Unit - 1
Java Language Basics
Q1) What is an object in Java?
A1) An entity that has state and behavior is known as an object e.g., chair, bike, marker, pen, table, car, etc. It can be physical or logical (tangible and intangible).
The example of an intangible object is the banking system.
An object has three characteristics:
a) State: represents the data (value) of an object.
b) Behavior: represents the behavior (functionality) of an object such as deposit, withdraw, etc.
c) Identity: An object identity is typically implemented via a unique ID. The value of the ID is not visible to the external user. It is used internally by the JVM to identify each uniquely.
An object is an instance of a class. A class is a template or blueprint from which objects are created. So, an object is the instance(result) of a class.
Object Definitions:
- An object is a real-world entity.
- An object is a runtime entity.
- The object is an entity which has state and behavior.
- The object is an instance of a class.
Q2) What is a class in Java?
A2) A class is a group of objects which have common properties. It is a template or blueprint from which objects are created. It is a logical entity. It can't be physical.
A class in Java can contain:
● Fields
● Methods
● Constructors
● Blocks
● Nested class and interface
Syntax of the class:
Class<class_name>
{ No. Of fields;
No.of methods;
}
Q3) What is the constructor?
A3) The constructor can be defined as the special type of method that is used to initialize the state of an object.
It is invoked when the class is instantiated, and the memory is allocated for the object. Every time, an object is created using the new keyword, the default constructor of the class is called. The name of the constructor must be similar to the class name. The constructor must not have an explicit return type.
There are two types of constructors in Java:
1. Default constructor (no-arg constructor)
A constructor is called "Default Constructor" when it doesn't have any parameter.
Syntax of default constructor:
<class_name>(){ }
Example of default constructor that displays the default values
//Let us see example of default constructor which displays the default values
Class Student3{
Int id;
String name;
//method to display the value of id and name
Void display()
{ System.out.println(id+" "+name); }
Public static void main(String args[])
{ //creating objects
Student3 s1=new Student3();
Student3 s2=new Student3();
//displaying values of the object
s1.display();
s2.display();
}
}
OUTPUT:
0 null
0 null
Explanation:
In the above class, you are not creating any constructor so compiler provides you a default constructor. Here 0 and null values are provided by default constructor.
2. Parameterized Constructor
A constructor which has a specific number of parameters is called a parameterized constructor.
The parameterized constructor is used to provide different values to distinct objects. However, you can provide the same values also.
Example of parameterized constructor
//Java Program to demonstrate the use of the parameterized constructor.
Class Student4{
Int id;
String name;
//creating a parameterized constructor
Student4(int i,String n){
Id = i;
Name = n;
}
//method to display the values
Void display(){System.out.println(id+" "+name);}
Public static void main(String args[])
{ //creating objects and passing values
Student4 s1 = new Student4(111,"Karan");
Student4 s2 = new Student4(222,"Aryan");
//calling method to display the values of object
s1.display();
s2.display();
}
}
OUTPUT:
111 Karan
222 Aryan
Rules for creating Java constructor
There are two rules defined for the constructor.
- Constructor name must be the same as its class name
- A Java constructor cannot be abstract, static, final, and synchronized
Q4) Explain the fundamentals of java?
A4) Java programming language was originally developed by Sun Microsystems which was initiated by James Gosling and released in 1995 as core component of Sun Microsystems' Java platform (Java 1.0 [J2SE]).
The latest release of the Java Standard Edition is Java SE 8. With the advancement of Java and its widespread popularity, multiple configurations were built to suit various types of platforms. For example: J2EE for Enterprise Applications, J2ME for Mobile Applications.
The new J2 versions were renamed as Java SE, Java EE, and Java ME respectively. Java is guaranteed to be Write Once, Run Anywhere.
Java is −
● Object Oriented− In Java, everything is an Object. Java can be easily extended since it is based on the Object model.
● Platform Independent− Unlike many other programming languages including C and C++, when Java is compiled, it is not compiled into platform specific machine, rather into platform independent byte code. This byte code is distributed over the web and interpreted by the Virtual Machine (JVM) on whichever platform it is being run on.
