Unit - 2
Structures, Union and File Handling
Q1) Define structure? Explain how to access elements in structures?
A1) Structure
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
• Ordinary variables can hold one piece of information
• arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
- Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
- Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Q2) Explain structure variable declaration?
A2) Structure variable
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1 ,e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Q3) How do you declare structures for multiple variables?
A3) Declaration
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Q4) How do you access structure elements in the array?
A4) Access structure element in array
Structure is a collection of different data types. An object of structure represents a single record in memory, if we want more than one record of structure type, we have to create an array of structure or object. As we know, an array is a collection of similar type, therefore an array can be of structure type.
Syntax for declaring structure array
Struct struct-name
{
Datatype var1;
Datatype var2;
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj [ size ];
Example for declaring structure array
#include<stdio.h>
Struct Employee
{
Int Id;
Char Name[25];
Int Age;
Long Salary;
};
Void main()
{
Int i;
Struct Employee Emp[ 3 ]; //Statement 1
For(i=0;i<3;i++)
{
Printf("\nEnter details of %d Employee",i+1);
Printf("\n\tEnter Employee Id : ");
Scanf("%d",&Emp[i].Id);
Printf("\n\tEnter Employee Name : ");
Scanf("%s",&Emp[i].Name);
Printf("\n\tEnter Employee Age : ");
Scanf("%d",&Emp[i].Age);
Printf("\n\tEnter Employee Salary : ");
Scanf("%ld",&Emp[i].Salary);
}
Printf("\nDetails of Employees");
For(i=0;i<3;i++)
Printf("\n%d\t%s\t%d\t%ld",Emp[i].Id,Emp[i].Name,Emp[i].Age,Emp[i].Salary);
}
Output:
Enter details of 1 Employee
Enter Employee Id : 101
Enter Employee Name : Suresh
Enter Employee Age : 29
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name : Mukesh
Enter Employee Age : 31
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Ramesh
Enter Employee Age : 28
Enter Employee Salary : 47000
Details of Employees
101 Suresh 29 45000
102 Mukesh 31 51000
103 Ramesh 28 47000
In the above example, we are getting and displaying the data of 3 employee using array of object. Statement 1 is creating an array of Employee Emp to store the records of 3 employees.
Array within Structure
As we know, structure is collection of different data type. Like normal data type, It can
Also store an array as well.
Syntax for array within structure
Struct struct-name
{
Datatype var1; // normal variable
Datatype array [size]; // array variable
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj;
Example for array within structure
Struct Student
{
Int Roll;
Char Name[25];
Int Marks[3]; //Statement 1 : array of marks
Int Total;
Float Avg;
};
Void main()
{
Int i;
Struct Student S;
Printf("\n\nEnter Student Roll : ");
Scanf("%d",&S.Roll);
Printf("\n\nEnter Student Name : ");
Scanf("%s",&S.Name);
S.Total = 0;
For(i=0;i<3;i++)
{
Printf("\n\nEnter Marks %d : ",i+1);
Scanf("%d",&S.Marks[i]);
S.Total = S.Total + S.Marks[i];
}
S.Avg = S.Total / 3;
Printf("\nRoll : %d",S.Roll);
Printf("\nName : %s",S.Name);
Printf("\nTotal : %d",S.Total);
Printf("\nAverage : %f",S.Avg);
}
Output:
Enter Student Roll : 10
Enter Student Name : Kumar
Enter Marks 1 : 78
Enter Marks 2 : 89
Enter Marks 3 : 56
Roll : 10
Name : Kumar
Total : 223
Average: 74.00000
In the above example, we have created an array Marks[ ] inside structure representing 3 marks of a single student. Marks[ ] is now a member of structure student and to access Marks[ ] we have used dot operator(.) along with object S.
Q5) Write a program to obtain the details of the 3 employees?
A5) Program
#include <iostream>
Using namespace std;
Struct employee {
String ename;
Int age, phn_no;
Int salary;
};
// Function to display details of all employees
Void display(struct employee emp[], int n)
{
Cout<< "Name\tAge\tPhone Number\tSalary\n";
For (int i = 0; i< n; i++) {
Cout<< emp[i].ename<< "\t" << emp[i].age << "\t"
<< emp[i].phn_no<< "\t" << emp[i].salary << "\n";
}
}
// Driver code
Int main()
{
Int n = 3;
// Array of structure objects
Struct employee emp[n];
// Details of employee 1
Emp[0].ename = "Chirag";
Emp[0].age = 24;
Emp[0].phn_no = 1234567788;
Emp[0].salary = 20000;
// Details of employee 2
Emp[1].ename = "Arnav";
Emp[1].age = 31;
Emp[1].phn_no = 1234567891;
Emp[1].salary = 56000;
// Details of employee 3
Emp[2].ename = "Shivam";
Emp[2].age = 45;
Emp[2].phn_no = 1100661111;
Emp[2].salary = 30500;
Display(emp, n);
Return 0;
}
Output:
Name Age Phone Number Salary
Chirag 24 1234567788 20000
Arnav 31 1234567891 56000
Shivam 45 8881101111 30500
Q6) Define union?
A6) Union
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Q7) How do you access the members of the union?
A7) Access member of union
● Array elements are accessed using the Subscript variable , Similarly Union members are accessed using dot [.] operator.
● (.) is called as “union member Operator”.
● Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P- garbage value, ABC
Q8) Compare structure and union?
A8) Structure Vs Union
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Q9) Write a program to store information of students and display it?
A9) Program
#include <stdio.h>
#include <string.h>
Union Student
{
Int age;
Char Name[50];
Char Department[20];
};
Int main()
{
Union student stud1;
Union Employee stud2;
Stud1.age = 28;
Strcpy(stud1.Name, "Chris");
Strcpy(stud1.Department, "Science");
Printf("\nDetails of the First Student \n");
Printf(" Student Age = %d \n", emp1.age);
Printf(" Student Name = %s \n", emp1.Name);
Printf(" Student Department = %s \n", emp1.Department);
Printf("Details of the Second Student \n" );
Stud2.age = 30;
Printf(" Student Age = %d \n", stud2.age );
Strcpy(stud2.Name, "David");
Printf(" Student Name = %s \n", stud2.Name );
Strcpy(stud2.Department, "Technology" );
Printf(" Student Department = %s \n ", stud2.Department );
Return 0;
}
OUTPUT:
Details of the first student
Student Age = 28
Student Name = Chris
Student Department = Science
Details of the Second Student
Student Age =30
Student Name = David
Student Department= Technology
Q10) Write the advantages and disadvantages of structure?
A10) Advantages of structure
● Structures gather more than one piece of data about the same subject together in the same place.
● It is helpful when you want to gather the data of similar data types and parameters like first name, last name, etc.
● It is very easy to maintain as we can represent the whole record by using a single name.
● In structure, we can pass a complete set of records to any function using a single parameter.
● You can use an array of structure to store more records with similar types.
Disadvantages of structure
● If the complexity of an IT project goes beyond the limit, it becomes hard to manage.
● Change of one data structure in a code necessitates changes at many other places. Therefore, the changes become hard to track.
● Structure is slower because it requires storage space for all the data.
● You can retrieve any member at a time in structure whereas you can access one member at a time in the union.
● Structure occupies space for each and every member written in inner parameters while union occupies space for a member having the highest size written in inner parameters.
● Structure supports a flexible array. Union does not support a flexible array.
Q11) Write an example for calloc ()?
A11) Program
Example of calloc()
#include<stdio.h>
#include<stdlib.h>
Int main()
{
Int n,i,*ptr,sum=0;
Printf("Enter number of elements: ");
Scanf("%d",&n);
Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
If(ptr==NULL)
{
Printf("Sorry! unable to allocate memory");
Exit(0);
}
Printf("Enter elements of array: ");
For(i=0;i<n;++i)
{
Scanf("%d",ptr+i);
Sum+=*(ptr+i);
}
Printf("Sum=%d",sum);
Free(ptr);
Return 0;
}
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Q12) Explain nested structure?
A12) The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address.
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by
Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Q13) Write a program for nested strcuture?
A13) Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
Q14) Describe an Array of structure with an example?
A14) In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
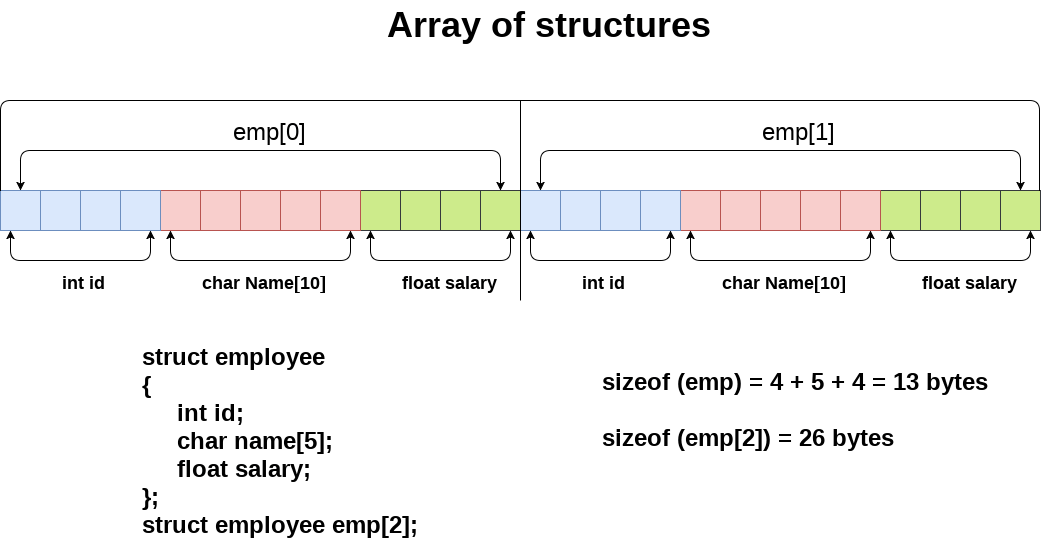
Fig 1: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Q15) Write a Program to access the structure member using the structure pointer and arrow (->) operator?
A15) Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include <stdio.h>
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
Q16) Write a Program to access the structure member using structure pointer and the dot operator?
A16) Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
Q17) What is sizeof ()?
A17) In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
Q18) Define typedef?
A18) typedef
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Q19) Write notes on - fseek(), ftell() and rewind()?
A19) fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
Q20) What are the formatted input?
A20) scanf()
We utilize the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.
Unit - 2
Structures, Union and File Handling
Q1) Define structure? Explain how to access elements in structures?
