Unit - 6
Ruby and Rails
Q1) Introduce Ruby?
A1) Yukihiro Matsumoto created Ruby, an object-oriented programming language. Ruby is a dynamic programming language with a grammar that is both complex and expressive. A core class library with a robust and powerful API is also available in Ruby.
Ruby is based on various low-level and object-oriented programming languages such as Lisp, Smalltalk, and Perl, and has a simple syntax that C and Java programmers can pick up quickly.
Ruby is a dynamic and open source programming language with a focus on productivity and simplicity. It features a beautiful syntax that is easy to understand and write.
Ruby is a simple language to programme in, but it is not a simple language.
Ruby is an object-oriented programming language in its purest form. Yukihiro Matsumoto of Japan invented it in 1993.
Yukihiro Matsumoto's name can be found on the Ruby mailing list at www.ruby-lang.org. In the Ruby community, Matsumoto is also known as Matz.
Ruby is referred to as "A Programmer's Best Friend."
Smalltalk, Perl, and Python all have features that Ruby does not. Scripting languages include Perl, Python, and Smalltalk. Smalltalk is an object-oriented programming language. Ruby is a wonderful object-oriented language, just like Smalltalk. Smalltalk syntax is substantially more difficult to learn than Ruby syntax.
Uses
Ruby is a programming language that may be used to develop a variety of web apps. It is currently one of the most used technologies for developing online apps.
Ruby has a fantastic functionality known as Ruby on Rails (RoR). It is a web framework that programmers use to save time and speed up the development process.
Ruby is most commonly used to create web apps. However, because it is a general-purpose language like Python, it may be used for data analysis, prototyping, and proof-of-concepts.
Rails web, a Ruby-based development framework, is probably the most visible Ruby implementation. Ruby is also used in Homebrew, a popular utility for installing software packages on macOS. Metasploit, a security software that allows you to test websites and applications for how easy they are to break into, is also free.
Ruby developers have produced a number of well-known Rails-based applications. AirBnB, a popular booking site, and Hulu, a TV streaming service, are among them. Github, Goodreads, and the calorie-tracking app MyFitnessPal are among the other apps.
Q2) What do you mean by scalar types?
A2) Scalars, arrays, and hashes are the three kinds of data in Ruby.
The most widely used types are:
Scalars data types
Scalar types are divided into two categories: numeric s and character strings. Literals with numeric and string values The Numeric class is the root of all numeric data types in Ruby. Numeric has two direct children: Float and Integer. Fixnum and Bignum are two child classes of the Integer class.
In Ruby, data types represent various sorts of data such as text, string, numbers, and so on. Because it is an Object-Oriented language, all data types are based on classes. The following are the various data types in Ruby:
● Numbers
● Boolean
● Strings
● Hashes
● Arrays
● Symbols
Numbers
A number is typically defined as a sequence of digits with a decimal mark of a dot. The underscore can be used as a separator if desired. Integers and floats are two separate types of numbers. Ruby is capable of working with both integers and floating point numbers. Integers are divided into two categories based on their size: Bignum and Fixnum.
Example
# Ruby program to illustrate the
# Numbers Data Type
# float type
Distance = 0.1
# both integer and float type
Time = 9.87 / 3600
Speed = distance / time
Puts "The average speed of a sprinter is #{speed} km/h"
Output:
The average speed of a sprinter is 36.474164133738604 km/h
Boolean
True or false is the only bit of information represented by the Boolean data type.
Example
# Ruby program to illustrate the
# Boolean Data Type
If true
Puts "It is True!"
Else
Puts "It is False!"
End
If nil
Puts "nil is True!"
Else
Puts "nil is False!"
End
If 0
Puts "0 is True!"
Else
Puts "0 is False!"
End
Output:
It is True!
Nil is False!
0 is True!
Strings
A string is a collection of letters that make up a sentence or a word. Strings are made up of text enclosed in single (") or double ("") quotations. To make strings, you can use both double and single quotes. Strings are objects that belong to the String class. Substitution and backslash notation are allowed in double-quoted strings, but not in single-quoted strings, which only allow backslash notation for \\ and \’.
Example
# Ruby program to illustrate the
# Strings Data Type
#!/usr/bin/ruby -w
Puts "String Data Type";
Puts 'escape using "\\"';
Puts 'That\'s right';
Output:
String Data Type
Escape using "\"
That's right
Hashes
The values of a hash are assigned to its key. The => symbol assigns a value to a key. A comma separates each key pair, and all of the pairings are surrounded within curly braces. In Ruby, a hash is similar to a JavaScript object literal or a PHP associative array. They're created in the same way that arrays are. A comma at the end of a sentence is ignored.
Example
# Ruby program to illustrate the
# Hashes Data Type
#!/usr/bin/ruby
Hsh = colors = { "red" => 0xf00, "green" => 0x0f0, "blue" => 0x00f }
Hsh.each do |key, value|
Print key, " is ", value, "\n"
End
Output:
Red is 3840
Green is 240
Blue is 15
Arrays
An array is a container for data or a list of data. It can hold any type of information. A comma separates the data in an array, which is then enclosed in square brackets. An array's elements are numbered from 0 to 1. A comma at the end of a sentence is ignored.
Example
# Ruby program to illustrate the
# Arrays Data Type
#!/usr/bin/ruby
Ary = [ "fred", 10, 3.14, "This is a string", "last element", ]
Ary.each do |i|
Puts i
End
Output:
Fred
10
3.14
This is a string
Last element
Symbols
Light-weight strings serve as symbols. A colon comes before a symbol (:). They're utilised instead of strings because they're substantially less in size. Symbols have a higher level of performance.
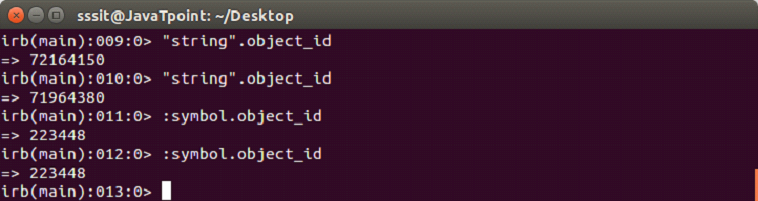
Q3) Write about input and output?
A3) Ruby I/O is a method of interacting with your computer. Bytes/characters are used to send data. In Ruby, the IO class is the foundation for all input and output. It can be duplexed, which means it can utilise several native operating system streams.
The File class is a subclass of I/O that allows Ruby to read and write files. The two classes are inextricably linked. The IO object represents readable/writable keyboard and screen interactions.
Common modes in I/O port
"r" - The default mode is "r," which starts at the beginning of the file.
"r+" - is a read-write mode that begins at the beginning of the file.
"w" - write-only mode, which either creates a new file or truncates an existing one to allow for writing.
"w+" - read-write mode, which either creates a new file or truncates an existing file to allow for reading and writing.
"a" - write-only mode; if the file already exists, it will be appended; otherwise, a new file for writing only will be created.
"a+" - read and write mode; if the file already exists, it will be appended; otherwise, a new file for writing and reading will be created.
The puts Statement
You gave values to variables and then used the puts statement to print the results.
The puts command tells the computer to display the value of the variable it's holding. Each line it writes will be followed by a new line.
Example
#!/usr/bin/ruby
Val1 = "This is variable one"
Val2 = "This is variable two"
Puts val1
Puts val2
This will produce the following result −
This is variable one
This is variable two
The gets Statement
The gets statement can be used to get any user input from the STDIN standard screen.
Example
The gets statement is demonstrated in the following code. This code will ask the user for a value, which will be saved in the variable val and then printed on STDOUT.
#!/usr/bin/ruby
Puts "Enter a value :"
Val = gets
Puts val
This will produce the following result −
Enter a value :
This is entered value
This is entered value
The putc Statement
Unlike the puts statement, which prints the entire string, the putc command allows you to print one character at a time.
Example
The following code produces only the letter H as an output.
#!/usr/bin/ruby
Str = "Hello Ruby!"
Putc str
This will produce the following result −
H
Reading and Writing Files
For all file objects, the same methods that we've been utilising for ‘simple I/O’ are available. As a result, gets and aFile both read a line from standard input. Reads a line from the aFile file object.
I/O objects, on the other hand, give an extra set of access methods to make our life easier.
The sysread Method
To read the contents of a file, use the sysread method. When using the technique sysread, you can open the file in any of the modes. For instance,
The input text file is as follows:
This is a simple text file for testing purposes.
Now let's try to read this file −
#!/usr/bin/ruby
AFile = File.new("input.txt", "r")
If aFile
Content = aFile.sysread(20)
Puts content
Else
Puts "Unable to open file!"
End
This statement will print the file's first 20 characters. The file pointer will now be set to the file's 21st character.
The syswrite Method
The method syswrite can be used to save the contents to a file. When utilising the syswrite approach, you must open the file in write mode. For instance,
#!/usr/bin/ruby
AFile = File.new("input.txt", "r+")
If aFile
AFile.syswrite("ABCDEF")
Else
Puts "Unable to open file!"
End
This command will append the letters "ABCDEF" to the file.
The each_byte Method
This method is part of the File class. Each byte is always used in conjunction with a block. Take a look at the following code example:
#!/usr/bin/ruby
AFile = File.new("input.txt", "r+")
If aFile
AFile.syswrite("ABCDEF")
AFile.each_byte {|ch| putc ch; putc ?. }
Else
Puts "Unable to open file!"
End
The variable ch is passed characters one by one and then shown on the screen as follows:
s. .a. .s.i.m.p.l.e. .t.e.x.t. .f.i.l.e. .f.o.r. .t.e.s.t.i.n.g. .p.u.r.p.o.s.e...
.
.
The IO.readlines Method
The File class is a subclass of the IO class. There are certain methods in the IO class that can be used to manipulate files.
IO.readlines is a method in the IO class. This method iterates through the file's contents line by line. The method IO.readlines is demonstrated in the following code.
#!/usr/bin/ruby
Arr = IO.readlines("input.txt")
Puts arr[0]
Puts arr[1]
The variable arr is an array in this code. In the array arr, each line of the file input.txt will be an element. As a result, arr[0] will contain the first line of the file, whereas arr[1] will have the second line.
The IO.foreach Method
This technique also returns line-by-line output. The method foreach differs from the method readlines in that it is coupled with a block. The method foreach, unlike the method readlines, does not return an array. For instance,
#!/usr/bin/ruby
IO.foreach("input.txt"){|block| puts block}
The contents of the file test will be passed line by line to the variable block in this code, and the output will be displayed on the screen.
Q4) Explain control statements?
A4) Ruby includes conditional structures that are ubiquitous in current programming languages. In this section, we'll go through all of Ruby's conditional statements and modifiers.
Ruby if...else Statement
If conditional execution is done with expressions The values false and nil are false, whereas the rest of the values are correct. Ruby utilises elsif instead of else if or elif.
If the conditional is true, the code is executed. The code supplied in the otherwise clause is run if the conditional is not true.
The reserved word then, a newline, or a semicolon separates the conditional part of an if statement from the code.
Syntax
If conditional [then]
Code...
[elsif conditional [then]
Code...]...
[else
Code...]
End
Example
#!/usr/bin/ruby
x = 1
If x > 2
Puts "x is greater than 2"
Elsif x <= 2 and x!=0
Puts "x is 1"
Else
Puts "I can't guess the number"
End
x is 1
Ruby case Statement
Using the === operator, compares the expressions supplied by case and when, and runs the code of the when clause that matches.
As the left operand, the expression indicated by the when clause is evaluated. Case performs the code of the else clause if no when clauses match.
Syntax
Case expression
[when expression [, expression ...] [then]
Code ]...
[else
Code ]
End
The reserved word then, a newline, or a semicolon separates the expression from the code in a when statement. As a result
Case expr0
When expr1, expr2
Stmt1
When expr3, expr4
Stmt2
Else
Stmt3
End
Example
#!/usr/bin/ruby
$age = 5
Case $age
When 0 .. 2
Puts "baby"
When 3 .. 6
Puts "little child"
When 7 .. 12
Puts "child"
When 13 .. 18
Puts "youth"
Else
Puts "adult"
End
This will produce the following result −
Little child
Ruby while Statement
While conditional is true, code is executed. The reserved word do, a newline, a backslash, or a semicolon ; separate the conditional in a while loop from the code.
Syntax
While conditional [do]
Code
End
Example
#!/usr/bin/ruby
$i = 0
$num = 5
While $i < $num do
Puts("Inside the loop i = #$i" )
$i +=1
End
This will produce the following result −
Inside the loop i = 0
Inside the loop i = 1
Inside the loop i = 2
Inside the loop i = 3
Inside the loop i = 4
Ruby while modifier
While conditional is true, code is executed.
When a while modifier is used after a begin statement that does not contain any rescue or assure clauses, the code is run once before the conditional is evaluated.
Syntax
Code while condition
OR
Begin
Code
End while conditional
Example
#!/usr/bin/ruby
$i = 0
$num = 5
Begin
Puts("Inside the loop i = #$i" )
$i +=1
End while $i < $num
This will produce the following result −
Inside the loop i = 0
Inside the loop i = 1
Inside the loop i = 2
Inside the loop i = 3
Inside the loop i = 4
Ruby for Loop
The for loop in Ruby iterates over a set of numbers. As a result, if a programme has a set number of iterations, the for loop is utilised.
For each element in the expression, the Ruby for loop will run once.
Syntax:
For variable [, variable ...] in expression [do]
Code
End
Ruby for loop using array
Example:
x = ["Blue", "Red", "Green", "Yellow", "White"]
For i in x do
Puts i
End
Output
Blue
Red
Green
Yellow
White
Ruby break Statement
The most internal loop is terminated. If called within the block, this method terminates a method with an associated block (with the method returning nil).
Syntax
Break
Example
#!/usr/bin/ruby
For i in 0..5
If i > 2 then
Break
End
Puts "Value of local variable is #{i}"
End
This will produce the following output −
Value of local variable is 0
Value of local variable is 1
Value of local variable is 2
Ruby next Statement
The most internal loop is advanced to the next iteration. If called within a block, it stops the execution of that block (with yield or call returning nil).
Syntax
Next
Example
#!/usr/bin/ruby
For i in 0..5
If i < 2 then
Next
End
Puts "Value of local variable is #{i}"
End
This will produce the following output −
Value of local variable is 2
Value of local variable is 3
Value of local variable is 4
Value of local variable is 5
Ruby redo Statement
The redo statement in Ruby is used to repeat the current loop iteration. The condition of the loop is not evaluated before the redo statement is executed.
Within a loop, the redo statement is utilised.
Syntax:
Redo
Example
i = 0
While(i < 5) # Prints "012345" instead of "01234"
Puts i
i += 1
Redo if i == 5
End
Output
0
1
2
3
4
5
Ruby retry Statement
The retry command in Ruby is used to restart the loop iteration from the beginning.
Within a loop, the retry command is utilized.
Syntax:
Retry
Q5) Explain the fundamentals of arrays?
A5) Ruby arrays are collections of any object that are sorted and integer-indexed. An index is assigned to each element in an array and is used to refer to it.
As in C or Java, array indexing begins at zero. A negative index is assumed relative to the array's end —- that is, an index of -1 denotes the array's last element, -2 indicates the array's next to last element, and so on.
String, Integer, Fixnum, Hash, Symbol, and even other Array objects can be stored in Ruby arrays. Arrays in Ruby aren't as rigid as those in other languages. While adding elements to a Ruby array, it grows automatically.
Creating array
An array can be created or initialised in a variety of ways. One technique is to use the new class method.
Names = Array.new
You can specify the size of an array when it is created.
Names = Array.new(20)
The names array now has a length of 20 entries. The size or length methods can be used to get the size of an array.
#!/usr/bin/ruby
Names = Array.new(20)
Puts names.size # This returns 20
Puts names.length # This also returns 20
As a result, you'll get the following:
20
20
You can give each element in the array a value by doing the following:
#!/usr/bin/ruby
Names = Array.new(4, "mac")
Puts "#{names}"
As a result, you'll get the following:
["mac", "mac", "mac", "mac"]
You may also use a block with new to populate each element with the value of the block.
#!/usr/bin/ruby
Nums = Array.new(10) { |e| e = e * 2 }
Puts "#{nums}"
As a result, you'll get the following:
[0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
There is another Array method, []. This is how it works:
Nums = Array.[](1, 2, 3, 4,5)
Another way to make an array is as follows:
Nums = Array[1, 2, 3, 4,5]
The Kernel module is a core module. The Array function in Ruby only takes a single argument. To produce an array of digits, the method takes a range as an input.
#!/usr/bin/ruby
Digits = Array(0..9)
Puts "#{digits}"
As a result, you'll get the following:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Array Built-in Methods
To call an Array method, we need an instance of the Array object. As we've seen, the method for creating an instance of the Array object is as follows:
Array.[](...) [or] Array[...] [or] [...]
This will create a new array with the specified objects in it. We can now call any available instance methods using the newly created object. For instance,
#!/usr/bin/ruby
Digits = Array(0..9)
Num = digits.at(6)
Puts "#{num}"
As a result, you'll get the following:
6
Q6) Define hashes?
A6) A Hash is a collection of key-value pairs like this: "employee" = > "salary". It's identical to an Array, except that instead of using an integer index, it indexes using arbitrary keys of any object type.
The order in which you traverse a hash by key or value may appear arbitrary, but it will almost always be different from the insertion order. The method will return nil if you try to access a hash with a key that does not exist.
Creating Hashes
Hashes can be created in a multitude of ways, just like arrays. The new class method can be used to produce an empty hash.
Months = Hash.new
You can also construct a hash with a default value, which is otherwise just nil, by using new.
Months = Hash.new( "month" )
Or
Months = Hash.new "month"
If a key or value in a hash contains a default value, accessing the hash will return the default value if the key or value doesn't exist.
#!/usr/bin/ruby
Months = Hash.new( "month" )
Puts "#{months[0]}"
Puts "#{months[72]}"
As a result, you'll get the following:
Month
Month
#!/usr/bin/ruby
H = Hash["a" => 100, "b" => 200]
Puts "#{H['a']}"
Puts "#{H['b']}"
As a result, you'll get the following:
100
200
Because any Ruby object, including an array, can be used as a key or value, the following example is valid:
[1,"jan"] => "January"
Hash Built-in Methods
To call a Hash method, we must have a Hash object instance. As we've seen, the method for creating a Hash object instance is as follows:
Hash[[key =>|, value]* ] or
Hash.new [or] Hash.new(obj) [or]
Hash.new { |hash, key| block }
This will create a new hash with the specified objects in it. We may now call any accessible instance methods using the newly formed object. For instance,
#!/usr/bin/ruby
$, = ", "
Months = Hash.new( "month" )
Months = {"1" => "January", "2" => "February"}
Keys = months.keys
Puts "#{keys}"
As a result, you'll get the following:
["1", "2"]
The public hash methods are listed below (assuming hash is an array object).
