Unit - 4
JSP and Web Services
Q1) Introduce JSP?
A1) JSP
● Java Server Pages (JSP) technology provides a simplified, fast way to create web pages that display dynamically-generated content. It is a technology used to create web applications.
● The JSP specification, developed through an industry-wide initiative led by Sun Microsystems in June, 1999, defines the interaction between the server and the JSP page, and describes the format and syntax of the page.
● JSP pages share the & quot; Write Once, Run Anywhere & quot; characteristics of Java technology. Java Server Pages are HTML pages embedded with snippets of Java code.
● It is an inverse of a Java Servlet. It provides additional features such as expression language, JSTL (JSP Standard Tag Library), custom tags etc.
● A JSP page is a page created by the web developer that includes JSP technology-specific and custom tags, in combination with other static (HTML or XML) tags.
● The JSP pages are easier to maintain than servlet because we can separate the designing and development part.
● A JSP page has the extension .jsp or .jspx. This signals to the web server that the JSP engine will process elements on this page. Using the web.xml deployment descriptor, additional extensions can be associated with the JSP engine.
● Java Server Pages often provides the same purpose as that of Common Gateway Interface (CGI). But JSP offers several advantages in comparison with the CGL.
● JSP allows embedding Dynamic Elements in HTML Pages itself instead of having separate CGI files which increases the performance.
● They are always compiled before they are processed by the server.
● Java Server Pages are built on top of the Java Servlets API, so JSP has access to all the powerful Enterprise Java APIs, including JDBC, EJB etc.
● Java Server Pages (JSP) is a technology which is used to develop web pages by inserting JAVA code into the HTML pages by making special JSP tags.
● The JSP tags which allow java code to be included into it are <% —-java code-—%>. Java Server Pages (JSP) separates the dynamic part of your pages from the static HTML.
Q2) Write any example of JSP?
A2) Example
HTML tags and text
<% Place JSP code here %>
HTML tags and text
i.e.
<U>
<%= request.getParameter("Welcome”) ⅝>
<∕U>
Q3) Write the difference between JSP and Servlet?
A3) Difference between JSP and servlet
JSP | Servlets |
JSP is an HTML-based programming language. | A java code is known as a servlet. |
Because JSP is java in HTML, it is simple to code. | Writing servlet code is more difficult than writing JSP code since servlet is html in Java. |
In the MVC architecture, the view for displaying output is JSP. | In the MVC paradigm, the servlet serves as a controller. |
Because the first step in the JSP lifecycle is to translate JSP to Java code and then compile, JSP is slower than Servlet. | Servlet is faster than JSP. |
Only http requests are accepted by JSP. | All protocol requests are accepted by Servlet. |
We can't override the service() method in JSP. | We can override the service() method in Servlet. |
Session management is enabled by default in JSP. | Session management is not enabled by default in Servlet; users must specifically enable it. |
JavaBeans are used to segregate business logic from presentation logic in JSP. | Everything, including business logic and display logic, must be implemented in a single servlet file in Servlet. |
JSP alteration is simple; all you have to do is click the refresh button. | Because Servlet modification necessitates reloading, recompiling, and restarting the server, it is a time-consuming process. |
Q4) What do you mean by java bean classes?
A4) A JavaBean is a custom Java class developed in Java and coded to the JavaBeans API requirements.
The following are the properties that set a JavaBean apart from other Java classes:
● It has a no-argument default function Object() { [native code] }.
● Serializable and capable of implementing the Serializable interface are required.
● It may have a variety of read-only or write-only characteristics.
● The properties may have a number of "getter" and "setter" methods.
JavaBeans property
A JavaBean property is a named attribute that the object's user can access. Any Java data type, including the classes you define, can be used for the attribute.
A property on a JavaBean can be read, write, read only, or write only. Two methods in the JavaBean's implementation class are used to access JavaBean properties.
● getPropertyName() - To read a property named firstName, for example, your method would be called getFirstName(). Accessor is the name of this technique.
● setPropertyName() - For example, if the property name is firstName, your method name to write that value would be setFirstName(). Mutator is the name of this method.
A read-only property will have only one method, getPropertyName(), and a write-only attribute will have only one method, setPropertyName().
Why use JavaBean?