A1) Structure
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
• Ordinary variables can hold one piece of information
• arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
- Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
- Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Q2) Explain structure variable declaration?
A2) Structure variable
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1 ,e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Q3) How do you declare structures for multiple variables?
A3) Declaration
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Q4) How do you access structure elements in the array?
A4) Access structure element in array
Structure is a collection of different data types. An object of structure represents a single record in memory, if we want more than one record of structure type, we have to create an array of structure or object. As we know, an array is a collection of similar type, therefore an array can be of structure type.
Syntax for declaring structure array
Struct struct-name
{
Datatype var1;
Datatype var2;
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj [ size ];
Example for declaring structure array
#include<stdio.h>
Struct Employee
{
Int Id;
Char Name[25];
Int Age;
Long Salary;
};
Void main()
{
Int i;
Struct Employee Emp[ 3 ]; //Statement 1
For(i=0;i<3;i++)
{
Printf("\nEnter details of %d Employee",i+1);
Printf("\n\tEnter Employee Id : ");
Scanf("%d",&Emp[i].Id);
Printf("\n\tEnter Employee Name : ");
Scanf("%s",&Emp[i].Name);
Printf("\n\tEnter Employee Age : ");
Scanf("%d",&Emp[i].Age);
Printf("\n\tEnter Employee Salary : ");
Scanf("%ld",&Emp[i].Salary);
}
Printf("\nDetails of Employees");
For(i=0;i<3;i++)
Printf("\n%d\t%s\t%d\t%ld",Emp[i].Id,Emp[i].Name,Emp[i].Age,Emp[i].Salary);
}
Output:
Enter details of 1 Employee
Enter Employee Id : 101
Enter Employee Name : Suresh
Enter Employee Age : 29
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name : Mukesh
Enter Employee Age : 31
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Ramesh
Enter Employee Age : 28
Enter Employee Salary : 47000
Details of Employees
101 Suresh 29 45000
102 Mukesh 31 51000
103 Ramesh 28 47000
In the above example, we are getting and displaying the data of 3 employee using array of object. Statement 1 is creating an array of Employee Emp to store the records of 3 employees.
Array within Structure
As we know, structure is collection of different data type. Like normal data type, It can
Also store an array as well.
Syntax for array within structure
Struct struct-name
{
Datatype var1; // normal variable
Datatype array [size]; // array variable
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj;
Example for array within structure
Struct Student
{
Int Roll;
Char Name[25];
Int Marks[3]; //Statement 1 : array of marks
Int Total;
Float Avg;
};
Void main()
{
Int i;
Struct Student S;
Printf("\n\nEnter Student Roll : ");
Scanf("%d",&S.Roll);
Printf("\n\nEnter Student Name : ");
Scanf("%s",&S.Name);
S.Total = 0;
For(i=0;i<3;i++)
{
Printf("\n\nEnter Marks %d : ",i+1);
Scanf("%d",&S.Marks[i]);
S.Total = S.Total + S.Marks[i];
}
S.Avg = S.Total / 3;
Printf("\nRoll : %d",S.Roll);
Printf("\nName : %s",S.Name);
Printf("\nTotal : %d",S.Total);
Printf("\nAverage : %f",S.Avg);
}
Output:
Enter Student Roll : 10
Enter Student Name : Kumar
Enter Marks 1 : 78
Enter Marks 2 : 89
Enter Marks 3 : 56
Roll : 10
Name : Kumar
Total : 223
Average: 74.00000
In the above example, we have created an array Marks[ ] inside structure representing 3 marks of a single student. Marks[ ] is now a member of structure student and to access Marks[ ] we have used dot operator(.) along with object S.
Q5) Write a program to obtain the details of the 3 employees?
A5) Program
#include <iostream>
Using namespace std;
Struct employee {
String ename;
Int age, phn_no;
Int salary;
};
// Function to display details of all employees
Void display(struct employee emp[], int n)
{
Cout<< "Name\tAge\tPhone Number\tSalary\n";
For (int i = 0; i< n; i++) {
Cout<< emp[i].ename<< "\t" << emp[i].age << "\t"
<< emp[i].phn_no<< "\t" << emp[i].salary << "\n";
}
}
// Driver code
Int main()
{
Int n = 3;
// Array of structure objects
Struct employee emp[n];
// Details of employee 1
Emp[0].ename = "Chirag";
Emp[0].age = 24;
Emp[0].phn_no = 1234567788;
Emp[0].salary = 20000;
// Details of employee 2
Emp[1].ename = "Arnav";
Emp[1].age = 31;
Emp[1].phn_no = 1234567891;
Emp[1].salary = 56000;
// Details of employee 3
Emp[2].ename = "Shivam";
Emp[2].age = 45;
Emp[2].phn_no = 1100661111;
Emp[2].salary = 30500;
Display(emp, n);
Return 0;
}
Output:
Name Age Phone Number Salary
Chirag 24 1234567788 20000
Arnav 31 1234567891 56000
Shivam 45 8881101111 30500
Q6) Define union?
A6) Union
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Q7) How do you access the members of the union?
A7) Access member of union
● Array elements are accessed using the Subscript variable , Similarly Union members are accessed using dot [.] operator.
● (.) is called as “union member Operator”.
● Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P- garbage value, ABC
Q8) Compare structure and union?
