Unit - 2
Shell Programming and Internet
Q1) Describe script basics?
A1) Shell Scripting
Shell Scripting is a free and open-source programming software that runs on the Unix/Linux shell. Shell Scripting is a program that allows you to write a series of commands to be executed by the shell. It can condense long and repetitive command sequences into a single, simple script that can be saved and executed at any time, reducing programming time.
Shells such as bash and korn in Linux support programming constructs that can be stored as scripts. Many Linux commands are scripted as a result of these scripts being shell commands.
To understand how their servers and applications are started, updated, maintained, or disabled, and to understand how a user interface is designed, a system administrator should have a basic understanding of scripting.
Shells are usually interactive, which means they accept user commands as input and execute them. However, there are times when we need to run a series of commands on a regular basis, and we must type all commands into the terminal each time.
We can write these commands in a file and execute them in shell to avoid this repetitive work because shell can take commands from files as input. Shell Scripts or Shell Programs are the names given to these files. The batch file in MS-DOS is identical to shell scripts. Each shell script has a.sh file extension, such as myscript.sh.
Shell scripts use the same syntax as other programming languages. It will be very simple to get started if you have previous experience with any programming language such as Python, C/C++, or others.
The following elements make up a shell script:
● Shell Keywords – if, else, break etc.
● Shell commands – cd, ls, echo, pwd, touch etc.
● Functions
● Control flow – if..then..else, case and shell loops etc.
Q2) How to create a shell script and generate output?
A2) Text editors are used to build Shell Scripts. Open a text editor program on your Linux system, create a new file, and begin typing a shell script or shell programming. Next, grant the shell permission to execute your shell script, and save your script anywhere the shell can find it.
Let's take a look at how to make a Shell Script:
● Using a vi editor, create a file (or any other editor). .sh is the extension for a script file.
● #! /bin/sh is a good way to start the script.
● Make a program.
● Filename.sh is the name of the script file.
● Type bash filename.sh to run the script.
"#!" is a shebang operator that sends the script to the interpreter's position. The script is guided to the bourne-shell if we use "#! /bin/sh."
Let's make a quick script -
#!/bin/bash
# My first script
Echo "Hello World!"
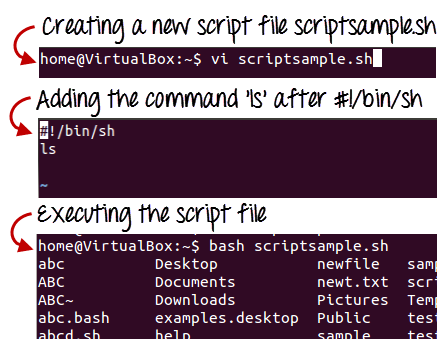
Fig 1: Steps to create shell scripting in linux
Generating output:
The read command is used in the following script to take input from the keyboard, allocate it to the variable Individual, and finally print it on STDOUT.
#!/bin/sh
# Author : Zara Ali
# Copyright (c) Tutorialspoint.com
# Script follows here:
Echo "What is your name?"
Read PERSON
Echo "Hello, $PERSON"
Output:
Here's an example of the script in action:
$./test.sh
What is your name?
Zara Ali
Hello, Zara Ali
$
Example:
The wc command in Bash is used to count a file's total number of lines, sentences, and characters. To generate the output, this command uses the options -c, -w, and -l, as well as the argument filename. To test the next script, create a text file called fruits.txt with the following details. Text file
Fruits.txt
Mango
Orange
Banana
Grape
Guava
Apple
To count and store the total number of words in the fruits.txt file into a variable, $count words, and print the value using the echo command, run the following commands.
$ count_words=wc -w fruits.txt
$ echo "Total words in fruits.txt is $count_words"
Output:

Q3) Explain conditional statements with examples?
A3) Normally, the shell runs the commands in a script in the order they are written in the text, one after the other.
Frequently, though, you will want to alter the way commands are executed.
Depending on the circumstances, you may want to run one command over and over, or you may want to run a command several times.
The shell provides a number of control mechanisms that can be used to change the order in which commands are executed.
There are two different types of selection mechanisms that allow you to choose between different commands:
● if/then/elif/else/fi
● case
The if statement
The if statement allows you to decide whether to run a command (or a group of commands) based on a condition.
The most basic version of this structure is as follows:
If conditional expression
Then
Command(s)
Fi
When the shell comes across a structure like this, it tests to see if the conditional expression is valid first.
If this is the case, the shell executes any commands found between the then and the fi (which is just if spelled backwards).
The shell skips the commands between then and fi if the conditional expression is false.
Example:
Here's a shell script that makes use of easy if statements:
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!
Fi
The test commands
In this conditional expression, we used the test instruction.
The phrase is:
Test $1 = Fri
The test command tests to see if the parameter $1 contains Fri; if it does, the condition is valid, and the message is printed.
The test command may run a number of tests; see the documentation for more details.
Using elif and else
Combining the if statement with the elif (“then if”) and else statements allows one to create even more complex selection structures.
Here's an easy example.
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!"
Elif test $1 = Sat 11 test $1 = Sun
Then
Echo "You should not be here working."
Echo "Log off and go home."
Else
Echo "It is not yet the weekend."
Echo "Get to work!"
Fi
The case Statement
On certain UNIX systems, the shell offers another selection structure that could be faster than the if argument.
This is the case statement, which takes the following form:
Case word in
Patternl) command(s) ;;
Pattern2) command(s) ;;
...
PatternN) command(s) ;;
Esac
The case statement compares word to pattern1, and if the two are equal, the shell executes the command(s) on the first line.
Otherwise, the shell goes through the remaining patterns one by one until it finds one that fits the expression, at which point it executes the command(s) on that line.
The default value is *.
Example:
Here's an example of a basic shell script that employs case statements:
#!/bin/sh
Set ‘date‘
Case $1 in
Fri) echo "Thank goodness it’s Friday!";;
Sat | Sun) echo "You should not be here working"; echo "Log off and go home!";;
*) echo "It is not yet the weekend."; echo "Get to work!";
Esac
Q4) Explain looping statements?
A4) for Loops
We sometimes want to repeat a command (or a set of commands).
Iteration, repetition, or looping are terms used to describe this method.
The for loop is the most common shell repetition structure, and it has the following general form:
For variable in list
Do
Command(s)
Done
Example:
Here's an easy example:
#!/bin/sh
For host in $*
Do
Ping $host
Done
While Loops
The while loop is described as follows:
While condition
Do
Command(s)
Done
The commands between the do and the done are executed as long as the condition is true.
Example:
#!/bin/sh
# Print a message ten times
Count=10
While test $count -gt 0
Do
Echo $*
Count=‘expr $count - 1
Done
Until Loops
The until loop is another form of iteration structure.
It takes the following general form:
Until condition
Do
Command
Done
Unless the condition is valid, this loop continues to execute the command(s) between do and done.
Instead of using while loops, we can rewrite the previous script using an until loop:
#!/bin/sh
# Print a message ten times
Count=10
Until test $count -eq 0
Do
Echo $*
Count=‘expr $count
Done
Conditional Execution
The Exit Code of the first command is used in the following two examples to conditionally execute the second command. The logical AND operator, &&, only runs the second command if the first one was good.
$ gcc myprog.c && a.out
If the first command fails, the logical OR operator || executes the second command.
$ gcc myprog.c || echo compilation failed
Q5) What do you mean by continue and break statement?
A5) Continue statement
The continue statement is identical to the break instruction, except that it only exits the current loop iteration, not the entire loop.
If an error occurs, but you still want to attempt to perform the next iteration of the loop, use this expression.
Syntax:
Continue
The continue instruction, like the break statement, can be given an integer argument to skip commands from nested loops.
Continue n
The nth enclosing loop to proceed from is defined by n.
Example:
The continue statement is used in the following loop, which returns from the continue statement and begins processing the next statement.
#!/bin/sh
NUMS="1 2 3 4 5 6 7"
For NUM in $NUMS
Do
Q=expr $NUM % 2
If [ $Q -eq 0 ]
Then
Echo "Number is an even number!!"
Continue
Fi
Echo "Found odd number"
Done
When you run it, you'll get the following result:
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Break statement
Since all of the lines of code up to the break statement have been executed, the break statement is used to end the loop's execution. It then descends to the code following the loop's conclusion.
Syntax:
To get out of a loop, use the break statement below.
Break
This format can also be used to exit a nested loop with the break command -
Break n
The nth enclosing loop to exit from is defined by n.
Example:
Here's a simple example that demonstrates how the loop ends when an equals 5.
#!/bin/sh
a=0
While [ $a -lt 10 ]
Do
Echo $a
If [ $a -eq 5 ]
Then
Break
Fi
a=expr $a + 1
Done
When you run it, you'll get the following result:
0
1
2
3
4
5
Here's a simple nested for loop illustration. If var1 equals 2 and var2 equals 0, this script exits all loops.
#!/bin/sh
For var1 in 1 2 3
Do
For var2 in 0 5
Do
If [ $var1 -eq 2 -a $var2 -eq 0 ]
Then
Break 2
Else
Echo "$var1 $var2"
Fi
Done
Done
When you run it, you'll get the following result. You have a break command with the argument 2 in the inner loop. This means that if a condition is met, you can exit the outer loop and eventually the inner loop as well.
1 0
1 5
Q6) Write history of the Internet?
A6) It is an interconnected computer network system that spans the globe. It makes use of the TCP/IP protocol, which is widely used on the internet. A unique IP address is assigned to every machine on the Internet. An IP Address (for example, 110.22.33.114) is a series of integers that identify the location of a computer.
A DNS (Domain Name Server) computer is used to give an IP Address a name so that a user can find a computer by name.
ARPANET was expanded to connect the Department of Defense with US colleges conducting defense-related research. It included most of the country's major universities. When University College London (UK) and Royal Radar Network (Norway) joined to the ARPANET and formed a network of networks, the concept of networking received a boost.
Stanford University's Vinton Cerf, Yogen Dalal, and Carl Sunshine invented the name "internet" to characterize this network of networks. They also collaborated on protocols to make information sharing via the Internet easier. The Transmission Control Protocol (TCP) is still the networking's backbone.
In the 1960s, the Internet was created as a means for government researchers to communicate information. In the 1960s, computers were enormous and stationary, and in order to access information stored on them, one had to either travel to the computer's location or have magnetic computer tapes transported through the mail system.
The escalation of the Cold War was another factor in the development of the Internet. The launch of the Sputnik satellite by the Soviet Union prompted the United States Defense Department to examine how information may be distributed even after a nuclear attack. This eventually led to the creation of the ARPANET (Advanced Research Projects Agency Network), which eventually evolved into the Internet we know today. ARPANET was a huge success, but it was only open to a few academic and research institutions with Defense Department contracts. As a result, new networks were formed to facilitate information sharing.
The Internet celebrates its formal birthday on January 1, 1983. Prior to this, there was no standard means for computer networks to communicate with one another. Transfer Control Protocol/Internetwork Protocol (TCP/IP) was created as a new communication protocol.
This allowed various types of computers on various networks to "speak" to one another. On January 1, 1983, ARPANET and the Defense Data Network switched to the TCP/IP standard, resulting in the birth of the Internet. A universal language could now connect all networks.
Q7) Explain SMTP?
A7) Simple Mail Transfer Protocol (SMTP)
E-mail system is implemented with the help of Message Transfer Agents (MTA). There are normally two MTAs in each mailing system. One for sending e-mails and another for receiving e-mails. The formal protocol that defines the MTA client and server in the internet is called Simple Mail Transfer Protocol (SMTP).
Fig shows the range of SMTP protocol.
By referring the above diagram, we can say that SMTP is used two times. That is between the sender and sender’s mail server and between the sender’s mail server and receiver’s mail server. Another protocol is used between the receiver’s mail server and receiver.
SMTP simply defines how commands and responses must be sent back and forth.
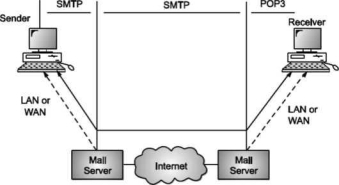
SMTP is a simple ASCII protocol
It establishes a TCP connection between a sender and port number 25 of the receiver. No checksums are generally required because TCP provides a reliable byte stream. After exchanging all the e-mail, the connection is released.
Commands and Responses
SMTP uses commands and responses to transfer messages between an MTA client and MTA server.
Each command or reply is terminated by a two-character (carriage return and line feed) end-of-line token.

Fig. Commands and Responses
Commands
Client sends commands to the server. SMTP defines 14 commands. Out of that first 5 commands are mandatory; every implementation must support these commands.
The next three commands are often used and are highly recommended. Last six commands are hardly used.
Q8) Explain POP?
A8) POP
Post Office Protocol, version 3 (POP3) is simple and feature-limited. The POP3 client software is installed on the receiving machine; the POP3 server software is installed on the mail server.
If the user wants to download e-mails from the mailbox on the mail server, mail access begins with the client. On TCP port 110, the client opens a connection to the server. In order to reach the mailbox, it then sends the user name and password. The user will then, one by one, list and retrieve the mail messages.
There are two modes for POP3: the delete mode and the hold mode. In the delete mode, after each retrieval, the mail is removed from the mailbox. In keep mode, after retrieval, the mail stays in the mailbox. When the user is operating on their permanent device, the delete mode is usually used and after reading or replying, the received mail can be saved and sorted. Normally, the keep mode is used when the user accesses her mail away from her main computer (e.g., a laptop). For later retrieval and organisation, the mail is read but kept in the system.
In some aspects, POP3 is deficient. It does not allow the user to arrange his mail on the server; it is impossible for the user to have multiple folders on the server. (The user will build directories on their own computer, of course.)
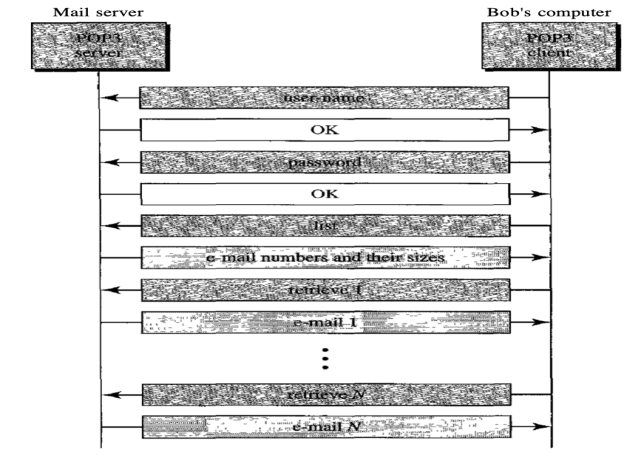
Fig: Command and response of POP3
Advantages of POP3
- As they are already saved on our PC, it provides easy and simple access to the emails.
- The size of the email that we receive or send has no limit.
- When all emails are saved on the local computer, it takes less server storage space.
- The maximum size of a mailbox is available, but the size of the hard disc is limited.
- It is a straightforward protocol, so it is one of the most common protocols used today.
- Configuring and using it is simple.
Disadvantages of POP3
- By default, if the emails are downloaded from the server, all the emails are deleted from the server. Mails will also not be accessed from other computers unless they are programmed to leave a mail copy on the server.
- It can be tough to move the mail folder from the local computer to another machine.
- As all of the attachments are placed on your local computer, if the virus scanner does not scan them, there is a high chance of a virus attack. Virus attacks can destroy your machine.
- There could also be corruption in the email folder that is downloaded from the mail server.
- Mails are saved on a local server, so the email folder can be accessed by someone who is sitting on your machine.
Q9) What is E-mail?
A9) E-mail is defined as the transmission of messages on the Internet. It is one of the most commonly used features over communications networks that may contain text, files, images, or other attachments. Generally, it is information that is stored on a computer sent through a network to a specified individual or group of individuals.
Email messages are conveyed through email servers; it uses multiple protocols within the TCP/IP suite. For example, SMTP is a protocol, stands for simple mail transfer protocol and used to send messages whereas other protocols IMAP or POP are used to retrieve messages from a mail server. If you want to login to your mail account, you just need to enter a valid email address, password, and the mail servers used to send and receive messages.
Although most of the webmail servers automatically configure your mail account, therefore, you only required to enter your email address and password. However, you may need to manually configure each account if you use an email client like Microsoft Outlook or Apple Mail. In addition, to enter the email address and password, you may also need to enter incoming and outgoing mail servers and the correct port numbers for each one.
Q10) What are the uses of email?
A10) Uses of email
Email can be used in different ways: it can be used to communicate either within an organization or personally, including between two people or a large group of people. Most people get benefit from communicating by email with colleagues or friends or individuals or small groups. It allows you to communicate with others around the world and send and receive images, documents, links, and other attachments. Additionally, it offers benefit users to communicate with the flexibility on their own schedule.
There is another benefit of using email; if you use it to communicate between two people or small groups that will beneficial to remind participants of approaching due dates and time-sensitive activities and send professional follow-up emails after appointments. Users can also use the email to quickly remind all upcoming events or inform the group of a time change. Furthermore, it can be used by companies or organizations to convey information to large numbers of employees or customers. Mainly, email is used for newsletters, where mailing list subscribers are sent email marketing campaigns directly and promoted content from a company.
Email can also be used to move a latent sale into a completed purchase or turn leads into paying customers. For example, a company may create an email that is used to send emails automatically to online customers who contain products in their shopping cart. This email can help to remind consumers that they have items in their cart and stimulate them to purchase those items before the items run out of stock. Also, emails are used to get reviews by customers after making a purchase. They can survey by including a question to review the quality of service.
Q11) Write advantages of Email?
A11) Advantages of Email
There are many advantages of email, which are as follows:
● Cost-effective: Email is a very cost-effective service to communicate with others as there are several email services available to individuals and organizations for free of cost. Once a user is online, it does not include any additional charge for the services.
● Email offers users the benefit of accessing email from anywhere at any time if they have an Internet connection.
● Email offers you an incurable communication process, which enables you to send a response at a convenient time. Also, it offers users a better option to communicate easily regardless of different schedules users.
● Speed and simplicity: Email can be composed very easily with the correct information and contacts. Also, minimum lag time, it can be exchanged quickly.
● Mass sending: You can send a message easily to large numbers of people through email.
● Email exchanges can be saved for future retrieval, which allows users to keep important conversations or confirmations in their records and can be searched and retrieved when they needed quickly.
● Email provides a simple user interface and enables users to categorize and filter their messages. This can help you recognize unwanted emails like junk and spam mail. Also, users can find specific messages easily when they are needed.
● As compared to traditional posts, emails are delivered extremely fast.
● Email is beneficial for the planet, as it is paperless. It reduces the cost of paper and helps to save the environment by reducing paper usage.
● It also offers a benefit to attaching the original message at the time you reply to an email. This is beneficial when you get hundreds of emails a day, and the recipient knows what you are talking about.
● Furthermore, emails are beneficial for advertising products. As email is a form of communication, organizations or companies can interact with a lot of people and inform them in a short time.
Q12) Explain the steps to compose and send e-mail?
A12) To write and send e-mail with data and messages, do the following:
Step 1: In order to create a new e-mail and send data through it a user has to click on “Compose” option available at the top of inbox option as shown in below picture.
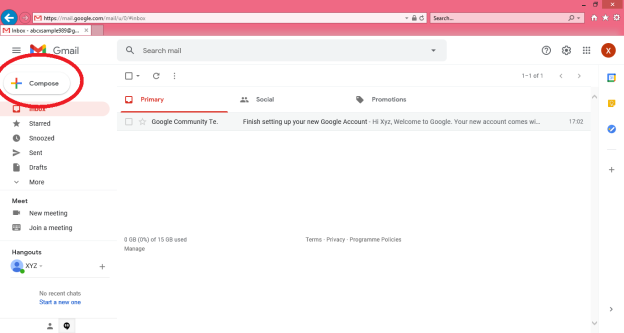
Step 2: A “New Message” box will appear as you can see in the below picture asking you to enter details in order to create a new e-mail message.
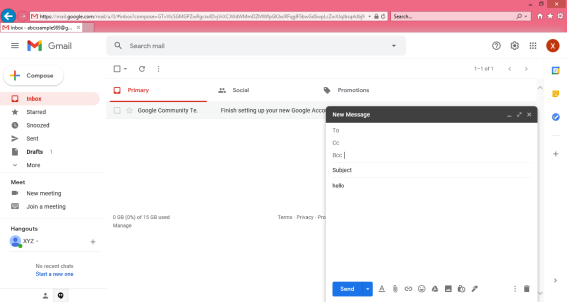
Note: New messages in an e-mail are stored in Inbox folder of the e-mail and all saved messages appears in draft folder of your e-mail.
Here, In the New Message box, you will see the following things:
To: Mail ID of the recipient
Cc: To send a Carbon copy i.e., send this mail to several people at the same time and also show everyone the email id’s of all the other people who have received it.
Bcc: To send a Blind Carbon copy i.e., send this mail to several people at the same time but without showing the person in Bcc list about who else has received this mail.
Subject: Is the main part which will be shown first to the person receiving the mail. It should be short and relevant to your mail and must tell the reason for your e-mail.
Body: The body of the e-mail is the blank white part where the user can type his message and can even customise it. In above picture we have written “Hello” to show the body of this e-mail.
Insert Bar: This bar is available at the bottom of the e-mail compose window. It includes various options to insert document, links, emoji’s, Google drive files, images, signature, delete the email etc.

