Unit - 1
Functions and Pointer
Q1) Explain function?
A1) Similar to other languages C language also provides the facility of function. Function is the block of code which is used to perform a specific task. In c language the complete program is composed of function.
Functions are useful to divide c programs into smaller modules. Programmer can invoked these modules anywhere inside c program for any number of times.
Functions are used to increase readability of the code. Size of program can be reduce by using functions. By using function, programmer can divide complex tasks into smaller manageable tasks and test them independently before using them together.
Functions of C language are defined with the type of function. The type of functions indicates the data type of value which will return by function. In order to use function in the program, initially programmer have to inform compiler about the function. This is also called as defining a function.
In C programme all the function definition present outside the main function. All function need to be declared and defined before use. Function declaration requires function name, argument list, and return type.
Return TypeFunction name (Argument list)
{
Statement 1;
Statement 2;
…………...
Statement n;
}
Q2) Explain User Defined Functions?
A2) User Defined Functions
User defined function is the block of code written by programmer to perform a particular task. As compiler doesn’t have any idea about the user define function so programmer has to define and declare these functions inside the program body. Programmer can define these function outside the main function but declaration of user define function should present in main function only. Whenever compiler executes function call (function declaration) then compiler shift the flow of program execution to the definition part of user define function.
Example
#include <stdio.h>
#include<conio.h>
Int add (int x, int y)
{
int sum;
sum = x + y;
return (sum);
}
Main ()
{
int a,b,c;
a = 15;
b = 25;
c = add(a,b);
Printf ("\n Addition is %d ", c);
}
Output:
Addition is 40
There are two ways to pass the parameters to the function
- Parameter Passing by value - In this mechanism, the value of the parameter is passed while calling the function.
- Parameter Passing by reference - In this mechanism, the address of the parameter is passed while calling the function.
Q3) Explain parameter passing in functions?
A3) This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q4) Write a program to swap two numbers using call by value and reference.
A4) Program
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q5) Explain how you pass arrays to functions?
A5) Array is a data structure which stores the collection of similar types of element in consecutive memory locations. Indexing of array always start with ‘0’ where as non-graphical variable ‘\0’ indicates the end of array.
Syntax for declaring array is
Data type array name[Maximum size];
● Data types are used to define type of element in an array. Data types are also useful in finding the size of total memory locations allocated for the array.
● All the rules of defining name of variable are also applicable for the array name.
● Maximum size indicates the total number of maximum elements that array can hold.
● Total memory allocated for the array is equal to the memory required to store one element of the array multiply by the total element in the array.
● In array, memory allocation is done at the time of declaration of an array.
Example:
Sr. No. | Instructions | Description |
#include<stdio.h> | Header file included | |
2. | #include<conio.h> | Header file included |
3. | Void main() | Execution of program begins |
4. | { | Memory is allocated for variable i,n and array a |
5. | Int i,n,a[10]; | |
6. | Clrscr(); | Clear the output of previous screen |
7. | Printf("enter a number"); | Print “enter a number” |
8. | Scanf("%d",&n); | Input value is stored at the addres of variable n |
9. | For(i=0;i<=10;i++) | For loop started from value of i=0 to i=10 |
10. | { | Compound statement(scope of for loop starts) |
11. | a[i]=n*i; | Result of multiplication of n and I is stored at the ith location of array ieasi=0 so it is stores at first location. |
12. | Printf("\n %d",a[i]); | Value of ith location of the array is printed |
13. | } | Compound statement(scope of for loop ends) |
14. | Printf("\n first element in array is %d",a[0]); | Value of first element of the array is printed |
15. | Printf("\n fifth element in array is %d",a[4]); | Value of fifth element of the array is printed |
16. | Printf("\n tenth element in array is %d",a[9]); | Value of tenth element of the array is printed |
17. | Getch(); | Used to hold the output screen |
18. | } | Indicates end of scope of main function |
This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q6) Write a program to find the sum of N natural numbers using functions?
A6) /* C Program to find Sum of N Numbers using Functions */
#include<stdio.h>
Int Sum_Of_Natural_Numbers(int Number);
Int main()
{
int Number, i, Sum = 0;
Printf("\nPlease Enter any Integer Value\n");
Scanf("%d", &Number);
Sum = Sum_Of_Natural_Numbers(Number);
Printf("Sum of Natural Numbers = %d", Sum);
return 0;
}
Int Sum_Of_Natural_Numbers(int Number)
{
Int i, Sum = 0;
If (Number == 0)
{
Return Number;
}
Else
{
Return (Number * (Number + 1) / 2);
}
}
Output:
Please enter any Integer Value
5
Sum of Natural Numbers = 15
Q7) Write a program to find the GCD of a number using functions?
A7) Program
#include<stdio.h>
Int main() {
int a, b;
Printf("Enter 2 numbers:");
Scanf("%d%d", & a, & b);
Printf("Greatest Common Divisor is %d", gcd(a, b));
return 0;
}
Int gcd(long a, long b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
Output:
Enter two positive integers: 81
153
GCD = 9
Q8) Write a program to find the factorial of a number using functions?
A8) Program
#include<stdio.h>
#include<math.h>
Void main()
{
//clrscr();
Printf("Enter a Number to Find Factorial: ");
Fact();
Getch();
}
Fact()
{
int i,fact=1,n;
Scanf("%d",&n);
For(i=1; i<=n; i++)
{
fact=fact*i;
}
Printf("\nFactorial of a Given Number is: %d ",fact);
return fact;
}
OUTPUT:
Enter a Number to Find Factorial: 5
Factorial of a Given Number is: 120
Q9) Write a program to check if the given number is prime or not?
A9) Program
#include <conio.h>
Void main()
{
Int num,res=0;
Clrscr();
Printf("\nENTER A NUMBER: ");
Scanf("%d",&num);
Res=prime(num);
If(res==0)
Printf("\n%d IS A PRIME NUMBER",num);
Else
Printf("\n%d IS NOT A PRIME NUMBER",num);
Getch();
}
Int prime(int n)
{
Int i;
For(i=2;i<=n/2;i++)
{
If(n%i!=0)
Continue;
Else
Return 1;
}
Return 0;
}
Output
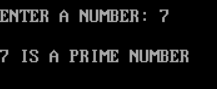
Q10) Write a program to convert a binary number to its equivalent decimal number?
A10) Program
#include <stdio.h>
Void main()
{
Int num, binary_val, decimal_val = 0, base = 1, rem;
Printf("Enter a binary number(1s and 0s) \n");
Scanf("%d", &num); /* maximum five digits */
Binary_val = num;
while (num> 0)
{
rem = num % 10;
Decimal_val = decimal_val + rem * base;
Num = num / 10 ;
base = base * 2;
}
Printf("The Binary number is = %d \n", binary_val);
Printf("Its decimal equivalent is = %d \n", decimal_val);
}
Enter a binary number(1s and 0s)
10101001
The Binary number is = 10101001
Its decimal equivalent is = 169
Q11) Define recursion?
A11) Recursion
In programming languages, if a program allows you to call a function inside the same function, then it is called a recursive call of the function.
Void recursion() {
Recursion(); /* function calls itself */
}
Int main() {
recursion();
}
The C programming language supports recursion, i.e., a function to call itself. But while using recursion, programmers has to be careful to define an exit condition from the function, otherwise it will go into an infinite loop.
Recursive functions are useful to solve many mathematical problems, such as calculating the factorial of a number, generating Fibonacci series, etc.
The following example calculates the factorial of a given number using a recursive function −
#include <stdio.h>
Unsigned long long int factorial(unsigned int i) {
if(i<= 1) {
return 1;
}
return i * factorial(i - 1);
}
Int main() {
int i = 12;
Printf("Factorial of %d is %d\n", i, factorial(i));
return 0;
}
When the above code is compiled and executed, it produces the following result–
Factorial of 12 is 479001600
The following example generates the Fibonacci series for a given number using a recursive function −
#include <stdio.h>
Int fibonacci(int i) {
if(i == 0) {
return 0;
}
if(i == 1) {
return 1;
}
return fibonacci(i-1) + fibonacci(i-2);
}
Int main() {
int i;
for (i = 0; i< 10; i++) {
Printf("%d\t\n", fibonacci(i));
}
return 0;
}
When the above code is compiled and executed, it produces the following result−
0
1
1
2
3
5
8
13
21
34
Q12) What is a pointer? How do you declare pointers?
A12) Pointer
One of the vital and heavily used feature ‘C’ is pointer. Most of the other programming languages also support pointers but only few of them use it freely. Support pointers but only few of them use it freely.
When we declare any variable in C language, there are three things associated with that variable.
- Data type of variable: Data type defines the type of data that variable can hold. Data type tells compiler about the amount of memory allocate to the variable.
- Address of Variable: Address of variable represent the exact address of memory location which is allocated to variable.
- Value of variable: It is the value of variable which is store at the address of memory location allocated to variable.
Example: int n = 5;
In the above example ‘int’ is the data type which tells compiler to allocate 2 bytes of memory to variable ‘n’.
Once the variable is declare compiler allocated two bytes of memory to variable ‘n’. Suppose the address of that memory location is 1020. At the address of memory location 1020 the value 5 is store.
Memory map for above declaration is as follows.
Use of &,/and ‘*’ operator
Consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c,
Printf (“Enter two numbers”);
Scanf (“%d%d”, &a,&b);
c=a+b;
Printf(“/n Addition is %d”, C);
Printf(“/n Address of variable C is %d”, &C);
Getch ();
}
Above program is the program for addition of two numbers in ‘C’ language. In the seventh instruction of above program we used operator ‘%’ and ‘&’ ‘%d’ is the access specifier which tells compiler to take integer value as input whereas. ‘&a’ tells compile to store the taken value at the address of variable ‘a’.
In the ninth instruction compiler will print me value of ‘C’ so the output of 9th instruction is as follows.
Addition is 5[Note : considering a = 2 and b = 3]
In the tenth instruction compiler will print me address of variable C.
So the output of length instruction is as follows. Address of variable C is 1020.
Now consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Printf(“Enter two numbers”);
Scanf(“%d %d”, & a, &b);
c=a+b;
Printf(“\n Addition is %d”,C);
Printf(“\n Address of variable C is %d”,&C);
Printf(‘\n value of variable C is %d”, *(&C));
Getch();
}
Now, we all are clear about the output of instruction 9 and 10.
In the 11th instruction, operator *and & is used. ‘*’ operator tells compile to pickup the value which is stored at the address of variable C. So the output of 11th instruction is as follow. Value of variable C is 5 [considering a = 24 b = 3;]
Q13) Write a program to add two numbers using pointers?
A13) Program
#include <stdio.h>
Int main()
{
int first, second, *p, *q, sum;
Printf("Enter two integers to add\n");
Scanf("%d%d", &first, &second);
p = &first;
q = &second;
sum = *p + *q;
Printf("Sum of the numbers = %d\n", sum);
return 0;
}
Output:
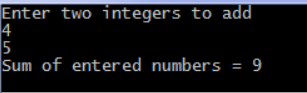
Q14) What is a pointer variable and give example?