A8) Structure Vs Union
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Q9) Write a program to store information of students and display it?
A9) Program
#include <stdio.h>
#include <string.h>
Union Student
{
Int age;
Char Name[50];
Char Department[20];
};
Int main()
{
Union student stud1;
Union Employee stud2;
Stud1.age = 28;
Strcpy(stud1.Name, "Chris");
Strcpy(stud1.Department, "Science");
Printf("\nDetails of the First Student \n");
Printf(" Student Age = %d \n", emp1.age);
Printf(" Student Name = %s \n", emp1.Name);
Printf(" Student Department = %s \n", emp1.Department);
Printf("Details of the Second Student \n" );
Stud2.age = 30;
Printf(" Student Age = %d \n", stud2.age );
Strcpy(stud2.Name, "David");
Printf(" Student Name = %s \n", stud2.Name );
Strcpy(stud2.Department, "Technology" );
Printf(" Student Department = %s \n ", stud2.Department );
Return 0;
}
OUTPUT:
Details of the first student
Student Age = 28
Student Name = Chris
Student Department = Science
Details of the Second Student
Student Age =30
Student Name = David
Student Department= Technology
Q10) Write the advantages and disadvantages of structure?
A10) Advantages of structure
● Structures gather more than one piece of data about the same subject together in the same place.
● It is helpful when you want to gather the data of similar data types and parameters like first name, last name, etc.
● It is very easy to maintain as we can represent the whole record by using a single name.
● In structure, we can pass a complete set of records to any function using a single parameter.
● You can use an array of structure to store more records with similar types.
Disadvantages of structure
● If the complexity of an IT project goes beyond the limit, it becomes hard to manage.
● Change of one data structure in a code necessitates changes at many other places. Therefore, the changes become hard to track.
● Structure is slower because it requires storage space for all the data.
● You can retrieve any member at a time in structure whereas you can access one member at a time in the union.
● Structure occupies space for each and every member written in inner parameters while union occupies space for a member having the highest size written in inner parameters.
● Structure supports a flexible array. Union does not support a flexible array.
Q11) Write an example for calloc ()?
A11) Program
Example of calloc()
#include<stdio.h>
#include<stdlib.h>
Int main()
{
Int n,i,*ptr,sum=0;
Printf("Enter number of elements: ");
Scanf("%d",&n);
Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
If(ptr==NULL)
{
Printf("Sorry! unable to allocate memory");
Exit(0);
}
Printf("Enter elements of array: ");
For(i=0;i<n;++i)
{
Scanf("%d",ptr+i);
Sum+=*(ptr+i);
}
Printf("Sum=%d",sum);
Free(ptr);
Return 0;
}
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Q12) Explain nested structure?
A12) The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address.
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by
Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Q13) Write a program for nested strcuture?
A13) Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
Q14) Describe an Array of structure with an example?
A14) In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
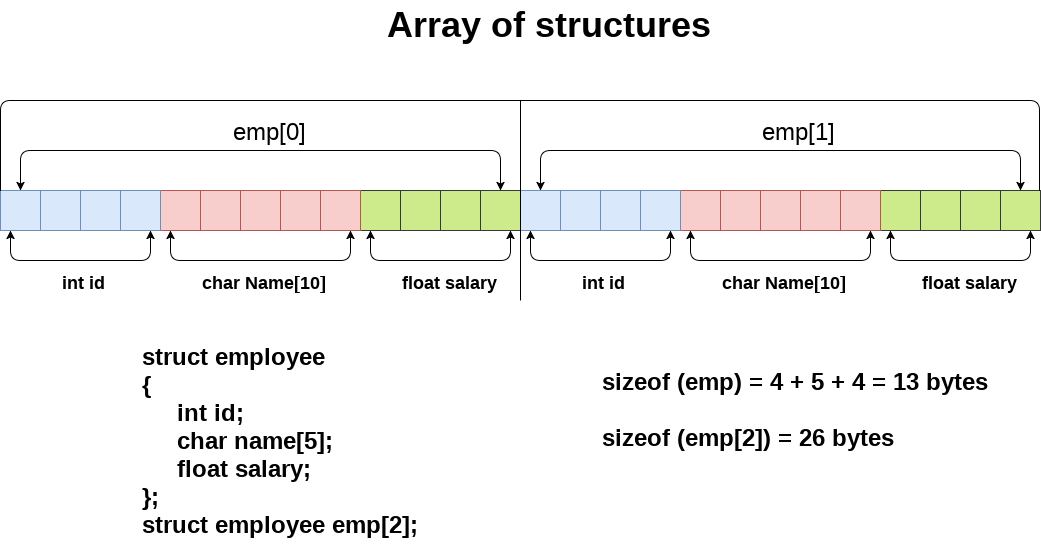
Fig 1: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Q15) Write a Program to access the structure member using the structure pointer and arrow (->) operator?
A15) Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include <stdio.h>
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
Q16) Write a Program to access the structure member using structure pointer and the dot operator?
A16) Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
Q17) What is sizeof ()?
A17) In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
Q18) Define typedef?
A18) typedef
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Q19) Write notes on - fseek(), ftell() and rewind()?
A19) fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
Q20) What are the formatted input?
A20) scanf()
We utilize the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.
Unit - 2
Structures, Union and File Handling
Q1) Define structure? Explain how to access elements in structures?