● Simple− Java is designed to be easy to learn. If you understand the basic concept of OOP Java, it would be easy to master.
● Secure− With Java's secure feature it enables to develop virus-free, tamper-free systems. Authentication techniques are based on public-key encryption.
● Architecture-neutral− Java compiler generates an architecture-neutral object file format, which makes the compiled code executable on many processors, with the presence of Java runtime system.
● Portable− Being architecture-neutral and having no implementation dependent aspects of the specification makes Java portable. Compiler in Java is written in ANSI C with a clean portability boundary, which is a POSIX subset.
● Robust− Java makes an effort to eliminate error prone situations by emphasizing mainly on compile time error checking and runtime checking.
● Multithreaded− With Java's multithreaded feature it is possible to write programs that can perform many tasks simultaneously. This design feature allows the developers to construct interactive applications that can run smoothly.
● Interpreted− Java byte code is translated on the fly to native machine instructions and is not stored anywhere. The development process is more rapid and analytical since the linking is an incremental and light-weight process.
● High Performance− With the use of Just-In-Time compilers, Java enables high performance.
● Distributed− Java is designed for the distributed environment of the internet.
● Dynamic− Java is considered to be more dynamic than C or C++ since it is designed to adapt to an evolving environment. Java programs can carry extensive amount of run-time information that can be used to verify and resolve accesses to objects on run-time.
Q5) Write the history of java?
A5) History of Java
James Gosling initiated Java language project in June 1991 for use in one of his many set-top box projects. The language, initially called ‘Oak’ after an oak tree that stood outside Gosling's office, also went by the name ‘Green’ and ended up later being renamed as Java, from a list of random words.
Sun released the first public implementation as Java 1.0 in 1995. It promised Write Once, Run Anywhere (WORA), providing no-cost run-times on popular platforms.
On 13 November, 2006, Sun released much of Java as free and open source software under the terms of the GNU General Public License (GPL).
On 8 May, 2007, Sun finished the process, making all of Java's core code free and open-source, aside from a small portion of code to which Sun did not hold the copyright.
Tools You Will Need
For performing the examples discussed in this tutorial, you will need a Pentium 200-MHz computer with a minimum of 64 MB of RAM (128 MB of RAM recommended).
You will also need the following software−
● Linux 7.1 or Windows xp/7/8 operating system
● Java JDK 8
● Microsoft Notepad or any other text editor
Q6) Explain array in java?
A6) Java array is an object which contains elements of a similar data type. Additionally, The elements of an array are stored in a contiguous memory location. It is a data structure where we store similar elements. We can store only a fixed set of elements in a Java array.
Array in Java is index-based, the first element of the array is stored at the 0th index, 2nd element is stored on 1st index and so on.
Unlike C/C++, we can get the length of the array using the length member. In C/C++, we need to use the sizeof operator.
In Java, array is an object of a dynamically generated class. Java array inherits the Object class, and implements the Serializable as well as Cloneable interfaces. We can store primitive values or objects in an array in Java. Like C/C++, we can also create single dimensional or multidimensional arrays in Java.
Moreover, Java provides the feature of anonymous arrays which is not available in C/C++.
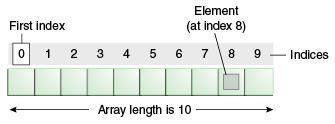
Advantages
● Code Optimization: It makes the code optimized, we can retrieve or sort the data efficiently.
● Random access: We can get any data located at an index position.
Disadvantages
● Size Limit: We can store only the fixed size of elements in the array. It doesn't grow its size at runtime. To solve this problem, collection framework is used in Java which grows automatically.
Q7) Write an Example of Java Array?
A7) Let's see the simple example of java array, where we are going to declare, instantiate, initialize and traverse an array.
- //Java Program to illustrate how to declare, instantiate, initialize
- //and traverse the Java array.
