Unit - 3
Constructors
Q1) Define Constructors.
A1)
In C++, constructor is a special method which is invoked automatically at the time of object creation. It is used to initialize the data members of new object generally. The constructor in C++ has the same name as class or structure.
- It is a member function of a class with same name as that of a class.
Class example
{
Int x,y;
Public:
Example() //constructor, same name as that of class
{
}
Void display()
{
:
}
};
- It is used to initialize data members of class.
Class example
{
Int x,y;
Public:
Example() //constructor initializing data members of class
{
x=10;
y=20;
}
Void display()
{
:
}
};
- It is always declared under public section.
Class example
{
Private:
Int x,y;
Example() // error, constructor declared under private section
{
x=10;
y=20;
}
:
:
};
- It does not have return type and hence cannot return any value
Class example
{
Int x, y;
Public:
Void example() // error, constructor does not have return type
{
x=10;
y=20;
}
:
:
};
- It is invoked automatically when object of class is created and initialize all data members of a class.
Class example
{
Int x, y;
Public:
Example() // constructor
{
x=10;
y=20;
}
Void display()
{
Cout<<x<<y;
}
};
Int main()
{
Example obj; //invokes constructor and initialize data
Member x and y to 10 and 20 respectively.
Obj.display();
Return 0 ;
}
- It cannot be declared as static, const and virtual.
- It can be defined inside a class or outside the class. While defining it outside the class scope resolution operator is used.
Class example
{
Int x, y;
Public:
Example(); // constructor declared
:
:
};
Example::example();// constructor defined outside class
{
x=10;
y=20;
}
Q2) What are the types of constructors?
A2)
Types of Constructors
Types of constructors with syntax
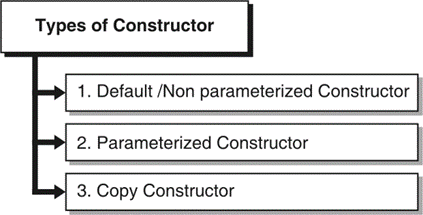
Fig: Types of Constructor
Default /Non parameterized Constructor
A constructor which has no argument is known as default constructor. It is invoked at the time of creating object.
- If constructor is not defined in program then compiler supplies default constructor.
Class Volume
{
Int x;
Public:
Volume () // default constructor
{
x=10;
}
Void display()
{
Cout<<x*x*x;
}
};
Void main()
{
Volume Obj; //invokes default constructor
Obj.display();
}
Let's see the simple example of C++ default Constructor.
- #include <iostream>
- Using namespace std;
- Class Employee
- {
- Public:
- Employee()
- {
- Cout<<"Default Constructor Invoked"<<endl;
- }
- };
- Int main(void)
- {
- Employee e1; //creating an object of Employee
- Employee e2;
- Return 0;
- }
Output:
Default Constructor Invoked
Default Constructor Invoked
Parameterized Constructor
A constructor which has parameters is called parameterized constructor. It is used to provide different values to distinct objects.
Class Volume {
Int x;
Public:
Volume ( int a) // parameterized constructor
{
x=a;
}
Void display()
{
Cout<<x*x*x;
}
};
Int main()
{
Volume obj(10); //invokes parameterized constructor
And pass argument 10 to it.
Obj.display();
}
Let's see the simple example of C++ Parameterized Constructor.
#include <iostream>
Using namespace std;
Class Employee {
public:
int id;//data member (also instance variable)
string name;//data member(also instance variable)
float salary;
Employee(int i, string n, float s)
{
id = i;
name = n;
salary = s;
}
void display()
{
cout<<id<<" "<<name<<" "<<salary<<endl;
}
};
Int main(void) {
Employee e1 =Employee(101, "Sonoo", 890000); //creating an object of Employee
Employee e2=Employee(102, "Nakul", 59000);
e1.display();
e2.display();
return 0;
}
Output:
101 Sonoo 890000
102 Nakul 59000
Copy Constructor
Copy constructor initializes an object using values of another object passed to it as a parameter.
Class Volume
{
Int x;
Public:
Volume ( int & P) // copy constructor
{
x=P.x;//copies value
}
Void display()
{
Cout<<x*x*x;
}
};
Int main()
{
Volume obj1; //invokes default constructor
Volume obj2(obj1);//calls copy constructor
Obj2.display();
}
Q3) What is Constructor Overloading?
A3)
Constructors can be overloaded just like member functions. Thus we can have multiple constructors in a class.
Write a C++ program to find volume of cube, cylinder.
Solution:
#include<iostream>
Using namespace std ;
Class Volume//Defines class Volume
{
Int x,y,z;
Public:
Volume ( int a) // Step 1Constructor with one argument
{
x=a;
}
Volume ( int a, int b) // Step 2 Constructor with two arguments
{
x=a;
y=b;
}
Void displaycube();
Void displaycylinder();
};
Void Volume::displaycube()
// Step 3 Function to calculate volume of cube
{
Cout<<x*x*x;
}
Void Volume::displaycylinder()
//Step 4 Function to calculate volume of cylinder
{
Cout<<3.14*x*x*y;
}
Int main()
{
Volume obj1(10);
// Step 5Calls constructor with one argument.
Volume obj2(10,5);
// Step 6 Calls constructor with two arguments.
Obj1. Displaycube ();//Step 7
Obj2. Displaycylinder ();
Return 0 ;
}
Explanation
This program calculates volume of cube and cylinder. For this we use two constructors in a class. One constructor is to initialize data member for cube and other to initialize data members for cylinder.
Step 1: Defines constructor with one argument. Here x stands for side of a cube.
Volume ( int a) { x=a; } | //Constructor with one argument
|
Step 2: Defines constructor with two arguments. Here x and y specifies radius and height.
Volume ( int a, int b) { x=a; y=b; } | //Constructor with two arguments
|
Step 3: Defines Function to calculate volume of cube.
Void Volume::displaycube() { Cout<<x*x*x; } | //Function to calculate volume of cube
|
Step 4: Defines Function to calculate volume of cylinder
Void Volume::displaycylinder() { Cout<<3.14*x*x*y; } | //Function to calculate volume of cylinder
|
Step 5: Here we want to initialize side of cube so we pass only one argument to invoke constructor with one argument.
Volume obj1(10);
Step 6: Here we want to initialize radius and height of cylinder so we pass two arguments to invoke constructor with two arguments.
Volume obj2(10,5);
Step 7: Calls functions to calculate volume of a cube and cylinder.
Obj1. Displaycube ();
Obj2. Displaycylinder ();
Q4) What are Destructors?
A4)
- It is a special member function that is called when lifetime of an object ends.
- Destructor is used to free the resources occupied by an object.
- It is called automatically when the object is no longer needed.
- It has same name as that of class preceded by tilde(~).
Class example
{
Public:
~example();//destructor
};
- It is always declared or defined under public section.
- It neither returns any value nor takes any argument.
- It cannot be declared as static const and volatile.
C++ Constructor and Destructor Example
Let's see an example of constructor and destructor in C++ which is called automatically.
- #include <iostream>
- Using namespace std;
- Class Employee
- {
- Public:
- Employee()
- {
- Cout<<"Constructor Invoked"<<endl;
- }
- ~Employee()
- {
- Cout<<"Destructor Invoked"<<endl;
- }
- };
- Int main(void)
- {
- Employee e1; //creating an object of Employee
- Employee e2; //creating an object of Employee
- Return 0;
- }
Output:
Constructor Invoked
Constructor Invoked
Destructor Invoked
Destructor Invoked
Q5) Explain Operator overloading.
A5)
C++ Operators Overloading
Operator overloading is a compile-time polymorphism in which the operator is overloaded to provide the special meaning to the user-defined data type. Operator overloading is used to overload or redefines most of the operators available in C++. It is used to perform the operation on the user-defined data type. For example, C++ provides the ability to add the variables of the user-defined data type that is applied to the built-in data types.
The advantage of Operators overloading is to perform different operations on the same operand.
Operator that cannot be overloaded are as follows:
- Scope operator (::)
- Sizeof
- Member selector(.)
- Member pointer selector(*)
- Ternary operator(?:)
Syntax of Operator Overloading
- Return_type class_name : : operator op(argument_list)
- {
- // body of the function.
- }
Where the return type is the type of value returned by the function.
Class_name is the name of the class.
Operator op is an operator function where op is the operator being overloaded, and the operator is the keyword.
Q6) Write a program in C++ to overload unary minus(-).
A6)
- #include <iostream>
- Using namespace std;
- Class Test
- {
- Private:
- Int num;
- Public:
- Test(): num(8){}
- Void operator ++() {
- Num = num+2;
- }
- Void Print() {
- Cout<<"The Count is: "<<num;
- }
- };
- Int main()
- {
- Test tt;
- ++tt; // calling of a function "void operator ++()"
- Tt.Print();
- Return 0;
- }
Output:
The Count is: 10
Q7) Write a program in C++ to overload binary operators.