A14) Pointers variable
Pointers in C language is a variable that stores/points the address of another variable. A Pointer in C is used to allocate memory dynamically i.e. at run time. The pointer variable might be belonging to any of the data type such as int, float, char, double, short etc.
Pointer Syntax: data_type *var_name; Example : int *p; char *p;
Where, * is used to denote that “p” is pointer variable and not a normal variable.
Normal variable stores the value whereas pointer variable stores the address of the variable.
The content of the C pointer always be a whole number i.e. address.
Always C pointer is initialized to null, i.e. int *p = null.
The value of null pointer is 0.
& symbol is used to get the address of the variable.
* symbol is used to get the value of the variable that the pointer is pointing to.
If a pointer in C is assigned to NULL, it means it is pointing to nothing.
Two pointers can be subtracted to know how many elements are available between these two pointers.
But, Pointer addition, multiplication, division are not allowed.
The size of any pointer is 2 byte (for 16 bit compiler).
Example:
#include <stdio.h>
Int main()
{
int *ptr, q;
q = 50;
/* address of q is assigned to ptr */
ptr = &q;
/* display q's value using ptr variable */
printf("%d", *ptr);
return 0;
}
Output:
1
2
3
4
5
6
7
8
9
10
11
Q15) What is Pointer Arithmetic?
A15) Pointer arithmetic
Pointer arithmetic is slightly different from arithmetic we normally use in our daily life. The only valid arithmetic operations applicable on pointers are:
- Addition of integer to a pointer
- Subtraction of integer to a pointer
- Subtracting two pointers of the same type
The pointer arithmetic is performed relative to the base type of the pointer. For example, if we have an integer pointer ip which contains address 1000, then on incrementing it by 1, we will get 1004 (i.e 1000 + 1 * 4) instead of 1001 because the size of the int data type is 4 bytes. If we had been using a system where the size of int is 2 bytes then we would get 1002 ( i.e 1000 + 1 * 2 ).
Similarly, on decrementing it we will get 996 (i.e 1000 - 1 * 4) instead of 999.
So, the expression ip + 4 will point to the address 1016 (i.e 1000 + 4 * 4).
Let's take some more examples.
1
2
3
Int i = 12, *ip = &i;
Double d = 2.3, *dp = &d;
Char ch = 'a', *cp = &ch;
Suppose the address of i, d and ch are 1000, 2000, 3000 respectively, therefore ip, dp and cp are at 1000, 2000, 3000 initially.
Q16) Explain in detail Dynamic memory allocation?
A16) Dynamic memory allocation
The concept of dynamic memory allocation in c language enables the C programmer to allocate memory at runtime. Dynamic memory allocation in c language is possible by 4 functions of stdlib.h header file.
- Malloc()
- Calloc()
- Realloc()
- Free()
Before learning above functions, let's understand the difference between static memory allocation and dynamic memory allocation.
Static memory allocation
Dynamic memory allocation
Memory is allocated at compile time.
Memory is allocated at run time.
Memory can't be increased while executing program.
Memory can be increased while executing program.
Used in array.
Used in linked list.
Now let's have a quick look at the methods used for dynamic memory allocation.
Malloc()
Allocates single block of requested memory.
Calloc()
Allocates multiple block of requested memory.
Realloc()
Reallocates the memory occupied by malloc() or calloc() functions.
Free()
Frees the dynamically allocated memory.
Malloc() function in C
The malloc() function allocates single block of requested memory.
It doesn't initialize memory at execution time, so it has garbage value initially.
It returns NULL if memory is not sufficient.
The syntax of malloc() function is given below:
Ptr=(cast-type*)malloc(byte-size)
Let's see the example of malloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)malloc(n*sizeof(int)); //memory allocated using malloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Calloc() function in C
The calloc() function allocates multiple block of requested memory.
It initially initialize all bytes to zero.
It returns NULL if memory is not sufficient.
The syntax of calloc() function is given below:
Ptr=(cast-type*)calloc(number, byte-size)
Let's see the example of calloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Realloc() function in C
If memory is not sufficient for malloc() or calloc(), you can reallocate the memory by realloc() function. In short, it changes the memory size.
Let's see the syntax of realloc() function.
Ptr=realloc(ptr, new-size)
Free() function in C
The memory occupied by malloc() or calloc() functions must be released by calling free() function. Otherwise, it will consume memory until program exit.
Let's see the syntax of free() function.
Free(ptr)
Q17) Write the difference between the local and global variable?
A17) Difference between Global variable and Local variable
Global Variable | Local Variable |
Outside of all the function blocks, global variables are declared. | Within a function block, local variables are declared. |
Throughout the programme, the scope is maintained. | The scope is restricted, and they can only be used in the function in which they are declared. |
Any modification to a global variable has an impact on the entire programme, regardless of where it is utilised. | Any changes to the local variable have no effect on the program's other functions.
|
A global variable is present in the programme for the duration of its execution. | After the function is executed, a local variable is created, and when the function is finished, the variable is destroyed. |
It can be accessed from anywhere in the programme using any of the program's functionalities. | It is only accessible through the function statements in which it is declared, not through other functions. |
If a global variable isn't initialised, it defaults to zero. | If a local variable isn't initialised, it defaults to a garbage value. |
The data segment of memory stores global variables. | Local variables are kept in memory on a stack. |
We can't use the same name for several variables. | Variables having the same name can be declared in several functions. |
Q18) Write short notes on local variable?
A18) Local variables
Local variables are variables that are declared within a function or block. They can only be utilised by statements that are included within that function or code block. Functions outside of their own are unaware of local variables. The following example demonstrates the use of local variables. The variables a, b, and c are all local to the function main().
#include <stdio.h>
Int main () {
/* local variable declaration */
int a, b;
int c;
/* actual initialization */
a = 10;
b = 20;
c = a + b;
printf ("value of a = %d, b = %d and c = %d\n", a, b, c);
return 0;
}
Advantages of Local Variable
● Because a local variable's name is only recognised by the function in which it is declared, it can be used in several functions with the same name.
● Local variables only require memory for the duration of the function; after that, the same memory address can be reused.
Disadvantages of Local Variables
● The local variable's scope is restricted to its function and it cannot be used by other functions.
● The local variable is not allowed to share data.
Q19) What do you mean by global variable?
A19) Global variables
Global variables are variables that are defined outside of a function, usually at the start of a programme. Global variables maintain their values throughout the life of your programme and can be accessed from any of the program's functions.
Any function has access to a global variable. That is, once a global variable is declared, it is available for use throughout your whole programme. The following programme demonstrates the use of global variables in a programme.
#include <stdio.h>
/* global variable declaration */
Int g;
Int main () {
/* local variable declaration */
int a, b;
/* actual initialization */
a = 10;
b = 20;
g = a + b;
printf ("value of a = %d, b = %d and g = %d\n", a, b, g);
return 0;
}
Local and global variables in a programme can have the same name, but the value of a local variable inside a function takes precedence. Here's an illustration:
Include <stdio.h>
/* global variable declaration */
Int g = 20;
Int main () {
/* local variable declaration */
int g = 10;
printf ("value of g = %d\n", g);
return 0;
}
When the preceding code is compiled and run, the following result is obtained:
Value of g = 10
Advantages of Global Variable
● Global variables can be accessible by all of the program's functions.
● It is just necessary to make a single declaration.
● If all of the functions are accessing the same data, this is quite handy.
Disadvantages of Global Variable
● Because it can be utilised by any function in the programme, the value of a global variable can be modified by accident.
● If we employ a big number of global variables, there is a good possibility that the programme may generate errors.
Q20) Describe storage classes with example?
A20) Storage classes
Within a C programme, a storage class defines the scope (visibility) and lifetime of variables and/or functions. They come before the type they modify. In a C programme, there are four different storage classes:
● auto
● register
● static
● extern
Auto
At runtime, memory is automatically allocated to automated variables.
The automated variables' visibility is confined to the block in which they are defined. The automated variables' scope is constrained to the block in which they are defined. By default, the automated variables are set to garbage.
When you depart the block, the memory allotted to automatic variables is released. The auto keyword is used to define automatic variables.
By default, every local variable in C is automatic.
For all local variables, the auto storage class is the default storage class.
{
int mount;
auto int month;
}
The preceding example declares two variables in the same storage class. The keyword 'auto' can only be used within functions, i.e. within local variables.
Example:
#include <stdio.h>
Int main()
{
Int a; //auto
Char b;
Float c;
Printf("%d %c %f",a,b,c); // printing initial default value of automatic variables a, b, and c.
Return 0;
}
Output:
Garbage garbage garbage
Register
The memory is allocated to the CPU registers by the variables declared as the registers, depending on the size of the memory remaining in the CPU.
We can't dereference the register variables, which means we can't use & operator for them. The register variables have a faster access time than the automatic variables. The register local variables have a default value of 0.
The variable that should be saved in the CPU register is denoted by the register keyword. Is it, nevertheless, a compiler? The variables can be stored in the register at the user's discretion. We can store pointers in registers, which means that a register can hold the address of a variable. Because we can't use more than one storage specifier for the same variable, static variables can't be stored in the register.
{
register int miles;
}
Example:
#include <stdio.h>
Int main()
{
Register int a; // variable a is allocated memory in the CPU register. The initial default value of a is 0.
Printf("%d",a);
}
Output:
0
Static
The static storage class tells the compiler to keep a local variable alive for the duration of the programme, rather than creating and destroying it every time it enters and exits scope. As a result, making local variables static ensures that their values are preserved between function calls.
The static modifier can be used on global variables as well. When you do this, the scope of that variable is limited to the file in which it is declared.
When the keyword static is applied on a global variable in C programming, just one copy of that member is shared by all objects in that class.
#include <stdio.h>
/* function declaration */
Void func(void);
Static int count = 5; /* global variable */
Main() {
while(count--) {
func();
}
return 0;
}
/* function definition */
Void func( void ) {
static int i = 5; /* local static variable */
i++;
printf("i is %d and count is %d\n", i, count);
}
When the above code is compiled and executed, it produces the following result −
i is 6 and count is 4
i is 7 and count is 3
i is 8 and count is 2
i is 9 and count is 1
i is 10 and count is 0
Extern
The extern storage class is used to provide a reference to a global variable that is shared by all application files. The variable cannot be initialised when you use 'extern,' but it does direct the variable name to a previously established storage location.
When you have many files and specify a global variable or function that will be used in other files, extern is used in another file to provide a reference to the defined variable or function. To clarify, extern is a keyword that is used to declare a global variable or function in a different file.
Example:
#include <stdio.h>
Int main()
{
Extern int a;
Printf("%d",a);
}
Output
Main.c:(.text+0x6): undefined reference to a'
Collect2: error: ld returned 1 exit status
Unit - 1
Functions and Pointer
Q1) Explain function?
A1) Similar to other languages C language also provides the facility of function. Function is the block of code which is used to perform a specific task. In c language the complete program is composed of function.
Functions are useful to divide c programs into smaller modules. Programmer can invoked these modules anywhere inside c program for any number of times.
Functions are used to increase readability of the code. Size of program can be reduce by using functions. By using function, programmer can divide complex tasks into smaller manageable tasks and test them independently before using them together.
Functions of C language are defined with the type of function. The type of functions indicates the data type of value which will return by function. In order to use function in the program, initially programmer have to inform compiler about the function. This is also called as defining a function.
In C programme all the function definition present outside the main function. All function need to be declared and defined before use. Function declaration requires function name, argument list, and return type.
Return TypeFunction name (Argument list)
{
Statement 1;
Statement 2;
…………...
Statement n;
}
Q2) Explain User Defined Functions?
A2) User Defined Functions
User defined function is the block of code written by programmer to perform a particular task. As compiler doesn’t have any idea about the user define function so programmer has to define and declare these functions inside the program body. Programmer can define these function outside the main function but declaration of user define function should present in main function only. Whenever compiler executes function call (function declaration) then compiler shift the flow of program execution to the definition part of user define function.
Example
#include <stdio.h>
#include<conio.h>
Int add (int x, int y)
{
int sum;
sum = x + y;
return (sum);
}
Main ()
{
int a,b,c;
a = 15;
b = 25;
c = add(a,b);
Printf ("\n Addition is %d ", c);
}
Output:
Addition is 40
There are two ways to pass the parameters to the function
- Parameter Passing by value - In this mechanism, the value of the parameter is passed while calling the function.
- Parameter Passing by reference - In this mechanism, the address of the parameter is passed while calling the function.
Q3) Explain parameter passing in functions?
A3) This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q4) Write a program to swap two numbers using call by value and reference.
A4) Program
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q5) Explain how you pass arrays to functions?
A5) Array is a data structure which stores the collection of similar types of element in consecutive memory locations. Indexing of array always start with ‘0’ where as non-graphical variable ‘\0’ indicates the end of array.
Syntax for declaring array is
Data type array name[Maximum size];
● Data types are used to define type of element in an array. Data types are also useful in finding the size of total memory locations allocated for the array.
● All the rules of defining name of variable are also applicable for the array name.
● Maximum size indicates the total number of maximum elements that array can hold.
● Total memory allocated for the array is equal to the memory required to store one element of the array multiply by the total element in the array.
● In array, memory allocation is done at the time of declaration of an array.
Example:
Sr. No. | Instructions | Description |
#include<stdio.h> | Header file included | |
2. | #include<conio.h> | Header file included |
3. | Void main() | Execution of program begins |
4. | { | Memory is allocated for variable i,n and array a |
5. | Int i,n,a[10]; | |
6. | Clrscr(); | Clear the output of previous screen |
7. | Printf("enter a number"); | Print “enter a number” |
8. | Scanf("%d",&n); | Input value is stored at the addres of variable n |
9. | For(i=0;i<=10;i++) | For loop started from value of i=0 to i=10 |
10. | { | Compound statement(scope of for loop starts) |
11. | a[i]=n*i; | Result of multiplication of n and I is stored at the ith location of array ieasi=0 so it is stores at first location. |
12. | Printf("\n %d",a[i]); | Value of ith location of the array is printed |
13. | } | Compound statement(scope of for loop ends) |
14. | Printf("\n first element in array is %d",a[0]); | Value of first element of the array is printed |
15. | Printf("\n fifth element in array is %d",a[4]); | Value of fifth element of the array is printed |
16. | Printf("\n tenth element in array is %d",a[9]); | Value of tenth element of the array is printed |
17. | Getch(); | Used to hold the output screen |
18. | } | Indicates end of scope of main function |
This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q6) Write a program to find the sum of N natural numbers using functions?
A6) /* C Program to find Sum of N Numbers using Functions */
#include<stdio.h>
Int Sum_Of_Natural_Numbers(int Number);
Int main()
{
int Number, i, Sum = 0;
Printf("\nPlease Enter any Integer Value\n");
Scanf("%d", &Number);
Sum = Sum_Of_Natural_Numbers(Number);
Printf("Sum of Natural Numbers = %d", Sum);
return 0;
}
Int Sum_Of_Natural_Numbers(int Number)
{
Int i, Sum = 0;
If (Number == 0)
{
Return Number;
}
Else
{
Return (Number * (Number + 1) / 2);
}
}
Output:
Please enter any Integer Value
5
Sum of Natural Numbers = 15
Q7) Write a program to find the GCD of a number using functions?
A7) Program
#include<stdio.h>
Int main() {
int a, b;
Printf("Enter 2 numbers:");
Scanf("%d%d", & a, & b);
Printf("Greatest Common Divisor is %d", gcd(a, b));
return 0;
}
Int gcd(long a, long b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
Output:
Enter two positive integers: 81
153
GCD = 9
Q8) Write a program to find the factorial of a number using functions?
A8) Program
#include<stdio.h>
#include<math.h>
Void main()
{
//clrscr();
Printf("Enter a Number to Find Factorial: ");
Fact();
Getch();
}
Fact()
{
int i,fact=1,n;
Scanf("%d",&n);
For(i=1; i<=n; i++)
{
fact=fact*i;
}
Printf("\nFactorial of a Given Number is: %d ",fact);
return fact;
}
OUTPUT:
Enter a Number to Find Factorial: 5
Factorial of a Given Number is: 120
Q9) Write a program to check if the given number is prime or not?
A9) Program
#include <conio.h>
Void main()
{
Int num,res=0;
Clrscr();
Printf("\nENTER A NUMBER: ");
Scanf("%d",&num);
Res=prime(num);
If(res==0)
Printf("\n%d IS A PRIME NUMBER",num);
Else
Printf("\n%d IS NOT A PRIME NUMBER",num);
Getch();
}
Int prime(int n)
{
Int i;
For(i=2;i<=n/2;i++)
{
If(n%i!=0)
Continue;
Else
Return 1;
}
Return 0;
}
Output
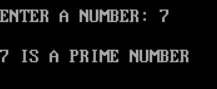
Q10) Write a program to convert a binary number to its equivalent decimal number?
A10) Program
#include <stdio.h>
Void main()
{
Int num, binary_val, decimal_val = 0, base = 1, rem;
Printf("Enter a binary number(1s and 0s) \n");
Scanf("%d", &num); /* maximum five digits */
Binary_val = num;
while (num> 0)
{
rem = num % 10;
Decimal_val = decimal_val + rem * base;
Num = num / 10 ;
base = base * 2;
}
Printf("The Binary number is = %d \n", binary_val);
Printf("Its decimal equivalent is = %d \n", decimal_val);
}
Enter a binary number(1s and 0s)
10101001
The Binary number is = 10101001
Its decimal equivalent is = 169
Q11) Define recursion?
A11) Recursion
In programming languages, if a program allows you to call a function inside the same function, then it is called a recursive call of the function.
Void recursion() {
Recursion(); /* function calls itself */
}
Int main() {
recursion();
}
The C programming language supports recursion, i.e., a function to call itself. But while using recursion, programmers has to be careful to define an exit condition from the function, otherwise it will go into an infinite loop.
Recursive functions are useful to solve many mathematical problems, such as calculating the factorial of a number, generating Fibonacci series, etc.
The following example calculates the factorial of a given number using a recursive function −
#include <stdio.h>
Unsigned long long int factorial(unsigned int i) {
if(i<= 1) {
return 1;
}
return i * factorial(i - 1);
}
Int main() {
int i = 12;
Printf("Factorial of %d is %d\n", i, factorial(i));
return 0;
}
When the above code is compiled and executed, it produces the following result–
Factorial of 12 is 479001600
The following example generates the Fibonacci series for a given number using a recursive function −
#include <stdio.h>
Int fibonacci(int i) {
if(i == 0) {
return 0;
}
if(i == 1) {
return 1;
}
return fibonacci(i-1) + fibonacci(i-2);
}
Int main() {
int i;
for (i = 0; i< 10; i++) {
Printf("%d\t\n", fibonacci(i));
}
return 0;
}
When the above code is compiled and executed, it produces the following result−
0
1
1
2
3
5
8
13
21
34
Q12) What is a pointer? How do you declare pointers?
A12) Pointer
One of the vital and heavily used feature ‘C’ is pointer. Most of the other programming languages also support pointers but only few of them use it freely. Support pointers but only few of them use it freely.
When we declare any variable in C language, there are three things associated with that variable.
- Data type of variable: Data type defines the type of data that variable can hold. Data type tells compiler about the amount of memory allocate to the variable.
- Address of Variable: Address of variable represent the exact address of memory location which is allocated to variable.
- Value of variable: It is the value of variable which is store at the address of memory location allocated to variable.
Example: int n = 5;
In the above example ‘int’ is the data type which tells compiler to allocate 2 bytes of memory to variable ‘n’.
Once the variable is declare compiler allocated two bytes of memory to variable ‘n’. Suppose the address of that memory location is 1020. At the address of memory location 1020 the value 5 is store.
Memory map for above declaration is as follows.
Use of &,/and ‘*’ operator
Consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c,
Printf (“Enter two numbers”);
Scanf (“%d%d”, &a,&b);
c=a+b;
Printf(“/n Addition is %d”, C);
Printf(“/n Address of variable C is %d”, &C);
Getch ();
}
Above program is the program for addition of two numbers in ‘C’ language. In the seventh instruction of above program we used operator ‘%’ and ‘&’ ‘%d’ is the access specifier which tells compiler to take integer value as input whereas. ‘&a’ tells compile to store the taken value at the address of variable ‘a’.
In the ninth instruction compiler will print me value of ‘C’ so the output of 9th instruction is as follows.
Addition is 5[Note : considering a = 2 and b = 3]
In the tenth instruction compiler will print me address of variable C.
So the output of length instruction is as follows. Address of variable C is 1020.
Now consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Printf(“Enter two numbers”);
Scanf(“%d %d”, & a, &b);
c=a+b;
Printf(“\n Addition is %d”,C);
Printf(“\n Address of variable C is %d”,&C);
Printf(‘\n value of variable C is %d”, *(&C));
Getch();
}
Now, we all are clear about the output of instruction 9 and 10.
In the 11th instruction, operator *and & is used. ‘*’ operator tells compile to pickup the value which is stored at the address of variable C. So the output of 11th instruction is as follow. Value of variable C is 5 [considering a = 24 b = 3;]
Q13) Write a program to add two numbers using pointers?
A13) Program
#include <stdio.h>
Int main()
{
int first, second, *p, *q, sum;
Printf("Enter two integers to add\n");
Scanf("%d%d", &first, &second);
p = &first;
q = &second;
sum = *p + *q;
Printf("Sum of the numbers = %d\n", sum);
return 0;
}
Output:
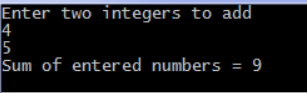
Q14) What is a pointer variable and give example?
A14) Pointers variable
Pointers in C language is a variable that stores/points the address of another variable. A Pointer in C is used to allocate memory dynamically i.e. at run time. The pointer variable might be belonging to any of the data type such as int, float, char, double, short etc.
Pointer Syntax: data_type *var_name; Example : int *p; char *p;
Where, * is used to denote that “p” is pointer variable and not a normal variable.
Normal variable stores the value whereas pointer variable stores the address of the variable.
The content of the C pointer always be a whole number i.e. address.
Always C pointer is initialized to null, i.e. int *p = null.
The value of null pointer is 0.
& symbol is used to get the address of the variable.
* symbol is used to get the value of the variable that the pointer is pointing to.
If a pointer in C is assigned to NULL, it means it is pointing to nothing.
Two pointers can be subtracted to know how many elements are available between these two pointers.
But, Pointer addition, multiplication, division are not allowed.
The size of any pointer is 2 byte (for 16 bit compiler).
Example:
#include <stdio.h>
Int main()
{
int *ptr, q;
q = 50;
/* address of q is assigned to ptr */
ptr = &q;
/* display q's value using ptr variable */
printf("%d", *ptr);
return 0;
}
Output:
1
2
3
4
5
6
7
8
9
10
11
Q15) What is Pointer Arithmetic?
A15) Pointer arithmetic
Pointer arithmetic is slightly different from arithmetic we normally use in our daily life. The only valid arithmetic operations applicable on pointers are:
- Addition of integer to a pointer
- Subtraction of integer to a pointer
- Subtracting two pointers of the same type
The pointer arithmetic is performed relative to the base type of the pointer. For example, if we have an integer pointer ip which contains address 1000, then on incrementing it by 1, we will get 1004 (i.e 1000 + 1 * 4) instead of 1001 because the size of the int data type is 4 bytes. If we had been using a system where the size of int is 2 bytes then we would get 1002 ( i.e 1000 + 1 * 2 ).
Similarly, on decrementing it we will get 996 (i.e 1000 - 1 * 4) instead of 999.
So, the expression ip + 4 will point to the address 1016 (i.e 1000 + 4 * 4).
Let's take some more examples.
1
2
3
Int i = 12, *ip = &i;
Double d = 2.3, *dp = &d;
Char ch = 'a', *cp = &ch;
Suppose the address of i, d and ch are 1000, 2000, 3000 respectively, therefore ip, dp and cp are at 1000, 2000, 3000 initially.
Q16) Explain in detail Dynamic memory allocation?
A16) Dynamic memory allocation
The concept of dynamic memory allocation in c language enables the C programmer to allocate memory at runtime. Dynamic memory allocation in c language is possible by 4 functions of stdlib.h header file.
- Malloc()
- Calloc()
- Realloc()
- Free()
Before learning above functions, let's understand the difference between static memory allocation and dynamic memory allocation.
Static memory allocation
Dynamic memory allocation
Memory is allocated at compile time.
Memory is allocated at run time.
Memory can't be increased while executing program.
Memory can be increased while executing program.
Used in array.
Used in linked list.
Now let's have a quick look at the methods used for dynamic memory allocation.
Malloc()
Allocates single block of requested memory.
Calloc()
Allocates multiple block of requested memory.
Realloc()
Reallocates the memory occupied by malloc() or calloc() functions.
Free()
Frees the dynamically allocated memory.
Malloc() function in C
The malloc() function allocates single block of requested memory.
It doesn't initialize memory at execution time, so it has garbage value initially.
It returns NULL if memory is not sufficient.
The syntax of malloc() function is given below:
Ptr=(cast-type*)malloc(byte-size)
Let's see the example of malloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)malloc(n*sizeof(int)); //memory allocated using malloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Calloc() function in C
The calloc() function allocates multiple block of requested memory.
It initially initialize all bytes to zero.
It returns NULL if memory is not sufficient.
The syntax of calloc() function is given below:
Ptr=(cast-type*)calloc(number, byte-size)
Let's see the example of calloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Realloc() function in C
If memory is not sufficient for malloc() or calloc(), you can reallocate the memory by realloc() function. In short, it changes the memory size.
Let's see the syntax of realloc() function.
Ptr=realloc(ptr, new-size)
Free() function in C
The memory occupied by malloc() or calloc() functions must be released by calling free() function. Otherwise, it will consume memory until program exit.
Let's see the syntax of free() function.
Free(ptr)
Q17) Write the difference between the local and global variable?
A17) Difference between Global variable and Local variable
Global Variable | Local Variable |
Outside of all the function blocks, global variables are declared. | Within a function block, local variables are declared. |
Throughout the programme, the scope is maintained. | The scope is restricted, and they can only be used in the function in which they are declared. |
Any modification to a global variable has an impact on the entire programme, regardless of where it is utilised. | Any changes to the local variable have no effect on the program's other functions.
|
A global variable is present in the programme for the duration of its execution. | After the function is executed, a local variable is created, and when the function is finished, the variable is destroyed. |
It can be accessed from anywhere in the programme using any of the program's functionalities. | It is only accessible through the function statements in which it is declared, not through other functions. |
If a global variable isn't initialised, it defaults to zero. | If a local variable isn't initialised, it defaults to a garbage value. |
The data segment of memory stores global variables. | Local variables are kept in memory on a stack. |
We can't use the same name for several variables. | Variables having the same name can be declared in several functions. |
Q18) Write short notes on local variable?
A18) Local variables
Local variables are variables that are declared within a function or block. They can only be utilised by statements that are included within that function or code block. Functions outside of their own are unaware of local variables. The following example demonstrates the use of local variables. The variables a, b, and c are all local to the function main().
#include <stdio.h>
Int main () {
/* local variable declaration */
int a, b;
int c;
/* actual initialization */
a = 10;
b = 20;
c = a + b;
printf ("value of a = %d, b = %d and c = %d\n", a, b, c);
return 0;
}
Advantages of Local Variable
● Because a local variable's name is only recognised by the function in which it is declared, it can be used in several functions with the same name.
● Local variables only require memory for the duration of the function; after that, the same memory address can be reused.
Disadvantages of Local Variables
● The local variable's scope is restricted to its function and it cannot be used by other functions.
● The local variable is not allowed to share data.
Q19) What do you mean by global variable?
A19) Global variables
Global variables are variables that are defined outside of a function, usually at the start of a programme. Global variables maintain their values throughout the life of your programme and can be accessed from any of the program's functions.
Any function has access to a global variable. That is, once a global variable is declared, it is available for use throughout your whole programme. The following programme demonstrates the use of global variables in a programme.
#include <stdio.h>
/* global variable declaration */
Int g;
Int main () {
/* local variable declaration */
int a, b;
/* actual initialization */
a = 10;
b = 20;
g = a + b;
printf ("value of a = %d, b = %d and g = %d\n", a, b, g);
return 0;
}
Local and global variables in a programme can have the same name, but the value of a local variable inside a function takes precedence. Here's an illustration:
Include <stdio.h>
/* global variable declaration */
Int g = 20;
Int main () {
/* local variable declaration */
int g = 10;
printf ("value of g = %d\n", g);
return 0;
}
When the preceding code is compiled and run, the following result is obtained:
Value of g = 10
Advantages of Global Variable
● Global variables can be accessible by all of the program's functions.
● It is just necessary to make a single declaration.
● If all of the functions are accessing the same data, this is quite handy.
Disadvantages of Global Variable
● Because it can be utilised by any function in the programme, the value of a global variable can be modified by accident.
● If we employ a big number of global variables, there is a good possibility that the programme may generate errors.
Q20) Describe storage classes with example?
A20) Storage classes
Within a C programme, a storage class defines the scope (visibility) and lifetime of variables and/or functions. They come before the type they modify. In a C programme, there are four different storage classes:
● auto
● register
● static
● extern
Auto
At runtime, memory is automatically allocated to automated variables.
The automated variables' visibility is confined to the block in which they are defined. The automated variables' scope is constrained to the block in which they are defined. By default, the automated variables are set to garbage.
When you depart the block, the memory allotted to automatic variables is released. The auto keyword is used to define automatic variables.
By default, every local variable in C is automatic.
For all local variables, the auto storage class is the default storage class.
{
int mount;
auto int month;
}
The preceding example declares two variables in the same storage class. The keyword 'auto' can only be used within functions, i.e. within local variables.
Example:
#include <stdio.h>
Int main()
{
Int a; //auto
Char b;
Float c;
Printf("%d %c %f",a,b,c); // printing initial default value of automatic variables a, b, and c.
Return 0;
}
Output:
Garbage garbage garbage
Register
The memory is allocated to the CPU registers by the variables declared as the registers, depending on the size of the memory remaining in the CPU.
We can't dereference the register variables, which means we can't use & operator for them. The register variables have a faster access time than the automatic variables. The register local variables have a default value of 0.
The variable that should be saved in the CPU register is denoted by the register keyword. Is it, nevertheless, a compiler? The variables can be stored in the register at the user's discretion. We can store pointers in registers, which means that a register can hold the address of a variable. Because we can't use more than one storage specifier for the same variable, static variables can't be stored in the register.
{
register int miles;
}
Example:
#include <stdio.h>
Int main()
{
Register int a; // variable a is allocated memory in the CPU register. The initial default value of a is 0.
Printf("%d",a);
}
Output:
0
Static
The static storage class tells the compiler to keep a local variable alive for the duration of the programme, rather than creating and destroying it every time it enters and exits scope. As a result, making local variables static ensures that their values are preserved between function calls.
The static modifier can be used on global variables as well. When you do this, the scope of that variable is limited to the file in which it is declared.
When the keyword static is applied on a global variable in C programming, just one copy of that member is shared by all objects in that class.
#include <stdio.h>
/* function declaration */
Void func(void);
Static int count = 5; /* global variable */
Main() {
while(count--) {
func();
}
return 0;
}
/* function definition */
Void func( void ) {
static int i = 5; /* local static variable */
i++;
printf("i is %d and count is %d\n", i, count);
}
When the above code is compiled and executed, it produces the following result −
i is 6 and count is 4
i is 7 and count is 3
i is 8 and count is 2
i is 9 and count is 1
i is 10 and count is 0
Extern
The extern storage class is used to provide a reference to a global variable that is shared by all application files. The variable cannot be initialised when you use 'extern,' but it does direct the variable name to a previously established storage location.
When you have many files and specify a global variable or function that will be used in other files, extern is used in another file to provide a reference to the defined variable or function. To clarify, extern is a keyword that is used to declare a global variable or function in a different file.
Example:
#include <stdio.h>
Int main()
{
Extern int a;
Printf("%d",a);
}
Output
Main.c:(.text+0x6): undefined reference to a'
Collect2: error: ld returned 1 exit status
Unit - 1
Functions and Pointer
Q1) Explain function?
A1) Similar to other languages C language also provides the facility of function. Function is the block of code which is used to perform a specific task. In c language the complete program is composed of function.
Functions are useful to divide c programs into smaller modules. Programmer can invoked these modules anywhere inside c program for any number of times.
Functions are used to increase readability of the code. Size of program can be reduce by using functions. By using function, programmer can divide complex tasks into smaller manageable tasks and test them independently before using them together.
Functions of C language are defined with the type of function. The type of functions indicates the data type of value which will return by function. In order to use function in the program, initially programmer have to inform compiler about the function. This is also called as defining a function.
In C programme all the function definition present outside the main function. All function need to be declared and defined before use. Function declaration requires function name, argument list, and return type.
Return TypeFunction name (Argument list)
{
Statement 1;
Statement 2;
…………...
Statement n;
}
Q2) Explain User Defined Functions?
A2) User Defined Functions
User defined function is the block of code written by programmer to perform a particular task. As compiler doesn’t have any idea about the user define function so programmer has to define and declare these functions inside the program body. Programmer can define these function outside the main function but declaration of user define function should present in main function only. Whenever compiler executes function call (function declaration) then compiler shift the flow of program execution to the definition part of user define function.
Example
#include <stdio.h>
#include<conio.h>
Int add (int x, int y)
{
int sum;
sum = x + y;
return (sum);
}
Main ()
{
int a,b,c;
a = 15;
b = 25;
c = add(a,b);
Printf ("\n Addition is %d ", c);
}
Output:
Addition is 40
There are two ways to pass the parameters to the function
- Parameter Passing by value - In this mechanism, the value of the parameter is passed while calling the function.
- Parameter Passing by reference - In this mechanism, the address of the parameter is passed while calling the function.
Q3) Explain parameter passing in functions?
A3) This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q4) Write a program to swap two numbers using call by value and reference.
A4) Program
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q5) Explain how you pass arrays to functions?
A5) Array is a data structure which stores the collection of similar types of element in consecutive memory locations. Indexing of array always start with ‘0’ where as non-graphical variable ‘\0’ indicates the end of array.
Syntax for declaring array is
Data type array name[Maximum size];
● Data types are used to define type of element in an array. Data types are also useful in finding the size of total memory locations allocated for the array.
● All the rules of defining name of variable are also applicable for the array name.
● Maximum size indicates the total number of maximum elements that array can hold.
● Total memory allocated for the array is equal to the memory required to store one element of the array multiply by the total element in the array.
● In array, memory allocation is done at the time of declaration of an array.
Example:
Sr. No. | Instructions | Description |
#include<stdio.h> | Header file included | |
2. | #include<conio.h> | Header file included |
3. | Void main() | Execution of program begins |
4. | { | Memory is allocated for variable i,n and array a |
5. | Int i,n,a[10]; | |
6. | Clrscr(); | Clear the output of previous screen |
7. | Printf("enter a number"); | Print “enter a number” |
8. | Scanf("%d",&n); | Input value is stored at the addres of variable n |
9. | For(i=0;i<=10;i++) | For loop started from value of i=0 to i=10 |
10. | { | Compound statement(scope of for loop starts) |
11. | a[i]=n*i; | Result of multiplication of n and I is stored at the ith location of array ieasi=0 so it is stores at first location. |
12. | Printf("\n %d",a[i]); | Value of ith location of the array is printed |
13. | } | Compound statement(scope of for loop ends) |
14. | Printf("\n first element in array is %d",a[0]); | Value of first element of the array is printed |
15. | Printf("\n fifth element in array is %d",a[4]); | Value of fifth element of the array is printed |
16. | Printf("\n tenth element in array is %d",a[9]); | Value of tenth element of the array is printed |
17. | Getch(); | Used to hold the output screen |
18. | } | Indicates end of scope of main function |
This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q6) Write a program to find the sum of N natural numbers using functions?
A6) /* C Program to find Sum of N Numbers using Functions */
#include<stdio.h>
Int Sum_Of_Natural_Numbers(int Number);
Int main()
{
int Number, i, Sum = 0;
Printf("\nPlease Enter any Integer Value\n");
Scanf("%d", &Number);
Sum = Sum_Of_Natural_Numbers(Number);
Printf("Sum of Natural Numbers = %d", Sum);
return 0;
}
Int Sum_Of_Natural_Numbers(int Number)
{
Int i, Sum = 0;
If (Number == 0)
{
Return Number;
}
Else
{
Return (Number * (Number + 1) / 2);
}
}
Output:
Please enter any Integer Value
5
Sum of Natural Numbers = 15
Q7) Write a program to find the GCD of a number using functions?
A7) Program
#include<stdio.h>
Int main() {
int a, b;
Printf("Enter 2 numbers:");
Scanf("%d%d", & a, & b);
Printf("Greatest Common Divisor is %d", gcd(a, b));
return 0;
}
Int gcd(long a, long b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
Output:
Enter two positive integers: 81
153
GCD = 9
Q8) Write a program to find the factorial of a number using functions?
A8) Program
#include<stdio.h>
#include<math.h>
Void main()
{
//clrscr();
Printf("Enter a Number to Find Factorial: ");
Fact();
Getch();
}
Fact()
{
int i,fact=1,n;
Scanf("%d",&n);
For(i=1; i<=n; i++)
{
fact=fact*i;
}
Printf("\nFactorial of a Given Number is: %d ",fact);
return fact;
}
OUTPUT:
Enter a Number to Find Factorial: 5
Factorial of a Given Number is: 120
Q9) Write a program to check if the given number is prime or not?
A9) Program
#include <conio.h>
Void main()
{
Int num,res=0;
Clrscr();
Printf("\nENTER A NUMBER: ");
Scanf("%d",&num);
Res=prime(num);
If(res==0)
Printf("\n%d IS A PRIME NUMBER",num);
Else
Printf("\n%d IS NOT A PRIME NUMBER",num);
Getch();
}
Int prime(int n)
{
Int i;
For(i=2;i<=n/2;i++)
{
If(n%i!=0)
Continue;
Else
Return 1;
}
Return 0;
}
Output
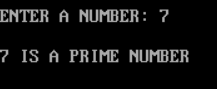
Q10) Write a program to convert a binary number to its equivalent decimal number?
A10) Program
#include <stdio.h>
Void main()
{
Int num, binary_val, decimal_val = 0, base = 1, rem;
Printf("Enter a binary number(1s and 0s) \n");
Scanf("%d", &num); /* maximum five digits */
Binary_val = num;
while (num> 0)
{
rem = num % 10;
Decimal_val = decimal_val + rem * base;
Num = num / 10 ;
base = base * 2;
}
Printf("The Binary number is = %d \n", binary_val);
Printf("Its decimal equivalent is = %d \n", decimal_val);
}
Enter a binary number(1s and 0s)
10101001
The Binary number is = 10101001
Its decimal equivalent is = 169
Q11) Define recursion?
A11) Recursion
In programming languages, if a program allows you to call a function inside the same function, then it is called a recursive call of the function.
Void recursion() {
Recursion(); /* function calls itself */
}
Int main() {
recursion();
}
The C programming language supports recursion, i.e., a function to call itself. But while using recursion, programmers has to be careful to define an exit condition from the function, otherwise it will go into an infinite loop.
Recursive functions are useful to solve many mathematical problems, such as calculating the factorial of a number, generating Fibonacci series, etc.
The following example calculates the factorial of a given number using a recursive function −
#include <stdio.h>
Unsigned long long int factorial(unsigned int i) {
if(i<= 1) {
return 1;
}
return i * factorial(i - 1);
}
Int main() {
int i = 12;
Printf("Factorial of %d is %d\n", i, factorial(i));
return 0;
}
When the above code is compiled and executed, it produces the following result–
Factorial of 12 is 479001600
The following example generates the Fibonacci series for a given number using a recursive function −
#include <stdio.h>
Int fibonacci(int i) {
if(i == 0) {
return 0;
}
if(i == 1) {
return 1;
}
return fibonacci(i-1) + fibonacci(i-2);
}
Int main() {
int i;
for (i = 0; i< 10; i++) {
Printf("%d\t\n", fibonacci(i));
}
return 0;
}
When the above code is compiled and executed, it produces the following result−
0
1
1
2
3
5
8
13
21
34
Q12) What is a pointer? How do you declare pointers?
A12) Pointer
One of the vital and heavily used feature ‘C’ is pointer. Most of the other programming languages also support pointers but only few of them use it freely. Support pointers but only few of them use it freely.
When we declare any variable in C language, there are three things associated with that variable.
- Data type of variable: Data type defines the type of data that variable can hold. Data type tells compiler about the amount of memory allocate to the variable.
- Address of Variable: Address of variable represent the exact address of memory location which is allocated to variable.
- Value of variable: It is the value of variable which is store at the address of memory location allocated to variable.
Example: int n = 5;
In the above example ‘int’ is the data type which tells compiler to allocate 2 bytes of memory to variable ‘n’.
Once the variable is declare compiler allocated two bytes of memory to variable ‘n’. Suppose the address of that memory location is 1020. At the address of memory location 1020 the value 5 is store.
Memory map for above declaration is as follows.
Use of &,/and ‘*’ operator
Consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c,
Printf (“Enter two numbers”);
Scanf (“%d%d”, &a,&b);
c=a+b;
Printf(“/n Addition is %d”, C);
Printf(“/n Address of variable C is %d”, &C);
Getch ();
}
Above program is the program for addition of two numbers in ‘C’ language. In the seventh instruction of above program we used operator ‘%’ and ‘&’ ‘%d’ is the access specifier which tells compiler to take integer value as input whereas. ‘&a’ tells compile to store the taken value at the address of variable ‘a’.
In the ninth instruction compiler will print me value of ‘C’ so the output of 9th instruction is as follows.
Addition is 5[Note : considering a = 2 and b = 3]
In the tenth instruction compiler will print me address of variable C.
So the output of length instruction is as follows. Address of variable C is 1020.
Now consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Printf(“Enter two numbers”);
Scanf(“%d %d”, & a, &b);
c=a+b;
Printf(“\n Addition is %d”,C);
Printf(“\n Address of variable C is %d”,&C);
Printf(‘\n value of variable C is %d”, *(&C));
Getch();
}
Now, we all are clear about the output of instruction 9 and 10.
In the 11th instruction, operator *and & is used. ‘*’ operator tells compile to pickup the value which is stored at the address of variable C. So the output of 11th instruction is as follow. Value of variable C is 5 [considering a = 24 b = 3;]
Q13) Write a program to add two numbers using pointers?
A13) Program
#include <stdio.h>
Int main()
{
int first, second, *p, *q, sum;
Printf("Enter two integers to add\n");
Scanf("%d%d", &first, &second);
p = &first;
q = &second;
sum = *p + *q;
Printf("Sum of the numbers = %d\n", sum);
return 0;
}
Output:
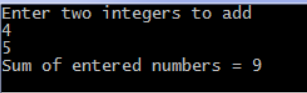
Q14) What is a pointer variable and give example?
A14) Pointers variable
Pointers in C language is a variable that stores/points the address of another variable. A Pointer in C is used to allocate memory dynamically i.e. at run time. The pointer variable might be belonging to any of the data type such as int, float, char, double, short etc.
