Unit - 1
Client server model & socket interface
Q.1) What do you mean by client server model and server design ?
A1)
The Client Server model and Software design
● TCP/IP allows a programmer to connect and transfer data back and forth between two applications.
● It is possible to run an application on the same computer or on different machines.
● The use of TCP/IP is known as the client-server model, as one organisational process dominates.
Motivation
● Since TCP/IP does not have any mechanisms that immediately generate running programmes when a message arrives, a programme must wait before any requests arrive to accept communication.
● Many system administrators arrange to have communication programmes start automatically when OS boots to ensure computers are ready to talk. Every single programme runs indefinitely.
Terminology and concept
Client :
- A client is the name of an application that initiates peer-to-peer contact.
- It contacts a server, sends a request, and awaits an answer any time a client application runs.
- Clients are much simpler to build than servers, and typically do not require any special machine privileges to run.
Sever :
- Any software waiting for incoming contact requests from a client is a server. The server receives a request from the client, performs the requisite calculation, and returns the result to the client.
- Privilege and intricacy.
- Servers must have code that addresses the following issues: authentication, authorization, security of data, privacy and protection.
- Standard Vs Non-standard client software
● Normal application services consist of TCP/IP defined services and TCP/IP defined services and well-known, widely accepted protocol port identifiers allocated.
● The non-standard application is a locally defined application service (e.g., a private database system of an institution)
● Only when interacting outside the local community is the distinction between standard services and non-standard applications significant.
● System administrators typically arrange to identify service names within a specified context in such a way that users do not differentiate between local and standard services.
● The difference must be recognised by programmers who develop network applications that will be used on other sites.
Parametrization of clients
● More generality than others is provided by some client applications.
● In particular, some client software allows the user to define both the remote machine on which the server is running and the port number of the protocol that the server is listening to.
● Here's an example:
- Telnet Machine-Port Name-Port Name
- Include parameters when developing client application software that allow the user to completely define the port number for the destination machine and destination protocol.
Connectionless Vs Connection-oriented server
● They must choose between two styles of interaction when programmers develop client-server software: a connectionless style or a connection-oriented style.
● TCP- Connection-oriented : This gives all the reliability needed to communicate over the internet.
- Verifies the information arrives, retransmits it automatically.
- Checksum computing over records.
- Delete the duplicated kit automatically.
● UDP - Protocol.connectionless user datagram. No assurances with respect to reliable delivery.
● Application programmes use UDP only if:
(1) The protocol for the programme states that UDP must be used.
(2) The programme depends on broadcast or multicast hardware, or multicast hardware.
(3) Computational overhead or delay required for TCP virtual circuits cannot be tolerated by the application.
● In summary : Beginners are strongly encouraged to use TCP when developing client-server apps, as it provides secure, connection-oriented communication. Programs only use UDP if redundancy is handled by the application protocol, hardware broadcast or multicast is needed by the application, or virtual circuit overhead may not be tolerated by the application.
Stateless Vs Stateful server
● The server stores details about the status of the ongoing client contact, called the stateful server.
● Stateless servers are called servers that do not maintain any state details.
● Why do we need servers with statements?
● State data allows a server to remember what client previously requested and to measure an incremental response as each new request arrives=> performance.
● Why do we need servers that are stateless?
● If messages are lost, duplicated, or delivered out of order or the client computer crashes and reboots, state information on a server can become incorrect. If the server uses incorrect information when an answer is computed, it will react incorrectly.
Server as clients a Program can be both client and server
● It may be necessary for servers to access network resources that enable them to act as clients.
● The time of day must be collected by the file server so that files can be stamped with the access time. Suppose there is no time-of-day clock in the device it runs on. To obtain a time-of-day server, by submitting a request to a time-of-day server, the server functions as a client.
Q.2)What do you mean by socket interface ?
A2)
The Socket Interface
Berkeley sockets
ARPA group's Berkeley Sockets Goal: to combine TCP/IP with UNIX
● Application Communication Interface (API) API: Use UNIX calls wherever possible and add new functionality to the API.
● API Sockets (first appeared in Berkeley UNIX release 4.1)
● The de facto standard for TCP/IP networking with Inter
Specifying a protocol interface
● The Sockets API offers generalised functions that, using several possible protocols, allow network communication.
● For the Sockets API (protocol family PF INET), TCP/IP is one alternative.
● Socket functions only define the type of operation for the protocol.
Provides functions that use several possible protocols to facilitate network communication.
● One protocol family, supported by sockets, is PF INET.
● The protocols in the PF INET family are TCP and UDP.
The socket abstraction
- Socket Descriptor and File Descriptor
● Issue an open call to build a descriptor for a file. To store references to internal data structures for already opened files, a process maintains a descriptor table. In the descriptor table, the OS uses the file or socket descriptor as an index.
● The socket descriptor is generated by the socket() call; the open() call is the file descriptor.