Sr.No. | Methods & Description |
1 | Hash == other_hash This function compares two hashes to see if they have the same amount of key-value pairs and if the key-value pairs match the corresponding pair in each hash. |
2 | Hash.[key] A value from hash is referenced using a key. If the key cannot be retrieved, a default value is returned. |
3 | Hash.[key] = value The value given by value is linked to the key given by key. |
4 | Hash.clear All key-value pairs are removed from the hash. |
5 | Hash.default(key = nil) If default= isn't specified, this function returns nil. (If the key does not exist in the hash, [] returns a default value.) |
6 | Hash.default = obj Sets the hash's default value. |
7 | Hash.default_proc If the hash was formed by a block, it returns a block. |
8 | Hash.delete(key) [or] Array.delete(key) { |key| block } By key, removes a key-value pair from the hash. If block is used, if pair is not found, returns the result of a block. Take a look at delete if. |
9 | Hash.delete_if { |key,value| block } For every pair in the hash that evaluates to true, a key-value pair is removed. |
10 | Hash.each { |key,value| block } Iterates through the hash, calling block once for each key and passing the key-value pair as a two-element array. |
11 | Hash.each_key { |key| block } Iterates through the hash, calling the block for each key once and passing the key as an argument. |
Q7) What are the methods of Ruby?
A7) Methods in Ruby are a lot like functions in other programming languages. Ruby methods are used to group together one or more recurring statements.
The name of the method should start with a lowercase letter. If you start a method name with an uppercase letter, Ruby may mistake it for a constant and wrongly process the call.
Methods should be defined before they are called; otherwise, Ruby will throw an exception for invoking an undefined method.
Syntax
Def method_name [( [arg [= default]]...[, * arg [, &expr ]])]
Expr..
End
As a result, you can create a simple procedure as follows:
Def method_name
Expr..
End
This is an example of a method that accepts parameters.
Def method_name (var1, var2)
Expr..
End
You can give the parameters default values, which will be used if the method is called without the needed parameters.
Def method_name (var1 = value1, var2 = value2)
Expr..
End
When calling the simple method, you only need to write the method name as follows:
Method_name
When calling a method with parameters, however, you must include the method name as well as the parameters, for example,
Method_name 25, 30
The most significant disadvantage of using methods with parameters is that you must keep track of the number of parameters each time you call them. If you send only two parameters to a method that supports three, Ruby will throw an error.
Example
#!/usr/bin/ruby
Def test(a1 = "Ruby", a2 = "Perl")
Puts "The programming language is #{a1}"
Puts "The programming language is #{a2}"
End
Test "C", "C++"
Test
This will produce the following output −
The programming language is C
The programming language is C++
The programming language is Ruby
The programming language is Perl
Return Values from Methods
By default, every Ruby method returns a value. The value of the previous statement will be returned. For instance,
Def test
i = 100
j = 10
k = 0
End
When called, this method returns the last declared variable k.
Ruby return Statement
Returning one or more values from a Ruby Method is done with the return statement.
Syntax
Return [expr[,' expr...]]
If more than two expressions are specified, the return value will be the array containing these values. If no expression is provided, the return value will be nil.
Example
Return
OR
Return 12
OR
Return 1,2,3
Take a look at this illustration.
#!/usr/bin/ruby
Def test
i = 100
j = 200
k = 300
Return i, j, k
End
Var = test
Puts var
As a result, you'll get the following:
100
200
300
Variable Number of Parameters
If you declare a method that takes two parameters, you must pass two parameters along with it anytime you call it.
Ruby, on the other hand, allows you to declare methods with a variable amount of parameters. Let's have a look at an example of this.
#!/usr/bin/ruby
Def sample (*test)
Puts "The number of parameters is #{test.length}"
For i in 0...test.length
Puts "The parameters are #{test[i]}"
End
End
Sample "Zara", "6", "F"
Sample "Mac", "36", "M", "MCA"
You've declared a method sample with one parameter test in this code. This parameter, however, is a changeable parameter. This signifies that any amount of variables can be passed into this parameter. As a result, the code above will yield the following result:
The number of parameters is 3
The parameters are Zara
The parameters are 6
The parameters are F
The number of parameters is 4
The parameters are Mac
The parameters are 36
The parameters are M
The parameters are MCA
Class Methods
By default, when a method is defined outside of the class declaration, it is designated as private. The methods defined in the class definition, on the other hand, are tagged as public by default. The default visibility and private mark of the methods can be altered by changing the Module's public or private property.
When you want to use a class's method, you must first instantiate the class. The object can then be used to access any member of the class.
Ruby provides a means to call a method without having to create a class. Let's have a look at how a class method is declared and used.
Class Accounts
Def reading_charge
End
Def Accounts.return_date
End
End
Ruby alias Statement
This grants methods or global variables aliases. Within the method body, aliases cannot be defined. Even when methods are overridden, the alias of the method keeps the current definition of the method.
It is forbidden to create aliases for the numbered global variables ($1, $2,...) It's possible that overriding the built-in global variables will cause major issues.
Syntax
Alias method-name method-name
Alias global-variable-name global-variable-name
Example
Alias foo bar
Alias $MATCH $&
The foo alias for bar is defined here, while $MATCH is an alias for $&.
Ruby undef Statement
This removes the method definition from the equation. The method body cannot contain an undef.
The interface of the class can be updated independently from the superclass by using undef and alias, but be aware that the internal method call to self may break applications.
Syntax
Undef method-name
Example
To make a method called bar undefined, do the following:
Undef bar
Q8) What is ruby classes?
A8) Ruby is a great language for object-oriented programming. Data encapsulation, polymorphism, inheritance, data abstraction, operator overloading, and other properties of an object-oriented programming language. Classes and objects play a vital role in object-oriented programming.
A class is a blueprint for the creation of objects. A class instance is another name for the object. Animals, for example, are a class, and mammals, birds, fish, reptiles, and amphibians are examples of it. Similarly, the sales department is the class, and the sales data, sales manager, and secretary are the class's objects.
Creating a class in Ruby:
Ruby makes it simple to construct classes and objects. Simply type the class keyword followed by the class name. The class name's initial letter should be written in capital letters.
Syntax:
Class Class_name
End
The end keyword terminates a class, and all data members are located between the class declaration and the end keyword.
Variables in a Ruby Class
Ruby has four different sorts of variables.
● Local Variables − The variables defined in a method are known as local variables. Outside of the method, local variables are not accessible. In the next chapter, you'll learn more about the process. Local variables begin with the letter or a lowercase letter.
● Instance Variables − For each given instance or object, instance variables are available across methods. As a result, instance variables differ from one object to the next. The at sign (@) precedes instance variables, which are then followed by the variable name.
● Class Variables − Variables from a class can be used across several objects. A class variable is a characteristic of a class that belongs to it. They have the @@ sign before them and the variable name after them.
● Global Variables − Class variables aren't shared between classes. You must define a global variable if you want a single variable that is accessible across all classes. The global variables are always preceded by the dollar sign ($).
Example
The number of objects being created can be determined using the class property @@no of customers. This enables the number of clients to be calculated.
Class Customer
@@no_of_customers = 0
End
Q9) What do you mean by pattern matching?
A9) Pattern matching is an experimental feature that allows for deep matching of structured data, including evaluating the structure and binding matched parts to local variables.
In functional programming languages, pattern matching is a feature that is often used. Pattern matching is "a tool for testing a value against a pattern," according to Scala documentation. A good match can also break down a value into its component elements."
Regex, string matching, and pattern recognition are not the same thing. Matching patterns has little to do with strings and everything to do with data structure. When I initially tried out Elixir two years ago, I ran into pattern matching for the first time. I was trying to solve algorithms with Elixir, which I was studying. When I looked at other people's solutions, I noticed that they employed pattern matching, which made their code a lot shorter and easier to comprehend.
The in operator, which can be used in a solo expression in Ruby, is used to match patterns.
<variable> in <pattern>
Or inside the +case+ clause:
Case <variable>
In <pattern1>
...
In <pattern2>
...
In <pattern3>
...
Else
...
End
A variable or an expression can be passed to the case statement, which will be tested against patterns in the in clause. After the pattern, you can add if or unless statements. Like the typical case statement, the equality check here employs ===. You can match subsets and instances of classes in this way. Here's an example of how to put it to use:
Patterns
Patterns can be anything from:
● any Ruby object (as in +when+) that is matched by the === operator;
● [subpattern>, subpattern>, subpattern>,...] array pattern
● hash pattern: key: subpattern>, key: subpattern>, key: subpattern>,...
● pattern combination with |
Where <subpattern> is supplied, any pattern can be nested inside array/hash patterns.
Array patterns correspond to arrays or objects that deconstruct (see below about the latter). Hash patterns correspond to hashes or objects that react to the deconstruct keys command. For the time being, only symbol keys are supported for hash patterns.
The behaviour of arrays and hash patterns differs in that arrays match just the entire array.
Case [1, 2, 3]
In [Integer, Integer]
"matched"
Else
"not matched"
End
#=> "not matched"
Even if there are more keys except the given section, the hash matches:
Case {a: 1, b: 2, c: 3}
In {a: Integer}
"matched"
Else
"not matched"
End
#=> "matched"
With **nil: no other keys in the matched hash except those expressly indicated by pattern, you can declare that there should be no other keys in the matched hash except those specifically stated by pattern.
Case {a: 1, b: 2}
In {a: Integer, **nil} # this will not match the pattern having keys other than a:
"matched a part"
In {a: Integer, b: Integer, **nil}
"matched a whole"
Else
"not matched"
End
#=> "matched a whole"
The "rest" specification is supported by both array and hash patterns:
Case [1, 2, 3]
In [Integer, *]
"matched"
Else
"not matched"
End
#=> "matched"
Case {a: 1, b: 2, c: 3}
In {a: Integer, **}
"matched"
Else
"not matched"
End
#=> "matched"
Parentheses around both types of patterns could be eliminated in case (but not in standalone in) statements.
Case [1, 2]
In Integer, Integer
"matched"
Else
"not matched"
End
#=> "matched"
Case {a: 1, b: 2, c: 3}
In a: Integer
"matched"
Else
"not matched"
End
#=> "matched"
Matching Hashes
Translation = {orig_lang: 'th', trans_lang: 'en', orig_txt: 'เต้', trans_txt: 'tae' }
Case translation
In {orig_lang: 'th', trans_lang: 'en', orig_txt: orig_txt, trans_txt: trans_txt}
Puts "#{orig_txt} => #{trans_txt}"
End
The translation variable is now a hash in the example above. In the in clause, it is compared to another hash. The case statement examines whether all of the keys in the pattern match the keys in the translation variable. It also ensures that all of the values for each key are identical. It then assigns the values to the hash variable.
Q10) Explain rails?
A10) Rails
● Framework for developing web applications that is incredibly productive.
● David Heinemeier Hansson wrote it in Ruby.
● With Rails, you could create a web application ten times faster than you could with a traditional Java framework.
● A Ruby framework for creating database-backed web applications that is open source.
● Use Database Schema to configure your code.
● There is no need for a compilation phase.
Rails is a server-side web application framework built in Ruby and released under the MIT License. Rails is a model–view–controller (MVC) framework that comes with default database, web service, and web page structures. It promotes and enables the use of web standards for data transfer, such as JSON or XML, and user interfaces, such as HTML, CSS, and JavaScript. Rails promotes the usage of additional well-known software engineering patterns and paradigms, such as convention over configuration (CoC), don't repeat yourself (DRY), and the active record pattern, in addition to MVC.
The introduction of Ruby on Rails in 2005 had a significant impact on web app development, thanks to new features such as seamless database table creation, migrations, and view scaffolding to enable rapid application development.
The influence of Ruby on Rails on other web frameworks can still be seen today, with Django in Python, Catalyst in Perl, Laravel, CakePHP, and Yii in PHP, Grails in Groovy, Phoenix in Elixir, Play in Scala, and Sails.js in Node.js all taking ideas from Ruby on Rails.
Ruby on Rails was first released in July 2004 but did not share commit rights until February 2005. Ruby on Rails would be included with Mac OS X 10.5 "Leopard" in August 2006. Rail 5.0.1, the most recent version of Ruby on Rail, was released on December 21, 2016. This version introduces the action cable, Turbolinks 5, and API mode.