Pointer Syntax: data_type *var_name; Example : int *p; char *p;
Where, * is used to denote that “p” is pointer variable and not a normal variable.
Normal variable stores the value whereas pointer variable stores the address of the variable.
The content of the C pointer always be a whole number i.e. address.
Always C pointer is initialized to null, i.e. int *p = null.
The value of null pointer is 0.
& symbol is used to get the address of the variable.
* symbol is used to get the value of the variable that the pointer is pointing to.
If a pointer in C is assigned to NULL, it means it is pointing to nothing.
Two pointers can be subtracted to know how many elements are available between these two pointers.
But, Pointer addition, multiplication, division are not allowed.
The size of any pointer is 2 byte (for 16 bit compiler).
Example:
#include <stdio.h>
Int main()
{
int *ptr, q;
q = 50;
/* address of q is assigned to ptr */
ptr = &q;
/* display q's value using ptr variable */
printf("%d", *ptr);
return 0;
}
Output:
1
2
3
4
5
6
7
8
9
10
11
Q15) What is Pointer Arithmetic?
A15) Pointer arithmetic
Pointer arithmetic is slightly different from arithmetic we normally use in our daily life. The only valid arithmetic operations applicable on pointers are:
- Addition of integer to a pointer
- Subtraction of integer to a pointer
- Subtracting two pointers of the same type
The pointer arithmetic is performed relative to the base type of the pointer. For example, if we have an integer pointer ip which contains address 1000, then on incrementing it by 1, we will get 1004 (i.e 1000 + 1 * 4) instead of 1001 because the size of the int data type is 4 bytes. If we had been using a system where the size of int is 2 bytes then we would get 1002 ( i.e 1000 + 1 * 2 ).
Similarly, on decrementing it we will get 996 (i.e 1000 - 1 * 4) instead of 999.
So, the expression ip + 4 will point to the address 1016 (i.e 1000 + 4 * 4).
Let's take some more examples.
1
2
3
Int i = 12, *ip = &i;
Double d = 2.3, *dp = &d;
Char ch = 'a', *cp = &ch;
Suppose the address of i, d and ch are 1000, 2000, 3000 respectively, therefore ip, dp and cp are at 1000, 2000, 3000 initially.
Q16) Explain in detail Dynamic memory allocation?
A16) Dynamic memory allocation
The concept of dynamic memory allocation in c language enables the C programmer to allocate memory at runtime. Dynamic memory allocation in c language is possible by 4 functions of stdlib.h header file.
- Malloc()
- Calloc()
- Realloc()
- Free()
Before learning above functions, let's understand the difference between static memory allocation and dynamic memory allocation.
Static memory allocation
Dynamic memory allocation
Memory is allocated at compile time.
Memory is allocated at run time.
Memory can't be increased while executing program.
Memory can be increased while executing program.
Used in array.
Used in linked list.
Now let's have a quick look at the methods used for dynamic memory allocation.
Malloc()
Allocates single block of requested memory.
Calloc()
Allocates multiple block of requested memory.
Realloc()
Reallocates the memory occupied by malloc() or calloc() functions.
Free()
Frees the dynamically allocated memory.
Malloc() function in C
The malloc() function allocates single block of requested memory.
It doesn't initialize memory at execution time, so it has garbage value initially.
It returns NULL if memory is not sufficient.
The syntax of malloc() function is given below:
Ptr=(cast-type*)malloc(byte-size)
Let's see the example of malloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)malloc(n*sizeof(int)); //memory allocated using malloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Calloc() function in C
The calloc() function allocates multiple block of requested memory.
It initially initialize all bytes to zero.
It returns NULL if memory is not sufficient.
The syntax of calloc() function is given below:
Ptr=(cast-type*)calloc(number, byte-size)
Let's see the example of calloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Realloc() function in C
If memory is not sufficient for malloc() or calloc(), you can reallocate the memory by realloc() function. In short, it changes the memory size.
Let's see the syntax of realloc() function.
Ptr=realloc(ptr, new-size)
Free() function in C
The memory occupied by malloc() or calloc() functions must be released by calling free() function. Otherwise, it will consume memory until program exit.
Let's see the syntax of free() function.
Free(ptr)
Q17) Write the difference between the local and global variable?
A17) Difference between Global variable and Local variable
Global Variable | Local Variable |
Outside of all the function blocks, global variables are declared. | Within a function block, local variables are declared. |
Throughout the programme, the scope is maintained. | The scope is restricted, and they can only be used in the function in which they are declared. |
Any modification to a global variable has an impact on the entire programme, regardless of where it is utilised. | Any changes to the local variable have no effect on the program's other functions.
|
A global variable is present in the programme for the duration of its execution. | After the function is executed, a local variable is created, and when the function is finished, the variable is destroyed. |
It can be accessed from anywhere in the programme using any of the program's functionalities. | It is only accessible through the function statements in which it is declared, not through other functions. |
If a global variable isn't initialised, it defaults to zero. | If a local variable isn't initialised, it defaults to a garbage value. |
The data segment of memory stores global variables. | Local variables are kept in memory on a stack. |
We can't use the same name for several variables. | Variables having the same name can be declared in several functions. |
Q18) Write short notes on local variable?
A18) Local variables
Local variables are variables that are declared within a function or block. They can only be utilised by statements that are included within that function or code block. Functions outside of their own are unaware of local variables. The following example demonstrates the use of local variables. The variables a, b, and c are all local to the function main().
#include <stdio.h>
Int main () {
/* local variable declaration */
int a, b;
int c;
/* actual initialization */
a = 10;
b = 20;
c = a + b;
printf ("value of a = %d, b = %d and c = %d\n", a, b, c);
return 0;
}
Advantages of Local Variable
● Because a local variable's name is only recognised by the function in which it is declared, it can be used in several functions with the same name.
● Local variables only require memory for the duration of the function; after that, the same memory address can be reused.
Disadvantages of Local Variables
● The local variable's scope is restricted to its function and it cannot be used by other functions.
● The local variable is not allowed to share data.
Q19) What do you mean by global variable?
A19) Global variables
Global variables are variables that are defined outside of a function, usually at the start of a programme. Global variables maintain their values throughout the life of your programme and can be accessed from any of the program's functions.
Any function has access to a global variable. That is, once a global variable is declared, it is available for use throughout your whole programme. The following programme demonstrates the use of global variables in a programme.
#include <stdio.h>
/* global variable declaration */
Int g;
Int main () {
/* local variable declaration */
int a, b;
/* actual initialization */
a = 10;
b = 20;
g = a + b;
printf ("value of a = %d, b = %d and g = %d\n", a, b, g);
return 0;
}
Local and global variables in a programme can have the same name, but the value of a local variable inside a function takes precedence. Here's an illustration:
Include <stdio.h>
/* global variable declaration */
Int g = 20;
Int main () {
/* local variable declaration */
int g = 10;
printf ("value of g = %d\n", g);
return 0;
}
When the preceding code is compiled and run, the following result is obtained:
Value of g = 10
Advantages of Global Variable
● Global variables can be accessible by all of the program's functions.
● It is just necessary to make a single declaration.
● If all of the functions are accessing the same data, this is quite handy.
Disadvantages of Global Variable
● Because it can be utilised by any function in the programme, the value of a global variable can be modified by accident.
● If we employ a big number of global variables, there is a good possibility that the programme may generate errors.
Q20) Describe storage classes with example?
A20) Storage classes
Within a C programme, a storage class defines the scope (visibility) and lifetime of variables and/or functions. They come before the type they modify. In a C programme, there are four different storage classes:
● auto
● register
● static
● extern
Auto
At runtime, memory is automatically allocated to automated variables.
The automated variables' visibility is confined to the block in which they are defined. The automated variables' scope is constrained to the block in which they are defined. By default, the automated variables are set to garbage.
When you depart the block, the memory allotted to automatic variables is released. The auto keyword is used to define automatic variables.
By default, every local variable in C is automatic.
For all local variables, the auto storage class is the default storage class.
{
int mount;
auto int month;
}
The preceding example declares two variables in the same storage class. The keyword 'auto' can only be used within functions, i.e. within local variables.
Example:
#include <stdio.h>
Int main()
{
Int a; //auto
Char b;
Float c;
Printf("%d %c %f",a,b,c); // printing initial default value of automatic variables a, b, and c.
Return 0;
}
Output:
Garbage garbage garbage
Register
The memory is allocated to the CPU registers by the variables declared as the registers, depending on the size of the memory remaining in the CPU.
We can't dereference the register variables, which means we can't use & operator for them. The register variables have a faster access time than the automatic variables. The register local variables have a default value of 0.
The variable that should be saved in the CPU register is denoted by the register keyword. Is it, nevertheless, a compiler? The variables can be stored in the register at the user's discretion. We can store pointers in registers, which means that a register can hold the address of a variable. Because we can't use more than one storage specifier for the same variable, static variables can't be stored in the register.
{
register int miles;
}
Example:
#include <stdio.h>
Int main()
{
Register int a; // variable a is allocated memory in the CPU register. The initial default value of a is 0.
Printf("%d",a);
}
Output:
0
Static
The static storage class tells the compiler to keep a local variable alive for the duration of the programme, rather than creating and destroying it every time it enters and exits scope. As a result, making local variables static ensures that their values are preserved between function calls.
The static modifier can be used on global variables as well. When you do this, the scope of that variable is limited to the file in which it is declared.
When the keyword static is applied on a global variable in C programming, just one copy of that member is shared by all objects in that class.
#include <stdio.h>
/* function declaration */
Void func(void);
Static int count = 5; /* global variable */
Main() {
while(count--) {
func();
}
return 0;
}
/* function definition */
Void func( void ) {
static int i = 5; /* local static variable */
i++;
printf("i is %d and count is %d\n", i, count);
}
When the above code is compiled and executed, it produces the following result −
i is 6 and count is 4
i is 7 and count is 3
i is 8 and count is 2
i is 9 and count is 1
i is 10 and count is 0
Extern
The extern storage class is used to provide a reference to a global variable that is shared by all application files. The variable cannot be initialised when you use 'extern,' but it does direct the variable name to a previously established storage location.
When you have many files and specify a global variable or function that will be used in other files, extern is used in another file to provide a reference to the defined variable or function. To clarify, extern is a keyword that is used to declare a global variable or function in a different file.
Example:
#include <stdio.h>
Int main()
{
Extern int a;
Printf("%d",a);
}
Output
Main.c:(.text+0x6): undefined reference to a'
Collect2: error: ld returned 1 exit status
Unit - 1
Functions and Pointer
Q1) Explain function?
A1) Similar to other languages C language also provides the facility of function. Function is the block of code which is used to perform a specific task. In c language the complete program is composed of function.
Functions are useful to divide c programs into smaller modules. Programmer can invoked these modules anywhere inside c program for any number of times.
Functions are used to increase readability of the code. Size of program can be reduce by using functions. By using function, programmer can divide complex tasks into smaller manageable tasks and test them independently before using them together.
