struct employee { int id; char name[50]; float salary; }; |
struct employee { int id; char name[50]; float salary; }; int main() { struct employee e1, e2; return 0; } |
struct employee { int id; char name[50]; float salary; }e1,e2; |
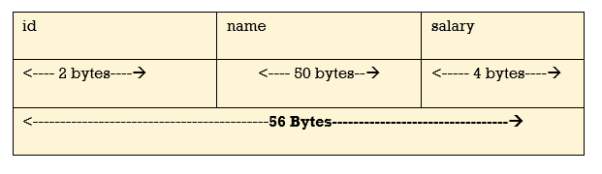
{ char name[20]; int roll; float marks; } std1 = {"Poonam" ,67, 78.3}; std2 = {"Vishal",62, 71.3}; |
{ int mark1; int mark2; int mark3; } sub1={67}; |
#include <stdio.h> // function to swap the two numbers void swap(int *x,int *y) { int t; t = *x; *x = *y; *y = t; } | ||
int main() { int num1,num2; printf("Enter value of num1: "); scanf("%d",&num1); printf("Enter value of num2: "); scanf("%d",&num2); //displaying numbers before swapping printf("Before Swapping: num1 is: %d, num2 is: %d\n",num1,num2); //calling the user defined function swap() swap(&num1,&num2); //displaying numbers after swapping printf("After Swapping: num1 is: %d, num2 is: %d\n",num1,num2); return 0; } | ||
/* Created a structure here. The name of the structure is * StudentData. */ struct StudentData{ char *stu_name; int stu_id; int stu_age; }; int main() { /* student is the variable of structure StudentData*/ struct StudentData student;
/*Assigning the values of each struct member here*/ student.stu_name = "Steve"; | |||
student.stu_id = 1234; student.stu_age = 30;
/* Displaying the values of struct members */ printf("Student Name is: %s", student.stu_name); printf("\nStudent Id is: %d", student.stu_id); printf("\nStudent Age is: %d", student.stu_age); return 0; } | |||
Output: Student Name is: Steve Student Id is: 1234 | |||
Student Age is: 30 | |||
Syntax:
- #include< file_name >
- where file_name is the name of file to be included. The ‘<‘ and ‘>’ brackets tells the compiler to look for the file in standard directory.
- user defined files: When a program becomes very large, it is good practice to divide it into smaller files and include whenever needed. These types of files are user defined files. These files can be included as:
- #include"filename"
3. #pragma Directive: This directive is a special purpose directive and is used to turn on or off some features. This type of directives are compiler-specific, i.e., they vary from compiler to compiler. Some of the #pragma directives are discussed below: