Unit - 3
Inheritance, packages and interfaces
Q1) What is inheritance in java?
A1) Inheritance in Java is a mechanism in which one object acquires all the properties and behaviour of a parent object. It is an important part of OOPs (Object Oriented programming system).
The idea behind inheritance in Java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of the parent class. Moreover, you can add new methods and fields in your current class also.
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
Why use inheritance in java
● For Method overriding (so runtime polymorphism can be achieved).
● For Code Reusability.
Terms used in Inheritance
● Class: A class is a group of objects which have common properties. It is a template or blueprint from which objects are created.
● Sub Class/Child Class: Subclass is a class which inherits the other class. It is also called a derived class, extended class, or child class.
● Super Class/Parent Class: Superclass is the class from where a subclass inherits the features. It is also called a base class or a parent class.
● Reusability: As the name specifies, reusability is a mechanism which facilitates you to reuse the fields and methods of the existing class when you create a new class. You can use the same fields and methods already defined in the previous class.
The syntax of Java Inheritance
- Class Subclass-name extends Superclass-name
- {
- //methods and fields
- }
The extends keyword indicates that you are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality.
In the terminology of Java, a class which is inherited is called a parent or superclass, and the new class is called child or subclass.
Q2) Write an example of java inheritance?
A2) Java Inheritance Example
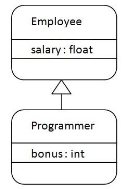
As displayed in the above figure, Programmer is the subclass and Employee is the superclass. The relationship between the two classes is Programmer IS-A Employee. It means that Programmer is a type of Employee.
- Class Employee{
- Float salary=40000;
- }
- Class Programmer extends Employee{
- Int bonus=10000;
- Public static void main(String args[]){
- Programmer p=new Programmer();
- System.out.println("Programmer salary is:"+p.salary);
- System.out.println("Bonus of Programmer is:"+p.bonus);
- }
- }
Programmer salary is:40000.0
Bonus of programmer is:10000
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
Q3) Explain types of inheritance in java?
A3) Types of inheritance in java
On the basis of class, there can be three types of inheritance in java: single, multilevel and hierarchical.
In java programming, multiple and hybrid inheritance is supported through interface only.
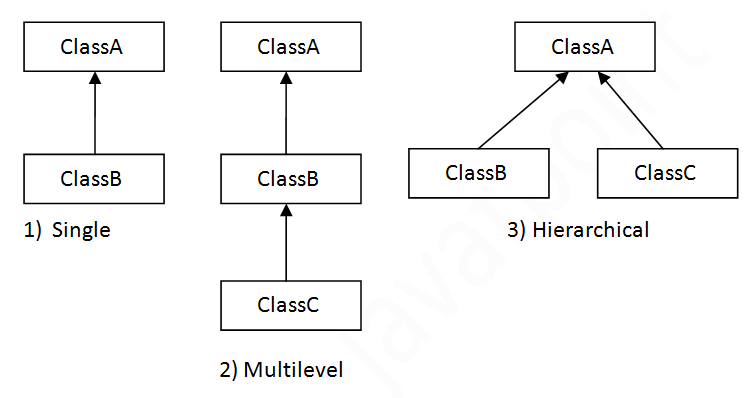
Note: Multiple inheritance is not supported in Java through class.
When one class inherits multiple classes, it is known as multiple inheritance.
For Example:
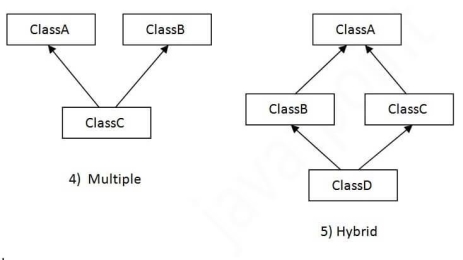
Single Inheritance Example
When a class inherits another class, it is known as a single inheritance. In the example given below, Dog class inherits the Animal class, so there is the single inheritance.
File: TestInheritance.java
- Class Animal{
- Void eat(){System.out.println("eating...");}
- }
- Class Dog extends Animal{
- Void bark(){System.out.println("barking...");}
- }
- Class TestInheritance{
- Public static void main(String args[]){
- Dog d=new Dog();
- d.bark();
- d.eat();
- }}
Output:
Barking...
Eating…
Multilevel Inheritance Example
When there is a chain of inheritance, it is known as multilevel inheritance. As you can see in the example given below, BabyDog class inherits the Dog class which again inherits the Animal class, so there is a multilevel inheritance.
File: TestInheritance2.java
- Class Animal{
- Void eat(){System.out.println("eating...");}
- }
- Class Dog extends Animal{
- Void bark(){System.out.println("barking...");}
- }
- Class BabyDog extends Dog{
- Void weep(){System.out.println("weeping...");}
- }
- Class TestInheritance2{
- Public static void main(String args[]){
- BabyDog d=new BabyDog();
- d.weep();
- d.bark();
- d.eat();
- }}
Output:
Weeping...
Barking...
Eating…
Hierarchical Inheritance Example
When two or more classes inherits a single class, it is known as hierarchical inheritance. In the example given below, Dog and Cat classes inherits the Animal class, so there is hierarchical inheritance.
File: TestInheritance3.java
- Class Animal{
- Void eat(){System.out.println("eating...");}
- }
- Class Dog extends Animal{
- Void bark(){System.out.println("barking...");}
- }
- Class Cat extends Animal{
- Void meow(){System.out.println("meowing...");}
- }
- Class TestInheritance3{
- Public static void main(String args[]){
- Cat c=new Cat();
- c.meow();
- c.eat();
- //c.bark();//C.T.Error
- }}
Output:
Meowing...
Eating...
Q4) Explain super keywords with an example?
A4) The super keyword
The super keyword is similar to this keyword. Following are the scenarios where the super keyword is used.
● It is used to differentiate the members of superclass from the members of subclass, if they have same names.
● It is used to invoke the superclass constructor from subclass.
Differentiating the Members
If a class is inheriting the properties of another class. And if the members of the superclass have the names same as the sub class, to differentiate these variables we use super keyword as shown below.
Super.variable
Super.method();
Sample Code
This section provides you with a program that demonstrates the usage of the super keyword.
In the given program, you have two classes namely Sub_class and Super_class, both have a method named display() with different implementations, and a variable named num with different values. We are invoking display() method of both classes and printing the value of the variable num of both classes. Here you can observe that we have used super keyword to differentiate the members of superclass from subclass.
Copy and paste the program in a file with name Sub_class.java.
Example
Class Super_class {
int num = 20;
// display method of superclass
public void display() {
System.out.println("This is the display method of superclass");
}
}
Public class Sub_class extends Super_class {
int num = 10;
// display method of sub class
public void display() {
System.out.println("This is the display method of subclass");
}
public void my_method() {
// Instantiating subclass
Sub_class sub = new Sub_class();
// Invoking the display() method of sub class
Sub.display();
// Invoking the display() method of superclass
Super.display();
// printing the value of variable num of subclass
System.out.println("value of the variable named num in sub class:"+ sub.num);
// printing the value of variable num of superclass
System.out.println("value of the variable named num in super class:"+ super.num);
}
public static void main(String args[]) {
Sub_classobj = new Sub_class();
Obj.my_method();
}
}
Compile and execute the above code using the following syntax.
JavacSuper_Demo
Java Super
On executing the program, you will get the following result −
Output
This is the display method of subclass
This is the display method of superclass
Value of the variable named num in sub class:10
Value of the variable named num in super class:20
Q5) What is this keyword in java?
A5) This keyword is a reference variable that refers to the current object. There are various uses of this keyword in Java.
It can be used to refer to current class properties such as instance methods, variable, constructors, etc.
It can also be passed as an argument into the methods or constructors. It can also be returned from the method as the current class instance.
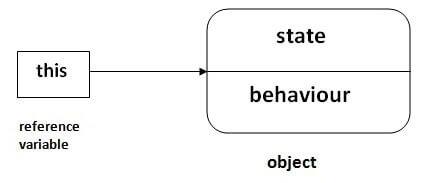
Q6) What are the main uses of this keyword?
A6) There are the following uses of this keyword.
- This can be used to refer to the current class instance variable.
- This can be used to invoke current class method (implicitly)
- This() can be used to invoke the current class constructor.
- This can be passed as an argument in the method call.
Example:
Class S2
{ void m(S2 obj)
{ System.out.println("method is invoked");
}
Void p(){
m(this);
}//method
Public static void main(String args[]){
S2 s1 = new S2();
s1.p();
}
}
OUTPUT:
Method is invoked
- This can be passed as an argument in the constructor call.