User simply has to click on one of these options to insert an object and a new window will appear that will ask you about the file location which you want to insert into this mail. After locating the file just click on insert and the file will start uploading to this e-mail, once it is done it can be seen in the e-mail window. You can also open or remove the file from the e-mail using the options available alongside your uploaded file.
Delete button: There is a dustbin icon at the end of this email which lets a user to delete or discard there typed mail. Once discarded the mail cannot be retrieved.
Note: The close option is also available at the top of the window but that option will not delete the e-mail you have typed it simply closes the window and save the typed e-mail in drafts folder for future editing or usage purpose.
Step 3: The send icon is the blue one which is used to send the e-mail once it is composed. All the files inserted along with the text will be sent to the e-mail addresses once the user clicks on this button.
Note: There are various other options available under this Mail compose window such as Print, Schedule send, Minimize and Maximize the window which can be used for better e-mail experience of the user.
Q13) What is SMTP working?
A13) SMTP Working
SMTP works in the following three stages:
a) Connection Establishment
b) Message Transfer
c) Connection Termination
These are explained below:
Connection Establishment
Once the TCP connection is made on port no. 25, SMTP server starts the connection phase. This phase involves following three steps which are explained in the Fig.
The server tells the client that it is ready to receive mail by using the code 220. If the server is not ready, it sends code 421 which tells that service is not available.
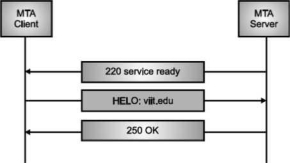
Fig. Connection Establishment
Once the server becomes ready to receive the mails, client sends HELO message to identify itself using the domain name address. This is important step which informs the server of the domain name of the client. Remember that during TCP connection establishment, the sender and receiver know each other through their IP addresses.
Server responds with code 250 which tells that the request command is completed. Message Transfer Once the connection has been established, SMTP server sends messages to SMTP receiver.
The messages are transferred in three stages
- A MAIL command identifies the message originator.
- RCPT command identifies the receiver of the message.
- DATA command transfers the message text.
Connection Closing
After the message is transferred successfully, the client terminates the connection. The connection is terminated in two steps The client sends the quit command.
The server responds with code 221 or some other appropriate code. After the connection termination phase, the TCP connection must be closed.
MIME Short for Multipurpose Internet Mail Extensions, a specification for formatting non-ASCII messages so that they can be sent over the Internet.
Many e-mail clients now support MIME, which enables them to send and receive graphics, audio, and video files via the Internet mail system. In addition, MIME supports messages in character sets other than ASCII.
There are many predefined MIME types, such as GIF graphics files and PostScript files. It is also possible to define your own MIME types.
In addition to e-mail applications, Web browsers also support various MIME types. This enables the browser to display or output files that are not in HTML format.
Q14) Write the disadvantages of email?
A14) Disadvantages of Email
● Impersonal: As compared to other forms of communication, emails are less personal. For example, when you talk to anyone over the phone or meeting face to face is more appropriate for communicating than email.
● Misunderstandings: As email includes only text, and there is no tone of voice or body language to provide context. Therefore, misunderstandings can occur easily with email. If someone sends a joke on email, it can be taken seriously. Also, well-meaning information can be quickly typed as rude or aggressive that can impact wrong. Additionally, if someone types with short abbreviations and descriptions to send content on the email, it can easily be misinterpreted.
● Malicious Use: As email can be sent by anyone if they have an only email address. Sometimes, an unauthorized person can send you mail, which can be harmful in terms of stealing your personal information. Thus, they can also use email to spread gossip or false information.
● Accidents Will Happen: With email, you can make fatal mistakes by clicking the wrong button in a hurry. For instance, instead of sending it to a single person, you can accidentally send sensitive information to a large group of people. Thus, the information can be disclosed, when you have clicked the wrong name in an address list. Therefore, it can be harmful and generate big trouble in the workplace.
● Spam: Although in recent days, the features of email have been improved, there are still big issues with unsolicited advertising arriving and spam through email. It can easily become overwhelming and takes time and energy to control.
● Information Overload: As it is very easy to send email to many people at a time, which can create information overload. In many modern workplaces, it is a major problem where it is required to move a lot of information and impossible to tell if an email is important. And, email needs organization and upkeep. The bad feeling is one of the other problems with email when you returned from vacation and found hundreds of unopened emails in your inbox.
● Viruses: Although there are many ways to travel viruses in the devices, email is one of the common ways to enter viruses and infect devices. Sometimes when you get a mail, it might be the virus come with an attached document. And, the virus can infect the system when you click on the email and open the attached link. Furthermore, an anonymous person or a trusted friend or contact can send infected emails.
● Pressure to Respond: If you get emails and you do not answer them, the sender can get annoyed and think you are ignoring them. Thus, this can be a reason to make pressure on your put to keep opening emails and then respond in some way.
● Time Consuming: When you get an email and read, write, and respond to emails that can take up vast amounts of time and energy. Many modern workers spend their most time with emails, which may be caused to take more time to complete work.
● Overlong Messages: Generally, email is a source of communication with the intention of brief messages. There are some people who write overlong messages that can take much time than required.
● Insecure: There are many hackers available that want to gain your important information, so email is a common source to seek sensitive data, such as political, financial, documents, or personal messages. In recent times, there have various high-profile cases occurred that shown how email is insecure about information theft.
Q15) Explain IMAP?
A15) Internet Message Access Protocol (IMAP) is an acronym for Internet Message Access Protocol. It's an application layer protocol that allows you to receive emails from a mail server. POP3 is one of the most widely used protocols for retrieving emails.
The client/server model is also used. On the one hand, we have an IMAP client, which is a computer programme. On the other hand, we have an IMAP server, which is likewise a computer-based operation. The two computers are linked through a network.
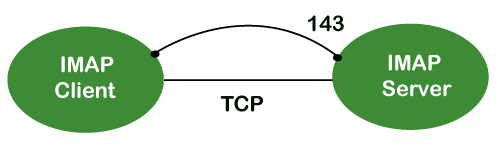
Because the IMAP protocol is based on the TCP/IP transport layer, it makes use of the protocol's dependability implicitly. The IMAP server listens to port 143 by default whenever a TCP connection is established between the IMAP client and the IMAP server, although this port number can be altered.
IMAP is configured to use two ports by default:
● Port 143: It is a non-encrypted IMAP port.
● Port 993: This port is used when IMAP client wants to connect through IMAP securely.
IMAP History and Standards
IMAP version 2 was the first version of IMAP to be formally documented as an internet standard in RFC 1064, which was published in July 1988. It was modified in August 1990, with the same version, in RFC 1176. As a result, they created IMAP3, a new version 3 document. In February 1991, the RFC 1203 was published. However, because IMAP3 was never embraced by the market, users continued to use IMAP2. IMAPb is, a protocol extension, was later built to add support for Multipurpose Internet Mail Extensions (MIME) to IMAP. Because of MIME's utility, this was a significant advance.
IMAPb is was never published as an RFC, despite this. This could be due to issues with the IMAP3 protocol. IMAP version 4, or IMAP4, was released in two RFCs in December 1994: RFC 1730, which described the basic protocol, and RFC 1731, which described the authentication mechanism for IMAP 4. IMAP 4 is the most recent and extensively used version of IMAP. It is still being developed, and the most recent version is known as IMAP4rev1 and is defined in RFC 2060. RFC 3501 is the most recent revision.
IMAP Features
IMAP was created for a specific purpose: to give users more flexibility in how they access their inbox. It can function in any of the three modes: online, offline, or disconnected. The offline and disconnected modes are of particular importance to most protocol users.
An IMAP protocol has the following characteristics:
● Access and retrieve mail from a distant server: The user can access and retrieve mail from a remote server while keeping the messages there.
● Set message flags: The user can keep track of which messages he has already viewed by setting message flags.
● Manage multiple mailboxes: The user has the ability to manage several mailboxes and transfer messages between them. For those working on numerous projects, the user can categorise them into various categories.
● Determine information prior to downloading: Before downloading the mail from the mail server, it decides whether to retrieve or not.
● Downloads a portion of a message: It allows you to download a component of a message, such as a single body part from the mime-multi part. This is useful when a message's short-text element contains huge multimedia files.
● Search: Users can conduct a search for the contents of emails.
● Check email-header: Examine the email header: Before downloading, users can check the email header.
Q16) Write the advantages of IMAP?
A16) Advantages
The following are some of the benefits of IMAP:
● It enables us to compose email messages from any location and on as many different devices as we like.
● Only when we click on a message can it be downloaded. As a result, we don't have to wait for the server to download all of our new messages before we can view them.
● With IMAP, attachments are not immediately downloaded. As a result, you can check your messages much faster and have more control over which attachments are accessed.
● IMAP, like the Post Office Protocol, can be utilised offline (POP).
● It allows you to delete messages, search for keywords in the body of emails, create and manage multiple mailboxes or folders, and display the headers of emails for quick visual searches.
Q17) Explain different web browsers?
A17) Web browsers are programmes that are installed on your computer. A web browser, such as Netscape Navigator, Microsoft Internet Explorer, or Mozilla Firefox, is required to access the Internet.
Currently, you must navigate through our website tutorialspoint.com using any type of Web browser. Web browsing or web surfing is the process of navigating through pages of information on the Internet.
There are four major web browsers: Internet Explorer, Firefox, Netscape, and Safari, but there are several others. Complete Browser Statistics may be of interest to you. Now we'll take a closer look at these browsers.
We should aim to make a site compatible with as many browsers as feasible when developing it. Especially sites should be adaptable to main browsers like Explorer, Firefox, Chrome, Netscape, Opera, and Safari.
Internet Explorer

Microsoft's Internet Explorer (IE) is a software product. This is the most widely used web browser on the planet. This was debuted in 1995 in conjunction with the release of Windows 95, and it surpassed Netscape in popularity in 1998.
Internet Explorer (also known as IE or MSIE) is a free web browser that allows users to view web pages on the internet. It can also be used to access online banking, internet marketing, listen to and watch streaming videos, and many other things. Microsoft first released it in 1995. It was created in response to Netscape Navigator, the first geographical browser.
For many years, from 1999 to 2012, Microsoft Internet Explorer was the most used web browser, surpassing Netscape Navigator. Network file sharing, multiple internet connections, active Scripting, and security settings are all included.
Google Chrome

Google created this web browser, and the beta version was published on September 2, 2008 for Microsoft Windows. Chrome is now one of the most popular web browsers, with a global market share of more than 50%.
Google Chrome is the most popular open-source internet browser for accessing information on the World Wide Web. It was released on December 11, 2008, by Google for Windows, Linux, Mac OS X, Android, and iOS users. It provides Web security through a sandboxing-based technique. It also adheres to web standards such as HTML5 and CSS (cascading style sheet).
Google Chrome was the first online browser to integrate the search box and address bar, a feature that was quickly replicated by other competitors. Google launched the Chrome Web Store in 2010, allowing users to purchase and install Web-based applications.
Mozilla Firefox

Mozilla has created a new browser called Firefox. It was first introduced in 2004 and has since evolved to become the Internet's second most used browser.
Mozilla Firefox is an open-source web browser that allows users to access information on the Internet. Firefox, the popular Web browser, has a simpler user interface and better download speeds than Internet Explorer. To translate web pages, it employs the Gecko layout engine, which follows current and predicted web standards.
Firefox was popular as a replacement for Internet Explorer 6.0 because it protected users from spyware and harmful websites. After Google Chrome, Apple Safari, and UC Browser, it was the fourth most popular web browser in 2017.
Safari

Safari is an Apple Inc. Web browser that comes standard with Mac OS X. It was originally made available to the public as a public beta in January 2003. Safari offers excellent compatibility for modern technologies like as XHTML and CSS2.
Safari is a web browser for the iPhone, iPad, and iPod Touch, as well as the Macintosh and Windows operating systems. Apple, Inc. Released it on June 30, 2003. It is the primary browser for Apple's operating systems, such as OS X for MacBook and Mac computers and iOS for iPad and iPhone smartphones and tablets. After Microsoft Internet Explorer, Mozilla Firefox, and Google Chrome, it is the fourth most used browser. It makes use of the WebKit engine, which handles font rendering, graphics display, page layout, and JavaScript execution.
Opera

Opera is a full-featured browser that is smaller and faster than most other browsers. With a keyboard interface, numerous windows, zoom functions, and more, it's quick and easy to use. There are Java and non-Java versions available. Ideal for novices to the Internet, schoolchildren, the handicapped, and as a CD-Rom and kiosk front-end.
Konqueror

Konqueror is an HTML 4.01 compliant web browser that also supports Java applets, JavaScript, CSS 1, CSS 2.1, and Netscape plugins. This programme functions as a file manager and offers basic file management on local UNIX filesystems, ranging from simple cut/copy/paste operations to advanced remote and local network file browsing.
Lynx

Lynx is a full-featured Web browser for Unix, VMS, and other platforms with cursor-addressable character-cell terminals or emulators.
Q18) Define read command?
A18) The read command in Linux is used to read a line's contents into a variable. For Linux systems, this is a built-in command. As a result, we won't need to install any more software. When writing a bash script, it's a simple tool to utilise to get user input. It's a useful tool, just like the echo command and the positional parameter. It's used to separate the words associated with the shell variable. It is mostly used to receive user input, but it can also be used to implement functions while receiving input.
Syntax:
The read command has the following basic syntax:
Read [options] [name...]
How to use the read command?
With and without arguments, the read command can be used. Let's have a look at how the read command might be used in different situations:
● Default Behaviour
If we run the read command without any arguments, it will accept a line as user input and save it in the 'REPLY' built-in variable. Run the command as follows:
Read
The command above will prompt the user for input. To save the user input, type it in and hit the ENTER key. Execute the command as follows to see the entered content:
Echo $REPLY
The above command will display the 'REPLY' variable's stored input.
● Specify the variables to store the values
We can define the variables that will be used to store the data. If the number of provided variables is less than the number of entered words, all leftover words will be stored in the last variable by default. Consider the command below:
Read var1 var2 var3
● read -p
For the prompt text, the '-p' option is used. It reads the data as well as some hints. This tip text guides us through the process of typing text, such as what to type. Consider the command below:
Read -p " Enter your name: "
The command above will prompt you for your name; type it in. The name will be saved in the variable 'REPLY.' Execute the following command to see the variable value:
Echo " My name is $REPLY"
● read -n
The '-n' option shortens the character length in the entered text. It will not allow you to type more than the number of characters specified. It will automatically cease reading after the maximum number of characters has been reached. Execute the following command to limit the number of characters to six:
Read -n 6 -p " Enter 6 characters only: "
We can't enter more than 6 characters with the instruction above.
● read -s
For security reasons, the '-s' option is utilised. It is used to read sensitive information. The entered text will not appear in the terminal if this option is selected. With this option, we can use additional options. In this option, the characters are read aloud. Its primary function is to read passwords from the keyboard. Consider the command below:
Read -s -p "Enter password: "
The above command will demand for a password, but the password will not be displayed on the terminal when we type it.
Q19) Explain echo statement?
A19) In Linux, the echo command is used to display a line of text/string that has been supplied as an argument. This is a built-in command that outputs status text to the screen or a file. It's typically used in shell scripts and batch files.
Syntax:
Echo [option] [string]
Displaying a text/string:
Syntax:
Echo [string]
Example:
Echo -e "World \bis \bBeautiful"
Output
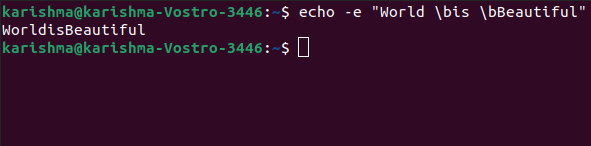
Implementations of the echo command
SymbOS, KolibriOS, HP MPE/iX, ReactOS, Microsoft Windows, IBM OS/2, Digital Research FlexOS, Acorn Computers Panos, Microwave OS-9, Zilog Z80-RIO, MetaComCo TRIPOS, TSC FLEX, Multics, Unix-like, and Unix operating systems all provide the echo command.
Several shells, including Csh-like shells (such as zsh or Bash), Bourne-like shells, COMMAND.COM, and cmd.exe, use the echo command as a built-in command.
The command also exists inside the EFI shell.
Echo Command Options
The echo command has a number of different options. The following alternatives are listed and explained:
1. b: Use this option to remove all spaces from the text/string.
Example
Echo -e "Flowers \bare \bBeautiful"
2.\c: This option is used in conjunction with the '-e' backspace interpreter to suppress the trailing new line and continue without emitting any new lines.
Example
Echo -e "World \cis Beautiful"
3. \n: This option is used to generate a new line, which will be formed from the location where it is used.
Example:
Echo -e "World \nis \nBeautiful"
4. Echo *: This option prints every folder or file in the system. It's the same as the Linux ls command.
Example:
Echo *
5. -n: This option is used to skip echoing new lines at the end.
Example:
Echo -n "World is Beautiful"
6. Print "Hello All!" on the terminal: Use the following command to print the text "Hello All!" on the terminal:
Example:
$ echo "Hello All!"
7. Print specified file types: For example, if we want to print every '.c' file, we can use the command below:
Example:
$ echo *.txt
Unit - 2
Shell Programming and Internet
Q1) Describe script basics?
A1) Shell Scripting
Shell Scripting is a free and open-source programming software that runs on the Unix/Linux shell. Shell Scripting is a program that allows you to write a series of commands to be executed by the shell. It can condense long and repetitive command sequences into a single, simple script that can be saved and executed at any time, reducing programming time.
Shells such as bash and korn in Linux support programming constructs that can be stored as scripts. Many Linux commands are scripted as a result of these scripts being shell commands.
To understand how their servers and applications are started, updated, maintained, or disabled, and to understand how a user interface is designed, a system administrator should have a basic understanding of scripting.
Shells are usually interactive, which means they accept user commands as input and execute them. However, there are times when we need to run a series of commands on a regular basis, and we must type all commands into the terminal each time.
We can write these commands in a file and execute them in shell to avoid this repetitive work because shell can take commands from files as input. Shell Scripts or Shell Programs are the names given to these files. The batch file in MS-DOS is identical to shell scripts. Each shell script has a.sh file extension, such as myscript.sh.
Shell scripts use the same syntax as other programming languages. It will be very simple to get started if you have previous experience with any programming language such as Python, C/C++, or others.
The following elements make up a shell script:
● Shell Keywords – if, else, break etc.
● Shell commands – cd, ls, echo, pwd, touch etc.
● Functions
● Control flow – if..then..else, case and shell loops etc.
Q2) How to create a shell script and generate output?
A2) Text editors are used to build Shell Scripts. Open a text editor program on your Linux system, create a new file, and begin typing a shell script or shell programming. Next, grant the shell permission to execute your shell script, and save your script anywhere the shell can find it.
Let's take a look at how to make a Shell Script:
● Using a vi editor, create a file (or any other editor). .sh is the extension for a script file.
● #! /bin/sh is a good way to start the script.
● Make a program.
● Filename.sh is the name of the script file.
● Type bash filename.sh to run the script.
"#!" is a shebang operator that sends the script to the interpreter's position. The script is guided to the bourne-shell if we use "#! /bin/sh."
Let's make a quick script -
#!/bin/bash
# My first script
Echo "Hello World!"
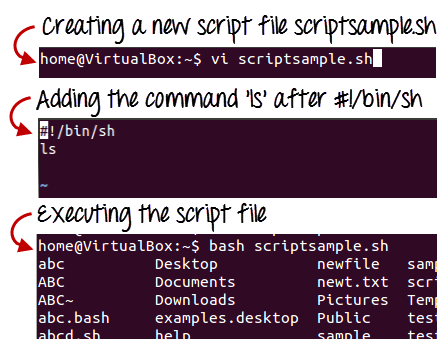
Fig 1: Steps to create shell scripting in linux
Generating output:
The read command is used in the following script to take input from the keyboard, allocate it to the variable Individual, and finally print it on STDOUT.
#!/bin/sh
# Author : Zara Ali
# Copyright (c) Tutorialspoint.com
# Script follows here:
Echo "What is your name?"
Read PERSON
Echo "Hello, $PERSON"
Output:
Here's an example of the script in action:
$./test.sh
What is your name?
Zara Ali
Hello, Zara Ali
$
Example:
The wc command in Bash is used to count a file's total number of lines, sentences, and characters. To generate the output, this command uses the options -c, -w, and -l, as well as the argument filename. To test the next script, create a text file called fruits.txt with the following details. Text file
Fruits.txt
Mango
Orange
Banana
Grape
Guava
Apple
To count and store the total number of words in the fruits.txt file into a variable, $count words, and print the value using the echo command, run the following commands.
$ count_words=wc -w fruits.txt
$ echo "Total words in fruits.txt is $count_words"
Output:

Q3) Explain conditional statements with examples?
A3) Normally, the shell runs the commands in a script in the order they are written in the text, one after the other.
Frequently, though, you will want to alter the way commands are executed.
Depending on the circumstances, you may want to run one command over and over, or you may want to run a command several times.
The shell provides a number of control mechanisms that can be used to change the order in which commands are executed.
There are two different types of selection mechanisms that allow you to choose between different commands:
● if/then/elif/else/fi
● case
The if statement
The if statement allows you to decide whether to run a command (or a group of commands) based on a condition.
The most basic version of this structure is as follows:
If conditional expression
Then
Command(s)
Fi
When the shell comes across a structure like this, it tests to see if the conditional expression is valid first.
If this is the case, the shell executes any commands found between the then and the fi (which is just if spelled backwards).
The shell skips the commands between then and fi if the conditional expression is false.
Example:
Here's a shell script that makes use of easy if statements:
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!
Fi
The test commands
In this conditional expression, we used the test instruction.
The phrase is:
Test $1 = Fri
The test command tests to see if the parameter $1 contains Fri; if it does, the condition is valid, and the message is printed.
The test command may run a number of tests; see the documentation for more details.
Using elif and else
Combining the if statement with the elif (“then if”) and else statements allows one to create even more complex selection structures.
Here's an easy example.
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!"
Elif test $1 = Sat 11 test $1 = Sun
Then
Echo "You should not be here working."
Echo "Log off and go home."
Else
Echo "It is not yet the weekend."
Echo "Get to work!"
Fi
The case Statement
On certain UNIX systems, the shell offers another selection structure that could be faster than the if argument.
This is the case statement, which takes the following form:
Case word in
Patternl) command(s) ;;
Pattern2) command(s) ;;
...
PatternN) command(s) ;;
Esac
The case statement compares word to pattern1, and if the two are equal, the shell executes the command(s) on the first line.
Otherwise, the shell goes through the remaining patterns one by one until it finds one that fits the expression, at which point it executes the command(s) on that line.
The default value is *.
Example:
Here's an example of a basic shell script that employs case statements:
#!/bin/sh
Set ‘date‘
Case $1 in
Fri) echo "Thank goodness it’s Friday!";;
Sat | Sun) echo "You should not be here working"; echo "Log off and go home!";;
*) echo "It is not yet the weekend."; echo "Get to work!";
Esac
Q4) Explain looping statements?
A4) for Loops
We sometimes want to repeat a command (or a set of commands).
Iteration, repetition, or looping are terms used to describe this method.
The for loop is the most common shell repetition structure, and it has the following general form:
For variable in list
Do
Command(s)
Done
Example:
Here's an easy example:
#!/bin/sh
For host in $*
Do
Ping $host
Done
While Loops
The while loop is described as follows:
While condition
Do
Command(s)
Done
The commands between the do and the done are executed as long as the condition is true.
Example:
#!/bin/sh
# Print a message ten times
Count=10
While test $count -gt 0
Do
Echo $*
Count=‘expr $count - 1
Done
Until Loops
The until loop is another form of iteration structure.
It takes the following general form:
Until condition
Do
Command
Done
Unless the condition is valid, this loop continues to execute the command(s) between do and done.
Instead of using while loops, we can rewrite the previous script using an until loop:
#!/bin/sh
# Print a message ten times
Count=10
Until test $count -eq 0
Do
Echo $*
Count=‘expr $count
Done
Conditional Execution
The Exit Code of the first command is used in the following two examples to conditionally execute the second command. The logical AND operator, &&, only runs the second command if the first one was good.
$ gcc myprog.c && a.out
If the first command fails, the logical OR operator || executes the second command.
$ gcc myprog.c || echo compilation failed
Q5) What do you mean by continue and break statement?
A5) Continue statement
The continue statement is identical to the break instruction, except that it only exits the current loop iteration, not the entire loop.
If an error occurs, but you still want to attempt to perform the next iteration of the loop, use this expression.
Syntax:
Continue
The continue instruction, like the break statement, can be given an integer argument to skip commands from nested loops.
Continue n
The nth enclosing loop to proceed from is defined by n.
Example:
The continue statement is used in the following loop, which returns from the continue statement and begins processing the next statement.
#!/bin/sh
NUMS="1 2 3 4 5 6 7"
For NUM in $NUMS
Do
Q=expr $NUM % 2
If [ $Q -eq 0 ]
Then
Echo "Number is an even number!!"
Continue
Fi
Echo "Found odd number"
Done
When you run it, you'll get the following result:
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Break statement
Since all of the lines of code up to the break statement have been executed, the break statement is used to end the loop's execution. It then descends to the code following the loop's conclusion.
Syntax:
To get out of a loop, use the break statement below.
Break
This format can also be used to exit a nested loop with the break command -
Break n
The nth enclosing loop to exit from is defined by n.
Example:
Here's a simple example that demonstrates how the loop ends when an equals 5.
#!/bin/sh
a=0
While [ $a -lt 10 ]
Do
Echo $a
If [ $a -eq 5 ]
Then
Break
Fi
a=expr $a + 1
Done
When you run it, you'll get the following result:
0
1
2
3
4
5
Here's a simple nested for loop illustration. If var1 equals 2 and var2 equals 0, this script exits all loops.
#!/bin/sh
For var1 in 1 2 3
Do
For var2 in 0 5
Do
If [ $var1 -eq 2 -a $var2 -eq 0 ]
Then
Break 2
Else
Echo "$var1 $var2"
Fi
Done
Done
When you run it, you'll get the following result. You have a break command with the argument 2 in the inner loop. This means that if a condition is met, you can exit the outer loop and eventually the inner loop as well.
1 0
1 5
Q6) Write history of the Internet?
A6) It is an interconnected computer network system that spans the globe. It makes use of the TCP/IP protocol, which is widely used on the internet. A unique IP address is assigned to every machine on the Internet. An IP Address (for example, 110.22.33.114) is a series of integers that identify the location of a computer.
A DNS (Domain Name Server) computer is used to give an IP Address a name so that a user can find a computer by name.
ARPANET was expanded to connect the Department of Defense with US colleges conducting defense-related research. It included most of the country's major universities. When University College London (UK) and Royal Radar Network (Norway) joined to the ARPANET and formed a network of networks, the concept of networking received a boost.
Stanford University's Vinton Cerf, Yogen Dalal, and Carl Sunshine invented the name "internet" to characterize this network of networks. They also collaborated on protocols to make information sharing via the Internet easier. The Transmission Control Protocol (TCP) is still the networking's backbone.
In the 1960s, the Internet was created as a means for government researchers to communicate information. In the 1960s, computers were enormous and stationary, and in order to access information stored on them, one had to either travel to the computer's location or have magnetic computer tapes transported through the mail system.
The escalation of the Cold War was another factor in the development of the Internet. The launch of the Sputnik satellite by the Soviet Union prompted the United States Defense Department to examine how information may be distributed even after a nuclear attack. This eventually led to the creation of the ARPANET (Advanced Research Projects Agency Network), which eventually evolved into the Internet we know today. ARPANET was a huge success, but it was only open to a few academic and research institutions with Defense Department contracts. As a result, new networks were formed to facilitate information sharing.
The Internet celebrates its formal birthday on January 1, 1983. Prior to this, there was no standard means for computer networks to communicate with one another. Transfer Control Protocol/Internetwork Protocol (TCP/IP) was created as a new communication protocol.
This allowed various types of computers on various networks to "speak" to one another. On January 1, 1983, ARPANET and the Defense Data Network switched to the TCP/IP standard, resulting in the birth of the Internet. A universal language could now connect all networks.
Q7) Explain SMTP?
A7) Simple Mail Transfer Protocol (SMTP)
E-mail system is implemented with the help of Message Transfer Agents (MTA). There are normally two MTAs in each mailing system. One for sending e-mails and another for receiving e-mails. The formal protocol that defines the MTA client and server in the internet is called Simple Mail Transfer Protocol (SMTP).
Fig shows the range of SMTP protocol.
By referring the above diagram, we can say that SMTP is used two times. That is between the sender and sender’s mail server and between the sender’s mail server and receiver’s mail server. Another protocol is used between the receiver’s mail server and receiver.
SMTP simply defines how commands and responses must be sent back and forth.
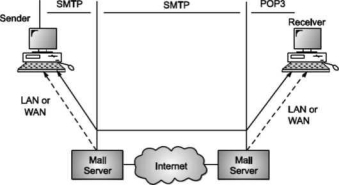
SMTP is a simple ASCII protocol
It establishes a TCP connection between a sender and port number 25 of the receiver. No checksums are generally required because TCP provides a reliable byte stream. After exchanging all the e-mail, the connection is released.
Commands and Responses
SMTP uses commands and responses to transfer messages between an MTA client and MTA server.
Each command or reply is terminated by a two-character (carriage return and line feed) end-of-line token.

Fig. Commands and Responses
Commands
Client sends commands to the server. SMTP defines 14 commands. Out of that first 5 commands are mandatory; every implementation must support these commands.
The next three commands are often used and are highly recommended. Last six commands are hardly used.
Q8) Explain POP?
A8) POP
Post Office Protocol, version 3 (POP3) is simple and feature-limited. The POP3 client software is installed on the receiving machine; the POP3 server software is installed on the mail server.
If the user wants to download e-mails from the mailbox on the mail server, mail access begins with the client. On TCP port 110, the client opens a connection to the server. In order to reach the mailbox, it then sends the user name and password. The user will then, one by one, list and retrieve the mail messages.
There are two modes for POP3: the delete mode and the hold mode. In the delete mode, after each retrieval, the mail is removed from the mailbox. In keep mode, after retrieval, the mail stays in the mailbox. When the user is operating on their permanent device, the delete mode is usually used and after reading or replying, the received mail can be saved and sorted. Normally, the keep mode is used when the user accesses her mail away from her main computer (e.g., a laptop). For later retrieval and organisation, the mail is read but kept in the system.
In some aspects, POP3 is deficient. It does not allow the user to arrange his mail on the server; it is impossible for the user to have multiple folders on the server. (The user will build directories on their own computer, of course.)
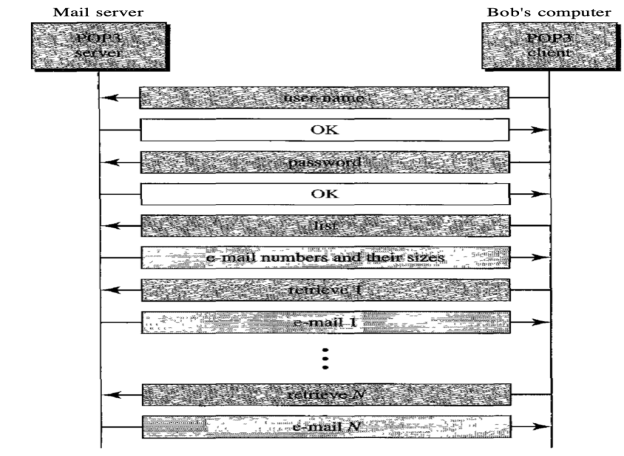
Fig: Command and response of POP3
Advantages of POP3
- As they are already saved on our PC, it provides easy and simple access to the emails.
- The size of the email that we receive or send has no limit.
- When all emails are saved on the local computer, it takes less server storage space.
- The maximum size of a mailbox is available, but the size of the hard disc is limited.
- It is a straightforward protocol, so it is one of the most common protocols used today.
- Configuring and using it is simple.
Disadvantages of POP3
- By default, if the emails are downloaded from the server, all the emails are deleted from the server. Mails will also not be accessed from other computers unless they are programmed to leave a mail copy on the server.
- It can be tough to move the mail folder from the local computer to another machine.
- As all of the attachments are placed on your local computer, if the virus scanner does not scan them, there is a high chance of a virus attack. Virus attacks can destroy your machine.
- There could also be corruption in the email folder that is downloaded from the mail server.
- Mails are saved on a local server, so the email folder can be accessed by someone who is sitting on your machine.
Q9) What is E-mail?
A9) E-mail is defined as the transmission of messages on the Internet. It is one of the most commonly used features over communications networks that may contain text, files, images, or other attachments. Generally, it is information that is stored on a computer sent through a network to a specified individual or group of individuals.
Email messages are conveyed through email servers; it uses multiple protocols within the TCP/IP suite. For example, SMTP is a protocol, stands for simple mail transfer protocol and used to send messages whereas other protocols IMAP or POP are used to retrieve messages from a mail server. If you want to login to your mail account, you just need to enter a valid email address, password, and the mail servers used to send and receive messages.
Although most of the webmail servers automatically configure your mail account, therefore, you only required to enter your email address and password. However, you may need to manually configure each account if you use an email client like Microsoft Outlook or Apple Mail. In addition, to enter the email address and password, you may also need to enter incoming and outgoing mail servers and the correct port numbers for each one.
Q10) What are the uses of email?
A10) Uses of email
Email can be used in different ways: it can be used to communicate either within an organization or personally, including between two people or a large group of people. Most people get benefit from communicating by email with colleagues or friends or individuals or small groups. It allows you to communicate with others around the world and send and receive images, documents, links, and other attachments. Additionally, it offers benefit users to communicate with the flexibility on their own schedule.
There is another benefit of using email; if you use it to communicate between two people or small groups that will beneficial to remind participants of approaching due dates and time-sensitive activities and send professional follow-up emails after appointments. Users can also use the email to quickly remind all upcoming events or inform the group of a time change. Furthermore, it can be used by companies or organizations to convey information to large numbers of employees or customers. Mainly, email is used for newsletters, where mailing list subscribers are sent email marketing campaigns directly and promoted content from a company.
Email can also be used to move a latent sale into a completed purchase or turn leads into paying customers. For example, a company may create an email that is used to send emails automatically to online customers who contain products in their shopping cart. This email can help to remind consumers that they have items in their cart and stimulate them to purchase those items before the items run out of stock. Also, emails are used to get reviews by customers after making a purchase. They can survey by including a question to review the quality of service.
Q11) Write advantages of Email?
A11) Advantages of Email
There are many advantages of email, which are as follows:
● Cost-effective: Email is a very cost-effective service to communicate with others as there are several email services available to individuals and organizations for free of cost. Once a user is online, it does not include any additional charge for the services.
● Email offers users the benefit of accessing email from anywhere at any time if they have an Internet connection.
● Email offers you an incurable communication process, which enables you to send a response at a convenient time. Also, it offers users a better option to communicate easily regardless of different schedules users.
● Speed and simplicity: Email can be composed very easily with the correct information and contacts. Also, minimum lag time, it can be exchanged quickly.
● Mass sending: You can send a message easily to large numbers of people through email.
● Email exchanges can be saved for future retrieval, which allows users to keep important conversations or confirmations in their records and can be searched and retrieved when they needed quickly.
● Email provides a simple user interface and enables users to categorize and filter their messages. This can help you recognize unwanted emails like junk and spam mail. Also, users can find specific messages easily when they are needed.
● As compared to traditional posts, emails are delivered extremely fast.
● Email is beneficial for the planet, as it is paperless. It reduces the cost of paper and helps to save the environment by reducing paper usage.
● It also offers a benefit to attaching the original message at the time you reply to an email. This is beneficial when you get hundreds of emails a day, and the recipient knows what you are talking about.
● Furthermore, emails are beneficial for advertising products. As email is a form of communication, organizations or companies can interact with a lot of people and inform them in a short time.
Q12) Explain the steps to compose and send e-mail?
A12) To write and send e-mail with data and messages, do the following:
Step 1: In order to create a new e-mail and send data through it a user has to click on “Compose” option available at the top of inbox option as shown in below picture.
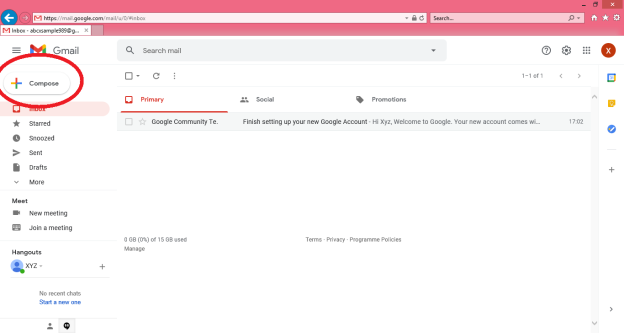
Step 2: A “New Message” box will appear as you can see in the below picture asking you to enter details in order to create a new e-mail message.
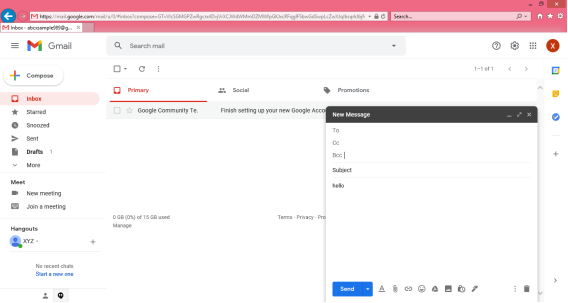
Note: New messages in an e-mail are stored in Inbox folder of the e-mail and all saved messages appears in draft folder of your e-mail.
Here, In the New Message box, you will see the following things:
To: Mail ID of the recipient
Cc: To send a Carbon copy i.e., send this mail to several people at the same time and also show everyone the email id’s of all the other people who have received it.
Bcc: To send a Blind Carbon copy i.e., send this mail to several people at the same time but without showing the person in Bcc list about who else has received this mail.
Subject: Is the main part which will be shown first to the person receiving the mail. It should be short and relevant to your mail and must tell the reason for your e-mail.
Body: The body of the e-mail is the blank white part where the user can type his message and can even customise it. In above picture we have written “Hello” to show the body of this e-mail.
Insert Bar: This bar is available at the bottom of the e-mail compose window. It includes various options to insert document, links, emoji’s, Google drive files, images, signature, delete the email etc.