It is a reusable programme component, according to the Java white paper. A bean wraps numerous things into a single object that may be accessed from multiple locations. Furthermore, it is simple to maintain.
Simple example of JavaBean class
//Employee.java
Package mypack;
Public class Employee implements java.io.Serializable{
Private int id;
Private String name;
Public Employee(){}
Public void setId(int id){this.id=id;}
Public int getId(){return id;}
Public void setName(String name){this.name=name;}
Public String getName(){return name;}
}
How to access the JavaBean class?
We should utilize getter and setter methods to access the JavaBean class.
Package mypack;
Public class Test{
Public static void main(String args[]){
Employee e=new Employee();//object is created
e.setName("Arjun");//setting value to the object
System.out.println(e.getName());
}}
Q5) Write the advantages and disadvantages of java beans?
A5) Advantages of JavaBean
The following are some of JavaBean's benefits:/p>
● Another programme can access the JavaBean attributes and methods.
● It makes reusing software components more easier.
Disadvantages of JavaBean
The following are some of JavaBean's drawbacks:
● JavaBeans can be changed. As a result, it is unable to benefit from immutable objects.
● The boilerplate code may result from creating the setter and getter methods for each property independently.
Q6) Define MVC (model view controller)?
A6) The model, view, and controller (MVC) paradigm splits a web application's logic into three discrete regions, or concerns. Each concern, in practise, has numerous components and files. Model classes, view templates, and controller classes are all present in a typical MVC application.
Here's how the various concerns operate:
Model - Logic is applied to data. Essentially, the model is responsible for the application's business logic as well as any other logic that isn't present in the display or controller. Data is represented and manipulated by model objects. The model in the CMS environment is based on XML data.
View - Data from the model is displayed. The view layer creates the application's user interface. A view template generates an HTML page to be served to a client using markup and JavaScript.
Controller - Handles the application's input logic. In response to user input, the controller transmits queries from the user to the model, and controller methods call views.
MVC is popular because it separates the application logic and user interface layer, allowing for separation of concerns. The Controller receives all application requests and then works with the Model to prepare any data that the View requires. The View then generates a final presentable response using the data prepared by the Controller. The MVC abstraction can be represented graphically as follows.
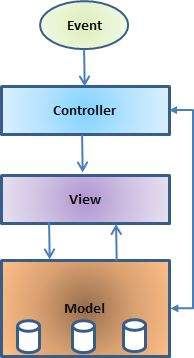
Fig 1: MVC
Q7) Write the advantages and disadvantages of MVC?
A7) Advantages
The following are some of the primary advantages of employing MVC architecture:
● Easy-to-maintain code that can easily be extended and expanded.
● Separately from the user, the MVC Model component can be tested.
● Support for new sorts of clients is made easier.
● Parallel development of the various components is possible.
● By splitting an application into three components, it aids in the avoidance of complexity. There's a model, a view, and a controller.
● It solely employs the Front Controller design, which uses a single controller to execute web application requests.
● Provides the best test-driven development support.
● It works effectively for online apps that have huge teams of web designers and engineers supporting them.
● Allows for a clear separation of concerns (SoC).
● Friendly to Search Engine Optimization (SEO).
● All classes and objects are self-contained, allowing you to test them independently.
● The MVC design pattern allows for the logical grouping of related controller actions.
Disadvantages
● This model is difficult to read, alter, unit test, and reuse.
● The navigation of the framework can be complicated at times since it introduces additional layers of abstraction, requiring users to adjust to the MVC decomposition requirements.
● There is no official validation support.
● Data has become more complex and inefficient.
● The challenge of using MVC with today's user interface.
● Parallel programming necessitates the use of numerous programmers.
● It is necessary to have a broad understanding of a variety of technologies.
● Keeping track of a large number of codes in the controller.
Q8) What are the features of MVC?
A8) Features of MVC
● Testability is simple and painless. Framework that is highly tested, extensible, and pluggable.
● The MVC pattern gives you complete control over your HTML and URLs when creating a web application architecture.
● Utilize existing features like as ASP.NET, JSP, Django, and others.
● Model, View, and Controller are clearly separated in terms of logic. Application activities, including as business logic, Ul logic, and input logic, are separated.