A1) Structure
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
• Ordinary variables can hold one piece of information
• arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
- Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
- Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Q2) Explain structure variable declaration?
A2) Structure variable
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1 ,e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Q3) How do you declare structures for multiple variables?
A3) Declaration
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Q4) How do you access structure elements in the array?
A4) Access structure element in array
Structure is a collection of different data types. An object of structure represents a single record in memory, if we want more than one record of structure type, we have to create an array of structure or object. As we know, an array is a collection of similar type, therefore an array can be of structure type.
Syntax for declaring structure array
Struct struct-name
{
Datatype var1;
Datatype var2;
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj [ size ];
Example for declaring structure array
#include<stdio.h>
Struct Employee
{
Int Id;
Char Name[25];
Int Age;
Long Salary;
};
Void main()
{
Int i;
Struct Employee Emp[ 3 ]; //Statement 1
For(i=0;i<3;i++)
{
Printf("\nEnter details of %d Employee",i+1);
Printf("\n\tEnter Employee Id : ");
Scanf("%d",&Emp[i].Id);
Printf("\n\tEnter Employee Name : ");
Scanf("%s",&Emp[i].Name);
Printf("\n\tEnter Employee Age : ");
Scanf("%d",&Emp[i].Age);
Printf("\n\tEnter Employee Salary : ");
Scanf("%ld",&Emp[i].Salary);
}
Printf("\nDetails of Employees");
For(i=0;i<3;i++)
Printf("\n%d\t%s\t%d\t%ld",Emp[i].Id,Emp[i].Name,Emp[i].Age,Emp[i].Salary);
}
Output:
Enter details of 1 Employee
Enter Employee Id : 101
Enter Employee Name : Suresh
Enter Employee Age : 29
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name : Mukesh
Enter Employee Age : 31
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Ramesh
Enter Employee Age : 28
Enter Employee Salary : 47000
Details of Employees
101 Suresh 29 45000
102 Mukesh 31 51000
103 Ramesh 28 47000
In the above example, we are getting and displaying the data of 3 employee using array of object. Statement 1 is creating an array of Employee Emp to store the records of 3 employees.
Array within Structure
As we know, structure is collection of different data type. Like normal data type, It can
Also store an array as well.
Syntax for array within structure
Struct struct-name
{
Datatype var1; // normal variable
Datatype array [size]; // array variable
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj;
Example for array within structure
Struct Student
{
Int Roll;
Char Name[25];
Int Marks[3]; //Statement 1 : array of marks
Int Total;
Float Avg;
};
Void main()
{
Int i;
Struct Student S;
Printf("\n\nEnter Student Roll : ");
Scanf("%d",&S.Roll);
Printf("\n\nEnter Student Name : ");
Scanf("%s",&S.Name);
S.Total = 0;
For(i=0;i<3;i++)
{
Printf("\n\nEnter Marks %d : ",i+1);
Scanf("%d",&S.Marks[i]);
S.Total = S.Total + S.Marks[i];
}
S.Avg = S.Total / 3;
Printf("\nRoll : %d",S.Roll);
Printf("\nName : %s",S.Name);
Printf("\nTotal : %d",S.Total);
Printf("\nAverage : %f",S.Avg);
}
Output:
Enter Student Roll : 10
Enter Student Name : Kumar
Enter Marks 1 : 78
Enter Marks 2 : 89
Enter Marks 3 : 56
Roll : 10
Name : Kumar
Total : 223
Average: 74.00000
In the above example, we have created an array Marks[ ] inside structure representing 3 marks of a single student. Marks[ ] is now a member of structure student and to access Marks[ ] we have used dot operator(.) along with object S.
Q5) Write a program to obtain the details of the 3 employees?
A5) Program
#include <iostream>
Using namespace std;
Struct employee {
String ename;
Int age, phn_no;
Int salary;
};
// Function to display details of all employees
Void display(struct employee emp[], int n)
{
Cout<< "Name\tAge\tPhone Number\tSalary\n";
For (int i = 0; i< n; i++) {
Cout<< emp[i].ename<< "\t" << emp[i].age << "\t"
<< emp[i].phn_no<< "\t" << emp[i].salary << "\n";
}
}
// Driver code
Int main()
{
Int n = 3;
// Array of structure objects
Struct employee emp[n];
// Details of employee 1
Emp[0].ename = "Chirag";
Emp[0].age = 24;
Emp[0].phn_no = 1234567788;
Emp[0].salary = 20000;
// Details of employee 2
Emp[1].ename = "Arnav";
Emp[1].age = 31;
Emp[1].phn_no = 1234567891;
Emp[1].salary = 56000;
// Details of employee 3
Emp[2].ename = "Shivam";
Emp[2].age = 45;
Emp[2].phn_no = 1100661111;
Emp[2].salary = 30500;
Display(emp, n);
Return 0;
}
Output:
Name Age Phone Number Salary
Chirag 24 1234567788 20000
Arnav 31 1234567891 56000
Shivam 45 8881101111 30500
Q6) Define union?
A6) Union
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Q7) How do you access the members of the union?
A7) Access member of union
● Array elements are accessed using the Subscript variable , Similarly Union members are accessed using dot [.] operator.
● (.) is called as “union member Operator”.
● Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P- garbage value, ABC
Q8) Compare structure and union?
A8) Structure Vs Union
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Q9) Write a program to store information of students and display it?