- Class Testarray{
- Public static void main(String args[]){
- Int a[]=new int[5];//declaration and instantiation
- a[0]=10;//initialization
- a[1]=20;
- a[2]=70;
- a[3]=40;
- a[4]=50;
- //traversing array
- For(int i=0;i<a.length;i++)//length is the property of array
- System.out.println(a[i]);
- }}
Output:
10
20
70
40
50
Q8) Explain multidimensional array in java?
A8) Multidimensional Array in Java
In such case, data is stored in row and column based index (also known as matrix form).
Syntax to Declare Multidimensional Array in Java
- DataType[][] arrayRefVar; (or)
- DataType [][]arrayRefVar; (or)
- DataType arrayRefVar[][]; (or)
- DataType []arrayRefVar[];
Example to instantiate Multidimensional Array in Java
- Int[][] arr=new int[3][3];//3 row and 3 column
Example to initialize Multidimensional Array in Java
- Arr[0][0]=1;
- Arr[0][1]=2;
- Arr[0][2]=3;
- Arr[1][0]=4;
- Arr[1][1]=5;
- Arr[1][2]=6;
- Arr[2][0]=7;
- Arr[2][1]=8;
- Arr[2][2]=9;
Example of Multidimensional Java Array
Let's see the simple example to declare, instantiate, initialize and print the 2 Dimensional array.
- //Java Program to illustrate the use of multidimensional array
- Class Testarray3{
- Public static void main(String args[]){
- //declaring and initializing 2D array
- Int arr[][]={{1,2,3},{2,4,5},{4,4,5}};
- //printing 2D array
- For(int i=0;i<3;i++){
- For(int j=0;j<3;j++){
- System.out.print(arr[i][j]+" ");
- }
- System.out.println();
- }
- }}
Output:
1 2 3
2 4 5
4 4 5
Q9) What are the main differences between the Java platform and other platforms?
A9) There are the following differences between the Java platform and other platforms
● Java is the software-based platform whereas other platforms may be the hardware platforms or software-based platforms.
● Java is executed on the top of other hardware platforms whereas other platforms can only have the hardware components.
Q10) List the features of Java Programming language?
A10) There are the following features in Java Programming Language.
- Simple: Java is easy to learn. The syntax of Java is based on C++ which makes easier to write the program in it.
- Object-Oriented: Java follows the object-oriented paradigm which allows us to maintain our code as the combination of different type of objects that incorporates both data and behavior.
- Portable: Java supports read-once-write-anywhere approach. We can execute the Java program on every machine. Java program (.java) is converted to bytecode (.class) which can be easily run on every machine.
- Platform Independent: Java is a platform independent programming language. It is different from other programming languages like C and C++ which needs a platform to be executed. Java comes with its platform on which its code is executed. Java doesn't depend upon the operating system to be executed.
- Interpreted: Java uses the Just-in-time (JIT) interpreter along with the compiler for the program execution.
- Secured: Java is secured because it doesn't use explicit pointers. Java also provides the concept of ByteCode and Exception handling which makes it more secured.
- Robust: Java is a strong programming language as it uses strong memory management. The concepts like Automatic garbage collection, Exception handling, etc. make it more robust.
- Architecture Neutral: Java is architectural neutral as it is not dependent on the architecture. In C, the size of data types may vary according to the architecture (32 bit or 64 bit) which doesn't exist in Java.
- High Performance: Java is faster than other traditional interpreted programming languages because Java bytecode is "close" to native code. It is still a little bit slower than a compiled language (e.g., C++).
- Multithreaded: We can write Java programs that deal with many tasks at once by defining multiple threads. The main advantage of multi-threading is that it doesn't occupy memory for each thread. It shares a common memory area. Threads are important for multimedia, Web applications, etc.
- Distributed: Java is distributed because it facilitates users to create distributed applications in Java. RMI and EJB are used for creating distributed applications. This feature of Java makes us able to access files by calling the methods from any machine on the internet.
- Dynamic: Java is a dynamic language. It supports dynamic loading of classes. It means classes are loaded on demand. It also supports functions from its native languages, i.e., C and C++.