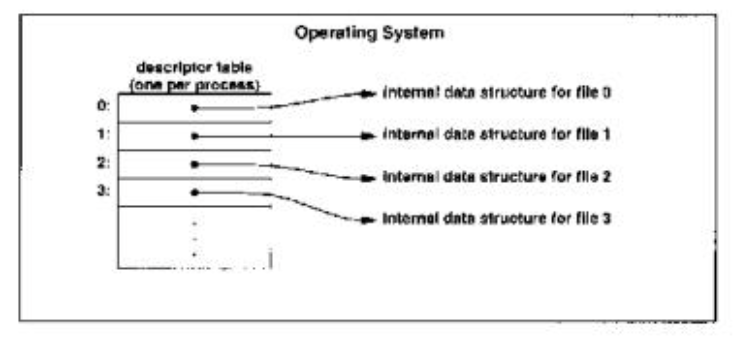
Fig : socket and file descriptor
b. System Data Structure For Socket
A pointer to the corresponding data structure is returned after calling socket () (with the arguments PF INET and SOCK STREAM).
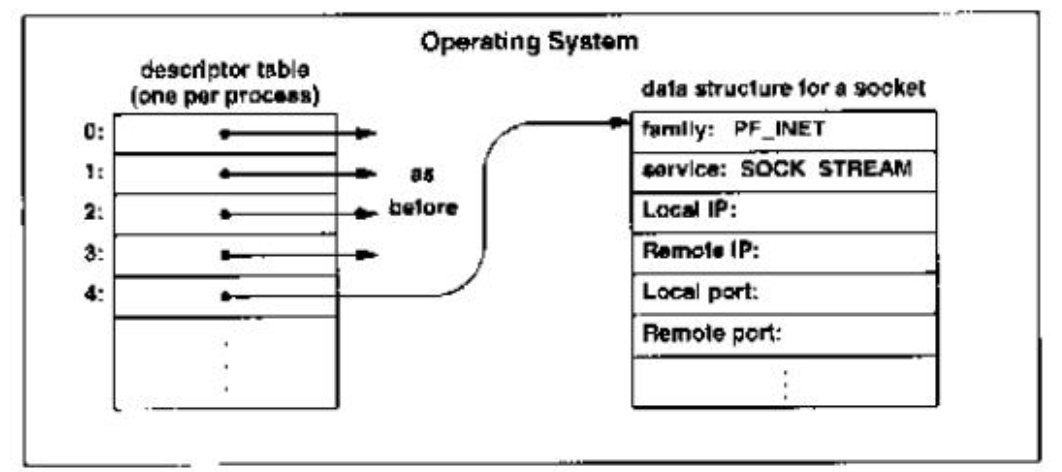
Fig : system data structure for socket
c. Using Socket
● On a passive socket, a server is waiting for an incoming connection.
● On an active socket, a client initiates a connection.
Major system calls used with sockets
Primary access calls for access to the underlying function.
Routines of services that assist the programmer.
● The Socket Call : In the newly created socket, socket() returns a descriptor. Arguments: the protocol family (e.g. PF INET or PF INET6) and the protocol or form (e.g. Stream or datagram).
● The Connect Call : To create an active connection to a remote server, a client calls Connect.
Argument: The remote endpoint.
● The Write Call : Writing is used by clients and servers to transfer data over a TCP connection.
Arguments: The socket descriptor, the address of the data to be sent, the data length.
● The Read Call : Reading is used by clients and servers to receive (block) data over a TCP link or to receive a datagram sent by UDP.
Arguments: The socket descriptor, the address of the buffer, the buffer length.
● The Close Call : Deallocation of a socket (The reference count will be decremented when many processes share the socket; delocation when reference count = 0)
Argument: A socket descriptor.
● The Bind Call : To define a local endpoint, the app calls connect.
Arguments: The socket and endpoint descriptor (in sockaddr in or sockaddr in6).
● The Listen Call : To put the socket in passive mode, a connection-oriented server calls to listen and makes it ready to accept incoming connections.
Argument: Defines the queue size to be used for the socket in question.
● The Accept Call : A connection-oriented server calls for listening and makes it ready to accept incoming connections to place the socket in passive mode.
Argument: Specifies the queue size for the socket in question to be used.
Socket device data structure
● family (e.g., PF INET)
● service (e.g., SOCK STREAM)
● Local IP address, Local Port
● Remote IP address, Remote Port
Q.3) Describe concurrent processing in client-server software?
A3)
Concurrent processing in client-server software
● The power of client-server computing is concurrent processing, but it also makes it difficult to design and construct.
● Concurrency refers to simultaneous computation that is actual or evident.
● Simultaneous processing in :
● Multi-user computer system : Time-sharing, a way to move a single processor between multiple computations quickly enough to give the impression of simultaneous computations, may achieve competition.
● Multiprocessing : A design in which multiple processors simultaneously conduct multiple computations.
● Many client software operates simultaneously since the underlying operating system enables users to execute client programmes simultaneously.
● Users run client applications simultaneously on several computers.
● In any conventional application, an individual client programme operates; it does not directly control concurrency.
Concurrency in network :
Real or apparent simultaneous computation refers to the term concurrency. For example, through time-sharing, a design that arranges to move a single processor between multiple computations quickly enough to give the impression of simultaneous development, a multi-user computer system may achieve concurrency; or through multiprocessing, a design in which multiple processors perform multiple computations simultaneously.
For distributed computing, simultaneous processing is fundamental and exists in several ways. Many pairs of application programmes may communicate simultaneously between machines on a single network, sharing the network that interconnects them.
Concurrency in clients
Within a given computer scheme, rivalry may also occur. Multiple users on a time sharing scheme, for instance, can each invoke a client programme that interacts on another computer with an application. A file may be transferred by one user while another user performs a remote login session. From the point of view of a user, it appears that all client programmes proceed concurrently.
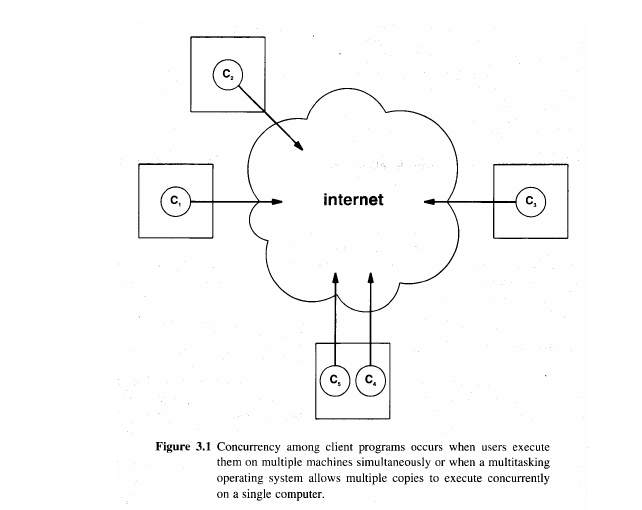
Fig : concurrency among client programs running on several machines.
In addition to the rivalry between clients on a single machine, the set will run simultaneously between all clients on a set of machines.
Usually, client software needs no special attention or effort on the part of the programmer to make it accessible simultaneously. Without regard to concurrent execution, the application programmer designs and builds each client programme; rivalry between multiple client programmes automatically arises because the operating system requires multiple users to simultaneously invoke a client each time.
Concurrency in server
Competition within a server requires significant effort, in comparison to concurrent client software.
Consider server operations that involve significant computation or communication to understand why concurrency is necessary. Think of a remote login server, for instance. It functions without competition, so it can handle only one remote login at a time. When a client contacts the server, subsequent requests must be ignored or refused by the server before the first user is done. Clearly, such a design restricts the server's utility and prohibits multiple remote users from simultaneously accessing a given computer.
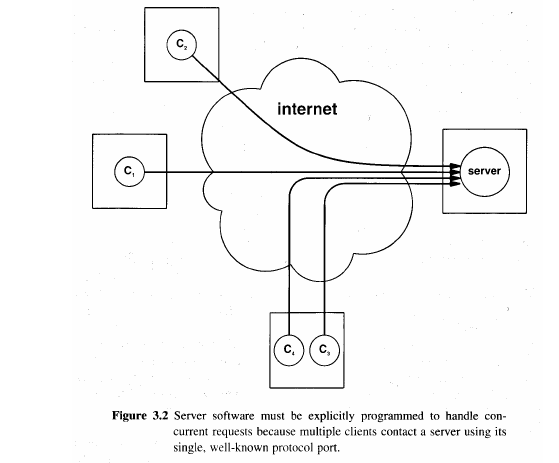
Fig : single server program must handle incoming requests concurrently.
Terminology and concept
● The Process concept : On a uniprocessor system = simultaneous execution, obviously. On a multiprocessor system = concurrent execution.
● Program Vs. Processes : Conventional Program = a piece of code executed at a time by one machine. A speed of code can be executed simultaneously by many processes, each process has an independent copy of the variables.
● Procedure calls : When many processes run a peace of code simultaneously, in a procedure-oriented language (like C). There is an individual run-time stack of procedure activation records for each process.
Executing new code
Context switch = OS stops running one process and moves to another context switch, creating an overhead processing to store the old process state and load the new one state. This overhead should be less than the gain of a server's implementation of concurrency.
Q.4) Difference between Connectionless Vs Connection-oriented server?
A4)
Connectionless Vs Connection-oriented server
● They must choose between two styles of interaction when programmers develop client-server software: a connectionless style or a connection-oriented style.
● TCP- Connection-oriented : This gives all the reliability needed to communicate over the internet.
- Verifies the information arrives, retransmits it automatically.
- Checksum computing over records.
- Delete the duplicated kit automatically.
● UDP - Protocol.connectionless user datagram. No assurances with respect to reliable delivery.
● Application programmes use UDP only if:
(1) The protocol for the programme states that UDP must be used.
(2) The programme depends on broadcast or multicast hardware, or multicast hardware.
(3) Computational overhead or delay required for TCP virtual circuits cannot be tolerated by the application.
● In summary : Beginners are strongly encouraged to use TCP when developing client-server apps, as it provides secure, connection-oriented communication. Programs only use UDP if redundancy is handled by the application protocol, hardware broadcast or multicast is needed by the application, or virtual circuit overhead may not be tolerated by the application.
Q.5) Describe algorithms and issues in client design ?
A5)
Algorithms and issue in Client design
Client Architecture
A consumer is easier than a server:
● It does not handle concurrent connections with multiple servers directly.
● Accessing ports or services does not require special privileges.
● Protection is not necessary to be implemented.
TCP or UDP may be used by a client.
Identifying the Location of a Server
● Having the domain name or IP address of the server as a constant
● The server needs to be identified by the user.
● Obtain the address of the server from a register.
● To find a suitable host, use a naming protocol.
Parsing an Address Argument
● Domain name of a computer: urd. Informatik. Hs-fulda.de
● corresponds to the IP address (dotted decimal notation):193.174.26.41
● A fully parameterized client software specifies the port of the service : urd.informatik.hs-fulda.de smtp
Or as a single argument: urd.informatik.hs-fulda.de:smtp
Looking Up a Domain Name
By converting the domain name or the dotted decimal notation to binary, a client specifies the IP address of the server using the sockaddr in structure:
● inet addr - An ASCII string is converted to binary using the dotted decimal notation.
● gethostbyname - This takes an ASCII string with a domain name and returns a binary IP address pointer to the host structure.
● inet pton() - An alphanumeric IPv4 or IPv6 address in binary (network order).
● inetntop() - An alphanumeric IPv4 or IPv6 binary address.
● gethostbyname - This takes an ASCII string with a domain name and returns a binary IPv4 or IPv6 address pointer to the host structure.
● gethostbyname2(myname, adrtype) - Same as above, but customised for IPv6.
● gethostbyaddr - This takes an IPv4 or IPv6 binary address and returns the string.
Struct hostent {
Char *h_name; /* official name of host */
Char **h_aliases; /* alias list */
Int h_addrtype; /* host address type */
Int h_length; /* length of address */
Char **h_addr_list; /* list of addresses */
};
#define h_addr h_addr_list[0] /* for backward compat */
Example:
Struct hostent *hptr;
Char *examplenam = „urd.informatik.hs-fulda.de“;
If ( hptr = gethostbyname ( examplenam ) ) {
/* IP address is now in hptr->h_addr */
} else {
/* error in name - handle it */
}
Switch (hptr->h_addrtype) {
Case AF_INET:
# ifdef AF_INET6
Case AF_INET6: .....
Allocating a Socket
Clients that use TCP:
#include <sys/types.h>
#include <sys/socket.h>
Int s; /* socket descriptor */
s = socket (PF_INET, SOCK_STREAM, 0); or
s = socket (PF_INET6, SOCK_STREAM, 0);
Connecting A TCP Socket To A Server
Connect() enables a link to be initiated by a TCP client (TCP 3-way handshake). The call does not return until the connection (return code 0) has been formed or the timeout is reached by TCP and fails (return code-1)
Retcode = connect (s, remaddr, remaddrlen)
(1) checks that socket s is correct and not already linked,
(2) fills the remote endpoint into a sockaddr in or sockaddr in6 style data structure handled by remaddr,
(3) chooses the local endpoint,
(4) initiates and waits for a TCP link if appropriate.
Closing a TCP Connection
● A Need for Partial Close : An application should gracefully terminate the connection and assign the socket after communication with the remote side. An application must know that all data has arrived from the remote side before terminating the connection.
● A Partial Close Operation : Shut down a TCP connection without allocating the socket in one direction:
Errcode = shutdown (s, direction);
After its last submission, a client issues a partial close. After its last response, the server then closes the connection.
Q.6) What do you mean by multiprotocol servers?
A6)
Multiprotocol Servers
The benefits of each protocol using a separate server:
● The same service, but with various transport protocols, such as TCP or UDP, can be used for the DAYTIME service.
● It is easy to maintain a single protocol server, but it results in duplication. The same service can provide the same piece of code with different protocols.
● Each single protocol server requires its own resources, resulting in process table entries and device resources being unnecessarily consumed.
Single thread multiprotocol
● Using asynchronous I/O for contact management.
● Open two sockets initially: one is for TCP and the other is for UDP.
● Wait until one of the two sockets is ready, then
- When TCP is ready, a new connection is made by the server using accept.
- If the UDP is ready, the server will support the client using the UDP service.
● If a UDP request is received by the server, it communicates with the client via sendto and recvfrom.
Process structure
At a given time, an iterative, multiprotocol server has a maximum of three sockets available. The third socket will be created for a given TCP-session by acceptance.
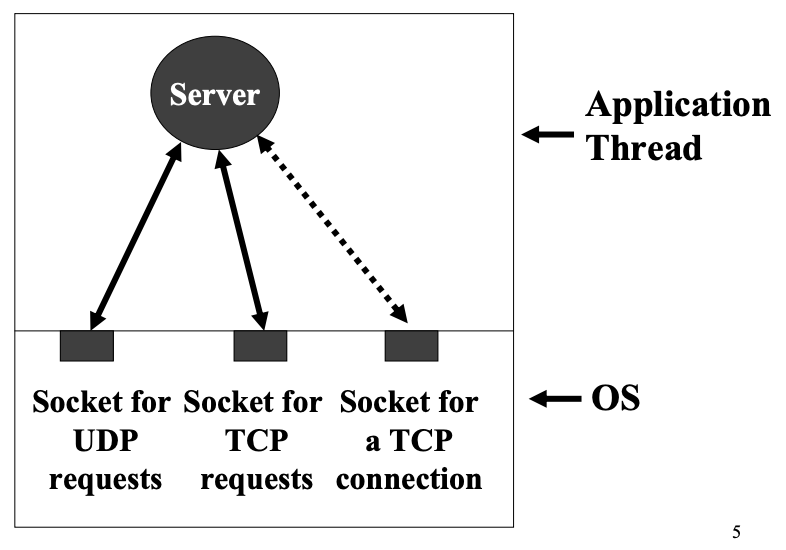
Fig : process structure
Concept of shared code
A single daytime procedure responds to requests for a specific service irrespective of whether requests arrive via UDP or TCP.