● Routing URLs for SEO-Friendly URLs URL mapping that is both comprehensible and searchable.
● Test-Driven Development (TDD) is aided by the following resources (TDD).
Q9) What is jsp related technology?
A9) JSP stands for JavaServer Pages, and it's a technique for creating dynamic and data-driven pages for your Java online applications. The Java Servlet specification governs JSP. Servlet and JSP technologies are used jointly in older Java projects. When writing servlets, you create Java code and include client-side markup (such as HTML) in it. You write the client-side script or markup and include JSP tags to connect your page to the Java backend while writing JSP.
HTML, XML, SOAP, and other document types are used to create JavaServer Pages.
In a word, JSP technology is a Servlet-based extension. We can use all of the Servlet's functionalities there. In addition, implicit objects, predefined tags, expression language, and Custom tags are all available in JSP, making JSP programming simple.
JSP, like Servlets, is hosted on a web server. Apache Tomcat, Jetty, Apache TomEE, Oracle WebLogic, WebSphere, Apache Geronimo, and others are examples of web servers. JSP is a Java servlet abstraction. JSP is a Servlet since it is transformed into Servlets at runtime.
The JavaServer Pages can be utilised on their own. It can also be used as the view component in a model–view–controller paradigm. It's typically used as a controller with the JavaBeans paradigm and Java servlets (or a framework like Apache Struts).
The JavaServer Pages technology allows you to create online content that includes both static and dynamic elements. Although JSP technology offers dynamic capabilities, it is a more natural way to create static information.
The following are the primary characteristics of JSP technology:
● JSP pages are text-based documents that outline how to process and generate a response to a request.
● You can use an expression language in JSP to access server-side objects.
● The JSP language has facilities for creating extensions.
The following are some of the benefits of JavaServer Pages over Servlets:
In JSP, we can use all of the Servlet's functionalities. In addition, implicit objects, predefined tags, expression language, and Custom tags are all available in JSP, making JSP programming simple.
JSP is less difficult to maintain. It's simple to manage because the business logic and presentation layers are readily separated. We combine our business and presentation logic with Servlet technology.
JSP development is faster than Servlet development because you don't have to recompile and redeploy them. We don't need to recompile and redeploy the project if the JSP page is changed.
Servlet technology has more code than JavaServer Pages technology. Many tags, such as action tags, JSTL, custom tags, and so on, can be used there. All of these things help to cut down on code. We can also employ expression language, implicit objects, and a variety of other features.
Because JavaServer Pages are based on the Java Servlets API, they have access to all of Enterprise Java's advanced technologies, such as JDBC, JNDI, EJB, JAXP, and so on.
JSP is part of the Java EE platform, which is a full platform for enterprise-class applications. This means that JSP may be used in the simplest as well as the most complicated and demanding applications.
Q10) What is the concept of web services?
A10) The Internet is the worldwide interconnection of hundreds of thousands of different types of computers that are connected to many networks. A web service is a defined technique for propagating messages between client and server applications on the World Wide Web. A web service is a software module that is designed to perform a set of specialised tasks. In cloud computing, web services can be found and invoked over the internet.
The client that invoked the web service would be able to receive functionality from the web service.
A web service is a collection of open protocols and standards that allow data to flow across different applications or systems. Web services can be used by software programmes written in a number of programming languages and running on a range of platforms to exchange data via computer networks like the Internet, much like inter-process communication on a single machine.
A web service is any software, application, or cloud technology that connects, interoperates, and exchanges data messages – often XML (Extensible Markup Language) – across the internet using standardised web protocols (HTTP or HTTPS).
Web services have the benefit of allowing programmes written in various languages to communicate with one another by sending data between clients and servers via a web service. A client makes an XML request to a web service, and the service responds with an XML response.
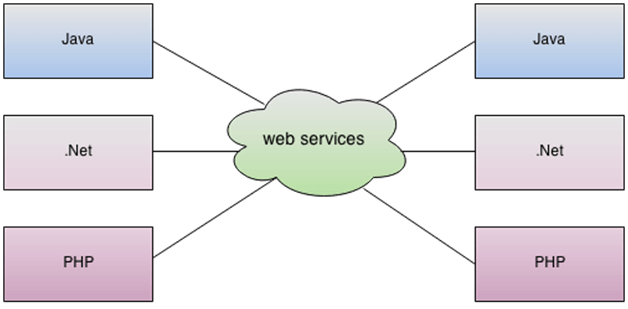
Fig 2: Web service
As seen in the diagram, Java, .net, and PHP programmes can connect with each other across the network via web services. The Java application, for example, can communicate with Java, .Net, and PHP applications. As a result, a web service is a language-independent communication method.