Advantages
● Rails provides tooling to assist us in delivering more features in less time.
● Libraries: There's a third-party module(gem) for almost anything we can imagine.
● Ruby code is substantially better than PHP or NodeJS alternatives in terms of quality.
● The Ruby community is very interested in test automation and testing.
● Ruby has a big following in the community.
● Ruby is exceptionally fast when compared to other languages in terms of productivity. It has a high rate of productivity.
Disadvantages
● Ruby on Rails is slower than Node.js and Golang when it comes to runtime speed.
● Lack of Flexibility: Because of its strict dependency between components and models, Ruby on Rails is best suited for typical web applications. However, adding new features and personalization to apps might be difficult.
● ROR also has a disadvantage in terms of boot speed. It takes some time to get started due to the volume of gem dependencies and files, which can stifle developer productivity.
● Documentation: Finding adequate documentation for lesser-known gems and libraries that rely heavily on mixins is difficult.
● Multithreading: Multithreading is supported by Ruby on Rails, although other IO libraries do not since they preserve the global interpreter lock. As a result, if you aren't attentive, your request will be queued behind other active ones, causing performance problems.
● Active Record: The domain becomes firmly tied to your persistence system due to the use of Active records in the ROR and hard reliance.
Q11) What is code blocks?
A11) You've seen how Ruby provides methods inside which you can include a number of statements before calling the method. Block is a concept in Ruby as well.
● A block is made up of code pieces.
● A block has a name that you give it.
● Braces () are always used to surround the code in the block.
● A function with the same name as the block is always used to call the block. This means that if you have a block named test, you should use the function test to call it.
● The yield statement is used to start a block.
Syntax
Block_name {
Statement1
Statement2
..........
}
You'll learn how to use a basic yield statement to invoke a block. You'll also learn how to invoke a block using a yield statement with parameters. Both sorts of yield statements will be tested in the sample code.
Blocks and Methods
You've seen how a block and a method can be linked to one another. In most cases, you call a block with the yield statement from a method with the same name as the block. As a result, you write
#!/usr/bin/ruby
Def test
Yield
End
Test{ puts "Hello world"}
This is the most basic approach to implement a block. The yield statement is used to invoke the test block.
However, if a method's last argument is preceded by &, you can pass a block to it, and it will be allocated to the last parameter. If the argument list contains both * and &, & should come first.
#!/usr/bin/ruby
Def test(&block)
Block.call
End
Test { puts "Hello World!"}
This will produce the following output −
Hello World!
BEGIN and END Blocks
Every Ruby source file can declare code blocks to run both while the file is being loaded (the BEGIN blocks) and after the programme has completed executing (the END blocks) (the END blocks).
#!/usr/bin/ruby
BEGIN {
# BEGIN block code
Puts "BEGIN code block"
}
END {
# END block code
Puts "END code block"
}
# MAIN block code
Puts "MAIN code block"
Multiple BEGIN and END blocks can be found in a programme. BEGIN blocks are performed in the order in which they appear. The order in which END blocks are performed is reversed. When the aforementioned programme is run, it produces the following result:
BEGIN code block
MAIN code block
END code block
Q12) Write about iterators?
A12) Iterators are just methods that are provided by collections. Collections are objects that store a group of data members. Arrays and hashes are considered collections in Ruby. Iterators iterate across a collection, returning each element one by one.
Each iterator iterates through an array or a hash, returning all of its elements.
Syntax
Collection.each do |variable|
Code
End
For each element in the collection, code is executed. A collection could be an array or a ruby hash in this case.
Example
#!/usr/bin/ruby
Ary = [1,2,3,4,5]
Ary.each do |i|
Puts i
End
This will produce the following output−
1
2
3
4
5
Each iterator is always associated with a block. It passes each value of the array to the block one by one. The result is saved in the variable I and then shown on the screen.
Collect iterator
The collect iterator returns all of a collection's elements.
Syntax
Collection = collection.collect
A block does not always have to be associated with the collect method. Regardless of whether the collection is an array or a hash, the collect method returns the complete collection.
Example
#!/usr/bin/ruby
a = [1,2,3,4,5]
b = Array.new
b = a.collect
Puts b
This will produce the following result −
1
2
3
4
5
Copying across arrays should not be done with the collect method. Another method, clone, should be used to replicate one array into another.
When you want to perform anything with each of the items in the new array, you usually use the collect method. This code, for example, creates an array b that contains 10 copies of each value in a.
#!/usr/bin/ruby
a = [1,2,3,4,5]
b = a.collect{|x| 10*x}
Puts b
This will produce the following result −
10
20
30
40
50
Q13) What is layouts?
A13) An HTML page's surroundings are defined by its layout. It's where you'll establish a consistent appearance and feel for your final product. The layout files are located in the app/views/layouts folder.
The procedure entails creating a layout template and then informing the controller that it exists and can be used. Let's start by making the template.
In app/views/layouts, create a new file called standard.html.erb. The name of the file tells the controllers what template to use, thus sticking to the same naming convention is recommended.
Save your changes by adding the following code to the new standard.html.erb file:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns = "http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv = "Content-Type" content = "text/html; charset = iso-8859-1" />
<meta http-equiv = "Content-Language" content = "en-us" />
<title>Library Info System</title>
<%= stylesheet_link_tag "style" %>
</head>
<body id = "library">
<div id = "container">
<div id = "header">
<h1>Library Info System</h1>
<h3>Library powered by Ruby on Rails</h3>
</div>
<div id = "content">
<%= yield -%>
</div>
<div id = "sidebar"></div>
</div>
</body>
</html>
Except for two lines, everything you just inserted was ordinary HTML. The auxiliary method stylesheet link tag returns a stylesheet link. We're linking the style.css style file in this case. The yield command instructs Rails to place the html.erb file for the method called here.
Now open book controller.rb and right below the first line, add the following code:
Class BookController < ApplicationController
Layout 'standard'
Def list
@books = Book.all
End
...................
It tells the controller that we want to utilise one of the layouts in standard.html.erb. Now try browsing books, which will result in the screen below.