Concurrent Multiprotocol Server
For programmes that require additional computing.
● A new process can be created by the server to manage each TCP connection.
● Apparent concurrency can be enforced by the server.
Q.7) What do you mean by multiservice servers ?
A7)
Multiservice Servers
Consolidating server
The same incentive for servers with multiprotocol.
Higher chance of using a single, multi-service server. Why?
Consolidating multiple resources into a single method for the server reduces
- The number of dramatically executed processes
- The required total code.
A single transport protocol is used by most multi-service servers.
Connectionless, Multiservice
● One socket for UDP, One Operation
● Internal mapper port (socket ←→ procedure)
● Select uses it to wait for requests.
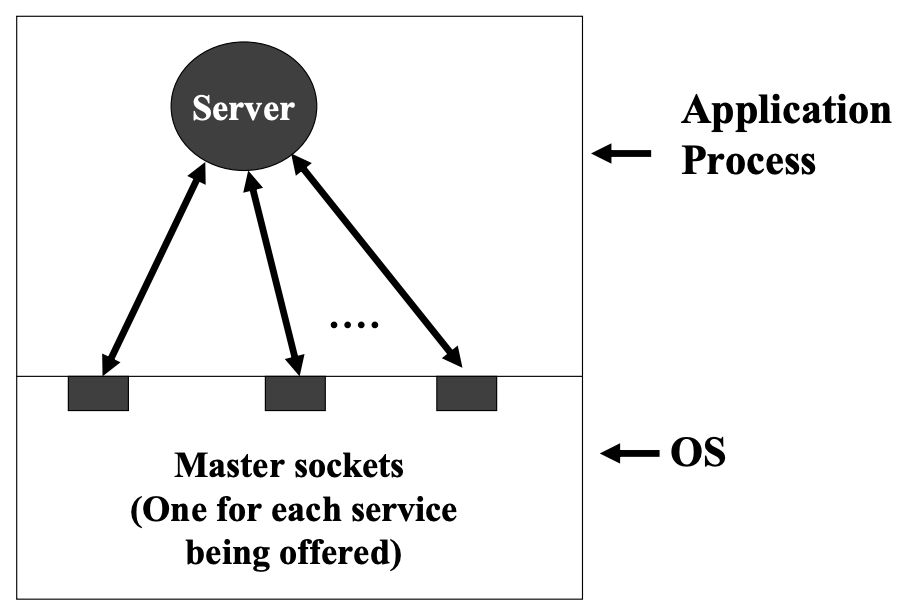
Fig : connection less multiservice
Connection - oriented Multiservice
● Multiservice servers may be configured to iteratively handle certain services and simultaneously handle other services.
● With several single-threaded procedures or a single-threaded operation, competition can be introduced.
● Process with multi-threads.
● Internal mapper port (socket ←→procedure)
● To wait for requests, it uses select
● To obtain a new relation, it calls accept
● To connect with the client, it generates (at most) one socket.
Concurrent connection - oriented multiservice
● One socket for TCP (master), one service
● Internal mapper port (socket ←→ procedure)
● To wait for requests, it uses select
● To obtain a new relation, it calls accept
● To connect with the client, it generates a slave process with one socket.
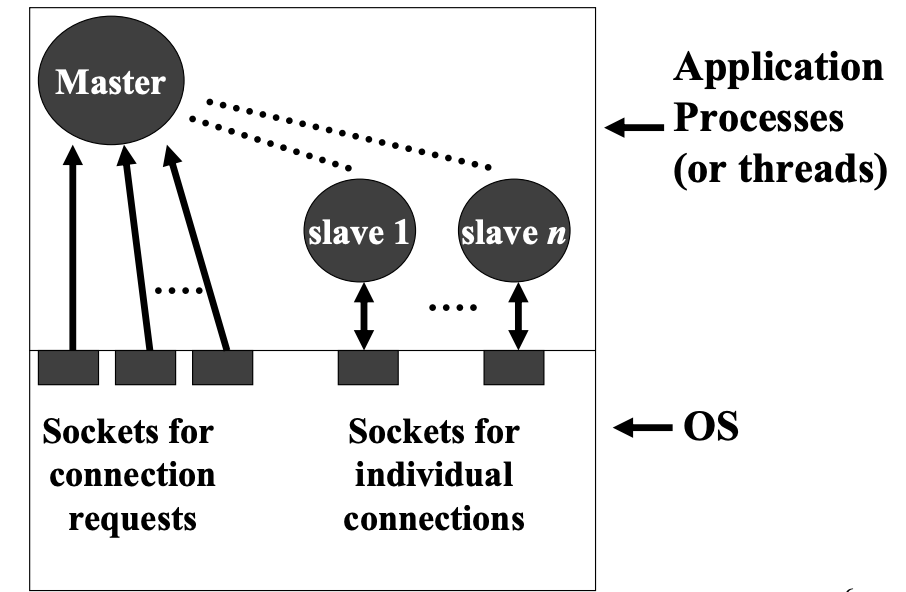
Fig : concurrent connection-oriented multiservice
Single process multiservice
● One TCP (master) socket, one private port mapper service (socket ⁇ process)
● To wait for requests, it uses select
● To obtain a new relation, it calls accept
● At least one socket is generated to connect with customers.