User simply has to click on one of these options to insert an object and a new window will appear that will ask you about the file location which you want to insert into this mail. After locating the file just click on insert and the file will start uploading to this e-mail, once it is done it can be seen in the e-mail window. You can also open or remove the file from the e-mail using the options available alongside your uploaded file.
Delete button: There is a dustbin icon at the end of this email which lets a user to delete or discard there typed mail. Once discarded the mail cannot be retrieved.
Note: The close option is also available at the top of the window but that option will not delete the e-mail you have typed it simply closes the window and save the typed e-mail in drafts folder for future editing or usage purpose.
Step 3: The send icon is the blue one which is used to send the e-mail once it is composed. All the files inserted along with the text will be sent to the e-mail addresses once the user clicks on this button.
Note: There are various other options available under this Mail compose window such as Print, Schedule send, Minimize and Maximize the window which can be used for better e-mail experience of the user.
Q13) What is SMTP working?
A13) SMTP Working
SMTP works in the following three stages:
a) Connection Establishment
b) Message Transfer
c) Connection Termination
These are explained below:
Connection Establishment
Once the TCP connection is made on port no. 25, SMTP server starts the connection phase. This phase involves following three steps which are explained in the Fig.
The server tells the client that it is ready to receive mail by using the code 220. If the server is not ready, it sends code 421 which tells that service is not available.
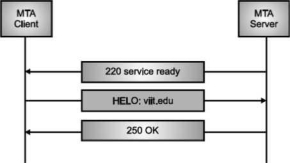
Fig. Connection Establishment
Once the server becomes ready to receive the mails, client sends HELO message to identify itself using the domain name address. This is important step which informs the server of the domain name of the client. Remember that during TCP connection establishment, the sender and receiver know each other through their IP addresses.
Server responds with code 250 which tells that the request command is completed. Message Transfer Once the connection has been established, SMTP server sends messages to SMTP receiver.
The messages are transferred in three stages
- A MAIL command identifies the message originator.
- RCPT command identifies the receiver of the message.
- DATA command transfers the message text.
Connection Closing
After the message is transferred successfully, the client terminates the connection. The connection is terminated in two steps The client sends the quit command.
The server responds with code 221 or some other appropriate code. After the connection termination phase, the TCP connection must be closed.
MIME Short for Multipurpose Internet Mail Extensions, a specification for formatting non-ASCII messages so that they can be sent over the Internet.
Many e-mail clients now support MIME, which enables them to send and receive graphics, audio, and video files via the Internet mail system. In addition, MIME supports messages in character sets other than ASCII.
There are many predefined MIME types, such as GIF graphics files and PostScript files. It is also possible to define your own MIME types.
In addition to e-mail applications, Web browsers also support various MIME types. This enables the browser to display or output files that are not in HTML format.
Q14) Write the disadvantages of email?
A14) Disadvantages of Email
● Impersonal: As compared to other forms of communication, emails are less personal. For example, when you talk to anyone over the phone or meeting face to face is more appropriate for communicating than email.
● Misunderstandings: As email includes only text, and there is no tone of voice or body language to provide context. Therefore, misunderstandings can occur easily with email. If someone sends a joke on email, it can be taken seriously. Also, well-meaning information can be quickly typed as rude or aggressive that can impact wrong. Additionally, if someone types with short abbreviations and descriptions to send content on the email, it can easily be misinterpreted.
● Malicious Use: As email can be sent by anyone if they have an only email address. Sometimes, an unauthorized person can send you mail, which can be harmful in terms of stealing your personal information. Thus, they can also use email to spread gossip or false information.
● Accidents Will Happen: With email, you can make fatal mistakes by clicking the wrong button in a hurry. For instance, instead of sending it to a single person, you can accidentally send sensitive information to a large group of people. Thus, the information can be disclosed, when you have clicked the wrong name in an address list. Therefore, it can be harmful and generate big trouble in the workplace.
● Spam: Although in recent days, the features of email have been improved, there are still big issues with unsolicited advertising arriving and spam through email. It can easily become overwhelming and takes time and energy to control.
● Information Overload: As it is very easy to send email to many people at a time, which can create information overload. In many modern workplaces, it is a major problem where it is required to move a lot of information and impossible to tell if an email is important. And, email needs organization and upkeep. The bad feeling is one of the other problems with email when you returned from vacation and found hundreds of unopened emails in your inbox.
● Viruses: Although there are many ways to travel viruses in the devices, email is one of the common ways to enter viruses and infect devices. Sometimes when you get a mail, it might be the virus come with an attached document. And, the virus can infect the system when you click on the email and open the attached link. Furthermore, an anonymous person or a trusted friend or contact can send infected emails.
● Pressure to Respond: If you get emails and you do not answer them, the sender can get annoyed and think you are ignoring them. Thus, this can be a reason to make pressure on your put to keep opening emails and then respond in some way.
● Time Consuming: When you get an email and read, write, and respond to emails that can take up vast amounts of time and energy. Many modern workers spend their most time with emails, which may be caused to take more time to complete work.
● Overlong Messages: Generally, email is a source of communication with the intention of brief messages. There are some people who write overlong messages that can take much time than required.
● Insecure: There are many hackers available that want to gain your important information, so email is a common source to seek sensitive data, such as political, financial, documents, or personal messages. In recent times, there have various high-profile cases occurred that shown how email is insecure about information theft.
Q15) Explain IMAP?
A15) Internet Message Access Protocol (IMAP) is an acronym for Internet Message Access Protocol. It's an application layer protocol that allows you to receive emails from a mail server. POP3 is one of the most widely used protocols for retrieving emails.
The client/server model is also used. On the one hand, we have an IMAP client, which is a computer programme. On the other hand, we have an IMAP server, which is likewise a computer-based operation. The two computers are linked through a network.
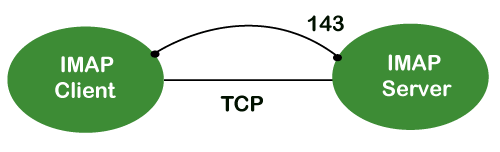
Because the IMAP protocol is based on the TCP/IP transport layer, it makes use of the protocol's dependability implicitly. The IMAP server listens to port 143 by default whenever a TCP connection is established between the IMAP client and the IMAP server, although this port number can be altered.
IMAP is configured to use two ports by default:
● Port 143: It is a non-encrypted IMAP port.
● Port 993: This port is used when IMAP client wants to connect through IMAP securely.
IMAP History and Standards
IMAP version 2 was the first version of IMAP to be formally documented as an internet standard in RFC 1064, which was published in July 1988. It was modified in August 1990, with the same version, in RFC 1176. As a result, they created IMAP3, a new version 3 document. In February 1991, the RFC 1203 was published. However, because IMAP3 was never embraced by the market, users continued to use IMAP2. IMAPb is, a protocol extension, was later built to add support for Multipurpose Internet Mail Extensions (MIME) to IMAP. Because of MIME's utility, this was a significant advance.
IMAPb is was never published as an RFC, despite this. This could be due to issues with the IMAP3 protocol. IMAP version 4, or IMAP4, was released in two RFCs in December 1994: RFC 1730, which described the basic protocol, and RFC 1731, which described the authentication mechanism for IMAP 4. IMAP 4 is the most recent and extensively used version of IMAP. It is still being developed, and the most recent version is known as IMAP4rev1 and is defined in RFC 2060. RFC 3501 is the most recent revision.
IMAP Features
IMAP was created for a specific purpose: to give users more flexibility in how they access their inbox. It can function in any of the three modes: online, offline, or disconnected. The offline and disconnected modes are of particular importance to most protocol users.
An IMAP protocol has the following characteristics:
● Access and retrieve mail from a distant server: The user can access and retrieve mail from a remote server while keeping the messages there.
● Set message flags: The user can keep track of which messages he has already viewed by setting message flags.
● Manage multiple mailboxes: The user has the ability to manage several mailboxes and transfer messages between them. For those working on numerous projects, the user can categorise them into various categories.
● Determine information prior to downloading: Before downloading the mail from the mail server, it decides whether to retrieve or not.
● Downloads a portion of a message: It allows you to download a component of a message, such as a single body part from the mime-multi part. This is useful when a message's short-text element contains huge multimedia files.
● Search: Users can conduct a search for the contents of emails.
● Check email-header: Examine the email header: Before downloading, users can check the email header.
Q16) Write the advantages of IMAP?
A16) Advantages
The following are some of the benefits of IMAP:
● It enables us to compose email messages from any location and on as many different devices as we like.
● Only when we click on a message can it be downloaded. As a result, we don't have to wait for the server to download all of our new messages before we can view them.
● With IMAP, attachments are not immediately downloaded. As a result, you can check your messages much faster and have more control over which attachments are accessed.
● IMAP, like the Post Office Protocol, can be utilised offline (POP).
● It allows you to delete messages, search for keywords in the body of emails, create and manage multiple mailboxes or folders, and display the headers of emails for quick visual searches.
Q17) Explain different web browsers?
A17) Web browsers are programmes that are installed on your computer. A web browser, such as Netscape Navigator, Microsoft Internet Explorer, or Mozilla Firefox, is required to access the Internet.
Currently, you must navigate through our website tutorialspoint.com using any type of Web browser. Web browsing or web surfing is the process of navigating through pages of information on the Internet.
There are four major web browsers: Internet Explorer, Firefox, Netscape, and Safari, but there are several others. Complete Browser Statistics may be of interest to you. Now we'll take a closer look at these browsers.
We should aim to make a site compatible with as many browsers as feasible when developing it. Especially sites should be adaptable to main browsers like Explorer, Firefox, Chrome, Netscape, Opera, and Safari.
Internet Explorer

Microsoft's Internet Explorer (IE) is a software product. This is the most widely used web browser on the planet. This was debuted in 1995 in conjunction with the release of Windows 95, and it surpassed Netscape in popularity in 1998.
Internet Explorer (also known as IE or MSIE) is a free web browser that allows users to view web pages on the internet. It can also be used to access online banking, internet marketing, listen to and watch streaming videos, and many other things. Microsoft first released it in 1995. It was created in response to Netscape Navigator, the first geographical browser.
For many years, from 1999 to 2012, Microsoft Internet Explorer was the most used web browser, surpassing Netscape Navigator. Network file sharing, multiple internet connections, active Scripting, and security settings are all included.
Google Chrome

Google created this web browser, and the beta version was published on September 2, 2008 for Microsoft Windows. Chrome is now one of the most popular web browsers, with a global market share of more than 50%.
Google Chrome is the most popular open-source internet browser for accessing information on the World Wide Web. It was released on December 11, 2008, by Google for Windows, Linux, Mac OS X, Android, and iOS users. It provides Web security through a sandboxing-based technique. It also adheres to web standards such as HTML5 and CSS (cascading style sheet).
Google Chrome was the first online browser to integrate the search box and address bar, a feature that was quickly replicated by other competitors. Google launched the Chrome Web Store in 2010, allowing users to purchase and install Web-based applications.
Mozilla Firefox

Mozilla has created a new browser called Firefox. It was first introduced in 2004 and has since evolved to become the Internet's second most used browser.
Mozilla Firefox is an open-source web browser that allows users to access information on the Internet. Firefox, the popular Web browser, has a simpler user interface and better download speeds than Internet Explorer. To translate web pages, it employs the Gecko layout engine, which follows current and predicted web standards.
Firefox was popular as a replacement for Internet Explorer 6.0 because it protected users from spyware and harmful websites. After Google Chrome, Apple Safari, and UC Browser, it was the fourth most popular web browser in 2017.
Safari

Safari is an Apple Inc. Web browser that comes standard with Mac OS X. It was originally made available to the public as a public beta in January 2003. Safari offers excellent compatibility for modern technologies like as XHTML and CSS2.
Safari is a web browser for the iPhone, iPad, and iPod Touch, as well as the Macintosh and Windows operating systems. Apple, Inc. Released it on June 30, 2003. It is the primary browser for Apple's operating systems, such as OS X for MacBook and Mac computers and iOS for iPad and iPhone smartphones and tablets. After Microsoft Internet Explorer, Mozilla Firefox, and Google Chrome, it is the fourth most used browser. It makes use of the WebKit engine, which handles font rendering, graphics display, page layout, and JavaScript execution.
Opera

Opera is a full-featured browser that is smaller and faster than most other browsers. With a keyboard interface, numerous windows, zoom functions, and more, it's quick and easy to use. There are Java and non-Java versions available. Ideal for novices to the Internet, schoolchildren, the handicapped, and as a CD-Rom and kiosk front-end.
Konqueror

Konqueror is an HTML 4.01 compliant web browser that also supports Java applets, JavaScript, CSS 1, CSS 2.1, and Netscape plugins. This programme functions as a file manager and offers basic file management on local UNIX filesystems, ranging from simple cut/copy/paste operations to advanced remote and local network file browsing.
Lynx

Lynx is a full-featured Web browser for Unix, VMS, and other platforms with cursor-addressable character-cell terminals or emulators.
Q18) Define read command?
A18) The read command in Linux is used to read a line's contents into a variable. For Linux systems, this is a built-in command. As a result, we won't need to install any more software. When writing a bash script, it's a simple tool to utilise to get user input. It's a useful tool, just like the echo command and the positional parameter. It's used to separate the words associated with the shell variable. It is mostly used to receive user input, but it can also be used to implement functions while receiving input.
Syntax:
The read command has the following basic syntax:
Read [options] [name...]
How to use the read command?
With and without arguments, the read command can be used. Let's have a look at how the read command might be used in different situations:
● Default Behaviour
If we run the read command without any arguments, it will accept a line as user input and save it in the 'REPLY' built-in variable. Run the command as follows:
Read
The command above will prompt the user for input. To save the user input, type it in and hit the ENTER key. Execute the command as follows to see the entered content:
Echo $REPLY
The above command will display the 'REPLY' variable's stored input.
● Specify the variables to store the values
We can define the variables that will be used to store the data. If the number of provided variables is less than the number of entered words, all leftover words will be stored in the last variable by default. Consider the command below:
Read var1 var2 var3
● read -p
For the prompt text, the '-p' option is used. It reads the data as well as some hints. This tip text guides us through the process of typing text, such as what to type. Consider the command below:
Read -p " Enter your name: "
The command above will prompt you for your name; type it in. The name will be saved in the variable 'REPLY.' Execute the following command to see the variable value:
Echo " My name is $REPLY"
● read -n
The '-n' option shortens the character length in the entered text. It will not allow you to type more than the number of characters specified. It will automatically cease reading after the maximum number of characters has been reached. Execute the following command to limit the number of characters to six:
Read -n 6 -p " Enter 6 characters only: "
We can't enter more than 6 characters with the instruction above.
● read -s
For security reasons, the '-s' option is utilised. It is used to read sensitive information. The entered text will not appear in the terminal if this option is selected. With this option, we can use additional options. In this option, the characters are read aloud. Its primary function is to read passwords from the keyboard. Consider the command below:
Read -s -p "Enter password: "
The above command will demand for a password, but the password will not be displayed on the terminal when we type it.
Q19) Explain echo statement?
A19) In Linux, the echo command is used to display a line of text/string that has been supplied as an argument. This is a built-in command that outputs status text to the screen or a file. It's typically used in shell scripts and batch files.
Syntax:
Echo [option] [string]
Displaying a text/string:
Syntax:
Echo [string]
Example:
Echo -e "World \bis \bBeautiful"
Output
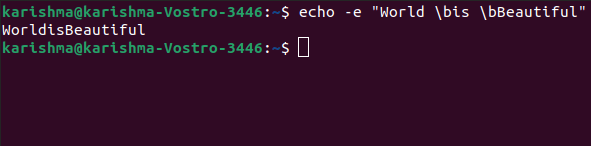
Implementations of the echo command
SymbOS, KolibriOS, HP MPE/iX, ReactOS, Microsoft Windows, IBM OS/2, Digital Research FlexOS, Acorn Computers Panos, Microwave OS-9, Zilog Z80-RIO, MetaComCo TRIPOS, TSC FLEX, Multics, Unix-like, and Unix operating systems all provide the echo command.
Several shells, including Csh-like shells (such as zsh or Bash), Bourne-like shells, COMMAND.COM, and cmd.exe, use the echo command as a built-in command.
The command also exists inside the EFI shell.
Echo Command Options
The echo command has a number of different options. The following alternatives are listed and explained:
1. b: Use this option to remove all spaces from the text/string.
Example
Echo -e "Flowers \bare \bBeautiful"
2.\c: This option is used in conjunction with the '-e' backspace interpreter to suppress the trailing new line and continue without emitting any new lines.
Example
Echo -e "World \cis Beautiful"
3. \n: This option is used to generate a new line, which will be formed from the location where it is used.
Example:
Echo -e "World \nis \nBeautiful"
4. Echo *: This option prints every folder or file in the system. It's the same as the Linux ls command.
Example:
Echo *
5. -n: This option is used to skip echoing new lines at the end.
Example:
Echo -n "World is Beautiful"
6. Print "Hello All!" on the terminal: Use the following command to print the text "Hello All!" on the terminal:
Example:
$ echo "Hello All!"
7. Print specified file types: For example, if we want to print every '.c' file, we can use the command below:
Example:
$ echo *.txt
Unit - 2
Shell Programming and Internet
Q1) Describe script basics?
A1) Shell Scripting
Shell Scripting is a free and open-source programming software that runs on the Unix/Linux shell. Shell Scripting is a program that allows you to write a series of commands to be executed by the shell. It can condense long and repetitive command sequences into a single, simple script that can be saved and executed at any time, reducing programming time.
Shells such as bash and korn in Linux support programming constructs that can be stored as scripts. Many Linux commands are scripted as a result of these scripts being shell commands.
To understand how their servers and applications are started, updated, maintained, or disabled, and to understand how a user interface is designed, a system administrator should have a basic understanding of scripting.
Shells are usually interactive, which means they accept user commands as input and execute them. However, there are times when we need to run a series of commands on a regular basis, and we must type all commands into the terminal each time.
We can write these commands in a file and execute them in shell to avoid this repetitive work because shell can take commands from files as input. Shell Scripts or Shell Programs are the names given to these files. The batch file in MS-DOS is identical to shell scripts. Each shell script has a.sh file extension, such as myscript.sh.
Shell scripts use the same syntax as other programming languages. It will be very simple to get started if you have previous experience with any programming language such as Python, C/C++, or others.
The following elements make up a shell script:
● Shell Keywords – if, else, break etc.
● Shell commands – cd, ls, echo, pwd, touch etc.
● Functions
● Control flow – if..then..else, case and shell loops etc.
Q2) How to create a shell script and generate output?
A2) Text editors are used to build Shell Scripts. Open a text editor program on your Linux system, create a new file, and begin typing a shell script or shell programming. Next, grant the shell permission to execute your shell script, and save your script anywhere the shell can find it.
Let's take a look at how to make a Shell Script:
● Using a vi editor, create a file (or any other editor). .sh is the extension for a script file.
● #! /bin/sh is a good way to start the script.
● Make a program.
● Filename.sh is the name of the script file.
● Type bash filename.sh to run the script.
"#!" is a shebang operator that sends the script to the interpreter's position. The script is guided to the bourne-shell if we use "#! /bin/sh."
Let's make a quick script -
#!/bin/bash
# My first script
Echo "Hello World!"
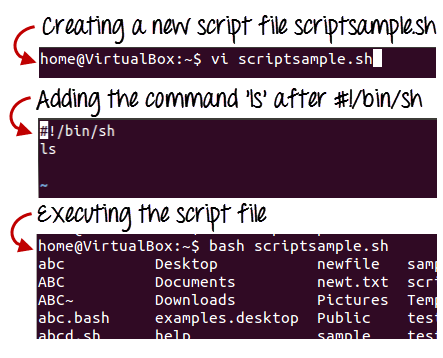
Fig 1: Steps to create shell scripting in linux
Generating output:
The read command is used in the following script to take input from the keyboard, allocate it to the variable Individual, and finally print it on STDOUT.
#!/bin/sh
# Author : Zara Ali
# Copyright (c) Tutorialspoint.com
# Script follows here:
Echo "What is your name?"
Read PERSON
Echo "Hello, $PERSON"
Output:
Here's an example of the script in action:
$./test.sh
What is your name?
Zara Ali
Hello, Zara Ali
$
Example:
The wc command in Bash is used to count a file's total number of lines, sentences, and characters. To generate the output, this command uses the options -c, -w, and -l, as well as the argument filename. To test the next script, create a text file called fruits.txt with the following details. Text file
Fruits.txt
Mango
Orange
Banana
Grape
Guava
Apple
To count and store the total number of words in the fruits.txt file into a variable, $count words, and print the value using the echo command, run the following commands.
$ count_words=wc -w fruits.txt
$ echo "Total words in fruits.txt is $count_words"
Output:

Q3) Explain conditional statements with examples?
A3) Normally, the shell runs the commands in a script in the order they are written in the text, one after the other.
Frequently, though, you will want to alter the way commands are executed.
Depending on the circumstances, you may want to run one command over and over, or you may want to run a command several times.
The shell provides a number of control mechanisms that can be used to change the order in which commands are executed.
There are two different types of selection mechanisms that allow you to choose between different commands:
● if/then/elif/else/fi
● case
The if statement
The if statement allows you to decide whether to run a command (or a group of commands) based on a condition.
The most basic version of this structure is as follows:
If conditional expression
Then
Command(s)
Fi
When the shell comes across a structure like this, it tests to see if the conditional expression is valid first.
If this is the case, the shell executes any commands found between the then and the fi (which is just if spelled backwards).
The shell skips the commands between then and fi if the conditional expression is false.
Example:
Here's a shell script that makes use of easy if statements:
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!
Fi
The test commands
In this conditional expression, we used the test instruction.
The phrase is:
Test $1 = Fri
The test command tests to see if the parameter $1 contains Fri; if it does, the condition is valid, and the message is printed.
The test command may run a number of tests; see the documentation for more details.
Using elif and else
Combining the if statement with the elif (“then if”) and else statements allows one to create even more complex selection structures.
Here's an easy example.
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!"
Elif test $1 = Sat 11 test $1 = Sun
Then
Echo "You should not be here working."
Echo "Log off and go home."
Else
Echo "It is not yet the weekend."
Echo "Get to work!"
Fi
The case Statement
On certain UNIX systems, the shell offers another selection structure that could be faster than the if argument.
This is the case statement, which takes the following form:
Case word in
Patternl) command(s) ;;
Pattern2) command(s) ;;
...
PatternN) command(s) ;;
Esac
The case statement compares word to pattern1, and if the two are equal, the shell executes the command(s) on the first line.
Otherwise, the shell goes through the remaining patterns one by one until it finds one that fits the expression, at which point it executes the command(s) on that line.
The default value is *.
Example:
Here's an example of a basic shell script that employs case statements:
#!/bin/sh
Set ‘date‘
Case $1 in
Fri) echo "Thank goodness it’s Friday!";;
Sat | Sun) echo "You should not be here working"; echo "Log off and go home!";;
*) echo "It is not yet the weekend."; echo "Get to work!";
Esac
Q4) Explain looping statements?
A4) for Loops
We sometimes want to repeat a command (or a set of commands).
Iteration, repetition, or looping are terms used to describe this method.
The for loop is the most common shell repetition structure, and it has the following general form:
For variable in list
Do
Command(s)
Done
Example:
Here's an easy example:
#!/bin/sh
For host in $*
Do
Ping $host
Done
While Loops
The while loop is described as follows:
While condition
Do
Command(s)
Done
The commands between the do and the done are executed as long as the condition is true.
Example:
#!/bin/sh
# Print a message ten times
Count=10
While test $count -gt 0
Do
Echo $*
Count=‘expr $count - 1
Done
Until Loops
The until loop is another form of iteration structure.
It takes the following general form:
Until condition
Do
Command
Done
Unless the condition is valid, this loop continues to execute the command(s) between do and done.
Instead of using while loops, we can rewrite the previous script using an until loop:
#!/bin/sh
# Print a message ten times
Count=10
Until test $count -eq 0
Do
Echo $*
Count=‘expr $count
Done
Conditional Execution
The Exit Code of the first command is used in the following two examples to conditionally execute the second command. The logical AND operator, &&, only runs the second command if the first one was good.
$ gcc myprog.c && a.out
If the first command fails, the logical OR operator || executes the second command.
$ gcc myprog.c || echo compilation failed
Q5) What do you mean by continue and break statement?
A5) Continue statement
The continue statement is identical to the break instruction, except that it only exits the current loop iteration, not the entire loop.
If an error occurs, but you still want to attempt to perform the next iteration of the loop, use this expression.
Syntax:
Continue
The continue instruction, like the break statement, can be given an integer argument to skip commands from nested loops.
Continue n
The nth enclosing loop to proceed from is defined by n.
Example:
The continue statement is used in the following loop, which returns from the continue statement and begins processing the next statement.
#!/bin/sh
NUMS="1 2 3 4 5 6 7"
For NUM in $NUMS
Do
Q=expr $NUM % 2
If [ $Q -eq 0 ]
Then
Echo "Number is an even number!!"
Continue
Fi
Echo "Found odd number"
Done
When you run it, you'll get the following result:
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Break statement
Since all of the lines of code up to the break statement have been executed, the break statement is used to end the loop's execution. It then descends to the code following the loop's conclusion.
Syntax:
To get out of a loop, use the break statement below.
Break
This format can also be used to exit a nested loop with the break command -
Break n
The nth enclosing loop to exit from is defined by n.
Example:
Here's a simple example that demonstrates how the loop ends when an equals 5.
#!/bin/sh
a=0
While [ $a -lt 10 ]
Do
Echo $a
If [ $a -eq 5 ]
Then
Break
Fi
a=expr $a + 1
Done
When you run it, you'll get the following result:
0
1
2
3
4
5
Here's a simple nested for loop illustration. If var1 equals 2 and var2 equals 0, this script exits all loops.
#!/bin/sh
For var1 in 1 2 3
Do
For var2 in 0 5
Do
If [ $var1 -eq 2 -a $var2 -eq 0 ]
Then
Break 2
Else
Echo "$var1 $var2"
Fi
Done
Done
When you run it, you'll get the following result. You have a break command with the argument 2 in the inner loop. This means that if a condition is met, you can exit the outer loop and eventually the inner loop as well.
1 0
1 5
Q6) Write history of the Internet?
A6) It is an interconnected computer network system that spans the globe. It makes use of the TCP/IP protocol, which is widely used on the internet. A unique IP address is assigned to every machine on the Internet. An IP Address (for example, 110.22.33.114) is a series of integers that identify the location of a computer.
A DNS (Domain Name Server) computer is used to give an IP Address a name so that a user can find a computer by name.
ARPANET was expanded to connect the Department of Defense with US colleges conducting defense-related research. It included most of the country's major universities. When University College London (UK) and Royal Radar Network (Norway) joined to the ARPANET and formed a network of networks, the concept of networking received a boost.
Stanford University's Vinton Cerf, Yogen Dalal, and Carl Sunshine invented the name "internet" to characterize this network of networks. They also collaborated on protocols to make information sharing via the Internet easier. The Transmission Control Protocol (TCP) is still the networking's backbone.
In the 1960s, the Internet was created as a means for government researchers to communicate information. In the 1960s, computers were enormous and stationary, and in order to access information stored on them, one had to either travel to the computer's location or have magnetic computer tapes transported through the mail system.
The escalation of the Cold War was another factor in the development of the Internet. The launch of the Sputnik satellite by the Soviet Union prompted the United States Defense Department to examine how information may be distributed even after a nuclear attack. This eventually led to the creation of the ARPANET (Advanced Research Projects Agency Network), which eventually evolved into the Internet we know today. ARPANET was a huge success, but it was only open to a few academic and research institutions with Defense Department contracts. As a result, new networks were formed to facilitate information sharing.
The Internet celebrates its formal birthday on January 1, 1983. Prior to this, there was no standard means for computer networks to communicate with one another. Transfer Control Protocol/Internetwork Protocol (TCP/IP) was created as a new communication protocol.
This allowed various types of computers on various networks to "speak" to one another. On January 1, 1983, ARPANET and the Defense Data Network switched to the TCP/IP standard, resulting in the birth of the Internet. A universal language could now connect all networks.
Q7) Explain SMTP?
A7) Simple Mail Transfer Protocol (SMTP)
E-mail system is implemented with the help of Message Transfer Agents (MTA). There are normally two MTAs in each mailing system. One for sending e-mails and another for receiving e-mails. The formal protocol that defines the MTA client and server in the internet is called Simple Mail Transfer Protocol (SMTP).
Fig shows the range of SMTP protocol.
By referring the above diagram, we can say that SMTP is used two times. That is between the sender and sender’s mail server and between the sender’s mail server and receiver’s mail server. Another protocol is used between the receiver’s mail server and receiver.
SMTP simply defines how commands and responses must be sent back and forth.
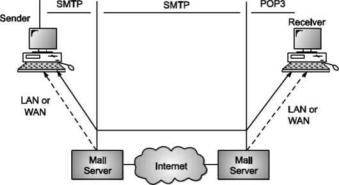
SMTP is a simple ASCII protocol
It establishes a TCP connection between a sender and port number 25 of the receiver. No checksums are generally required because TCP provides a reliable byte stream. After exchanging all the e-mail, the connection is released.
Commands and Responses
SMTP uses commands and responses to transfer messages between an MTA client and MTA server.
Each command or reply is terminated by a two-character (carriage return and line feed) end-of-line token.

Fig. Commands and Responses
Commands
Client sends commands to the server. SMTP defines 14 commands. Out of that first 5 commands are mandatory; every implementation must support these commands.
The next three commands are often used and are highly recommended. Last six commands are hardly used.
Q8) Explain POP?
A8) POP
Post Office Protocol, version 3 (POP3) is simple and feature-limited. The POP3 client software is installed on the receiving machine; the POP3 server software is installed on the mail server.
If the user wants to download e-mails from the mailbox on the mail server, mail access begins with the client. On TCP port 110, the client opens a connection to the server. In order to reach the mailbox, it then sends the user name and password. The user will then, one by one, list and retrieve the mail messages.
There are two modes for POP3: the delete mode and the hold mode. In the delete mode, after each retrieval, the mail is removed from the mailbox. In keep mode, after retrieval, the mail stays in the mailbox. When the user is operating on their permanent device, the delete mode is usually used and after reading or replying, the received mail can be saved and sorted. Normally, the keep mode is used when the user accesses her mail away from her main computer (e.g., a laptop). For later retrieval and organisation, the mail is read but kept in the system.
In some aspects, POP3 is deficient. It does not allow the user to arrange his mail on the server; it is impossible for the user to have multiple folders on the server. (The user will build directories on their own computer, of course.)
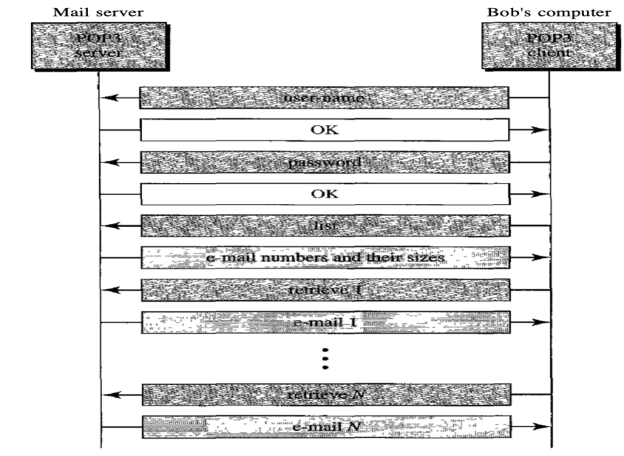
Fig: Command and response of POP3
Advantages of POP3
- As they are already saved on our PC, it provides easy and simple access to the emails.
- The size of the email that we receive or send has no limit.
- When all emails are saved on the local computer, it takes less server storage space.
- The maximum size of a mailbox is available, but the size of the hard disc is limited.
- It is a straightforward protocol, so it is one of the most common protocols used today.
- Configuring and using it is simple.
Disadvantages of POP3
- By default, if the emails are downloaded from the server, all the emails are deleted from the server. Mails will also not be accessed from other computers unless they are programmed to leave a mail copy on the server.
- It can be tough to move the mail folder from the local computer to another machine.
- As all of the attachments are placed on your local computer, if the virus scanner does not scan them, there is a high chance of a virus attack. Virus attacks can destroy your machine.
- There could also be corruption in the email folder that is downloaded from the mail server.
- Mails are saved on a local server, so the email folder can be accessed by someone who is sitting on your machine.
Q9) What is E-mail?
A9) E-mail is defined as the transmission of messages on the Internet. It is one of the most commonly used features over communications networks that may contain text, files, images, or other attachments. Generally, it is information that is stored on a computer sent through a network to a specified individual or group of individuals.
Email messages are conveyed through email servers; it uses multiple protocols within the TCP/IP suite. For example, SMTP is a protocol, stands for simple mail transfer protocol and used to send messages whereas other protocols IMAP or POP are used to retrieve messages from a mail server. If you want to login to your mail account, you just need to enter a valid email address, password, and the mail servers used to send and receive messages.
Although most of the webmail servers automatically configure your mail account, therefore, you only required to enter your email address and password. However, you may need to manually configure each account if you use an email client like Microsoft Outlook or Apple Mail. In addition, to enter the email address and password, you may also need to enter incoming and outgoing mail servers and the correct port numbers for each one.
Q10) What are the uses of email?
A10) Uses of email
Email can be used in different ways: it can be used to communicate either within an organization or personally, including between two people or a large group of people. Most people get benefit from communicating by email with colleagues or friends or individuals or small groups. It allows you to communicate with others around the world and send and receive images, documents, links, and other attachments. Additionally, it offers benefit users to communicate with the flexibility on their own schedule.
There is another benefit of using email; if you use it to communicate between two people or small groups that will beneficial to remind participants of approaching due dates and time-sensitive activities and send professional follow-up emails after appointments. Users can also use the email to quickly remind all upcoming events or inform the group of a time change. Furthermore, it can be used by companies or organizations to convey information to large numbers of employees or customers. Mainly, email is used for newsletters, where mailing list subscribers are sent email marketing campaigns directly and promoted content from a company.
Email can also be used to move a latent sale into a completed purchase or turn leads into paying customers. For example, a company may create an email that is used to send emails automatically to online customers who contain products in their shopping cart. This email can help to remind consumers that they have items in their cart and stimulate them to purchase those items before the items run out of stock. Also, emails are used to get reviews by customers after making a purchase. They can survey by including a question to review the quality of service.
Q11) Write advantages of Email?
A11) Advantages of Email
There are many advantages of email, which are as follows:
● Cost-effective: Email is a very cost-effective service to communicate with others as there are several email services available to individuals and organizations for free of cost. Once a user is online, it does not include any additional charge for the services.
● Email offers users the benefit of accessing email from anywhere at any time if they have an Internet connection.
● Email offers you an incurable communication process, which enables you to send a response at a convenient time. Also, it offers users a better option to communicate easily regardless of different schedules users.
● Speed and simplicity: Email can be composed very easily with the correct information and contacts. Also, minimum lag time, it can be exchanged quickly.
● Mass sending: You can send a message easily to large numbers of people through email.
● Email exchanges can be saved for future retrieval, which allows users to keep important conversations or confirmations in their records and can be searched and retrieved when they needed quickly.
● Email provides a simple user interface and enables users to categorize and filter their messages. This can help you recognize unwanted emails like junk and spam mail. Also, users can find specific messages easily when they are needed.
● As compared to traditional posts, emails are delivered extremely fast.
● Email is beneficial for the planet, as it is paperless. It reduces the cost of paper and helps to save the environment by reducing paper usage.
● It also offers a benefit to attaching the original message at the time you reply to an email. This is beneficial when you get hundreds of emails a day, and the recipient knows what you are talking about.
● Furthermore, emails are beneficial for advertising products. As email is a form of communication, organizations or companies can interact with a lot of people and inform them in a short time.
Q12) Explain the steps to compose and send e-mail?
A12) To write and send e-mail with data and messages, do the following:
Step 1: In order to create a new e-mail and send data through it a user has to click on “Compose” option available at the top of inbox option as shown in below picture.
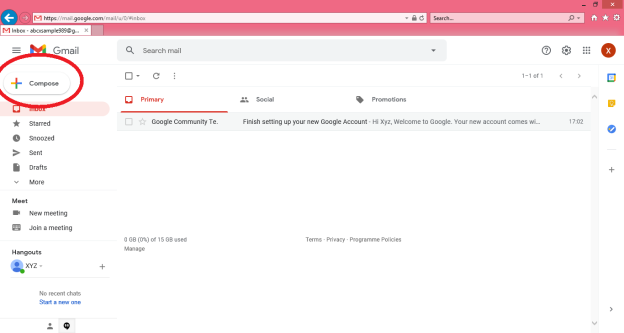
Step 2: A “New Message” box will appear as you can see in the below picture asking you to enter details in order to create a new e-mail message.
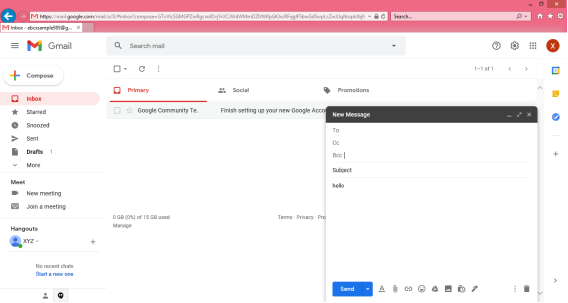
Note: New messages in an e-mail are stored in Inbox folder of the e-mail and all saved messages appears in draft folder of your e-mail.
Here, In the New Message box, you will see the following things:
To: Mail ID of the recipient
Cc: To send a Carbon copy i.e., send this mail to several people at the same time and also show everyone the email id’s of all the other people who have received it.
Bcc: To send a Blind Carbon copy i.e., send this mail to several people at the same time but without showing the person in Bcc list about who else has received this mail.
Subject: Is the main part which will be shown first to the person receiving the mail. It should be short and relevant to your mail and must tell the reason for your e-mail.
Body: The body of the e-mail is the blank white part where the user can type his message and can even customise it. In above picture we have written “Hello” to show the body of this e-mail.
Insert Bar: This bar is available at the bottom of the e-mail compose window. It includes various options to insert document, links, emoji’s, Google drive files, images, signature, delete the email etc.