Working of web service
A web service uses open standards like HTML, XML, WSDL, and SOAP to allow communication between different applications. A web service relies on the assistance of
● XML to tag the data
● SOAP to transfer a message
● WSDL to describe the availability of service.
On Solaris, you can create a Java-based web service that can be accessed from your Windows Visual Basic programme.
You may also use C# to create new web services on Windows that can be called from your JavaServer Pages (JSP)-based web application running on Linux.
Q11) What are the components and features of web services?
A11) Features
● It can be accessed through the internet or intranet networks.
● A standardised XML communicating protocol.
● Independent of the operating system or programming language.
● It is self-descriptive when using the XML standard.
● It can be found using a simple locating method.
Components
XML + HTTP is the most fundamental web services platform. The following components are used by all typical web services:
● SOAP (Simple Object Access Protocol)
● UDDI (Universal Description, Discovery and Integration)
● WSDL (Web Services Description Language)
Q12) Describe WSDL?
A12) Web Services Description Language (WSDL) is an acronym for Web Services Description Language. It's the industry standard for characterising web services. Microsoft and IBM collaborated to create WSDL.
The Web Services Description Language (WSDL) is an XML-based file that explains what the web service does to the client application. The WSDL file is used to describe the web service in a nutshell and provides the client with all of the information needed to connect to the web service and use all of its capability.
The WSDL file is used to describe the web service in a nutshell and provides the client with all of the information needed to connect to the web service and use all of its capability.
History
In March 2001, Ariba, IBM, and Microsoft submitted WSDL 1.1 as a W3C Note for describing services for the W3C XML Activity on XML Protocols.
The World Wide Web Consortium (W3C) has not endorsed WSDL 1.1, but it has just released a draught for version 2.0, which will be a recommendation (an official standard) and thus endorsed by the W3C.
Usage
To provide web services via the Internet, WSDL is frequently used with SOAP and XML Schema. The WSDL can be read by a client software connecting to a web service to determine what functions are available on the server. XML Schema is used to encapsulate any particular datatypes used in the WSDL file. After that, the client can use SOAP to call one of the functions provided in the WSDL.
Q13) Write the features of WSDL?
A13) Features
The Web Services Description Language (WSDL) is an XML-based framework for exchanging data in decentralised and distributed contexts.
The WSDL defines how to use a web service and what actions it will do.
The Web Services Description Language (WSDL) is a language for specifying how to interact with XML-based services.
Universal Description, Discovery, and Integration (UDDI), an XML-based global business registry, includes WSDL.
UDDI is written in the WSDL language.
WSDL is spelt W-S-D-L and is pronounced 'wiz-dull.'
Q14) Explain SOAP?
A14) The acronym SOAP refers to the Simple Object Access Protocol. It's a web service access protocol based on XML.
SOAP (Simple Object Access Protocol) is a W3C recommendation for communication between two applications.
SOAP is a protocol that is based on XML. It works on any platform and in any language. You will be able to interface with other programming language applications by using SOAP.
In today's world, there are a plethora of applications created using several programming languages. There could be a web application written in Java, another in.Net, and yet another in PHP, for example.
In today's networked world, data exchange between applications is critical. However, data transmission between these disparate applications would be difficult. The complexity of the programming required to carry out this data interchange will also be high.
The use of XML (Extensible Markup Language) as an intermediate language for sharing data between programmes is one of the approaches tried to fight this complexity.
The XML markup language can be understood by any programming language. As a result, XML was chosen as the data interchange medium.
However, there are no common specifications for using XML for data sharing across all computer languages. SOAP software can help with this.
SOAP was created to deal with XML over HTTP and to establish a standard that could be applied to any applications.