Example
Class B{
A4 obj;
B(A4 obj) {
This.obj=obj;
}
Void display() {
System.out.println(obj.data);//using data member of A4 class
}
}
Class A4{
Int data=10;
A4(){
B b=new B(this);
b.display();
}
Public static void main(String args[]){
A4 a=new A4();
}
}
Output:
10
- This can be used to return the current class instance from the method.
We are using this keyword to distinguish local variable and instance variable.
Class Student{
Int rollno;
String name;
Float fee;
Student(int rollno,String name,float fee)
{ this.rollno=rollno;
This.name=name;
This.fee=fee;
}
Void display(){System.out.println(rollno+" "+name+" "+fee);}
}
Class TestThis2
{
Public static void main(String args[])
{
Student s1=new Student(111,"ankit",5000f);
Student s2=new Student(112,"sumit",6000f);
s1.display();
s2.display();
}//void main
} //class TestThis2
Output:
111 ankit 5000f
112 sumit 6000f
Q7) Why is Inheritance used in Java?
A7) There are various advantages of using inheritance in Java that is given below:
- Inheritance provides code reusability. The derived class does not need to redefine the method of base class unless it needs to provide the specific implementation of the method.
- Runtime polymorphism cannot be achieved without using inheritance.
- We can simulate the inheritance of classes with the real-time objects which makes OOPs more realistic.
- Inheritance provides data hiding. The base class can hide some data from the derived class by making it private.
- Method overriding cannot be achieved without inheritance. By method overriding, we can give a specific implementation of some basic method contained by the base class.
Q8) What is super in java?
A8) The super keyword in Java is a reference variable that is used to refer to the immediate parent class object.
Whenever we create the instance of the subclass, an instance of the parent class is created implicitly which is referred by super reference variable.
The super() is called in the class constructor implicitly by the compiler if there is no super or this.
Class Animal
{ Animal()
{ System.out.println("animal is created");}
}
Class Dog extends Animal
{ Dog()
{
System.out.println("dog is created");
}
}
Class TestSuper4 {
Public static void main(String args[]) {
Dog d=new Dog();
}
}
Output:
Animal is created
Dog is created
Q9) What are the differences between this and super keyword?
A9) There are the following differences between this and super keyword
- The super keyword always points to the parent class contexts whereas this keyword always points to the current class context.
- The super keyword is primarily used for initializing the base class variables within the derived class constructor whereas this keyword primarily used to differentiate between local and instance variables when passed in the class constructor.
- The super and this must be the first statement inside constructor otherwise the compiler will throw an error.
Q10) Describe method overloading with example?
A10) Java allows us to create multiple methods in a class even with the same method name, until their parameters are different. In this case methods are said to be overloaded and this complete process is known as method overloading.
Method overloading is an implementation of polymorphism in a code which is “One interface, multiple methods” paradigm.
To invoke an overloaded method, it is important to pass the right number or arguments or parameters; since this will determine which version of method to actually call.
All the overloaded methods have different return type but we cant distinguish method based on the type of data they return.
Consider a simple example of method overloading:
Class Addition {
// Overloaded method takes two int parameters
public int add(int a, int b)
{
return (a + b);
}
// Overloaded method takes three int parameters
public int add(int a, int b, int c)
{
return (a + b + c);
}
// Overloaded method takes two double parameters
public double add(double a, double b)
{
return (a + b);
}
public static void main(String args[])
{
Addition a = new Addition();
System.out.println(a.add(100, 20));
System.out.println(a.add(11, 2, 3));
System.out.println(a.add(0.5, 2.5));
}
}
Output:
120
16
3.0
While creating a overloaded method it s not necessary that all the methods must relate to one another. For example one add() method can do addition, while other add() method can concatenate string; since each version of a method perform a separate activity you desire.
However, it is preferable to see a relationship between overloaded method as shown in the above example, where all add() method doing addition.
Q11) Write the benefits of method overloading?
A11) Benefit of method overloading
● Technique over-burdening builds the coherence of the program.
● This gives adaptability to developers with the goal that they can call similar strategy for various sorts of information.
● This makes the code look spotless.
● This lessens the execution time in light of the fact that the limiting is done in accumulation time itself.
● Technique over-burdening limits the intricacy of the code.
● With this, we can utilize the code once more, which saves memory.
Q12) Define method overriding with example?
A12) In Java, method overriding occurs when a subclass (child class) has the same method as the parent class.
In other words, method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
Usage of Java Method Overriding
● Method overriding is a technique for providing a particular implementation of a method that has already been implemented by its superclass.