User simply has to click on one of these options to insert an object and a new window will appear that will ask you about the file location which you want to insert into this mail. After locating the file just click on insert and the file will start uploading to this e-mail, once it is done it can be seen in the e-mail window. You can also open or remove the file from the e-mail using the options available alongside your uploaded file.
Delete button: There is a dustbin icon at the end of this email which lets a user to delete or discard there typed mail. Once discarded the mail cannot be retrieved.
Note: The close option is also available at the top of the window but that option will not delete the e-mail you have typed it simply closes the window and save the typed e-mail in drafts folder for future editing or usage purpose.
Step 3: The send icon is the blue one which is used to send the e-mail once it is composed. All the files inserted along with the text will be sent to the e-mail addresses once the user clicks on this button.
Note: There are various other options available under this Mail compose window such as Print, Schedule send, Minimize and Maximize the window which can be used for better e-mail experience of the user.
Q13) What is SMTP working?
A13) SMTP Working
SMTP works in the following three stages:
a) Connection Establishment
b) Message Transfer
c) Connection Termination
These are explained below:
Connection Establishment
Once the TCP connection is made on port no. 25, SMTP server starts the connection phase. This phase involves following three steps which are explained in the Fig.
The server tells the client that it is ready to receive mail by using the code 220. If the server is not ready, it sends code 421 which tells that service is not available.
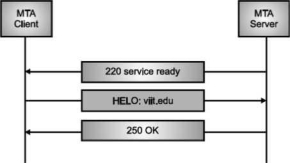
Fig. Connection Establishment
Once the server becomes ready to receive the mails, client sends HELO message to identify itself using the domain name address. This is important step which informs the server of the domain name of the client. Remember that during TCP connection establishment, the sender and receiver know each other through their IP addresses.
Server responds with code 250 which tells that the request command is completed. Message Transfer Once the connection has been established, SMTP server sends messages to SMTP receiver.
The messages are transferred in three stages
- A MAIL command identifies the message originator.
- RCPT command identifies the receiver of the message.
- DATA command transfers the message text.
Connection Closing
After the message is transferred successfully, the client terminates the connection. The connection is terminated in two steps The client sends the quit command.
The server responds with code 221 or some other appropriate code. After the connection termination phase, the TCP connection must be closed.
MIME Short for Multipurpose Internet Mail Extensions, a specification for formatting non-ASCII messages so that they can be sent over the Internet.
Many e-mail clients now support MIME, which enables them to send and receive graphics, audio, and video files via the Internet mail system. In addition, MIME supports messages in character sets other than ASCII.
There are many predefined MIME types, such as GIF graphics files and PostScript files. It is also possible to define your own MIME types.
In addition to e-mail applications, Web browsers also support various MIME types. This enables the browser to display or output files that are not in HTML format.
Q14) Write the disadvantages of email?
A14) Disadvantages of Email
● Impersonal: As compared to other forms of communication, emails are less personal. For example, when you talk to anyone over the phone or meeting face to face is more appropriate for communicating than email.
● Misunderstandings: As email includes only text, and there is no tone of voice or body language to provide context. Therefore, misunderstandings can occur easily with email. If someone sends a joke on email, it can be taken seriously. Also, well-meaning information can be quickly typed as rude or aggressive that can impact wrong. Additionally, if someone types with short abbreviations and descriptions to send content on the email, it can easily be misinterpreted.
● Malicious Use: As email can be sent by anyone if they have an only email address. Sometimes, an unauthorized person can send you mail, which can be harmful in terms of stealing your personal information. Thus, they can also use email to spread gossip or false information.
● Accidents Will Happen: With email, you can make fatal mistakes by clicking the wrong button in a hurry. For instance, instead of sending it to a single person, you can accidentally send sensitive information to a large group of people. Thus, the information can be disclosed, when you have clicked the wrong name in an address list. Therefore, it can be harmful and generate big trouble in the workplace.
● Spam: Although in recent days, the features of email have been improved, there are still big issues with unsolicited advertising arriving and spam through email. It can easily become overwhelming and takes time and energy to control.
● Information Overload: As it is very easy to send email to many people at a time, which can create information overload. In many modern workplaces, it is a major problem where it is required to move a lot of information and impossible to tell if an email is important. And, email needs organization and upkeep. The bad feeling is one of the other problems with email when you returned from vacation and found hundreds of unopened emails in your inbox.
● Viruses: Although there are many ways to travel viruses in the devices, email is one of the common ways to enter viruses and infect devices. Sometimes when you get a mail, it might be the virus come with an attached document. And, the virus can infect the system when you click on the email and open the attached link. Furthermore, an anonymous person or a trusted friend or contact can send infected emails.
● Pressure to Respond: If you get emails and you do not answer them, the sender can get annoyed and think you are ignoring them. Thus, this can be a reason to make pressure on your put to keep opening emails and then respond in some way.
● Time Consuming: When you get an email and read, write, and respond to emails that can take up vast amounts of time and energy. Many modern workers spend their most time with emails, which may be caused to take more time to complete work.
● Overlong Messages: Generally, email is a source of communication with the intention of brief messages. There are some people who write overlong messages that can take much time than required.
● Insecure: There are many hackers available that want to gain your important information, so email is a common source to seek sensitive data, such as political, financial, documents, or personal messages. In recent times, there have various high-profile cases occurred that shown how email is insecure about information theft.
Q15) Explain IMAP?
A15) Internet Message Access Protocol (IMAP) is an acronym for Internet Message Access Protocol. It's an application layer protocol that allows you to receive emails from a mail server. POP3 is one of the most widely used protocols for retrieving emails.
The client/server model is also used. On the one hand, we have an IMAP client, which is a computer programme. On the other hand, we have an IMAP server, which is likewise a computer-based operation. The two computers are linked through a network.
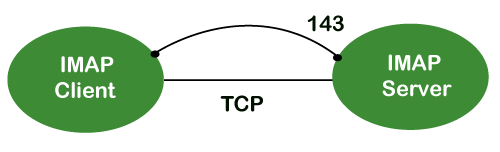
Because the IMAP protocol is based on the TCP/IP transport layer, it makes use of the protocol's dependability implicitly. The IMAP server listens to port 143 by default whenever a TCP connection is established between the IMAP client and the IMAP server, although this port number can be altered.
IMAP is configured to use two ports by default:
● Port 143: It is a non-encrypted IMAP port.
● Port 993: This port is used when IMAP client wants to connect through IMAP securely.
IMAP History and Standards
IMAP version 2 was the first version of IMAP to be formally documented as an internet standard in RFC 1064, which was published in July 1988. It was modified in August 1990, with the same version, in RFC 1176. As a result, they created IMAP3, a new version 3 document. In February 1991, the RFC 1203 was published. However, because IMAP3 was never embraced by the market, users continued to use IMAP2. IMAPb is, a protocol extension, was later built to add support for Multipurpose Internet Mail Extensions (MIME) to IMAP. Because of MIME's utility, this was a significant advance.
IMAPb is was never published as an RFC, despite this. This could be due to issues with the IMAP3 protocol. IMAP version 4, or IMAP4, was released in two RFCs in December 1994: RFC 1730, which described the basic protocol, and RFC 1731, which described the authentication mechanism for IMAP 4. IMAP 4 is the most recent and extensively used version of IMAP. It is still being developed, and the most recent version is known as IMAP4rev1 and is defined in RFC 2060. RFC 3501 is the most recent revision.
IMAP Features
IMAP was created for a specific purpose: to give users more flexibility in how they access their inbox. It can function in any of the three modes: online, offline, or disconnected. The offline and disconnected modes are of particular importance to most protocol users.
An IMAP protocol has the following characteristics:
● Access and retrieve mail from a distant server: The user can access and retrieve mail from a remote server while keeping the messages there.
● Set message flags: The user can keep track of which messages he has already viewed by setting message flags.
● Manage multiple mailboxes: The user has the ability to manage several mailboxes and transfer messages between them. For those working on numerous projects, the user can categorise them into various categories.
● Determine information prior to downloading: Before downloading the mail from the mail server, it decides whether to retrieve or not.
● Downloads a portion of a message: It allows you to download a component of a message, such as a single body part from the mime-multi part. This is useful when a message's short-text element contains huge multimedia files.
● Search: Users can conduct a search for the contents of emails.
● Check email-header: Examine the email header: Before downloading, users can check the email header.
Q16) Write the advantages of IMAP?
A16) Advantages
The following are some of the benefits of IMAP:
● It enables us to compose email messages from any location and on as many different devices as we like.
● Only when we click on a message can it be downloaded. As a result, we don't have to wait for the server to download all of our new messages before we can view them.
● With IMAP, attachments are not immediately downloaded. As a result, you can check your messages much faster and have more control over which attachments are accessed.
● IMAP, like the Post Office Protocol, can be utilised offline (POP).
● It allows you to delete messages, search for keywords in the body of emails, create and manage multiple mailboxes or folders, and display the headers of emails for quick visual searches.
Q17) Explain different web browsers?
A17) Web browsers are programmes that are installed on your computer. A web browser, such as Netscape Navigator, Microsoft Internet Explorer, or Mozilla Firefox, is required to access the Internet.
Currently, you must navigate through our website tutorialspoint.com using any type of Web browser. Web browsing or web surfing is the process of navigating through pages of information on the Internet.
There are four major web browsers: Internet Explorer, Firefox, Netscape, and Safari, but there are several others. Complete Browser Statistics may be of interest to you. Now we'll take a closer look at these browsers.
We should aim to make a site compatible with as many browsers as feasible when developing it. Especially sites should be adaptable to main browsers like Explorer, Firefox, Chrome, Netscape, Opera, and Safari.
Internet Explorer

Microsoft's Internet Explorer (IE) is a software product. This is the most widely used web browser on the planet. This was debuted in 1995 in conjunction with the release of Windows 95, and it surpassed Netscape in popularity in 1998.
Internet Explorer (also known as IE or MSIE) is a free web browser that allows users to view web pages on the internet. It can also be used to access online banking, internet marketing, listen to and watch streaming videos, and many other things. Microsoft first released it in 1995. It was created in response to Netscape Navigator, the first geographical browser.
For many years, from 1999 to 2012, Microsoft Internet Explorer was the most used web browser, surpassing Netscape Navigator. Network file sharing, multiple internet connections, active Scripting, and security settings are all included.
Google Chrome

Google created this web browser, and the beta version was published on September 2, 2008 for Microsoft Windows. Chrome is now one of the most popular web browsers, with a global market share of more than 50%.
Google Chrome is the most popular open-source internet browser for accessing information on the World Wide Web. It was released on December 11, 2008, by Google for Windows, Linux, Mac OS X, Android, and iOS users. It provides Web security through a sandboxing-based technique. It also adheres to web standards such as HTML5 and CSS (cascading style sheet).
Google Chrome was the first online browser to integrate the search box and address bar, a feature that was quickly replicated by other competitors. Google launched the Chrome Web Store in 2010, allowing users to purchase and install Web-based applications.
Mozilla Firefox

Mozilla has created a new browser called Firefox. It was first introduced in 2004 and has since evolved to become the Internet's second most used browser.
Mozilla Firefox is an open-source web browser that allows users to access information on the Internet. Firefox, the popular Web browser, has a simpler user interface and better download speeds than Internet Explorer. To translate web pages, it employs the Gecko layout engine, which follows current and predicted web standards.
Firefox was popular as a replacement for Internet Explorer 6.0 because it protected users from spyware and harmful websites. After Google Chrome, Apple Safari, and UC Browser, it was the fourth most popular web browser in 2017.
Safari

Safari is an Apple Inc. Web browser that comes standard with Mac OS X. It was originally made available to the public as a public beta in January 2003. Safari offers excellent compatibility for modern technologies like as XHTML and CSS2.
Safari is a web browser for the iPhone, iPad, and iPod Touch, as well as the Macintosh and Windows operating systems. Apple, Inc. Released it on June 30, 2003. It is the primary browser for Apple's operating systems, such as OS X for MacBook and Mac computers and iOS for iPad and iPhone smartphones and tablets. After Microsoft Internet Explorer, Mozilla Firefox, and Google Chrome, it is the fourth most used browser. It makes use of the WebKit engine, which handles font rendering, graphics display, page layout, and JavaScript execution.
Opera

Opera is a full-featured browser that is smaller and faster than most other browsers. With a keyboard interface, numerous windows, zoom functions, and more, it's quick and easy to use. There are Java and non-Java versions available. Ideal for novices to the Internet, schoolchildren, the handicapped, and as a CD-Rom and kiosk front-end.
Konqueror

Konqueror is an HTML 4.01 compliant web browser that also supports Java applets, JavaScript, CSS 1, CSS 2.1, and Netscape plugins. This programme functions as a file manager and offers basic file management on local UNIX filesystems, ranging from simple cut/copy/paste operations to advanced remote and local network file browsing.
Lynx

Lynx is a full-featured Web browser for Unix, VMS, and other platforms with cursor-addressable character-cell terminals or emulators.
Q18) Define read command?
A18) The read command in Linux is used to read a line's contents into a variable. For Linux systems, this is a built-in command. As a result, we won't need to install any more software. When writing a bash script, it's a simple tool to utilise to get user input. It's a useful tool, just like the echo command and the positional parameter. It's used to separate the words associated with the shell variable. It is mostly used to receive user input, but it can also be used to implement functions while receiving input.
Syntax:
The read command has the following basic syntax:
Read [options] [name...]
How to use the read command?
With and without arguments, the read command can be used. Let's have a look at how the read command might be used in different situations:
● Default Behaviour
If we run the read command without any arguments, it will accept a line as user input and save it in the 'REPLY' built-in variable. Run the command as follows:
Read
The command above will prompt the user for input. To save the user input, type it in and hit the ENTER key. Execute the command as follows to see the entered content:
Echo $REPLY
The above command will display the 'REPLY' variable's stored input.
● Specify the variables to store the values
We can define the variables that will be used to store the data. If the number of provided variables is less than the number of entered words, all leftover words will be stored in the last variable by default. Consider the command below:
Read var1 var2 var3
● read -p
For the prompt text, the '-p' option is used. It reads the data as well as some hints. This tip text guides us through the process of typing text, such as what to type. Consider the command below:
Read -p " Enter your name: "
The command above will prompt you for your name; type it in. The name will be saved in the variable 'REPLY.' Execute the following command to see the variable value:
Echo " My name is $REPLY"
● read -n
The '-n' option shortens the character length in the entered text. It will not allow you to type more than the number of characters specified. It will automatically cease reading after the maximum number of characters has been reached. Execute the following command to limit the number of characters to six:
Read -n 6 -p " Enter 6 characters only: "
We can't enter more than 6 characters with the instruction above.
● read -s
For security reasons, the '-s' option is utilised. It is used to read sensitive information. The entered text will not appear in the terminal if this option is selected. With this option, we can use additional options. In this option, the characters are read aloud. Its primary function is to read passwords from the keyboard. Consider the command below:
Read -s -p "Enter password: "
The above command will demand for a password, but the password will not be displayed on the terminal when we type it.
Q19) Explain echo statement?
A19) In Linux, the echo command is used to display a line of text/string that has been supplied as an argument. This is a built-in command that outputs status text to the screen or a file. It's typically used in shell scripts and batch files.
Syntax:
Echo [option] [string]
Displaying a text/string:
Syntax:
Echo [string]
Example:
Echo -e "World \bis \bBeautiful"
Output
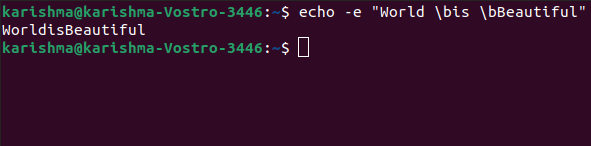
Implementations of the echo command
SymbOS, KolibriOS, HP MPE/iX, ReactOS, Microsoft Windows, IBM OS/2, Digital Research FlexOS, Acorn Computers Panos, Microwave OS-9, Zilog Z80-RIO, MetaComCo TRIPOS, TSC FLEX, Multics, Unix-like, and Unix operating systems all provide the echo command.
Several shells, including Csh-like shells (such as zsh or Bash), Bourne-like shells, COMMAND.COM, and cmd.exe, use the echo command as a built-in command.
The command also exists inside the EFI shell.
Echo Command Options
The echo command has a number of different options. The following alternatives are listed and explained:
1. b: Use this option to remove all spaces from the text/string.
Example
Echo -e "Flowers \bare \bBeautiful"
2.\c: This option is used in conjunction with the '-e' backspace interpreter to suppress the trailing new line and continue without emitting any new lines.
Example
Echo -e "World \cis Beautiful"
3. \n: This option is used to generate a new line, which will be formed from the location where it is used.
Example:
Echo -e "World \nis \nBeautiful"
4. Echo *: This option prints every folder or file in the system. It's the same as the Linux ls command.
Example:
Echo *
5. -n: This option is used to skip echoing new lines at the end.
Example:
Echo -n "World is Beautiful"
6. Print "Hello All!" on the terminal: Use the following command to print the text "Hello All!" on the terminal:
Example:
$ echo "Hello All!"
7. Print specified file types: For example, if we want to print every '.c' file, we can use the command below:
Example:
$ echo *.txt
Unit - 2
Shell Programming and Internet
Q1) Describe script basics?
A1) Shell Scripting
Shell Scripting is a free and open-source programming software that runs on the Unix/Linux shell. Shell Scripting is a program that allows you to write a series of commands to be executed by the shell. It can condense long and repetitive command sequences into a single, simple script that can be saved and executed at any time, reducing programming time.
Shells such as bash and korn in Linux support programming constructs that can be stored as scripts. Many Linux commands are scripted as a result of these scripts being shell commands.
To understand how their servers and applications are started, updated, maintained, or disabled, and to understand how a user interface is designed, a system administrator should have a basic understanding of scripting.
Shells are usually interactive, which means they accept user commands as input and execute them. However, there are times when we need to run a series of commands on a regular basis, and we must type all commands into the terminal each time.
We can write these commands in a file and execute them in shell to avoid this repetitive work because shell can take commands from files as input. Shell Scripts or Shell Programs are the names given to these files. The batch file in MS-DOS is identical to shell scripts. Each shell script has a.sh file extension, such as myscript.sh.
Shell scripts use the same syntax as other programming languages. It will be very simple to get started if you have previous experience with any programming language such as Python, C/C++, or others.
The following elements make up a shell script:
● Shell Keywords – if, else, break etc.
● Shell commands – cd, ls, echo, pwd, touch etc.
● Functions
● Control flow – if..then..else, case and shell loops etc.
Q2) How to create a shell script and generate output?
A2) Text editors are used to build Shell Scripts. Open a text editor program on your Linux system, create a new file, and begin typing a shell script or shell programming. Next, grant the shell permission to execute your shell script, and save your script anywhere the shell can find it.
Let's take a look at how to make a Shell Script:
● Using a vi editor, create a file (or any other editor). .sh is the extension for a script file.
● #! /bin/sh is a good way to start the script.
● Make a program.
● Filename.sh is the name of the script file.
● Type bash filename.sh to run the script.
"#!" is a shebang operator that sends the script to the interpreter's position. The script is guided to the bourne-shell if we use "#! /bin/sh."
Let's make a quick script -
#!/bin/bash
# My first script
Echo "Hello World!"
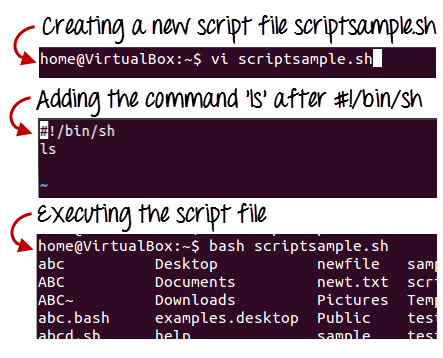
Fig 1: Steps to create shell scripting in linux
Generating output:
The read command is used in the following script to take input from the keyboard, allocate it to the variable Individual, and finally print it on STDOUT.
#!/bin/sh
# Author : Zara Ali
# Copyright (c) Tutorialspoint.com
# Script follows here:
Echo "What is your name?"
Read PERSON
Echo "Hello, $PERSON"
Output:
Here's an example of the script in action:
$./test.sh
What is your name?
Zara Ali
Hello, Zara Ali
$
Example:
The wc command in Bash is used to count a file's total number of lines, sentences, and characters. To generate the output, this command uses the options -c, -w, and -l, as well as the argument filename. To test the next script, create a text file called fruits.txt with the following details. Text file
Fruits.txt
Mango
Orange
Banana
Grape
Guava
Apple
To count and store the total number of words in the fruits.txt file into a variable, $count words, and print the value using the echo command, run the following commands.
$ count_words=wc -w fruits.txt
$ echo "Total words in fruits.txt is $count_words"
Output:

Q3) Explain conditional statements with examples?
A3) Normally, the shell runs the commands in a script in the order they are written in the text, one after the other.
Frequently, though, you will want to alter the way commands are executed.
Depending on the circumstances, you may want to run one command over and over, or you may want to run a command several times.
The shell provides a number of control mechanisms that can be used to change the order in which commands are executed.
There are two different types of selection mechanisms that allow you to choose between different commands:
● if/then/elif/else/fi
● case
The if statement
The if statement allows you to decide whether to run a command (or a group of commands) based on a condition.
The most basic version of this structure is as follows:
If conditional expression
Then
Command(s)
Fi
When the shell comes across a structure like this, it tests to see if the conditional expression is valid first.
If this is the case, the shell executes any commands found between the then and the fi (which is just if spelled backwards).
The shell skips the commands between then and fi if the conditional expression is false.
Example:
Here's a shell script that makes use of easy if statements:
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!
Fi
The test commands
In this conditional expression, we used the test instruction.
The phrase is:
Test $1 = Fri
The test command tests to see if the parameter $1 contains Fri; if it does, the condition is valid, and the message is printed.
The test command may run a number of tests; see the documentation for more details.
Using elif and else
Combining the if statement with the elif (“then if”) and else statements allows one to create even more complex selection structures.
Here's an easy example.
#!/bin/sh
Set ‘date‘
If test $1 = Fri
Then
Echo "Thank goodness it’s Friday!"
Elif test $1 = Sat 11 test $1 = Sun
Then
Echo "You should not be here working."
Echo "Log off and go home."
Else
Echo "It is not yet the weekend."
Echo "Get to work!"
Fi
The case Statement
On certain UNIX systems, the shell offers another selection structure that could be faster than the if argument.
This is the case statement, which takes the following form:
Case word in
Patternl) command(s) ;;
Pattern2) command(s) ;;
...
PatternN) command(s) ;;
Esac
The case statement compares word to pattern1, and if the two are equal, the shell executes the command(s) on the first line.
Otherwise, the shell goes through the remaining patterns one by one until it finds one that fits the expression, at which point it executes the command(s) on that line.
The default value is *.
Example:
Here's an example of a basic shell script that employs case statements:
#!/bin/sh
Set ‘date‘
Case $1 in
Fri) echo "Thank goodness it’s Friday!";;
Sat | Sun) echo "You should not be here working"; echo "Log off and go home!";;
*) echo "It is not yet the weekend."; echo "Get to work!";
Esac
Q4) Explain looping statements?
A4) for Loops
We sometimes want to repeat a command (or a set of commands).
Iteration, repetition, or looping are terms used to describe this method.
The for loop is the most common shell repetition structure, and it has the following general form:
For variable in list
Do
Command(s)
Done
Example:
Here's an easy example:
#!/bin/sh
For host in $*
Do
Ping $host
Done
While Loops
The while loop is described as follows:
While condition
Do
Command(s)
Done
The commands between the do and the done are executed as long as the condition is true.
Example:
#!/bin/sh
# Print a message ten times
Count=10
While test $count -gt 0
Do
Echo $*
Count=‘expr $count - 1
Done
Until Loops
The until loop is another form of iteration structure.
It takes the following general form:
Until condition
Do
Command
Done
Unless the condition is valid, this loop continues to execute the command(s) between do and done.
Instead of using while loops, we can rewrite the previous script using an until loop:
#!/bin/sh
# Print a message ten times
Count=10
Until test $count -eq 0
Do
Echo $*
Count=‘expr $count
Done
Conditional Execution
The Exit Code of the first command is used in the following two examples to conditionally execute the second command. The logical AND operator, &&, only runs the second command if the first one was good.
$ gcc myprog.c && a.out
If the first command fails, the logical OR operator || executes the second command.
$ gcc myprog.c || echo compilation failed
Q5) What do you mean by continue and break statement?
A5) Continue statement
The continue statement is identical to the break instruction, except that it only exits the current loop iteration, not the entire loop.
If an error occurs, but you still want to attempt to perform the next iteration of the loop, use this expression.
Syntax:
Continue
The continue instruction, like the break statement, can be given an integer argument to skip commands from nested loops.
Continue n
The nth enclosing loop to proceed from is defined by n.
Example:
The continue statement is used in the following loop, which returns from the continue statement and begins processing the next statement.
#!/bin/sh
NUMS="1 2 3 4 5 6 7"
For NUM in $NUMS
Do
Q=expr $NUM % 2
If [ $Q -eq 0 ]
Then
Echo "Number is an even number!!"
Continue
Fi
Echo "Found odd number"
Done
When you run it, you'll get the following result:
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Number is an even number!!
Found odd number
Break statement
Since all of the lines of code up to the break statement have been executed, the break statement is used to end the loop's execution. It then descends to the code following the loop's conclusion.
Syntax:
To get out of a loop, use the break statement below.
Break
This format can also be used to exit a nested loop with the break command -
Break n
The nth enclosing loop to exit from is defined by n.
Example:
Here's a simple example that demonstrates how the loop ends when an equals 5.
#!/bin/sh
a=0
While [ $a -lt 10 ]
Do
Echo $a
If [ $a -eq 5 ]
Then
Break
Fi
a=expr $a + 1
Done
When you run it, you'll get the following result:
0
1
2
3
4
5
Here's a simple nested for loop illustration. If var1 equals 2 and var2 equals 0, this script exits all loops.
#!/bin/sh
For var1 in 1 2 3
Do
For var2 in 0 5
Do
If [ $var1 -eq 2 -a $var2 -eq 0 ]
Then
Break 2
Else
Echo "$var1 $var2"
Fi
Done
Done
When you run it, you'll get the following result. You have a break command with the argument 2 in the inner loop. This means that if a condition is met, you can exit the outer loop and eventually the inner loop as well.
1 0
1 5
Q6) Write history of the Internet?
A6) It is an interconnected computer network system that spans the globe. It makes use of the TCP/IP protocol, which is widely used on the internet. A unique IP address is assigned to every machine on the Internet. An IP Address (for example, 110.22.33.114) is a series of integers that identify the location of a computer.
A DNS (Domain Name Server) computer is used to give an IP Address a name so that a user can find a computer by name.
ARPANET was expanded to connect the Department of Defense with US colleges conducting defense-related research. It included most of the country's major universities. When University College London (UK) and Royal Radar Network (Norway) joined to the ARPANET and formed a network of networks, the concept of networking received a boost.
Stanford University's Vinton Cerf, Yogen Dalal, and Carl Sunshine invented the name "internet" to characterize this network of networks. They also collaborated on protocols to make information sharing via the Internet easier. The Transmission Control Protocol (TCP) is still the networking's backbone.
In the 1960s, the Internet was created as a means for government researchers to communicate information. In the 1960s, computers were enormous and stationary, and in order to access information stored on them, one had to either travel to the computer's location or have magnetic computer tapes transported through the mail system.
The escalation of the Cold War was another factor in the development of the Internet. The launch of the Sputnik satellite by the Soviet Union prompted the United States Defense Department to examine how information may be distributed even after a nuclear attack. This eventually led to the creation of the ARPANET (Advanced Research Projects Agency Network), which eventually evolved into the Internet we know today. ARPANET was a huge success, but it was only open to a few academic and research institutions with Defense Department contracts. As a result, new networks were formed to facilitate information sharing.
The Internet celebrates its formal birthday on January 1, 1983. Prior to this, there was no standard means for computer networks to communicate with one another. Transfer Control Protocol/Internetwork Protocol (TCP/IP) was created as a new communication protocol.
This allowed various types of computers on various networks to "speak" to one another. On January 1, 1983, ARPANET and the Defense Data Network switched to the TCP/IP standard, resulting in the birth of the Internet. A universal language could now connect all networks.
Q7) Explain SMTP?
A7) Simple Mail Transfer Protocol (SMTP)
E-mail system is implemented with the help of Message Transfer Agents (MTA). There are normally two MTAs in each mailing system. One for sending e-mails and another for receiving e-mails. The formal protocol that defines the MTA client and server in the internet is called Simple Mail Transfer Protocol (SMTP).
Fig shows the range of SMTP protocol.
By referring the above diagram, we can say that SMTP is used two times. That is between the sender and sender’s mail server and between the sender’s mail server and receiver’s mail server. Another protocol is used between the receiver’s mail server and receiver.
SMTP simply defines how commands and responses must be sent back and forth.
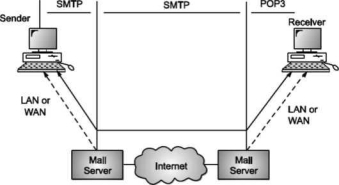
SMTP is a simple ASCII protocol
It establishes a TCP connection between a sender and port number 25 of the receiver. No checksums are generally required because TCP provides a reliable byte stream. After exchanging all the e-mail, the connection is released.
Commands and Responses
SMTP uses commands and responses to transfer messages between an MTA client and MTA server.
Each command or reply is terminated by a two-character (carriage return and line feed) end-of-line token.

Fig. Commands and Responses
Commands
Client sends commands to the server. SMTP defines 14 commands. Out of that first 5 commands are mandatory; every implementation must support these commands.
The next three commands are often used and are highly recommended. Last six commands are hardly used.
Q8) Explain POP?
A8) POP
Post Office Protocol, version 3 (POP3) is simple and feature-limited. The POP3 client software is installed on the receiving machine; the POP3 server software is installed on the mail server.
If the user wants to download e-mails from the mailbox on the mail server, mail access begins with the client. On TCP port 110, the client opens a connection to the server. In order to reach the mailbox, it then sends the user name and password. The user will then, one by one, list and retrieve the mail messages.
There are two modes for POP3: the delete mode and the hold mode. In the delete mode, after each retrieval, the mail is removed from the mailbox. In keep mode, after retrieval, the mail stays in the mailbox. When the user is operating on their permanent device, the delete mode is usually used and after reading or replying, the received mail can be saved and sorted. Normally, the keep mode is used when the user accesses her mail away from her main computer (e.g., a laptop). For later retrieval and organisation, the mail is read but kept in the system.
In some aspects, POP3 is deficient. It does not allow the user to arrange his mail on the server; it is impossible for the user to have multiple folders on the server. (The user will build directories on their own computer, of course.)
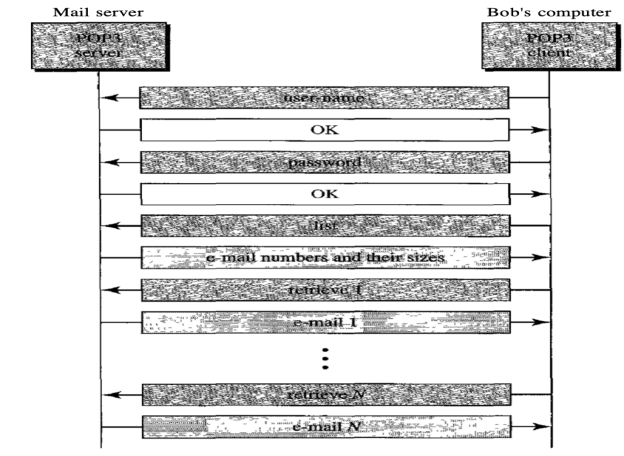
Fig: Command and response of POP3
Advantages of POP3
- As they are already saved on our PC, it provides easy and simple access to the emails.
- The size of the email that we receive or send has no limit.
- When all emails are saved on the local computer, it takes less server storage space.
- The maximum size of a mailbox is available, but the size of the hard disc is limited.
- It is a straightforward protocol, so it is one of the most common protocols used today.
- Configuring and using it is simple.
Disadvantages of POP3
- By default, if the emails are downloaded from the server, all the emails are deleted from the server. Mails will also not be accessed from other computers unless they are programmed to leave a mail copy on the server.
- It can be tough to move the mail folder from the local computer to another machine.
- As all of the attachments are placed on your local computer, if the virus scanner does not scan them, there is a high chance of a virus attack. Virus attacks can destroy your machine.
- There could also be corruption in the email folder that is downloaded from the mail server.
- Mails are saved on a local server, so the email folder can be accessed by someone who is sitting on your machine.
Q9) What is E-mail?
A9) E-mail is defined as the transmission of messages on the Internet. It is one of the most commonly used features over communications networks that may contain text, files, images, or other attachments. Generally, it is information that is stored on a computer sent through a network to a specified individual or group of individuals.
Email messages are conveyed through email servers; it uses multiple protocols within the TCP/IP suite. For example, SMTP is a protocol, stands for simple mail transfer protocol and used to send messages whereas other protocols IMAP or POP are used to retrieve messages from a mail server. If you want to login to your mail account, you just need to enter a valid email address, password, and the mail servers used to send and receive messages.
Although most of the webmail servers automatically configure your mail account, therefore, you only required to enter your email address and password. However, you may need to manually configure each account if you use an email client like Microsoft Outlook or Apple Mail. In addition, to enter the email address and password, you may also need to enter incoming and outgoing mail servers and the correct port numbers for each one.
Q10) What are the uses of email?
A10) Uses of email
Email can be used in different ways: it can be used to communicate either within an organization or personally, including between two people or a large group of people. Most people get benefit from communicating by email with colleagues or friends or individuals or small groups. It allows you to communicate with others around the world and send and receive images, documents, links, and other attachments. Additionally, it offers benefit users to communicate with the flexibility on their own schedule.
There is another benefit of using email; if you use it to communicate between two people or small groups that will beneficial to remind participants of approaching due dates and time-sensitive activities and send professional follow-up emails after appointments. Users can also use the email to quickly remind all upcoming events or inform the group of a time change. Furthermore, it can be used by companies or organizations to convey information to large numbers of employees or customers. Mainly, email is used for newsletters, where mailing list subscribers are sent email marketing campaigns directly and promoted content from a company.
Email can also be used to move a latent sale into a completed purchase or turn leads into paying customers. For example, a company may create an email that is used to send emails automatically to online customers who contain products in their shopping cart. This email can help to remind consumers that they have items in their cart and stimulate them to purchase those items before the items run out of stock. Also, emails are used to get reviews by customers after making a purchase. They can survey by including a question to review the quality of service.
Q11) Write advantages of Email?
A11) Advantages of Email
There are many advantages of email, which are as follows:
● Cost-effective: Email is a very cost-effective service to communicate with others as there are several email services available to individuals and organizations for free of cost. Once a user is online, it does not include any additional charge for the services.
● Email offers users the benefit of accessing email from anywhere at any time if they have an Internet connection.
● Email offers you an incurable communication process, which enables you to send a response at a convenient time. Also, it offers users a better option to communicate easily regardless of different schedules users.
● Speed and simplicity: Email can be composed very easily with the correct information and contacts. Also, minimum lag time, it can be exchanged quickly.
● Mass sending: You can send a message easily to large numbers of people through email.
● Email exchanges can be saved for future retrieval, which allows users to keep important conversations or confirmations in their records and can be searched and retrieved when they needed quickly.
● Email provides a simple user interface and enables users to categorize and filter their messages. This can help you recognize unwanted emails like junk and spam mail. Also, users can find specific messages easily when they are needed.
● As compared to traditional posts, emails are delivered extremely fast.
● Email is beneficial for the planet, as it is paperless. It reduces the cost of paper and helps to save the environment by reducing paper usage.
● It also offers a benefit to attaching the original message at the time you reply to an email. This is beneficial when you get hundreds of emails a day, and the recipient knows what you are talking about.
● Furthermore, emails are beneficial for advertising products. As email is a form of communication, organizations or companies can interact with a lot of people and inform them in a short time.
Q12) Explain the steps to compose and send e-mail?
A12) To write and send e-mail with data and messages, do the following:
Step 1: In order to create a new e-mail and send data through it a user has to click on “Compose” option available at the top of inbox option as shown in below picture.
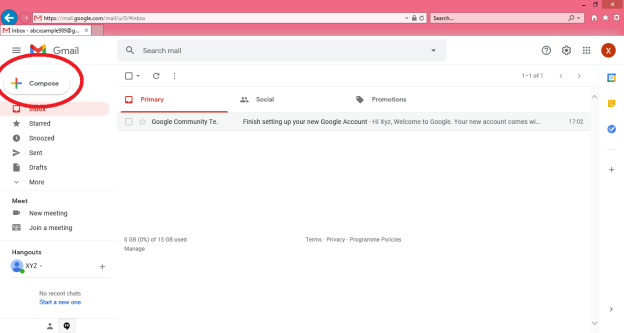
Step 2: A “New Message” box will appear as you can see in the below picture asking you to enter details in order to create a new e-mail message.
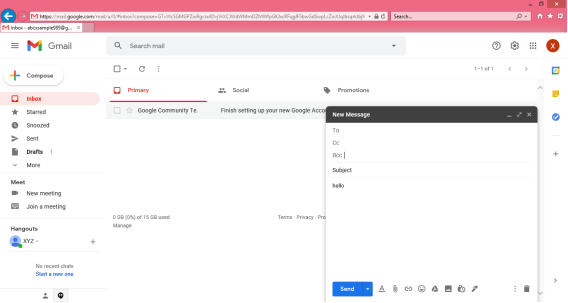
Note: New messages in an e-mail are stored in Inbox folder of the e-mail and all saved messages appears in draft folder of your e-mail.
Here, In the New Message box, you will see the following things:
To: Mail ID of the recipient
Cc: To send a Carbon copy i.e., send this mail to several people at the same time and also show everyone the email id’s of all the other people who have received it.
Bcc: To send a Blind Carbon copy i.e., send this mail to several people at the same time but without showing the person in Bcc list about who else has received this mail.
Subject: Is the main part which will be shown first to the person receiving the mail. It should be short and relevant to your mail and must tell the reason for your e-mail.
Body: The body of the e-mail is the blank white part where the user can type his message and can even customise it. In above picture we have written “Hello” to show the body of this e-mail.
Insert Bar: This bar is available at the bottom of the e-mail compose window. It includes various options to insert document, links, emoji’s, Google drive files, images, signature, delete the email etc.