A9) Program
#include <stdio.h>
#include <string.h>
Union Student
{
Int age;
Char Name[50];
Char Department[20];
};
Int main()
{
Union student stud1;
Union Employee stud2;
Stud1.age = 28;
Strcpy(stud1.Name, "Chris");
Strcpy(stud1.Department, "Science");
Printf("\nDetails of the First Student \n");
Printf(" Student Age = %d \n", emp1.age);
Printf(" Student Name = %s \n", emp1.Name);
Printf(" Student Department = %s \n", emp1.Department);
Printf("Details of the Second Student \n" );
Stud2.age = 30;
Printf(" Student Age = %d \n", stud2.age );
Strcpy(stud2.Name, "David");
Printf(" Student Name = %s \n", stud2.Name );
Strcpy(stud2.Department, "Technology" );
Printf(" Student Department = %s \n ", stud2.Department );
Return 0;
}
OUTPUT:
Details of the first student
Student Age = 28
Student Name = Chris
Student Department = Science
Details of the Second Student
Student Age =30
Student Name = David
Student Department= Technology
Q10) Write the advantages and disadvantages of structure?
A10) Advantages of structure
● Structures gather more than one piece of data about the same subject together in the same place.
● It is helpful when you want to gather the data of similar data types and parameters like first name, last name, etc.
● It is very easy to maintain as we can represent the whole record by using a single name.
● In structure, we can pass a complete set of records to any function using a single parameter.
● You can use an array of structure to store more records with similar types.
Disadvantages of structure
● If the complexity of an IT project goes beyond the limit, it becomes hard to manage.
● Change of one data structure in a code necessitates changes at many other places. Therefore, the changes become hard to track.
● Structure is slower because it requires storage space for all the data.
● You can retrieve any member at a time in structure whereas you can access one member at a time in the union.
● Structure occupies space for each and every member written in inner parameters while union occupies space for a member having the highest size written in inner parameters.
● Structure supports a flexible array. Union does not support a flexible array.
Q11) Write an example for calloc ()?
A11) Program
Example of calloc()
#include<stdio.h>
#include<stdlib.h>
Int main()
{
Int n,i,*ptr,sum=0;
Printf("Enter number of elements: ");
Scanf("%d",&n);
Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
If(ptr==NULL)
{
Printf("Sorry! unable to allocate memory");
Exit(0);
}
Printf("Enter elements of array: ");
For(i=0;i<n;++i)
{
Scanf("%d",ptr+i);
Sum+=*(ptr+i);
}
Printf("Sum=%d",sum);
Free(ptr);
Return 0;
}
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Q12) Explain nested structure?
A12) The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address.
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by
Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Q13) Write a program for nested strcuture?
A13) Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
Q14) Describe an Array of structure with an example?
A14) In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
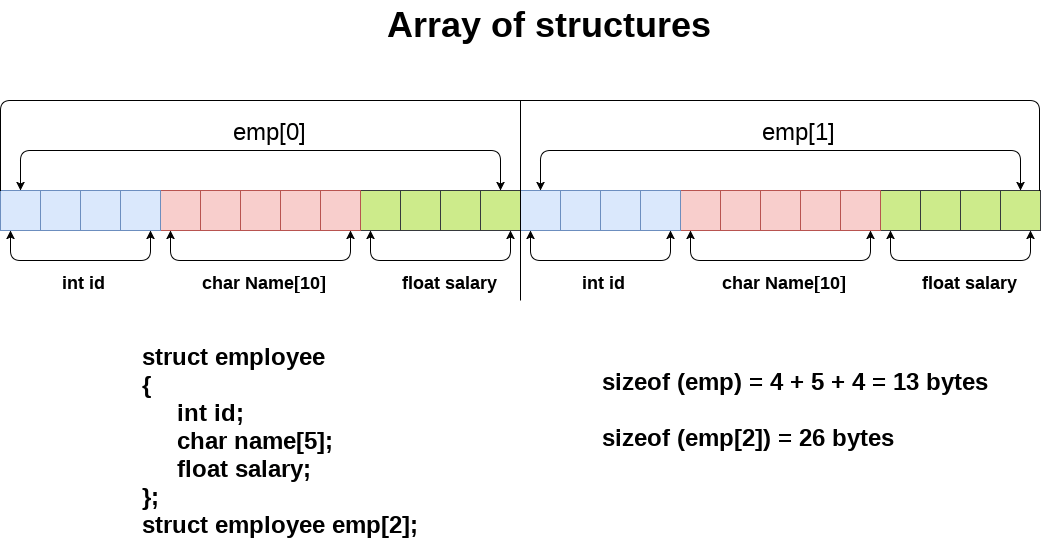
Fig 1: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Q15) Write a Program to access the structure member using the structure pointer and arrow (->) operator?
A15) Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include <stdio.h>
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
Q16) Write a Program to access the structure member using structure pointer and the dot operator?
A16) Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
Q17) What is sizeof ()?
A17) In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
Q18) Define typedef?
A18) typedef
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Q19) Write notes on - fseek(), ftell() and rewind()?
A19) fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
Q20) What are the formatted input?
A20) scanf()
We utilize the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.
Unit - 2
Structures, Union and File Handling
Q1) Define structure? Explain how to access elements in structures?
A1) Structure
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
• Ordinary variables can hold one piece of information
• arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
- Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
- Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Q2) Explain structure variable declaration?