A7)
- #include <iostream>
- Using namespace std;
- Class A
- {
- Int x;
- Public:
- A(){}
- A(int i)
- {
- x=i;
- }
- Void operator+(A);
- Void display();
- };
- Void A :: operator+(A a)
- {
- Int m = x+a.x;
- Cout<<"The result of the addition of two objects is : "<<m;
- }
- Int main()
- {
- A a1(5);
- A a2(4);
- a1+a2;
- Return 0;
- }
Output:
The result of the addition of two objects is : 9
Q8) What is the need of Operator Overloading?
A8)
Introduction and Need of Operator Overloading
- Operators have fixed meaning and functionalities for primitive data types like int, float, double etc.
- These operators cannot be used for user defined data types like classes.
- But the functionality of these operators can be extended to user defined data types like classes.
- Operator ‘+’ is used to add two integers but we can add two objects by extending the meaning of ‘+’ operator.
- This is called as operator overloading.
- We can overload operators so that they can perform operation on objects.
- Operator overloading provides special meaning to built in operators to perform some specific computation when the operator is used on objects of that class.
- All C++ operators can be overloaded except following:
- Class member access operator(.*)
- Conditional operator(?:)
- Scope resolution operator(::)
- Size of operator(sizeof)
Q9) Explain Operator Function.
A9)
- Operator is overloaded with the help of operator function.
- It defines the operations to be performed by overloaded operator.
- This operator function can be of two types
1. Member operator function
2. Non Member operator function
- Non member operator functions are usually friend functions of class. Operator functions are discussed in preceding section.
Overloading using Member Operator Function
Syntax of an operator function
- Member operator function is a member of the class.
- It is declared in a class as follows:
Return-type operator OP(argument list);
Where OP -> operator to be overloaded.
- Number of parameters in an argument list depends on the type of operator.
Type of Operator | No. Of Arguments |
Unary | 0 |
Binary | 1 |
- Thus according to above table, overloading unary operators using member functions takes no argument and binary operator takes one argument.
- It is defined as follows:
Return-type class-name :: operator OP(argument list)
{
Operations that overloaded operator performs
}
Q10) Write a program in C++ to overload unary minus(-).
A10)
#include<iostream> Using namespace std ; Class Example { Int x,y,z; Public:
Example(int p,int q,int r) { x=p; y=q; z=r; } Void display() { Cout<<x<<” ”<<y<<” ”<<z; } Void operator-() { x=-x; y=-y; z=-z; } }; Int main() { Example obj (10,-20,30); Cout<<”Original numbers are” Obj.display(); - obj; Obj.display(); Return 0; } |
//Defines class Example
//Parameterized constructor
// Step 1 Operator function to overload minus
// Step 2 Invokes operator function
Cout<<”Numbers after applying unary minus” |
Explanation
- This program overloads unary – on data members of class Example.
- It defines operator function to overload unary – so that it can be applied to object. See step 1.
Void operator-() { x=-x; y=-y; z=-z; } | //Operator function to overload minus
|
- This operator function is invoked by applying unary – on object ‘obj’. See step 2.
- obj; Cout<<”Numbers after applying unary minus” | //Invokes operator function |
Output
Original numbers are
10 -20 30
Numbers after applying unary minus
-10 20 -30
Q11) Write a C++ program to overload binary +, - .
A11)
#include<iostream> Using namespace std ; Class Example { Int a,b; Public: Example(int p, int q) { a=p; b=q; } Void display() { Cout<<a<<” ”<<b<<endl; } Example operator+(Example X); Example operator-(Example X); }; Example Example::operator+(Example X) { Example E; E.a= a+X.a; E.b= b+X.b; Return E } Example Example:: operator-(Example X) { Example F; F.a= a-X.a; F.b= b-X.b; Return F } Int main() { Example obj1(10,20); Example obj2(5,5); Example obj3(0,0); Cout<<”Addition is” Obj3= obj1+ obj2; Obj3.display(); Cout<<”Subtraction is” Obj3= obj1- obj2; Obj3.display(); Return 0 ; } |
// Step 1 overloads + // Step 1 overloads -
// Step 2 Defines operator function to overload +
//Defines operator function to overload -
// Step 3 //Invokes overloaded binary +
// Step4 //Invokes overloaded binary -
|
Explanation
- This program overloads binary + and -. For this operator functions are declared in class. See step 1.
- This operator function returns objects which stores addition of two objects.
Return type of an object is always a class so it is declared as follows
Example operator+(Example X); Example operator-(Example X); | //overloads + //overloads - |
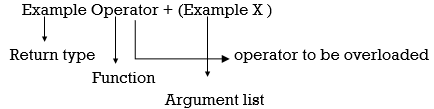
- Operator functions are defined outside class using scope resolution operator. See step 2.
Example Example:: operator+(Example X) { Example E; E.a= a+X.a; E.b= b+X.b; Return E; } Example Example:: operator-(Example X) { Example F; F.a= a-X.a; F.b= b-X.b; Return F; } | //Defines operator function to overload +
//Defines operator function to overload -
|
- Operator function takes only one argument as left hand side operand is passed implicitly via this pointer.
- This operator function returns object where addition is stored.
- Return type of an object is always a class so it is declared as follows:
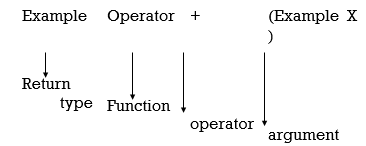
- Operator function for addition is activated when two objects are added using +. See
step 3.
O3= O1+ O2; //Invokes overloaded binary +
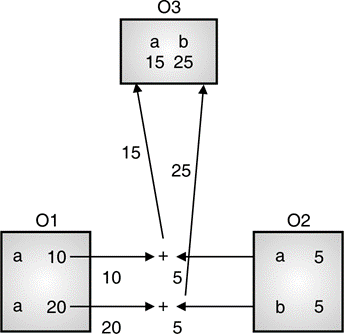
- Operator function for subtraction is activated when two objects are subtracted using -. See step 4.
O3= O1- O2;//Invokes overloaded binary -
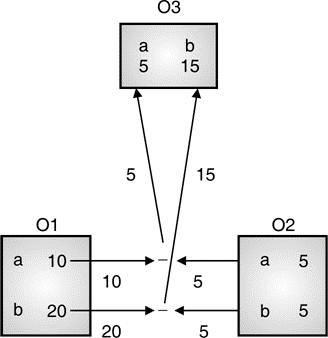
Thus the output will be:
Output
Cout<<”Addition is”
a 15 b 25
Cout<<”Subtraction is”
a 5 b 15
Q12) Write C++ program to demonstrate use of binary (“+”) operator overloading to add two complex numbers using member function.
A12)
#include<iostream> Using namespace std ; Class Demo { Int real,imag; Public: Demo(int p, int q) { Real=p; Imag=q; } Void display() { Cout<<real<<” +j”<<imag<<endl; } Demo operator+(Demo X); }; Demo Demo::operator+(Demo X) { Demo D; D.real=real+X.real; D.imag=imag+D.imag; Return D } Int main() { Demo obj1(10,20); Demo obj2(5,5); Demo obj3(0,0); Cout<<”Addition is” Obj3= obj1+ obj2; Obj3.display(); Return 0 ; } |
//……1overloads +
//.2 Defines operator function to overload +
//Defines operator function to overload -
//…..3 //Invokes overloaded binary +
|
Q13) Explain Binary Operators.
A13)
- Operator function to overload binary operator using friend function takes two arguments.
- E.g. Friend void operator-(Example O1, Example O2);
#include<iostream> Using namespace std ; Class Point { Int x,y; Public: Point() {} Point(int p, int q) { x=p; y=q; } Void display() { Cout<<x<<” ”<<y<<endl; } Friend Point operator+(Point P1,Point P2); Friend Point operator-(Point P1,Point P2); }; Point operator+(Point P1,Point P2) { Point E; E.x= P1.x+P2.x; E.y= P1.y+P2.y; Return E; } Point operator-(Point P1,Point P2) { Point E; E.x= P1.x-P2.x; E.y= P1.y-P2.y; Return E; } Int main() { Example obj1(10,20); Example obj2(5,5); Example obj3(0,0); Obj3= obj1+ obj2; Obj3.display(); Obj3= obj1- obj2; Obj3.display(); Return 0; } |
//Step 1 overloads + using friend
// Step 2 overloads – using friend //Defines operator function to overload +
//Defines operator function to overload -
//Invokes overloaded binary +
//Invokes overloaded binary - |
Explanation
- Here we are overloading binary operators using friend, hence it takes two arguments. Here arguments are objects as usually friend functions take object as arguments. See steps 1 and 2.