Q11) What is JVM?
A11) Java virtual Machine (JVM) is a virtual Machine that provides runtime environment to execute java byte code.
● The JVM doesn't understand Java typo, that's why you compile your *.java files to obtain *.class files that contain the bytecodes understandable by the JVM.
● JVM control execution of every Java program.
It enables features such as automated exception handling, Garbage-collected heap.
JVM Architecture
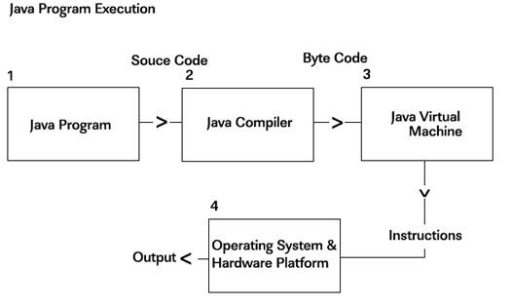
Q12) What are the various access specifiers in Java?
A12) In Java, there are four access specifiers given below:
a) Public: The classes, methods, or variables which are defined as public, can be accessed by any class or method.
b) Protected: Protected can be accessed by the class of the same package, or by the sub-class of this class, or within the same class.
c) Default: Default are accessible within the package only. By default, all the classes, methods, and variables are of default scope.
d) Private: The private class, methods, or variables defined as private can be accessed within the class only.
Q13) What are Operators? Different types of operators used in java?
A13) Operator in Java is a symbol that is used to perform operations. For example: +, -, *, / etc.
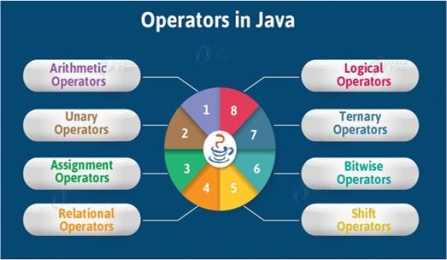
Operator Type | Category | Precedence |
Unary | Postfix | Expr++ expr-- |
Prefix | ++expr --expr +expr -expr ~! | |
Arithmetic | Multiplicative | * / % |
Additive | + - | |
Shift | Shift | << >> >>> |
Relational | Comparison | < > <= >= instance of |
Equality | == != | |
Bitwise | Bitwise AND | & |
Bitwise exclusive OR | ^ | |
Bitwise inclusive OR | | | |
Logical | Logical AND | && |
Logical OR | || | |
Ternary | Ternary | ? : |
Assignment | Assignment | = += -= *= /= %= &= ^= |= <<= >>= >>>= |
Q14) What is the output of the following Java program?
Class Test
{
Public static void main (String args[])
{
System.out.println(10 * 20 + "Javamy");
System.out.println("Javamy" + 10 * 20);
}
}
A14) The output of the above code will be
200Javamy
Javamy200
Explanation
In the first case, the numbers 10 and 20 will be multiplied first and then the result 200 is treated as the string and concatenated with the string Javamy to produce the output 200Javamy.
In the second case, the numbers 10 and 20 will be multiplied first to be 200 because the precedence of the multiplication is higher than addition. The result 200 will be treated as the string and concatenated with the string Javamy to produce the output as Javamy.
Q15) What are variables? Different types of variables used in java?
A15) A variable is the name of a reserved area allocated in memory. In other words, it is a name of the memory location.
1)Local Variable
A variable declared inside the body of the method is called local variable. You can use this variable only within that method and the other methods in the class aren't even aware that the variable exists.
A local variable cannot be defined with "static" keyword.
2) Instance Variable
A variable declared inside the class but outside the body of the method, is called an instance variable. It is not declared as static.
It is called an instance variable because its value is instance-specific and is not shared among instances.
3) Static variable
A variable that is declared as static is called a static variable. It cannot be local. You can create a single copy of the static variable and share it among all the instances of the class. Memory allocation for static variables happens only once when the class is loaded in the memory.