A2) Structure variable
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1 ,e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Q3) How do you declare structures for multiple variables?
A3) Declaration
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Q4) How do you access structure elements in the array?
A4) Access structure element in array
Structure is a collection of different data types. An object of structure represents a single record in memory, if we want more than one record of structure type, we have to create an array of structure or object. As we know, an array is a collection of similar type, therefore an array can be of structure type.
Syntax for declaring structure array
Struct struct-name
{
Datatype var1;
Datatype var2;
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj [ size ];
Example for declaring structure array
#include<stdio.h>
Struct Employee
{
Int Id;
Char Name[25];
Int Age;
Long Salary;
};
Void main()
{
Int i;
Struct Employee Emp[ 3 ]; //Statement 1
For(i=0;i<3;i++)
{
Printf("\nEnter details of %d Employee",i+1);
Printf("\n\tEnter Employee Id : ");
Scanf("%d",&Emp[i].Id);
Printf("\n\tEnter Employee Name : ");
Scanf("%s",&Emp[i].Name);
Printf("\n\tEnter Employee Age : ");
Scanf("%d",&Emp[i].Age);
Printf("\n\tEnter Employee Salary : ");
Scanf("%ld",&Emp[i].Salary);
}
Printf("\nDetails of Employees");
For(i=0;i<3;i++)
Printf("\n%d\t%s\t%d\t%ld",Emp[i].Id,Emp[i].Name,Emp[i].Age,Emp[i].Salary);
}
Output:
Enter details of 1 Employee
Enter Employee Id : 101
Enter Employee Name : Suresh
Enter Employee Age : 29
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name : Mukesh
Enter Employee Age : 31
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Ramesh
Enter Employee Age : 28
Enter Employee Salary : 47000
Details of Employees
101 Suresh 29 45000
102 Mukesh 31 51000
103 Ramesh 28 47000
In the above example, we are getting and displaying the data of 3 employee using array of object. Statement 1 is creating an array of Employee Emp to store the records of 3 employees.
Array within Structure
As we know, structure is collection of different data type. Like normal data type, It can
Also store an array as well.
Syntax for array within structure
Struct struct-name
{
Datatype var1; // normal variable
Datatype array [size]; // array variable
- - - - - - - - - -
- - - - - - - - - -
Datatype varN;
};
Struct struct-name obj;
Example for array within structure
Struct Student
{
Int Roll;
Char Name[25];
Int Marks[3]; //Statement 1 : array of marks
Int Total;
Float Avg;
};
Void main()
{
Int i;
Struct Student S;
Printf("\n\nEnter Student Roll : ");
Scanf("%d",&S.Roll);
Printf("\n\nEnter Student Name : ");
Scanf("%s",&S.Name);
S.Total = 0;
For(i=0;i<3;i++)
{
Printf("\n\nEnter Marks %d : ",i+1);
Scanf("%d",&S.Marks[i]);
S.Total = S.Total + S.Marks[i];
}
S.Avg = S.Total / 3;
Printf("\nRoll : %d",S.Roll);
Printf("\nName : %s",S.Name);
Printf("\nTotal : %d",S.Total);
Printf("\nAverage : %f",S.Avg);
}
Output:
Enter Student Roll : 10
Enter Student Name : Kumar
Enter Marks 1 : 78
Enter Marks 2 : 89
Enter Marks 3 : 56
Roll : 10
Name : Kumar
Total : 223
Average: 74.00000
In the above example, we have created an array Marks[ ] inside structure representing 3 marks of a single student. Marks[ ] is now a member of structure student and to access Marks[ ] we have used dot operator(.) along with object S.
Q5) Write a program to obtain the details of the 3 employees?
A5) Program
#include <iostream>
Using namespace std;
Struct employee {
String ename;
Int age, phn_no;
Int salary;
};
// Function to display details of all employees
Void display(struct employee emp[], int n)
{
Cout<< "Name\tAge\tPhone Number\tSalary\n";
For (int i = 0; i< n; i++) {
Cout<< emp[i].ename<< "\t" << emp[i].age << "\t"
<< emp[i].phn_no<< "\t" << emp[i].salary << "\n";
}
}
// Driver code
Int main()
{
Int n = 3;
// Array of structure objects
Struct employee emp[n];
// Details of employee 1
Emp[0].ename = "Chirag";
Emp[0].age = 24;
Emp[0].phn_no = 1234567788;
Emp[0].salary = 20000;
// Details of employee 2
Emp[1].ename = "Arnav";
Emp[1].age = 31;
Emp[1].phn_no = 1234567891;
Emp[1].salary = 56000;
// Details of employee 3
Emp[2].ename = "Shivam";
Emp[2].age = 45;
Emp[2].phn_no = 1100661111;
Emp[2].salary = 30500;
Display(emp, n);
Return 0;
}
Output:
Name Age Phone Number Salary
Chirag 24 1234567788 20000
Arnav 31 1234567891 56000
Shivam 45 8881101111 30500
Q6) Define union?
A6) Union
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Q7) How do you access the members of the union?
A7) Access member of union
● Array elements are accessed using the Subscript variable , Similarly Union members are accessed using dot [.] operator.
● (.) is called as “union member Operator”.
● Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P- garbage value, ABC
Q8) Compare structure and union?
A8) Structure Vs Union
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Q9) Write a program to store information of students and display it?