● It uses selection to wait for requests to read the data on the master sockets or on the slave sockets and submit the response.
Invoking separate programs
● Core Multi-Service Server Flaw-Inflexibility
- Changing small pieces of code allows the entire server to be recompiled.
- Preventing the recompilation of the server bothers clients
● How will this be overcome?
- Split a server into different components
- Then treat each component separately,
- Substitute with execve for the original code
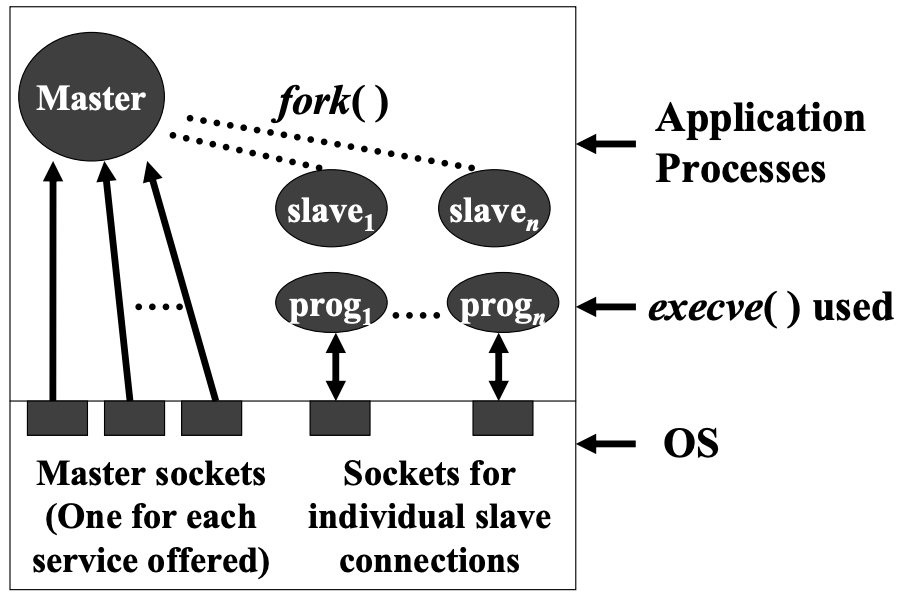
Fig : multiprocess , multiservice
Multiservice , Multiprotocol design
● As mentioned before, it is possible to build a multi-service server that has multi-protocol.
● Multi-service, multi-protocol server: mega server
- Open one or two sockets for each service initially,
- Master sockets are UDP or TCP referring to
- Use select device call to wait for the readiness of any socket
- If there is a ready UDP socket, read, process and respond
- If a TCP socket is ready, deal directly with the link.
Or build a method to manage it (iteratively) or (concurrently)
Server configuration
Static configuration from a configuration file when execution starts with the server process.
Dynamic configuration when the server is running: The administrator updates the configuration file and then notifies the server of the need for reconfiguration.
If the configuration file introduces a new service, the server opens new sockets to accept requests for them. This information is provided by a signal from a control socket.
If the configuration file deletes the service, the server gracefully closes the corresponding sockets.
Q.8) What are the advantages of concurrency?
A8)
The Advantages of concurrency
For servers :
● The response time observed can be enhanced.
● Might remove possible deadlocks
● Supports the configuration of the muliprotocol or multiservice
● Works on uniprocessors and systems with multiprocessors.
For clients:
● Asynchronously (handling of multiple tasks simultaneously)
● Easier to programme because they differentiate between functions
● Easier to retain end-extension services (modularity)
● Adjust parameters, inquire about client status or dynamically monitor processing.
Motivation
SEPARATE CONTROL FROM THE Usual PROCESSING For Exercising ControlAsynchrony.Concurrency allows the user to continue to communicate with the client while the client waits for a response. The user can assume a deadlock, repeat the request or gracefully terminate the communication.
Concurrent contact with multiple servers
● At the same time, the client will contact multiple servers and report when they receive a response from each of them.
● Example: Parallel calculation of the response times of multiple servers.
Implementing concurrent clients
Two strategies:
● The customer is split into two or more systems, each handling one role.
● The client consists of a single process that uses selection to asynchronously manage multiple I/O events.
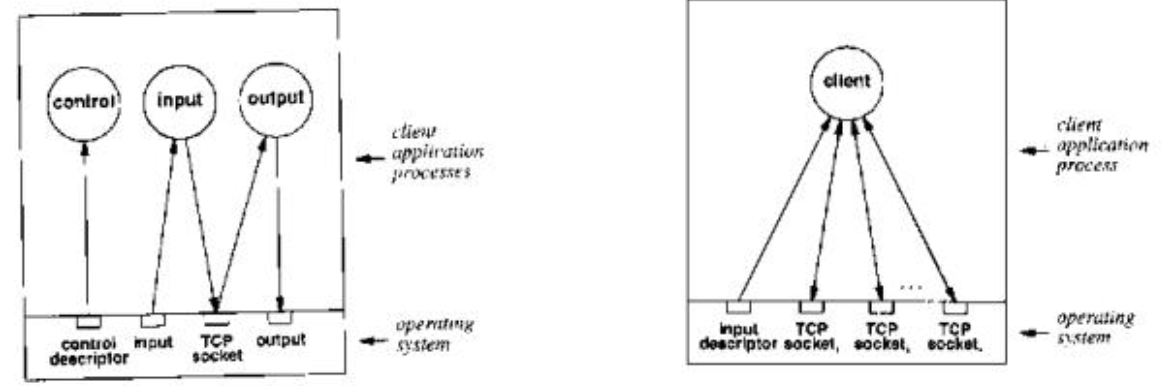
Fig : concurrent clients
Single process implementation
Many UNIX implementations do not allow memory to be shared by different processes. Concurrent clients designed for UNIX implement the single-process algorithm (# 8.5) The clients use select to wait for the readiness of one of their descriptors.