Q16) How many data types are used in java?
A16) Data types specify the different sizes and values that can be stored in the variable. There are two types of data types in Java:
- Primitive data types: The primitive data types include boolean, char, byte, short, int, long, float and double.
- Non-primitive data types: The non-primitive data types include Classes, Interfaces, and Arrays.
Data Type | Default Value | Default size |
Boolean | False | 1 bit |
Char | '\u0000' | 2 byte |
Byte | 0 | 1 byte |
Short | 0 | 2 byte |
Int | 0 | 4 byte |
Long | 0L | 8 byte |
Float | 0.0f | 4 byte |
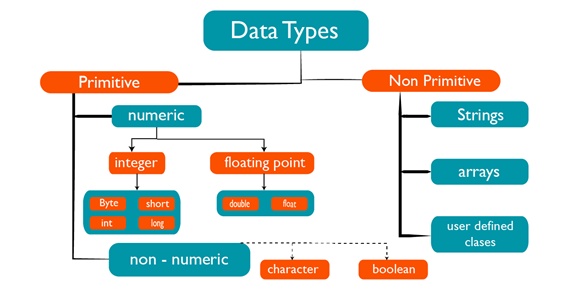
Q17) Describe java methods?
A17) In general, a method is a manner of accomplishing a goal. In Java, a method is a collection of instructions that accomplishes a specified goal. It ensures that code can be reused. Methods can also be used to easily alter code. We'll study what a method is in Java, the different sorts of methods, how to declare a method, and how to invoke a method in Java in this part.
What is a method in Java?
A method is a collection of statements or a series of statements organised together to conduct a specific task or action. It's a technique for making code more reusable. We create a method once and then use it repeatedly. We don't have to write code over and over again.
It also allows for easy code modification and readability by simply adding or removing code chunks. Only when we call or invoke the method is it executed.
Method Declaration
Method properties such as visibility, return-type, name, and parameters are all stated in the method declaration. As seen in the following diagram, it consists of six components known as method headers.
Fig: Method declaration
Method Signature: A method signature is a description of a method. It's included in the method declaration. It contains the method name as well as a list of parameters.
Access Specifier: The method's access specifier or modifier is the method's access type. It specifies the method's visibility. There are four different types of access specifiers in Java:
● public - When we utilise the public specifier in our application, all classes can access the method.
● private - The method is only accessible in the classes in which it is declared when we use a private access specifier.
● protected - The method is accessible within the same package or subclasses in a different package when we use the protected access specifier.
● default - When no access specifier is specified in the method declaration, Java uses the default access specifier. It is visible only from the same package only.
Return Type: The data type that the method returns is known as the return type. It could be a primitive data type, an object, a collection, or void, for example. The void keyword is used when a method does not return anything.
Method Name: It's a one-of-a-kind moniker that's used to specify a method's name. It must be appropriate for the method's functionality. If we're making a method for subtracting two numbers, the name of the method must be subtraction (). The name of a method is used to call it.
Parameter List: It consists of a list of parameters separated by a comma and contained in parentheses. It specifies the data type as well as the name of the variable. Leave the parenthesis blank if the method has no parameters.
Method Body: It's included in the method declaration. It contains all of the actions that must be completed. It is protected by a pair of curly braces.
Q18) Write the types of methods?
A18) There are two types of methods in Java:
● Predefined Method
● User-defined Method
Predefined Method
Predefined methods in Java are methods that are previously defined in the Java class libraries. It's also known as the built-in method or the standard library approach. We can use these methods directly by calling them at any time in the application. Length(), equals(), compareTo(), sqrt(), and other predefined methods are examples. When we call any of the predefined methods in our software, a set of codes relevant to that method run in the background, which are already saved in the library.
Every predefined method is contained within a class. The java.io.PrintStream class, for example, defines the print() function. It outputs the statement we typed into the method. For example, print("Java"), it prints Java on the console.
Example
Demo.java
Public class Demo
{
Public static void main(String[] args)
{
// using the max() method of Math class
System.out.print("The maximum number is: " + Math.max(9,7));
}
}
Output
The maximum number is: 9
We utilized three predefined methods in the preceding example: main(), print(), and max() (). Because these methods are predefined, we have utilised them without declaring them. The PrintStream class has a print() function that prints the result to the console. The greater of two numbers is returned by the max() function of the Math class.
User-defined Method
A user-defined method is a method that is written by the user or programmer. These strategies are tweaked to meet the needs of the situation.
How to Create a User-defined Method
Let's build a user-defined mechanism for determining whether an integer is even or odd. We'll start by defining the method.
//user defined method
Public static void findEvenOdd(int num)
{
//method body
If(num%2==0)
System.out.println(num+" is even");
Else
System.out.println(num+" is odd");
}
The following method, entitled find even odd, has been defined (). It has an int-type parameter called num. We used void because the method does not return any value. The procedures to determine whether a number is even or odd are contained in the method body. If the number is even, it will be printed as such; otherwise, it will be printed as odd.
Q19) Explain constructor overloading with an example?
A19) Constructor Overloading
Like method overloading we can also do constructor overloading. Overloaded constructors in Java can be implemented in the same way as method overloading. We can have more than one constructor with different parameter list with same name. Each constructor performs a different task.
Compiler differentiates between each constructor on the basis of their parameter list.
Example of constructor loading is given below:
Class Add {
int x;
int y;
// Overloaded constructor takes two int parameters
Add(int a, int b)
{
x = a;
y = b;
}
// Overloaded constructor single int parameters
Add(int a)
{
x = y = a;
}
// Overloaded constructor takes no parameters
Add()
{
x = 6;
y = 5;
}
int sum() {
return x + y;
}
}
Class OverloadAdd{
public static void main(String args[])
{
// create objects using the various constructors
Add cons1 = new Add(23, 1);
Add cons2 = new Add(8);
Add cons3 = new Add();
int s;
s = cons1.sum()
System.out.println(“Sum of constructor1 =” + s);
s = cons2.sum()
System.out.println(“Sum of constructor2 =” + s);
s = cons3.sum()
System.out.println(“Sum of constructor3 =” + s);
}
}
Output
Sum of constructor1 = 24
Sum of constructor2 = 16
Sum of constructor3 = 11
Q20) Why Method Overloading is not possible by changing the return type of method only?
A20) In java, method overloading is not possible by changing the return type of the method only because of ambiguity. Let's see how ambiguity may occur:
- Class Adder{
- Static int add(int a,int b){return a+b;}
- Static double add(int a,int b){return a+b;}
- }
- Class TestOverloading3{
- Public static void main(String[] args){
- System.out.println(Adder.add(11,11));//ambiguity
- }}
Output:
Compile Time Error: method add(int,int) is already defined in class Adder
System.out.println(Adder.add(11,11)); //Here, how can java determine which sum() method should be called?
Note: Compile Time Error is better than Run Time Error. So, java compiler renders compiler time error if you declare the same method having same parameters.
Q21) Write the difference between constructor overloading and method overloading?
A 21) Difference between Constructor overloading and Method overloading
Constructor overloading | Method overloading |
Constructor Overloading is the practice of writing many constructors in a class, each with a distinct set of parameters. | Method overloading is the practise of writing many methods within a class, each with its own set of parameters. |
The class name will appear in all constructors. | The names of all methods must be the same. |
Overloaded constructor will be executed at the time of instantiating an object. | If the method is not static, it can only be invoked after the object has been created according to the requirements. |
An overloaded constructor cannot be static as a constructor relates to the creation of an object. | It is possible for an overloaded method to be static and to be accessed without having to create an object. |
An overloaded constructor cannot be final as the constructor is not derived by subclasses; it won't make sense. | To prevent subclasses from overriding an overloaded method, it can be made final. |
An overloaded constructor can be private to prevent using it for instantiating from outside of the class. | An overloaded method can be made private in order to prohibit outsiders from calling it. |