User simply has to click on one of these options to insert an object and a new window will appear that will ask you about the file location which you want to insert into this mail. After locating the file just click on insert and the file will start uploading to this e-mail, once it is done it can be seen in the e-mail window. You can also open or remove the file from the e-mail using the options available alongside your uploaded file.
Delete button: There is a dustbin icon at the end of this email which lets a user to delete or discard there typed mail. Once discarded the mail cannot be retrieved.
Note: The close option is also available at the top of the window but that option will not delete the e-mail you have typed it simply closes the window and save the typed e-mail in drafts folder for future editing or usage purpose.
Step 3: The send icon is the blue one which is used to send the e-mail once it is composed. All the files inserted along with the text will be sent to the e-mail addresses once the user clicks on this button.
Note: There are various other options available under this Mail compose window such as Print, Schedule send, Minimize and Maximize the window which can be used for better e-mail experience of the user.
Q13) What is SMTP working?
A13) SMTP Working
SMTP works in the following three stages:
a) Connection Establishment
b) Message Transfer
c) Connection Termination
These are explained below:
Connection Establishment
Once the TCP connection is made on port no. 25, SMTP server starts the connection phase. This phase involves following three steps which are explained in the Fig.
The server tells the client that it is ready to receive mail by using the code 220. If the server is not ready, it sends code 421 which tells that service is not available.
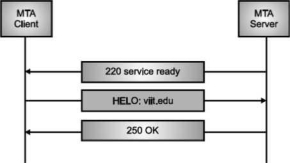
Fig. Connection Establishment
Once the server becomes ready to receive the mails, client sends HELO message to identify itself using the domain name address. This is important step which informs the server of the domain name of the client. Remember that during TCP connection establishment, the sender and receiver know each other through their IP addresses.
Server responds with code 250 which tells that the request command is completed. Message Transfer Once the connection has been established, SMTP server sends messages to SMTP receiver.
The messages are transferred in three stages
- A MAIL command identifies the message originator.
- RCPT command identifies the receiver of the message.
- DATA command transfers the message text.
Connection Closing
After the message is transferred successfully, the client terminates the connection. The connection is terminated in two steps The client sends the quit command.
The server responds with code 221 or some other appropriate code. After the connection termination phase, the TCP connection must be closed.
MIME Short for Multipurpose Internet Mail Extensions, a specification for formatting non-ASCII messages so that they can be sent over the Internet.
Many e-mail clients now support MIME, which enables them to send and receive graphics, audio, and video files via the Internet mail system. In addition, MIME supports messages in character sets other than ASCII.
There are many predefined MIME types, such as GIF graphics files and PostScript files. It is also possible to define your own MIME types.
In addition to e-mail applications, Web browsers also support various MIME types. This enables the browser to display or output files that are not in HTML format.
Q14) Write the disadvantages of email?
A14) Disadvantages of Email
● Impersonal: As compared to other forms of communication, emails are less personal. For example, when you talk to anyone over the phone or meeting face to face is more appropriate for communicating than email.
● Misunderstandings: As email includes only text, and there is no tone of voice or body language to provide context. Therefore, misunderstandings can occur easily with email. If someone sends a joke on email, it can be taken seriously. Also, well-meaning information can be quickly typed as rude or aggressive that can impact wrong. Additionally, if someone types with short abbreviations and descriptions to send content on the email, it can easily be misinterpreted.
● Malicious Use: As email can be sent by anyone if they have an only email address. Sometimes, an unauthorized person can send you mail, which can be harmful in terms of stealing your personal information. Thus, they can also use email to spread gossip or false information.
● Accidents Will Happen: With email, you can make fatal mistakes by clicking the wrong button in a hurry. For instance, instead of sending it to a single person, you can accidentally send sensitive information to a large group of people. Thus, the information can be disclosed, when you have clicked the wrong name in an address list. Therefore, it can be harmful and generate big trouble in the workplace.
● Spam: Although in recent days, the features of email have been improved, there are still big issues with unsolicited advertising arriving and spam through email. It can easily become overwhelming and takes time and energy to control.
● Information Overload: As it is very easy to send email to many people at a time, which can create information overload. In many modern workplaces, it is a major problem where it is required to move a lot of information and impossible to tell if an email is important. And, email needs organization and upkeep. The bad feeling is one of the other problems with email when you returned from vacation and found hundreds of unopened emails in your inbox.
● Viruses: Although there are many ways to travel viruses in the devices, email is one of the common ways to enter viruses and infect devices. Sometimes when you get a mail, it might be the virus come with an attached document. And, the virus can infect the system when you click on the email and open the attached link. Furthermore, an anonymous person or a trusted friend or contact can send infected emails.
● Pressure to Respond: If you get emails and you do not answer them, the sender can get annoyed and think you are ignoring them. Thus, this can be a reason to make pressure on your put to keep opening emails and then respond in some way.
● Time Consuming: When you get an email and read, write, and respond to emails that can take up vast amounts of time and energy. Many modern workers spend their most time with emails, which may be caused to take more time to complete work.
● Overlong Messages: Generally, email is a source of communication with the intention of brief messages. There are some people who write overlong messages that can take much time than required.
● Insecure: There are many hackers available that want to gain your important information, so email is a common source to seek sensitive data, such as political, financial, documents, or personal messages. In recent times, there have various high-profile cases occurred that shown how email is insecure about information theft.
Q15) Explain IMAP?
A15) Internet Message Access Protocol (IMAP) is an acronym for Internet Message Access Protocol. It's an application layer protocol that allows you to receive emails from a mail server. POP3 is one of the most widely used protocols for retrieving emails.
The client/server model is also used. On the one hand, we have an IMAP client, which is a computer programme. On the other hand, we have an IMAP server, which is likewise a computer-based operation. The two computers are linked through a network.
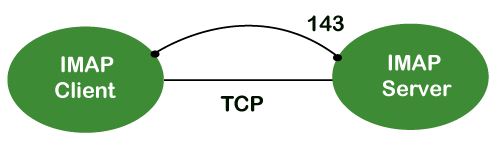
Because the IMAP protocol is based on the TCP/IP transport layer, it makes use of the protocol's dependability implicitly. The IMAP server listens to port 143 by default whenever a TCP connection is established between the IMAP client and the IMAP server, although this port number can be altered.
IMAP is configured to use two ports by default:
● Port 143: It is a non-encrypted IMAP port.
● Port 993: This port is used when IMAP client wants to connect through IMAP securely.
IMAP History and Standards
IMAP version 2 was the first version of IMAP to be formally documented as an internet standard in RFC 1064, which was published in July 1988. It was modified in August 1990, with the same version, in RFC 1176. As a result, they created IMAP3, a new version 3 document. In February 1991, the RFC 1203 was published. However, because IMAP3 was never embraced by the market, users continued to use IMAP2. IMAPb is, a protocol extension, was later built to add support for Multipurpose Internet Mail Extensions (MIME) to IMAP. Because of MIME's utility, this was a significant advance.
IMAPb is was never published as an RFC, despite this. This could be due to issues with the IMAP3 protocol. IMAP version 4, or IMAP4, was released in two RFCs in December 1994: RFC 1730, which described the basic protocol, and RFC 1731, which described the authentication mechanism for IMAP 4. IMAP 4 is the most recent and extensively used version of IMAP. It is still being developed, and the most recent version is known as IMAP4rev1 and is defined in RFC 2060. RFC 3501 is the most recent revision.
IMAP Features
IMAP was created for a specific purpose: to give users more flexibility in how they access their inbox. It can function in any of the three modes: online, offline, or disconnected. The offline and disconnected modes are of particular importance to most protocol users.
An IMAP protocol has the following characteristics:
● Access and retrieve mail from a distant server: The user can access and retrieve mail from a remote server while keeping the messages there.
● Set message flags: The user can keep track of which messages he has already viewed by setting message flags.
● Manage multiple mailboxes: The user has the ability to manage several mailboxes and transfer messages between them. For those working on numerous projects, the user can categorise them into various categories.
● Determine information prior to downloading: Before downloading the mail from the mail server, it decides whether to retrieve or not.
● Downloads a portion of a message: It allows you to download a component of a message, such as a single body part from the mime-multi part. This is useful when a message's short-text element contains huge multimedia files.
● Search: Users can conduct a search for the contents of emails.
● Check email-header: Examine the email header: Before downloading, users can check the email header.
Q16) Write the advantages of IMAP?
A16) Advantages
The following are some of the benefits of IMAP:
● It enables us to compose email messages from any location and on as many different devices as we like.
● Only when we click on a message can it be downloaded. As a result, we don't have to wait for the server to download all of our new messages before we can view them.
● With IMAP, attachments are not immediately downloaded. As a result, you can check your messages much faster and have more control over which attachments are accessed.
● IMAP, like the Post Office Protocol, can be utilised offline (POP).
● It allows you to delete messages, search for keywords in the body of emails, create and manage multiple mailboxes or folders, and display the headers of emails for quick visual searches.
Q17) Explain different web browsers?
A17) Web browsers are programmes that are installed on your computer. A web browser, such as Netscape Navigator, Microsoft Internet Explorer, or Mozilla Firefox, is required to access the Internet.
Currently, you must navigate through our website tutorialspoint.com using any type of Web browser. Web browsing or web surfing is the process of navigating through pages of information on the Internet.
There are four major web browsers: Internet Explorer, Firefox, Netscape, and Safari, but there are several others. Complete Browser Statistics may be of interest to you. Now we'll take a closer look at these browsers.
We should aim to make a site compatible with as many browsers as feasible when developing it. Especially sites should be adaptable to main browsers like Explorer, Firefox, Chrome, Netscape, Opera, and Safari.
Internet Explorer

Microsoft's Internet Explorer (IE) is a software product. This is the most widely used web browser on the planet. This was debuted in 1995 in conjunction with the release of Windows 95, and it surpassed Netscape in popularity in 1998.
Internet Explorer (also known as IE or MSIE) is a free web browser that allows users to view web pages on the internet. It can also be used to access online banking, internet marketing, listen to and watch streaming videos, and many other things. Microsoft first released it in 1995. It was created in response to Netscape Navigator, the first geographical browser.
For many years, from 1999 to 2012, Microsoft Internet Explorer was the most used web browser, surpassing Netscape Navigator. Network file sharing, multiple internet connections, active Scripting, and security settings are all included.
Google Chrome

Google created this web browser, and the beta version was published on September 2, 2008 for Microsoft Windows. Chrome is now one of the most popular web browsers, with a global market share of more than 50%.
Google Chrome is the most popular open-source internet browser for accessing information on the World Wide Web. It was released on December 11, 2008, by Google for Windows, Linux, Mac OS X, Android, and iOS users. It provides Web security through a sandboxing-based technique. It also adheres to web standards such as HTML5 and CSS (cascading style sheet).
Google Chrome was the first online browser to integrate the search box and address bar, a feature that was quickly replicated by other competitors. Google launched the Chrome Web Store in 2010, allowing users to purchase and install Web-based applications.
Mozilla Firefox

Mozilla has created a new browser called Firefox. It was first introduced in 2004 and has since evolved to become the Internet's second most used browser.
Mozilla Firefox is an open-source web browser that allows users to access information on the Internet. Firefox, the popular Web browser, has a simpler user interface and better download speeds than Internet Explorer. To translate web pages, it employs the Gecko layout engine, which follows current and predicted web standards.
Firefox was popular as a replacement for Internet Explorer 6.0 because it protected users from spyware and harmful websites. After Google Chrome, Apple Safari, and UC Browser, it was the fourth most popular web browser in 2017.
Safari

Safari is an Apple Inc. Web browser that comes standard with Mac OS X. It was originally made available to the public as a public beta in January 2003. Safari offers excellent compatibility for modern technologies like as XHTML and CSS2.
Safari is a web browser for the iPhone, iPad, and iPod Touch, as well as the Macintosh and Windows operating systems. Apple, Inc. Released it on June 30, 2003. It is the primary browser for Apple's operating systems, such as OS X for MacBook and Mac computers and iOS for iPad and iPhone smartphones and tablets. After Microsoft Internet Explorer, Mozilla Firefox, and Google Chrome, it is the fourth most used browser. It makes use of the WebKit engine, which handles font rendering, graphics display, page layout, and JavaScript execution.
Opera

Opera is a full-featured browser that is smaller and faster than most other browsers. With a keyboard interface, numerous windows, zoom functions, and more, it's quick and easy to use. There are Java and non-Java versions available. Ideal for novices to the Internet, schoolchildren, the handicapped, and as a CD-Rom and kiosk front-end.
Konqueror

Konqueror is an HTML 4.01 compliant web browser that also supports Java applets, JavaScript, CSS 1, CSS 2.1, and Netscape plugins. This programme functions as a file manager and offers basic file management on local UNIX filesystems, ranging from simple cut/copy/paste operations to advanced remote and local network file browsing.
Lynx

Lynx is a full-featured Web browser for Unix, VMS, and other platforms with cursor-addressable character-cell terminals or emulators.
Q18) Define read command?
A18) The read command in Linux is used to read a line's contents into a variable. For Linux systems, this is a built-in command. As a result, we won't need to install any more software. When writing a bash script, it's a simple tool to utilise to get user input. It's a useful tool, just like the echo command and the positional parameter. It's used to separate the words associated with the shell variable. It is mostly used to receive user input, but it can also be used to implement functions while receiving input.
Syntax:
The read command has the following basic syntax:
Read [options] [name...]
How to use the read command?
With and without arguments, the read command can be used. Let's have a look at how the read command might be used in different situations:
● Default Behaviour
If we run the read command without any arguments, it will accept a line as user input and save it in the 'REPLY' built-in variable. Run the command as follows:
Read
The command above will prompt the user for input. To save the user input, type it in and hit the ENTER key. Execute the command as follows to see the entered content:
Echo $REPLY
The above command will display the 'REPLY' variable's stored input.
● Specify the variables to store the values
We can define the variables that will be used to store the data. If the number of provided variables is less than the number of entered words, all leftover words will be stored in the last variable by default. Consider the command below:
Read var1 var2 var3
● read -p
For the prompt text, the '-p' option is used. It reads the data as well as some hints. This tip text guides us through the process of typing text, such as what to type. Consider the command below:
Read -p " Enter your name: "
The command above will prompt you for your name; type it in. The name will be saved in the variable 'REPLY.' Execute the following command to see the variable value:
Echo " My name is $REPLY"
● read -n
The '-n' option shortens the character length in the entered text. It will not allow you to type more than the number of characters specified. It will automatically cease reading after the maximum number of characters has been reached. Execute the following command to limit the number of characters to six:
Read -n 6 -p " Enter 6 characters only: "
We can't enter more than 6 characters with the instruction above.
● read -s
For security reasons, the '-s' option is utilised. It is used to read sensitive information. The entered text will not appear in the terminal if this option is selected. With this option, we can use additional options. In this option, the characters are read aloud. Its primary function is to read passwords from the keyboard. Consider the command below:
Read -s -p "Enter password: "
The above command will demand for a password, but the password will not be displayed on the terminal when we type it.
Q19) Explain echo statement?
A19) In Linux, the echo command is used to display a line of text/string that has been supplied as an argument. This is a built-in command that outputs status text to the screen or a file. It's typically used in shell scripts and batch files.
Syntax:
Echo [option] [string]
Displaying a text/string:
Syntax:
Echo [string]
Example:
Echo -e "World \bis \bBeautiful"
Output
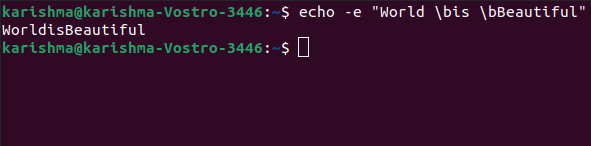
Implementations of the echo command
SymbOS, KolibriOS, HP MPE/iX, ReactOS, Microsoft Windows, IBM OS/2, Digital Research FlexOS, Acorn Computers Panos, Microwave OS-9, Zilog Z80-RIO, MetaComCo TRIPOS, TSC FLEX, Multics, Unix-like, and Unix operating systems all provide the echo command.
Several shells, including Csh-like shells (such as zsh or Bash), Bourne-like shells, COMMAND.COM, and cmd.exe, use the echo command as a built-in command.
The command also exists inside the EFI shell.
Echo Command Options
The echo command has a number of different options. The following alternatives are listed and explained:
1. b: Use this option to remove all spaces from the text/string.
Example
Echo -e "Flowers \bare \bBeautiful"
2.\c: This option is used in conjunction with the '-e' backspace interpreter to suppress the trailing new line and continue without emitting any new lines.
Example
Echo -e "World \cis Beautiful"
3. \n: This option is used to generate a new line, which will be formed from the location where it is used.
Example:
Echo -e "World \nis \nBeautiful"
4. Echo *: This option prints every folder or file in the system. It's the same as the Linux ls command.
Example:
Echo *
5. -n: This option is used to skip echoing new lines at the end.
Example:
Echo -n "World is Beautiful"
6. Print "Hello All!" on the terminal: Use the following command to print the text "Hello All!" on the terminal:
Example:
$ echo "Hello All!"
7. Print specified file types: For example, if we want to print every '.c' file, we can use the command below:
Example:
$ echo *.txt