Uses
What is the purpose of a standard like SOAP? Two applications can transmit rich, structured information by exchanging XML documents over HTTP without the need for an extra standard, such as SOAP, to explicitly provide a message envelope format and a mechanism to encode structured content.
SOAP provides a standard that eliminates the need for developers to create their own XML message format for each service they want to make available. The SOAP specification specifies an unambiguous XML message format based on the signature of the service method to be invoked. By getting the following service data, any developer familiar with the SOAP specification, working in any programming language, can make a correct SOAP XML request for a specific service and comprehend the answer from the service.
● Service name
● Method names implemented by the service
● Method signature of each method
● Address of the service implementation (expressed as a URI)
Because the method signature of the service indicates the XML document structure used for both the request and the response, using SOAP simplifies the process of exposing an existing software component as a Web service.
Q15) Write the advantages and disadvantages of SOAP?
A15) Advantages
● SOAP defines its own security, referred to as WS Security.
● SOAP web services are language and platform agnostic. They can be built in any programming language and run on any platform.
Disadvantages
● SOAP is slow because it employs an XML format that must be digested before it can be read. It establishes a number of guidelines that must be followed while designing SOAP applications. As a result, it is slow and uses a lot of bandwidth and resources.
● SOAP is WSDL-reliant, as it lacks any other mechanism for discovering services.
Q16) Define struts?
A16) Struts
● The struts framework is an open source framework for creating well-structured web based applications.
● The struts framework was initially created by Craig McClanahan and donated to Apache Foundation in May, 2000 and Struts 1.0 was released in June 2001.
● The current stable release of Struts is Struts 2.3.16.1 in March 2, 2014.
● The Struts 2 framework is used to develop MVC (Model View Controller) based web applications. Struts 2 is the combination of webwork framework and struts
● The struts framework is based on the Model View Controller (MVC) standard which distinctly separates all the three layers - Model which represents the state of the application, View represents presentation and Controller shows controlling the application flow.
● The struts framework is a complete web framework as it provides complete web form components, validators, error handling, internationalization, tiles and more.
● Struts framework provides its own Controller component. It integrates with other technologies for both Model and View components.
● Struts can integrate well with Java Server Pages (JSP), Java Server Faces (JSF), JSTL, Velocity templates and many other presentation technologies for View. For Model, Struts works great with data access technologies like JDBC, Hibernate, EJB and many more.
Why struts! so popular
● A strut supports extensive validations where other frame works doesn’t
● Having inbuilt support for Il 8N
● Struts 2 actions classes are spring friendly so we can easily integrate
● In build AJAX themes to make the applications more dynamic
● It is good frame work for front end based applications.
Q17) What are the struts architecture?
A17) Struts Architecture
● In Struts architecture, there is only one controller servlet for the entire web application. This controller servlet is called ActionServlet and resides in the package org.apache.struts.action.
● It intercepts every client request and populates an ActionForm from the HTTP request parameters.
● ActionForm is a normal JavaBeans class. It has several attributes corresponding to the HTTP request parameters and getter, setter methods for those attributes. You have to create your own ActionForm for every HTTP request handled through the Struts framework by extending the org.apache.struts.action.ActionForm class as shown in the below architecture.
● Consider the following HTTP request for FirstApp web application:
Http://localhost:8080/FirstApp/create.do?firstName=AnandfelastName=Mustary
● The ActionForm class for this HTTP request is shown in the below example. The class MyForm extends the org.apache.struts.action.
● ActionForm class and contains two attributes: fιrstName and IastName. It also has getter and setter methods for these attributes. Use classes such as ActionForm for View Data Transfer Object. View Data Transfer Object is an object that holds the data from html page and transfers it around in the web tier framework and application classes.
∕∕ ActionForm
Public class MyForm extends ActionForm {
Private String firStName;
Private String IastName;
Public MyForm() {
fιrstName = IastName =
}
Public String getFirstName() {
Return firstName;
}
Public void setFirstName(String s) {
This, fir StName = s;
}
Public String getLastName() {
Return IastName;
}
Public void setLastName(String s) {
This.IastName = s;
}
}
● The ActionServlet then instantiates a Handler. The Handler class name is obtained from an XML file based on the URL path information. This XML file is referred to as Struts configuration file and by default named as struts-config.xml.