Functions of C language are defined with the type of function. The type of functions indicates the data type of value which will return by function. In order to use function in the program, initially programmer have to inform compiler about the function. This is also called as defining a function.
In C programme all the function definition present outside the main function. All function need to be declared and defined before use. Function declaration requires function name, argument list, and return type.
Return TypeFunction name (Argument list)
{
Statement 1;
Statement 2;
…………...
Statement n;
}
Q2) Explain User Defined Functions?
A2) User Defined Functions
User defined function is the block of code written by programmer to perform a particular task. As compiler doesn’t have any idea about the user define function so programmer has to define and declare these functions inside the program body. Programmer can define these function outside the main function but declaration of user define function should present in main function only. Whenever compiler executes function call (function declaration) then compiler shift the flow of program execution to the definition part of user define function.
Example
#include <stdio.h>
#include<conio.h>
Int add (int x, int y)
{
int sum;
sum = x + y;
return (sum);
}
Main ()
{
int a,b,c;
a = 15;
b = 25;
c = add(a,b);
Printf ("\n Addition is %d ", c);
}
Output:
Addition is 40
There are two ways to pass the parameters to the function
- Parameter Passing by value - In this mechanism, the value of the parameter is passed while calling the function.
- Parameter Passing by reference - In this mechanism, the address of the parameter is passed while calling the function.
Q3) Explain parameter passing in functions?
A3) This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q4) Write a program to swap two numbers using call by value and reference.
A4) Program
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q5) Explain how you pass arrays to functions?
A5) Array is a data structure which stores the collection of similar types of element in consecutive memory locations. Indexing of array always start with ‘0’ where as non-graphical variable ‘\0’ indicates the end of array.
Syntax for declaring array is
Data type array name[Maximum size];
● Data types are used to define type of element in an array. Data types are also useful in finding the size of total memory locations allocated for the array.
● All the rules of defining name of variable are also applicable for the array name.
● Maximum size indicates the total number of maximum elements that array can hold.
● Total memory allocated for the array is equal to the memory required to store one element of the array multiply by the total element in the array.
● In array, memory allocation is done at the time of declaration of an array.
Example:
Sr. No. | Instructions | Description |
#include<stdio.h> | Header file included | |
2. | #include<conio.h> | Header file included |
3. | Void main() | Execution of program begins |
4. | { | Memory is allocated for variable i,n and array a |
5. | Int i,n,a[10]; | |
6. | Clrscr(); | Clear the output of previous screen |
7. | Printf("enter a number"); | Print “enter a number” |
8. | Scanf("%d",&n); | Input value is stored at the addres of variable n |
9. | For(i=0;i<=10;i++) | For loop started from value of i=0 to i=10 |
10. | { | Compound statement(scope of for loop starts) |
11. | a[i]=n*i; | Result of multiplication of n and I is stored at the ith location of array ieasi=0 so it is stores at first location. |
12. | Printf("\n %d",a[i]); | Value of ith location of the array is printed |
13. | } | Compound statement(scope of for loop ends) |
14. | Printf("\n first element in array is %d",a[0]); | Value of first element of the array is printed |
15. | Printf("\n fifth element in array is %d",a[4]); | Value of fifth element of the array is printed |
16. | Printf("\n tenth element in array is %d",a[9]); | Value of tenth element of the array is printed |
17. | Getch(); | Used to hold the output screen |
18. | } | Indicates end of scope of main function |
This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output:
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Q6) Write a program to find the sum of N natural numbers using functions?
A6) /* C Program to find Sum of N Numbers using Functions */
#include<stdio.h>
Int Sum_Of_Natural_Numbers(int Number);
Int main()
{
int Number, i, Sum = 0;
Printf("\nPlease Enter any Integer Value\n");
Scanf("%d", &Number);
Sum = Sum_Of_Natural_Numbers(Number);
Printf("Sum of Natural Numbers = %d", Sum);
return 0;
}
Int Sum_Of_Natural_Numbers(int Number)
{
Int i, Sum = 0;
If (Number == 0)
{
Return Number;
}
Else
{
Return (Number * (Number + 1) / 2);
}
}
Output:
Please enter any Integer Value
5
Sum of Natural Numbers = 15
Q7) Write a program to find the GCD of a number using functions?
A7) Program
#include<stdio.h>
Int main() {
int a, b;
Printf("Enter 2 numbers:");
Scanf("%d%d", & a, & b);
Printf("Greatest Common Divisor is %d", gcd(a, b));
return 0;
}
Int gcd(long a, long b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
Output:
Enter two positive integers: 81
153
GCD = 9
Q8) Write a program to find the factorial of a number using functions?
A8) Program
#include<stdio.h>
#include<math.h>
Void main()
{
//clrscr();
Printf("Enter a Number to Find Factorial: ");
Fact();
Getch();
}
Fact()
{
int i,fact=1,n;
Scanf("%d",&n);
For(i=1; i<=n; i++)
{
fact=fact*i;
}
Printf("\nFactorial of a Given Number is: %d ",fact);
return fact;
}
OUTPUT:
Enter a Number to Find Factorial: 5
Factorial of a Given Number is: 120
Q9) Write a program to check if the given number is prime or not?
A9) Program
#include <conio.h>
Void main()
{
Int num,res=0;
Clrscr();
Printf("\nENTER A NUMBER: ");
Scanf("%d",&num);
Res=prime(num);
If(res==0)
Printf("\n%d IS A PRIME NUMBER",num);
Else
Printf("\n%d IS NOT A PRIME NUMBER",num);
Getch();
}
Int prime(int n)
{
Int i;
For(i=2;i<=n/2;i++)
{
If(n%i!=0)
Continue;
Else
Return 1;
}
Return 0;
}
Output
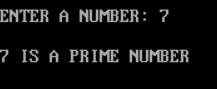
Q10) Write a program to convert a binary number to its equivalent decimal number?
A10) Program
#include <stdio.h>
Void main()
{
Int num, binary_val, decimal_val = 0, base = 1, rem;
Printf("Enter a binary number(1s and 0s) \n");
Scanf("%d", &num); /* maximum five digits */
Binary_val = num;
while (num> 0)
{
rem = num % 10;
Decimal_val = decimal_val + rem * base;
Num = num / 10 ;
base = base * 2;
}
Printf("The Binary number is = %d \n", binary_val);
Printf("Its decimal equivalent is = %d \n", decimal_val);
}
Enter a binary number(1s and 0s)
10101001
The Binary number is = 10101001
Its decimal equivalent is = 169
Q11) Define recursion?
A11) Recursion
In programming languages, if a program allows you to call a function inside the same function, then it is called a recursive call of the function.
Void recursion() {
Recursion(); /* function calls itself */
}
Int main() {
recursion();
}
The C programming language supports recursion, i.e., a function to call itself. But while using recursion, programmers has to be careful to define an exit condition from the function, otherwise it will go into an infinite loop.
Recursive functions are useful to solve many mathematical problems, such as calculating the factorial of a number, generating Fibonacci series, etc.
The following example calculates the factorial of a given number using a recursive function −
#include <stdio.h>
Unsigned long long int factorial(unsigned int i) {
if(i<= 1) {
return 1;
}
return i * factorial(i - 1);
}
Int main() {
int i = 12;
Printf("Factorial of %d is %d\n", i, factorial(i));
return 0;
}
When the above code is compiled and executed, it produces the following result–
Factorial of 12 is 479001600
The following example generates the Fibonacci series for a given number using a recursive function −
#include <stdio.h>
Int fibonacci(int i) {
if(i == 0) {
return 0;
}
if(i == 1) {
return 1;
}
return fibonacci(i-1) + fibonacci(i-2);
}
Int main() {
int i;
for (i = 0; i< 10; i++) {
Printf("%d\t\n", fibonacci(i));
}
return 0;
}
When the above code is compiled and executed, it produces the following result−
0
1
1
2
3
5
8
13
21
34
Q12) What is a pointer? How do you declare pointers?
A12) Pointer
One of the vital and heavily used feature ‘C’ is pointer. Most of the other programming languages also support pointers but only few of them use it freely. Support pointers but only few of them use it freely.
When we declare any variable in C language, there are three things associated with that variable.
- Data type of variable: Data type defines the type of data that variable can hold. Data type tells compiler about the amount of memory allocate to the variable.
- Address of Variable: Address of variable represent the exact address of memory location which is allocated to variable.
- Value of variable: It is the value of variable which is store at the address of memory location allocated to variable.
Example: int n = 5;
In the above example ‘int’ is the data type which tells compiler to allocate 2 bytes of memory to variable ‘n’.
Once the variable is declare compiler allocated two bytes of memory to variable ‘n’. Suppose the address of that memory location is 1020. At the address of memory location 1020 the value 5 is store.
Memory map for above declaration is as follows.
Use of &,/and ‘*’ operator
Consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c,
Printf (“Enter two numbers”);
Scanf (“%d%d”, &a,&b);
c=a+b;
Printf(“/n Addition is %d”, C);
Printf(“/n Address of variable C is %d”, &C);
Getch ();
}
Above program is the program for addition of two numbers in ‘C’ language. In the seventh instruction of above program we used operator ‘%’ and ‘&’ ‘%d’ is the access specifier which tells compiler to take integer value as input whereas. ‘&a’ tells compile to store the taken value at the address of variable ‘a’.
In the ninth instruction compiler will print me value of ‘C’ so the output of 9th instruction is as follows.
Addition is 5[Note : considering a = 2 and b = 3]
In the tenth instruction compiler will print me address of variable C.
So the output of length instruction is as follows. Address of variable C is 1020.
Now consider the following program.
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Printf(“Enter two numbers”);
Scanf(“%d %d”, & a, &b);
c=a+b;
Printf(“\n Addition is %d”,C);
Printf(“\n Address of variable C is %d”,&C);
Printf(‘\n value of variable C is %d”, *(&C));
Getch();
}
Now, we all are clear about the output of instruction 9 and 10.
In the 11th instruction, operator *and & is used. ‘*’ operator tells compile to pickup the value which is stored at the address of variable C. So the output of 11th instruction is as follow. Value of variable C is 5 [considering a = 24 b = 3;]
Q13) Write a program to add two numbers using pointers?
A13) Program
#include <stdio.h>
Int main()
{
int first, second, *p, *q, sum;
Printf("Enter two integers to add\n");
Scanf("%d%d", &first, &second);
p = &first;
q = &second;
sum = *p + *q;
Printf("Sum of the numbers = %d\n", sum);
return 0;
}
Output:
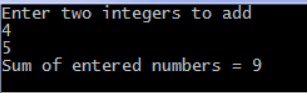
Q14) What is a pointer variable and give example?
A14) Pointers variable
Pointers in C language is a variable that stores/points the address of another variable. A Pointer in C is used to allocate memory dynamically i.e. at run time. The pointer variable might be belonging to any of the data type such as int, float, char, double, short etc.
Pointer Syntax: data_type *var_name; Example : int *p; char *p;
Where, * is used to denote that “p” is pointer variable and not a normal variable.
Normal variable stores the value whereas pointer variable stores the address of the variable.
The content of the C pointer always be a whole number i.e. address.
Always C pointer is initialized to null, i.e. int *p = null.
The value of null pointer is 0.
& symbol is used to get the address of the variable.
* symbol is used to get the value of the variable that the pointer is pointing to.
If a pointer in C is assigned to NULL, it means it is pointing to nothing.
Two pointers can be subtracted to know how many elements are available between these two pointers.
But, Pointer addition, multiplication, division are not allowed.
The size of any pointer is 2 byte (for 16 bit compiler).
Example:
#include <stdio.h>
Int main()
{
int *ptr, q;
q = 50;
/* address of q is assigned to ptr */
ptr = &q;
/* display q's value using ptr variable */
printf("%d", *ptr);
return 0;
}
Output:
1
2
3
4
5
6
7
8
9
10
11
Q15) What is Pointer Arithmetic?
A15) Pointer arithmetic
Pointer arithmetic is slightly different from arithmetic we normally use in our daily life. The only valid arithmetic operations applicable on pointers are:
- Addition of integer to a pointer
- Subtraction of integer to a pointer
- Subtracting two pointers of the same type
The pointer arithmetic is performed relative to the base type of the pointer. For example, if we have an integer pointer ip which contains address 1000, then on incrementing it by 1, we will get 1004 (i.e 1000 + 1 * 4) instead of 1001 because the size of the int data type is 4 bytes. If we had been using a system where the size of int is 2 bytes then we would get 1002 ( i.e 1000 + 1 * 2 ).
Similarly, on decrementing it we will get 996 (i.e 1000 - 1 * 4) instead of 999.
So, the expression ip + 4 will point to the address 1016 (i.e 1000 + 4 * 4).
Let's take some more examples.
1
2
3
Int i = 12, *ip = &i;
Double d = 2.3, *dp = &d;
Char ch = 'a', *cp = &ch;
Suppose the address of i, d and ch are 1000, 2000, 3000 respectively, therefore ip, dp and cp are at 1000, 2000, 3000 initially.
Q16) Explain in detail Dynamic memory allocation?
A16) Dynamic memory allocation
The concept of dynamic memory allocation in c language enables the C programmer to allocate memory at runtime. Dynamic memory allocation in c language is possible by 4 functions of stdlib.h header file.
- Malloc()
- Calloc()
- Realloc()
- Free()
Before learning above functions, let's understand the difference between static memory allocation and dynamic memory allocation.
Static memory allocation
Dynamic memory allocation
Memory is allocated at compile time.
Memory is allocated at run time.
Memory can't be increased while executing program.
Memory can be increased while executing program.
Used in array.
Used in linked list.
Now let's have a quick look at the methods used for dynamic memory allocation.
Malloc()
Allocates single block of requested memory.
Calloc()
Allocates multiple block of requested memory.
Realloc()
Reallocates the memory occupied by malloc() or calloc() functions.
Free()
Frees the dynamically allocated memory.
Malloc() function in C
The malloc() function allocates single block of requested memory.
It doesn't initialize memory at execution time, so it has garbage value initially.
It returns NULL if memory is not sufficient.
The syntax of malloc() function is given below:
Ptr=(cast-type*)malloc(byte-size)
Let's see the example of malloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)malloc(n*sizeof(int)); //memory allocated using malloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Calloc() function in C
The calloc() function allocates multiple block of requested memory.
It initially initialize all bytes to zero.
It returns NULL if memory is not sufficient.
The syntax of calloc() function is given below:
Ptr=(cast-type*)calloc(number, byte-size)
Let's see the example of calloc() function.
- #include<stdio.h>
- #include<stdlib.h>
- Int main(){
- Int n,i,*ptr,sum=0;
- Printf("Enter number of elements: ");
- Scanf("%d",&n);
- Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
- If(ptr==NULL)
- {
- Printf("Sorry! unable to allocate memory");
- Exit(0);
- }
- Printf("Enter elements of array: ");
- For(i=0;i<n;++i)
- {
- Scanf("%d",ptr+i);
- Sum+=*(ptr+i);
- }
- Printf("Sum=%d",sum);
- Free(ptr);
- Return 0;
- }
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Realloc() function in C
If memory is not sufficient for malloc() or calloc(), you can reallocate the memory by realloc() function. In short, it changes the memory size.
Let's see the syntax of realloc() function.
Ptr=realloc(ptr, new-size)
Free() function in C
The memory occupied by malloc() or calloc() functions must be released by calling free() function. Otherwise, it will consume memory until program exit.
Let's see the syntax of free() function.
Free(ptr)
Q17) Write the difference between the local and global variable?
A17) Difference between Global variable and Local variable
Global Variable | Local Variable |
Outside of all the function blocks, global variables are declared. | Within a function block, local variables are declared. |
Throughout the programme, the scope is maintained. | The scope is restricted, and they can only be used in the function in which they are declared. |
Any modification to a global variable has an impact on the entire programme, regardless of where it is utilised. | Any changes to the local variable have no effect on the program's other functions.
|
A global variable is present in the programme for the duration of its execution. | After the function is executed, a local variable is created, and when the function is finished, the variable is destroyed. |
It can be accessed from anywhere in the programme using any of the program's functionalities. | It is only accessible through the function statements in which it is declared, not through other functions. |
If a global variable isn't initialised, it defaults to zero. | If a local variable isn't initialised, it defaults to a garbage value. |
The data segment of memory stores global variables. | Local variables are kept in memory on a stack. |
We can't use the same name for several variables. | Variables having the same name can be declared in several functions. |
Q18) Write short notes on local variable?
A18) Local variables
Local variables are variables that are declared within a function or block. They can only be utilised by statements that are included within that function or code block. Functions outside of their own are unaware of local variables. The following example demonstrates the use of local variables. The variables a, b, and c are all local to the function main().
#include <stdio.h>
Int main () {
/* local variable declaration */
int a, b;
int c;
/* actual initialization */
a = 10;
b = 20;
c = a + b;
printf ("value of a = %d, b = %d and c = %d\n", a, b, c);
return 0;
}
Advantages of Local Variable
● Because a local variable's name is only recognised by the function in which it is declared, it can be used in several functions with the same name.
● Local variables only require memory for the duration of the function; after that, the same memory address can be reused.
Disadvantages of Local Variables
● The local variable's scope is restricted to its function and it cannot be used by other functions.
● The local variable is not allowed to share data.
Q19) What do you mean by global variable?
A19) Global variables
Global variables are variables that are defined outside of a function, usually at the start of a programme. Global variables maintain their values throughout the life of your programme and can be accessed from any of the program's functions.
Any function has access to a global variable. That is, once a global variable is declared, it is available for use throughout your whole programme. The following programme demonstrates the use of global variables in a programme.
#include <stdio.h>
/* global variable declaration */
Int g;
Int main () {
/* local variable declaration */
int a, b;
/* actual initialization */
a = 10;
b = 20;
g = a + b;
printf ("value of a = %d, b = %d and g = %d\n", a, b, g);
return 0;
}
Local and global variables in a programme can have the same name, but the value of a local variable inside a function takes precedence. Here's an illustration:
Include <stdio.h>
/* global variable declaration */
Int g = 20;
Int main () {
/* local variable declaration */
int g = 10;
printf ("value of g = %d\n", g);
return 0;
}
When the preceding code is compiled and run, the following result is obtained:
Value of g = 10
Advantages of Global Variable
● Global variables can be accessible by all of the program's functions.
● It is just necessary to make a single declaration.
● If all of the functions are accessing the same data, this is quite handy.
Disadvantages of Global Variable
● Because it can be utilised by any function in the programme, the value of a global variable can be modified by accident.
● If we employ a big number of global variables, there is a good possibility that the programme may generate errors.
Q20) Describe storage classes with example?
A20) Storage classes
Within a C programme, a storage class defines the scope (visibility) and lifetime of variables and/or functions. They come before the type they modify. In a C programme, there are four different storage classes:
● auto
● register
● static
● extern
Auto
At runtime, memory is automatically allocated to automated variables.
The automated variables' visibility is confined to the block in which they are defined. The automated variables' scope is constrained to the block in which they are defined. By default, the automated variables are set to garbage.
When you depart the block, the memory allotted to automatic variables is released. The auto keyword is used to define automatic variables.
By default, every local variable in C is automatic.
For all local variables, the auto storage class is the default storage class.
{
int mount;
auto int month;
}
The preceding example declares two variables in the same storage class. The keyword 'auto' can only be used within functions, i.e. within local variables.
Example:
#include <stdio.h>
Int main()
{
Int a; //auto
Char b;
Float c;
Printf("%d %c %f",a,b,c); // printing initial default value of automatic variables a, b, and c.
Return 0;
}
Output:
Garbage garbage garbage
Register
The memory is allocated to the CPU registers by the variables declared as the registers, depending on the size of the memory remaining in the CPU.
We can't dereference the register variables, which means we can't use & operator for them. The register variables have a faster access time than the automatic variables. The register local variables have a default value of 0.
The variable that should be saved in the CPU register is denoted by the register keyword. Is it, nevertheless, a compiler? The variables can be stored in the register at the user's discretion. We can store pointers in registers, which means that a register can hold the address of a variable. Because we can't use more than one storage specifier for the same variable, static variables can't be stored in the register.
{
register int miles;
}
Example:
#include <stdio.h>
Int main()
{
Register int a; // variable a is allocated memory in the CPU register. The initial default value of a is 0.
Printf("%d",a);
}
Output:
0
Static
The static storage class tells the compiler to keep a local variable alive for the duration of the programme, rather than creating and destroying it every time it enters and exits scope. As a result, making local variables static ensures that their values are preserved between function calls.
The static modifier can be used on global variables as well. When you do this, the scope of that variable is limited to the file in which it is declared.
When the keyword static is applied on a global variable in C programming, just one copy of that member is shared by all objects in that class.
#include <stdio.h>
/* function declaration */
Void func(void);
Static int count = 5; /* global variable */
Main() {
while(count--) {
func();
}
return 0;
}
/* function definition */
Void func( void ) {
static int i = 5; /* local static variable */
i++;
printf("i is %d and count is %d\n", i, count);
}
When the above code is compiled and executed, it produces the following result −
i is 6 and count is 4
i is 7 and count is 3
i is 8 and count is 2
i is 9 and count is 1
i is 10 and count is 0
Extern
The extern storage class is used to provide a reference to a global variable that is shared by all application files. The variable cannot be initialised when you use 'extern,' but it does direct the variable name to a previously established storage location.
When you have many files and specify a global variable or function that will be used in other files, extern is used in another file to provide a reference to the defined variable or function. To clarify, extern is a keyword that is used to declare a global variable or function in a different file.
Example:
#include <stdio.h>
Int main()
{
Extern int a;
Printf("%d",a);
}
Output
Main.c:(.text+0x6): undefined reference to a'
Collect2: error: ld returned 1 exit status