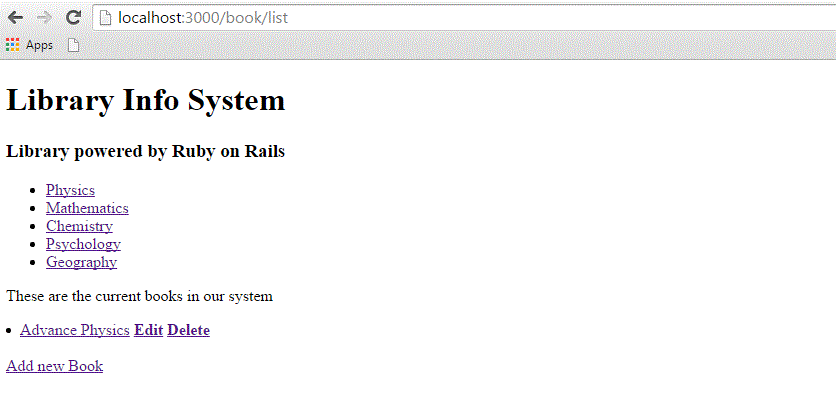
Adding Style Sheet
Because we haven't built a style sheet yet, Rails is utilising the default one. Now we'll make a new style.css file and save it in /public/stylesheets. To this file, add the following code.
Body {
Font-family: Helvetica, Geneva, Arial, sans-serif;
Font-size: small;
Font-color: #000;
Background-color: #fff;
}
a:link, a:active, a:visited {
Color: #CD0000;
}
Input {
Margin-bottom: 5px;
}
p {
Line-height: 150%;
}
Div#container {
Width: 760px;
Margin: 0 auto;
}
Div#header {
Text-align: center;
Padding-bottom: 15px;
}
Div#content {
Float: left;
Width: 450px;
Padding: 10px;
}
Div#content h3 {
Margin-top: 15px;
}
Ul#books {
List-style-type: none;
}
Ul#books li {
Line-height: 140%;
}
Div#sidebar {
Width: 200px;
Margin-left: 480px;
}
Ul#subjects {
Width: 700px;
Text-align: center;
Padding: 5px;
Background-color: #ececec;
Border: 1px solid #ccc;
Margin-bottom: 20px;
}
Ul#subjects li {
Display: inline;
Padding-left: 5px;
}
Now, go ahead and reload your browser to observe the difference.
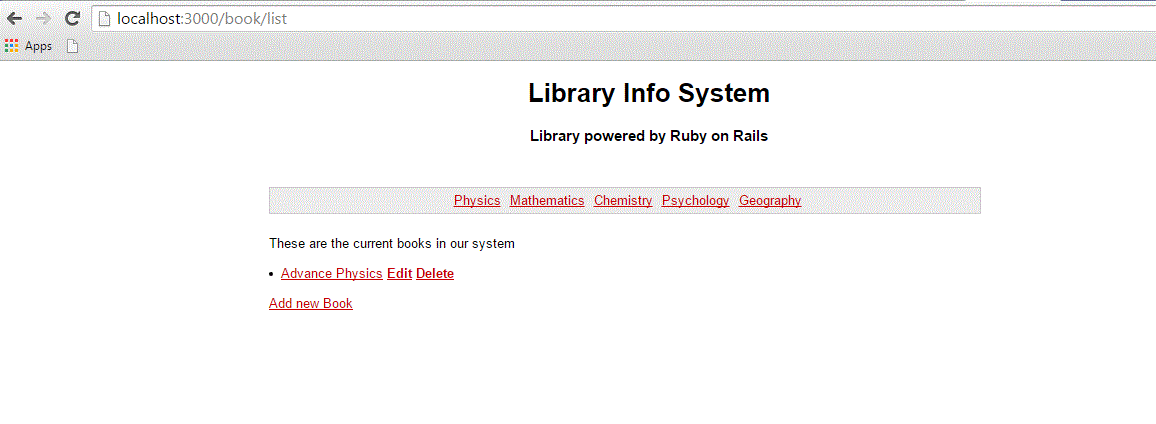
Q14) What do you mean by rails with Ajax?
A14) Ajax refers to a combination of asynchronous JavaScript and XML. Ajax is a collection of technologies rather than a single technology. The following are included in Ajax:
● XHTML is a markup language for online pages.
● The styling is done with CSS.
● DOM-based dynamic display and interaction.
● XML is used to manipulate and exchange data.
● XMLHttpRequest is used to retrieve data.
● JavaScript serves as the glue that holds everything together.
Ajax allows you to retrieve information from a web page without having to reload the full page. The user clicks a link or fills out a form in the most basic web design. The form is sent to the server, which responds with an answer. The user is then presented with the response on a separate page.
An Ajax-powered web page launches an Ajax engine in the background when you interact with it. The engine, which is built in JavaScript, is responsible for communicating with the web server as well as displaying the results to the user. When you submit data using an Ajax-powered form, the server delivers an HTML fragment containing the server's response and only displays the data that has changed or been added, rather than reloading the entire page.
How Rails Implements Ajax
The way Rails supports Ajax operations is simple and consistent. Different user actions force the browser to display a new web page (like any regular web application) or initiate an Ajax activity after the original web page has been produced and displayed.
Some trigger fires − This could be a user clicking on a button or link, a user changing data on a form or in a field, or just a recurring trigger (based on a timer).
The web client calls the server − The XMLHttpRequest JavaScript function transmits data associated with the trigger to a server action handler. The data may be a checkbox's ID, the text in an entry field, or the entire form.
The server does processing −The server-side action handler (Rails controller action) manipulates the data and sends an HTML fragment to the web client.
The client receives the response − The HTML fragment is received by the client-side JavaScript, which Rails generates automatically, and it is used to alter a specific area of the current page's HTML, usually the content of a div> element.
These are the most basic steps for using Ajax in a Rails application, but with a little more effort, you can have the server provide any type of data in response to an Ajax request, and you can utilise custom JavaScript in the browser to do more complex interactions.
Example of AJAX
This example uses scaffold, but the Destroy idea uses ajax.
We shall supply, list, show, and create operations on the ponies table in this example. If you're unfamiliar with scaffolding technology, and then moving on to AJAX on Rails.
Creating An Application
Let's begin with the development of an application. It will be carried out as follows:
Rails new ponies
The above command builds an application; now we must use the cd command to navigate to the app directory. It'll go into an application directory, and then we'll have to run the scaffold command. It will be carried out as follows:
Rails generate scaffold Pony name:string profession:string
The above command creates a scaffold with columns for name and profession. The database must be migrated using the command below.