Friend Point operator+(Point P1,Point P2); //overloads + using friend
Friend Point operator-(Point P1,Point P2);//overloads – using friend
- Operator function returns object E. Return type of object is always a class. Hence return type of operation function will be class “Point” to which object “E” belongs.
- Hence friend function is declared as follows:
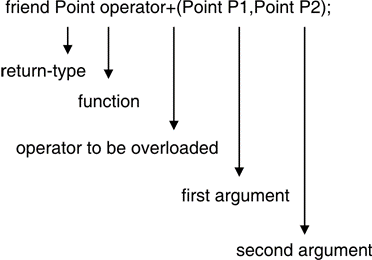
- Following operators cannot be overloaded using friends:
1.() Function call operator
2.= Assignment operator
3.[] subscripting operator
4.-> class member access operator
- The chart given below summarizes no of arguments in operator function:
Type of operator Type Of operator Function | Unary | Binary |
Member Function | 0 | 1 |
Friend Function | 1 | 2 |
Q14) Write a C++ program to add the complex numbers using friend function.
A14)
#include<iostream> Using namespace std ; Class Example { Int real, imag; Public: Example(int p, int q) { Real=p; Imag=q; } Void display() { Cout<<real<<“”<<imag<<endl; } Friend Example operator+(Example X1,Example X2); }; Example operator+(Example X1, Example X2) { Example E; E.real= X1.real+X2.real; E.imag= X1.imag+X2.imag; Return E; } Int main() { Example obj1(10,20); Example obj2(5,5); Example obj3(0,0); Cout<<“Addition is” Obj3= obj1+ obj2; Obj 3.display(); Return 0; }
|
//Defines operator function to overload+
//Invokes overloaded binary+
|
Q15) Write a C++ program to subtract 2 complex numbers using concept of overloading using friends function.
A15)
#include<iostream> Using namespace std ; Class Example { Int real, imag; Public: Example(int p, int q) { Real=p; Imag=q; } Void display() { Cout<<real<<”+j”<<imag<<endl; } Friend Example operator-(Example X1,Example X2); }; Example operator-(Example X1, Example X2) { Example E; E.real= X1.real-X2.real; E.imag= X1.imag-X2.imag; Return E; } Int main() { Example obj1(10,20); Example obj2(5,5); Example obj3(0,0); Cout<<“Subtraction is” Obj3= obj1- obj2; Obj3.display(); Return 0; } |
//Defines operator function to overload -
//Invokes overloaded binary -
|
Q16) Write about Overloading operators using friend function.
A16)
- Friend function using operator overloading offers better flexibility to the class.
- These functions are not a members of the class and they do not have 'this' pointer.
- When you overload a unary operator you have to pass one argument.
- When you overload a binary operator you have to pass two arguments.
- Friend function can access private members of a class directly.
Syntax:
Friend return-type operator operator-symbol (Variable 1, Varibale2)
{
//Statements;
}
Example: Program demonstrating Unary operator overloading using Friend function
#include<iostream>
Using namespace std;
Class UnaryFriend
{
int a=10;
int b=20;
int c=30;
public:
void getvalues()
{
cout<<"Values of A, B & C\n";
cout<<a<<"\n"<<b<<"\n"<<c<<"\n"<<endl;
}
void show()
{
cout<<a<<"\n"<<b<<"\n"<<c<<"\n"<<endl;
}
void friend operator-(UnaryFriend &x); //Pass by reference
};
Void operator-(UnaryFriend &x)
{
x.a = -x.a; //Object name must be used as it is a friend function
x.b = -x.b;
x.c = -x.c;
}
Int main()
{
UnaryFriend x1;
x1.getvalues();
cout<<"Before Overloading\n";
x1.show();
cout<<"After Overloading \n";
-x1;
x1.show();
return 0;
}
Output:
Values of A, B & C
10
20
30
Before Overloading
10
20
30
After Overloading
-10
-20
-30
In the above program, operator – is overloaded using friend function. The operator() function is defined as a Friend function. The statement -x1 invokes the operator() function. The object x1 is created of class UnaryFriend. The object itself acts as a source and destination object. This can be accomplished by sending reference of an object. The object x1 is a reference of object x. The values of object x1 are replaced by itself by applying negation.