Execution of the concurrent client
● TCPtecho accepts as arguments several system names. It opens a TCP link for each computer, sends account characters, reads the bytes it receives, and prints the necessary total time.
● it can be used for the calculation of current output.
% TCPtecho oak apple elm
Oak: 602 ms
Apple: 4921 ms
Elm: 11110 ms
Concurrency in the example code
Advantages :
● Get a more detailed calculation of the necessary time.
● Measurement by parallel.
Q.9) What do you mean by unix internet super server ?
A9)
Unix Internet Super server (inetd)
● "A "SuperServer" is given by most Unix systems that solves the problem:
● Executes the startup code that a bunch of servers need.
● Waits for incoming requests for the same server package.
● When a request arrives, the correct server starts up and provides the request.
Unix Super Server, inetd
● The super server, inetd, manages a wide range of resources on most UNIX systems, including LINUX,
● INETD motivation-Less consumption of energy
● Configurable Dynamically
- The newer Linux versions replace inetd with xinetd
● When the SIGHUP (-1) signal is received by a configuration file with six or more fields, Inetd is dynamically reconfigurable:
Service name - (must appear in the service database of the system)
Type of socket - (e.g. Stream or dgram)
Protocol - (e.g. Tcp or udp)
Wait status - wait for concurrent request handling
Userid - sequentially or no wait user id in which the service can run (e.g. Root)
Server programme - the programme name or the internal name of the code within the programme.
Inetd arguments - reasons for the service to be passed on.
Steps:-
* For each new operation, Build a master socket
* Consider a Service Port Number
* Handling consumer demands iteratively or concurrently,
* Execute the programme for operation.
Inetd children
● Inetd forks a child that executes and manages the request and exits with the real server software.
● After the fork the inetd process remains running
● The infant device closes all unwanted sockets.
● Inetd uses the wait status to decide how to proceed once a service has been started.
/ etc/inted.conf
Inetd reads a configuration file listing all the resources it wants to manage.
For each service specified, inetd generates a socket and adds the socket to fd set
Enabled to pick ().
Inetd service specification
INETD wants to know about each service:
● The port number and protocol for transport.
● Wait/wait flag now.
● The login name should be run as a method.
● True server programme pathname.
● Server software command line arguments.
Wait /nowait
● Specifying WAIT means that once the child (the actual server) has terminated, inetd does not check for new clients for the service.
● Currently, TCP servers define Nowaita
- this implies that inetd is able to start several copies of the TCP server software
- provides competition
UDP & Wait /nowait
● Most UDP services run on inetd, which is told to wait until the child server is dead.
● What's going to happen if:
- inetd did not wait for the death of a UDP server
- inetd gets a time slice until a request datagram is read by the real server?
Super inetd
There are some variants of inetd that have server code to manage basic services such as :
Echo server,
Daytime server,
Chargen server,
…..
Xinetd
Xinetd can be used for the purpose of
● Giving access only to individual hosts
● Denying access to unique hosts
● Provide access at a certain time to a service
Etc..
The configure file is /etc/xinetd.conf
Q.10) What is the Algorithm and Issues In Server Software Design ?
A10)
Algorithm and Issues In Server Software Design
The Conceptual Server Algorithm
"Problem: equal scheduling of "large" and "small" client requests. Creates a socket, connects the socket to the (well-known) port on which it wants to accept requests, waits for requests from a client, processes the request, formulates the reply, and sends the reply back.
Concurrent Vs. Iterative Servers
Iterative: One request at a time is handled by the server.
- It is simpler to build, but can result in poor customer performance.
Concurrent: Several requests are treated at one time by the Server. This does not apply to whether there are several concurrent processes used in the underlying implementation.
- A single process can concurrently use multiple asynchronous I/Os.
- It is hard to build, but offers better performance.
Connection - Oriented Severs
Advantages:
It is easier to programme connection-oriented communication, since TCP automatically manages packet loss, errors and out-of-order delivery.
Disadvantages:
● Each link needs a separate socket. An overhead is generated by socket allocation and link management.
● Because of the overhead of the 3-way handshake used to build and terminate connections, TCP is costly compared to UDP.
● Most significantly, a crashed client leaves allocated server resources (structures of data, buffers, etc.) that cannot be retrieved. The server will run out of resources if clients or connections crash repeatedly.
Connectionless Servers
Benefits:
No issue with resource depletion and multicast connectivity support.
