- Integer
- Character
- Boolean
- Floating Point
- Double Floating Point
- Valueless or Void
- Wide Character
- Function
- Array
- Pointer
- Reference
- Class
- Structure
- Union
- Enumeration
- Typedef defined Datatype
For Example: Consider the Class of Cars. There may be many cars with different names and brand but all of them will share some common properties like all of them will have 4 wheels, Speed Limit, Mileage range etc. So here, Car is the class and wheels, speed limits, mileage are their properties.
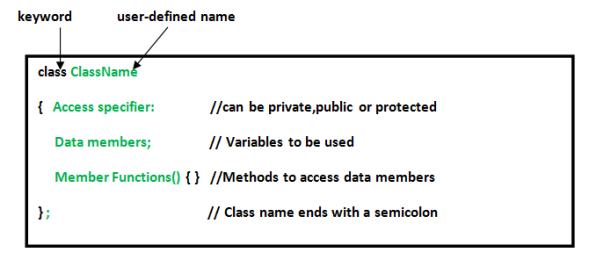
BASIS FOR COMPARISON | OBJECT | CLASS |
Definition | An instance of a class is known as Object. | A template or blueprint with which objects are created is known as Class. |
Type of entity | Physical | Logical |
Creation | Object is invoked by new keyword. | Class is declared by using class keyword. |
Memory allocation | Creation of object consumes memory. | The formation of a class doesn't allocate memory. |
#include <iostream>
using namespace std;
double division(int a, int b) {
if( b == 0 ) {
throw "Division by zero condition!";
}
return (a/b);
}
int main () {
int x = 50;
int y = 0;
double z = 0;
try {
z = division(x, y);
cout << z << endl;
} catch (const char* msg) {
cerr << msg << endl;
}
return 0;
}
Because we are raising an exception of type const char*, so while catching this exception, we have to use const char* in catch block. If we compile and run above code, this would produce the following result − Division by zero condition! Question. What is Access modifier in C++? Define each type and also differentiate between these.Answer: The access modifiers of C++ are public, private, and protected.One of the main features of object-oriented programming languages such as C++ is data hiding.Data hiding refers to restricting access to data members of a class. This is to prevent other functions and classes from tampering with the class data.However, it is also important to make some member functions and member data accessible so that the hidden data can be manipulated indirectly.The access modifiers of C++ allows us to determine which class members are accessible to other classes and functions, and which are not.For example,class Patient {
private:
int patientNumber;
string diagnosis;
public:
void billing() {
// code
}
void makeAppointment() {
// code
}
};
Here, the variables patientNumber and diagnosis of the Patient class are hidden using the private keyword, while the member functions are made accessible using the public keyword.Types of C++ Access ModifiersIn C++, there are 3 access modifiers:
public Access Modifier
Example 1: C++ public Access Modifier
using namespace std;
// define a class
class Sample {
// public elements
public:
int age;
void displayAge() {
cout << "Age = " << age << endl;
}
};
int main() {
// declare a class object
Sample obj1;
cout << "Enter your age: ";
// store input in age of the obj1 object
cin >> obj1.age;
// call class function
obj1.displayAge();
return 0;
}
Output:Enter your age: 20
Age = 20
In this program, we have created a class named Sample, which contains a public variable age and a public function displayAge().In main(), we have created an object of the Sample class named obj1. We then access the public elements directly by using the codes obj.age and obj.displayAge().private Access Modifier
Example 2: C++ private Access Specifier
using namespace std;
// define a class
class Sample {
// private elements
private:
int age;
// public elements
public:
void displayAge(int a) {
age = a;
cout << "Age = " << age << endl;
}
};
int main() {
int ageInput;
// declare an object
Sample obj1;
cout << "Enter your age: ";
cin >> ageInput;
// call function and pass ageInput as argument
obj1.displayAge(ageInput);
return 0;
}
Output:Enter your age: 20
Age = 20
In main(), the object obj1 cannot directly access the class variable age.// error
cin >> obj1.age;
We can only indirectly manipulate age through the public function displayAge(), since this function assigns age to the argument passed into it i.e. the function parameter int a.protected Access ModifierBefore we learn about the protected access specifier, make sure you know about inheritance in C++.
Example 3: C++ protected Access Specifier
using namespace std;
// declare parent class
class Sample {
// protected elements
protected:
int age;
};
// declare child class
class SampleChild : public Sample {
public:
void displayAge(int a) {
age = a;
cout << "Age = " << age << endl;
}
};
int main() {
int ageInput;
// declare object of child class
SampleChild child;
cout << "Enter your age: ";
cin >> ageInput;
// call child class function
// pass ageInput as argument
child.displayAge(ageInput);
return 0;
}
Output:Enter your age: 20
Age = 20
Here, ChildSample is an inherited class that is derived from Sample. The variable age is declared in Sample with the protected keyword.This means that ChildSample can access age since Sample is its parent class.We see this as we have assigned the value of age in ChildSample even though age is declared in the Sample class.public elements can be accessed by all other classes and functions.