● For runtime polymorphism, method overriding is employed.
Example
//Java Program to illustrate the use of Java Method Overriding
//Creating a parent class.
Class Vehicle{
//defining a method
void run(){System.out.println("Vehicle is running");}
}
//Creating a child class
Class Bike2 extends Vehicle{
//defining the same method as in the parent class
void run(){System.out.println("Bike is running safely");}
public static void main(String args[]){
Bike2 obj = new Bike2();//creating object
obj.run();//calling method
}
}
Output
Bike is running safely
Q13) What are the rules for method overriding?
A13) Rules for method overriding
● The argument list should match that of the overridden method exactly.
● The return type must be the same as or a subtype of the return type stated in the superclass's original overridden method.
● The access level must be lower than the access level of the overridden method. For instance, if the superclass method is marked public, the subclass overriding method cannot be either private or protected.
● Instance methods can only be overridden if the subclass inherits them.
● It is not possible to override a method that has been declared final.
● A static method cannot be overridden, but it can be re-declared.
● A method can't be overridden if it can't be inherited.
● Any superclass function that is not declared private or final can be overridden by a subclass in the same package as the instance's superclass.
● Only non-final methods labeled public or protected can be overridden by a subclass from a different package.
● Regardless of whether the overridden method throws exceptions or not, an overriding method can throw any unchecked exceptions. The overriding method, on the other hand, should not throw any checked exceptions that aren't specified by the overridden method. In comparison to the overridden method, the overriding method can throw fewer or narrower exceptions.
● Overriding constructors is not possible.
Q14) What do you mean by abstract classes?
A14) Abstract classes
● Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
● It can only be inherited by the concrete classes (descendent classes), where a concrete class can be instantiated.
● Abstract class act as a generic class having attributes and methods which can be commonly shared and accessed by the concrete classes.
● The attributes and methods in the abstract class are non-static, accessible by inheriting classes.
● Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class
● It can be represented as below
Abstract public class RentalCar {
// your code goes here
}
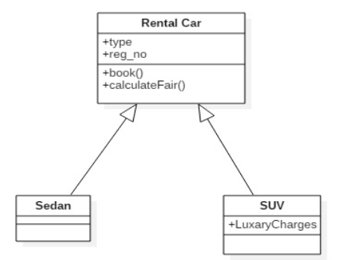
Fig: Example of abstract class
Q15) Write short notes on final keyword?
A15) The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
When you want a variable to constantly store the same value, such as PI, the final keyword comes in handy (3.14159...).
The last keyword is referred to as a "modifier."
Example
Set a variable to final, to prevent it from being overridden/modified:
Public class Main {
final int x = 10;
public static void main(String[] args) {
Main myObj = new Main();
myObj.x = 25; // will generate an error: cannot assign a value to a final variable
System.out.println(myObj.x);
}
}
Using Final with Inheritance
● The final keyword is final, which means we can't change anything.
● Variables, methods, and classes can all benefit from final keywords.
● If we use the final keyword for inheritance, that is, if we declare any method in the base class with the final keyword, the final method's implementation will be the same as in the derived class.
● If any other class extends this subclass, we can declare the final method in any subclass we wish.
Q16) Write a program to declare the final method with inheritance?
A16) Declare final methods with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final method parent of void type i.e
the implementation of this method is fixed throughout
all the derived classes or not overridden*/
final void parent() {
System.out.println("Hello , we are in parent method");
}
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of final method child of void type i.e
the implementation of this method is not fixed throughout
all the derived classes or not overridden*/
void child() {
System.out.println("Hello , we are in child method");
}
}
Class Test {
public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a method parent() by parent object
p.parent();
// Creation of Child class object
Child c = new Child();
// Calling a method child() by Child class object
c.child();
// Calling a method parent() by child object
c.parent();
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, we are in parent method
Hello, we are in child method
Hello, we are in parent method
Q17) How to declare the final variable with inheritance?
A17) Declare final variable with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final variable pa of string type i.e
the value of this variable is fixed throughout all
the derived classes or not overridden*/
final String pa = "Hello , We are in parent class variable";
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of variable ch of string type i.e
the value of this variable is not fixed throughout all
the derived classes or overridden*/
String ch = "Hello , We are in child class variable";
}
Class Test {
public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a variable pa by parent object
System.out.println(p.pa);
// Creation of Child class object
Child c = new Child();
// Calling a variable ch by Child object
System.out.println(c.ch);
// Calling a variable pa by Child object
System.out.println(c.pa);
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, We are in parent class variable
Hello, We are in child class variable
Hello, We are in parent class variable
Q18) Write the difference between method overloading and overriding?
