Object Model.
● It also represents an XML document as a tree structure. It provides access to XML document structure through a set of objects.
● Writing an application that accesses an XML document through DOM requires:
○ AnXMLParser
○ DOM
● Using DOM to create and manipulate XML documents helps avoid:
○ Unclosedtags
○ Improperly Nested Tags
● DOM helps easily move information between a database and an XML file.
Although the DOM is not a language, there can be several language bindings for it
● In particular, there is a Javascript binding.
● That is, one can access the DOM from JavaScript
Q9) Define DOM history and levels?
A9) The World Wide Web Consortium (W3C) created the Document Object Model to give programming languages access to a Web document's underlying structure. A computer can use the DOM to access any element in the page, determine and change attributes, and even remove, add, or rearrange elements at will.
It's vital to remember that the DOM is an application programme interface (API) that gives any programming language access to a Web document's structure. The ability to manipulate a page without having to go to the document's server is the fundamental benefit of using the DOM. As a result, client-side technologies like JavaScript often access and use the DOM.
At the same time as JavaScript and early browsers, the first DOM specification (Level 0) was created. From Netscape 2 onwards, it is supported.
Netscape 4 and later, as well as Microsoft Internet Explorer (IE) versions 4 and 5, allowed two intermediate DOMs. The two sides of the browser coin — Netscape and Microsoft Internet Explorer — had their own DOMs. The former made use of a collection of elements that were linked together in a document. The former used a document.all object, while the latter used a layers object. A script should try to cover both of these DOMs rather than just one to be truly cross-browser compatible.
Level
DOM Levels are the versions of the specification for defining how the Document Object Model should work. W3C specifies the W3C DOM Recommendation which is a model for parsing XML documents. The three levels of DOM interface are having following status:
1. The W3C DOM 1 Recommendation (DOMl):- Oct. 1998
Interfaces for representing XML and HTML document
● Document
● Element
● Attribute
● Node
● Text interfaces
DOM Core Interfaces
Fundamental interfaces
● basic interfaces to structured documents
● Extended interfaces
○ XML specific: CDATASection, DocumentType, Notation, Entity, EntityReference, Processinginstruction
II: DOM HTML Interfaces
● More convenient to access HTML documents
● Level 1: basic representation and manipulation of document structure and content (No access to the contents of a DTD)
2. The W3C DOM 2 Recommendation (DOM2):- Nov.2000
It contains following different specifications
● D0M2 core
● Views
● Events
● Style
● Traversal and Range
● D0M2HTML
DOM Level 2 adds
● Support for namespaces
● Accessing elements by ID attribute values
● Interfaces to document views and style sheets
● An event model for example: user actions on elements
● Methods for traversing the document tree and manipulating regions of document
3. The W3C DOM 3 Recommendation (DOM3):- January 2002
It contains following different specifications
● D0M3 core
● Load and Save
● Events
● Validation
● Xpath
Q10) How to modify element style?
A10) One of the ways the DOM can be utilised is to change an element's style (CSS) attributes. The onfocus event handler method, for example, is used in the code below to alter the style of the input> element when the user clicks inside it. The element's background colour will change to purple when this event occurs.
<!DOCTYPE html>
<html>
<body>
Email: <input type="text" id="outline" onfocus="background()">
<p>Click in the text input to focus the element.<br/>
This will trigger the 'background' function, which changes the element's background color to purple.</p>
<script>
Function background() {
Document.getElementById("outline").style.background = "purple";
}
The DOM is used to make all of the changes mentioned in this lecture. Take note of the document. In the example, the getElementById() function is part of the JavaScript function. The getElementById() method informs the browser to isolate only the HTML element with a specified ID, in this case outline, from the rest of the HTML document. The style method is used to define the intended change once the desired element has been identified.
Q11) What do you mean by document tree?
A11) When a browser loads a page, it produces a hierarchical representation of its contents that closely follows the structure of the HTML code. This creates a tree-like structure of nodes, each of which represents an element, attribute, content, or other object.
Nodes
These many object kinds will each have their own set of methods and properties. However, each implements the Node interface as well. This is a collection of methods and properties that are all linked to document tree structure. Take a look at the diagram below, which depicts a simple node tree, to better comprehend this interface.
The Document Root
The root of this node tree is the document object. The Node interface is implemented as well. As the initial node, it will have child nodes but no parent or sibling nodes. It implements the Document interface in addition to being a Node.
Other nodes in the document tree can be accessed and created using this interface. Here are a few examples:
+ getElementById()
+ getElementsByTagName()
+ createElement()
+ createAttribute()
+ createTextNode()
Q12) What is JQuery?
A12) JQuery
● JQuery is a fast and concise JavaScript Library created by John Resig in 2006 with a nice motto - Write less, do more, jQuery simplifies HTML document traversing, event handling, animating, and Ajax interactions for speedy website development with an easy-to-use API that works across a multitude of browsers.
● With a combination of versatility and extensibility, jQuery has changed the way that millions of people write JavaScript. JQuery greatly simplifies JavaScript programming. It is easy to learn.
● JQuery is a JavaScript toolkit designed to simplify various tasks by writing less code. Here i the list of important core features supported by jQuery -
● DOM Manipulation: The jQuery made it easy to select DOM elements, traverse them and modifying their content by using cross-browser open source selector engine called Sizzle.
● Event Handling: The jQuery offers an well-designed way to capture a wide variety of events, such as a user clicking on a link, without the need to clutter the HTML code itself with event handlers.
● AJAX Support: The jQuery helps you a lot to develop a responsive and feature-rich site using AJAX technology.