Q17) What are the rules for overloading operator?
A17)
Operators which cannot be overloaded
All C++ operators can be overloaded except following
- Class member access operator(.*)
- Conditional operator(?:)
- Scope resolution operator(::)
- Size of operator(sizeof)
Operators which cannot be overloaded by using Friend Function
Following operators cannot be overloaded using friends
- () Function call operator
- = Assignment operator
- [] subscripting operator
- -> class member access operator
Rules for operator overloading
- Some operators like (assignment)=, (address)& and comma (,) are by default overloaded.
- We cannot create new operators for overloading. Only built in operators can be overloaded.
- We cannot redefine meaning of in built operators i.e we cannot change the meaning +, - etc.
- Precedence and associativity of operators cannot be changed.
- Overloaded operators cannot have default arguments.
- Operators overloaded using member functions takes no argument for unary and one argument for binary operator.
- Operators overloaded using friend functions takes one argument for unary and two arguments for binary operator.
Q18) Write a C++ program to find volume of cube, cylinder.
A18)
#include<iostream>
Using namespace std ;
Class Volume//Defines class Volume
{
Int x,y,z;
Public:
Volume ( int a) // Step 1Constructor with one argument
{
x=a;
}
Volume ( int a, int b) // Step 2 Constructor with two arguments
{
x=a;
y=b;
}
Void displaycube();
Void displaycylinder();
};
Void Volume::displaycube()
// Step 3 Function to calculate volume of cube
{
Cout<<x*x*x;
}
Void Volume::displaycylinder()
//Step 4 Function to calculate volume of cylinder
{
Cout<<3.14*x*x*y;
}
Int main()
{
Volume obj1(10);
// Step 5Calls constructor with one argument.
Volume obj2(10,5);
// Step 6 Calls constructor with two arguments.
Obj1. Displaycube ();//Step 7
Obj2. Displaycylinder ();
Return 0 ;
}
Explanation
This program calculates volume of cube and cylinder. For this we use two constructors in a class. One constructor is to initialize data member for cube and other to initialize data members for cylinder.
Step 1: Defines constructor with one argument. Here x stands for side of a cube.
Volume ( int a) { x=a; } | //Constructor with one argument
|
Step 2: Defines constructor with two arguments. Here x and y specifies radius and height.
Volume ( int a, int b) { x=a; y=b; } | //Constructor with two arguments
|
Step 3: Defines Function to calculate volume of cube.
Void Volume::displaycube() { Cout<<x*x*x; } | //Function to calculate volume of cube
|
Step 4: Defines Function to calculate volume of cylinder
Void Volume::displaycylinder() { Cout<<3.14*x*x*y; } | //Function to calculate volume of cylinder
|
Step 5: Here we want to initialize side of cube so we pass only one argument to invoke constructor with one argument.
Volume obj1(10);
Step 6: Here we want to initialize radius and height of cylinder so we pass two arguments to invoke constructor with two arguments.
Volume obj2(10,5);
Step 7: Calls functions to calculate volume of a cube and cylinder.
Obj1. Displaycube ();
Obj2. Displaycylinder ();
Q19) Write example of C++ Constructor and Destructor.
A19)
Let's see an example of constructor and destructor in C++ which is called automatically.
21. #include <iostream>
22. using namespace std;
23. class Employee
24. {
25. public:
26. Employee()
27. {
28. cout<<"Constructor Invoked"<<endl;
29. }
30. ~Employee()
31. {
32. cout<<"Destructor Invoked"<<endl;
33. }
34. };
35. int main(void)
36. {
37. Employee e1; //creating an object of Employee
38. Employee e2; //creating an object of Employee
39. return 0;
40. }
Output:
Constructor Invoked
Constructor Invoked
Destructor Invoked
Destructor Invoked
Q20) Write Syntax of an operator function.
A20)
Syntax of an operator function
- Member operator function is a member of the class.
- It is declared in a class as follows:
Return-type operator OP(argument list);
Where OP -> operator to be overloaded.
- Number of parameters in an argument list depends on the type of operator.
Type of Operator | No. Of Arguments |
Unary | 0 |
Binary | 1 |
- Thus according to above table, overloading unary operators using member functions takes no argument and binary operator takes one argument.
- It is defined as follows:
Return-type class-name :: operator OP(argument list)
{
Operations that overloaded operator performs
}