A18) Difference between Method Overloading and Method Overriding are:
S.NO | Method Overloading | Method Overriding |
1. | Method overloading is a compile-time polymorphism. | Method overriding is a run-time polymorphism. |
2. | It helps to increase the readability of the program. | It is used to grant the specific implementation of the method which is already provided by its parent class or superclass. |
3. | It occurs within the class. | It is performed in two classes with inheritance relationships. |
4. | Method overloading may or may not require inheritance. | Method overriding always needs inheritance. |
5. | In method overloading, methods must have the same name and different signatures. | In method overriding, methods must have the same name and same signature. |
6. | In method overloading, the return type can or can not be the same, but we just have to change the parameter. | In method overriding, the return type must be the same or co-variant. |
Q19) How to inherit the abstract class?
A19) Inheriting the Abstract class
We may inherit the properties of the Employee class in the same way that we can inherit the properties of concrete classes in the following method:
Example
/* File name : Salary.java */
Public class Salary extends Employee {
private double salary; // Annual salary
public Salary(String name, String address, int number, double salary) {
super(name, address, number);
setSalary(salary);
}
public void mailCheck() {
System.out.println("Within mailCheck of Salary class ");
System.out.println("Mailing check to " + getName() + " with salary " + salary);
}
public double getSalary() {
return salary;
}
public void setSalary(double newSalary) {
if(newSalary >= 0.0) {
salary = newSalary;
}
}
public double computePay() {
System.out.println("Computing salary pay for " + getName());
return salary/52;
}
}
You can't instantiate the Employee class here, but you can instantiate the Salary Class, and you can access all three fields and seven methods of the Employee class using this instance, as seen below.
/* File name : AbstractDemo.java */
Public class AbstractDemo {
public static void main(String [] args) {
Salary s = new Salary("Mohd Mohtashim", "Ambehta, UP", 3, 3600.00);
Employee e = new Salary("John Adams", "Boston, MA", 2, 2400.00);
System.out.println("Call mailCheck using Salary reference --");
s.mailCheck();
System.out.println("\n Call mailCheck using Employee reference--");
e.mailCheck();
}
}
Output
Constructing an Employee
Constructing an Employee
Call mailCheck using Salary reference --
Within mailCheck of Salary class
Mailing check to Mohd Mohtashim with salary 3600.0
Call mailCheck using Employee reference--
Within mailCheck of Salary class
Mailing check to John Adams with salary 2400.0
Q20) Write a program to overload three methods with the same name?
A20) Program to overload three methods with the same name
Public class Add{
Public int add(int a , int b){
return (a + b);
}
Public int add(int a , int b , int c){
return (a + b + c) ;
}
Public double add(double a , double b){
return (a + b);
}
Public static void main( String args[]){
Add ob = new Add();
Ob.add(15,25);
Ob.add(15,25,35);
Ob.add(11.5 , 22.5);
}
}
Output:
40
75
34
Q21) Introduce interface?
A21) In Java, an interface is a reference type. It's comparable to a class. It consists of a set of abstract methods. When a class implements an interface, it inherits the interface's abstract methods.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods. Method bodies are only available for default and static methods.
The process of creating an interface is identical to that of creating a class. A class, on the other hand, describes an object's features and behaviors. An interface, on the other hand, contains the behaviors that a class implements.
Unless the interface's implementation class is abstract, all of the interface's methods must be declared in the class.
In the following ways, an interface is analogous to a class:
● Any number of methods can be included in an interface.
● An interface is written in a file with the extension.java, with the interface's name matching the file's name.
● A.class file contains the byte code for an interface.
● Packages have interfaces, and the bytecode file for each must be in the same directory structure as the package name.
Why do we use interfaces?
There are three primary reasons to utilize an interface. Below is a list of them.
● It's a technique for achieving abstraction.
● We can offer various inheritance functionality via an interface.
● It's useful for achieving loose coupling.
Q22) How to declare an interface?
A22) The interface keyword is used to declare an interface. All methods in an interface are declared with an empty body, and all fields are public, static, and final by default. A class that implements an interface is required to implement all of the interface's functions.
Syntax
Interface <interface_name>{
// declare constant fields
// declare methods that abstract
// by default.
}
Q23) How to implement an interface?