● The Handler is called Action in the Struts terminology. And you guessed it right! This class is created by extending the Action class in org.apache.struts.action package.
● The Action class is abstract and defines a single method called execute(). You override this method in your own Actions and invoke the business logic in this method. The execute() method returns the name of next view (JSP) to be shown to the user. The ActionServlet forwards to the selected view.
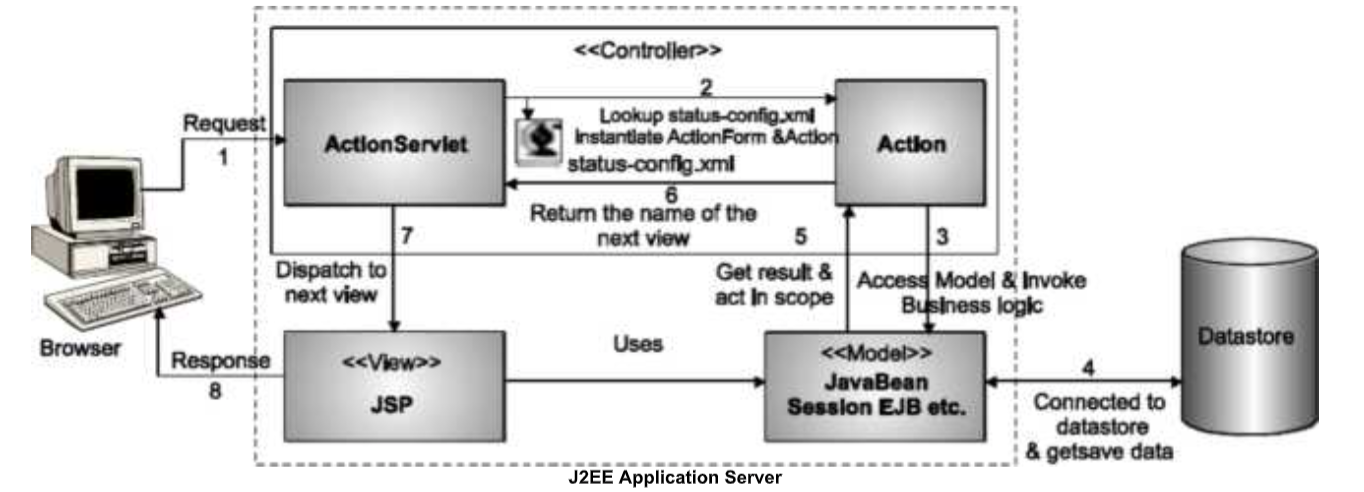
Fig 3: Struts architecture
Q18) What are the key distinctions between JSP custom tags and Java Beans?
A18) Primary differences between the JSP custom tags and java beans
● Beans cannot change JSP content, but custom tags may.
● With custom tags, complex operations can be simplified to a much simpler form than with beans.
● Setting up custom tags takes a lot more time than setting up beans.
● Beans can be utilised in all JSP 1.x versions, however custom tags are only accessible in JSP 1.1 and later.
Q19) In the JSP, how may the applets be displayed? Give an example to illustrate your point.
A19) To embed an applet in a JSP file, use the jsp:plugin action tag. The jsp:plugin action tag downloads a plugin to run an applet or bean on the client side.
In JSP, an example of showing an applet is shown.
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Mouse Drag</title>
</head>
<body bgcolor="khaki">
<h1>Mouse Drag Example</h1>
<jsp:plugin align="middle"
Type="applet" code="MouseDrag.class" name="clock" codebase="."/>
</body>
</html>
Q20) What are the many characteristics of a Java Bean?
A20) There are five different forms of property:
● Simple property: To set a simple property, a pair of accessor, i.e. getXXX (), and mutator, i.e setXXX(), methods are employed.
● Boolean Property: A simple property with boolean values – true or false – set in mutator method.
● Indexed property: An indexed property when a single property can hold an array of values using public void set propertyName (propertyType[] list) method.
● Bound property: A Bean that has a bound property generates an event when the property is changed. The event is of type propertychangeEvent and is sent to objects that previously registered an interest in receiving such notifications.
● Constrained property: A Bean that has a constrained property generates an event when an attempt is made to change its value. The event is of type propertychangeEvent.