Rake db:migrate
Now run the Rails application using the commands below.
Rails s
Now open a web browser and type http://localhost:3000/ponies/new into the address bar. The final result will be as follows:
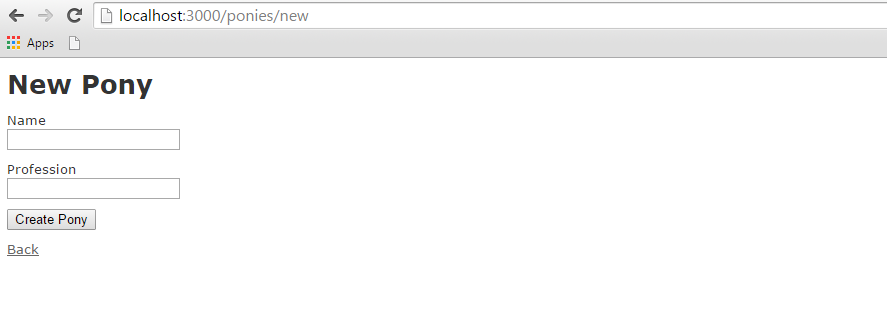
Q15) Define EJB?
A15) EJB stands for Enterprise Java Beans. EJB is an essential part of a J2EE platform. J2EE platform has component based architecture to provide multi-tiered, distributed and highly transactional features to enterprise level applications.

Fig: Multitier Layered Architecture
● Consider the three-layer architecture shown in Fig. 6.6 consisting of user interface, application logic, and database layers. If the database layer is changed, only the application logic layer is affected.
● The application logic layer shields the user interface layer from changes to the database layer.
● This facilitates ongoing maintenance of the application and increases the application’s ability to incorporate new technologies in its layers.
● These layers provide an excellent model of how EJBs fit into your overall program design. EJBs provide an application logic layer and a JavaBeans-Like abstraction of the database layer. The application logic layer is also known as the middle tier.
● It is a server side and Industry standard distributed component model written in Java Language which incorporates the business logic of an application.
Types
There are three types Of Enterprise Java Beans namely:
1. Session Beans
2. Entity Beans
3. Message driven beans
Session Beans
● Session beans are intended to allow the application author to easily implement portions of application code in middleware and to simplify access to this code.
● Represents a single client inside the server
● The client calls the session bean to invoke methods of an application on the server
● Perform works for its client, hiding the complexity of interaction with other objects in the server
● Is not shared
● Is not persistent
● When the client stops the session, the bean can be assigned to another client from the server Session beans are divided into two types:
(i) Stateless Session Bean: Stateless Session Bean is intended to be simple and “light weight” components. The client, thereby making the server highly scalable, if required, maintains any state. Since no state is maintained in this bean type, stateless session beans are not tied to any specific client, hence any available instance of a stateless session bean can be used to service a client.
● Not maintain a conversational state for a client
● Contains values only for the duration of the single invocation
● Except during method invocation, all instances of stateless session bean are equivalent
(ii) Stateful Session Bean: Stateful Session Bean provides easy and transparent state management on the server side. Because state is maintained in this bean type, the application server manages client/bean pairs. Stateful session beans can access persistent resources on behalf of the client, but unlike entity beans, they do not actually represent the data.
Difference between Stateless and Stateful EJB are as follows,
Stateless:
1. Normally data members are not put in stateless session bean
2. Stateless beans are pooled
3. No effort for keeping client specific data
4. No Activation Z Passivation in stateless session bean
Stateful:
5. Data members that represent state are present in Stateful session bean
6. Stateful beans are cached
7. Setting the tag idle-timeout-seconds determines how long data is maintained in
Stateful session bean
Q16) Write the Difference between Stateless and Stateful EJB?
A16) Difference between Stateless and Stateful EJB are as follows,
Stateless:
1. Normally data members are not put in stateless session bean
2. Stateless beans are pooled
3. No effort for keeping client specific data
4. No Activation Z Passivation in stateless session bean
Stateful:
5. Data members that represent state are present in Stateful session bean
6. Stateful beans are cached
7. Setting the tag idle-timeout-seconds determines how long data is maintained in
Stateful session bean
Q17) Why Use EJBs?
A17) Some of the features of an application server include the following:
● Client Communication: The client, which is often a user interface, must be able to call the methods of objects on the application server via agreed-upon protocols.
● Session State Management: You'll recall our discussions on this topic in the context of JSP (JavaServer Pages) and servlet development.
● Transaction Management: Some operations, for example, when updating data, must occur as a unit of work. If one update fails, they all should fail.
● Database Connection Management: An application server must connect to a database, often using pools of database connections for optimizing resources.
● User Authentication and Role-Based Authorization: Users of an application must often log in for security purposes. The functionality of an application to which a user is allowed access is often based on the role associated with a user ID.
● Asynchronous Messaging: Applications often need to communicate with other systems in an asynchronous manner; that is, without waiting for the other system to respond. This requires an underlying messaging system that provides guaranteed delivery of these asynchronous messages.
● Application Server Administration: Application servers must be administered. For example, they need to be monitored and tuned.
Q18) Describe the similarities and distinctions between Ruby and Python?
A18) Similarities:
● Language at a high level
● Multiple platforms are supported.
● Use the irb Server Side Scripting Language interactive prompt.
Differences:
● Python is not totally object oriented, whereas Ruby is.
● Ruby has support for EclipseIDE, but Python has support for a variety of IDEs.
● Mixins are used in Ruby, but not in Python.
● Python does not support blocks, procs, or lambdas, although Ruby does.
Q19) In how many ways items can be removed from array in Ruby?
A19) Ruby array elements can be removed in different ways.
● pop
● shift
● delete
● uniq
Q20) What are versions of EJB?
A20) Version of EJB
● EJB 3.1
● EJB 3.2
● EJB 3.2 Final Release (2013-05-28)
● EJB 3.1 Final Release (2009-12-10)
● EJB 3.0 Final Release (2006-05-11)
● EJB 2.1, Final Release (2003-11-24)
● EJB 2.0 Final Release (2001-08-22)
● EJB 1.1 Final Release (1999-12-17)
● EJB 1.0 (1998-03-24)