A23) When a class implements an interface, it's like the class signing a contract, agreeing to perform the interface's defined actions. If a class doesn't perform all of the interface's actions, it must declare itself abstract.
To implement an interface, a class utilizes the implements keyword. Following the extends component of the declaration, the implements keyword appears in the class declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
public void eat() {
System.out.println("Mammal eats");
}
public void travel() {
System.out.println("Mammal travels");
}
public int noOfLegs() {
return 0;
}
public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
Output
Mammal eats
Mammal travels
Q24) How to access implementations through interface references?
A24) Variables can be declared as object references that use an interface instead of a class.
Such a variable can refer to any instance that implements the stated interface.
When you use one of these references to invoke a method, the right version is used based on the actual instance of the interface being referred to.
This is one of the most important characteristics of interfaces. The method to be executed is dynamically looked up at runtime, allowing classes to be built after the code that calls methods on them.
This is analogous to accessing a subclass object using a superclass reference.
The callback() function is invoked in the following example using an interface reference variable:
Interface Callback {
void callback(int param);
}
Class Client implements Callback {
// Implement Callback's interface
public void callback(int p) {
System.out.println("callback called with " + p);
}
void aa() {
System.out.println("hi.");
}
}
Public class Main{
public static void main(String args[]) {
Callback c = new Client();
c.callback(42);
}
}
The following is the program's output:
Despite the fact that the variable c is of the interface type Callback, it has been assigned an instance of Client.
The callback() method can be used using c, but it cannot access any other members of the Client class.
Only the methods declared by the interface declaration are known to an interface reference variable.
As a result, because aa() is defined by Client but not Callback, c could not be used to access it.
Q25) What is the package?
A25) A java package is a group of similar types of classes, interfaces and sub-packages.
Package in java can be categorized in two form, built-in package and user-defined package.
There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
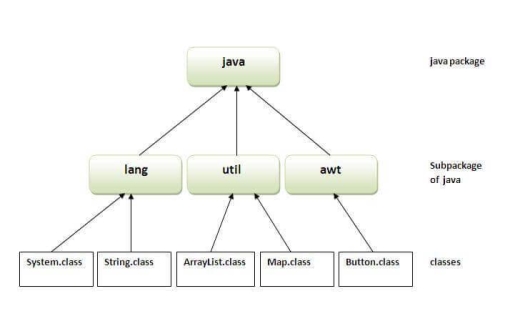
Fig - Package
Simple example of java package
The package keyword is used to create a package in java.
- //save as Simple.java
- Package mypack;
- Public class Simple{
- Public static void main(String args[]){
- System.out.println("Welcome to package");
- }
- }
How to compile java package
If you are not using any IDE, you need to follow the syntax given below:
- Javac -d directory javafilename
For example
- Javac -d . Simple.java
The -d switch specifies the destination where to put the generated class file. You can use any directory name like /home (in case of Linux), d:/abc (in case of windows) etc. If you want to keep the package within the same directory, you can use . (dot).
How to run java package program
You need to use fully qualified name e.g. Mypack.Simple etc to run the class.
To Compile: javac -d . Simple.java
To Run: java mypack.Simple
Output: Welcome to package
The -d is a switch that tells the compiler where to put the class file i.e. it represents destination. The . Represents the current folder.
Q26) Write the advantages of package?
A26) Advantage of Java Package
1) Java package is used to categorize the classes and interfaces so that they can be easily maintained.
2) Java package provides access protection.
3) Java package removes naming collision.
Q27) Write a sample program to implement an interface in Java?
A27) Program
Interface Results
{
final static float pi = 3.14f;
float areaOf(float l, float b);
}
Class Rectangle implements Results
{
public float areaOf(float l, float b)
{
return (l * b);
}
}
Class Square implements Results
{
public float areaOf(float l, float b)
{
return (l * l);
}
}
Class Circle implements Results
{
public float areaOf(float r, float b)
{
return (pi * r * r);
}
}
Public class InterfaceDemo
{
public static void main(String args[])
{
Rectangle rect = new Rectangle();
Square square = new Square();
Circle circle = new Circle();
System.out.println("Area of Rectangle: "+rect.areaOf(20.3f, 28.7f));
System.out.println("Are of square: "+square.areaOf(10.0f, 10.0f));
System.out.println("Area of Circle: "+circle.areaOf(5.2f, 0));
}
}
Output:
Area of Rectangle: 582.61
Are of square: 100.0
Area of Circle: 84.905594