Specifiers | Same Class | Derived Class | Outside Class |
public | Yes | Yes | Yes |
private | Yes | No | No |
protected | Yes | Yes | No |
#include <iostream>
using namespace std;
class Adder {
public:
// constructor
Adder(int i = 0) {
total = i;
}
// interface to outside world
void addNum(int number) {
total += number;
}
// interface to outside world
int getTotal() {
return total;
};
private:
// hidden data from outside world
int total;
};
int main() {
Adder a;
a.addNum(10);
a.addNum(20);
a.addNum(30);
cout << "Total " << a.getTotal() <<endl;
return 0;
}
When the above code is compiled and executed, it produces the following result −Total 60Above class adds numbers together, and returns the sum. The public members - addNum and getTotal are the interfaces to the outside world and a user needs to know them to use the class. The private member total is something that the user doesn't need to know about, but is needed for the class to operate properly.b) Overriding: C++ Function OverridingIf derived class defines same function as defined in its base class, it is known as function overriding in C++. It is used to achieve runtime polymorphism. It enables you to provide specific implementation of the function which is already provided by its base class.C++ Function Overriding ExampleLet's see a simple example of Function overriding in C++. In this example, we are overriding the eat() function.#include <iostream>
using namespace std;
class Adder {
public:
// constructor
Adder(int i = 0) {
total = i;
}
// interface to outside world
void addNum(int number) {
total += number;
}
// interface to outside world
int getTotal() {
return total;
};
private:
// hidden data from outside world
int total;
};
int main() {
Adder a;
a.addNum(10);
a.addNum(20);
a.addNum(30);
cout << "Total " << a.getTotal() <<endl;
return 0;
}
When the above code is compiled and executed, it produces the following result −Total 60Above class adds numbers together, and returns the sum. The public members addNum and getTotal are the interfaces to the outside world and a user needs to know them to use the class. The private member total is something that is hidden from the outside world, but is needed for the class to operate properly.Designing StrategyMost of us have learnt to make class members private by default unless we really need to expose them. That's just good encapsulation.This is applied most frequently to data members, but it applies equally to all members, including virtual functions.d) Virtual Functioning: A virtual function is a member function in the base class that we expect to redefine in derived classes.Basically, a virtual function is used in the base class in order to ensure that the function is overridden. This especially applies to cases where a pointer of base class points to an object of a derived class.For example, consider the code below:class Base {
public:
void print() {
// code
}
};
class Derived : public Base {
public:
void print() {
// code
}
};
Later, if we create a pointer of Base type to point to an object of Derived class and call the print() function, it calls the print() function of the Base class.In other words, the member function of Base is not overridden.int main() {
Derived derived1;
Base* base1 = &derived1;
// calls function of Base class
base1->print();
return 0;
}
In order to avoid this, we declare the print() function of the Base class as virtual by using the virtual keyword.class Base {
public:
virtual void print() {
// code
}
};
Virtual functions are an integral part of polymorphism in C++. To learn more, check our tutorial on C++ Polymorphism.Example 1: C++ virtual Function
using namespace std;
class Base {
public:
virtual void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1;
// pointer of Base type that points to derived1
Base* base1 = &derived1;
// calls member function of Derived class
base1->print();
return 0;
}
OutputDerived FunctionHere, we have declared the print() function of Base as virtual.So, this function is overridden even when we use a pointer of Base type that points to the Derived object derived1.e) Constructor and destructor: Constructors and Destructors in C++ConstructorA Constructor is a member function of a class. It is mainly used to initialize the objects of the class. It has the same name as the class. When an object is created, the constructor is automatically called. It is a special kind of member function of a class.Difference Between Constructor and Other Member Functions:1. The Constructor has the same name as the class name.2. The Constructor is called when an object of the class is created.
3. A Constructor does not have a return type.
4. When a constructor is not specified, the compiler generates a default constructor which does nothing.
5. There are 3 types of constructors:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <iostream> using namespace std; class test { public: int y, z; test() { y = 7; z = 13; } }; int main() { test a; cout <<"the sum is: "<< a.y+a.z; return 1; } |
The main function has an object of class test called a. When this object is created the constructor is called and the variables y and z are given values. The main function has a cout statement or a print statement. In this statement, the sum is printed. With respect to the object of the class, we access the public members of the class, that is, a.y gives the value of y and the same for z. We display the sum of y and z.
The default constructor works this way. When we do not provide a default constructor, the compiler generates a default constructor which does not operate.
Next, let us take a look at parameterized constructor.