A9) Program
#include <stdio.h>
#include <string.h>
Union Student
{
Int age;
Char Name[50];
Char Department[20];
};
Int main()
{
Union student stud1;
Union Employee stud2;
Stud1.age = 28;
Strcpy(stud1.Name, "Chris");
Strcpy(stud1.Department, "Science");
Printf("\nDetails of the First Student \n");
Printf(" Student Age = %d \n", emp1.age);
Printf(" Student Name = %s \n", emp1.Name);
Printf(" Student Department = %s \n", emp1.Department);
Printf("Details of the Second Student \n" );
Stud2.age = 30;
Printf(" Student Age = %d \n", stud2.age );
Strcpy(stud2.Name, "David");
Printf(" Student Name = %s \n", stud2.Name );
Strcpy(stud2.Department, "Technology" );
Printf(" Student Department = %s \n ", stud2.Department );
Return 0;
}
OUTPUT:
Details of the first student
Student Age = 28
Student Name = Chris
Student Department = Science
Details of the Second Student
Student Age =30
Student Name = David
Student Department= Technology
Q10) Write the advantages and disadvantages of structure?
A10) Advantages of structure
● Structures gather more than one piece of data about the same subject together in the same place.
● It is helpful when you want to gather the data of similar data types and parameters like first name, last name, etc.
● It is very easy to maintain as we can represent the whole record by using a single name.
● In structure, we can pass a complete set of records to any function using a single parameter.
● You can use an array of structure to store more records with similar types.
Disadvantages of structure
● If the complexity of an IT project goes beyond the limit, it becomes hard to manage.
● Change of one data structure in a code necessitates changes at many other places. Therefore, the changes become hard to track.
● Structure is slower because it requires storage space for all the data.
● You can retrieve any member at a time in structure whereas you can access one member at a time in the union.
● Structure occupies space for each and every member written in inner parameters while union occupies space for a member having the highest size written in inner parameters.
● Structure supports a flexible array. Union does not support a flexible array.
Q11) Write an example for calloc ()?
A11) Program
Example of calloc()
#include<stdio.h>
#include<stdlib.h>
Int main()
{
Int n,i,*ptr,sum=0;
Printf("Enter number of elements: ");
Scanf("%d",&n);
Ptr=(int*)calloc(n,sizeof(int)); //memory allocated using calloc
If(ptr==NULL)
{
Printf("Sorry! unable to allocate memory");
Exit(0);
}
Printf("Enter elements of array: ");
For(i=0;i<n;++i)
{
Scanf("%d",ptr+i);
Sum+=*(ptr+i);
}
Printf("Sum=%d",sum);
Free(ptr);
Return 0;
}
Output
Enter elements of array: 3
Enter elements of array: 10
10
10
Sum=30
Q12) Explain nested structure?
A12) The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address.
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by
Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Q13) Write a program for nested strcuture?
A13) Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
Q14) Describe an Array of structure with an example?
A14) In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
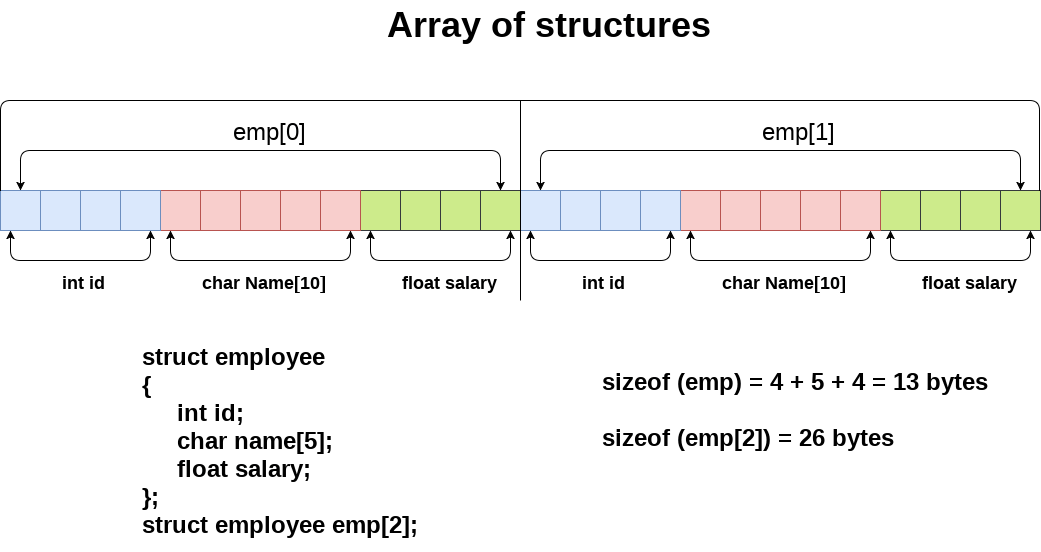
Fig 1: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Q15) Write a Program to access the structure member using the structure pointer and arrow (->) operator?
A15) Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include <stdio.h>
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
Q16) Write a Program to access the structure member using structure pointer and the dot operator?
A16) Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
Q17) What is sizeof ()?
A17) In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
Q18) Define typedef?
A18) typedef
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Q19) Write notes on - fseek(), ftell() and rewind()?
A19) fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
Q20) What are the formatted input?
A20) scanf()
We utilize the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.