Disadvantages:
Allows the application protocol to provide stability (e.g. The programmer). Reliability is extremely difficult through time-outs and retransmission, since adaptive retransmission is required for end-to-end delays that can change rapidly. This requires extensive expertise.
Optimizing Stateless Servers
Example of the stateless file server: Each request is treated independently: open the file, search for location, read bytes, submit the data, and close the file.
Problems: The overhead for opening and closing files is high, with only a small number of bytes per request, and clients sequentially prefer to read files. RAM reads are, in comparison, many orders of magnitude faster than disc reads.
Solutions: IP address + port is used as an index to a large RAM buffer for file data → caching for subsequent access to an entire file block, without opening the file.
New issues: The server retains state information (IP address + port + file name), which can consume resources if clients regularly crash and reboot or if messages are duplicated or delayed by the underlying network.
Four Basic Types of Servers
- Iterative , Connectionless
- Iterative , Connection - Oriented
- Concurrent , Connectionless
- Concurrent , Connection - Oriented
Iterative Server Algorithm
It is simple to design, programme, debug, and change the server. If this option provides the anticipated load with a sufficiently rapid response, the programmer must investigate. For simple services using a connectionless protocol, this is typically the case.
Iterative , connection - oriented server algorithm
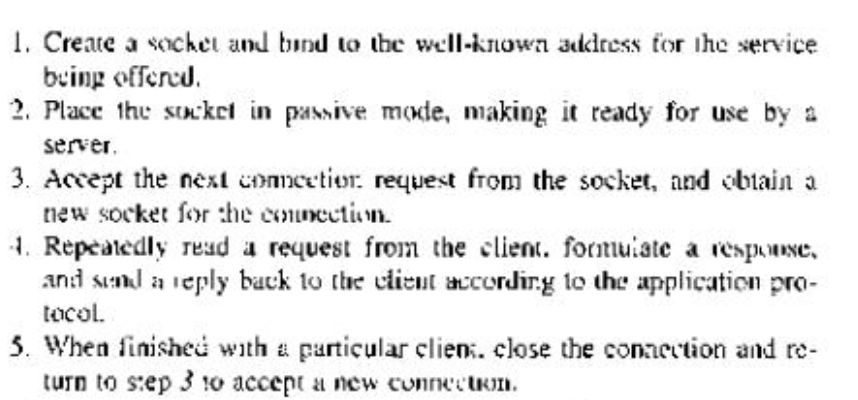
Concurrent Server Algorithm
Quicker reaction to multiple clients if:
● Forming an answer requires substantial I/O
● The processing time varies greatly.
● The server operates a multi-processor computer.
Master and Slave Process
Several processes are used by most parallel servers:
● The master server process waits for requests and generates a slave server process to handle each request.
● Each slave process processes, sends a response and exits the request.
Concurrent , Connectionless Server Algorithm
Since it is costly to build processes, few connectionless servers have simultaneous implementations.
Algorithm :
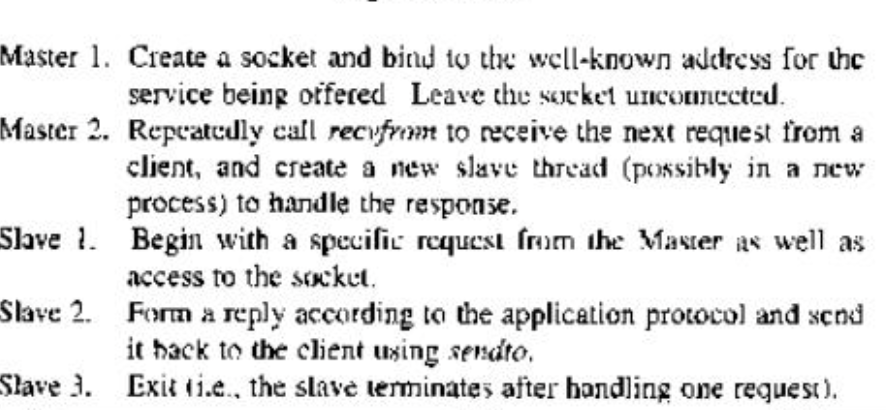
Concurrent , Connection - Oriented Server Algorithm
The servers maintain continuity between links rather than between individual requests.
Algorithm
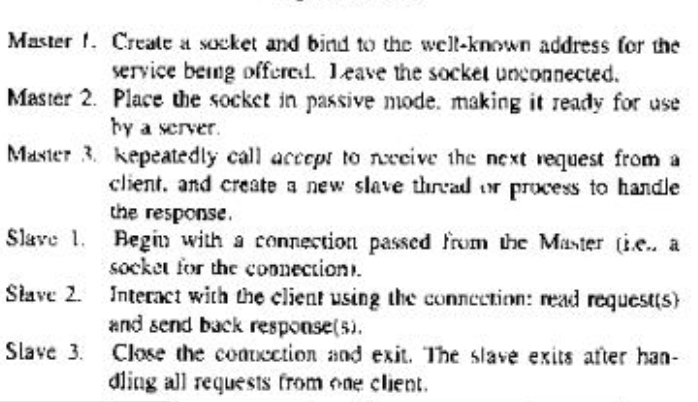
Using Seperate Program as Slave
In cases where separate programmes for master and slave processes are used, the master calls are executed after the fork call.