● Animations: The jQuery comes with plenty of built-in animation effects which you can use in your websites.
● Lightweight: The jQuery is very lightweight library
● Cross Browser Support: The jQuery has cross-browser support, and works well in IE 6.0+, FF 2.0+, Safari 3.0+, Chrome and Opera 9.0+
● Latest Technology: The jQuery supports CSS3 selectors and basic XPath syntax.
Why jQuery?
● There are lots of other JavaScript frameworks in the market, but jQuery seems to be the most popular, and also the most extendable.
● The purpose Of jQuery is to make it much easier to use JavaScript on website.
● jQuery takes a lot of common tasks that require many lines of JavaScript code to accomplish, and wraps them into methods that you can call with a single line of code.
● jQuery also simplifies a lot of the complicated things from JavaScript, like AJAX calls and DOM manipulation.
Q13) Describe AngularJS?
A13) AngularJS is a free and open-source framework for building online applications. Misko Hevery and Adam Abrons came up with the idea in 2009. It is now Google's responsibility to keep it up to date. The most recent version is 1.2.21.
According to its official documentation, AngularJS is defined as follows:
“AngularJS is a structural framework for dynamic web applications. It lets you use HTML as your template language and lets you extend HTML's syntax to express your application components clearly and succinctly. Its data binding and dependency injection eliminate much of the code you currently have to write. And it all happens within the browser, making it an ideal partner with any server technology.”
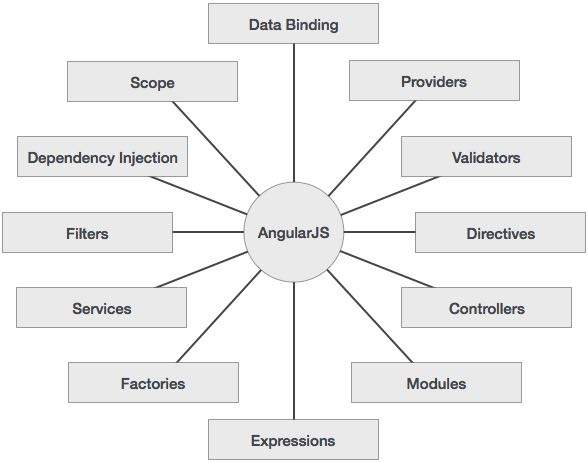
Fig 2: Parts of AngularJS
General features
The following are some of AngularJS's main features:
● AngularJS is a powerful framework for building Rich Internet Applications (RIA).
● AngularJS gives developers the ability to construct elegant Model View Controller (MVC) client-side apps using JavaScript.
● AngularJS-based applications are cross-browser compatible. AngularJS processes JavaScript code in a browser-agnostic manner.
● AngularJS is an open source framework that is absolutely free to use, and it is utilised by thousands of developers all over the world. It is distributed under the Apache License 2.0.
AngularJS is a framework for developing large-scale, high-performance, and simple-to-maintain web applications.
Q14) Write the advantages and disadvantages of AngularJs?
A14) Advantages
AngularJS has a number of advantages.
● It allows you to design Single Page Applications that are both tidy and easy to maintain.
● It gives HTML the ability to bind data. As a result, the user has a rich and responsive experience.
● The code in AngularJS may be unit tested.
● Separation of concerns and dependency injection are used in AngularJS.
● Reusable components are provided by AngularJS.
● Developers can get greater functionality with less code with AngularJS.
● Views in AngularJS are simply HTML pages, with business processing handled by controllers written in JavaScript.
On top of that, AngularJS applications are compatible with all major browsers and smartphones, including Android and iOS devices.
Disadvantages
AngularJS has a lot of advantages, however there are a few things to be concerned about.
● Not secure - Because AngularJS is a JavaScript-only framework, applications created with it are not secure. To keep an application safe, server-side authentication and permission are required.
● Not degradable - If the user of your application disables JavaScript, only the basic page will be viewable.
Q15) Write the code for adding new elements dynamically?
A15) Code
<html>
<head>
<title>t1</title>
<script type="text/javascript">
Function addNode () { var newP = document. CreateElement("p");
Var textNode = document.createTextNode(" This is a new text node");
NewP.appendChild(textNode); document.getElementById("firstP").appendChild(newP); }
</script> </head>
<body> <p id="firstP">firstP<p> </body>
</html>
Q16) How closures work in JavaScript?
A16) The closure is a locally declared variable related to a function that stays in memory when it has returned.
For example:
Function greet(message) {
Console.log(message);
}
Function greeter(name, age) {
Return name + " says howdy!! He is " + age + " years old";
}
// Generate the message
Var message = greeter("James", 23);
// Pass it explicitly to greet
Greet(message);
This function can be better represented by using closures
Function greeter(name, age) {
Var message = name + " says howdy!! He is " + age + " years old";
Return function greet() {
Console.log(message);
};
}
// Generate the closure
Var JamesGreeter = greeter("James", 23);
// Use the closure
JamesGreeter();
Q17) How to Changed Font and Colour Of A Text Element?
A17) You can change font and text of an element by using a function.
Example:
<html>
<head>
<script type="text/javascript">
Function ChangeText()
{
Document.getElementById("welcome").style.color="red";
Document.getElementById("welcome").style.fontFamily="Times New Roman";
Document.getElementById("welcome").style.fontSize="20";
}
</script>
</head>
<body>
<p id="welcome">R4R Welcomes You!</p>
<input type="button" onclick="ChangeText()"
Value="When you click on button text font and color will change">
</body>
</html>